Python Functions: How to Use Them Effectively?
Python is a versatile and powerful programming language that has become increasingly popular over the years. One of its most important features is the ability to define and use functions.
Functions are a fundamental building block of any program, allowing us to encapsulate a piece of code that can be called and reused multiple times. In other words, functions are like mini-programs that can be called from within our main program.
But functions are not just a way to organize our code. They can also help us write more efficient and maintainable code, reduce the likelihood of bugs, and make our programs more flexible and adaptable to different situations. In fact, understanding how to use functions effectively is one of the key skills that separates a beginner Python developer from an expert.
In this blog, we will explore the different features and options available to us when working with Python functions. We will cover everything from the basics of defining and calling functions to more advanced topics such as handling different types of arguments.
Let's get started!
Table of contents
- What is a Python Function?
- Function Parameters and Arguments
- Number of Arguments
- Default Arguments
- Keyword Arguments
- Arbitrary Arguments
- Python Function Best Practices and Examples
What is a Python Function?
In Python, a function is a block of code that performs a specific task and runs only when it is called. Functions can take input arguments, perform operations on those arguments, and return an output value.
There are two types of functions in python,
- Built-in
- User-defined
1. Built-in functions
These are pre-defined functions in python, which are readily available for use without any additional code. They are designed in order to perform common tasks that are frequently encountered by the programmers. Here are some of the examples,
print()
: used to print outputlen()
: used to get the length of a list, tuple, or stringrange()
: used to generate a sequence of numberstype()
: used to get the data type of a variable or valueinput()
: used to get user input
Here is a simple example of using len()
function, which is a built-in function in python that returns the length.
Example:
string = "Hello, world!"
length = len(string)
print(length)
Output:

In this example, a string "Hello, world!" is assigned to the variable string. The built-in len()
function is then used to calculate the length of the string. Finally, the value of the length is Printed using the print()
function.
2. User-defined functions
These are the functions in python, created to perform a specific task by the programmer. User-defined functions are defined using the def keyword, followed by the function name, input parameters, and function code. They can be called and used anywhere in the program after they have been defined.
Have a look at the simple code to understand the user-defined function better.
Example:
def multiply_numbers(num1, num2):
product = num1 * num2
return product
result = multiply_numbers(3, 4)
print(result)
Output:
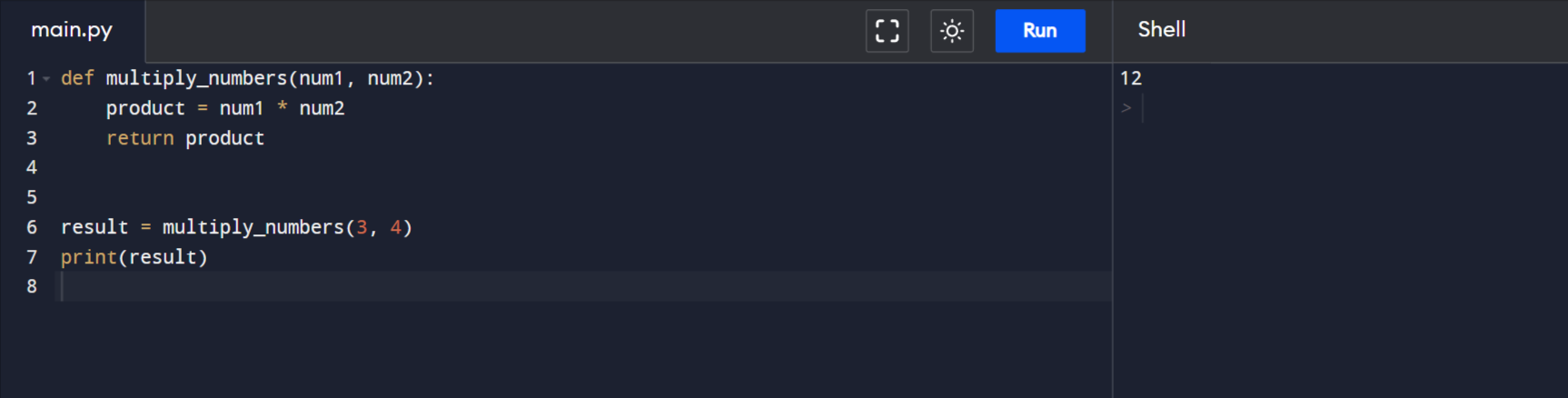
In this example, functions are defined using the def keyword, followed by the function name multiply_numbers
which takes two parameters num1
and num2
. Inside the function, we create a variable called product that stores the product of num1
and num2
. Finally, a return statement is used to return the value of the product. To call a function, use the function name, followed by parenthesis. Here when the function multiply_numbers(3, 4)
is called, it multiplies 3 and 4 together and returns the result.
Function Parameters and Arguments
In Python, function parameters and arguments refer to the values that are passed into a function.
A parameter is a variable in the function definition that represents a value that the function expects to receive when it is called. When you define a function, you can specify one or more parameters inside the parentheses after the function name.
Example:
def greet(name):
print("Hello, " + name + "!")
In this example, name is a parameter that is defined inside the parentheses after the greet
function name.
An argument is a value that is passed into a function when it is called. When you call a function, you can pass one or more arguments inside the parentheses.
Example:
def greet(name):
print("Hello, " + name + "!")
greet("Alice")
Output:
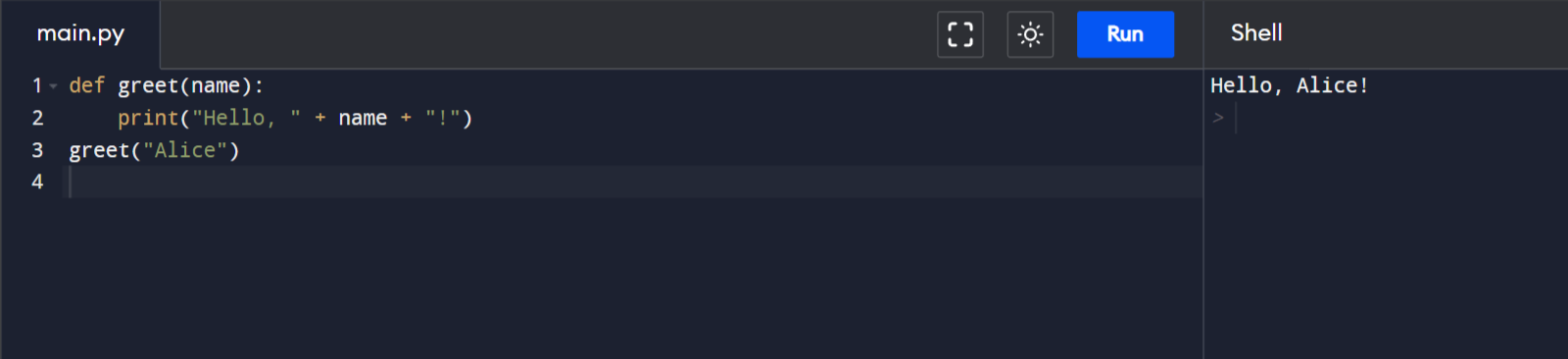
In this example, "Alice" is an argument that is passed into the greet
function when it is called.
The parameter(s) in the function definition are used to define the function and to specify what kind of input the function expects to receive. The argument(s) that are passed into the function when it is called are the actual values that are used by the function to perform its task.
It's important to note that the number of arguments passed into a function must match the number of parameters specified in the function definition. If you pass too few or too many arguments, you will get an error.
Number of Arguments
In Python, you can define a function that takes a specific number of arguments, or you can define a function that takes a variable number of arguments.
To define a function that takes a specific number of arguments, you simply specify the number of parameters in the function definition.
Example:
def add_numbers(x, y):
return x + y
sum = add_numbers(2, 3)
print(sum)
Output:
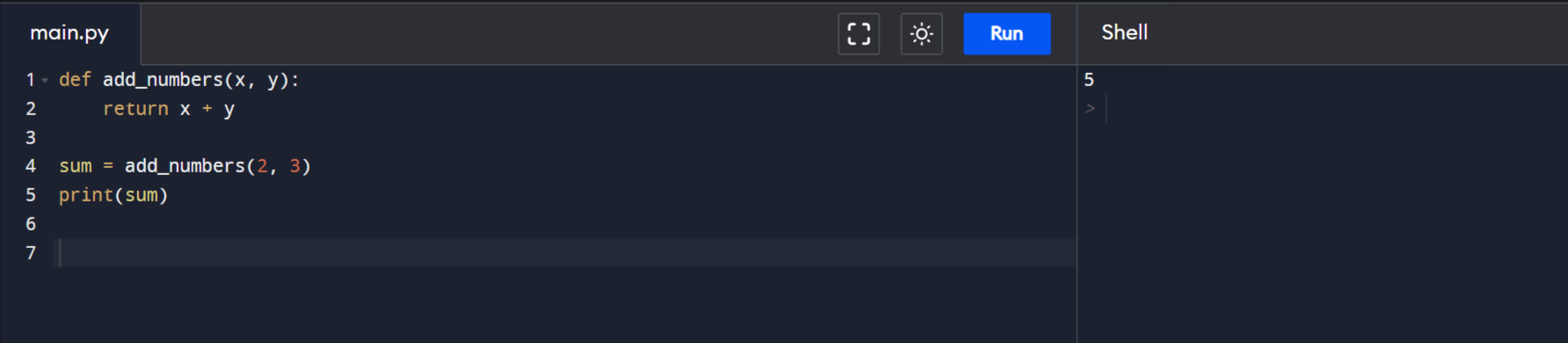
In this example, the add_numbers()
function takes two arguments, x and y. To call this function, you must pass exactly two arguments. In the given example, 2 is assigned to x, and 3 is assigned to y. The function then adds these two values and returns the result, which is assigned to the variable sum.
If you want to define a function that takes a variable number of arguments, you can use the *args
syntax in the function definition.
Example:
def print_arguments(*args):
for arg in args:
print(arg)
In this example, the print_arguments()
function takes any number of arguments and prints each argument on a separate line. To call this function, you can pass any number of arguments.
Example:
def print_arguments(*args):
for arg in args:
print(arg)
print_arguments("Hello", "world", 42)
Output:
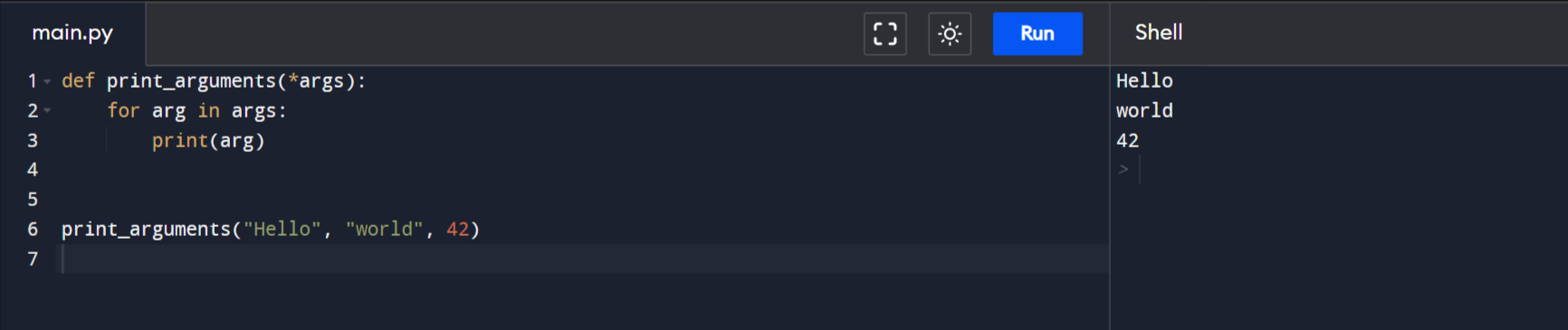
Default Arguments
Default arguments are arguments that are given a default value in a function definition, so that they can be omitted when the function is called. If the caller does not provide a value for a default argument, the default value is used instead.
Example:
def greet(name, greeting="Hello"):
print(greeting, name)
greet("Alice")
greet("Sam", "Hi there")
Output:
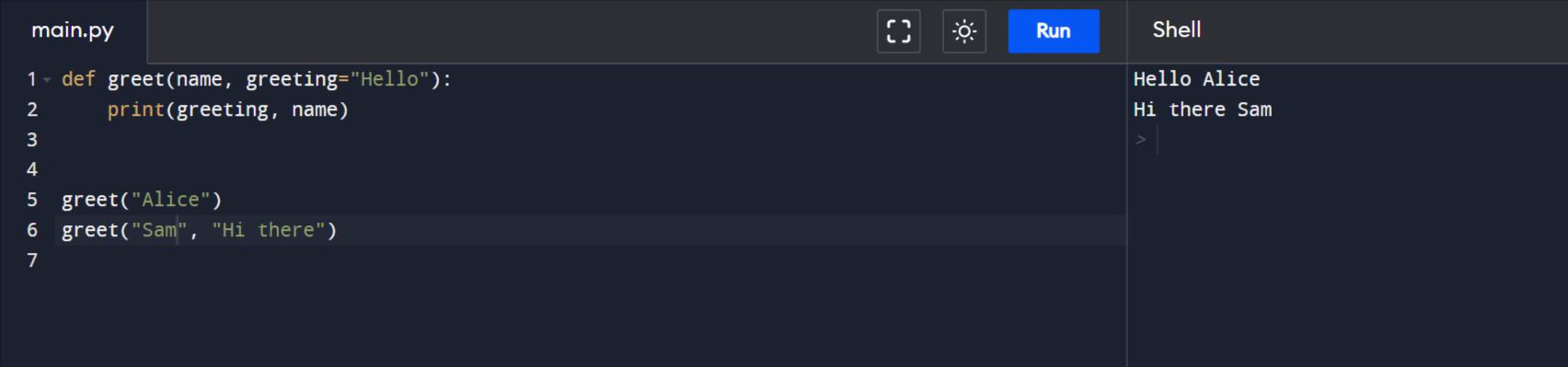
In this example, the greet()
function takes two arguments: name and greeting. The greeting argument has a default value of "Hello", so if it is not provided when the function is called, "Hello" will be used.
When we call greet("Alice")
, we only provide a value for name, so the default value of "Hello" is used for greeting, and the function prints "Hello Alice".
When we call greet("Sam", "Hi there")
, we provide values for both name and greeting, so the function prints "Hi there Sam".
Keyword Arguments
In Python, keyword arguments are a way to pass arguments to a function by specifying the name of the parameter, along with its value. This allows for greater flexibility and readability when calling functions with many arguments, as it is possible to explicitly state which argument is being passed to which parameter.
Here is an example of a function that takes two arguments and returns their sum.
Example:
def add_numbers(a, b):
return a + b
To call this function, you would need to pass in two arguments in the correct order.
def add_numbers(a, b):
return a + b
result = add_numbers(2, 3)
print(result)
Output:
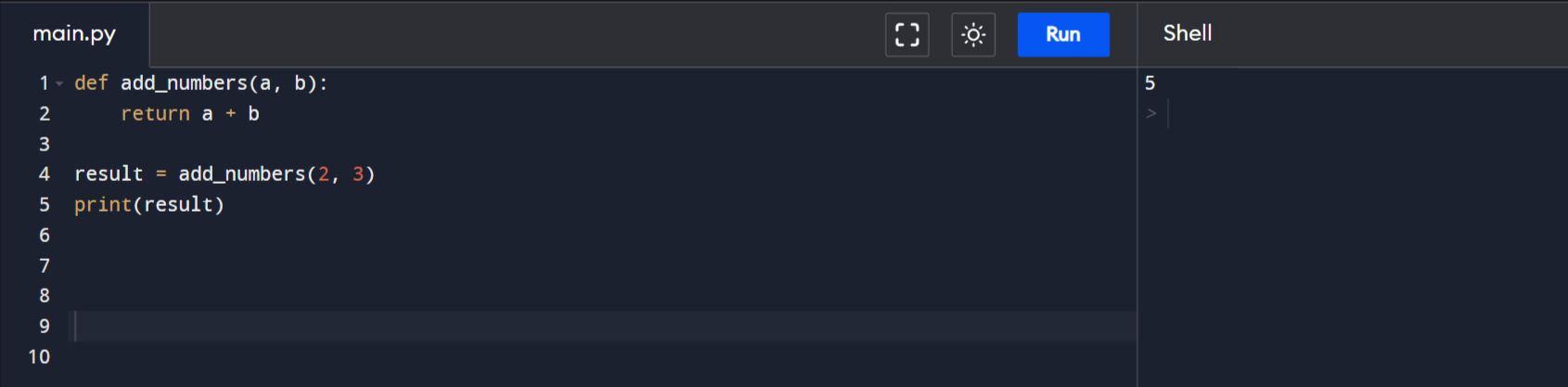
However, using keyword arguments, you can specify the parameter names explicitly as shown below.
def add_numbers(a, b):
return a + b
result = add_numbers(a=2, b=3)
print(result)
This code produces the same output as before, but it is now much clearer which argument is being passed to which parameter. It is also possible to pass the arguments in a different order as shown below.
def add_numbers(a, b):
return a + b
result = add_numbers(b=3, a=2)
print(result)
This is especially useful when a function has many arguments or when the order of the arguments is not immediately obvious. Also, note that the keyword arguments can also be used in combination with default parameter values.
Arbitrary Arguments
In Python, arbitrary arguments allow a function to accept an arbitrary number of arguments. There are two ways to define arbitrary arguments in Python: using the *args
syntax or using the **kwargs
syntax.
Using *args
, a function can accept a variable number of positional arguments. The *args
syntax allows you to pass any number of arguments to the function, which are then collected into a tuple.
Example:
def calculate_sum(*args):
total = 0
for num in args:
total += num
return total
# Call the function with different numbers of arguments
print(calculate_sum(1, 2, 3)) # Output: 6
print(calculate_sum(4, 5, 6, 7, 8)) # Output: 30
print(calculate_sum()) # Output: 0
Output:
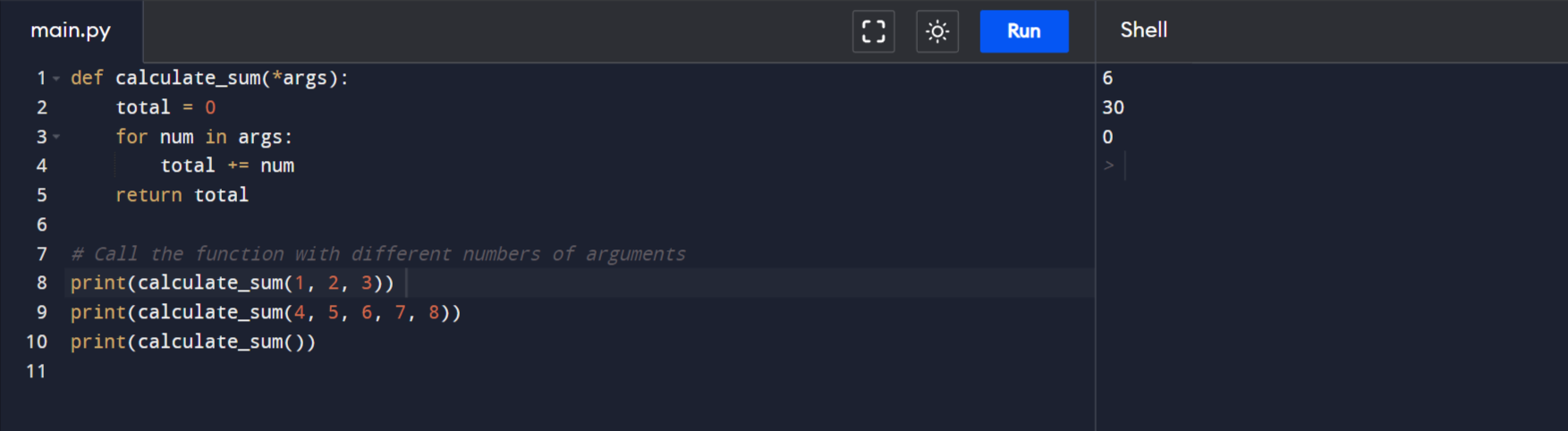
In this example, the function calculate_sum
takes an arbitrary number of arguments using the *args
syntax. It then loops through each argument and adds them up to calculate the total sum. Finally, the function returns the total.
Python Functions Best Practices and Examples
Here are some tips for using Python functions effectively, along with some examples:
1. Use descriptive function names: A function's name should clearly convey its purpose. This makes the code easier to read and understand. For example:
# Bad function name
def func(x):
return x ** 2
# Good function name
def calculate_square(x):
return x ** 2
2. Keep functions short and focused: Functions should generally perform a single task or a related group of tasks. This makes the code more modular and easier to understand. For example:
# Bad function
def process_data(data):
# Do a bunch of unrelated tasks
return result
# Good functions
def clean_data(data):
# Clean the data
return cleaned_data
def analyze_data(data):
# Analyze the data
return analysis_result
3. Use default argument values: Default argument values can simplify function calls and make the code more concise. For example:
# Without default arguments
def greet(name, greeting):
print(greeting, name)
greet("Alice", "Hello") # Prints "Hello Alice"
greet("Bob", "Hi there") # Prints "Hi there Bob"
# With default arguments
def greet(name, greeting="Hello"):
print(greeting, name)
greet("Alice") # Prints "Hello Alice"
greet("Bob", "Hi there") # Prints "Hi there Bob"
4. Use keyword arguments: Keyword arguments can make function calls more readable and help prevent errors due to argument order. For example:
# Without keyword arguments
def calc_total(price, tax_rate):
return price + price * tax_rate
total = calc_total(100, 0.08) # Price first, then tax rate
# With keyword arguments
def calc_total(price, tax_rate):
return price + price * tax_rate
total = calc_total(price=100, tax_rate=0.08) # Arguments by name
5. Use docstrings: Docstrings are used to document the purpose and usage of a function. They make the code more understandable and are also used by tools such as help(). For example:
def calculate_square(x):
"""
Calculates the square of a number.
Parameters:
x (float): The number to be squared.
Returns:
float: The square of the input number.
"""
return x ** 2
These are just a few examples of how to use Python functions effectively. By following these guidelines, you can write cleaner, more efficient, and more reusable code.
Conclusion
Functions are an essential part of any programming language, including Python. They allow us to encapsulate a piece of code that can be reused multiple times and to organize our code in a more manageable way.
In Python, we can define functions using the def keyword, followed by the function name and its parameters. We can also specify a return value using the return statement. Python functions can take both positional and keyword arguments, and we can also define default values for our parameters.
In addition, we can use arbitrary arguments to allow our functions to accept an arbitrary number of arguments. We can use this feature to simplify our code and make it more flexible.
Python functions are a powerful tool that can help us write more maintainable and efficient code. By understanding the different features and options available to us, we can create functions that are both reusable and adaptable to different situations.
Atatus: Python Performance Monitoring
Atatus is an Application Performance Management (APM) solution that collects all requests to your Python applications without requiring you to change your source code. However, the tool does more than just keep track of your application's performance.
Monitor logs from all of your Python applications and systems into a centralized and easy-to-navigate user interface, allowing you to troubleshoot faster using Python monitoring.
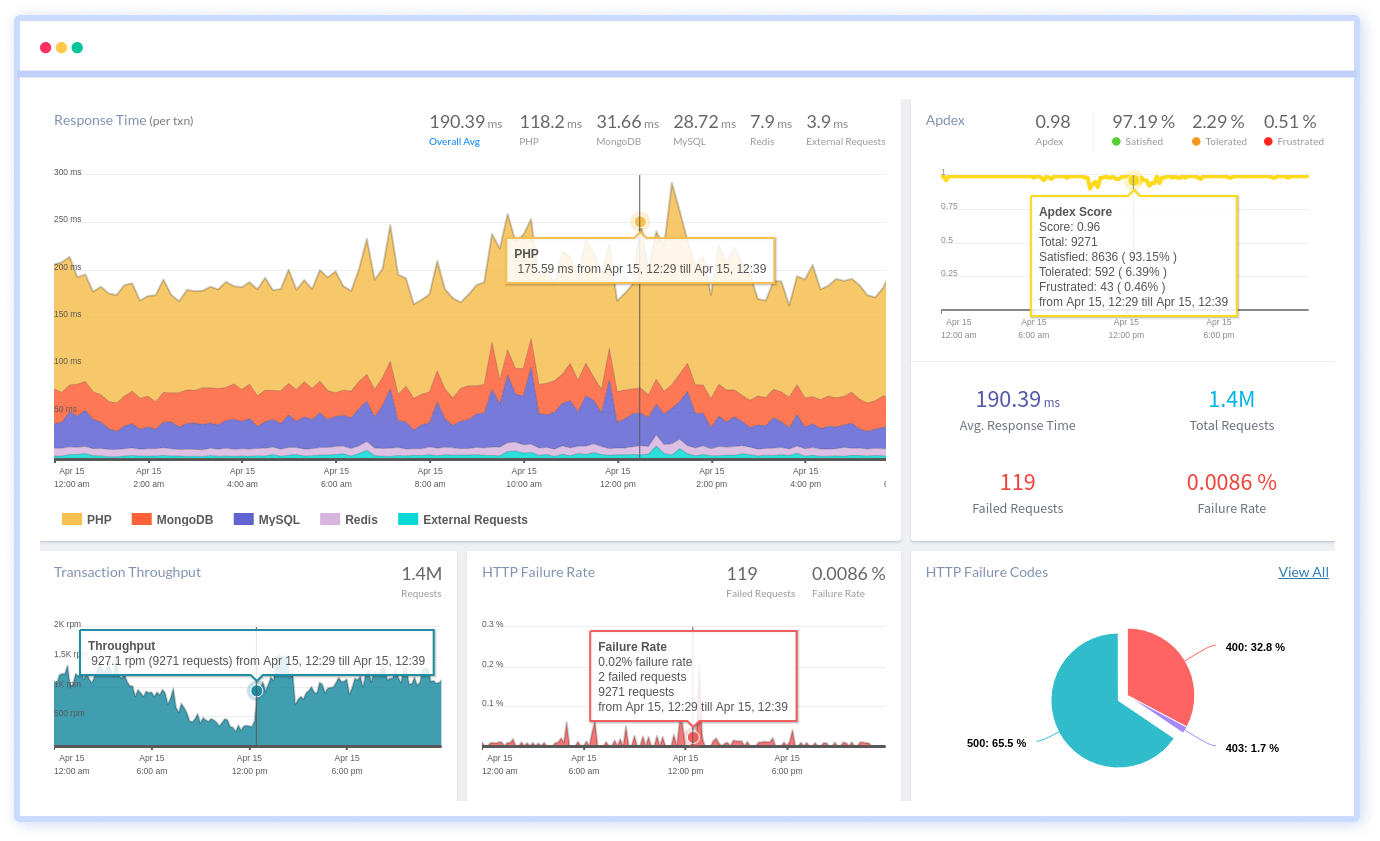
We give a cost-effective, scalable method to centralized Python logging, so you can obtain total insight across your complex architecture. To cut through the noise and focus on the key events that matter, you can search the logs by hostname, service, source, messages, and more. When you can correlate log events with APM slow traces and errors, troubleshooting becomes easy.
#1 Solution for Logs, Traces & Metrics
APM
Kubernetes
Logs
Synthetics
RUM
Serverless
Security
More