Simplifying Code with PHP Shorthand Techniques
Are you tired of typing out long lines of code? Do you wish there was a faster way to write code without sacrificing readability? Look no further than shorthand programming! Shorthand allows you to write code in a more abbreviated syntax, saving your time and effort.
But what exactly is shorthand programming and how can you use it to your advantage? In programming, shorthand refers to a way of writing code using abbreviated syntax.
Hope by now, you would have got some clarity about shorthand programming. In this blog, we are going to particularly look into the concept of Shorthand comparisons in PHP using an abbreviated syntax, saving your time and effort.
Lets get started!
Table of contents
- Null Coalescing Operator
- Null Coalescing on Arrays
- Null Coalesce Chaining
- Nested Coalescing
- Spaceship Operator
- Ternary Operator
- PHP if Shorthand
Shorthand Comparison in PHP
Shorthand comparison, also known as the ternary operator, is a shorthand way of writing an if-else statement in PHP. It is used to assign a value to a variable based on a condition.
You may be familiar with some of the comparison operators in PHP, such as the ternary operator ?:
, the null coalescing operator ??
, and the spaceship operator <=>
. By understanding these operators in more detail, you can use them more effectively and write more concise and readable code.
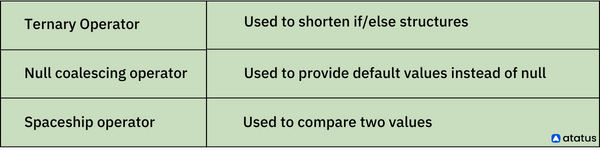
1. Null Coalescing Operator
The null coalescing operator is a shorthand way of checking if a variable is null and returning a default value if it is. The null coalescing operator is denoted by ??
. The double question marks were chosen to avoid conflicts with other operators and to make the operator easy to remember and type. This is available in PHP starting from version 7.0.
Syntax:
$variableName = $valueToCheck ?? $defaultValue;
In this syntax:
$valueToCheck
is the variable that you want to check for null.$defaultValue
is the value to return if$valueToCheck
is null.
Let us look into some examples to understand the concept of null coalescing better.
Example: In the below example, we use isset()
to check if the $_POST
variables are set, and then assign the appropriate value to the $firstName
and $lastName
variables.
<?php
if (isset($_POST["firstName"])) {
$firstName = $_POST["firstName"];
} else {
$firstName = "Unknown";
}
if (isset($_POST["lastName"])) {
$lastName = $_POST["lastName"];
} else {
$lastName = "Unknown";
}
echo "Welcome, {$firstName} {$lastName}!";
?>
Here is an equivalent example code that achieves the same result with using the null coalescing operator.
<?php
$firstName = $_POST["firstName"] ?? "Unknown";
$lastName = $_POST["lastName"] ?? "Unknown";
echo "Welcome, {$firstName} {$lastName}!";
?>
In this example, we use the null coalescing operator to assign a default value of Unknown
to the $firstName
and $lastName
variables if they are not set in the $_POST
super global array.
2. Null Coalescing on Arrays
In PHP, the Null coalescing operator ??
can be used with arrays to provide a shorthand way of assigning a default value to a variable if the array element being accessed is null.
Example: Assigning a default value to an array element.
<?php
$array = [null, "hello", null, "world"];
$value = $array[0] ?? "default";
echo $value;
?>
In this example, an array is created with four elements, including two null values. This code assigns the value of the first element of the array to the variable $value
, but if the value of the first element is null
, it assigns the default value of default
to the variable instead. This is achieved using the null coalescing operator ??
.

In this case, since the value of the first element of the array is null, the null coalescing operator assigns the value default
to the variable $value
.
Example: Assigning a default value to an array if it is null.
<?php
$array = null;
$value = $array ?? ["default"];
print_r($value);
?>
This code assigns the value of $array
to the variable $value
, but if the value of $array
is null
, it assigns the default value of ["default"]
to the variable instead. This is achieved using the null coalescing operator ??
.

In this case, since the value of $array
is null
, the null coalescing operator assigns the default value of ["default"]
to the variable $value
.
3. Null Coalesce Chaining
Null coalesce chaining allows you to chain multiple null coalescing operations together, so that if any one of them returns a non-null value, the entire expression will evaluate to that value.
Here is an example of using null coalesce chaining:
'name' => 'John Doe'
'email' => null
'phone' => null
The code defines an associative array $userData
which represents the user data. The array has three key-value pairs. The name
key has a non-null value, while the email
and phone
keys have null values. The code uses null coalesce chaining to set the values of three variables.
$displayName = $userData['displayName'] ?? $userData['name'] ?? 'Guest';
$displayName
is set to the value of $userData['displayName']
, or if that value is null, the value of $userData['name']
, or if that value is also null, the string Guest
. The null coalesce operator (??)
allows the code to check each value in turn until a non-null value is found. In this case, since $userData['name']
is non-null, it is used as the value of $displayName
.
$userEmail = $userData['email'] ?? 'No email provided';
$userEmail
is set to the value of $userData['email']
, or if that value is null, the string No email provided
. This line is straightforward: if the email is not provided, the string No email provided
is used as a default value.
$userPhone = $userData['phone'] ?? 'No phone number provided';
$userPhone
is set to the value of $userData['phone']
, or if that value is null, the string 'No phone number provided'
. This line is similar to the previous line, but for the phone number.
echo $displayName;
echo $userEmail;
echo $userPhone;
And finally, the code uses echo
statements to output the values of each variable.
<?php
$userData = [
"name" => "John Doe",
"email" => null,
"phone" => null,
];
$displayName = $userData["displayName"] ?? ($userData["name"] ?? "Guest");
$userEmail = $userData["email"] ?? "No email provided";
$userPhone = $userData["phone"] ?? "No phone number provided";
echo $displayName;
echo $userEmail;
echo $userPhone;
?>
Output:
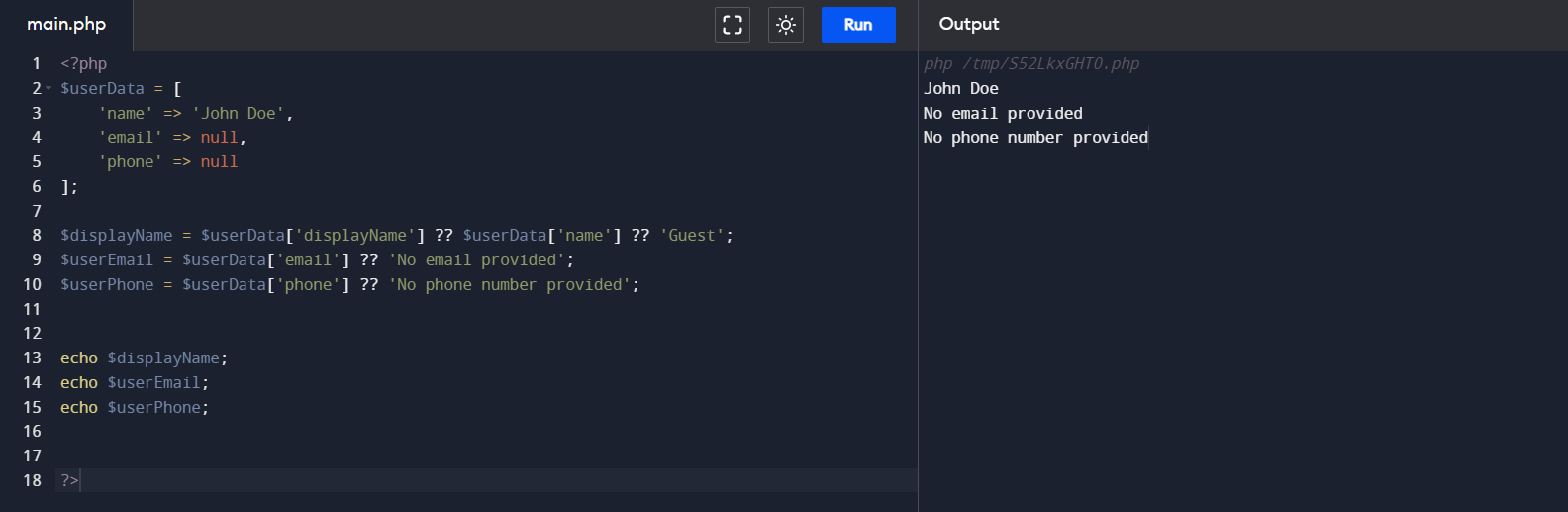
4. Nested Coalescing
Nested coalescing is a technique in PHP that allows you to check for nested null values and return a default value if any of the values are null. This technique involves using multiple null coalescing operators (??)
to check each nested value in turn until a non-null value is found.
<?php
$userData = [
"name" => "John Doe",
"address" => [
"street" => null,
"city" => "New York",
"state" => "NY",
],
];
$street = $userData["address"]["street"] ?? "Unknown";
$city = $userData["address"]["city"] ?? "Unknown";
$state = $userData["address"]["state"] ?? "Unknown";
echo $street . ", " . $city . ", " . $state;
?>
In this example, the $userData
array contains an inner array under the address key. The street key in the inner array has a null value, while the city and state keys have non-null values.
$street = $userData['address']['street'] ?? 'Unknown';
$city = $userData['address']['city'] ?? 'Unknown';
$state = $userData['address']['state'] ?? 'Unknown';
The code uses nested null coalescing operators to check each nested value in turn. The first line checks the value of $userData['address']['street']
. Since this value is null, the default value of 'Unknown'
is used instead.
The second and third lines check the values of $userData['address']['city']
and $userData['address']['state']
, respectively. Since these values are non-null, they are used as the values of $city
and $state
, respectively.
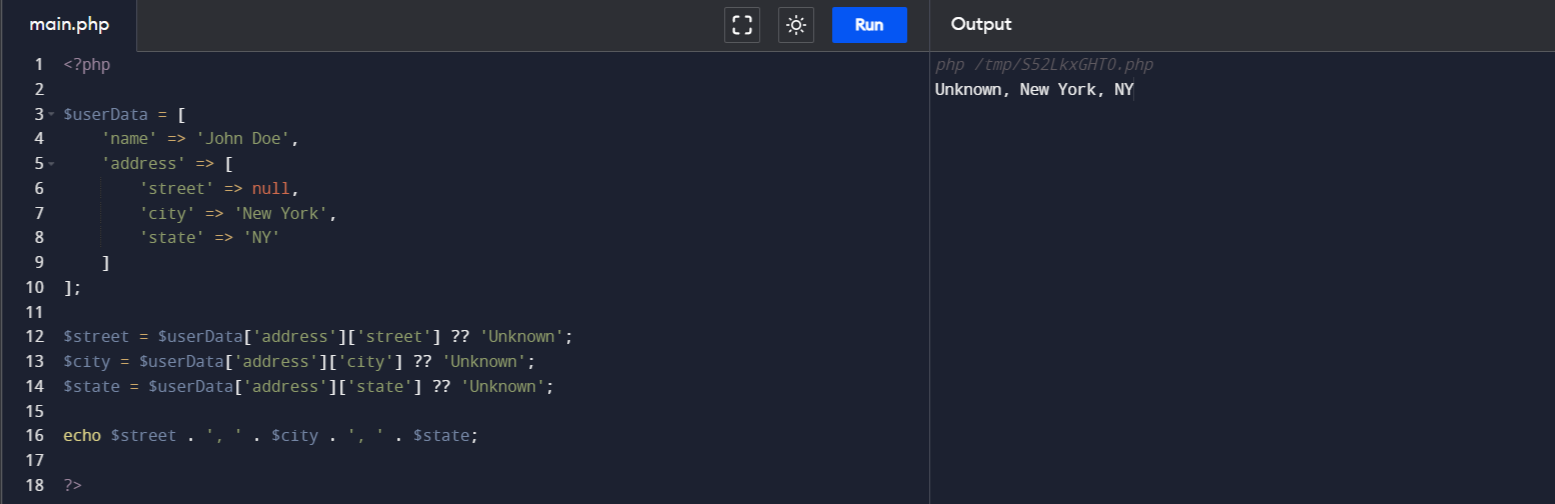
5. Spaceship Operator
The spaceship operator (<=>)
is a comparison operator introduced in PHP 7. It compares two values and returns an integer value that indicates the relationship between the values. The spaceship operator is also known as the "three-way comparison operator" because it can return one of three possible values: -1, 0, or 1.
The spaceship operator has the following syntax:
$value1 <=> $value2
The operator returns one of three possible values:
(i) -1: if $value1
is less than $value2
(ii) 0: if $value1
is equal to $value2
(iii) 1: if $value1
is greater than $value2
The spaceship operator can be used to compare values of different types, including integers, floats, strings, and arrays. When comparing values of different types, the operator uses the same rules as the comparison operators (<, <=, >, >=), but returns the integer value instead of a boolean value.
<?php
$a = 10;
$b = 20;
$result = $a <=> $b;
if ($result == -1) {
echo "$a is less than $b";
} elseif ($result == 0) {
echo "$a is equal to $b";
} else {
echo "$a is greater than $b";
}
?>
In this example, $a
is assigned a value of 10 and $b
is assigned a value of 20. The spaceship operator is used to compare these two values.
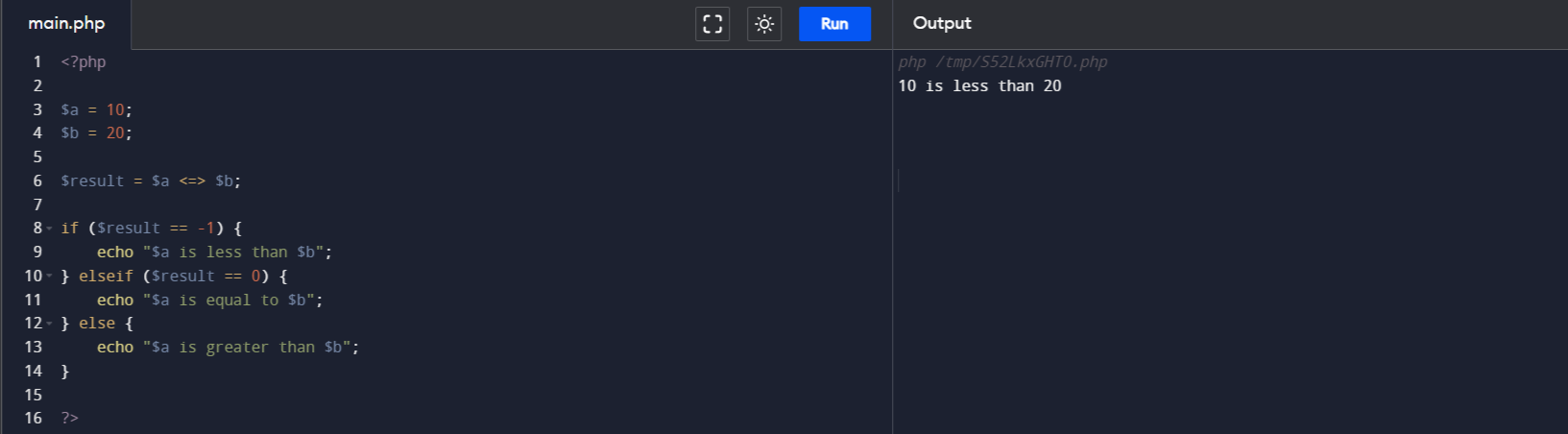
6. Ternary Operator
The ternary operator is a shorthand way of writing an if-else
statement. The ternary operator is also known as the conditional operator or the shorthand if statement. The ternary operator is denoted by ?:
Syntax: The syntax of the ternary operator in PHP is as follows
(condition) ? 'value if true' : 'value if false';
The ternary operator consists of three parts:
- The condition to be evaluated, enclosed in parentheses.
- The value to be returned if the condition is true, separated from the condition by a question mark (?).
- The value to be returned if the condition is false, separated from the true value by a colon (:).
7. PHP if Shorthand
PHP if shorthand refers to using a shortened syntax for the if statement in PHP code. The shorthand syntax for if statements in PHP is called the ternary operator. It allows you to write a conditional statement in a single line of code.
<?php
$score = 75;
if ($score >= 60)
{
$result = 'Pass';
}
else
{
$result = 'Fail';
}
echo $result;
?>
In this version of the code, we use a traditional if
statement to check if the $score variable is greater than or equal to 60. If it is, we set the $result
variable to "Pass"; otherwise, we set it to "Fail". The value of the $result
variable is then shown on the screen.
Here is the same code as in the previous one, but using the ternary operator. The ternary operator uses the following syntax.
(condition) ? 'value if true' : 'value if false';
Let us try writing the code with the ternary operator using the above syntax in PHP
<?php
$score = 75;
$result = ($score >= 60) ? 'Pass' : 'Fail';
echo $result; // output: Pass
?>
In this example, we use the ternary operator to check if the $score
variable is greater than or equal to 60. If it is, the result variable is set to "Pass"; otherwise, it is set to "Fail".
Using the ternary operator can make your code more concise and easier to read, especially if you have a simple if
statement with only one action to perform. However, it's important to use it appropriately and not to overuse it, as it can make your code harder to understand if it becomes too complex.
Key Takeaways
Shorthand PHP, on one hand, can make code shorter and easier to read, but on the other hand, it can make code harder to understand and maintain. One of the benefits of shorthand PHP is that it can help reduce the amount of code needed to accomplish a task. This can be particularly useful in cases where code readability is not a top priority.
However, it can also make code harder to understand, particularly for beginners or developers who are not familiar with the shorthand notation. This can lead to bugs, errors, and other issues, which can be difficult to troubleshoot and fix.
Using shorthand PHP depends on a number of factors, including the specific use case, the developer's skill level, and the project's overall goals and constraints. Although shorthand PHP can offer significant benefits when used correctly, it is crucial to remain aware of its limitations and use it prudently to prevent potential problems in the future.
Atatus: PHP Performance Monitoring and Log Management
Atatus is an Application Performance Management (APM) solution that collects all requests to your PHP applications without requiring you to change your source code. However, the tool does more than just keep track of your application's performance.
Monitor logs from all of your PHP applications and systems into a centralized and easy-to-navigate user interface, allowing you to troubleshoot faster using PHP monitoring.
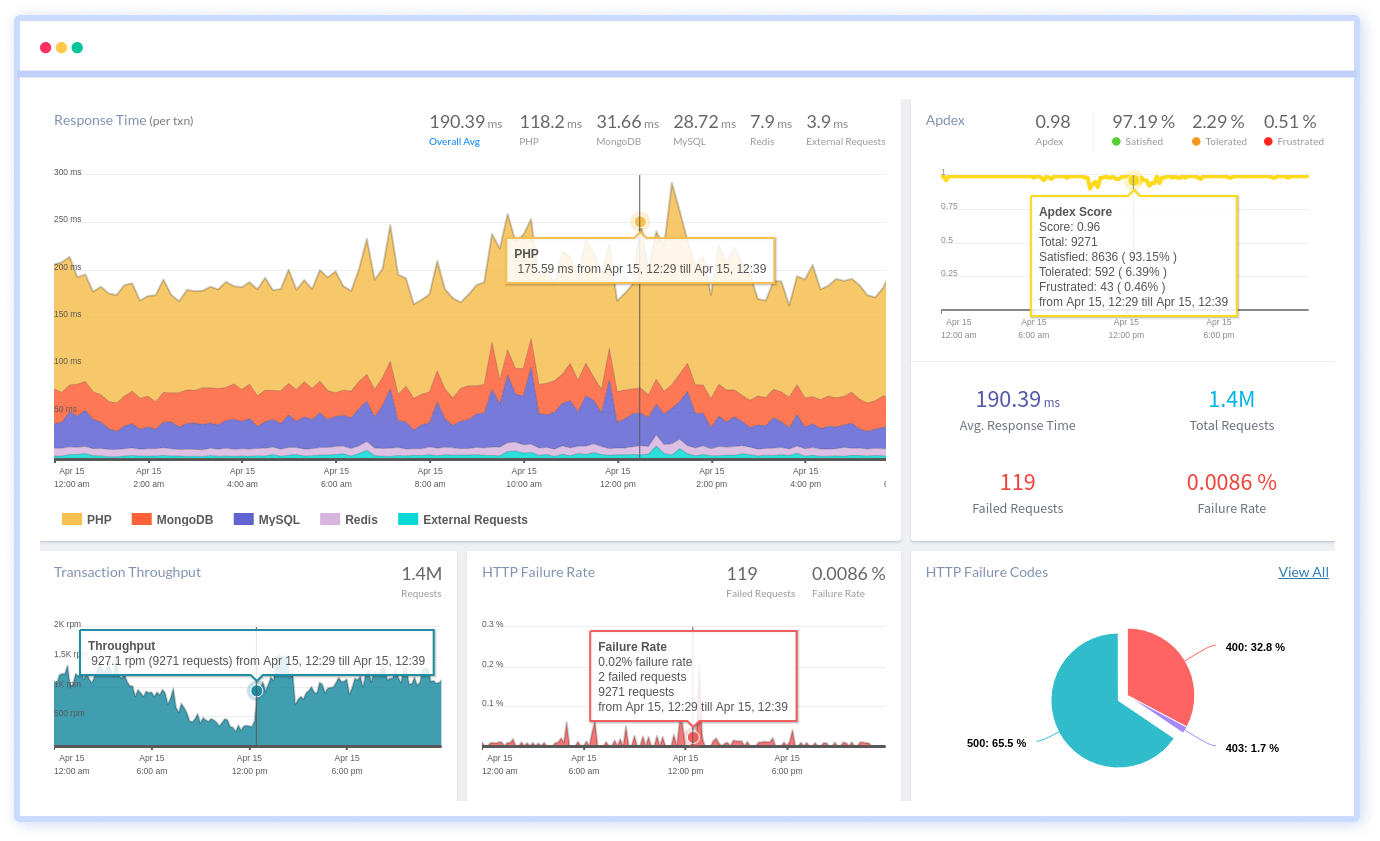
We give a cost-effective, scalable method to centralized PHP logging, so you can obtain total insight across your complex architecture. To cut through the noise and focus on the key events that matter, you can search the logs by hostname, service, source, messages, and more. When you can correlate log events with APM slow traces and errors, troubleshooting becomes easy.
#1 Solution for Logs, Traces & Metrics
APM
Kubernetes
Logs
Synthetics
RUM
Serverless
Security
More