A Guide to Math.random() in Java
The Math.random() method in Java is a built-in function which is a part of the java.Math class. One of the main benefits of the Math.random() method is its ease of use. It can be used to quickly generate random numbers within a specified range, making it a popular choice.
Another advantage of Math.random() is its performance. It is optimized to generate random numbers quickly, making it an ideal choice for high-performance applications where speed is a concern.
In this blog, we will discuss the basics of the Math.random() method and how to use it in Java to generate random numbers.
Table of Contents
- What are Random Numbers?
- Java Math.random() Method
- Application of random numbers
- Advantages of using Math.random() in Java
- Disadvantages of using Math.random() in Java
What are random numbers?
Random numbers are numbers that use a large set of numbers and select a single number from them using a mathematical algorithm. It satisfies the following two conditions:
- The generated values are uniformly distributed over a definite interval.
- It is impossible to guess the future value based on current and past values.
Java Math.random() Method
The Math.random()
method in Java is a static method in the java.lang.Math
class that returns a random floating-point number between 0.0 (inclusive) and 1.0 (exclusive).
The Math.random()
method generates a pseudo-random number using a deterministic algorithm, meaning that the sequence of numbers generated will be the same if the same seed is used. However, the default seed is based on the current time, which makes the generated numbers appear random.
The returned value of Math.random()
can be used in a variety of ways, such as generating random numbers within a specific range, shuffling arrays, and more.
Method Signature
public static double random( )
Syntax
Math.random( )
Return values
It returns a pseudorandom double value greater than or equal to 0.0 and less than 1.0
Parameters
There are no parameters to the Math.random() function
Java Math.random() Example
To understand Math.random() better, let’s look at some examples,
Example 1:
This code is an example of a simple Java program that uses the Math.random()
method to generate a random floating-point number and print it to the console.
class Main {
public static void main(String[] args) {
double number = Math.random();
System.out.println("Random number: " + number);
}
}
The main
method declares a double variable number
and assigns it to the result of calling the Math.random()
method. This method returns a random floating-point number between 0.0 and 1.0.
Finally, the code prints the value of the number
variable to the console using the System.out.println
method, which outputs a string to the console. The string "Random number: " is concatenated with the value of number
to produce the final output.
Each time this code is run, it generates a new random number and prints it to the console.
Output:

Generate random number between two numbers using Math.random() in Java
However, the random number obtained in Example 1 is not very useful in its current form. In most cases, we would not want to have a decimal number.
In order to produce a whole number with the pseudorandom number generator, we can multiply the random number by another number and round it to the nearest whole number.
For instance, suppose we want to generate a random number between 10 and 20. We could do so using this code:
Example 2:
class Main {
public static void main(String[] args) {
int upperBound = 20;
int lowerBound = 10;
// upperBound 20 will also be included
int range = (upperBound - lowerBound) + 1;
System.out.println("Random Numbers between 10 and 20:");
for (int i = 0; i < 10; i++) {
// generate random number
// (int) convert double value to int
// Math.random() generate value between 0.0 and 1.0
int random = (int)(Math.random() * range) + lowerBound;
System.out.print(Random integer + ", ");
}
}
}
In the main
method we have declared two integer variables upperBound
and lowerBound
with values 20 and 10 respectively.
Next, we have calculated the range of possible values between lowerBound
and upperBound
using the formula (upperBound - lowerBound) + 1
. This is because the Math.random()
method returns a value between 0.0 (inclusive) and 1.0 (exclusive), so to generate a random number within the specified range, we need to multiply the result of Math.random()
by the range and then add lowerBound
.
The code then uses a for loop to generate 10 random numbers within the specified range. The loop runs 10 times, and on each iteration, a new random number is generated by calling Math.random()
and multiplying the result by range
, then adding lowerBound
. The generated number is then cast to an integer using the (int)
operator, since Math.random()
returns a floating-point number, and we only want integers.
Output:
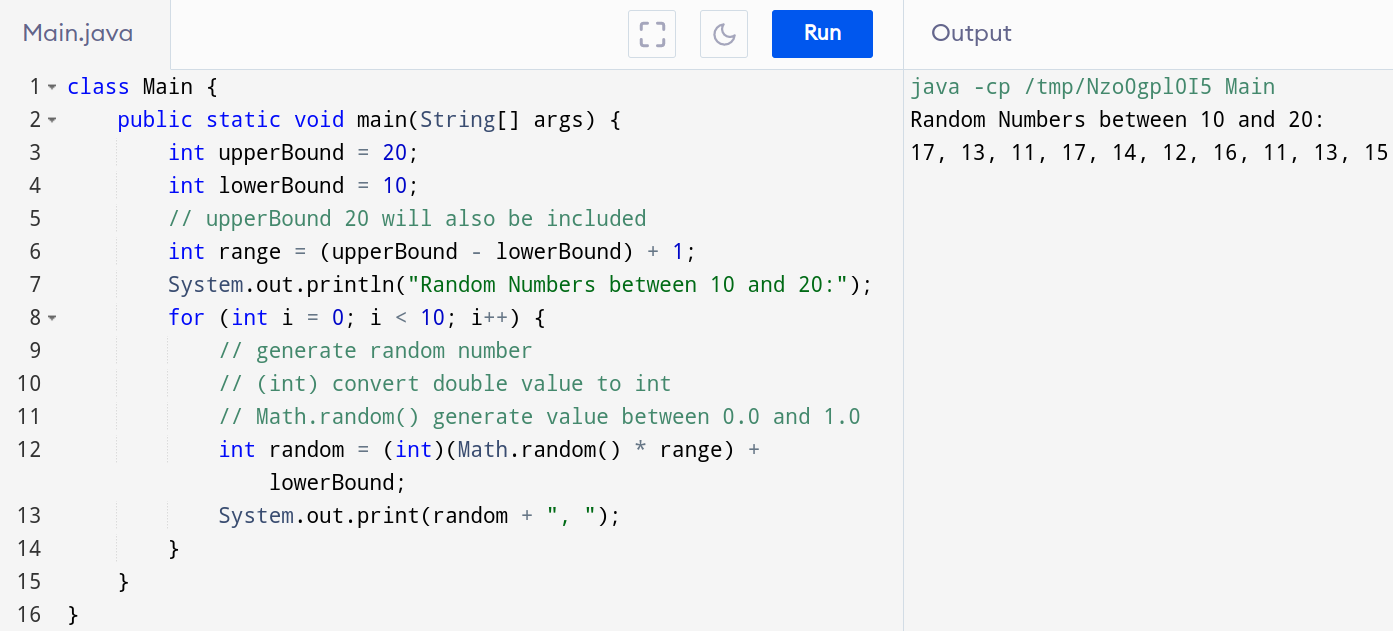
Example 3:
Suppose we are building an app that generates random numbers which will be used to distinguish a customer’s order at a cruise line. These numbers will be added to the end of a customer’s name.
For instance, the number we want to generate should be between 300 and 600. In order to generate this number and prepare the customer’s order reference, we could use this code
class Main {
public static int generateTicketNumber(int min, int max) {
int range = (max - min) + 1;
return (int)(Math.random() * range) + min;
}
public static void main(String args[]) {
String customerName = "Emmawatson";
int randomNumber = generateTicketNumber(300, 600);
System.out.println(customerName + randomNumber);
}
}
Output:
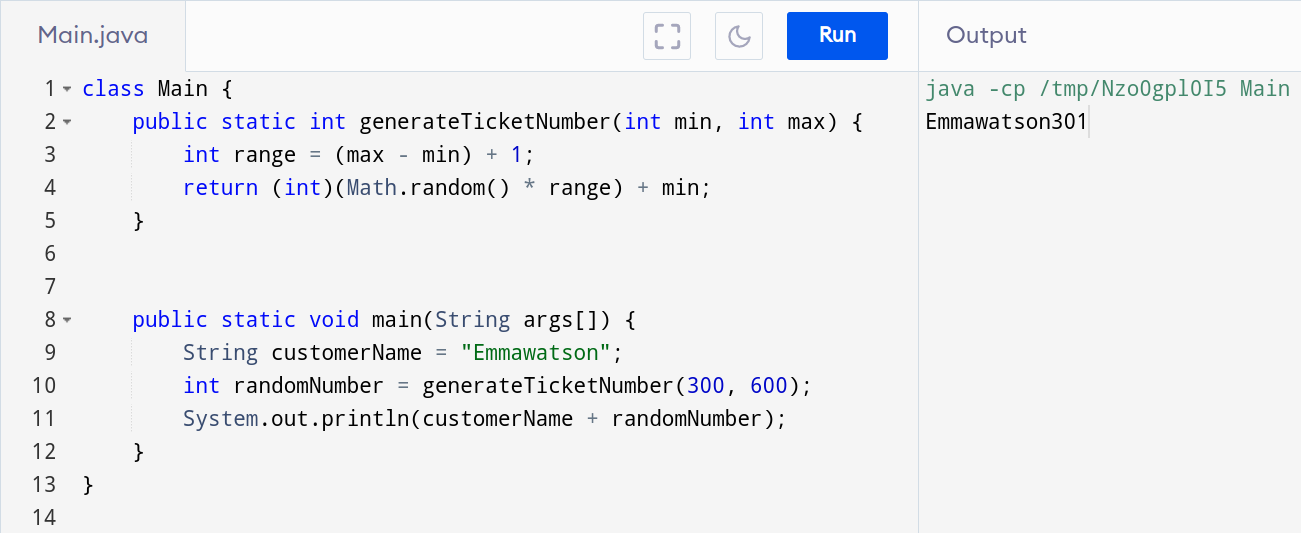
Application of random numbers
There are times when you need to generate a random number in programming. For example, say that you are operating a cruise line, as a booking reference, you may want to add a random number to a customer's order.
The Math.random() method in Java has many applications in various fields and industries. Some of the most common use cases for Math.random() include:
i.) Gaming: The Math.random() method is often used to generate random numbers for game development, such as random events, dice rolls, or card shuffling.
ii.) Statistical Analysis: Random numbers generated by Math.random() can be used in statistical analysis, such as Monte Carlo simulations, to model and predict outcomes.
iii.) Cryptography: In cryptography, random numbers generated by Math.random() can be used as keys or seeds to encrypt or decrypt sensitive information.
iv.) Testing: Math.random() can be used to generate random test data for software development, allowing developers to test their applications in a variety of scenarios.
v.) Artificial Intelligence: Math.random() can also be used in artificial intelligence and machine learning applications, such as genetic algorithms and neural networks, to generate random inputs for training and testing.
Advantages of using Math.random() in Java
- Simplicity: Math.random() is a simple and easy to use method for generating random numbers.
- Flexibility: It can be used to generate random numbers of various types, such as integers or decimals, within a specified range.
- Widely used: It is widely used in various applications, such as gaming, simulation, and statistical analysis.
Disadvantages of using Math.random() in Java
- Predictability: The sequence of random numbers generated by Math.random() can be predictable if not used correctly.
- Limited range: Math.random() only generates random numbers between 0 and 1, and the range must be scaled to meet the needs of a specific application.
- Non-uniform distribution: Math.random() generates random numbers with a non-uniform distribution, which can affect the accuracy of certain applications.
- Seed dependence: The random numbers generated by Math.random() are dependent on the seed value, and if the same seed is used, the same sequence of random numbers will be generated.
Conclusion
Math.random() method in Java is a convenient and efficient way to generate random numbers within a specified range.
If you need to generate random numbers for tasks like data analysis, games, or simulations, the Math.random() method is a handy tool.
However, it is important to note that the results generated by Math.random() are not completely random and may have some patterns or biases. For applications that require truly random numbers, it is recommended to use a specialized library.
Regardless of its limitations, Math.random() remains a widely used method for generating random numbers in Java and is a valuable tool for developers.
Monitor your Java application for errors & exceptions with Atatus
With Atatus Java performance monitoring, you can monitor the performance and availability of your Java application in real-time and receive alerts when issues arise. This allows you to quickly identify and troubleshoot problems, ensuring that your application is always running smoothly.
One of the key features of Atatus is its ability to monitor the performance of your Java application down to the individual request level. This allows you to see exactly how long each request is taking to process, as well as any errors or exceptions that may have occurred. You can also see a breakdown of the different components of your application, such as the web server, database, and external services, to see how they are affecting performance.
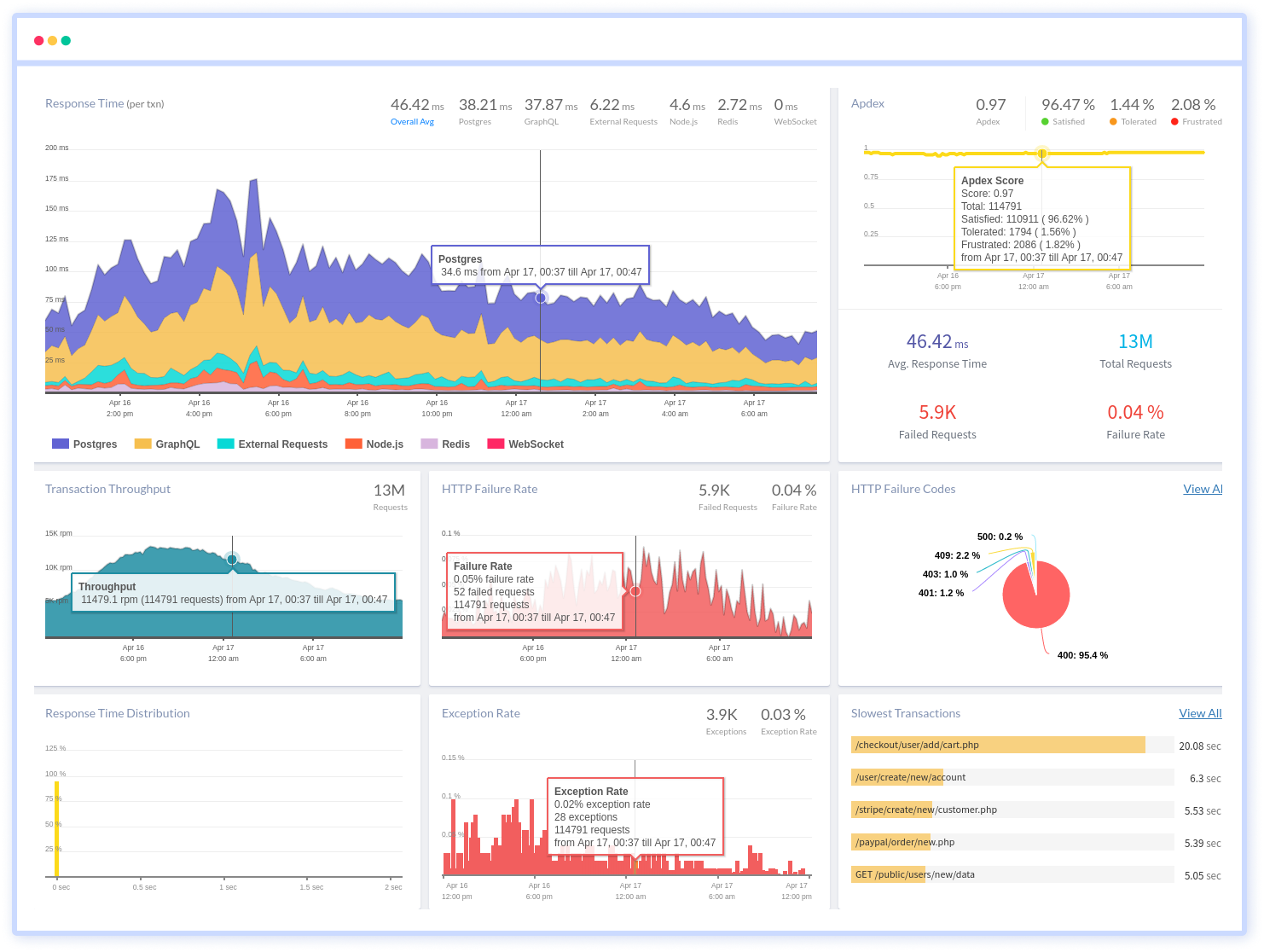
In addition to monitoring performance, Atatus also allows you to track the availability of your Java application. This means that you can see when your application is down or experiencing errors, and receive alerts when this occurs. You can also see how long your application has been running, as well as any uptime or downtime trends over time.
Atatus also offers a range of tools and integrations that can help you to get the most out of your monitoring. For example, you can integrate Atatus with popular tools like Slack, PagerDuty, and Datadog to receive alerts and notifications in your preferred channels. You can also use Atatus's APIs and SDKs to customize your monitoring and build custom integrations with your own tools and systems.
Overall, Atatus is a powerful solution for monitoring and managing the performance and availability of your Java application. By using Atatus, you can ensure that your application is always running smoothly and that any issues are quickly identified and resolved.
If you are not yet a Atatus customer, you can sign up for a 14-day free trial .
#1 Solution for Logs, Traces & Metrics
APM
Kubernetes
Logs
Synthetics
RUM
Serverless
Security
More