How to Check if a File Exists in Linux Bash Script?
As a developer or system administrator, you may encounter situations where you need to check whether a file exists in a Linux or Unix system. Knowing how to do this efficiently can save you time and effort, especially when dealing with multiple files.
Bash, the default shell in most Linux and Unix systems, provides several ways to check for file existence. In this blog post, we will explore some of the most commonly used methods to check if a file exists in bash.
We will start by introducing the basic syntax of the test
command, which is a built-in command used to perform various tests on files and directories. We will then move on to the [ ]
and [[ ]]
syntax, which are used to test expressions and conditions, including file existence.
Finally, we will discuss the if
statement and how it can be used to check for file existence and perform actions based on the result.
By the end of this post, you will have a solid understanding of how to check if a file exists in bash, and you will be able to use this skill in your future coding projects.
Table Of Contents:-
- Using 'test' command to check if the file exists
- Using double brackets [[ to check if the file exists
- Using 'if' statement with an '-e' to check if the file exists
- Verifying if the file is a regular file using '-f'
- File test operators
- Using 'stat' command to check if the file exists
- Error handling with '-f' when the file does not exist
1. Using 'test' command to check if the file exists
The test command (or its equivalent [ ] brackets) in Bash is a built-in utility used for performing various conditional tests. The test command is a straightforward and commonly used method for checking file existence in Bash. It offers a simple way to determine if a file is present before proceeding with any operations on that file within your script.
Syntax:
if test -e "/path/to/file"; then
# Code to execute if the file exists
else
# Code to execute if the file does not exist
fi
- test
-e "/path/to/file"
is the command that checks if the file at the specified path exists. if
...; then initiates a conditional statement to evaluate the file's existence.- If the file exists, the code within the if block is executed.
- If the file does not exist, the code within the else block is executed.
Additionally, you can use various other options with test to perform different types of file checks, such as checking if a file is a regular file, a directory, or has specific permissions.
2. Using double brackets [[ to check if the file exists
Using double brackets [[ ... ]] is a more powerful and flexible way to check if a file exists in Bash compared to the traditional [ ... ] test command. Here's an explanation of how to use double brackets to check for the existence of a file:
if [[ -e "/path/to/file" ]]; then
echo "File exists."
else
echo "File does not exist."
fi
In this syntax:
[[ -e "/path/to/file" ]]
checks if the file at the specified path exists. You can replace"/path/to/file"
with the actual path to the file you want to check.- If the file exists, the code within the if block is executed.
- If the file does not exist, the code within the else block is executed.
3. Using 'if' statement with an '-e' to check if the file exists
In Bash scripting, one of the simplest ways to determine the existence of a file is by employing the if statement in combination with the -e
option. This method allows you to create conditional logic that reacts based on whether a specified file is present or not.
Syntax:
if [ -e "/path/to/file" ]; then
echo "File exists."
else
echo "File does not exist."
fi
[ -e "/path/to/file" ]
checks if the file at the specified path exists.- If the file exists, the code within the if block is executed.
- If the file does not exist, the code within the else block is executed.
This approach is widely used and effective for basic file existence checks in Bash scripts. However, for more advanced scripting needs or when dealing with different file types, other methods like [[ ]]
, test, or stat might be more suitable.
4. Verifying if the file is a regular file using '-f'
It's often essential not only to check if a file exists but also to confirm that the file is a regular file (i.e., not a directory, symbolic link, or special file). You can achieve this by using the -f option in combination with conditional statements.
if test -f "/path/to/file"; then
echo "File exists and is a regular file."
else
echo "File does not exist or is not a regular file."
fi
[ -f "/path/to/file" ]
checks if the file at the specified path exists and is a regular file.- If the file exists and is a regular file, the code within the if block is executed.
- If the file does not exist or is not a regular file (e.g., it's a directory or symbolic link), the code within the else block is executed.
By using the -f
option, you ensure that your script not only verifies the file's existence but also checks if it's the specific type of file you're expecting before proceeding with any operations on it. This level of validation can be crucial in many Bash scripting scenarios.
You can also use double brackets to check if a file exists and is a regular file (not a directory, symbolic link, or special file):
if [[ -f "/path/to/file" ]]; then
# Code to execute if the file exists and is a regular file
else
# Code to execute if the file does not exist or is not a regular file
fi
In this case, [[ -f "/path/to/file" ]]
checks both existence and regular file status.
Using double brackets with -e
or -f
provides several advantages:
- Improved readability: The syntax is more intuitive and readable, making your code easier to understand.
- Logical operators: You can use logical operators (e.g., && and ||) within double brackets for more complex conditional tests.
- No need for variable expansion: You don't need to enclose variables in double quotes (e.g., "$file") as you do with the [ ... ] test command.
Overall, double brackets [[ ... ]]
is recommended when checking for file existence and attributes in Bash scripts, as it offers greater flexibility and readability.
5. File test operators
We can test for particular types of files using the following File Operators:
File Check | Returns | Description |
---|---|---|
-b File | True | If the file exists as a block special file. |
-c File | True | If the file exists as a special character file. |
-d File | True | If the file exists as a directory. |
-e File | True | If the file exists as a file, regardless of type (node, directory, socket, etc.). |
-f File | True | If the file exists as a regular file (not a directory or device). |
-G File | True | If the file exists and contains the same group as the user is running the command. |
-h File | True | If the file exists as a symbolic link. |
-g File | True | If the file exists and contains the set-group-id (sgid) flag set. |
-k File | True | If the file exists and contains a sticky bit flag set. |
-L File | True | If the file exists as a symbolic link. |
-O File | True | If the file exists and is owned by the user who is running the command. |
-p File | True | If the file exists as a pipe. |
-r File | True | If the file exists as a readable file. |
-S File | True | If the file exists as a socket. |
-s File | True | If the file exists and has a nonzero size. |
-u File | True | If the file exists, and the set-user-id (suid) flag is set. |
6. Using 'stat' command to check if the file exists
The stat command in Bash is a versatile utility that provides detailed information about files and directories. You can use it to check if a file exists and gather additional file-related information.
if stat "/path/to/file" >/dev/null 2>&1; then
echo "File exists."
else
echo "File does not exist."
fi
- stat
"/path/to/file"
is the command that retrieves information about the file at the specified path. >/dev/null 2>&1
is used to suppress the output and error messages generated by the stat command, ensuring that the command doesn't produce any visible output.if
...; then begins the conditional statement to check the existence of the file.- If the file exists, the code within the if block is executed.
- If the file does not exist, the code within the else block is executed.
The stat command is particularly useful when you need more than just a simple existence check. It allows you to collect various details about the file, such as its size, permissions, and timestamps, in addition to verifying whether the file exists.
The command's flexibility makes it suitable for scenarios where you require a comprehensive assessment of a file's properties before proceeding with specific actions in your Bash script.
7. Error handling with '-f' when the file does not exist
Error handling when using the -f
option to check if a file exists and the file does not exist can be done in Bash using conditional statements and appropriate messages. Here's an example of how to handle errors when the file specified does not exist:
#!/bin/bash
file="/path/to/nonexistentfile"
if [ -f "$file" ]; then
echo "File exists and is a regular file."
# Your code to process the file goes here
else
echo "Error: The file '$file' does not exist or is not a regular file."
# Additional error handling code can go here
fi
In the code above:
- We specify the path to the file we want to check in the file variable. Replace
"/path/to/nonexistentfile"
with the actual path to the file you want to check. - We use the
-f
option in the[ -f "$file" ]
conditional statement to check if the file exists and is a regular file. If the file exists and is a regular file, the code inside the if block is executed. - If the file does not exist or is not a regular file (e.g., it's a directory or doesn't exist), we print an error message using echo in the else block.
- You can add additional error-handling code inside the else block, such as exiting the script, logging the error, or taking other appropriate actions based on your specific needs.
By providing a clear error message, you make it easier to diagnose issues when the file is missing, allowing you to handle such situations gracefully in your Bash script.
Conclusion
There are several ways to check if a file exists in bash, ranging from the simple to the more complex. Whether you prefer to use the test
command, double brackets, or the if
statement with the -e
option, it is important to verify that the file you are looking for actually exists before attempting to access or manipulate it.
Additionally, using file test operators such as -f
can help you determine if a file is a regular file or not before performing any actions on it.
By familiarizing yourself with these methods and incorporating error handling with -f
when necessary, you can streamline your bash scripting and avoid potential errors.
Atatus Logs Monitoring and Management
Atatus offers a Logs Monitoring solution which is delivered as a fully managed cloud service with minimal setup at any scale that requires no maintenance. It monitors logs from all of your systems and applications into a centralized and easy-to-navigate user interface, allowing you to troubleshoot faster.
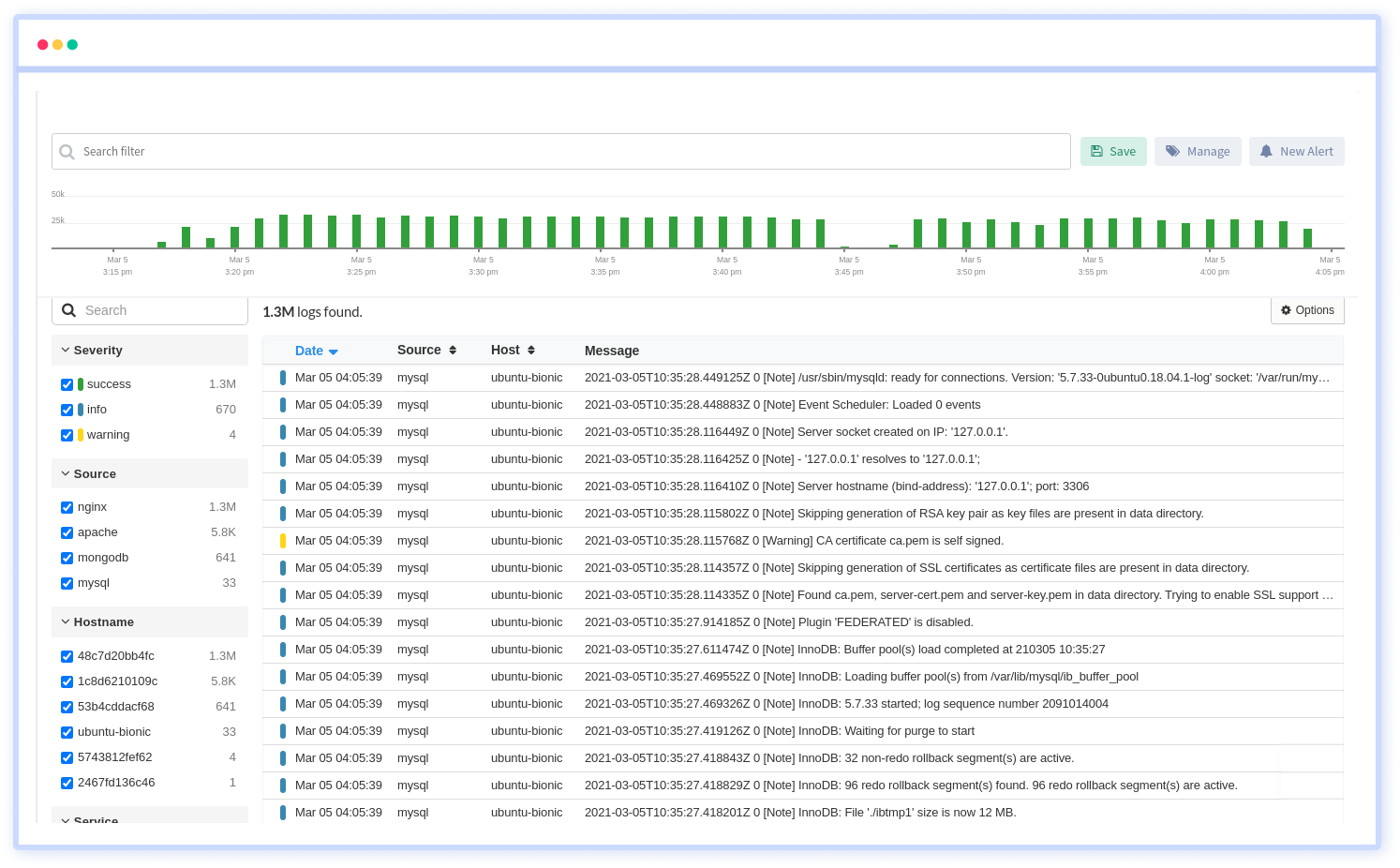
We give a cost-effective, scalable method to centralized logging, so you can obtain total insight across your complex architecture. To cut through the noise and focus on the key events that matter, you can search the logs by hostname, service, source, messages, and more. When you can correlate log events with APM slow traces and errors, troubleshooting becomes easy.
#1 Solution for Logs, Traces & Metrics
APM
Kubernetes
Logs
Synthetics
RUM
Serverless
Security
More