Ever found yourself frustrated by glitches while shopping online? Picture this, you are about to make that crucial purchase, but suddenly, an error message pops up, stopping your transaction.
What's behind these digital glitches, and how can they be fixed ? That is where Golang logging steps in!
When you encounter an error during checkout, Golang logging jumps into action, capturing crucial details about what went wrong.
From there, developers can analyse these logs, identify the root cause, and swiftly implement solutions, ensuring smoother shopping experiences for all.
In this blog, we'll explore Golang logging. We will start by explaining what logging is. Then we'll talk about why logging is so important and how it helps catch errors and track what's happening.
We'll also discuss how you can tell if your logging is doing a good job and give you tips on how to measure it. So, if you want to learn more about how logging works and why it matters, stick around!
Table of Contents
- What is Golang logging?
- Importance of Golang Logging
- Measuring Golang Logs
- How Logging in Golang Operate?
- Golang Logging Best Practices
What is Golang logging?
Golang logging is the practice of recording important log events and information during the execution of a Go program. It helps developers track what their program is doing, find and fix issues, and understand how it behaves in different situations.
For example, if you are building a web application in Go, you might use logging to track user requests, record errors, and monitor performance. Here's a basic example.
package main
import
(
"log"
)
func main()
{
// Log a message
log.Println("Hello, logging!")
// Log an error
log.Println("Error: Something went wrong!")
}
The first call logs the message "Hello, logging!"
and the second logs "Error: Something went wrong!"
. This code shows how to log messages in Go, which helps developers track what their program is doing and diagnose issues during execution.
Importance of Golang Logging
Logging in programming languages, like Go (Golang), is really important for many reasons. Let's see just how important it is.
- Debugging: This print messages to track program flow and identify errors easily during development.
fmt.Println("Debug message: Something happened here")
- Monitoring: Log performance metrics like response times to keep track of application health and identify issues in real-time.
log.Printf("Response time: %v milliseconds", responseTime)
- Auditing: Records user actions or system events to ensure compliance with regulations and maintain accountability.
auditLog.Printf("User %s performed action %s", username, action)
- Performance Optimization: Measure execution times and resource usage through logs to identify and improve inefficient parts of the code.
log.Printf("Function execution time: %v milliseconds", elapsedTime)
- Security: Log suspicious activities or unauthorized access attempts to enhance system security and detect potential threats.
securityLog.Printf("Unauthorized access attempt from IP: %s", ipAddress)
Thus, it provides developers and system administrators with the visibility and insights needed to effectively manage and troubleshoot complex systems.
Measuring Golang Logs
In simple terms, measuring Golang logs means,
- Counting how many log messages are generated.
- Checking if logs provide useful information.
- Evaluating how logging affects application performance.
- Monitoring log patterns and errors for insights.
Here are the steps to measure your logs,
- Identify Log Types: Determine what information you want in your logs, like performance metrics or user actions.
- Use Logging Frameworks: Choose Go logging frameworks such as Logrus or Zap, which come equipped with inherent metrics and instrumentation functionalities.
- Configure Logging Levels: Set up levels like debug, info, and error to control the amount of detail in your logs.
- Incorporate Custom Metrics: If necessary, include additional performance metrics using Golang's built-in expvar package or other tools.
- Pick a Logs Monitoring Tool: Select a tool like Atatus or Splunk to analyse and visualize your logs, aiding in understanding application behaviour.
- Configure Log Shipping: Send your logs to the chosen analysis tool by configuring your logging package to write to a file or stream and then forwarding it to the analysis tool.
- Analyse Log Data: Examine patterns and trends in your logs to identify areas for optimization or troubleshoot issues.
- Set up alerts: Establish alerts based on specific log events or metrics to proactively address potential problems, like notifying you if error rates exceed a threshold.
How Golang Logging Operate?
Understanding how logging functions in Golang operate is crucial for effective application development. Lets see the mechanics behind Golang logging, including its built-in capabilities and how to customize logging behaviour.
1. Select Logging Approach
Go's default logging package is like the basic toolbox - it's there and it gets the job done for simple tasks.
But if you need more features or flexibility, you might consider getting a more advanced set of tools, like external logging tools, which offer extra capabilities and options to fit your specific needs.
Consider trying Atatus, a comprehensive monitoring and error tracking solution with numerous features tailored for Go applications. With Atatus, you can pinpoint slow response times, identify performance bottlenecks, and gain real-time observability into your Go application.
Additionally, Atatus offers smart notifications and can deliver weekly and monthly summaries directly to your inbox, providing valuable insights to optimize your application's performance and reliability.
It is all about picking what works best for your project and what you are comfortable with.
2. Utilize Logging Functions
In Go's logging framework, there are different functions available for logging messages, and they are categorized based on the severity level of the message they handle.
i.) Print(), Printf(), Println()
These functions are used for logging messages with an INFO severity level.
Print()
simply prints the provided message to the log.Printf()
allows you to format the log message with additional data using formatting verbs, similar tofmt.Printf()
function.Println()
prints the message followed by a newline character.
ii.) Fatal(), Fatalf()
These functions are used for logging messages with a severity level of FATAL, which indicates a critical error that halts the program's execution.
Fatal()
prints the provided message and then terminates the program immediately.Fatalf()
allows you to format the log message with additional data before terminating the program.
3. Customize Logging Output
The Go logging package supports customization, allowing developers to tailor various logging aspects.
- Prefix: Define a prefix for each log message.
- Output Destination: Specify where log messages should be directed, such as the console or a designated file.
- Formatting: Customize log message format, including options for timestamps and additional data.
4. Implement Logging in Your Application
To begin logging in your Go application, you create a logger object using special functions provided by the logging package. This logger helps capture important information about what your program is doing, like recording events or errors.
It's like having a journal that keeps track of everything happening in your program, helping you understand and troubleshoot any issues that might arise.
import
(
"log"
)
func main()
{
log.Println("This is an informational message")
log.Printf("This is a formatted message with a variable: %v\n", "value")
log.Fatalf("This is a fatal error message")
}
To learn more about GoLang logging and monitoring, click on Atatus for further information.
Golang Logging Best Practices
Golang logging is essential for monitoring application behaviour and debugging issues. By following best practices, we can make our logs more useful, efficient, and manageable, ensuring smoother development and maintenance processes.
(i). Regular Log Review
Consistently analysing logs is crucial as it allows you to detect recurring patterns, pinpoint errors, enhance application performance, and mitigate potential security vulnerabilities.
package main
import
(
"bufio"
"fmt"
"log"
"os"
)
func main()
{
// Open the log file for reading
file, err := os.Open("logfile.log")
if err != nil {
log.Fatal(err)
}
defer file.Close()
// Create a scanner to read the log file line by line
scanner := bufio.NewScanner(file)
// Iterate through each line in the log file
for scanner.Scan() {
// Process each log entry
logEntry := scanner.Text()
fmt.Println(logEntry) // Print the log entry (you can perform further processing here)
}
// Check for any errors encountered during scanning
if err := scanner.Err(); err != nil {
log.Fatal(err)
}
}
Output:

(ii). Utilize Log Levels
Implementing log levels such as INFO, WARNING, and ERROR enables you to categorize log messages based on their severity, allowing for more granular control over the verbosity of logging output.
package main
import
(
"log"
)
func main()
{
// Set log level to INFO
log.SetFlags(log.LstdFlags | log.Lshortfile)
log.SetPrefix("INFO: ")
log.Println("This is an info message")
// Set log level to ERROR
log.SetFlags(log.LstdFlags | log.Lshortfile)
log.SetPrefix("ERROR: ")
log.Println("This is an error message")
}
Output:

(iii). Conditional Logging
By incorporating conditions into your logging statements, you can selectively log messages based on specific criteria or situations, ensuring that only relevant information is captured.
package main
import
(
"log"
)
func main(){
condition := true
if condition {
log.Println("This message will only be logged if the condition is true")
}
}
(iv). Log Rotation
Introducing log rotation mechanisms helps manage log file size by periodically archiving or rotating old log files, preventing them from consuming excessive disk space and ensuring smoother log management.
package main
import
(
"log"
"os"
"gopkg.in/natefinch/lumberjack.v2" // Import lumberjack for log rotation
)
func main()
{
logger := &lumberjack.Logger{
Filename: "logfile.log",
MaxSize: 10, // MB
MaxBackups: 3,
MaxAge: 28, // days
}
defer logger.Close()
log.SetOutput(logger)
log.Println("This message will be logged with log rotation")
}
(v). Optimize Log Settings
Fine-tuning log settings allows you to strike a balance between providing detailed information for troubleshooting purposes and efficiently utilizing system resources, optimizing the overall logging process.
For example, if you're troubleshooting a problem with your website, you might want detailed logs when testing new features. But once everything's working well, you can reduce the logging to save disk space and processing power.
This way, you ensure your logs are helpful when needed but not too heavy on your system.
Conclusion
Golang logging is about keeping track of what your program is doing. It's like taking notes as your program runs, helping you find and fix issues, understand its behaviour, and keep things running smoothly.
Golang logging is crucial for debugging, monitoring performance, ensuring security, and meeting compliance requirements.
To effectively use Golang logging, you need to pick the right tools, set up different log levels for severity, customize log output, and review logs regularly.
By categorizing log messages, selectively logging based on conditions, and optimizing log settings, you can make your logs more useful and efficient. Remember to rotate logs periodically to manage file size and system resources effectively.
Ultimately, good logging practices make your development and maintenance processes smoother, ensuring your application runs as intended.
Monitor Your Go Applications with Atatus
Atatus provides developers with insights into the performance of their Golang applications. By tracking requests and backend performance, Atatus helps identify bottlenecks in the application and enables developers to diagnose and fix errors more efficiently.
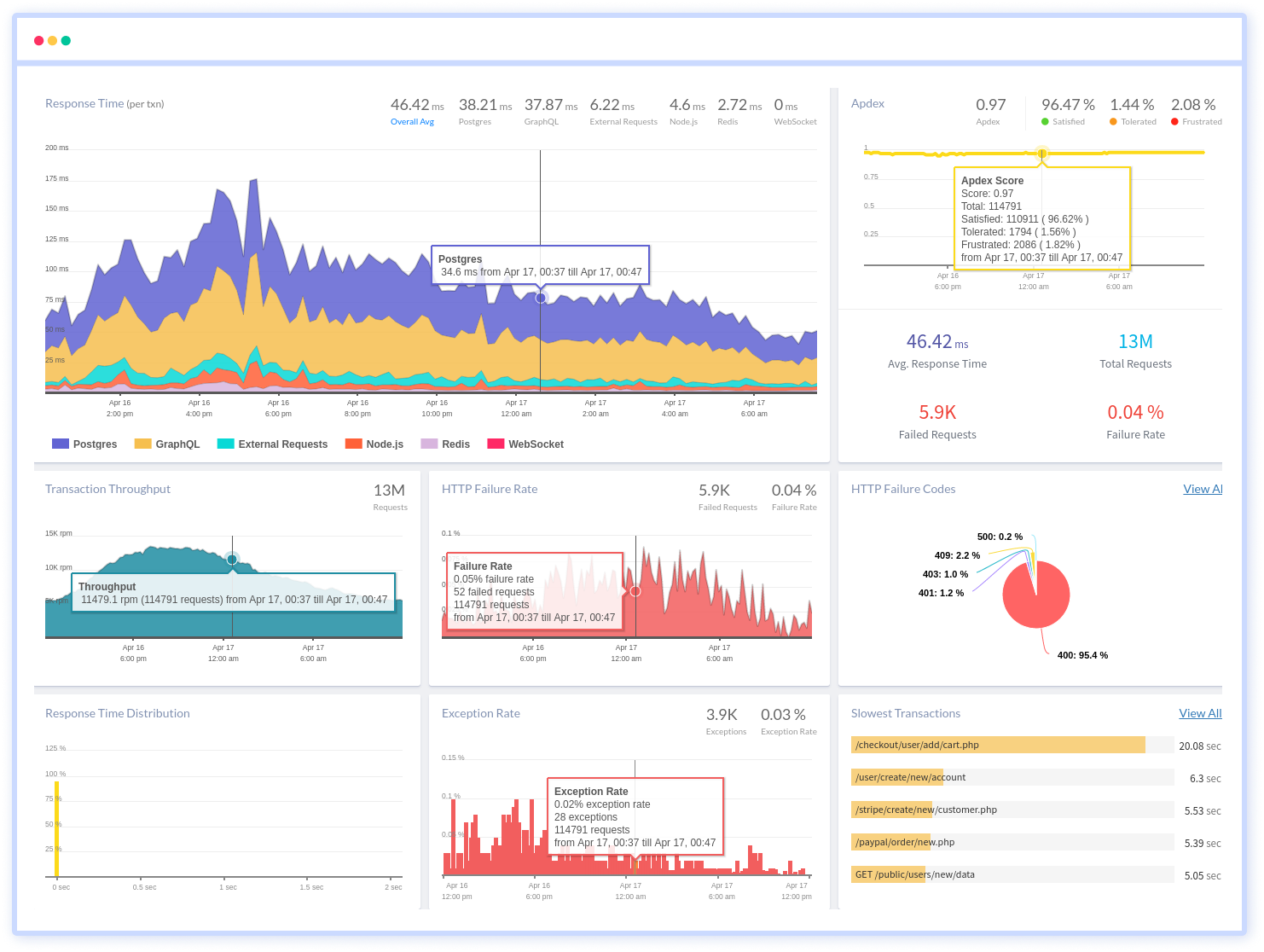
Golang monitoring captures errors and exceptions that occur in Golang applications and provides a detailed stack trace, enabling developers to quickly pinpoint the exact location of the error and address it.
We provide flexible alerting options, such as email, Slack, PagerDuty, or webhooks, to notify developers of Golang errors and exceptions in real-time. This enables developers to address issues promptly and minimize any negative impact on the end-user experience.