An Introduction to PHP 8.0's Null Safe Operator
Have you ever come across situations in your code where you had to check for null values before performing operations? Did you find it tedious to keep writing these checks over and over again, leading to code that is hard to read and maintain? Well, what if I told you there is a solution that can simplify your code and reduce the chances of introducing bugs?
Introducing the null-safe operator! This operator is a new feature in PHP 8 that provides a concise and convenient way to perform null checks. With the null-safe operator, you can chain method and property accesses on potentially null values without having to check for null each time. This makes your code more concise, easier to read, and less error-prone.
So, how does the null-safe operator work? Well! In this article, we will explore the concept of null safe operator in PHP and understand its importance in modern PHP programming. So, let's get started!
Table of Contents
- What is Null Safe Operator in PHP ?
- Using the Null Safe Operator in Practice
- Difference between null coalescing and null safe operator
- When to use the null safe operator
- Common Pitfalls
What is Null Safe Operator in PHP ?
The null safe operator in PHP 8.0 helps to simplify the code and make it more readable. It is represented by the question mark '?' followed by the '->' operator, and it allows developers to perform operations on variables that may be null without throwing an error.
Before the null safe operator, developers had to perform explicit checks to see if a variable was null before attempting to use it. This led to an excessive amount of repetitive code, which not only made the code harder to read but also increased the risk of introducing bugs.
With the null safe operator, developers can now use a simple syntax to access a property or method of an object, even if the object is null. If the object is null, the operation simply returns null without throwing any errors.
Using the Null Safe Operator in Practice
Let us consider a scenario where we have an array of objects and we want to access a property of an object if it exists.
$users = getUsers();
if ($users !== null && count($users) > 0 && isset($users[0]->name)) {
$name = $users[0]->name;
}
In this code, we first check if the returned array is not null, then we check if it has at least one element, and finally, we check if the name
property of the first element exists before accessing it. This code is quite long and can be error-prone.
With the null safe operator, we can simplify the above code,
$name = getUsers()?.[0]?->name;
Here, we use the null safe operator ?.
to check whether the getUsers()
function returns a non-null value before accessing the first element of the array with [0]
, and then we use ?->
to access the name
property of the object. If any of these checks fail, the entire expression evaluates to null without throwing an error. This simplifies the code and makes it more readable and less error-prone.
Additionally, it can help prevent runtime errors and make the code more maintainable, as it reduces the likelihood of having to fix null-related issues in the future.
Difference between null coalescing and null safe operator
Both null coalescing and null safe operator are used to handle null values in PHP, but they work in different ways.
Null coalescing operator ??
is used to assign a default value to a variable when the value is null. The operator checks if the value on the left is null, and if it is, it returns the value on the right. If the value on the left is not null, it returns that value instead.
$myVar = $someVar ?? "default value";
In this example, if $someVar
is null, $myVar
will be assigned the value "default value". If $someVar
is not null, $myVar
will be assigned the value of $someVar
.
On the other hand, null safe operator ?->
is used to access properties or methods of an object when the object may be null. It works by checking if the object is null, and if it is, it returns null without trying to access the property or method. If the object is not null, it tries to access the property or method as usual.
$myObj = new MyClass();
$myVar = $myObj?->myProperty?->myMethod();
In this example, $myVar
will be assigned the result of calling the myMethod()
method on the myProperty
property of the $myObj
object. However, if $myObj
is null or myProperty
is null, $myVar
will be assigned null instead of throwing an error.
The key difference between the two operators is that null coalescing operator is used to assign a default value to a variable, while null safe operator is used to access properties or methods of an object that may be null.
In general, null coalescing operator is useful when you want to assign a default value to a variable when it is not set or null, while null safe operator is useful when you want to access properties or methods of an object without worrying about whether the object is null or not.
It is important to note that null safe operator is only available in PHP 8 or later, so if you are using an earlier version of PHP, you will need to use alternative methods to handle null values.
When to use the null safe operator
So when should developers use the null safe operator? Let's explore some scenarios where it can be particularly useful.
1. Chaining method calls on null objects
In traditional PHP code, chaining method calls on null objects would result in a fatal error. With the null safe operator, developers can safely chain method calls on null objects without worrying about errors.
For example, let's say we have a User object with a getAccount method that returns an Account object, which in turn has a getBalance method. Without the null safe operator, we would need to write code like this to avoid errors.
if ($user !== null && $user->getAccount() !== null) {
$balance = $user->getAccount()->getBalance();
}
With the null safe operator, we can simplify the code to,
$balance = $user?->getAccount()?->getBalance();
2. Accessing object properties on null objects
Similar to the first scenario, accessing object properties on null objects can also be made safer and more concise with the null safe operator.
For example, let's say we have a Config object with a $settings property that contains an array of configuration settings. Without the null safe operator, we would need to write code like this,
if ($config !== null && isset($config->settings)) {
$timezone = $config->settings['timezone'] ?? 'UTC';
}
With the null safe operator, the code can be simplified as given below,
$timezone = $config?->settings['timezone'] ?? 'UTC';
3. Simplifying code with optional method parameters
Sometimes, a method may have optional parameters that can be null. In traditional PHP code, we would need to perform null checks on these parameters to avoid errors. For example, let's say we have a method that takes an optional User object parameter. Without the null safe operator,
function getUserName($user = null)
{
$name = '';
if ($user !== null && $user->getName() !== null)
{
$name = $user->getName();
}
return $name;
}
With the null safe operator, the code can be simply written as
function getUserName($user = null)
{
return $user?->getName() ?? '';
}
4. Working with external data sources
When working with external data sources, such as APIs or databases, there may be cases where data is missing or incomplete. In such cases, the null safe operator can be used to handle null data gracefully. For example, let's say we have an API that returns a JSON response with nested data.
Without null safe operator,
$response = json_decode($json, true);
if (isset($response['data']['user']['email']))
{
$email = $response['data']['user']['email'];
}
With null safe operator,
$response = json_decode($json);
$email = $response?->data?->user?->email ?? '';
It is important to consider the context of the code and whether null values are likely to be encountered in a particular situation before deciding to use the null safe operator. Ultimately, the decision to use the null safe operator should be based on a careful evaluation of the specific situation and the potential benefits and drawbacks of using it.
Common Pitfalls
- Overusing null safe operators: While null safe operators can be a helpful tool, it is important not to overuse them. Overusing null safe operators can make your code harder to read and maintain, and can also mask underlying issues with your code. Only use null safe operators when they are truly necessary.
- Dependency on language version: Null safe operators were introduced in PHP 8.0, so if you are using an earlier version of PHP, you will not be able to use this feature. Be aware of this dependency when deciding whether to use null safe operators in your code.
- Unintended consequences: When using the null safe operator, it's important to ensure that it's not masking other issues or problems in the code.
- Code maintenance: If the codebase has a mix of null safe and traditional null checks, it can be harder to maintain and understand the code.
- Potential performance impact: While null safe operators can improve the readability of your code, they can also have a slight performance impact. This is because the operator must check whether a value is null before performing the operation. Be aware of this potential impact when deciding whether to use null safe operators in performance-critical parts of your code.
Conclusion
The null safe operator is a useful feature that can simplify code and reduce the risk of errors when dealing with potentially null values. It provides a concise way to check for null values without the need for a larger code. The key advantage of using the null safe operator is that it allows for cleaner and more readable code, as well as reducing the likelihood of null-related errors.
However, it is important to use the null safe operator judiciously, as overuse can make code harder to understand and maintain. It is also important to be aware of the potential pitfalls, such as the risk of masking errors or failing to handle unexpected null values. Careful testing and code review can help to mitigate these risks.
Overall, the null safe operator can be a powerful tool in the PHP developer's toolkit when used properly. Developers can leverage the benefits of the null safe operator to write cleaner, more maintainable, and less error-prone code by adhering to the best practices and being mindful of the possible pitfalls.
Atatus: PHP Performance Monitoring and Error Tracking
Atatus is an Application Performance Management (APM) solution that collects all requests to your PHP applications without requiring you to change your source code. However, the tool does more than just keep track of your application's performance.
Atatus PHP monitoring, tracks and provides real-time notifications for every error, exception, and performance problem that occurs within the application. .
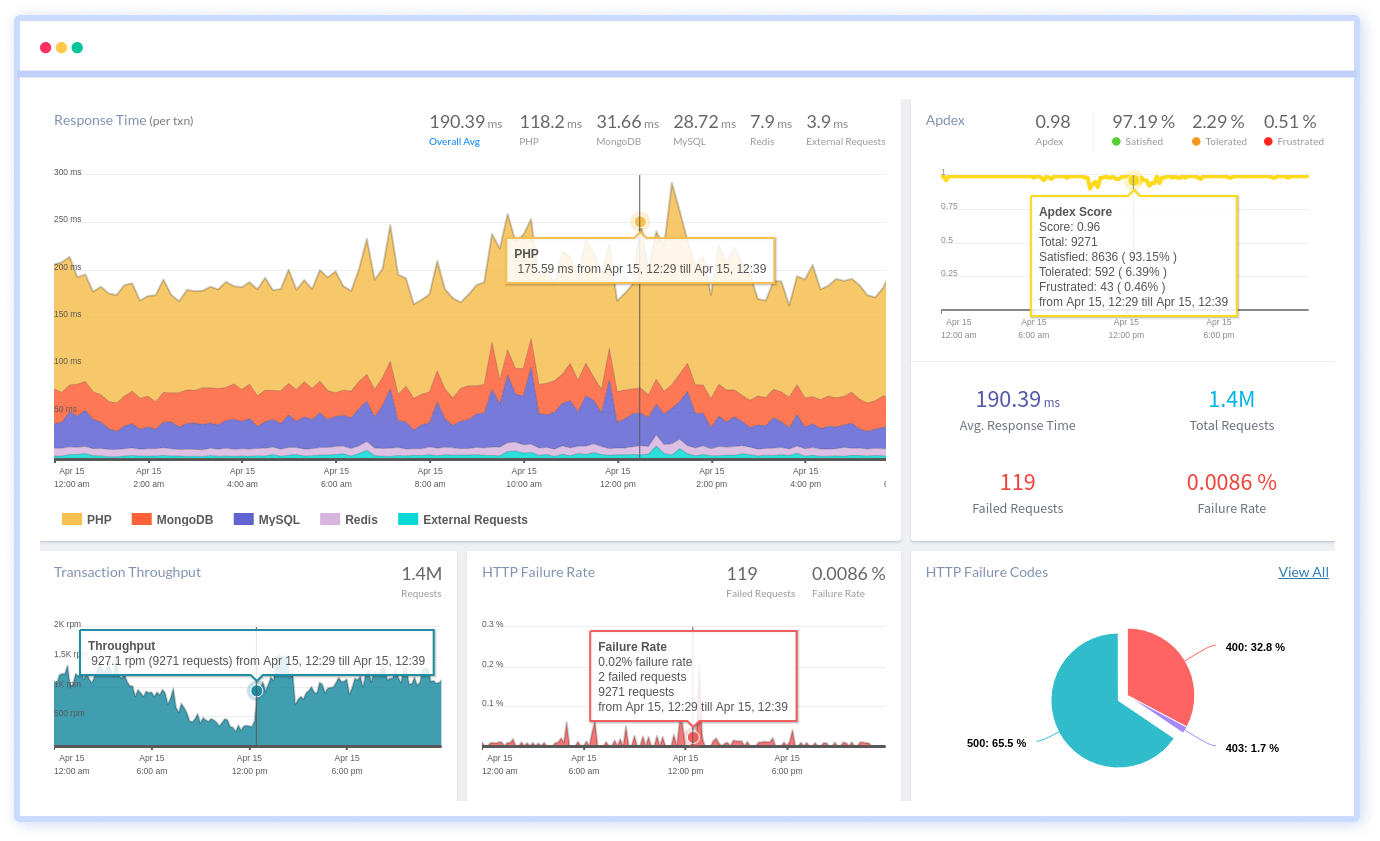
By tracking KPIs such as response times, throughput, HTTP failure rates, and error rates, it can help users identify and address any performance issues before they impact the application's users.
Furthermore, Atatus supports multiple PHP framework such as WordPress, Magento, Laravel, CodeIgniter, CakePHP, Symfony, Yii and Slim making it an adaptable tool that can be used to monitor applications across different frameworks and platforms.
#1 Solution for Logs, Traces & Metrics
APM
Kubernetes
Logs
Synthetics
RUM
Serverless
Security
More