Creating a Typesafe Dictionary: A Beginner's Guide
Have you ever messed up the data structure within your objects and as a result came across irresolvable errors during run-time? If yes, you’re not alone, we have all been there as developers. When the deadline approaches and you have way too many modules to work on somehow these blunders end up becoming a rampant source of misery and confusion.
This makes having Typesafe dictionaries and using them throughout your web development project essential. Whether you’re a frontend developer, Node.js Developer, or a Backend engineer creating a TypeSafe dictionary for you JS can help you maintain an easy-to-read codebase.
With this blog, we will take an in-depth look into how you can easily build a reliable typesafe dictionary for your web development projects. But before we dive into that let’s take a look at the reasons for building a TypeSafe dictionary.
- What is a Typesafe Dictionary?
- Create TypeSafe Dictionary using Object Type
- Create TypeSafe Dictionary with built-in ES6 maps And TS maps
- Create TypeSafe Dictionary through Record Type
- Conceptual Understanding of TypeSafe Dictionaries
What is a Typesafe Dictionary?
Typesafe dictionaries generally hold the data in key-value pairs and assist developers in easily accessing them. However, to successfully build a TypeSafe dictionary developer must follow the mentioned below rules of creating them:
- The developer must use a unique key for their dictionary
- Every key must have relevant values attached to them. These can be either string values or numbers as needed.
Usually, Dictionary type is used to create a Typesafe dictionary. However, if you’re using javascript this option might not be available for you in such scenarios you can use the following methods to create a typesafe dictionary for your codebase:
- Using Object in Javascript
- With built-in ES6 maps in Javascript
- Through Record Type, if you’re integrating TypeScript
By using a typesafe dictionary, you can ensure that the keys and values in the dictionary are of the correct types, which can help prevent bugs and make your code more robust.
Let’s now further look into each of their implementations with real-time examples for detailed understanding:
Create TypeSafe Dictionary using Object Type
The use of Object type is one of the most popular and easiest ways to create typesafe dictionaries in JS/TS
. This will allow you to create static key-value pairs for your code. You can further access these values in your function whenever needed and add new values to them as well.
To further understand how this works, let's create a dictionary named NewDictionary
as mentioned below:
let NewDictionary = {
'Name': 'Jhon',
'MiddleName': 'Doe',
'country': 'Sicily'
};
If you wish to add a new value you can achieve this in two ways as shown below:
- You can add a new value with a bracket Syntax:
NewDictionary['Name'] = 'Alice';
- You can also achieve this via property Name as well:
NewDictionary.Name = 'Alice';
Although this works fairly well in Javascript if you’re using Typescript using the same approach might not be functional and can lead to multiple errors.
Instead, you can use indexed object notations for creating typesafe dictionaries as this will help the Typescript to check the data type which is necessary for creating functional TS-based typesafe Dictionaries. In real-time using indexed object notation would look as the example below:
type NewUser = {
Name: string;
City: string;
}
const dictionary: { [key: number]: NewUser} = {};
dictionary[1] = {
Name: 'John',
City: 'Paris'
};
dictionary[2] = {
Name: 'Tom',
City: 'New York',
}
Create TypeSafe Dictionary with built-in ES6 maps And TS maps
The ES6 version of Javascript offers developers a smarter way to hold key-value pairs in the TypeSafe dictionary through Maps. The functionality of Maps operates quite similarly to JS-Objects.
However, with Maps in TS ot JS, you’ll not only hold the key-value pairs but it’ll also help your function remember the original order in which the keys were inserted for creating the Typesafe dictionary. Further, maps also provide innovative insertion methods which make working with the TypeSafe dictionary faster and easier for web developers.
Maps will usually follow the standard syntax mentioned below if you’re using TypeScript:
let map = new Map()([
["keyA", "valueA"],
["keyB", "valueB"],
["keyC", "valueC"],
]);
However, for JS developers the implementation of Map functionality would look something similar to this:
const my_dict = new Map<string, string>();
my_dict.set('keyA', 'valueA');
my_dict.set('keyB', 'valueB');
console.log(my_dict.has('keyA')); //To verify the key
console.log(my_dict.get('keyA')); //To get the value of key
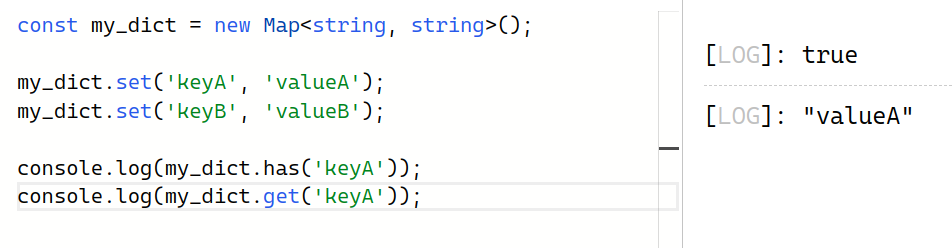
Create TypeSafe Dictionary through Record Type
If instead of JS, you’re integrating Typescript apart from the above-mentioned methods object and map you can also use Record Type for creating a TypeSafe Dictionary and work with the functionalities assigned for it.
Record Type basically constructs object type which instead of key-value pairs holds the data in Key-Types.
Here’s a working typesafe dictionary created using Record Type which holds name and city of three users:
interface Information {
age: number;
breed: string;
}
type Information = "Bruno" | "Welma" | "Molly";
const info: Record<CatName, CatInfo> = {
Bruno: { age: 13, breed: "Persian" },
Welma: { age: 15, breed: "Maine Coon" },
Molly: { age: 12, breed: "British Shorthair" },
};
console.log(info.Bruno);
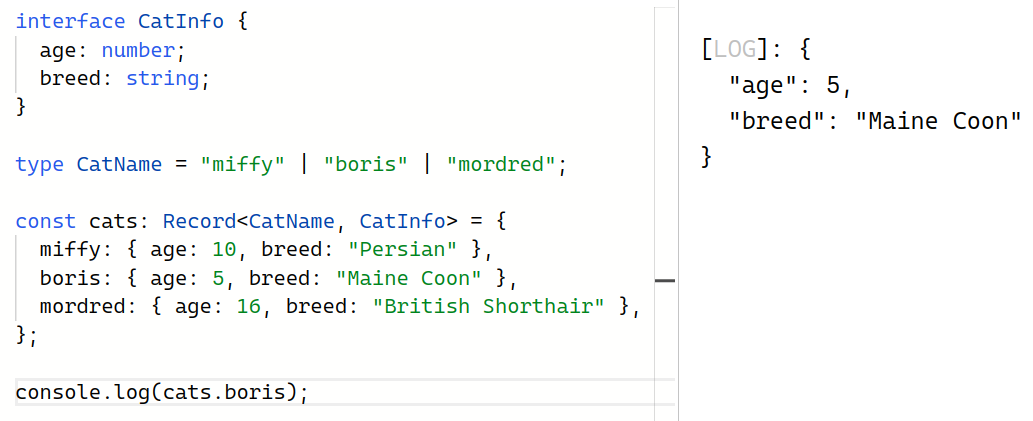
Conceptual Understanding of TypeSafe Dictionaries
Typesafe dictionaries are a must if you’re working with complex datasets. So far, by implementing different methods we can extract a fe foundational concepts which will make creating Typesafe dictionary easier.
Here are a few bullet points on basic concepts of Typesafe Dictionaries:
- Typesafe dictionaries basically hold data sets in key-value pairs to help developers create a one-stop collection of their relevant data sets.
- The Keys are used as a index to identify the values that are stored within the typesafe dictionary.
- Now, the Keys of these pairs is initially declared which means the dictionary will hold pre-defined data types only and you can not add a third or fourth key externally.
- However, there are multiple options which you can use to access, print, add, and alter values given to each key.
- Developers can create typesafe dictionaries in both Javascript and Typescript. However, with Typescript since there’s strict type checking declaring the data type and verifying them is essential.
Final Words
Creating Typesafe dictionaries instead of working with static data can be a game changer for developers. This not only allows you to hold the data within your codebase but also helps you access them via the designated key whenever necessary.
Hence, making the journey for using data error-free and more structured. Be it simple JS functions or complex code that implements useful functionality having a Typesafe dictionary can make implementations faster and easier.
Also apart from JS and Typescript, you can integrate Typesafe Dictionary in modern frontend languages such as React, Vue, Angular, etc. as well making the feature a must-know foundational concept for an effortless development experience.
So, without further adieu, open your editor and try out the above examples right away to gain a better understanding of Typesafe dictionaries.
Once you get a hang of these examples and can apply basic Typesafe dictionary-related functions, you can use the feature in real time within your JS and Typescript code base in order to access reliable and faster coding experience.
Atatus Front-end Performance Monitoring
Atatus is a scalable end-user experience monitoring system that allows you to see which areas of your website are underperforming and affecting your users. Understand the causes of your front-end performance issues and how to improve the user experience.
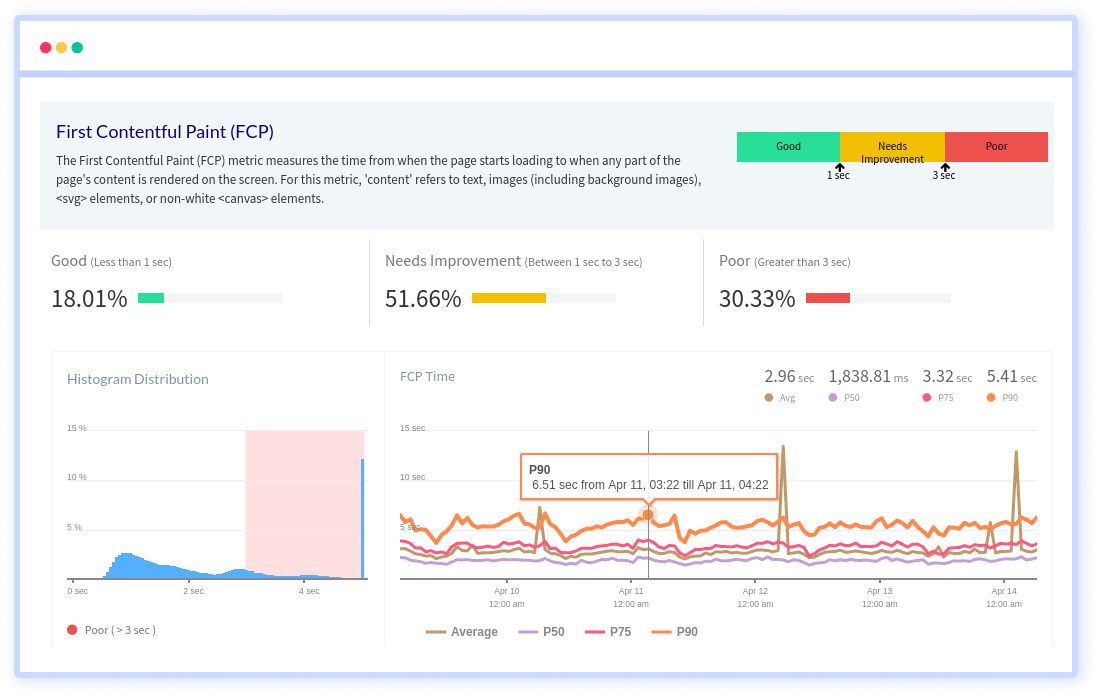
By understanding the complicated frontend performance issues that develop due to slow page loads, route modifications, delayed static assets, poor XMLHttpRequest, JS errors, core web vitals and more, you can discover and fix poor end-user performance with Real User Monitoring (RUM).
You can get a detailed view of each page-load event to quickly detect and fix frontend performance issues affecting actual users. With filterable data by URL, connection type, device, country, and more, you examine a detailed complete resource waterfall view to see which assets are slowing down your pages.
#1 Solution for Logs, Traces & Metrics
APM
Kubernetes
Logs
Synthetics
RUM
Serverless
Security
More