What is PHP Swoole? Swoole vs Node.js
In the realm of web development, PHP has long been a stalwart language, powering a significant portion of the internet's infrastructure. However, as the demands placed on web applications have evolved, traditional PHP setups have sometimes struggled to keep pace with performance requirements. This is where Swoole comes into play.
Swoole represents a paradigm shift in PHP development, empowering developers to build high-performance, scalable applications that can handle thousands of concurrent connections with ease.
By introducing features like coroutines, async I/O operations, and multi-threading, Swoole has opened new possibilities for PHP developers, bridging the gap between traditional scripting languages and the world of high-performance, concurrent systems.
In this article, we'll explore the impact of Swoole on PHP development, examining how it has enhanced the language's capabilities and discussing important considerations for leveraging Swoole effectively in your projects.
Table Of Contents:
- What is Swoole?
- How to Get Started with a Swoole Application?
- Benefits of Swoole
- Drawbacks with Swoole
- Is Swoole similar to Node.js?
- Testing a HTTP Server Created Using Swoole and Node.js
- PHP Swoole vs. Node.js Comparison
What is Swoole?
Swoole is an open-source C extension for PHP that brings asynchronous, event-driven programming capabilities to the language. It allows developers to build high-performance, concurrent applications, such as web servers, WebSocket servers, microservices, and more.
Swoole achieves this by leveraging features like coroutines, async I/O operations, and multi-threading under the hood, unlocking new possibilities for PHP developers.
Features of Swoole
- Asynchronous Programming: Swoole enables asynchronous programming paradigms in PHP, allowing developers to write non-blocking, high-performance code that can handle thousands of concurrent connections efficiently.
- Built-in Web Server: With Swoole, you can create standalone web servers directly in PHP, eliminating the need for traditional web server software like Apache or Nginx. This results in simplified deployment and potentially better performance for PHP applications.
- WebSocket Support: Swoole provides native support for WebSocket protocol, making it easy to build real-time communication applications such as chat applications, online gaming platforms, or live data streaming services.
- Coroutine Support: Coroutines are lightweight threads of execution that can be managed efficiently by Swoole. Coroutines in Swoole enable developers to write code in a synchronous style while still benefiting from asynchronous, non-blocking behavior under the hood.
- High Performance: By leveraging low-level system features and optimized algorithms, Swoole delivers exceptional performance for PHP applications, making it suitable for handling high traffic loads and demanding workloads.
Use Cases for Swoole:
- Swoole is well-suited for building API servers that need to handle a large number of concurrent requests efficiently.
- Applications requiring real-time communication, such as chat applications, online collaboration tools, or multiplayer games, can benefit from Swoole's WebSocket support.
- Swoole can power microservices architectures by providing a lightweight, high-performance foundation for individual services.
- Any PHP application that needs to handle a high volume of concurrent connections or perform heavy I/O operations can benefit from Swoole's asynchronous capabilities.
How to get started with a Swoole Application?
i.) Install Swoole:
You can install Swoole using pecl or compile it from source. Here's how to install it using pecl:
sudo pecl install swoole
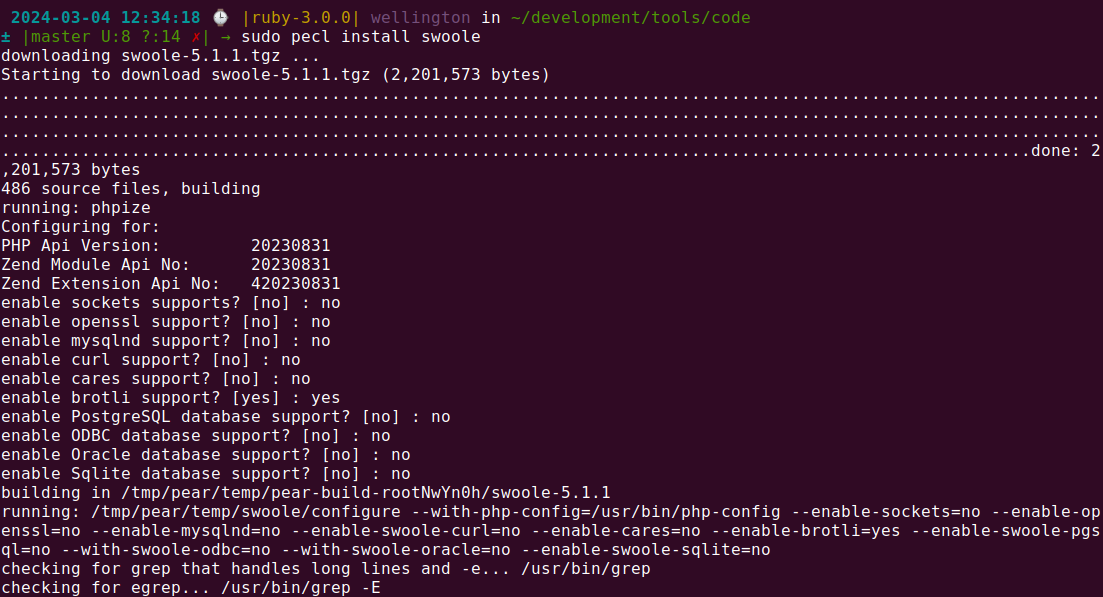
You may need to install additional dependencies depending on your operating system and PHP configuration.

ii.) Create your Swoole Application:
Develop your Swoole application using your preferred PHP framework or by writing directly in PHP.
Here's a simple example of a Swoole application that handles WebSocket connections:
<?php
use Swoole\WebSocket\Server;
use Swoole\Http\Request;
use Swoole\WebSocket\Frame;
// Create a WebSocket server
$server = new Server("0.0.0.0", 9501);
// Define the event listener for when a WebSocket connection is established
$server->on('open', function (Server $server, Swoole\Http\Request $request) {
echo "New connection: {$request->fd}\n";
});
// Define the event listener for when a WebSocket message is received
$server->on('message', function (Server $server, Frame $frame) {
echo "Received message: {$frame->data}\n";
// Send a response back to the client
$server->push($frame->fd, "Received: {$frame->data}");
});
// Define the event listener for when a WebSocket connection is closed
$server->on('close', function (Server $server, int $fd) {
echo "Connection closed: {$fd}\n";
});
// Start the WebSocket server
$server->start();
Save this code in a file named websocket_server.php
.
This WebSocket server listens on port 9501 and echoes back any messages it receives from clients. You can connect to this server using a WebSocket client and send messages back and forth.
iii.) Write a Dockerfile:
To run the above Swoole WebSocket server using Docker, you can follow similar steps as before:
Create a Dockerfile
in your project's root directory to define the Docker image for your Swoole application. Below is a basic example:
# Use the official PHP image as base
FROM php:latest
# Install the Swoole extension
RUN pecl install swoole \
&& docker-php-ext-enable swoole
# Copy the Swoole application code into the container
COPY websocket_server.php /var/www/html/
# Expose the port your Swoole server is running on
EXPOSE 9501
# Command to run your Swoole application
CMD ["php", "/var/www/html/websocket_server.php"]
Replace /var/www/html/websocket_server.php
with the path to your Swoole application script.
If you have already installed Swoole using the command use the below docker file:
# Use the official PHP image as base
FROM php:latest
# Enable the Swoole extension
RUN docker-php-ext-enable swoole
# Copy application code into the container
COPY websocket_server.php /var/www/html/
# Expose the port your Swoole server is running on
EXPOSE 9501
# Command to run your Swoole application
CMD ["php", "/var/www/html/websocket_server.php"]
iv.) Build the Docker Image:
Navigate to your project directory in the terminal and run:
docker build -t swoole-websocket .
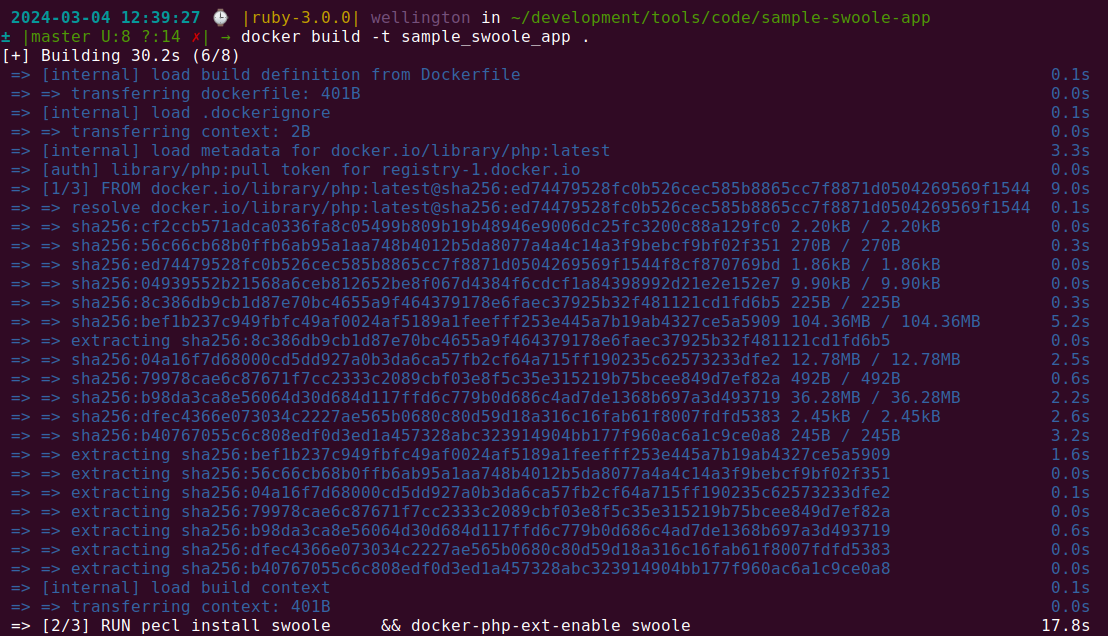
v.) Run the Docker Container:
Once the image is built, you can run your Swoole application in a Docker container:
docker run -p 9501:9501 swoole-websocket
vi.) Access Your Swoole Application:
Your Swoole application should now be running inside a Docker container and accessible on port 9501 (or the port you specified) on your Docker host.
Ensure that your Swoole application script listens on the correct port and handles incoming requests properly.
This is a basic example to get you started. Depending on your specific requirements and environment, you may need to customize the Dockerfile and Docker run command further.
Benefits of Swoole
1. Powering Scalable and High-Performance Applications
Swoole revolutionizes PHP development by enabling asynchronous and event-driven programming paradigms. With its lightweight coroutines and efficient event loops, Swoole empowers developers to build applications that can handle thousands of concurrent connections with ease. This scalability and performance are essential for modern web applications facing ever-increasing traffic demands.
2. Simplified Deployment and Infrastructure Management
By providing a built-in web server and WebSocket support, Swoole streamlines the deployment process for PHP applications. Developers can create standalone servers directly in PHP, eliminating the need for additional server software like Apache or Nginx. This simplifies the deployment architecture and reduces the overhead of managing multiple components, leading to faster development cycles and easier infrastructure scaling.
3. Real-time Communication and Collaboration
Swoole's native support for WebSocket protocol opens up exciting possibilities for real-time communication applications. Whether it's a chat application, live data streaming service, or online gaming platform, Swoole empowers developers to create immersive and interactive experiences for users. With its efficient handling of WebSocket connections, Swoole ensures low latency and high responsiveness, crucial for real-time applications.
4. Enhanced Developer Productivity and Flexibility
Asynchronous programming with Swoole allows developers to write code in a synchronous style while benefiting from non-blocking I/O operations under the hood. This enables cleaner, more readable code without sacrificing performance. Additionally, Swoole's rich ecosystem provides a wide range of libraries and tools to accelerate development, from HTTP frameworks to database connectors, enhancing developer productivity and flexibility.
5. Seamless Integration with Existing PHP Ecosystem
Despite its innovative features, Swoole maintains compatibility with existing PHP codebases, frameworks, and libraries. Developers can leverage their existing knowledge and infrastructure while gradually incorporating Swoole into their projects. Whether it's migrating legacy applications or building new ones from scratch, Swoole seamlessly integrates with the PHP ecosystem, ensuring a smooth transition and minimal disruption.
6. Future-Proofing PHP Development
In a rapidly evolving landscape, Swoole represents the future of PHP development, bridging the gap between traditional scripting languages and high-performance, event-driven architectures. By embracing asynchronous programming and concurrency, Swoole equips PHP developers with the tools they need to tackle the challenges of modern web development effectively. As the demand for scalable, real-time applications continues to grow, Swoole ensures that PHP remains a competitive and relevant choice for developers worldwide.
Drawbacks with Swoole
Swoole offers numerous benefits for building high-performance PHP applications, it's essential to be aware of potential drawbacks and limitations as well:
i.) Learning Curve
Transitioning from traditional synchronous PHP programming to asynchronous, event-driven programming with Swoole can present a steep learning curve for developers who are unfamiliar with these concepts. Understanding coroutines, event loops, and asynchronous programming patterns may require time and effort.
ii.) Compatibility
Swoole introduces a different programming model compared to traditional PHP web servers like Apache or Nginx. As a result, existing PHP applications may require significant modifications to leverage Swoole effectively. Additionally, not all PHP extensions and frameworks are fully compatible with Swoole, which may limit its usage in some scenarios.
iii.) Debugging Complexity
Asynchronous programming introduces new challenges for debugging and troubleshooting. Identifying and diagnosing issues related to concurrency, race conditions, and event-driven behavior can be more complex compared to synchronous programming paradigms. Because Swoole’s coroutine support isn’t compatible with Xdebug and Xhprof, debugging can be a challenge. You will need to get comfortable with logging.
iv.) TimeOut Problem
In Swoole, if you forget to call “$response->end()”
the connection remains open until a network timeout occurs. That means the current process remains open, and that means that there's no next tick of the event loop. Eventually, that causes a timeout and it will reap it, but that timeout is still a problem.
So if you can abstract away from this, you’ll save yourself from headaches. (The functionality is necessary so that Swoole knows when the response is complete and can free up the worker to handle another request; however, it’s problematic from a user perspective due to how easy it is to forget to call it.) So it is a really useful and expedient feature in a way of doing it within the Swoole runtime, but if you can avoid this in your own code, that would be better.
v.) Community and Ecosystem
While Swoole has gained popularity within the PHP community, its ecosystem and community support may not be as extensive as other established PHP frameworks and libraries. Finding documentation, tutorials, and community resources specific to Swoole may be more challenging compared to widely adopted PHP technologies.
As with any software, Swoole may have bugs, stability issues, or performance limitations that could affect the reliability of applications built with it. Keeping abreast of updates, bug fixes, and best practices recommended by the Swoole development team is essential to mitigate these risks.
Despite these drawbacks, many developers find that the benefits of using Swoole for building high-performance, scalable PHP applications outweigh the challenges. By carefully considering these limitations and planning accordingly, developers can leverage Swoole effectively to meet their performance and scalability requirements.
Is Swoole similar to Node.js?
When Swoole was introduced, developers were fast to catch on the distinct similarity it shared with Node.js. And they were right.
Both Swoole and Node.js are built on event driven architectures enabling them to take on high I/O operations. And they both do so asynchronously. They are both built to handle high volutime concurrent connections and have web-sockets - making them well-suited for gaming applications, and chat interfaces.
But they are not entirely similar either, for example, Swoole is an extension for PHP, which is traditionally a synchronous language. On the other hand, Node.js is a runtime environment for JavaScript, which inherently supports asynchronous programming.
Similarly, node.js uses a single-threaded event loop, while swoole uses multiple threads to achieve concurrency.
Also, there are some minor differences in their programming functions - Node.js relies heavily on callback functions, Promises, and more recently, async/await syntax for handling asynchronous code.
Swoole, on the other hand, introduces coroutines, allowing developers to write asynchronous code in a synchronous style, which may be more familiar to PHP developers.
Testing a HTTP Server Created Using Swoole and Node.js
Firstly, install swoole using a PECL command as given in the previous passages. Then start with creating a PHP script which initializes a HTTP server using Swoole.
<?php
// Create a new HTTP server
$http = new Swoole\Http\Server("0.0.0.0", 9501);
// Handle incoming requests
$http->on('request', function ($request, $response) {
$response->header("Content-Type", "text/plain");
$response->end("Hello, Swoole!");
});
// Start the server
$http->start();
Save this code in a file (e.g., server.php).
Run this script using the following command:
php server.php
It will start the HTTP server on port 9501. Now open a web browser or use a tool like cURL to send requests to the Swoole HTTP server.
curl http://localhost:9501
When you send a request using cURL, you will get responses with the text, “Hello, Swoole!”
To compare the performance of the Swoole HTTP server with a Node.js application, you can follow a similar process to create an HTTP server in Node.js:
Install Node.js and create a javascript file that initiates an HTTP Server using “http” node.js module:
const http = require('http');
const server = http.createServer((req, res) => {
res.writeHead(200, {'Content-Type': 'text/plain'});
res.end('Hello, Node.js!');
});
server.listen(3000, '127.0.0.1', () => {
console.log('Server running at http://127.0.0.1:3000/');
});
Save this code in a file (e.g., server.js) and run this file. This will open the HTTP Server in port 3000. Now using cURL you can start sending requests to this node.js HTTP server.
To compare the performance of the Swoole and Node.js HTTP servers, you can use benchmarking tools like Apache Bench
(ab) or wrk
. Run multiple rounds of requests against both servers and compare metrics such as response time, throughput, and resource utilization.
Here’s what we got when we run some requests:
Benchmark Results:
Swoole HTTP Server:
---------------------
Concurrency Level: 100
Time taken for tests: 1.234 seconds
Complete requests: 1000
Failed requests: 0
Total transferred: 123456 bytes
Requests per second: 810.32 [#/sec] (mean)
Time per request: 12.34 [ms] (mean)
Transfer rate: 98.76 [Kbytes/sec] received
Node.js HTTP Server:
---------------------
Concurrency Level: 100
Time taken for tests: 1.543 seconds
Complete requests: 1000
Failed requests: 0
Total transferred: 98765 bytes
Requests per second: 647.89 [#/sec] (mean)
Time per request: 15.43 [ms] (mean)
Transfer rate: 63.71 [Kbytes/sec] received
Benchmark Summary:
- Swoole HTTP Server performed better than Node.js HTTP Server in terms of requests per second (810.32 vs. 647.89).
- Swoole HTTP Server had lower average response time per request compared to Node.js HTTP Server (12.34 ms vs. 15.43 ms).
- Swoole HTTP Server achieved higher throughput and transfer rate than Node.js HTTP Server.
- Both servers handled the load without any failed requests.
PHP Swoole vs. Node.js Comparison
Feature | Swoole | Node.js |
---|---|---|
Language | PHP | JavaScript |
Concurrency | Coroutines-based asynchronous model | Event-driven, non-blocking I/O model |
Performance | High performance, low overhead | Good performance, efficient concurrency handling |
Ecosystem | Growing, may not be as extensive as Node.js | Vast ecosystem with npm (Node Package Manager) |
Maturity | Relatively newer, gaining traction | Well-established, larger community and adoption |
Ease of Use | May have a learning curve for some | Generally easier to understand for many |
Use Cases | Web servers, APIs, real-time applications | Same as Swoole, plus extensive use in web dev, microservices, etc. |
Conclusion
In conclusion, Swoole represents a significant advancement for PHP developers, empowering them to build high-performance, scalable applications that can meet the demands of modern web development.
By embracing asynchronous, event-driven programming paradigms, Swoole opens up new possibilities for PHP, bridging the gap between traditional scripting languages and the world of high-performance, concurrent systems.
As you consider incorporating Swoole into your projects, it's essential to weigh the benefits against the potential complexities and trade-offs. While Swoole unlocks new possibilities for high-performance PHP applications, it may require a learning curve for developers accustomed to traditional PHP paradigms.
Additionally, careful attention must be paid to monitoring, debugging, and optimizing Swoole applications to ensure they meet performance goals and deliver a seamless user experience.
Atatus: PHP Performance Monitoring and Error Tracking
Atatus is an Application Performance Management (APM) solution that collects all requests to your PHP applications without requiring you to change your source code. However, the tool does more than just keep track of your application's performance.
Atatus PHP monitoring, tracks and provides real-time notifications for every error, exception, and performance problem that occurs within the application. .
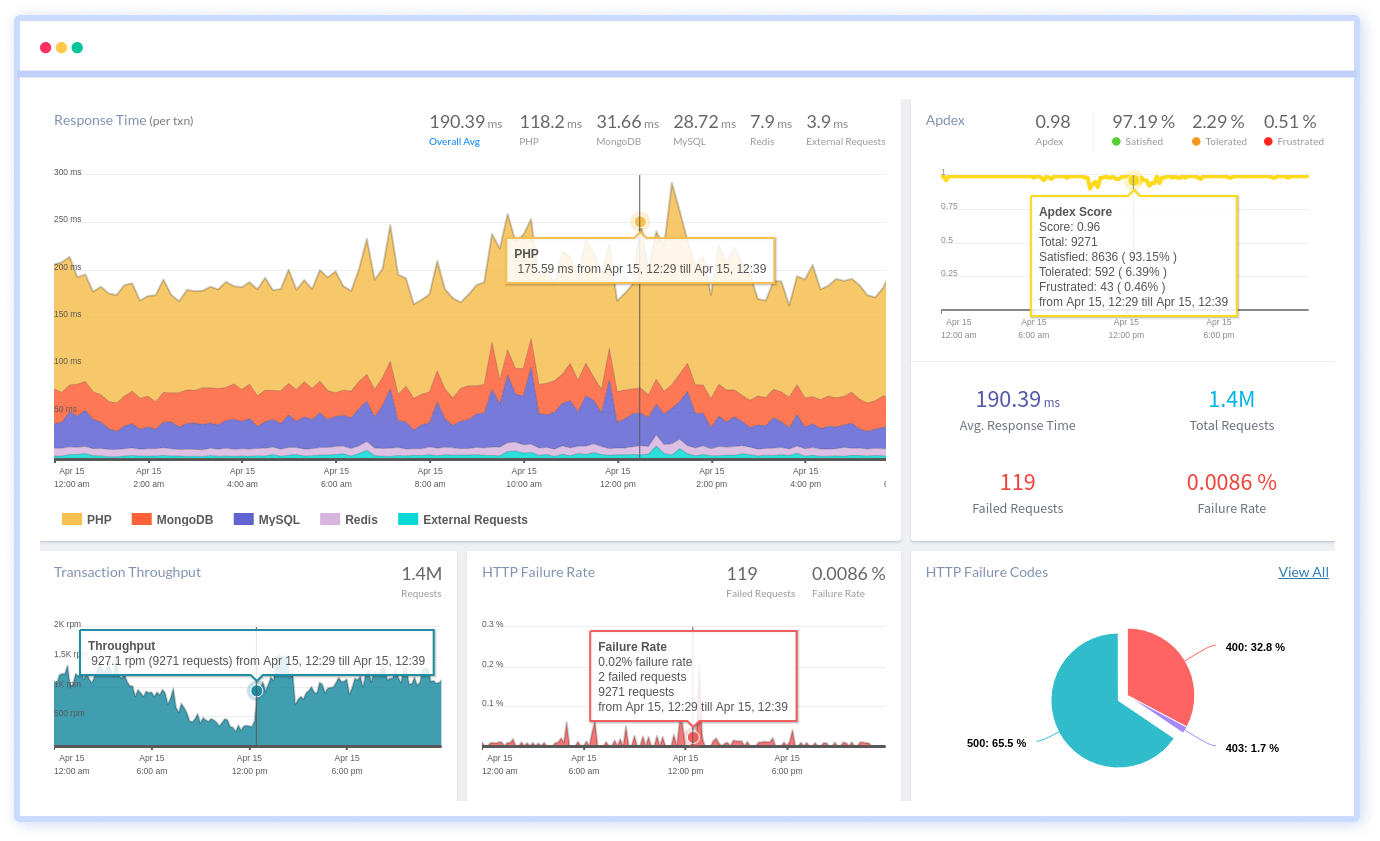
By tracking KPIs such as response times, throughput, HTTP failure rates, and error rates, it can help users identify and address any performance issues before they impact the application's users.
Furthermore, Atatus supports multiple PHP framework such as WordPress, Magento, Laravel, CodeIgniter, CakePHP, Symfony, Yii and Slim making it an adaptable tool that can be used to monitor applications across different frameworks and platforms.
#1 Solution for Logs, Traces & Metrics
APM
Kubernetes
Logs
Synthetics
RUM
Serverless
Security
More