How to use React Hook Form for Crafting Interactive Forms?
Forms are a vital part of any digital solution, be it a gaming app or a simple online store crafting an interactive and easy-to-use form is a must to ensure user engagement.
However, while working with core React preparing and managing forms with multiple fields usually becomes a hassle.
As in core React, developers have to add lengthy lines of code to implement basic form features. This not only makes the code a hassle to work with but also complicates the overall codebase with unnecessary clutter.
For example, a typical React Form usually looks like this:
import React, { useState } from "react";
import "./styles.css";
export default function App() {
const [state, setState] = useState({
username: "",
password: ""
});
const handleInputChange = (event) => {
setState((prevProps) => ({
...prevProps,
[event.target.name]: event.target.value
}));
};
const handleSubmit = (event) => {
event.preventDefault();
console.log(state);
};
return (
<div className="Application">
<form onSubmit={handleSubmit}>
<div className="form-control">
<label>UserName</label>
<input
type="text"
name="username"
value={state.username}
onChange={handleInputChange}
/>
</div>
<div className="form-control">
<label>Password</label>
<input
type="password"
name="password"
value={state.password}
onChange={handleInputChange}
/>
</div>
<div className="form-control">
<label></label>
<button type="submit">Get Started</button>
</div>
</form>
</div>
);
}
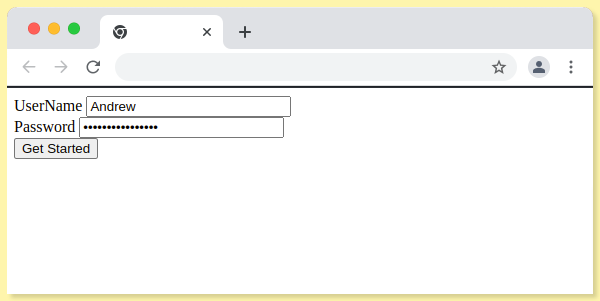
Now if we further add new fields and complex validation for making the form interactive, the codebase would end up getting cluttered. In the long run, this would make codebase maintenance and scalability a hassle.
To help developers speed up the form development process while ensuring the coding experience remains smooth React have introduced multiple libraries such as Formik, Redux-Form, and react hook form.
In the following React Hook form guide, we will take an in-depth look into how we can use react hook form to craft unique and interactive forms effectively without expanding the overall codebase.
- What is React Hook Form?
- How to use React Hook Form?
- How to validate fields Using React Hook Forms?
- Why React Hook Form is better than similar alternatives available?
What is React Hook Form?
The React Hook form is a simplistic React-based library that follows HTML standards for creating and validating forms without expanding the application codebase exponentially. Further, with the use of react hook form Library, you can easily improve the performance of your application forms and integrate them with major UI libraries since it supports the ref
attribute.
Adapting to the react hook form library helps developers avail unique perks which enhance their overall development experience and performance. Here’s a list of unique perks of using react hook form library:
Benefits of React Hook Form
- Lightweight: The react hook form is a lightweight library without any external dependencies. In the long run, this helps developers with improving the overall performance of their application forms and making their apps lightweight.
- Easy To Adapt: As the form state is inherently local you can easily use the library in your forms without any dependencies and speed up the overall development process with ease.
- Enhanced UX: The react hook form library helps in implementing validation strategies consistently which makes using the form an easier and more interactive experience for the users.
- Improved Performance: By using the react hook form library developers can easily minimize the number of re-renders and validate computations. For this, it uses uncontrolled inputs making use of “ref” in place of typically relying on the state to control overall inputs.
How to use React Hook Form?
Now that you have a clear insight into how react hook form can assist in creating eye-catching and performant React forms with ease let’s get a look into how you can start using it. You can follow the step-wise tutorial mentioned below to start integrating react hook forms with your current projects to make them lightweight and enhance their overall performance.
Step 1: To be able to use react hook form in your web and React Native application you’ll have to first install it with the command below:
npm install react-hook-form
Step 2: Once the package is installed, import the useform
from the react hook form which is a custom hook and can take an object as an optional argument to make managing the form easier.
import { useForm } from 'react-hook-form';
Step 3: After the useform
is imported its time to use the hook within the component as mentioned below:
const { register, handleSubmit } = useForm();
In this, the register method registers the input, and handleinput
method takes care of the form submission.
Step 4: Now in order to register the input let us pass it through input fields as below:
<input type='text' name='firstName' {...register('firstName')} />
Further, use the handlesubmit method for catering to further submissions. There are two functions that the handleSubmit
method can accept as arguments. When the form validation is successful, the first function supplied as an argument will be called along with the registered field values. When the validation fails, the second function is invoked with warnings.
const onFormSubmit = data => console.log(data);
const onErrors = errors => console.error(errors);
<form onSubmit={handleSubmit(onFormSubmit, onErrors)}>
{/* ... */}
</form>
Now the final form after following through with the steps to implement the react hook form library would look something as below.
import React from "react";
import { useForm } from "react-hook-form";
const RegisterForm = () => {
const { register, handleSubmit } = useForm();
const handleRegistration = (data) => console.log(data);
return (
<form onSubmit={handleSubmit(handleRegistration)}>
<div>
<label>First Name</label>
<input name="name" {...register('name')} />
</div>
<div>
<label>Last Name</label>
<input name="name" {...register('name')} />
</div>
<div>
<label>Phone Number</label>
<input name="number" {...register('phone')} />
</div>
<div>
<label>Email ID</label>
<input type="email" name="email" {...register('email')} />
</div>
<div>
<label>Password</label>
<input type="password" name="password" {...register('password')} />
</div>
<button>Get Started</button>
</form>
);
};
export default RegisterForm;
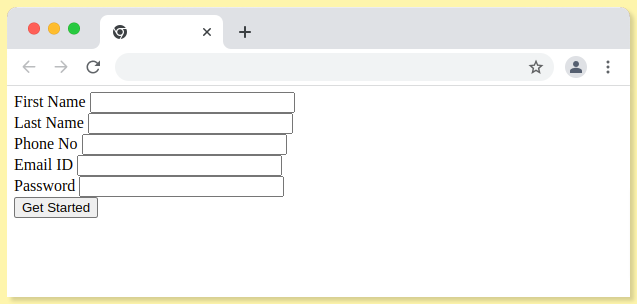
The code here unlike the example shown earlier looks more clear, concise, and easy to work with as no component had to be imported. Additionally, be it any UI library, you can integrate the React-Hook-Form with ease for crafting forms.
How to validate fields Using React Hook Forms?
Now that you are capable of creating a react hook form let us take things a step further by adding validations to the form. Validations guide the user to log in and signup effectively for using the app and makes the first touchpoints in the solution more interactive.
To add validation in our current form we will use two parameters as mentioned below:
- Required: Denoting the field is needed
- Maxlength: Signifying the maximum length of characters the user can input in the form.
Step 1:
To validate a text field, you can set both the parameters as follows:
<input placeholder="First Name" type="text" {...register("FirstName", { required: true, maxLength: 10 })} />
Further, you can also add in an error message as given below to inform the user and guide them in filling up the form efficiently.
{errors.firstName && <p>Please check the First Name</p>}
Step 2:
At times, validating a format-based field such as email and password is also essential to ensure secure access and an efficient login process. For this, you can use the pattern
parameter as mentioned below:
Validating the Email Fields:
<Form.Field>
<label>Email</label>
<input
placeholder='Email'
type="email"
{...register("email", {
required: true,
pattern: /^(([^<>()\[\]\\.,;:\s@"]+(\.[^<>()\[\]\\.,;:\s@"]+)*)|(".+"))@((\[[0-9]{1,3}\.[0-9]{1,3}\.[0-9]{1,3}\.[0-9]{1,3}\])|(([a-zA-Z\-0-9]+\.)+[a-zA-Z]{2,}))$/
}) } />
</Form.Field>
Note: The pattern mentioned above is in accordance with the industry standard for email verification.
Validating The Password Field:
<Form.Field>
<label>Password</label>
<input
placeholder='Password'
type="password"
{...register("password", {
required: true,
pattern: /^(?=.*\d)(?=.*[a-z])(?=.*[A-Z]).{6,15}$/
}) } />
</Form.Field>
{errors.password && <p>Please check the Password</p>}
Step 3:
After adding the required fields our final form would look something like this:
import React from 'react';
import { Form, Button } from 'semantic-ui-react';
import { useForm } from "react-hook-form";
export default function FormValidation() {
const { register, handleSubmit, formState: { errors } } = useForm();
const onSubmit = (data) => {
console.log(data);
}
return (
<div>
<Form onSubmit={handleSubmit(onSubmit)}>
<Form.Field>
<label>FirstName</label>
<input placeholder='First Name' type="text"
{...register("firstName", { required: true, maxLength: 8})} />
</Form.Field>
{errors.firstName &&
<p>Please Re-enter the First Name</p>
}
<Form.Field>
<label>mail</label>
<input placeholder='mail' type="email"
{...register("mail",
{
required: true,
pattern: /^(([^<>()\[\]\\.,;:\s@"]+(\.[^<>()\ [\]\\.,;:\s@"]+)*)|(".+"))@((\[[0-9]{1,3}\. [0-9]{1,3}\.[0-9]{1,3}\.[0-9]{1,3}\])|(([a- zA-Z\-0-9]+\.)+[a-zA-Z]{2,}))$/
})} />
</Form.Field>
{errors.email &&
<p>Please re-enter Email</p>
}
<Form.Field>
<label>Password</label>
<input placeholder='Password' type="password"
{...register("password", { required: true,
pattern: /^(?=.*\d)(?=.*[a-z])(?=.*[A-Z]).{6,15}$/
})}
/>
</Form.Field>
{errors.password &&
<p>Please Enter a Stronger Password</p>
}
<Button type='submit'>Get Started</Button>
</Form>
</div>
)
}
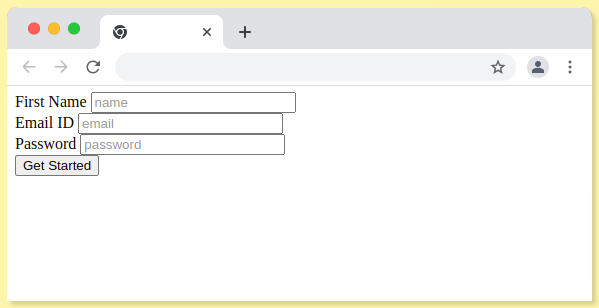
Why React Hook Form is better than similar alternatives available?
Although React offers multiple libraries to assist developers in crafting interactive forms react hook form is one of the majorly adapted options. Here’s how the react hook form library stands apart from the rest of the available alternatives:
- React-hook-form helps developers to create and maintain a cleaner codebase as compared to alternatives such as Formix and Redux-forms.
- React-Hook-Form allows developers to combine their validation schemas by integrating with the
yup
library. - The number of re-renders and mounting time is less compared to similar alternatives which makes react hook form more performant.
To Wrap Up
From the in-depth guide and discussion we had above, we can conclude that the right use of react hook form libraries can help developers move past development roadblocks.
Integrating react hook form can help React developers answer some of the common challenges of core React such as expanding codebase, redundancy, and integration complexity.
Since the library is solely focused on enhancing the overall development experience, it makes the overall development process a fun journey as well.
So if you’re looking for a way out of pesky code expansion in your React form along with removing validation complexity try out the react hook form for your projects right away.
Atatus Real User Monitoring
Atatus is a scalable end-user experience monitoring system that allows you to see which areas of your website are underperforming and affecting your users. Understand the causes of your React app performance issues and how to improve the user experience.
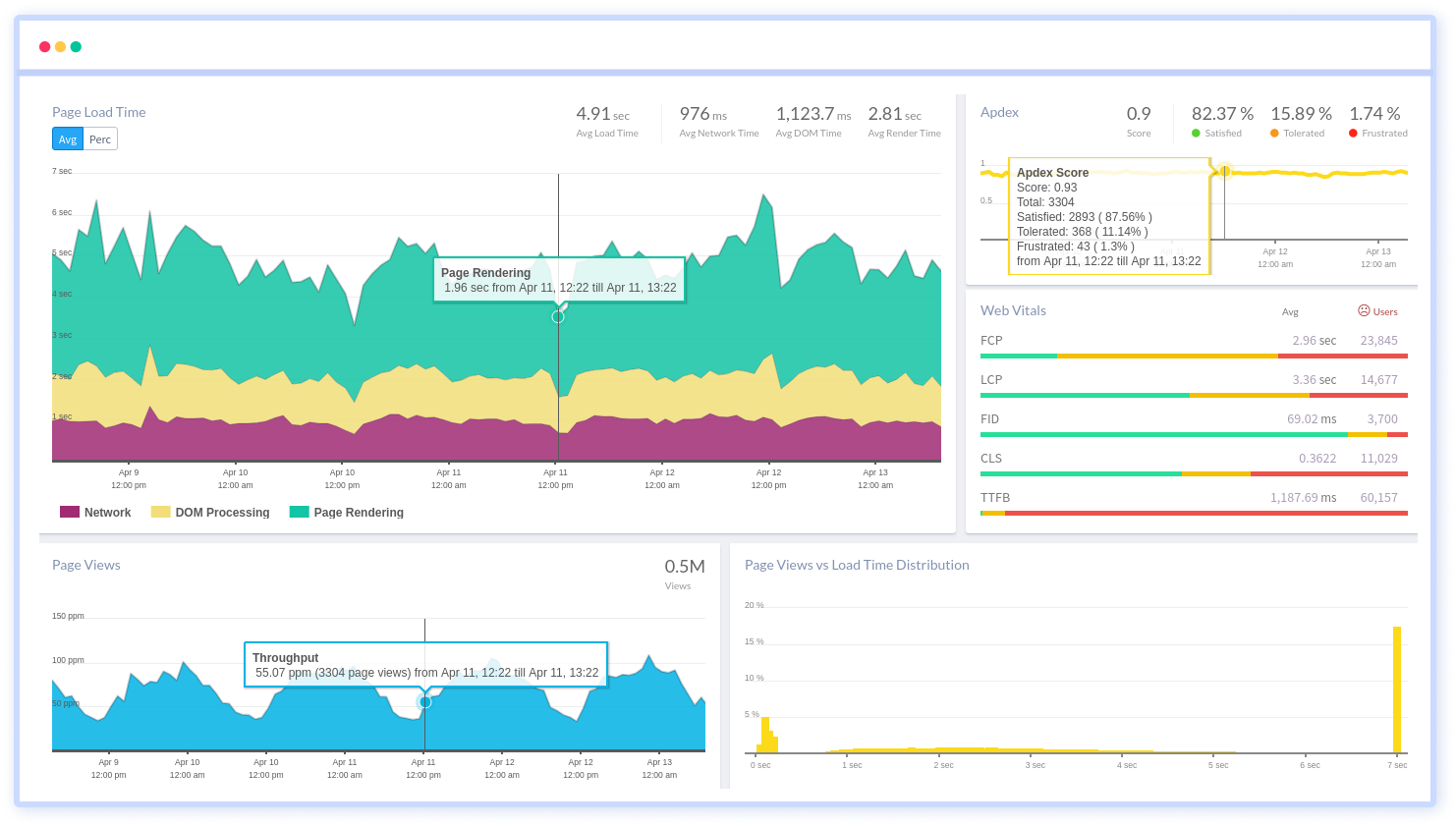
By understanding the complicated frontend performance issues that develop due to slow page loads, route modifications, delayed static assets, poor XMLHttpRequest, JS errors, and more, you can discover and fix poor end-user performance of your React app with React End User Monitoring.
You can get a detailed view of each page-load event to quickly detect and fix frontend performance issues affecting actual users. With filterable data by URL, connection type, device, country, and more, you examine a detailed complete resource waterfall view to see which assets are slowing down your pages.
#1 Solution for Logs, Traces & Metrics
APM
Kubernetes
Logs
Synthetics
RUM
Serverless
Security
More