JavaScript Source Maps: Everything You Should Know
JavaScript has become an essential programming language for web developers. It is used to develop complex web applications with dynamic and interactive features.
However, debugging and troubleshooting JavaScript code can be a daunting task, especially when the codebase is large. This is where JavaScript Source Maps come in handy.
They provide a way to map the minified or transpiled code back to its original source code, making it easier to debug and troubleshoot issues.
When a browser encounters an error in the minified code, it uses the source map to locate the original source file and line number where the error occurred. This makes it easier for developers to debug and troubleshoot issues in their code.
In this blog post, we will explore the concept of JavaScript Source Maps and how to get started with them. We'll also be exploring the different types of Source Maps and how to generate them for your own code.
Table of Content
- What are JavaScript Source Maps?
- Generating JavaScript Source Maps
- Breakdown of a JS Source Map File
- Debugging Made Easier with Source Maps
- Browser Support for Source Maps
What are JavaScript Source Maps?
Source maps are files that provide a mapping between the minified or transpiled code that is executed in the browser and the original, unminified source code written by developers. They include information about the original code's file structure, variable names, and line-by-line correspondence with the minified code.
You can search in the source map and get the initial position when your generated JavaScript makes a query for a specific line and column number.
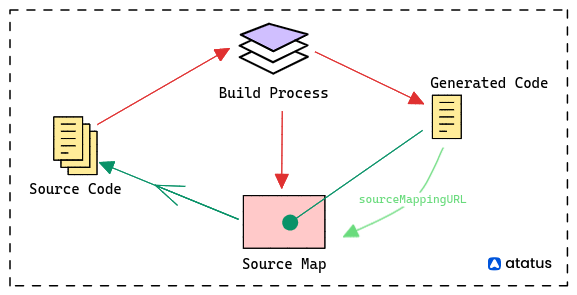
The source map can be immediately parsed by developer tools (currently WebKit nightly builds, Google Chrome, or Firefox 23+), giving the impression that you are operating unminified and uncombined files.
Necessity of Source Maps
Although there have been discussions about compiled-to-JavaScript languages like CoffeeScript and the potential to add support for CSS preprocessors like SASS or LESS, source mapping currently only functions between uncompressed/combined JavaScript to compressed/uncombined JavaScript, the future is looking bright.
A source map is a relationship between one or more initial source files and the produced, transpired, or minified JavaScript file. Sourcemaps are primarily used to assist in troubleshooting. In essence, the map can identify the position of the initial source file if the produced code file contains errors.
These source files are therefore for programmers. They are resources you'll likely use almost daily. Primarily aimed at aiding software development, these files play a crucial role in facilitating efficient teamwork and problem resolution.-
The folders known as source maps are used to transfer your source (original) code to your generated code. To put it another way, a line within your produced code represents the line of the source code that source maps identify.
Generating JavaScript Source Maps using uglify-js
You will need a utility that can assist in creating a source map as part of creating a minified version of your JavaScript before you can begin. Personally, we use UglifyJS and think it's a fantastic utility.
If you already have Node.js installed (even if you're not a Node developer), you can execute the following command to install it:
npm install uglify-js -g
The above command will install Uglify JS globally, so you can use it from the command line.
Let's assume you have a JavaScript file named example.js
with the following content:
function add(a, b) {
return a + b;
}
console.log(add(2, 3));
To generate the minified code along with a source map, you can use the uglifyjs
command-line tool as follows:
uglifyjs example.js --compress --mangle --output example.min.js --source-map
example.js
is the input JavaScript file.--compress
and--mangle
options enable code compression and variable name mangling to reduce the file size.--output
specifies the name of the output file where the minified code will be saved (example.min.js
).--source-map
instructsuglify-js
to generate a source map alongside the minified code.
After running this command, you'll have two files: example.min.js
(the minified code) and a sourceMappingURL
comment at the end of the minified file, which points to the source map file (example.min.js.map
).
//# sourceMappingURL=example.min.js.map
This comment is essential because it tells the browser where to find the corresponding source map when debugging. It associates the minified code with its source map, enabling developers to debug using the original source code.
In your HTML file, you can include the minified JavaScript file and link to the source map using the following code:
<script src="example.min.js"></script>
<script src="example.min.js.map"></script>
The browser will use the source map to map errors and debugging information back to the original example.js
file.
With this setup, when an error occurs or you need to debug the code, your browser's developer tools will display the error and stack trace in the context of the original source code (example.js
), making it easier to identify and fix issues in your code.
By following these steps, you can generate JavaScript source maps using uglify-js
to improve the debugging experience for your minified JavaScript code.
Breakdown of a Typical Source Map File
A source map is represented as JSON data. It includes essential components such as:
"version"
: Denoting the source map version.file
: The name of the generated code file (e.g., "app.min.js")."sources"
: An array of paths to the original source files."names"
: An array of identifiers and variable names."mappings"
: A string containing encoded mapping information."sourcesContent"
: The actual content of the original source files.sourceRoot
: An optional field that specifies the base path for resolving source file paths....
(other optional fields): Some source maps might include additional information like a list of included modules, metadata, etc.
Here is an illustration of a source map:
{
"version": 3,
"file": "app.min.js",
"sources": ["src/file1.js", "src/file2.js"],
"sourcesContent": ["/* Contents of file1.js */", "/* Contents of file2.js */"],
"mappings": "AAAA;ACAA;AAAA;...",
"names": ["console", "log", "add"],
"sourceRoot": "/source-maps"
}
In practice, the "mappings" section is often the most complex part of the source map. It uses a base64 VLQ (Variable Length Quantity) encoding to efficiently represent the mapping data, including information about lines, columns, source files, and symbol names.
By combining the information in the source map with the original source code, browsers and debugging tools can accurately display the original source code, set breakpoints, and step through code as if it were not minified or transpiled.
Debugging Made Easier with Source Maps
Imagine you have a minified JavaScript or CSS file and an associated source map. When an error occurs, the browser can utilize the source map to map the error back to the exact location in the original source code. This means developers can identify the problematic line, column, and even variable names, vastly simplifying the debugging process.
Source maps provide several benefits for easier debugging:
- Original Code View: Easily debug your original, human-readable code, making issue identification and changes simpler.
- Precise Error Location: Source maps link minified code errors to exact original code line and column numbers, ensuring accurate debugging.
- Readable Variable Names: Map minified variable and function names back to their original, understandable forms, facilitating code inspection.
- Efficient Workflow: Developers can debug minified code as if it were the original, with breakpoints, variable inspection, and familiar tools.
- Faster Debugging: Source maps speed up issue identification, reducing debugging time and enhancing productivity and code quality.
- Cross-Browser Support: Modern browsers, like Chrome, Firefox, Safari, and Edge, support source maps, ensuring a consistent debugging experience.
When debugging with source maps, there are three common types or strategies for including and using source maps:
- Inline source map
- External source map
- Hidden source
1. Inline Source Map
An inline source map is embedded within the compiled file using a data URL. This eliminates the need for an additional HTTP request but slightly increases the file size. Developers can easily relate the compiled code to the original source by referencing this inline source map comment.
Usage: These are useful when you want a single, self-contained file that includes both the minified code and the source map.
Advantages:
- Simplicity: No need to manage separate source map files.
- Convenience: Easier to distribute a single file.
Disadvantages:
- Increased File Size: The inline source map increases the size of the JavaScript file.
- Cache Inefficiency: Changes to the source code or source map require regenerating and redistributing the entire file.
Example of an inline source map comment at the end of a JavaScript file:
//# sourceMappingURL=data:application/json;base64, ...
This unique comment instructs the web browser how to connect the code that was compiled to the initial version and is added to your regular JavaScript file.
2. External Source Map
An external source map offers an alternative approach. In this scenario, the source map is stored in a separate file. This technique is particularly beneficial for production environments where the real-time loading of source maps isn't necessary.
By opting for external source maps, you can maintain smaller production files without sacrificing the ability to debug. This separation simplifies the application's structure, making it easier to examine in production.
In certain cases, you might choose not to generate a source map for your production package, which can also contribute to a level of obfuscation by disabling source maps. This approach proves valuable during testing to determine whether enabling source maps for production is desirable.
Location: External source maps are stored in separate files with a .map
file extension (e.g., script.js
and script.js.map
).
Usage: These are commonly used in development environments where source maps are generated during a build process, but only the minified JavaScript is served to users.
Advantages:
- Smaller File Size: The minified JavaScript file remains smaller, reducing network transfer times.
- Better Caching: Changes to the source code or source map don't require redistributing the entire JavaScript file.
Disadvantages:
- Slightly More Complex Setup: Managing separate source map files.
Example of linking to an external source map in a JavaScript file:
//# sourceMappingURL=/path/to/script.js.map
3. Hidden Source Map
Hidden source maps serve a specific purpose: they provide essential stack trace information when errors occur within a deployed application.
These maps are particularly valuable for pinpointing the causes of failures and aiding in the resolution of problematic scenarios. To leverage this information, developers can link hidden source maps to a tracking service.
While not the most ideal solution, this practice ensures that even in live environments, you can gain insights into potential issues. The benefit of being aware of potential problems outweighs the drawback of not having an optimal solution readily available.
Location: Hidden source maps are stored on the server and are not accessible to the client's browser. They are used primarily for security reasons to keep the original source code confidential.
Usage: These are essential when you want to protect your intellectual property or sensitive code while still benefiting from source maps during development.
Advantages:
- Code Protection: Prevents users from accessing the original source code, even though source maps are used for development.
Disadvantages:
- Debugging Limitations: Developers can't use source maps in their browsers for debugging, as the source map isn't accessible to the client.
Example of using hidden source maps: The source map is stored securely on the server, and it's not referenced or exposed in the client's code.
Browser Support for Source Maps
Source maps have gained widespread acceptance among modern web browsers, resulting in built-in support that ensures a consistent and effective debugging experience across various development environments
Here's an overview of source map compatibility in popular browsers:
1. Google Chrome
Google Chrome is widely used by developers and offers robust support for source maps. When you encounter an error in the Chrome developer tools, it automatically loads the associated source map if available. This allows you to inspect and debug your original source code directly in the browser.
2. Mozilla Firefox
Firefox is another browser that fully supports source maps. It seamlessly integrates them into its developer tools, enabling developers to navigate and debug their code with ease. Firefox provides a user-friendly debugging experience with source map integration.
3. Safari
Apple's Safari browser also supports source maps, allowing developers using macOS and iOS devices to benefit from an efficient debugging workflow. Similar to Chrome and Firefox, Safari's developer tools make good use of source maps for accurate debugging.
4. Microsoft Edge
Microsoft Edge, the successor to Internet Explorer, has adopted modern web development practices and supports source maps. Developers using Edge can expect a consistent and productive debugging experience when working with minified or transpiled code.
Conclusion
The ability to fix code quickly and effectively, connect back to the original source files, and see better what the browser is doing is made possible by source maps. It is even simpler to debug and examine problems immediately when source maps are combined with the developer tools which Chrome and Firefox have.
In this blog, we have seen the necessity of source maps and what makes debugging easier when we use a source map. Also the types of source maps and how they work with other browsers.
We can now, easily, map between a minified form of our JavaScript and the un-minified code. Any processed file can use Source Maps, a JSON-based mapping format, to establish a mapping connection between the source and the processed result.
For our minified JavaScript, our output from CoffeeScript, our output from Less, and our output from Sass, we can use source maps! Our goal is to create a JavaScript source map that will be used to manage localization of our minified JavaScript.
Atatus Real User Monitoring
Atatus is a scalable end-user experience monitoring system that allows you to see which areas of your website are underperforming and affecting your users. Understand the causes of your front-end performance issues and how to improve the user experience.
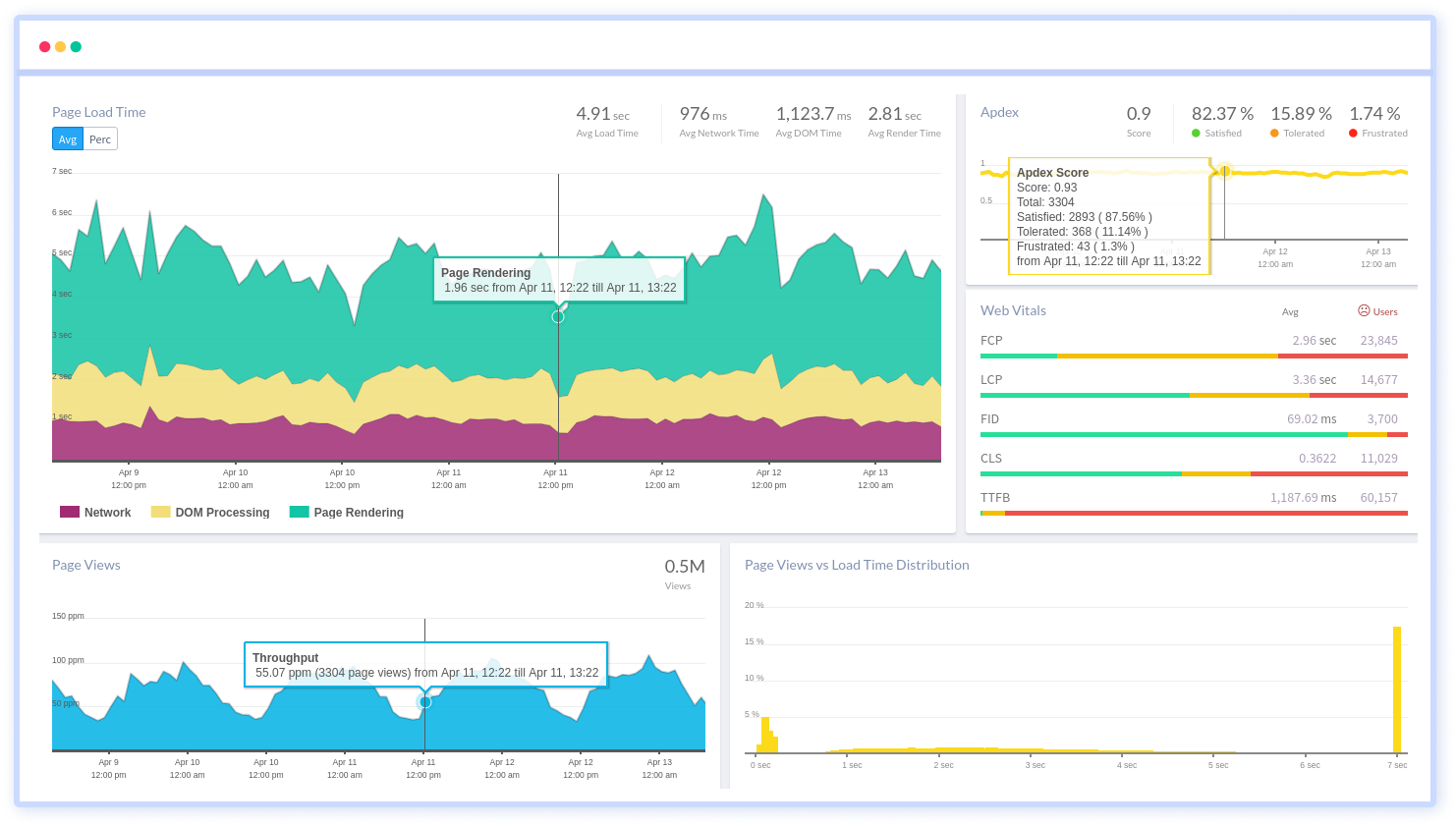
By understanding the complicated frontend performance issues that develop due to slow page loads, route modifications, delayed static assets, poor XMLHttpRequest, JS errors, core web vitals and more, you can discover and fix poor end-user performance with Real User Monitoring (RUM).
You can get a detailed view of each page-load event to quickly detect and fix frontend performance issues affecting actual users. With filterable data by URL, connection type, device, country, and more, you examine a detailed complete resource waterfall view to see which assets are slowing down your pages.
#1 Solution for Logs, Traces & Metrics
APM
Kubernetes
Logs
Synthetics
RUM
Serverless
Security
More