JWT Authentication: When and How To Use It
JWT stands for JSON Web Token, is a famous technology that comes with its own controversy. Few people find it quite beneficial, while others feel one must never use it. Nonetheless, in this article, we shall discuss the reason behind this controversy, understand JWT in detail, and when and why one must use it.
We'll cover the following section in this blog.
- What is JWT?: A brief introduction
- How It Works?
- Structure of Json Web Token
- Use of JWT for API Authentication
- When Should you use Json Web Tokens?
- How can one expire a single token?
- How to store JWTs securely?
- Use of JWT for SPA authentication
- Use of JWT to authorize operations across server
- Choosing the perfect JWT library
- When one must not use JWTs: Session tokens for regular web applications
#1 What is JWT?: A brief introduction
JWT (JSON Web Token) authentication is a process or method used to verify the owner of JSON data. It is a URL safe encoded string that is cryptographically signed and, unlike a cookie, contains an unlimited amount of data.
When a server receives a JWT, it guarantees and ensures that the data contained within the JWT can be trusted, as a source signs it. No person or a middleman can make changes or modify a JWT once it is sent to a server.
Many people get confused that JWT provides encryption, which is incorrect. JWT does not provide encryption but guarantees data ownership. It is important to note that anyone who intercepts the token can view the JSON data that is stored in a JWT. It is because the data is serialized and not encrypted.
This is the reason why, for safety purposes, it is greatly recommended to use HTTPS (HyperText Transfer Protocol Secure) with the JWTs.
#2 How It Works?
In a nutshell, JWT works like this way:
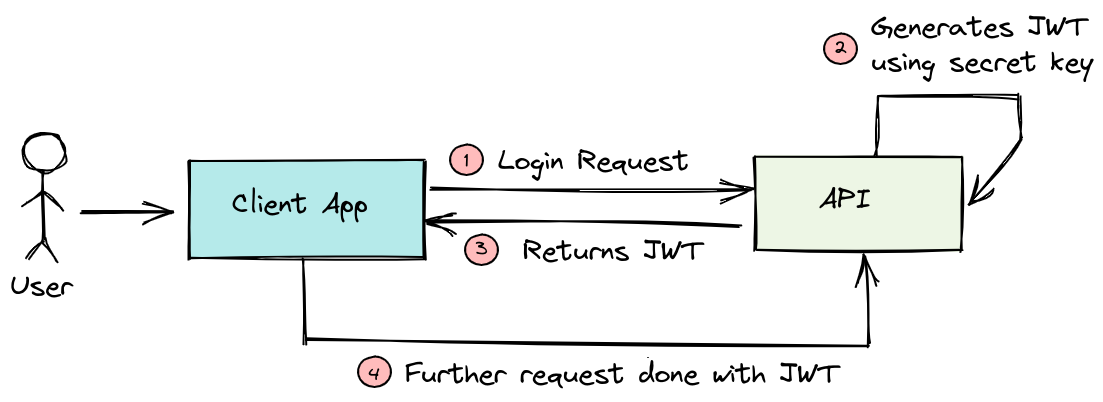
- Client send a post request i.e the login request with the username and password.
- Once it is successfully authenticated the server/API will create a JWT token which will be signed with a secret key.
- After creating the JSON Web Token, the API will return it back to the Client Application.
- After receiving the token by the client app, it is verified to ensure it is authentic and then only it will be used on every subsequent requests to authenticate the user so that user do not have to send the credentials anymore.
#3 Structure of Json Web Token
Lets dig deep into the structure of the Json Web Tokens to understand how secure and how useful it is to use.
The token which has been returned by the API is an encoded string which is composed of three different portions, detached from one another with the dot representation.
header.payload.signature
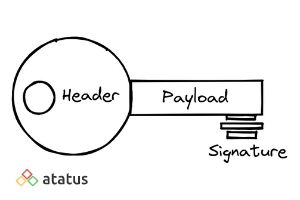
i.) Header
The header portion contains two fields the hashing algorithm generated for creating the JW Token and the type of the token. The hashing algorithm depends the security standard and measures you are looking for.
The most commonly used algorithms are HS256 and RS256. The tokens are signed by using either the private or the public key.
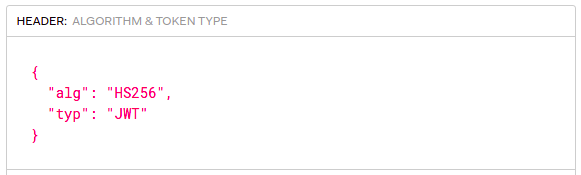
ii.) Payload
It is the base64 encoded JSON object which carries the bulk of our JWT, often known as JWT claims. We can insert the data we want to transmit and the additional information about the token within this payload.
You can decode this function using the atob() method which gives you a JSON string and with the json.parse() metod you can parse the respective JSON string into an object.
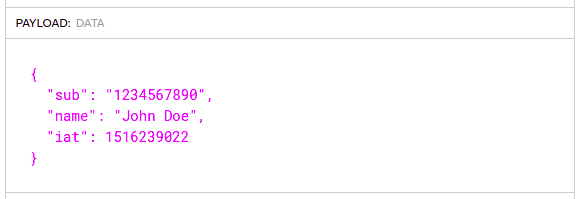
When we talk about JWT claims, a question would arise like what are JWT claims and how it will look like? 🤔
JWT claims are nothing but the piece of information asserted about an entity. They are key-value pair which contains information about the user.
There are multiple claims we can provide inside the payload.
- Registered Claims - Predefined claims which are not mandatory which can used to provide a set of interoperable claims. Some of the registered claims are: iss (issuer), exp (expiration time), sub (subject), aud (audience) and so on.
- Public Claims - Claims that we create by ourselves which contains generic informations like user name, email, address and so on.
Some of the public claims in IANA JSON Web Token Claims Registry registered by OpenId Connect(OICD) are: auth_time, acr, nonce. - Private Claims - These are the custom claims which can be created to share information. This could be the employee Id, department name or any other specific information.
iii.) Signature
To create a signature you will need the encoded header, payload, a secret key, the algorithm specified in the header and sign that.
Say for example, you want use the HMAC SHA256 algorithm, the signature could be created using the below method.
HMACSHA256(
base64UrlEncode(header) + "." +
base64UrlEncode(payload),
secret)
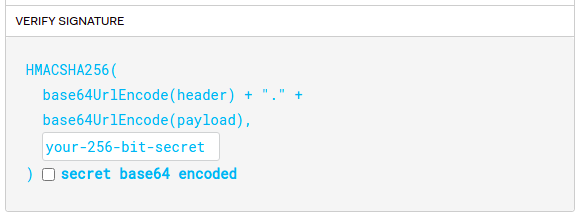
#4 Use of JWT for API Authentication
JWT token’s most common use is to use it as an API (Application Programming Interface) authentication mechanism. Therefore, it is usually recommended that one must only use a JWT token for this purpose.
JWT for API authentication is so well known and popular that even Google uses it to let its users authenticate to their APIs.
When one sets up an API, they receive a secret token from the service. Using the secret token, one can then create the token using several libraries on the client-side to sign it. One can pass the token as a part of the API request, and the server correctly identifies the specific client, as the request is signed with its unique identifier. Signed tokens verify the integrity of the claims that it contains within.
#5 When Should you use Json Web Tokens?
Here are few scenarios on when should we consider using the Json Web tokens.
a.) Authentication
One of the most common scenario of using JWT is authentication. When an user logs in with the credentials like username and password, each subsequent request will include the JWT, which allows the user to access routes, services and resources. JWT has been widely used in a function called Single Sign On (SSO) because of its small overhead and also it can be easily used across different domains.
b.) Information Exchange
Any kind of data can be transmitted securely between parties since they can be signed, which means you will know who the senders are and furthermore the structure of JWT allows users to verify whether the transmitted data has been tampered with or not.
#6 How can one expire a single token?
When one needs to invalidate a single token, a simple and effortless solution is to change the secret server key. Changing the secret server key invalidates all the tokens. Although this is an effortless solution, it is not ideal for users who do not want to have their single token expired without any reason.
Then what is the correct solution?
One way to expire a single token is to add a property to the user object present in the server database. For example, this method references the date and time the server was first created at.
It is the iat property in which the token stores this value automatically. Thus, each time a user checks the token, they can compare the server-side user property with the iat value.
In order to invalidate the single token, the user must simply update the server-side value. Likewise, if the iat value is older than the server-side value, the user must simply reject the particular single token.
Another alternative to expire the single token is to have a blacklist in the user’s database cached in memory or a whitelist (which is much better and preferred).
#7 How to store JWTs securely?
One is required to store a JWT inside a user’s browser in a safe place. However, storing it inside a LocalStorage is not safe, as any script can access it inside a page. This can lead to an external attacker accessing your token.
Thus, one must never store it in session storage or local storage. Furthermore, if the third-party scripts included in your page are compromised or hacked by the attacker, it is able to access all your user’s token.
Therefore, one must save and store a JWT inside an httpOnly Cookie. This cookie is a special type of cookie that is sent to a server only in HTTP requests. This cookie is also not accessible for reading or writing from JavaScript running in the browser.
JWT ensures a secure exchange of data and information between the two servers. Furthermore, as JWT is signed, the receiver rests assured that the client is really the one they think they are.
#8 Use of JWT for SPA authentication
JWTs are also used as authentication mechanisms that do not require a database. The server does not use or refrain from using a database because the data or information stored in the JWT is safely sent to the client.
#9 Use of JWT to authorize operations across server
Suppose you are logged in to a server that redirects you to another server to perform some type of operation. The first server issues a JWT to the user that authorizes them to the other user. There is no requirement for those two servers to share a common session for the user’s authentication purpose. This makes the token perfect and ideal for this use case.
#10 Choosing the perfect JWT library
To choose an ideal JWT library, the user must head to jwt.io and look at the available Libraries list. In addition, the web page contains a list of the most used and famous libraries that implement JWT.
The user must select the language of choice and pick the preferred library that consists of a higher number of green checks.
#11 When one must not use JWTs: Session tokens for regular web applications
Using JWTs for session tokens might seem like an ideal choice at first because of the following:
- It allows you to store any kind of user details on the client
- The server trusts the client because of the signed JWT, and there is no need for it to call the database to retrieve the data and information that is already stored in the JWT.
- The user need not coordinate the sessions in a centralized database when eventually the user gets to the problem of horizontal scaling.
If a user already has a database for their application, they can use the session table and regular sessions that are provided by the chosen server-side framework.
JWTs are sent to the server for each request, increasing the cost compared to the server-side sessions.
Moreover, despite the reduced security risks, sending JWTs using HTTPS, data deciphering and intercepting are possible, exposing the user’s private and confidential data and information.
Conclusion
JSON Web token is a famous web standard used by users to trust the requests using signatures and exchange data and information between the parties. It allows you to perform safe authentication in your applications.
One must ensure and make sure when it is the right choice to use it, when to avoid it, and when and how to avoid the most basic security issues.
#1 Solution for Logs, Traces & Metrics
APM
Kubernetes
Logs
Synthetics
RUM
Serverless
Security
More