Comments in Python 3: How to write them?
Python was created by Guido van Rossum in the early 90's and is an interpreted, high-level programming language that is used by coders of all types.
It is a widely-used programming language because of its readability and the fact that it is easy to learn for novice programmers. It supports multiple programming paradigms, including object-oriented, functional, and imperative/procedural styles.
The most common use for Python is for web development, but it can be used for just about anything you can think of. It has an excellent community, a wide variety of applications, and a strong ecosystem of libraries.
What are Comments in Python?
What do you do if you can't understand Python code?
Giving variables clear, explicit names, and organizing your code are all good ways is an easy way to understand your python code.
Despite Python's simplicity, sometimes we are unable to understand what a particular function does. For this reason, comments are used. Comment is simply a way of explaining what's going on in a Python program.
Comments are text notes that we all make in our code to explain things that we don't want to forget. They are great for teams, for anyone who has to work through someone else's code and wants more detail, and for anyone who wants to read a beautiful codebase.
These are considered non-executable statements. A comment does not execute when a program runs, so you will not see any indication of it in the output.
An example is shown below on how comments work:
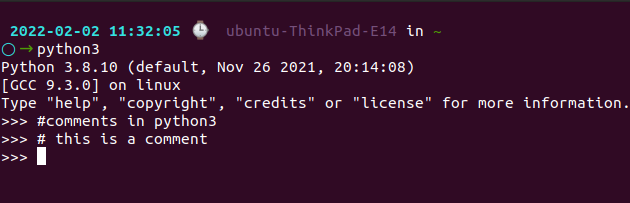
Syntax:
# this is a comment
Usage of Commenting:
The purpose of code comments is multifaceted. They can
- describe what a particular function does
- includes information, the reader may not be aware of
- enhance the readability
- make the code easier to understand
- provide a prompt for future changes
Good Vs Bad Comments
Inadequate programming skills and inadequately named variables are excuses for bad comments.
For ex:
# variable "a" that has been assigned to a string value of "comment"
a = "comment"
There is not much value in this comment. The fact that the variable "a" has a value of "comment" is obvious from the line of code. This does not require a comment.
Here are some examples of bad comments:
- Over commenting
- Lack of comments
- Misleading comments
Types of Comments in Python 3
There are many ways to write a comment. Comments in Python are preceded by # and followed by text. Below is a list of different types of comments:
- Single-Line comment
- Block comments
- Inline comments
- Docstring comments
#1 Single-Line Comment
The Python single line comment uses the hashtag symbol (#) without any white space. Put a hashtag on the new line and continue the comment if the comment exceeds one line.
Example:
# This is the syntax of a comment in Python
print("hello")
The first line in the code is the comment.
# define tools variable as a list of strings
tools = ['apm', 'rum', 'infra', 'logs', 'synthetics']
# for loop that iterates over tools list and prints each string item
for tool in tools:
print(tool)
#2 Block Comments
Python does not support multi-line comments from the start, unlike most other programming languages.
Here are some ways to use multi-line comments in Python.
- Consecutive Single-Line comment
- Using Multi-line string as comment
Example for consecutive single-line comment:
# This type of comments can serve
# both as a single-line as well
# as multi-line (block) in Python.
Example for multi-line string comment:
# Python code here
""" Multi-line comment using string
literal can be written
like this"""
#3 Inline Comments
Inline comments appear after the code on the same line. It is useful when attempting to explain tricky or complex elements of code.
x = 1
for i in range(10):
x = x + 1
print x # Print the value in the variable x
#4 Docstring Comments
Docstrings are string literals that are written at the end of Python definitions such as functions, methods, classes, and modules.
def add(a, b):
'''Takes in 2 numbers a and b, returns their sum'''
return a+b
By calling the __doc__ method of the object or by using the help function, you can access the docstrings.
def string_reverse(text):
'''
Returns the reversed String.
Parameters:
text: The string which is to be reversed.
Returns:
reverse_text: The string which gets reversed.
'''
reverse_text = ''
i = len(text)
while i > 0:
reverse_text += text[i - 1]
i = i - 1
return reverse_text
print(string_reverse('Application Performance Monitoring'))
Output:

To access the docstring:
print(string_reverse.__doc__)
Output:
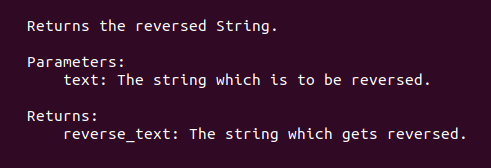
We can also read objects' docstrings with the help() function.
help(string_reverse)
Output:
Help on function string_reverse in module __main__:
string_reverse(text)
Returns the reversed String.
Parameters:
text: The string which is to be reversed.
Returns:
reverse_text: The string which gets reversed.
Conclusion
In summary, comments are a great way to leave a note for yourself after doing some work in an endeavour to make your code more readable.
Comments aren't more than a few lines of text attached to function or code. They can explain the function purpose, parameters, usage, or any other details that can help you understand why the function was implemented, how it works, and how to use it.
Python Comments can be made clearer and more relevant by using them in the proper way. This will help to make your code more readable and easier to collaborate with other developers.
Monitor your python application with Atatus
Atatus lets you detect, analyze and resolve python performance bottlenecks and failures with Python Application Performance Monitoring.
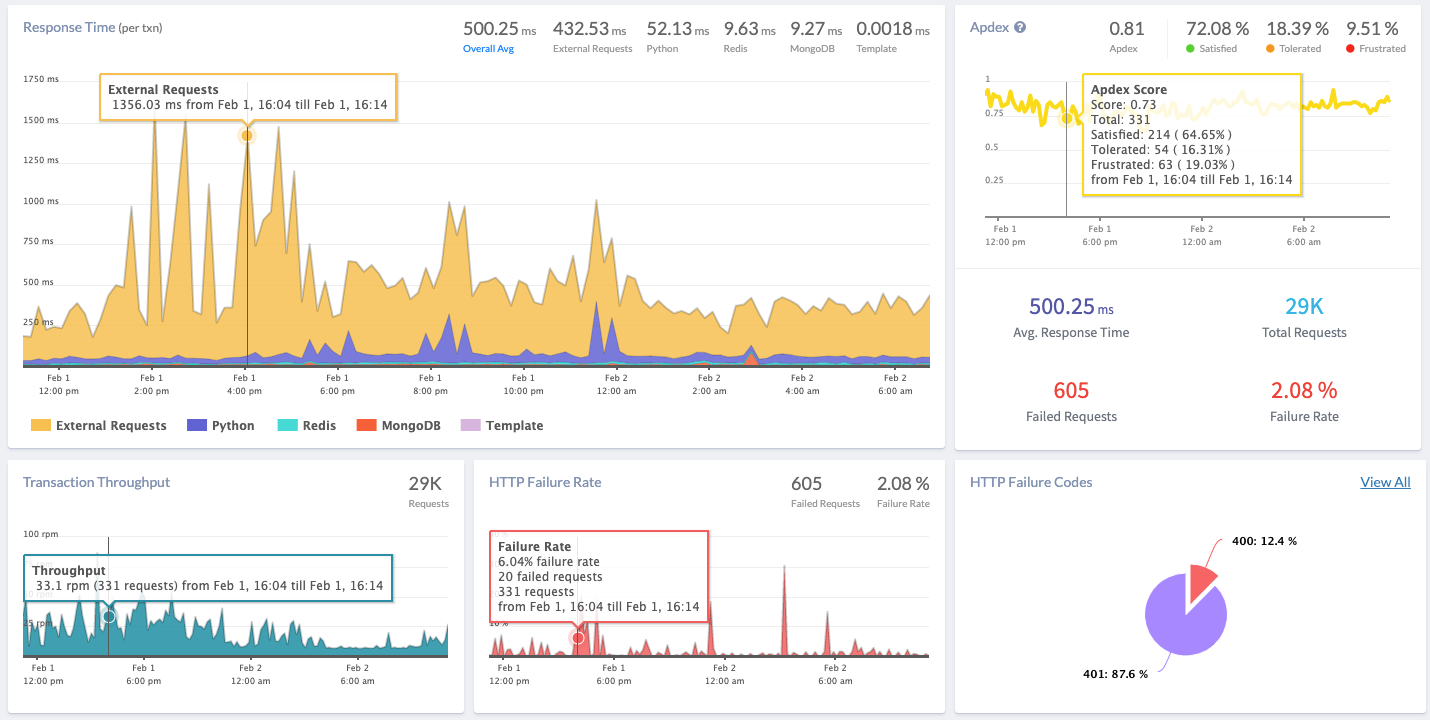
Monitoring Python applications in real time is the key to diagnosing performance bottlenecks, analyzing exceptions with stack traces, confirming that transactions traverse your distributed environment, and preventing errors from happening.
With built-in support for Python frameworks such as Django and Flask, you can start developing your Python app without errors.
Sign up for a free trial at Atatus and enjoy 14 days of free service.
#1 Solution for Logs, Traces & Metrics
APM
Kubernetes
Logs
Synthetics
RUM
Serverless
Security
More