Integrating Bootstrap with React - Beginner's Guide
Responsive and robust web apps have become an unsaid necessity for modern businesses. For this, the use of the traditional tech stack of JS, HTML, and CSS in the website frontend is not enough.
Instead, developers must shift to more modern and robust development alternatives to create web applications that answer complex business needs while guaranteeing effortless performance.
A majority of developers are using React with Bootstrap to achieve this goal in their web development projects. If you’re wondering how you can craft robust and responsive web apps, this blog is just the right place to begin.
In this blog, we will discuss how you can use React and Bootstrap effectively to create robust and mobile-friendly web apps for your projects.
Table of contents
Why use React with Bootstrap?
React is one of the most popular JS libraries for front-end development. It assists developers in crafting robust websites and web apps using reusable frontend components and a wide variety of frontend libraries.
Bootstrap is a well-known CSS library and can assist developers in crafting unique responsive websites at a rapid pace effectively.
By combining the usability of React with Bootstrap’s capability, developers can easily create responsive websites using React.
It helps frontend developers in moving beyond the constraints of CSS such as explosive codebase, redundancy, etc.
How to integrate Bootstrap with React?
Now that you know how Bootstrap can fastrack the React-based development process, let’s proceed to integrate React & Bootstrap into your projects. You can achieve this in three major ways, as discussed below:
- With Bootstrap CDN
- Using Bootstrap as a React dependency
- With React-Bootstrap packages such as react-strap and react-bootstrap
a.) Integrate with Bootstrap CDN
Integrating Bootstrap with the help of Bootstrap CDN is one of the fastest ways to use Bootstrap within your React-based web development projects effectively. As with CDN, you can avoid downloading external React dependencies and get started with using Bootstrap within your projects instantly. In order to effectively integrate Bootstrap you can follow the steps below:
Step 1: Supposed you created your React app using create-react-app
, we’ll just need to include the Bootstrap’s current version’s link given below to the CDN in the head section within public/index.html
<link rel="stylesheet" href="https://cdn.jsdelivr.net/npm/bootstrap@5.1.3/dist/css/bootstrap.min.css" integrity="sha384-1BmE4kWBq78iYhFldvKuhfTAU6auU8tT94WrHftjDbrCEXSU1oBoqyl2QvZ6jIW3" crossorigin="anonymous">
Step 2: To ensure you use Bootstrap in your React project effortlessly you’ll need Javascript components that ship with bootstrap. For this, we’ll link to the bootstrap.bundle.min.js
as described below within the script tag and place it just before the end of the body tag:
<script src="https://cdn.jsdelivr.net/npm/bootstrap@5.1.3/dist/js/bootstrap.bundle.min.js" integrity="sha384-ka7Sk0Gln4gmtz2MlQnikT1wXgYsOg+OMhuP+IlRH9sENBO0LRn5q+8nbTov4+1p" crossorigin="anonymous"></script>
Step 3: After placing the links in the public/index.html file, the complete codebase will look like the example shown below, and you’ll be ready to use Bootstrap functionality.
<!DOCTYPE html>
<html>
<head>
<meta charset="utf-8" />
<link rel="icon" href="%PUBLIC_URL%/favicon.ico" />
<meta name="viewport" content="width=device-width, initial-scale=1" />
<meta name="theme-color" content="#000000" />
<meta
name="Details"
content="Its a site crafted with create-react-app"
/>
<link rel="apple-touch-icon" href="%public_url%/logo192.png" />
<link rel="manifest" href="%public_url%/manifest.json" />
<title>React App</title>
<link
rel="stylesheet"
href="https://cdn.jsdelivr.net/npm/bootstrap@5.1.3/dist/css/bootstrap.min.css"
integrity="sha384-1BmE4kWBq78iYhFldvKuhfTAU6auU8tT94WrHftjDbrCEXSU1oBoqyl2QvZ6jIW3"
crossorigin="anonymous"
/>
</head>
<body>
<noscript>You need to enable JavaScript to run this app.</noscript>
<div id="root"></div>
<script
src="https://cdn.jsdelivr.net/npm/bootstrap@5.1.3/dist/js/bootstrap.bundle.min.js"
integrity="sha384-ka7Sk0Gln4gmtz2MlQnikT1wXgYsOg+OMhuP+IlRH9sENBO0LRn5q+8nbTov4+1p"
crossorigin="anonymous"
></script>
</body>
</html>
b.) Integrating BootStrap as React Dependency
For developers using a build tool or module bundlers such as webpack to create their React project, using React dependency in order to integrate bootstrap would be the easiest way to get started. To integrate Bootstrap as a React dependency, you can follow the steps mentioned below:
Step 1: To begin the dependency installation, you can run the command mentioned below in your React app to install the latest version of Bootstrap efficiently:
npm install bootstrap
Step 2: After Bootstrap dependency is installed, we can include Bootstrap CSS and JS components in our app entry package as shown below:
// Acess Bootstrap CSS with the link below
import "bootstrap/dist/css/bootstrap.min.css";
// Acess Bootstrap Bundle JS with the link below
import "bootstrap/dist/js/bootstrap.bundle.min";
Step 3: If you have used create-react-app to build your project, to install Bootstrap as a react dependency, go to the src/index.js file to import bootstrap. It should look something similar to the example given below:
import React from "react";
import ReactDOM from "react-dom/client";
// Bootstrap CSS
import "bootstrap/dist/css/bootstrap.min.css";
// Bootstrap Bundle JS
import "bootstrap/dist/js/bootstrap.bundle.min";
import "./index.css";
import App from "./App";
import reportWebVitals from "./reportWebVitals";
const root = ReactDOM.createRoot(document.getElementById("root"));
root.render(
<React.StrictMode>
<App />
</React.StrictMode>
);
reportWebVitals();
Once the installation is complete, you can easily use Bootstrap to craft efficient and unique React-based projects.
c.) Integrate using Bootstrap packages such as react-bootstrap and reactstrap
The react-bootstrap and reactstrap assist developers to use Bootstrap in the form of React components in their projects. One of the biggest perks of using such packages is that it comes with all the Bootstrap elements bundled as react components, making them much easier to use for users.
It further allows developers to directly use Bootstrap on react components and elements of your class. To understand how the bootstrap packages work directly on React elements, let us try to create a react app using each package.
i.) React App using React-Bootstrap package
To begin using react-bootstrap, we’ll start with importing the package with the command mentioned below:
npm install react-bootstrap bootstrap
Once the installation is complete, you’re ready to use the react-bootstrap package. Now let’s try to create a simple react app that uses the react-bootstrap component by using the codebase below:
import React, { useState } from "react";
import Jumbotron from "react-bootstrap/Jumbotron";
import Toast from "react-bootstrap/Toast";
import Container from "react-bootstrap/Container";
import Button from "react-bootstrap/Button";
import "./App.css";
const ExampleToast = ({ children }) => {
const [show, toggleShow] = useState(true);
return (
<>
{!show && <Button onClick={() => toggleShow(true)}>Show Toast</Button>}
<Toast show={show} onClose={() => toggleShow(false)}>
<Toast.Header>
<strong className="mr-auto">React-Bootstrap</strong>
</Toast.Header>
<Toast.Body>{children}</Toast.Body>
</Toast>
</>
);
};
const App = () => (
<Container className="p-3">
<Jumbotron>
<h2 className="header">This is the work of React Bootstrap</h2>
<ExampleToast>
Congrats Now You know How To use React-Bootstrap package{" "}
<span role="img" aria-label="tada">
🎉
</span>
</ExampleToast>
</Jumbotron>
</Container>
);
export default App;
From the above example, we can conclude that developers can easily import necessary Bootstrap components and use them with React elements.
ii.) React-App Using Reactstrap
The reactstrap is another react package that you can use to integrate bootstrap within your React application. However, before we start using reactstrap, it is essential to keep in mind that the package itself does not include Bootstrap CSS. Hence they should be installed separately.
To install reactstrap, use the command below:
npm install --save bootstrap
npm install --save reactstrap react react-dom
To further include Bootstrap CSS, you’ll have to import it by using the following command:
import 'bootstrap/dist/css/bootstrap.min.css';
After the installation, you’re ready to use reactstrap components effectively in your react projects. Let’s try out using reactstrap with the following examples:
import React from "react";
import { Button } from "reactstrap";
function App() {
return (
<div className="App">
<Button color="primary">primary</Button>{" "}
<Button color="secondary">secondary</Button>{" "}
<Button color="success">success</Button>{" "}
<Button color="info">info</Button>{" "}
<Button color="warning">warning</Button>{" "}
<Button color="danger">danger</Button> <Button color="link">link</Button>
</div>
);
}
export default App;
Final Words On Implementing React With Bootstrap
With the tutorial above you can now easily use Bootstrap components with React for creating a unique application frontend for your projects.
Be it by using CDNs, React Dependencies, or premade components, by including bootstrap components with React, you can easily enhance the quality of your web apps with ease.
However, while choosing the method to include Bootstrap, always be aware of your unique development needs and individual capabilities.
For example, if you're in-hand with bootstrap using CDN would be a good choice. However, for faster development using React packages as mentioned above is essential.
So, get started with using Bootstrap right away with your choice of methods using our tutorial and create interactive and user-friendly React applications for your clients effortlessly.
React Performance Monitoring with Atatus
Visualize React errors and performance issues influencing your end-user experience. With React performance monitoring of Atatus you can identify and resolve problems faster with in-depth data points that assist you in analyzing and resolving them.
Using Atatus, you can get an overview of how your customers experience your React app. Learn how to identify the root cause of a slow load time, route change, and more on the front end by identifying performance bottlenecks in the front end.
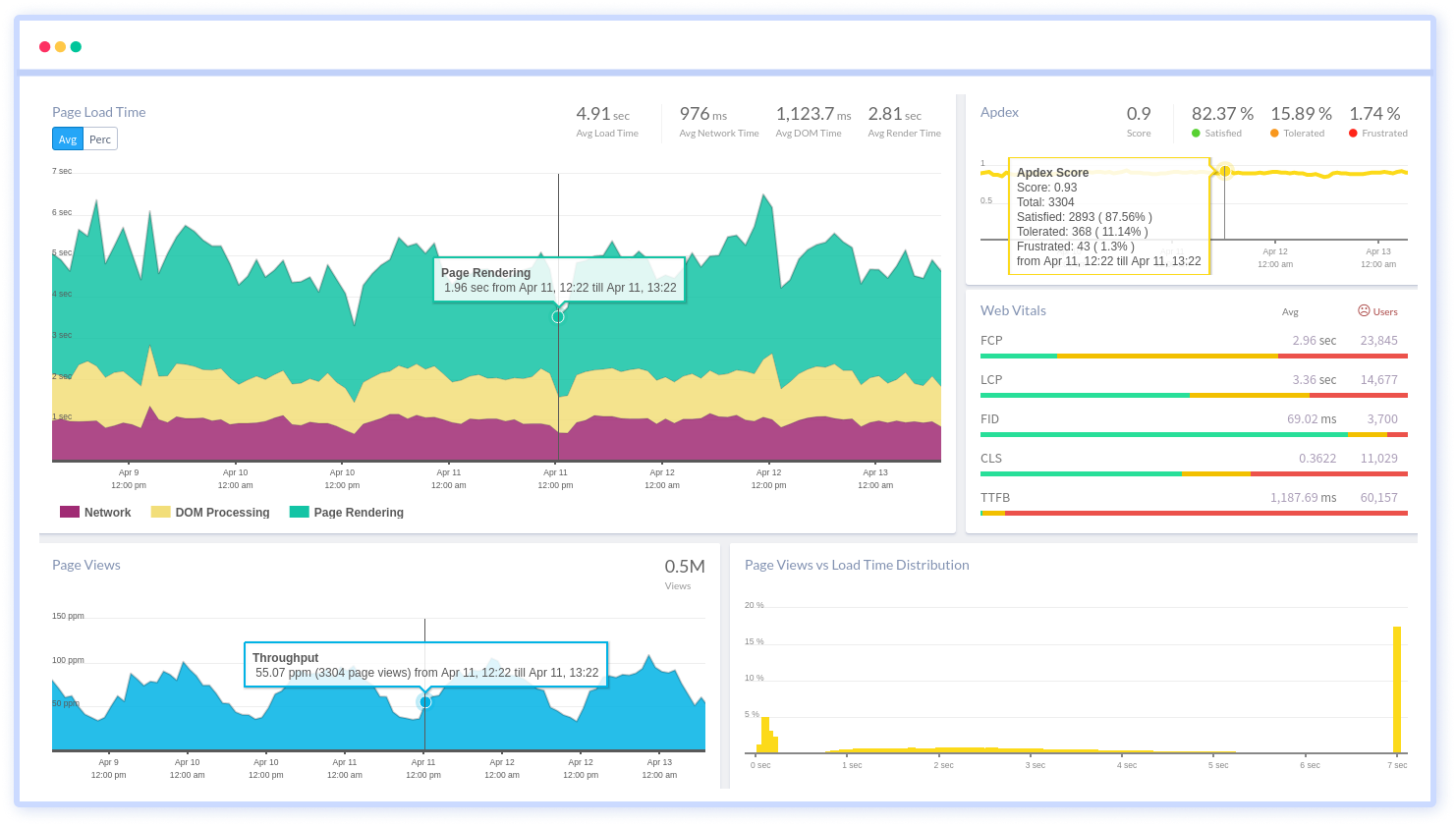
Ensure that your React app sends all XHR requests. Monitoring and measuring the response times and failure rates of XHR calls. Optimize AJAX request performance by identifying slow and failed calls. Analyze AJAX calls in real time based on the browser, the user, the version, the tag, and other attributes.
Identify the reasons behind bad front-end performance and slow page loading that are impacting your customers. Inspect individual users who are experiencing poor performance because of slow pages, React exceptions or a failing AJAX method.
Identify how your end users' experience is impacted by the network or geography. In addition to identifying slow React assets, long load times, and errors in sessions, session traces provide a waterfall view of the session.
Take a closer look at your React app's performance with Atatus. To get started activate Atatus free-trial for 14 days!
#1 Solution for Logs, Traces & Metrics
APM
Kubernetes
Logs
Synthetics
RUM
Serverless
Security
More