A Comprehensive Guide to Tuples in Python
When working with data collection, we occasionally encounter situations where we want to ensure it is impossible to change the sequence of objects after creation. Many data processing algorithms and functions expect data to be in a specific order since the order of the data affects the results of the processing.
Python has a built-in sequence data type to store data in an unchangeable object, called a tuple. Tuple will let you store an ordered sequence of items.
To understand tuple better let us see a very simple example, If suppose you are building an application for a restaurant, where you want to store information about the dish, such as its name, and price etc.,
Since tuple is immutable, you can be sure that the information given will not be accidentally changed or corrupted.
In this blog we will delve into the basics of tuples, how to work with tuples in python, its uses, and much more.
Are you ready to learn more, Let's dive in!
Table of Contents
- What is a Python Tuple?
- Python Tuple Methods
- Python Tuple Types
- Tuple Slicing
- Changing Tuple Values
- Deleting a Python Tuple
- Performing Operations in Python Tuple
- Difference Between List and Tuple
What is a Python Tuple?
Python tuple is a data structure that stores an ordered sequence of elements of any type. When we say that tuples are ordered, it means that the items have a defined order. A tuple is an immutable object, which means it cannot be changed, and we use it to represent fixed collections of items.
Tuples are commonly used to group related data together and can contain elements of different data types (integer, float, list, string, etc.).
They are defined using parentheses () and comma-separated values.
Let's see some examples,
- () — an empty tuple
- (1,2,3) — a tuple containing three integers
- ("Blue", "Yellow", "Pink") — a tuple containing three string objects
- ("1", 100, False) — a tuple containing a string, an integer, and a Boolean object
Python Tuple Methods
Python Tuples have several built-in methods to manipulate them. Here are some of the most commonly used tuple methods in Python:
1. Tuple length
Python tuple method len()
returns the number of elements in the tuple.
Syntax
len(tuple)
Example:
tuple1, tuple2 = ("Java", "Python", "C"), ("Windows", "linux")
print(len(tuple1))
Output:
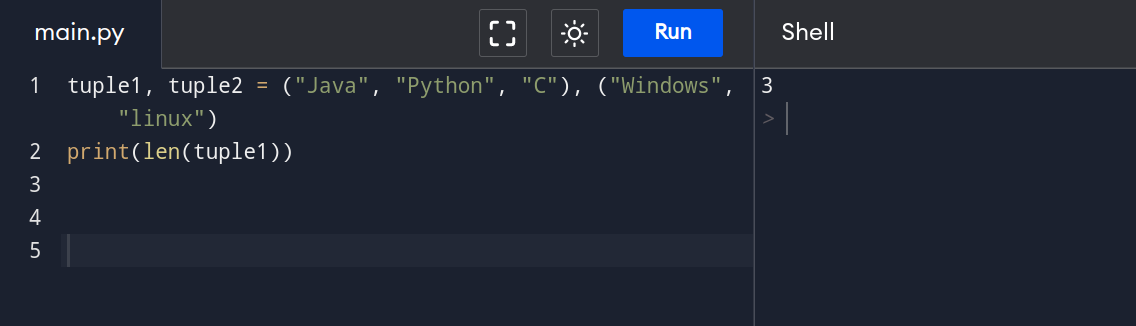
2. Tuple count
The count()
method returns the number of times a specified value appears in the tuple.
Syntax
tuple.count(value)
Example:
tuple = (1, 3, 7, 8, 7, 5, 4, 5, 6, 8, 5)
count = tuple.count(5)
print(count)
Output:
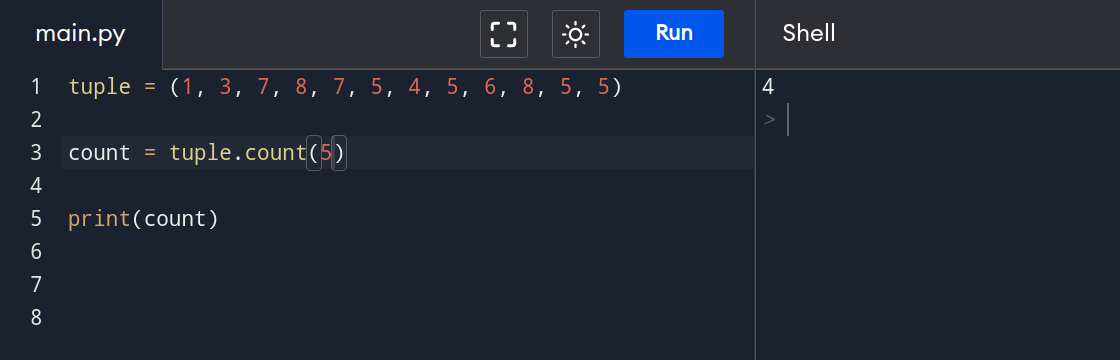
3. Tuple Max
The max()
method in Python returns the largest element in an iterable. For tuples, it returns the largest element based on the elements' natural order.
Example 1:
numbers = (1, 2, 3, 4, 5)
max = max(numbers)
print(max)
Output:
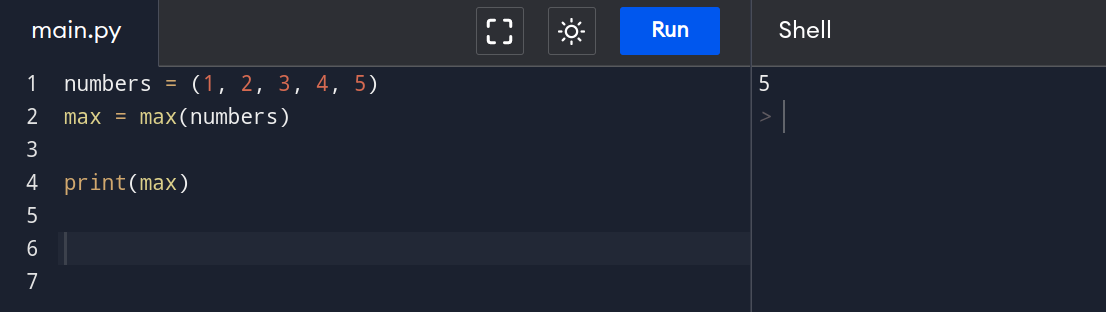
If the tuple contains elements of different data types, such as integers and strings, the max()
method will raise a TypeError
. To handle this situation, you can pass the tuple to the sorted()
function, which returns a sorted list, and then apply the max()
method to the sorted list.
Example 2:
Here's an example of using the max()
method on a tuple of integers and strings:
mixed = (1, "hello", 3, "world")
sorted_mixed = sorted(mixed, key=lambda x: str(x))
print(sorted_mixed)
max = max(sorted_mixed, key=lambda x: str(x))
print(max)
Output:
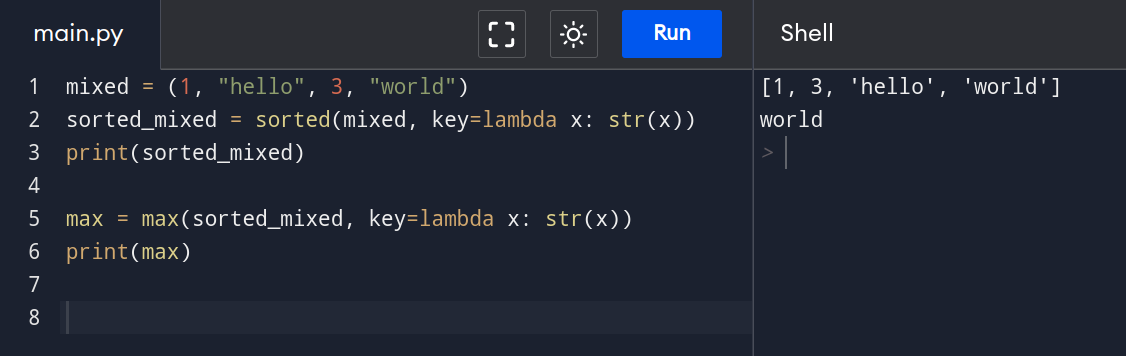
4. Tuple Min
The min()
method in Python returns the smallest element in an iterable.
Here's an example of using the min()
method on a tuple of integers:
Example 1:
numbers = (5, 3, 1, 4, 2)
min = min(numbers)
print(min)
Output:
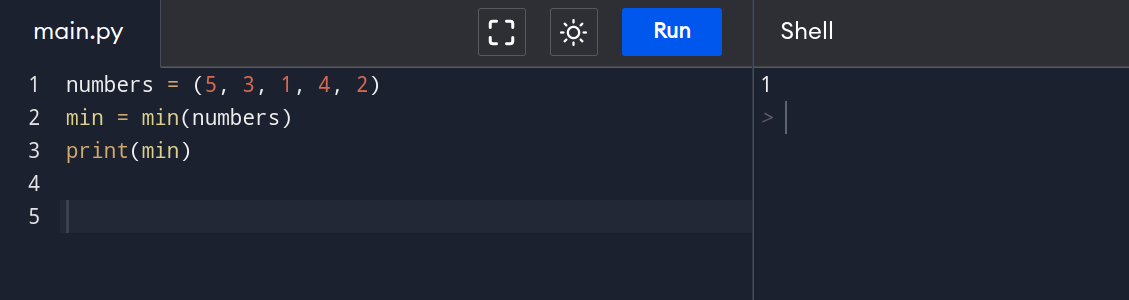
If the tuple contains elements of different data types, such as integers and strings, the min()
method will raise a TypeError
. To handle this situation, you can pass the tuple to the sorted()
function, which returns a sorted list, and then apply the min()
method to the sorted list.
Here's an example of using the min()
method on a tuple of integers and strings:
Example 2:
mixed = (1, "hello", 3, "world")
sorted_mixed = sorted(mixed, key=lambda x: str(x))
print(sorted_mixed)
min = min(sorted_mixed, key=lambda x: str(x))
print(min)
Output:
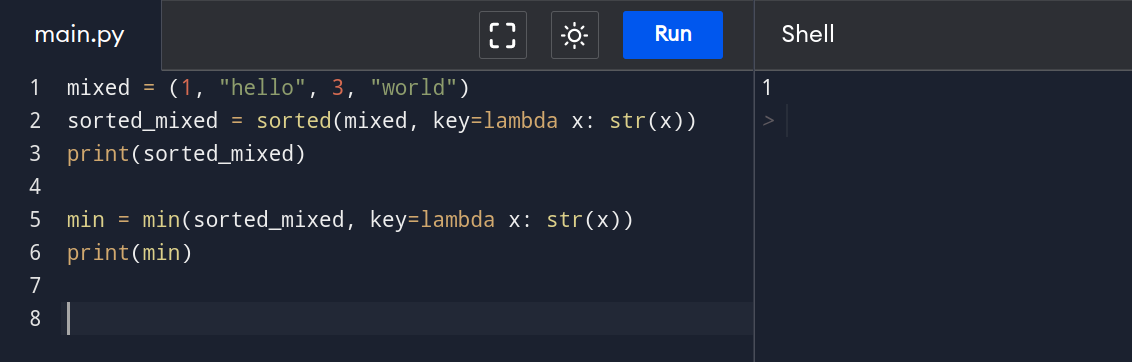
5. Tuple sorted()
The sorted()
function in Python returns a sorted list of elements from a given iterable. This function can also be used on tuples to sort the elements in ascending order based on the elements' natural order. The natural order is determined by the comparison operators <
, >
, <=
, >=
, ==
, and !=
.
Here's an example of using the sorted()
function on a tuple of integers:
Example 1:
numbers = (5, 3, 1, 4, 2)
sorted_num = sorted(numbers)
print(sorted_num)
Output:
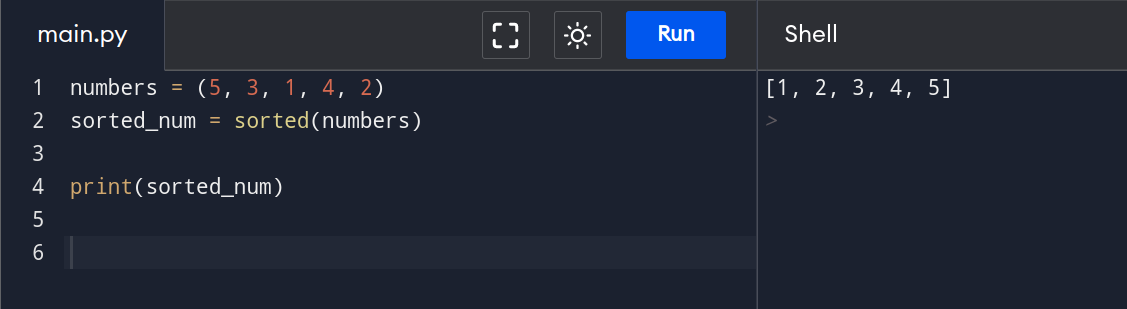
If the tuple contains elements of different data types, such as integers and strings, the sorted()
function will raise a TypeError
. To handle this situation, you can pass the key
argument to the sorted()
function, which specifies a function to extract a comparison key from each element in the iterable.
Here's an example of using the sorted()
function on a tuple of integers and strings:
Example 2:
mixed = (1, "hello", 3, "world")
sorted = sorted(mixed, key=lambda x: str(x))
print(sorted)
Output:
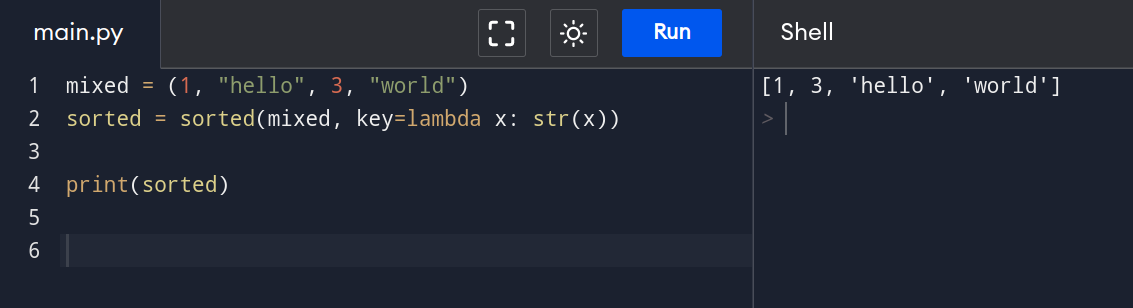
Note that the sorted()
function returns a sorted list, and the original tuple remains unchanged. If you need to sort the elements in a tuple in-place, you can convert the tuple to a list, sort the list, and then convert the list back to a tuple.
6. Tuple Index
In Python, you can access elements of a tuple by using square brackets and providing the index of the element you want to access. Indexing starts from 0, so the first element of a tuple has an index of 0, the second has an index of 1, and so on.
Example 1:
Since indexing starts from 0, in this example, the first element, Application performance monitoring has an index of 0 and the second element, logs monitoring has an index of 1, and so on.
You can use the following code for accessing the tuple elements,
monitoring_tools = (
"Application performance monitoring",
"Logs monitoring",
"Real user monitoring",
"Server monitoring",
"Infrastructure monitoring",
)
print(monitoring_tools[1])
print(monitoring_tools[2])
Output:
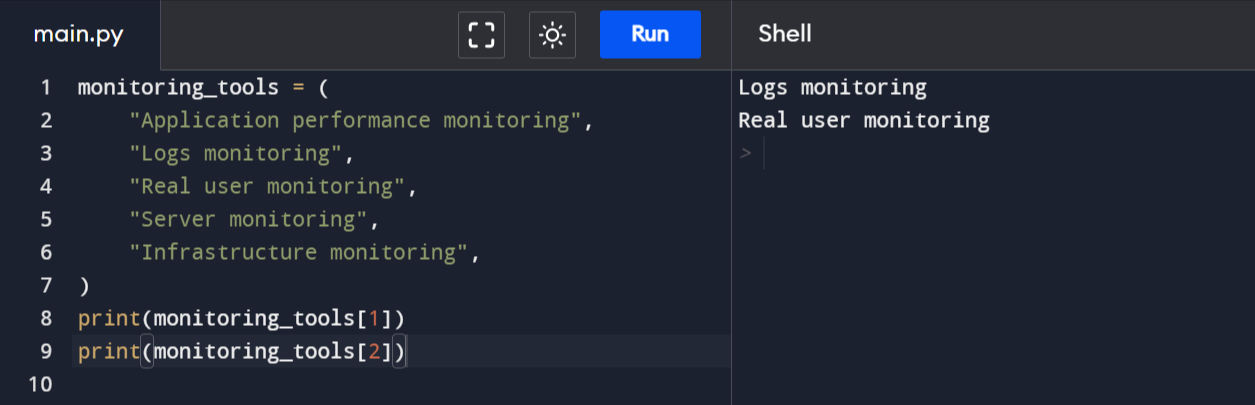
Similarly, you can modify and use the same code for accessing all the tuple elements.
Example: 2
The code used in example 1 can be modified as mentioned below to get the same output
monitoring_tools = (
"Application performance monitoring",
"Logs monitoring",
"Real user monitoring",
"Server monitoring",
"Infrastructure monitoring",
)
print(monitoring_tools[0 + 1])
print(monitoring_tools[1 + 1])
Output:
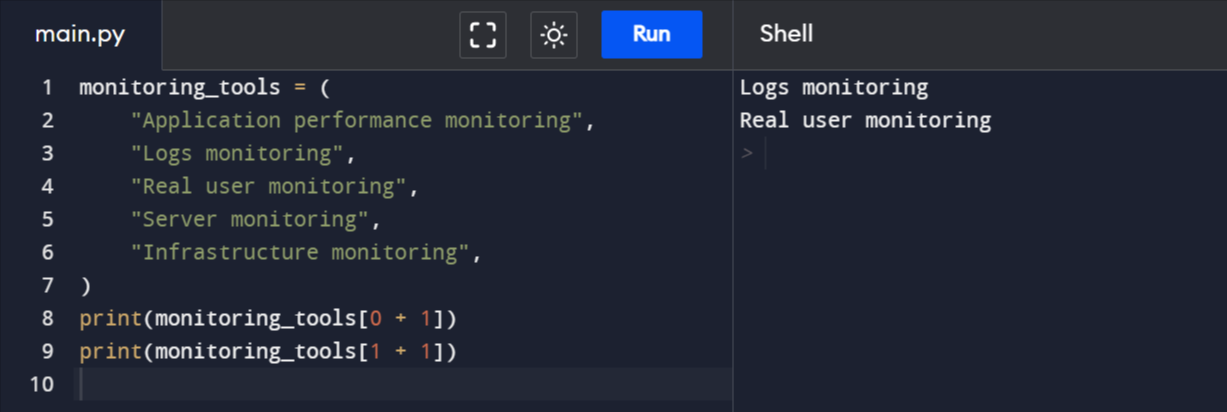
The code returns the same output as the previous example.
Example 3:
You can also use negative indices to access elements from the end of the tuple. For example, monitoring_tools[-1] returns the last element of the tuple and monitoring_tools[-2] returns the second to last element.
monitoring_tools = (
"Application performance monitoring",
"Logs monitoring",
"Real user monitoring",
"Server monitoring",
"Infrastructure monitoring",
)
print(monitoring_tools[-1])
print(monitoring_tools[-2])
Output:
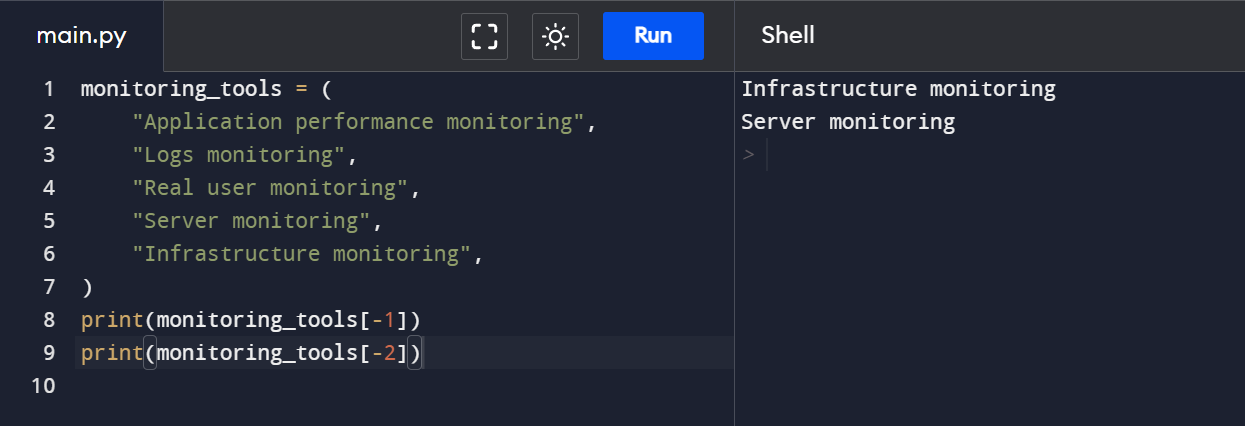
Example 4:
Tuple index out of range.
monitoring_tools = (
"Application performance monitoring",
"Logs monitoring",
"Real user monitoring",
"Server monitoring",
"Infrastructure monitoring",
)
print(monitoring_tools[10])
Output:
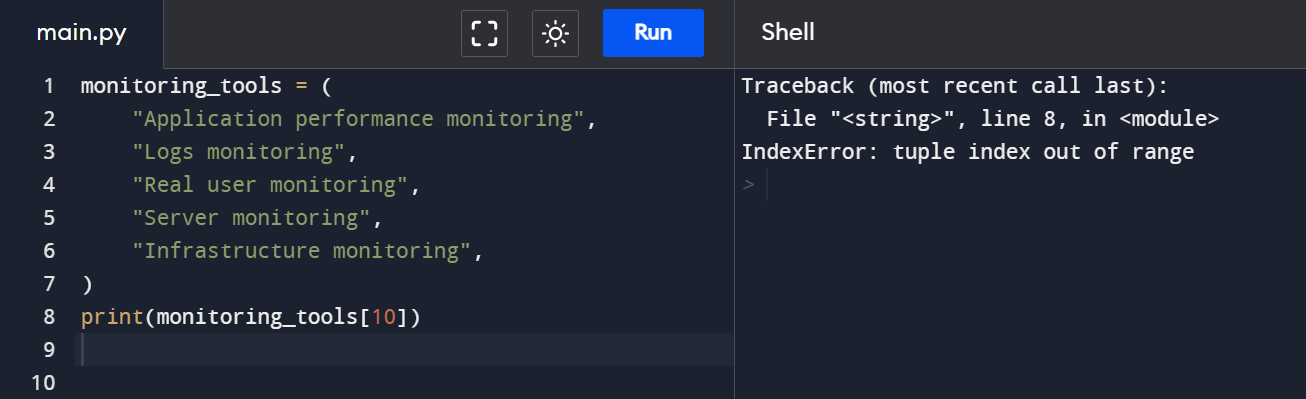
The code gives an error as the index is only up to 4.
Python Tuple Types
There are two main types, which includes named tuple and an unnamed tuple.
1. Named Tuple
It is used to assign name to elements in a tuple and these elements can be accessed using a dot notation. Named tuples are located in the standard library module called collections.
Example:
from collections import namedtuple
Person = namedtuple("Person", "name age country")
# creating named tuple
p1 = Person("Olivia", 25, "India")
# accessing elements using property name
print("Name:", p1.name)
print("Age:", p1.age)
print("Country:", p1.country)
Output:
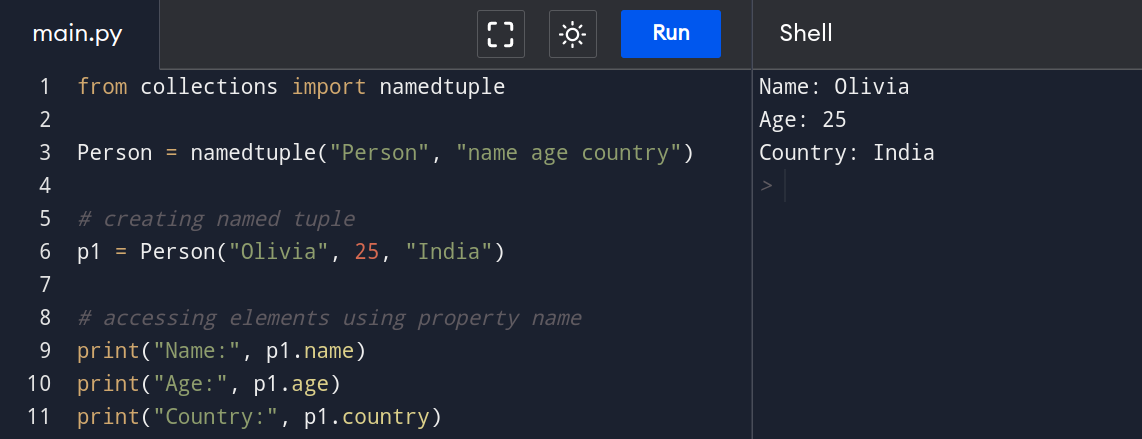
2. Unnamed Tuple
Unlike named tuples, unnamed tuples do not have named fields and can only be accessed using indexing.
Example:
person = ("Olivia", 25)
print("Name:", person[0])
print("Age:", person[1])
Output:
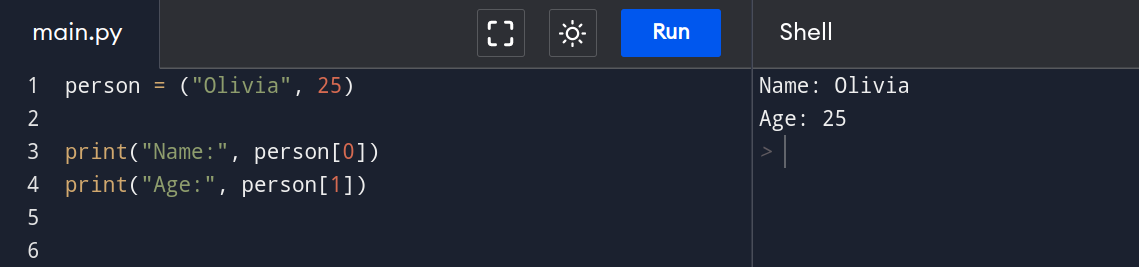
Tuple Slicing
Tuple slicing is the process of extracting a part of a tuple by specifying a range of indices. The syntax for tuple slicing is tuple[start:stop:step], where start is the starting index (included), stop is the stopping index (excluded), and step is the step size (default value is 1).
Let us see some examples to understand slicing better,
Example 1:
monitoring_tools = (
"Application performance monitoring",
"Logs monitoring",
"Real user monitoring",
"Server monitoring",
"Infrastructure monitoring",
)
print(monitoring_tools[1:4])
Output:
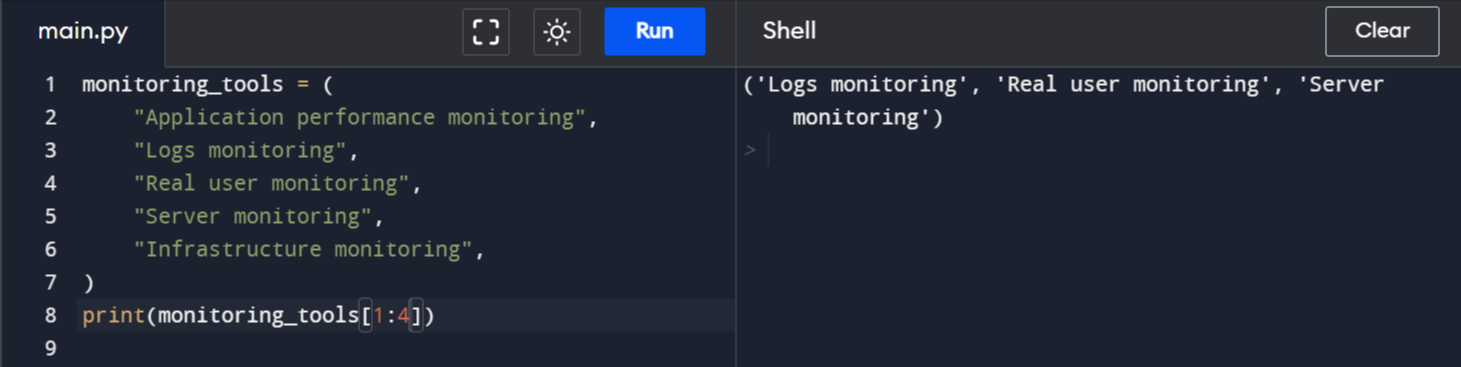
Example 2:
Here, the default starting value for the range, in the absence of an specified starting point, is the first term.
monitoring_tools = (
"Application performance monitoring",
"Logs monitoring",
"Real user monitoring",
"Server monitoring",
"Infrastructure monitoring",
)
print(monitoring_tools[:2])
Output:
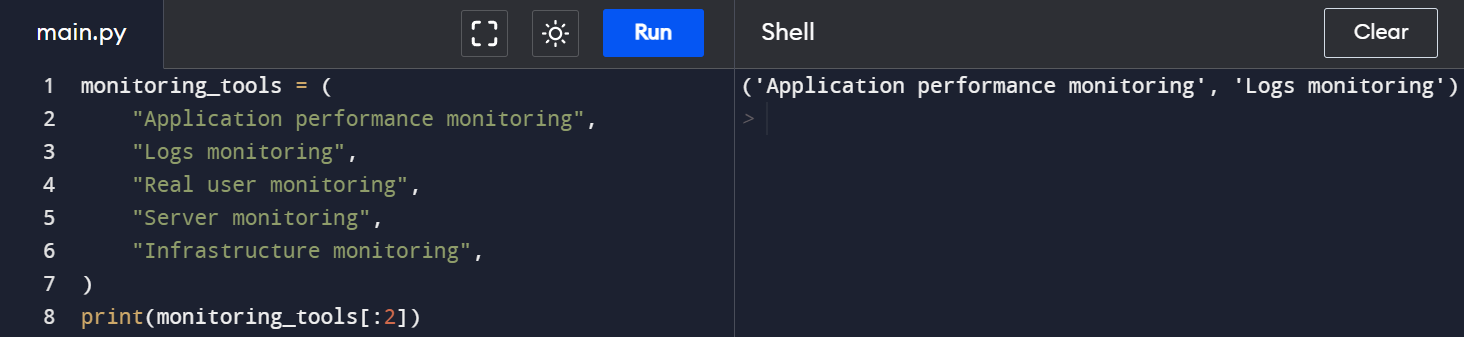
Example 3:
By default the range ends at the last term, if we do not specify the end value.
monitoring_tools = (
"Application performance monitoring",
"Logs monitoring",
"Real user monitoring",
"Server monitoring",
"Infrastructure monitoring",
)
print(monitoring_tools[1:])
Output:
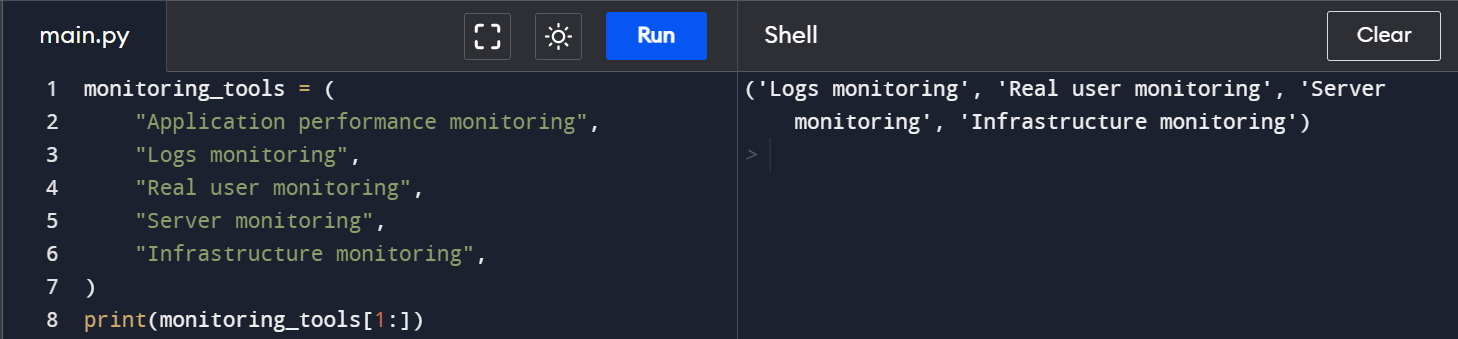
Example 4:
By default, if both the start and stop values of the range are not specified, it will start from the first term and end at the last term.
monitoring_tools = (
"Application performance monitoring",
"Logs monitoring",
"Real user monitoring",
"Server monitoring",
"Infrastructure monitoring",
)
print(monitoring_tools[:])
Output:
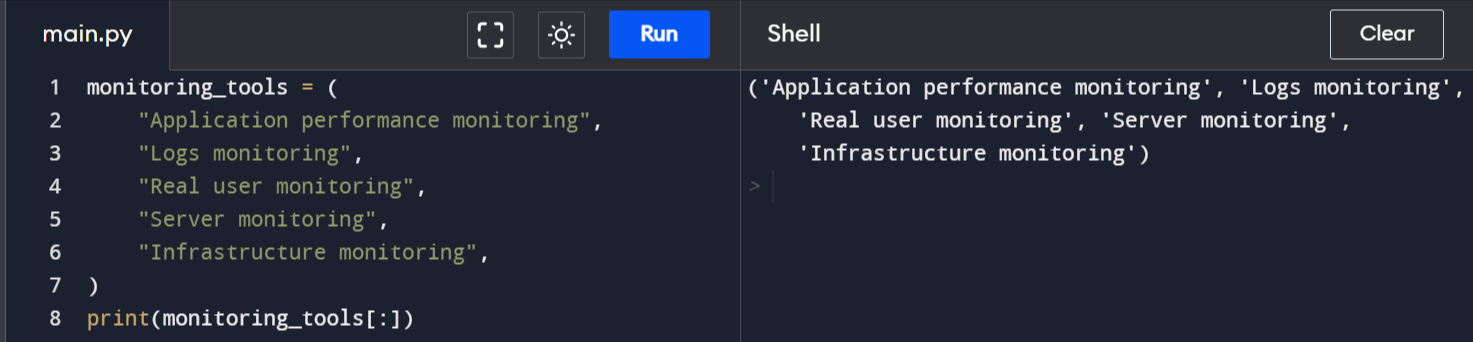
Example 5:
In the below code, the first element is printed to the last element with a step of 2, i.e. alternate elements are printed.
monitoring_tools = (
"Application performance monitoring",
"Logs monitoring",
"Real user monitoring",
"Server monitoring",
"Infrastructure monitoring",
)
print(monitoring_tools[::2])
Output:
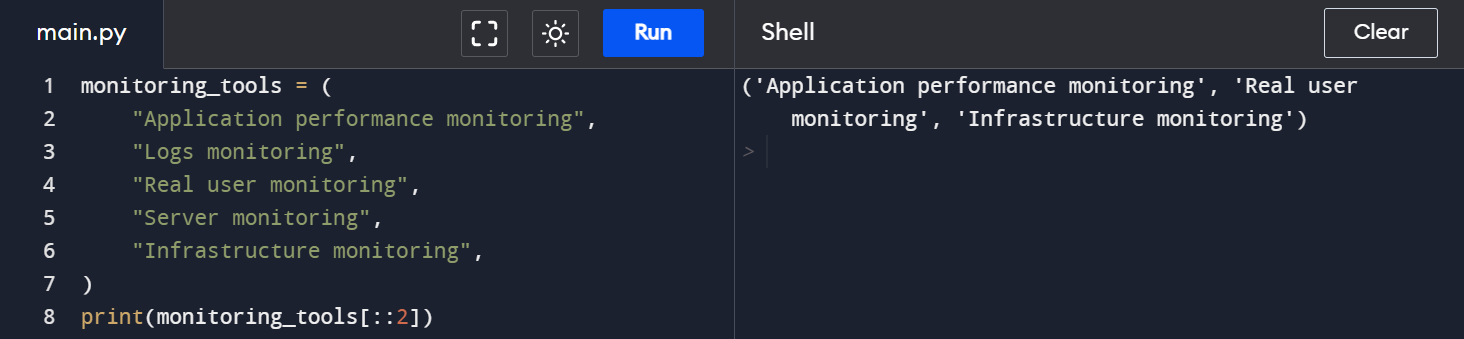
Example 6:
Additionally, tuple slicing offers a stride feature. It determines the interval between elements in the sliced tuple. The stride value can be positive, negative, or zero, and it determines how many indices to skip when selecting elements from the original tuple.
monitoring_tools = (
"Application performance monitoring",
"Logs monitoring",
"Real user monitoring",
"Server monitoring",
"Infrastructure monitoring",
)
print(monitoring_tools[0:5:2])
Output:
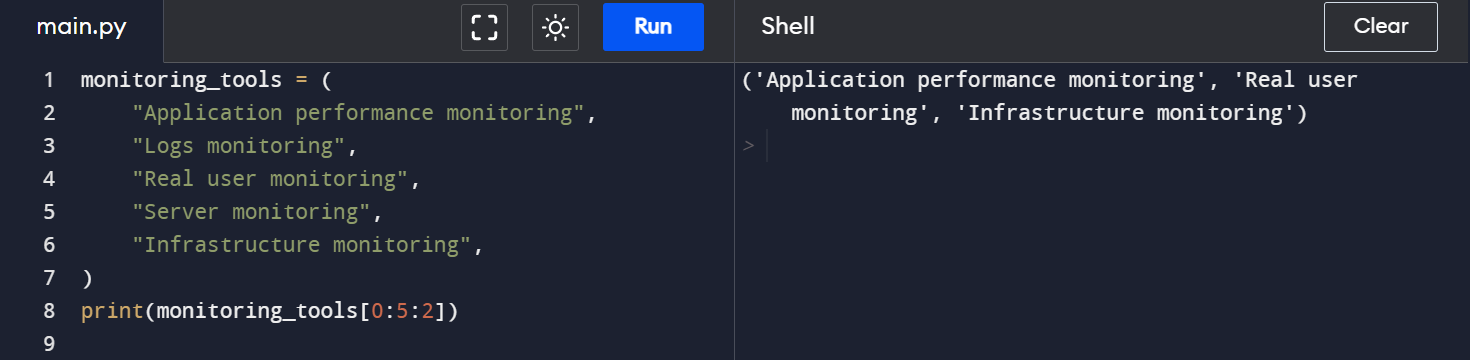
Example 7:
In addition, you can use negative index numbers when you’re slicing tuples. The below example returns the last two items in our list:
monitoring_tools = (
"Application performance monitoring",
"Logs monitoring",
"Real user monitoring",
"Server monitoring",
"Infrastructure monitoring",
)
print(monitoring_tools[-2:])
Output:
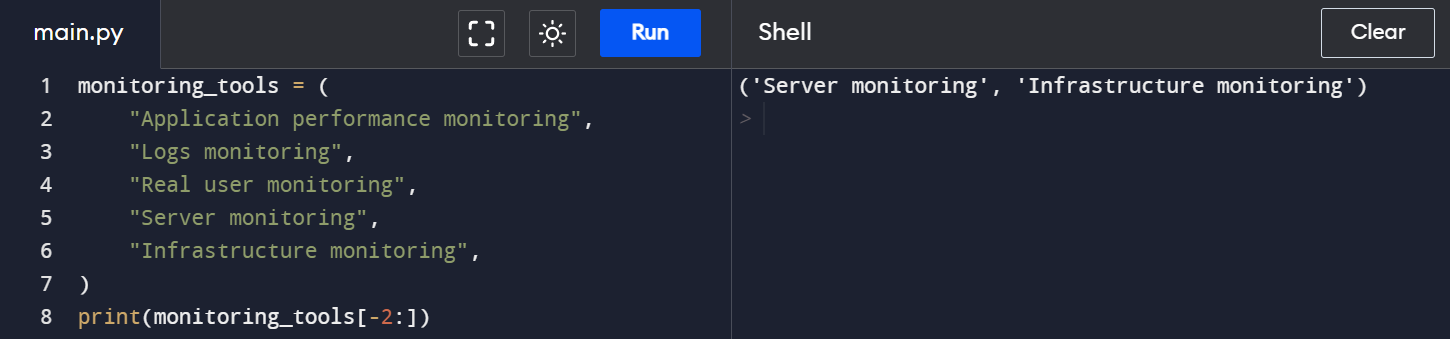
Changing Tuple Values
As mentioned earlier, you cannot modify the tuple values. But there is a workaround, You can convert the tuple to a list, modify the values in the list, and convert the list back to a tuple.
Similar to a tuple, lists are one of the built-in data types in Python which is used to store multiple items in a single variable.
Example:
programming_languages_tuple = ("Java", "Python", "C")
programming_languages_list = list(programming_languages_tuple)
programming_languages_list[1] = "Go"
programming_languages_tuple = tuple(programming_languages_list)
print(programming_languages_tuple)
Output:
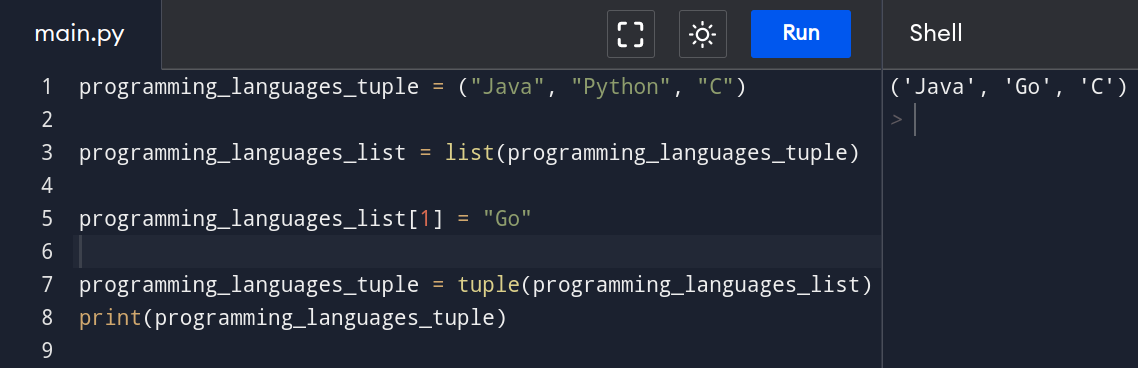
Deleting a Python Tuple
Deleting a particular element in a tuple is not possible. But, the whole tuple can be deleted using the del keyword as shown in the following example:
Example:
tup1 = ("Java", "Python", "C")
print (tup1)
del tup1
print (tup1)
Output:

Performing Operations in Python Tuple
In Python, there are several operators that can be used with tuples. Let us take a closer look at each aspect of the topic.
Addition operator
The +
operator is used to concatenate two tuples.
Example 1:
monitoring_tools_1 = (
"Application performance monitoring",
"Logs monitoring",
)
monitoring_tools_2 = (
"Real user monitoring",
"Server monitoring",
"Infrastructure monitoring",
)
monitoring_tools = monitoring_tools_1 + monitoring_tools_2
print(monitoring_tools)
Output:
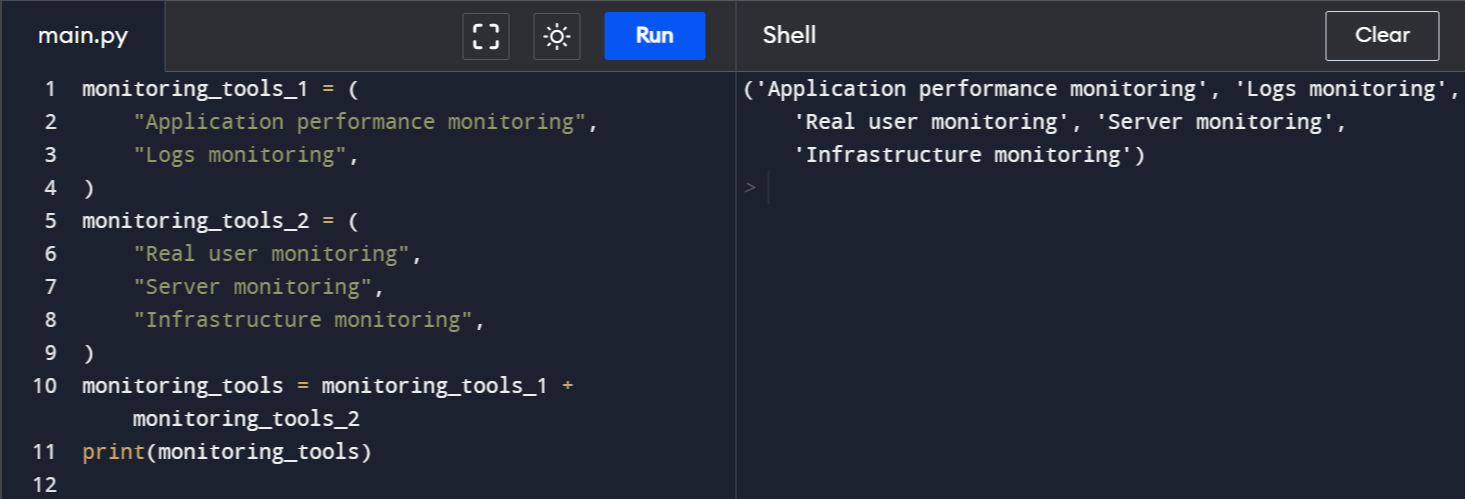
Repetition operator
The *
operator is used to repeat a tuple.
Example 2:
monitoring_tools_1 = (
"Application performance monitoring",
"Logs monitoring",
)
monitoring_tools = monitoring_tools_1 *3
print(monitoring_tools)
Output:
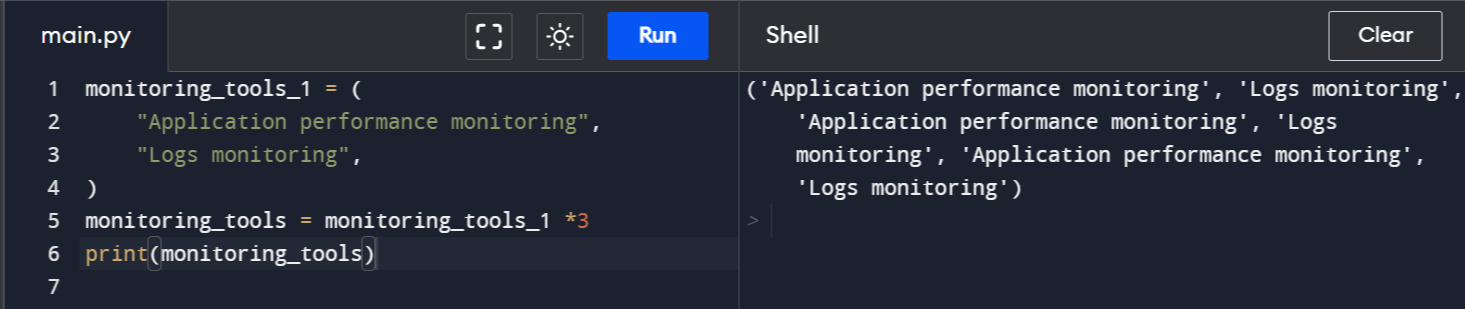
in operator
The in
operator is used to check if an element is present in a tuple. It returns True
if an element is present in the tuple, and False
otherwise.
Example 3:
monitoring_tools = (
"Application monitoring tool",
"Logs monitoring",
"server monitoring",
)
print("Logs monitoring" in monitoring_tools)
print("Real user monitoring" in monitoring_tools)
Output:
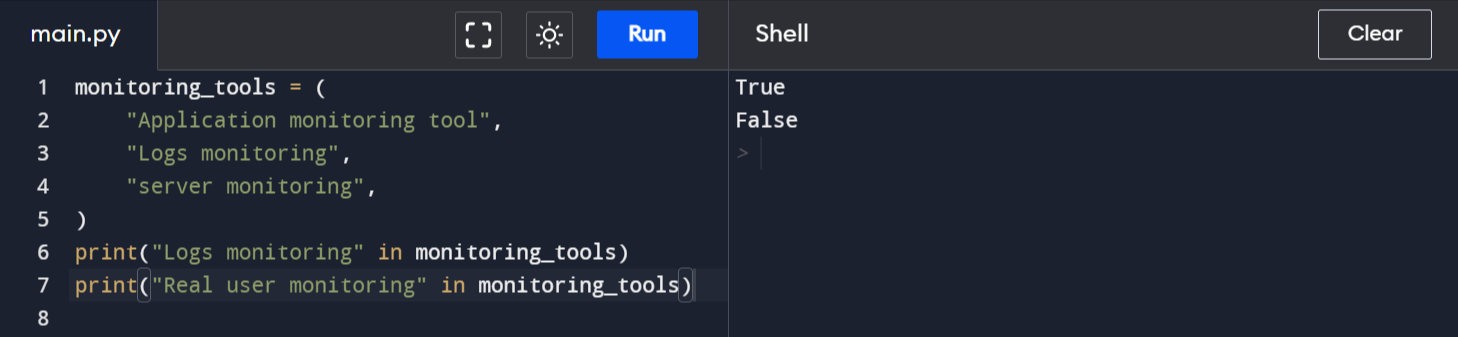
not in operator
The not in
operator is used to check if an element is not present in a tuple. It returns True
if an element is not present in the tuple, and False
otherwise.
Example 4:
monitoring_tools = (
"Application monitoring tool",
"Logs monitoring",
"server monitoring",
)
print("Logs monitoring" not in monitoring_tools)
print("Real user monitoring" not in monitoring_tools)
Output:

Less than operator
The <
operator is used to compare two tuples element by element, returns True
if the tuple is smaller than the second tuple.
Example 5:
monitoring_tools_1 = (
"Application performance monitoring",
"Logs monitoring",
)
monitoring_tools_2 = (
"Real user monitoring",
"Server monitoring",
"Infrastructure monitoring",
)
monitoring_tools = monitoring_tools_1 < monitoring_tools_2
print(monitoring_tools)
Output:
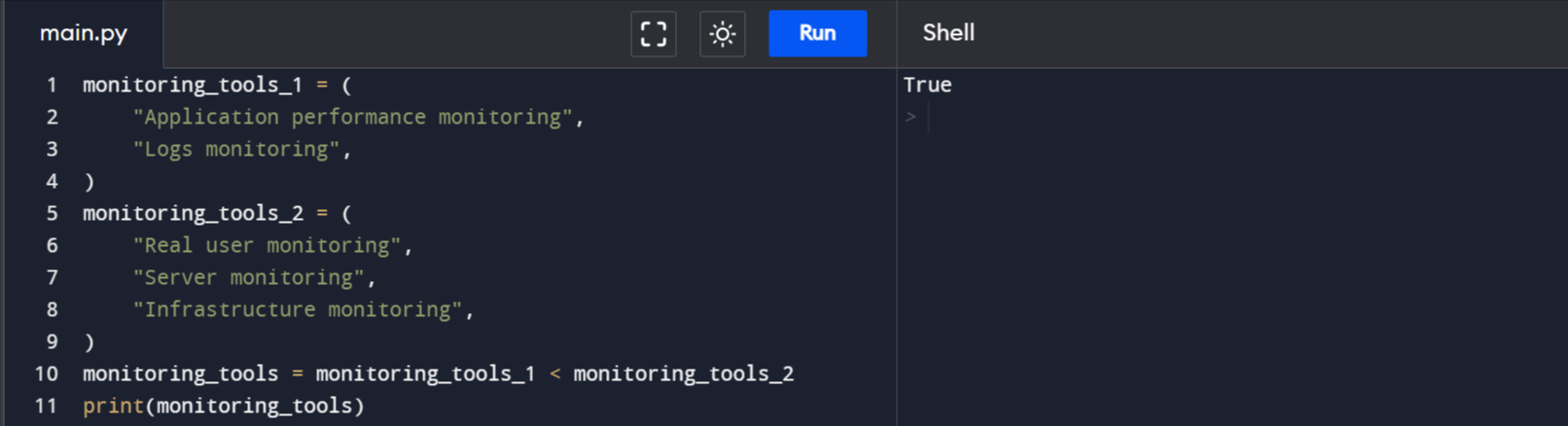
Greater than operator
The >
operator is used to compare two tuples element by element, returns True
if the tuple is larger than the second tuple.
Example 6:
monitoring_tools_1 = (
"Application performance monitoring",
"Logs monitoring",
)
monitoring_tools_2 = (
"Real user monitoring",
"Server monitoring",
"Infrastructure monitoring",
)
monitoring_tools = monitoring_tools_1 > monitoring_tools_2
print(monitoring_tools)
Output:
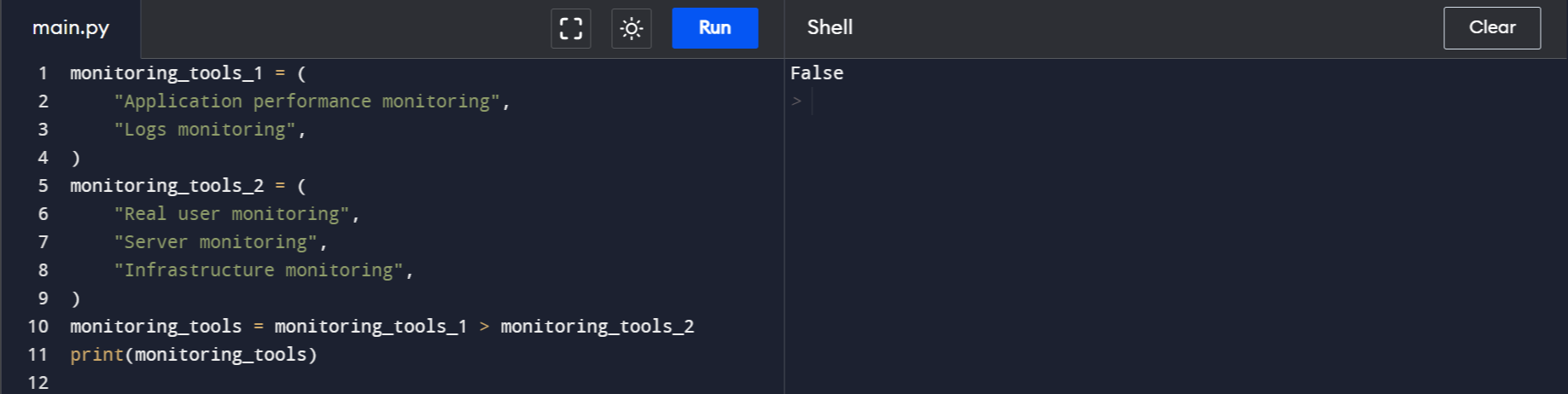
Difference Between List and Tuple
Python Lists | Python Tuples |
List are mutable | Tuples are immutable |
Iterations are time-consuming | Iterations are comparatively faster |
It consumes more memory | It consumes less memory than the list |
The List can delete any particular element | Tuples cannot delete elements rather it can delete a whole tuple |
Good for insertion-deletion | Good for accessing elements |
A unexpected change or error is more likely to occur in a list. | In a tuple, changes and errors don't usually occur because of immutability. |
Outcome
Python tuples are an essential component of implementation of several key ideas, including functional programming, data structures, and algorithms.
Did you know that tuples differ from other data structures in the language by virtue of a special property?
Tuples are hashable in Python, allowing for their use as keys in dictionaries. A hash value is a fixed-size integer that is used to identify an object, typically through a hash function.
Consider an object is having a hash value, it means that the value of the object does not change during its lifetime. This feature makes tuples an excellent option for utilisation.
Whether you're working on a small project or a large-scale software system, tuples can help you organize data in a way that makes it easy to access.
So, next time when you are working with related data, don't forget the power of tuples and their multifunctionality in Python programming!
Atatus: Python Performance Monitoring
Atatus is an Application Performance Management (APM) solution that collects all requests to your Python applications without requiring you to change your source code. However, the tool does more than just keep track of your application's performance.
Monitor logs from all of your Python applications and systems into a centralized and easy-to-navigate user interface, allowing you to troubleshoot faster using Python monitoring.
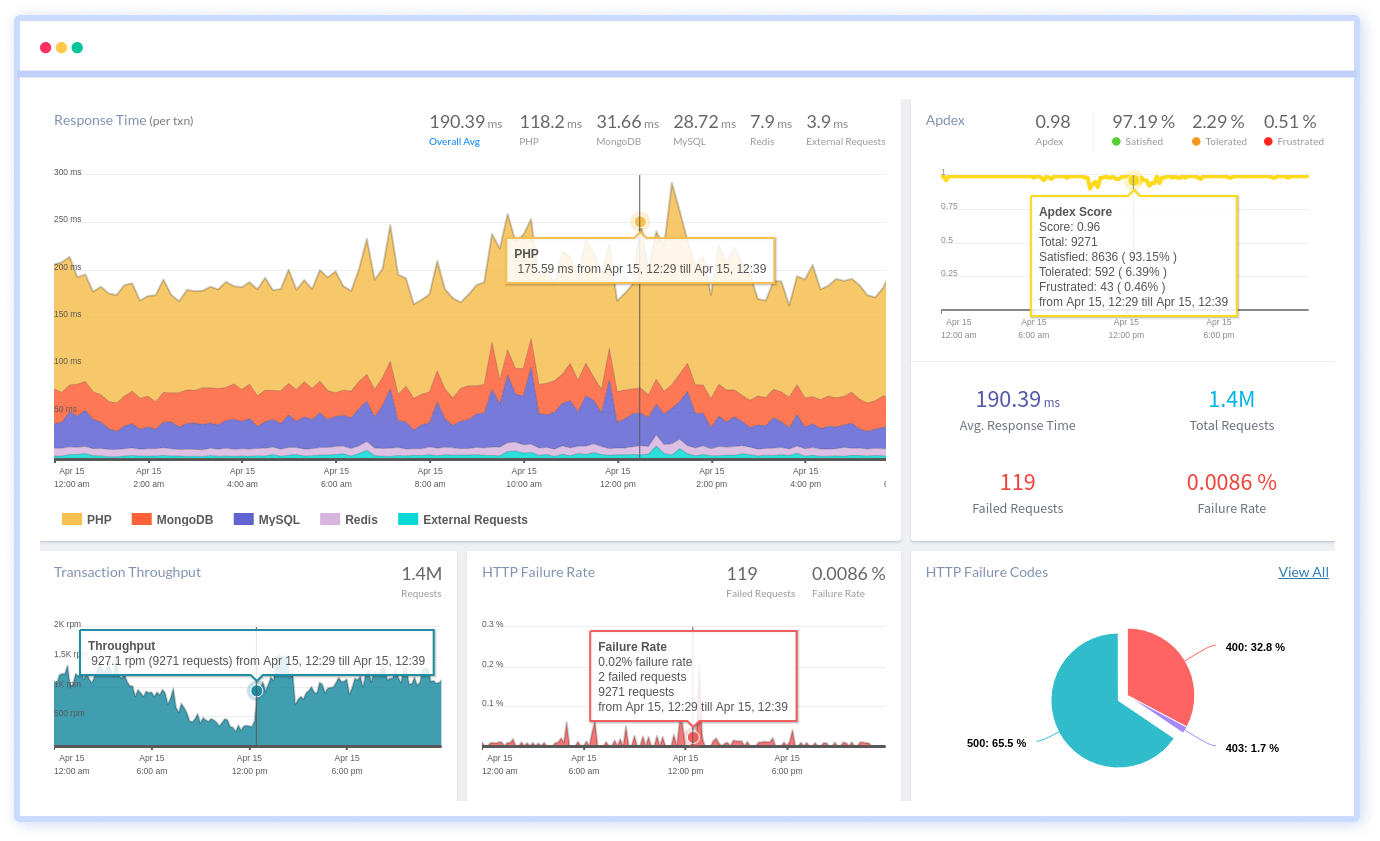
We give a cost-effective, scalable method to centralized Python logging, so you can obtain total insight across your complex architecture. To cut through the noise and focus on the key events that matter, you can search the logs by hostname, service, source, messages, and more. When you can correlate log events with APM slow traces and errors, troubleshooting becomes easy.
Try your 14-day free trial of Atatus.
#1 Solution for Logs, Traces & Metrics
APM
Kubernetes
Logs
Synthetics
RUM
Serverless
Security
More