View Models vs. View Composers: Understanding the Differences in Laravel
Are you familiar with Laravel, the popular PHP web application framework? If so, you may have heard of two key concepts in Laravel's view system: view models and view composers. While these terms may sound similar at first, they actually represent two distinct approaches to organizing and preparing data for views.
Understanding the differences between view models and view composers can help you structure your Laravel projects more effectively and improve the performance and maintainability of your code.
In this context, we will explore the key differences between view models and view composers in Laravel, highlighting their respective strengths and use cases. By the end of this discussion, you will have a clear understanding of when to use each approach and how to implement them in your own projects.
So let's dive in and explore the world of Laravel view models and view composers!
Table of Content
- Laravel's View System and its working
- View Models vs View Composers
- When to Use View Models and When to Use View Composers?
- How to Implement View Models and View Composers in Laravel?
- Performance Considerations for Using View Models and View Composers
Laravel's View System and its working
Laravel's view system is a way to render HTML templates and display them in a web browser. It allows developers to separate the presentation layer from the application logic, making it easier to maintain and modify the code.
The view system in Laravel is based on a template engine called Blade, which allows for easy and powerful template inheritance, conditional statements, loops, and more. The Laravel view system works by combining the power of the Blade template engine with the MVC (Model-View-Controller) architecture.
When a user sends a request to a Laravel application, the request is first routed to a controller. The controller retrieves the data required for the view from the model and passes it to the view.
The view is then responsible for rendering the HTML that will be displayed to the user. The view contains a mix of HTML and PHP code, and it is responsible for displaying the data passed to it by the controller. The Blade template engine allows for easy and powerful template inheritance, conditional statements, loops, and more.
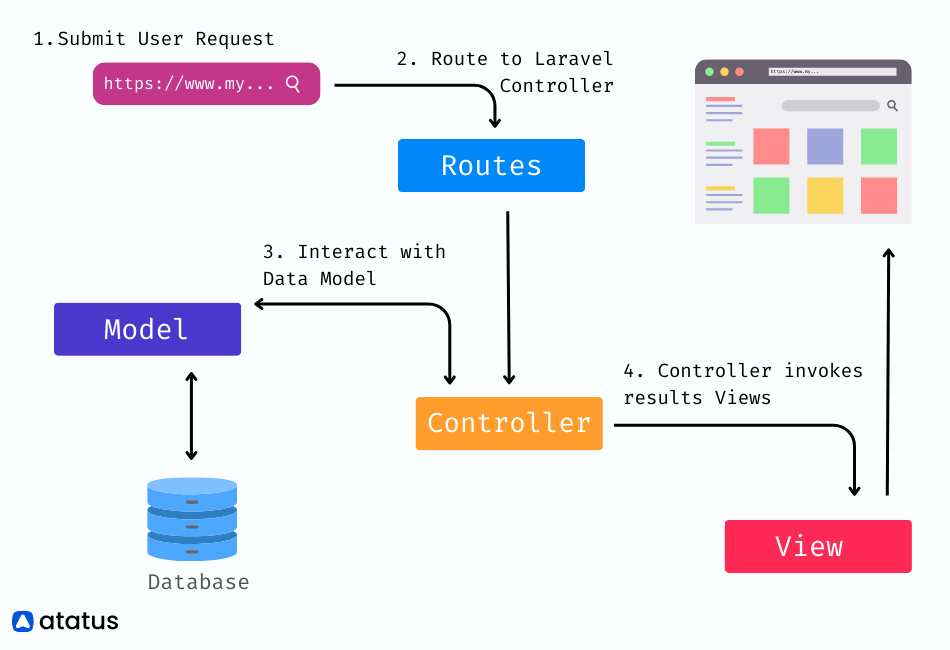
Before the view is rendered, it is compiled into PHP code by the Blade template engine. This makes it much faster to render views since the compiled code can be cached and reused. Laravel's view system also supports view composers and view models. View composers are used to share data across multiple views, while view models are used to prepare data specifically required by a single view. By using these techniques, developers can make their views more modular and easier to maintain.
View Models vs View Composers
In Laravel, the terms "view models" and "view composers" have slightly different meanings than in general software development.
In Laravel, a view model is a class that is responsible for providing data to a view. It is often used to encapsulate the logic needed to retrieve and format data from a database or other source. The view model is typically instantiated and passed to the view when it is rendered, allowing the view to access the data it needs without having to know where it came from.
A view composer, on the other hand, is a callback function or class method that is executed when a view is being rendered. It is used to inject data or objects into the view before it is returned to the browser. A view composer can be used to set up global data that should be available to all views, or to customize the behaviour of specific views.
The main difference between a view model and a view composer in Laravel is that a view model is used to encapsulate data and provide a structured way to pass it to a view, while a view composer is used to inject data or objects into a view on a per-view basis. A view composer can also be used to define global data that is available to all views.
Features | View Model | View Composer |
---|---|---|
Data organization | View models organize data into a single object. | View composers organize data in separate variables. |
Method of invocation | View models are typically invoked from a controller method and returned to a view. | View composers are registered in a service provider and automatically applied to all views. |
Scope | View models are specific to a single view. | View composers can be applied to multiple views or all views in a given application. |
Purpose | View models are used to isolate and organize data manipulation logic. | View composers are used to add common data or functionality to multiple views. |
Granularity | View models can be as granular as necessary, with each model representing a single view or component. | View composers are typically used for broad, cross-cutting concerns. |
Data binding | View models can bind data directly to a view. | View composers cannot bind directly to a view. |
Use of dependency injection | View models can leverage dependency injection to facilitate data access and manipulation. | View composers cannot leverage dependency injection. |
Performance | View models may have a performance overhead due to their creation and manipulation. | View composers are typically lightweight and do not have a significant performance impact. |
Testing | View models are easily testable using unit tests. | View composers may require more complex integration tests. |
When to Use View Models and When to Use View Composers?
View Models and View Composers serve different purposes in Laravel, and their usage depends on specific requirements and design considerations:
When to use View Models?
View Models are ideal for bundling both data and presentation logic dedicated to a specific view. They are beneficial in situations where:
- When you need to retrieve data from multiple models and combine them to create a view.
- When you need to format or transform data before displaying it in the view.
- When you want to provide a structured way to pass data to the view and avoid cluttering the view with complex code.
- When you need to provide a consistent interface for working with data across multiple views.
For example, if you have an e-commerce site, you might create a view model that retrieves information about a product, including its name, description, and price, from different database tables. The view model would then format the data into a structure that can be easily displayed in the view.
When to use View Composers?
View Composers come into play when you need to automatically bind data to multiple views. They are well-suited for the following scenarios:
- When you need to inject data or objects into a view based on a specific condition.
- When you want to define global data that should be available to all views.
- When you need to customize the behaviour of a specific view.
- When you want to avoid repeating the same code in multiple views.
For example, if you have a website with a navigation menu, you might create a view composer that injects the list of menu items into the view before it is rendered. The view composer would retrieve the list of menu items from the database and inject them into the view, ensuring that the menu is consistent across all pages.
How to Implement View Models and View Composers in Laravel?
To implement view models and view composers in Laravel, you can follow these steps:
Laravel View Models Implementation
Implementing View Models in Laravel involves creating separate classes to encapsulate the data and logic for specific views. Here's the step-by-step guide to implementing View Models in Laravel using PHP:
Step-1: Create a new directory to store your View Models, for example, ViewModels
, inside the app
directory.
Step-2: Create a new PHP class inside the ViewModels
directory. For example, if your view is named example.blade.php
, you can create a corresponding View Model like this:
// app/ViewModels/ExampleViewModel.php
namespace App\ViewModels;
class ExampleViewModel
{
public function getData()
{
// Put your data retrieval and manipulation logic here
return [
'name' => 'John Doe',
'age' => 30,
// Add more data properties as needed
];
}
}
Step-3: In your controller, instantiate the View Model and pass its data to the view:
// app/Http/Controllers/ExampleController.php
namespace App\Http\Controllers;
use App\Http\Controllers\Controller;
use App\ViewModels\ExampleViewModel;
class ExampleController extends Controller
{
public function show()
{
$viewModel = new ExampleViewModel();
$data = $viewModel->getData();
return view('example', $data);
}
}
Now, you can access the data in your example.blade.php
view file as regular variables:
<!-- resources/views/example.blade.php -->
<h1>Hello, {{ $name }}</h1>
<p>Your age is {{ $age }}</p>
Laravel View Composer Implementation
Implementing a View Composer in Laravel involves using a service provider to bind data to specific views automatically. Here's a step-by-step guide to implementing a View Composer in Laravel using PHP:
Step-1: To create a new Service Provider in Laravel, you can use the following Artisan command:
php artisan make:provider ViewComposerServiceProvider
Step-2: After generating the ViewComposerServiceProvider.php
file, you can locate it in the app/Providers
directory. In this file, you will find a boot()
method. Within the boot()
method, you can define your View Composers and indicate the views they should be associated with.
// app/Providers/ViewComposerServiceProvider.php
namespace App\Providers;
use Illuminate\Support\ServiceProvider;
use View;
use App\ViewModels\ExampleViewModel;
class ViewComposerServiceProvider extends ServiceProvider
{
public function boot()
{
// Bind the ExampleViewModel to the example.blade.php view
View::composer('example', function ($view) {
$viewModel = new ExampleViewModel();
$data = $viewModel->getData();
$view->with($data);
});
}
public function register()
{
//
}
}
You can register your View Composers using Laravel's View::composer()
method in the boot()
method of the ViewComposerServiceProvider
. Specify the view name to which the data should be bound, and define the logic for retrieving the data to be passed to that view within the closure function of View::composer()
.
With this approach, Laravel automatically associates the specified data with the designated views each time they are rendered. This practice helps you maintain well-organized view-related logic and facilitates data sharing across multiple views using View Composers.
Step-3: Register your new Service Provider in the config/app.php
file by adding it to the providers
array:
// config/app.php
return [
// ...
'providers' => [
// ...
App\Providers\ViewComposerServiceProvider::class,
],
// ...
];
Step-4: Now, the data from the View Model (ExampleViewModel
) will be available in the example.blade.php
view automatically, and you don't need to manually pass it from the controller:
<!-- resources/views/example.blade.php -->
<h1>Hello, {{ $name }}</h1>
<p>Your age is {{ $age }}</p>
Whenever the example.blade.php
view is rendered, it will automatically have access to the data provided by the ExampleViewModel
. This helps to keep your view-specific logic separate and makes it easier to share common data across views.
Performance Considerations for Using View Models and View Composers
When you utilize View Models and View Composers in your Laravel PHP application, it's important to take into account certain performance considerations to ensure that your application functions efficiently and seamlessly:
- Optimize Queries: Ensure that data retrieval operations in View Models are optimized by using eager loading and selecting only necessary columns. Avoid N+1 query issues that could lead to excessive database queries.
- Caching: Consider caching data fetched by View Models if it doesn't change frequently. Implement caching mechanisms like Redis or Memcached to improve performance and reduce database hits.
- Lazy Loading: Be cautious with View Composers to avoid loading unnecessary data for views that don't require it. Load data only when needed to minimize overhead.
- Database Indexing: Optimize database performance by indexing relevant columns used in data retrieval.
- Code Optimization: Keep your View Models and View Composers optimized by removing redundant or inefficient code. Aim for efficient data retrieval and processing.
- Service Providers: Carefully manage the number of registered service providers as each one gets booted on each request. Use only necessary providers to avoid unnecessary performance impact.
- View Caching: Leverage Laravel's view caching feature to store compiled views, reducing the overhead of compiling Blade templates on each request.
- Minimize Eloquent Queries: When using Eloquent models, be mindful of the number of queries executed. Utilize eager loading and reduce query counts for related data.
- Testing: Consider the impact of View Models and View Composers on test performance. Use mock objects to prevent unnecessary database hits during testing.
- Database Transactions: Exercise caution with transactions involving View Models and View Composers to avoid database deadlocks or performance issues.
Conclusion
View models and view composers are two powerful features that Laravel developers can use to prepare and organize data for views. While they share some similarities, such as their ability to abstract data manipulation logic away from views, they each have their own distinct strengths and use cases.
By leveraging view models and view composers effectively, developers can improve the performance and maintainability of their Laravel applications, while also creating cleaner and more modular code.
Whether you are working on a small personal project or a large-scale enterprise application, understanding the differences between view models and view composers is an important part of building high-quality Laravel applications.
So take time to explore these concepts in depth and see how they can help you write better code and deliver better results. With the right approach and a solid understanding of these concepts, you can take your Laravel development skills to the next level and create amazing web applications that truly stand out.
Atatus: Laravel Performance Monitoring
Atatus is an Application Performance Management (APM) solution that collects all requests to your PHP applications without requiring you to change your source code. However, the tool does more than just keep track of your application's performance.
Atatus Laravel monitoring, tracks and provides real-time notifications for every error, exception, and performance problem that occurs within the application.
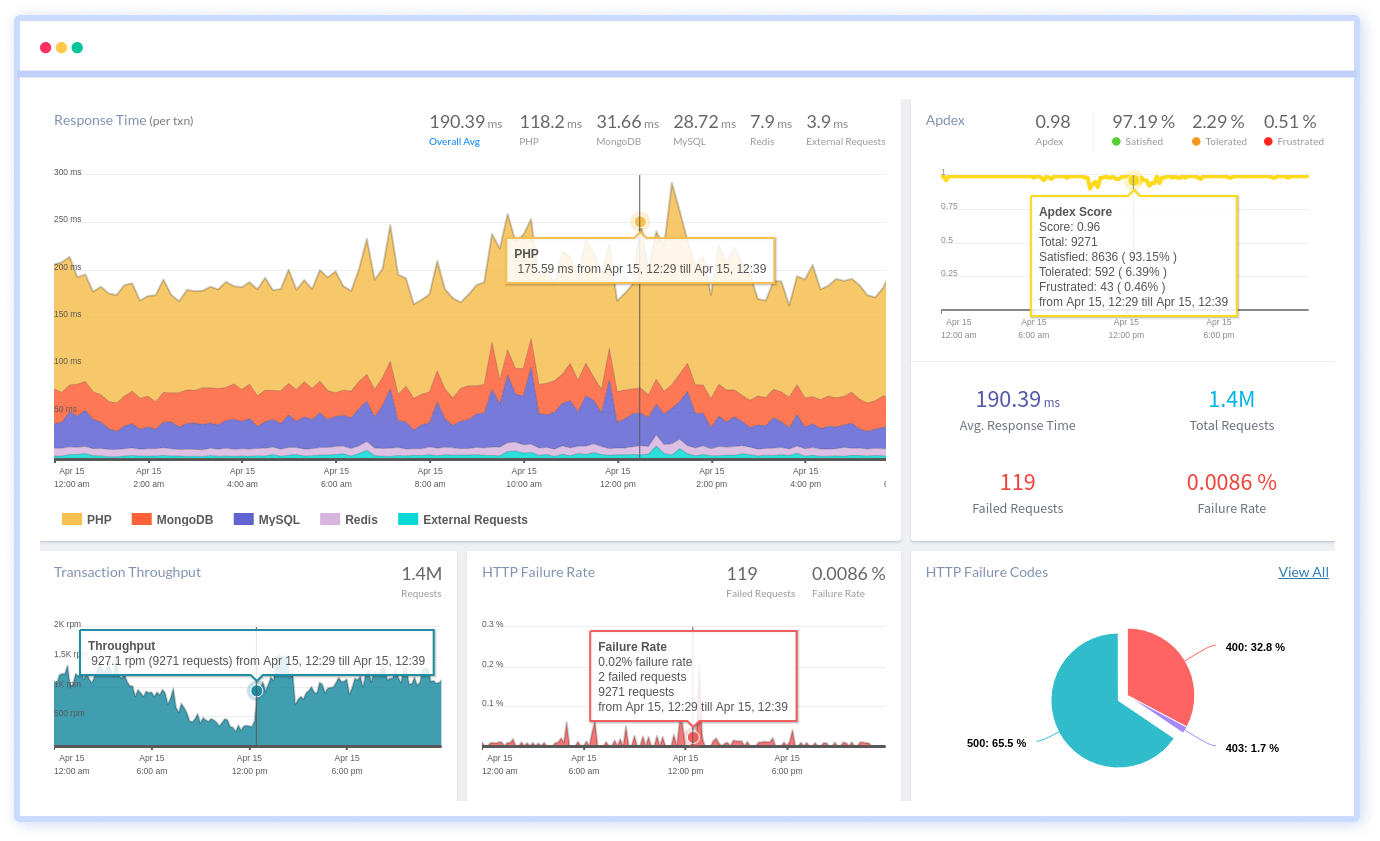
By tracking KPIs such as response times, throughput, HTTP failure rates, and error rates, it can help users identify and address any performance issues before they impact the application's users.
Furthermore, Atatus supports multiple PHP framework such as WordPress, Magento, CodeIgniter, CakePHP, Symfony, Yii and Slim making it an adaptable tool that can be used to monitor applications across different frameworks and platforms.
#1 Solution for Logs, Traces & Metrics
APM
Kubernetes
Logs
Synthetics
RUM
Serverless
Security
More