Troubleshooting PHP Segmentation Faults: Effective Debugging Tips
PHP, one of the world's most popular server-side scripting languages, powers a significant portion of the internet's dynamic web applications.
While PHP is renowned for its flexibility and ease of use, it is not immune to the challenges that come with complex software development. One such challenge is the occurrence of segmentation faults, elusive errors that can leave developers scratching their heads in frustration.
A segmentation fault, or segfault, is a stern signal from the operating system that something has gone awry within your PHP application, leading to an abrupt and often mystifying crash.
However, understanding the origins of these faults and mastering the art of debugging them can transform them from enigmatic foes into conquerable obstacles.
In this article, we will delve into the world of PHP segmentation faults, exploring their causes and, most importantly, providing you with effective strategies and tools to troubleshoot and resolve these issues, ensuring the stability and reliability of your PHP applications.
Table Of Contents:-
- What is PHP Segmentation Fault?
- Troubleshooting the cause of PHP Segfault
- Diagnosing PHP Segfaults
- Atatus PHP Performance Monitoring
- Process of Debugging PHP Builds
- Measures to Prevent Segfaults
What is PHP Segmentation Fault?
A "Segmentation Fault" (often abbreviated as "segfault") is a type of error that occurs when a program tries to access a memory location that it is not allowed to access.
This typically happens when a program tries to read or write to a memory address that it doesn't have permission to access, or when it tries to access memory that has been freed or is otherwise invalid. When a segmentation fault occurs, the operating system terminates the program to prevent it from causing further issues.
In the context of PHP, a segmentation fault is a severe error that can happen when running PHP scripts or web applications. It usually indicates a bug or issue in the PHP interpreter or one of the extensions/modules loaded by PHP.
How does it occur?
Common causes of segmentation faults in PHP include:
- Bugs in PHP itself: PHP is written in C, and like any software, it can contain bugs. A segmentation fault in PHP may be due to a bug in the PHP interpreter.
- Bugs in PHP extensions/modules: PHP can be extended with additional modules and extensions, and if one of these extensions has a bug, it can lead to a segmentation fault when the extension is used.
- Invalid memory access: If a PHP script tries to access memory that it doesn't have permission to access, it can result in a segmentation fault. This could happen due to programming errors, such as accessing an array element that doesn't exist or dereferencing a null pointer.
- Server misconfigurations: In some cases, server misconfigurations, such as incorrect permissions on files or directories, can lead to segmentation faults when PHP scripts are executed.
- Incompatible libraries: Using incompatible or outdated libraries with PHP can sometimes lead to segmentation faults if there are compatibility issues.
Troubleshooting the cause of PHP Segfault
Finding the cause of a PHP segmentation fault can be a challenging and complex task, as it often involves debugging at a low level. Here are steps you can take to help identify and troubleshoot the cause of a PHP segfault:
- Start by checking the PHP error logs (usually found in /var/log/php/error.log or a similar location). Look for any error messages or information related to the segmentation fault. Sometimes, PHP itself may log details about the crash.
- Try to reproduce the segfault consistently. Identify the specific PHP script or operation that triggers the segmentation fault. Narrowing down the issue to a specific code path can be extremely helpful.
- If you suspect that a specific extension or module is causing the segfault, try disabling them one by one and see if the issue persists. This can help pinpoint the problematic extension.
- Employ debugging tools like GDB (GNU Debugger) to attach to the PHP process when it crashes. You can use GDB to generate a backtrace and get more information about where the crash occurred. Here's a basic example of how to use GDB:
gdb /path/to/php
(gdb) run -f /path/to/your/script.php
(Wait for the segmentation fault to occur)
(gdb) bt
The bt
command will display a backtrace, which shows the call stack leading up to the crash.
If PHP generates a core dump when it crashes, you can analyze it with GDB to get more detailed information about the crash. You'll need to enable core dumps on your system if they are not already enabled.
ulimit -c unlimited # Enable core dumps
Then, you can analyze the core dump using GDB:
gdb /path/to/php /path/to/coredump
(gdb) bt
Seek help from the PHP community and forums. Others might have encountered similar issues and can provide guidance or solutions. If you are unable to identify and resolve the issue on your own, consider seeking professional support from experienced PHP developers or consulting services that specialize in debugging and troubleshooting PHP applications.
Remember that debugging segmentation faults can be a time-consuming process, and patience is often required. Additionally, it's essential to keep your server and PHP environment updated to minimize the chances of encountering known bugs that have already been fixed in newer versions.
Diagnosing PHP Segfaults
When you encounter a segmentation fault in PHP, it can be challenging to diagnose and fix because it often requires debugging at a lower level, involving C code or the PHP interpreter itself. To address such issues, you may need to:
- Check for any recent changes in your PHP code, extensions, or server configuration that might have triggered the segmentation fault.
- Update PHP and its extensions to the latest stable versions to ensure that you are not encountering a known bug that has already been fixed.
- Disable or remove recently added extensions or modules one by one to identify if a specific extension is causing the issue.
- Use debugging tools like GDB (GNU Debugger) to trace the segmentation fault and pinpoint the exact location of the issue in the code.
- Review PHP error logs and system logs for any additional information about the segmentation fault.
- Seek help from the PHP community or a knowledgeable developer who may have experience debugging such issues.
Process of Debugging PHP Builds
PHP segfaults can be debugged both in the live environment as well as after an error has occurred .i.e Post-mortem debugging. Let's look at these two in a bit more detail below:
1. Live Debugging Segfaults
Runtime debugging of a PHP segmentation fault (segfault) can help you pinpoint where and why a crash is occurring. Let's go over a step-by-step approach to debug a PHP segfault at runtime:
Before you begin debugging, ensure that core dumps are enabled on your system. Core dumps capture the memory content of a crashing process, which can then be analyzed to determine the cause of the crash. On Unix/Linux systems, use the ulimit command:
ulimit -c unlimited
Ensure that your system's configuration also allows for core dumps, which might involve tweaking /etc/security/limits.conf
or similar files.
Identify the exact PHP script or a specific section of the script causing the segmentation fault. Being able to reproduce the segfault consistently is crucial for effective debugging.
To get a backtrace from a segfault, you can use the GNU Debugger (GDB). Run the PHP script that produces the segfault with GDB:
gdb --args php /path/to/script.php
Then, within GDB:
(gdb) run
When PHP segfaults, you'll get back control in GDB. After a segfault occurs within the GDB session, generate a backtrace:
(gdb) bt
This backtrace will show the call stack at the time of the segfault, helping you trace the exact line of code or function that triggered it.
If PHP crashes and produces a core dump (e.g., core.12345), you can inspect it with GDB:
gdb php /path/to/core.dump
Once inside the GDB session, generate a backtrace as before:
(gdb) bt
Using GDB, you can inspect the values of variables at the time of the crash. For example:
(gdb) print variable_name
You can also inspect memory regions, call functions, and more. Tools like Valgrind can help identify memory-related issues such as overflows, underflows, and leaks:
valgrind php /path/to/script.php
If you suspect a PHP extension is the cause, you can disable them one by one and see if the segfault stops. Extensions can be disabled by editing the php.ini file or using the command line.
2. Post-mortem Debugging Segfaults
Post-mortem debugging of segfaults involves analyzing the core dump generated by a crashed process after the fact to identify the cause of the segmentation fault. This can be particularly useful when you cannot reproduce the issue in a live debugging session or when the crash occurs in a production environment.
Before you can perform post-mortem debugging, ensure that core dumps are enabled on your system. Then, if you have a way to reproduce the segfault, do so to generate a core dump. If not, you'll need to rely on any existing core dumps from previous crashes.
The core dump file is usually named "core" followed by the process ID (e.g., core.12345). It is typically generated in the same directory where the crashing process was executed or in the system's core dump directory (e.g., /var/core). Use the find command to search for core dump files:
find / -name "core.*"
Use the GNU Debugger (GDB) to analyze the core dump:
gdb /path/to/php /path/to/core.dump
Once inside GDB, you can analyze the core dump to get a backtrace:
(gdb) bt
Review the backtrace and the information from GDB to identify the root cause of the segmentation fault. Look for any invalid memory access, null pointer dereferencing, or other issues in your code or libraries.
Once you've identified the cause of the segmentation fault, you can work on fixing the issue in your code or, if it's a bug in a library or PHP itself, consider applying patches or updating the affected software components.
Measures to Prevent PHP Segfaults
Preventing segmentation faults (segfaults) in your programs, including those written in PHP, involves careful coding practices and adherence to memory management principles. Here are some tips to help you prevent segfaults in your PHP code and applications:
1. Check for NULL Pointers
Ensure that you never dereference a NULL pointer. Before accessing a variable, object, or array element, verify that it exists and has been properly initialized.
if ($variable !== null) {
// Safe to use $variable here
}
2. Array and String Bounds Checking
When working with arrays or strings, always check array indices and string lengths before accessing specific elements or characters. Avoid going beyond the bounds of an array or string.
if (isset($array[$index])) {
// Access $array[$index] safely
}
3. Memory Management
If you're dealing with manual memory management in PHP, such as with the malloc and free functions in C extensions, make sure to allocate and deallocate memory correctly. Avoid double-freeing memory or accessing freed memory.
4. Error Handling
Implement robust error handling in your PHP code using constructs like try...catch and if...else to gracefully handle unexpected conditions and prevent crashes.
try {
// Code that might cause errors
} catch (Exception $e) {
// Handle the error gracefully
}
5. Type Checking and Validation
Ensure that variables and data you receive from external sources, such as user inputs, are properly validated and cast to the correct data types before using them. Avoid type mismatches that can lead to undefined behavior.
6. Use PHP's Built-in Functions Safely
Be aware of the requirements and potential pitfalls of PHP's built-in functions. For example, when using functions like unset, make sure you pass valid variables and avoid double unsetting.
if (isset($variable)) {
unset($variable);
}
7. Third-Party Libraries and Extensions
Be cautious when using third-party libraries and extensions, as they may have bugs that can lead to segfaults. Keep these libraries and extensions up-to-date and monitor their release notes for security fixes and bug patches.
8. Memory Leaks
Watch out for memory leaks in long-running PHP scripts or applications. Ensure that you properly release resources and memory when they are no longer needed. Use tools like Valgrind or memory profiling extensions like Xdebug to detect memory leaks.
9. Logging and Monitoring
Implement comprehensive logging and monitoring in your applications to capture errors and unusual behavior. Regularly review logs to catch and address issues before they become critical.
10. Testing and Quality Assurance
Thoroughly test your code through unit tests, integration tests, and user acceptance testing. Automated testing can help catch many potential issues before they reach production. Use static analysis tools and conduct code reviews to identify potential issues in your codebase. Tools like PHPStan and PHP_CodeSniffer can help catch common programming mistakes.
Keep your PHP version and extensions/modules up-to-date. Many segfault-related bugs are fixed in newer versions of PHP and its extensions.
Atatus PHP Performance Monitoring
Atatus is an Application Performance Management (APM) collects all requests to your PHP applications without requiring you to change your source code. However, the tool does more than just keep track of your application's performance.
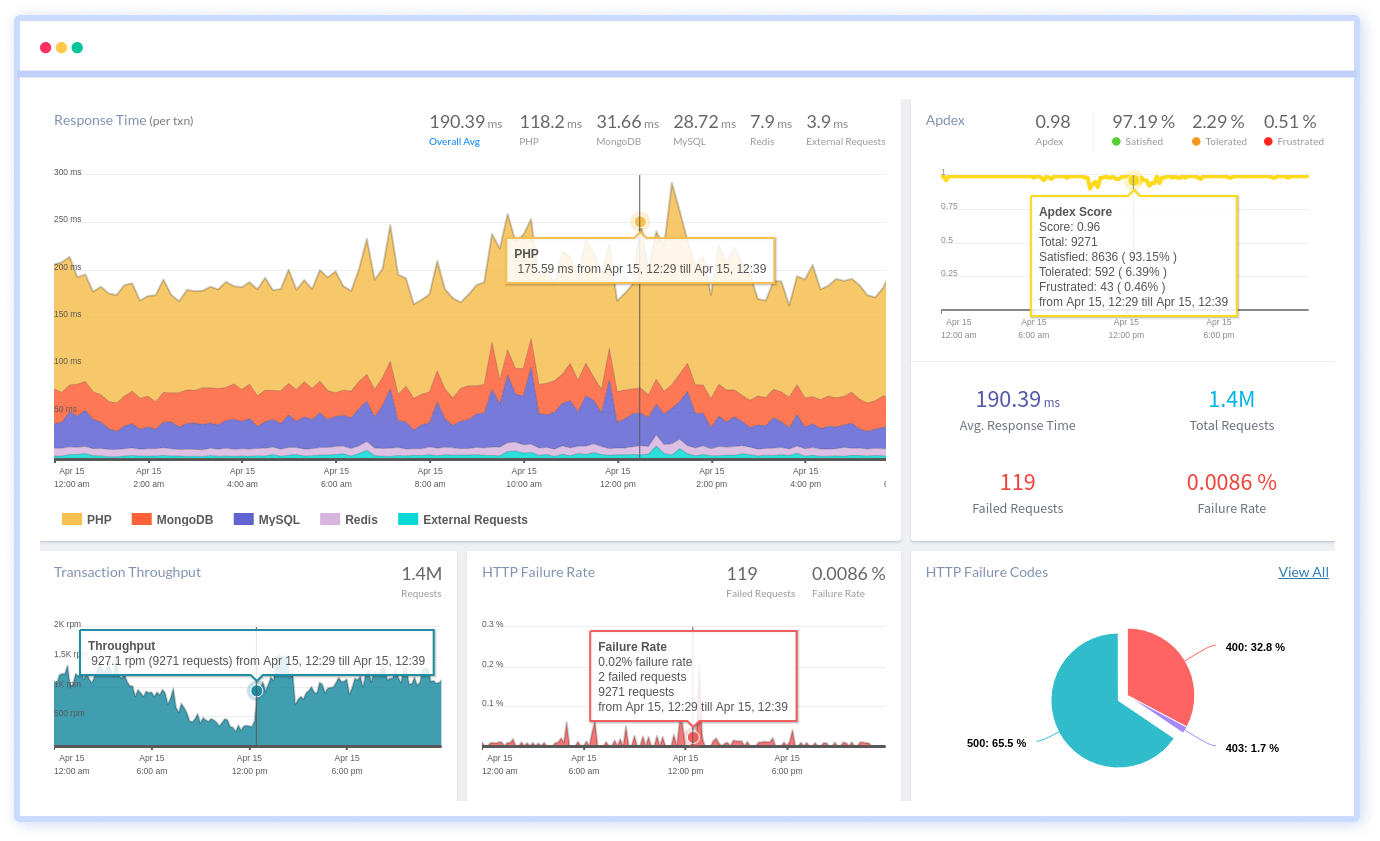
It monitors logs from all of your PHP applications and systems into a centralized and easy-to-navigate user interface, allowing you to troubleshoot faster using PHP monitoring. Atatus gives a cost-effective, scalable method to centralized logging, so that you can obtain total insight across your complex architecture.
To cut through the noise and focus on the key events that matter, you can search the logs by hostname, service, source, messages, and more. When you can correlate log events with APM slow traces and errors, troubleshooting becomes easy.
Try your 14-day free trial of Atatus.
Conclusion
We've discussed the importance of thorough debugging techniques, including runtime and post-mortem approaches, to uncover the root causes of segmentation faults in this article.
Armed with insights into the PHP source code, debugging tools like GDB, and best practices in memory management, developers can significantly reduce the likelihood of segmentation faults in their PHP projects.
While debugging segmentation faults may seem like a daunting task, it is a critical skill for PHP developers, enabling them to create robust and reliable applications.
By maintaining a proactive attitude towards debugging, staying informed about PHP updates and extensions, and fostering a culture of continuous improvement, developers can navigate the challenges presented by segmentation faults, ultimately ensuring the stability and performance of their PHP-based web applications.
#1 Solution for Logs, Traces & Metrics
APM
Kubernetes
Logs
Synthetics
RUM
Serverless
Security
More