Named arguments in PHP 8.0
PHP is a popular server-side programming language used to build dynamic websites and applications. With the release of PHP 8.0, there are several exciting new features that developers can take advantage of to improve their code and make it more efficient.
One such feature is named arguments, which allows developers to specify function arguments by name rather than just by position. This can make code more readable and flexible, but it also raises some compatibility issues when working with older versions of PHP.
In this topic, we will explore named arguments in PHP 8.0 and how they can be used to make code more expressive and maintainable.
Table of Content
- What are Named Arguments?
- Positional Arguments vs Named Arguments
- Default Values for Named Arguments
- Passing Arguments by Reference
- Mixing Positional and Named Arguments
- Best Practices for Named Arguments
- Compatibility of Named Arguments with Previous Versions
What are Named Arguments?
Named arguments is a new feature introduced in PHP 8.0, which allows you to pass arguments to a function or method by specifying the parameter name along with the value. This feature provides developers with more flexibility and improves code readability.
Traditionally, in PHP, function parameters are passed by position, which means the order in which arguments are passed to a function must match the order of the parameters in the function signature. In contrast, named arguments allow you to specify which parameter you are passing the argument to, without being constrained by the order.
Let us understand named arguments with a simple example, Let's say you have a function in PHP that takes multiple arguments, like this,
function createUser($username, $email, $password, $firstName, $lastName)
{
// Code to create user goes here
}
If you call this function without using named arguments, you have to make sure the arguments are in the correct order.
createUser('johndoe', 'johndoe@example.com', 'password123', 'John', 'Doe');
But what if you accidentally switch the order of the arguments, like this,
createUser('johndoe', 'password123', 'johndoe@example.com', 'John', 'Doe');
This will cause errors and the user won't be created properly, because the email and password are in the wrong positions. However, if you use named arguments, you can specify which argument corresponds to which parameter in the function, like this,
createUser(username: 'johndoe',email: 'johndoe@example.com',password: 'password123',firstName: 'John',lastName: 'Doe');
This way, even if you mix up the order of the arguments, the function will still work correctly because each argument is explicitly named.
In addition to avoiding errors, named arguments also make code more readable and self-documenting, because it's clear from the function call what each argument represents.
Positional Arguments vs Named Arguments
Positional arguments: Positional arguments are passed to a function based on their position or order in the argument list. The first argument is passed to the first parameter, the second argument is passed to the second parameter, and so on. The order of the arguments matters, and it's up to the caller to make sure that the correct value is passed to the correct parameter.
<?php
function multiply($a, $b)
{
return $a * $b;
}
$result = multiply(3, 4);
echo $result;
?>
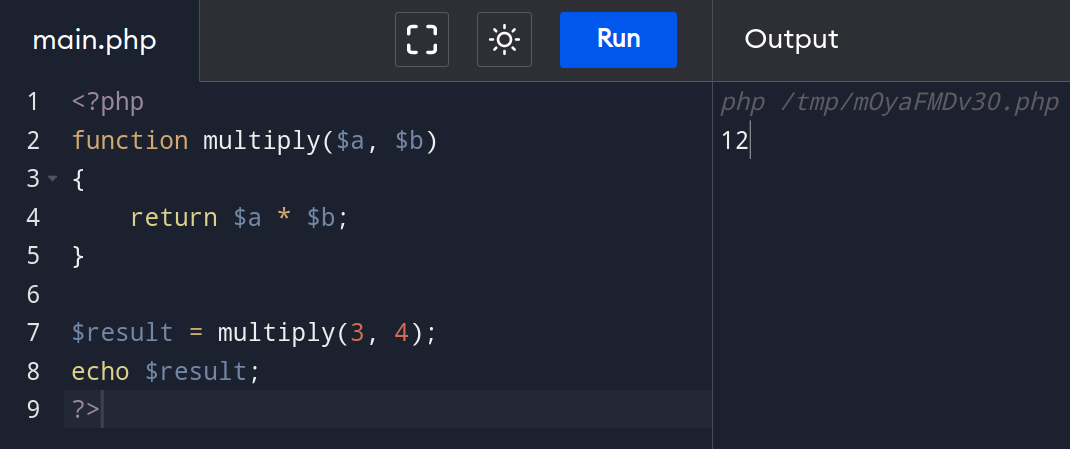
In this example, we have a function multiply()
that takes two parameters $a
and $b
, and returns the result of multiplying them together. When we call the function, we pass in two arguments 3
and 4
. These arguments are passed positionally, which means that the first argument 3
is assigned to the first parameter $a
, and the second argument 4
is assigned to the second parameter $b
. The function then returns the result of multiplying the two arguments together, which is 12
.
Named arguments: Named arguments, on the other hand, are passed to a function based on their names or labels, rather than their position. This allows the caller to specify which argument corresponds to which parameter explicitly, using the parameter name. This means that the order of the arguments does not matter, as long as each argument is labelled with the correct parameter name.
<?php
function multiply($a, $b)
{
return $a * $b;
}
$result = multiply(b: 4, a: 3);
echo $result;
?>
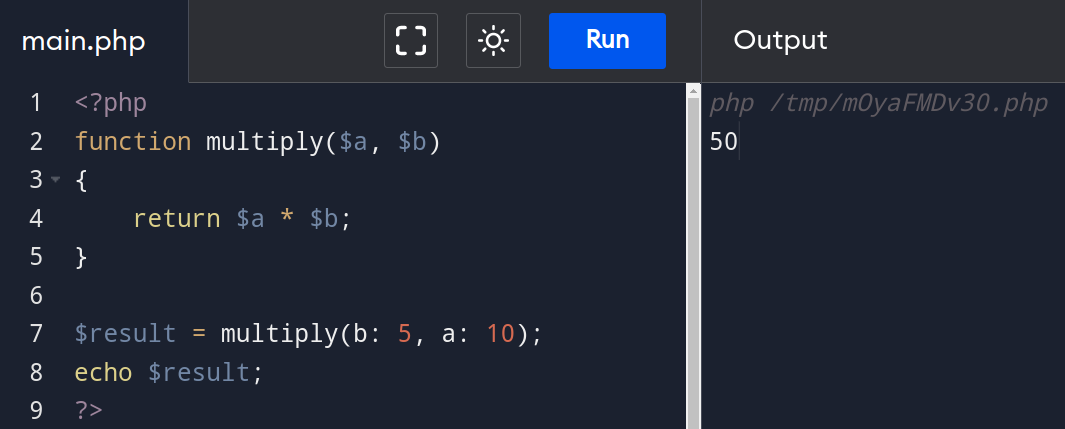
In this example, we have the same multiply()
function as before, but when we call it, we use named arguments instead of positional arguments. We pass in two arguments b: 4
and a: 3
. These arguments are passed by name, which means that we are explicitly specifying which argument corresponds to which parameter.
The order in which we pass the arguments doesn't matter anymore. The function assigns the value of 4
to the parameter $b
, and the value of 3
to the parameter $a
. The function then returns the result of multiplying the two arguments together, which is still 12
.
Overall, named arguments can make the code more readable and less prone to errors, especially when dealing with functions that have many parameters. By explicitly specifying the names of the arguments, it becomes easier to understand what each argument represents and what it's used for.
This can be particularly useful in codebases where there are many functions with similar parameters, or when working with code written by other developers.
Default Values for Named Arguments
In PHP 8.0, you can specify default values for named arguments in function definitions. Default values are values that are assigned to a parameter if no argument is passed for that parameter when the function is called.
Example: In this example, we have a function greet()
that takes one parameter $name
, which has a default value of "World"
.
<?php
function greet($name = "World")
{
echo "Hello, $name!";
}
greet();
?>
If no argument is passed for $name
when the function is called, the default value of "World"
is used. When we call the function with no arguments, it uses the default value of "World"
as shown below.
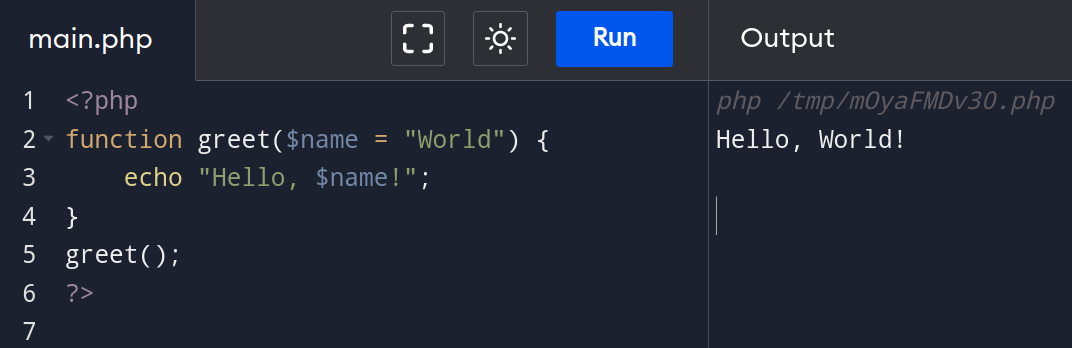
When we call the function with the argument "John"
, it overrides the default value and uses the value "John"
instead.
<?php
function greet($name = "World") {
echo "Hello, $name!";
}
greet("john");
?>
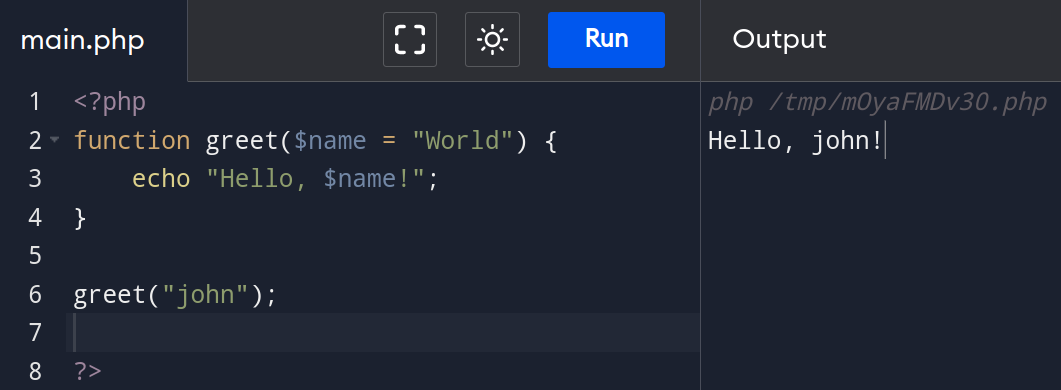
Overall, using default values for named arguments can make your code more flexible, especially when you have functions with many parameters. It allows you to provide sensible default values for parameters, while still allowing the caller to override them if necessary.
Passing Arguments by Reference
Passing arguments by reference in PHP 8.0 means that you can pass a variable to a function and modify its value directly, without creating a copy of the variable. When you pass a variable by reference using the &
symbol, any changes made to the variable inside the function will affect the original value of the variable outside the function as well.
This can be useful when you want to modify the value of a variable inside a function and have those changes reflected outside the function. However, it's important to be careful when using pass-by-reference in PHP, as it can lead to unexpected behaviour if not used correctly.
In this example, we have a function updateString()
that takes one parameter $str
by reference using the &
symbol. The function appends the string " updated" to the value of $str
.
<?php
function updateString(&$str)
{
$str .= " updated";
}
$text = "This is a test string";
updateString($text);
echo $text;
?>
We then set the variable $text
to "This is a test string". Next, we call the function updateString()
and pass $text
as the argument. Since we passed the argument by reference, any changes made to $str
inside the function will affect the original value of $text
outside the function as well. The function appends the string " updated" to the value of $str
, so the resulting value of $text
is "This is a test string updated".
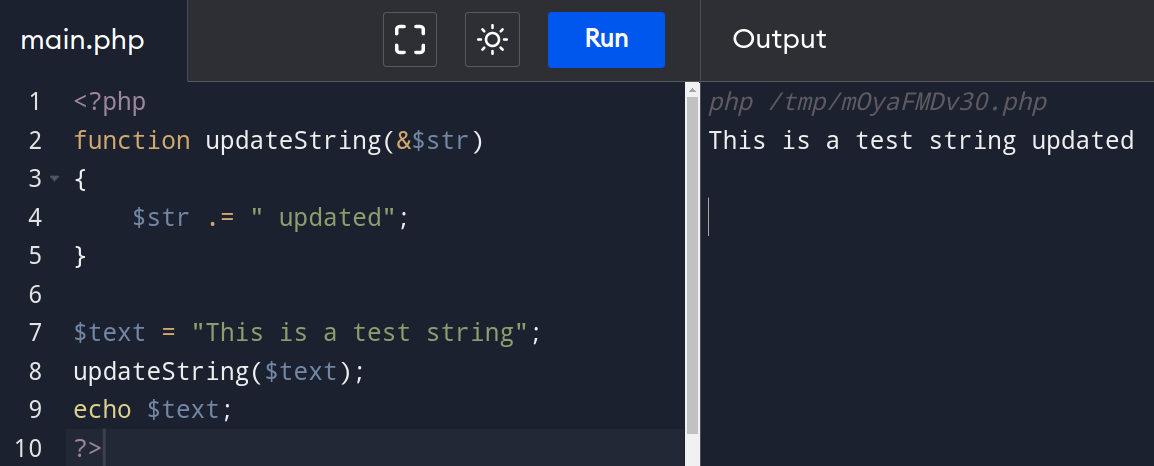
This example demonstrates how passing arguments by reference can be useful for modifying variables outside the function.
Mixing Positional and Named Arguments
In PHP 8.0, you can mix positional and named arguments in a function call. This means that you can pass some arguments by position, and some by name.
This can be useful when you have a long list of arguments and want to pass some of them by position and others by name. Mixing positional and named arguments can make your code more readable and easier to understand, as you can explicitly specify the names of the arguments that you are passing.
Let's take a look at an example to see how this works in practice.
function calculateTotal($price, $quantity, $discount = 0)
{
$total = $price * $quantity * (1 - $discount);
return $total;
}
// Mix positional and named arguments
$subtotal = calculateTotal(10, quantity: 5, discount: 0.2);
In this example, we have a function called calculateTotal()
that takes three parameters: $price
, $quantity
, and $discount
. The $discount
parameter has a default value of 0
.
In the function call, we pass the $price
argument by position with a value of 10
. Then, we pass the $quantity
argument by name using the syntax quantity: 5
, and we pass the $discount
argument by name using the syntax discount: 0.2
.
When we run the function call, it will calculate the total price after the discount and return 40
.
Notice that we used a default value for the $discount
parameter, so we didn't have to pass it explicitly in the function call. This can be useful when some arguments are optional or have a common default value.
Best Practices for Named Arguments
Here are some recommended practices for using named arguments in PHP 8.0:
- Use clear and descriptive argument names: To improve the readability of your code, it is important to use clear and descriptive argument names that convey their intended purpose. Avoid generic names like
$arg1
and$arg2
, and instead use names that accurately reflect their function, such as$username
,$password
, or$remember_me
. - Avoid using argument names that are reserved keywords: Since PHP has reserved keywords that cannot be used as variable names, make sure to avoid using them as argument names to prevent potential issues.
- Test your functions and methods with various argument combinations: As named arguments provide greater flexibility in how arguments are passed to functions and methods, it is essential to test your code with various argument combinations, including required and optional arguments, as well as combinations of named and positional arguments, to ensure correct functionality.
- Use named arguments judiciously: Although named arguments can be helpful in certain circumstances, they should not be overused. In general, use named arguments when dealing with many arguments or optional arguments that might be skipped. For simple functions with only a few arguments, positional arguments may be more suitable.
- Document your functions and methods with clear examples of using named arguments: To assist other developers in understanding how to use your code properly, make sure to document your functions and methods with clear examples that illustrate how to use named arguments. This can prevent confusion and errors when using your code.
Compatibility of Named Arguments with Previous Versions
Named arguments is a new feature introduced in PHP 8.0 that allows developers to specify function arguments by name, rather than just by position. This feature can make the code more readable and flexible, especially when functions have many parameters or optional arguments.
However, using named arguments in PHP 8.0 may not be compatible with older versions of PHP. If you use named arguments in your code, it will result in a syntax error when running on an older version of PHP.
To handle backwards compatibility issues, there are a few approaches you can take:
- Avoid using named arguments in your code altogether. If your code needs to be compatible with older versions of PHP, you can simply stick to the traditional way of passing function arguments by position.
- Use named arguments conditionally. You can use the
PHP_VERSION_ID
constant to check if the current PHP version is 8.0 or later, and use named arguments only if it is. If the version is older, you can use positional arguments instead. - Use a compatibility layer. You can create a compatibility layer that uses named arguments in PHP 8.0 and positional arguments in earlier versions. This way, your code can be written using named arguments, but it will still work on older versions of PHP.
Overall, named arguments can be a useful feature in PHP 8.0, but it's important to keep backwards compatibility in mind if you need your code to run on older versions of PHP. By using conditional statements or a compatibility layer, you can make sure your code works across different PHP versions.
Conclusion
In conclusion, we have explored several important topics related to PHP 8.0, including named arguments, passing arguments by reference, and mixing positional and named arguments. We have seen how named arguments can make code more readable and flexible, but also discussed the compatibility issues it can raise when working with older versions of PHP.
We also examined how passing arguments by reference can impact the behavior of functions and how to avoid common pitfalls. Finally, we explored how mixing positional and named arguments can be used to provide a balance between clarity and flexibility.
Overall, PHP 8.0 provides developers with powerful new features and functionality that can help streamline their code and improve its readability. However, it's important to be aware of the potential compatibility issues and to take steps to ensure that code works across different PHP versions. By keeping these topics in mind, developers can take full advantage of the benefits of PHP 8.0 while avoiding common mistakes and pitfalls.
Atatus: PHP Performance Monitoring and Log Management
Atatus is an Application Performance Management (APM) solution that collects all requests to your PHP applications without requiring you to change your source code. However, the tool does more than just keep track of your application's performance.
Monitor logs from all of your PHP applications and systems into a centralized and easy-to-navigate user interface, allowing you to troubleshoot faster using PHP monitoring.
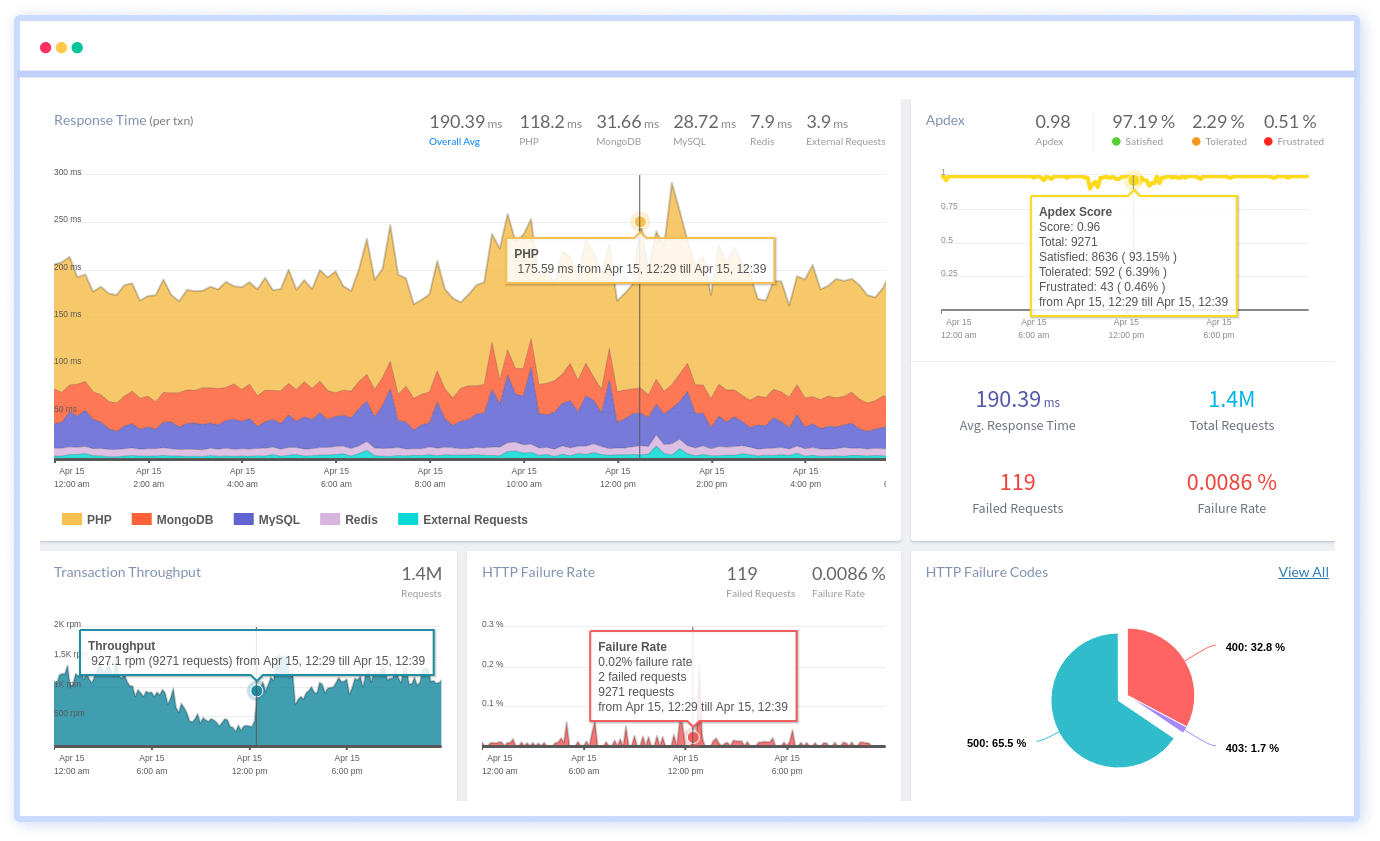
We give a cost-effective, scalable method to centralized PHP logging, so you can obtain total insight across your complex architecture. To cut through the noise and focus on the key events that matter, you can search the logs by hostname, service, source, messages, and more. When you can correlate log events with APM slow traces and errors, troubleshooting becomes easy.
#1 Solution for Logs, Traces & Metrics
APM
Kubernetes
Logs
Synthetics
RUM
Serverless
Security
More