A Deep Dive Into PHP 8.1 New Feature
We all know the updated features in PHP 8 that was released a few months earlier. Now there is a new set of features expected to release on 25 November 2021. It is one of the most used programming languages nowadays. With the recent updates in the 8th version, the usage of PHP language has become increased.
Developers at PHP are continuously working to improvise the features. The team has recently announced the list of features that are going to be released in the upcoming version of PHP.
Scroll down the article to check the latest features to be released.
New Features in PHP 8.1
They have decided to introduce some exciting features which would make PHP a more popular language in the market.
- Enum
- Fibers
- String-keyed array unpack
- Readonly properties
- Final class constants
- Intersection Types
- Never return type
- fsync and fdatasync functions
- array_is_list function
- Explicit octal numeral notation
Let us take a deep look with it usage about the new features.
#1 Enum
Enum is a user-defined type with a set of named constants called enumerators which is mainly used to assigning names to integral constants.
With enums, it will be easy to read and maintain the entire program. The variables should be equal to one of the predefined values such as days in a week(sunday, monday etc..), months(January, February etc..), cards(Heart, Diamond, etc..)
Example:
enum Day {
case SUNDAY;
case MONDAY;
case TUESDAY;
case WEDNESDAY;
case THURSDAY;
case FRIDAY;
case SATURDAY;
}
Usage:
class Post
{
public function __construct(
private Day $day = Day::MONDAY;
) {}
public function setDay(Day $day): void
{
echo "Today is Monday"
}
}
$post->setDay(Day::Active);
Enums are declared with the keyword enum which is more similar to classes and interfaces. You can use enums when you have a variable with small set of possible values.
#2 Fibers
Fibres are more similar to green or virtual threads which are used to manage parallelism. It maintains variables and state which is more like its own stack that can be started, suspended or terminated cooperatively.
Operating system schedules thread but it does not guarantee that when and at which point the threads should resume or pause.
Fiber can simply suspend the code block by the program itself which returns any data back to the main program. From the point it was suspended the main program can resume the fiber.
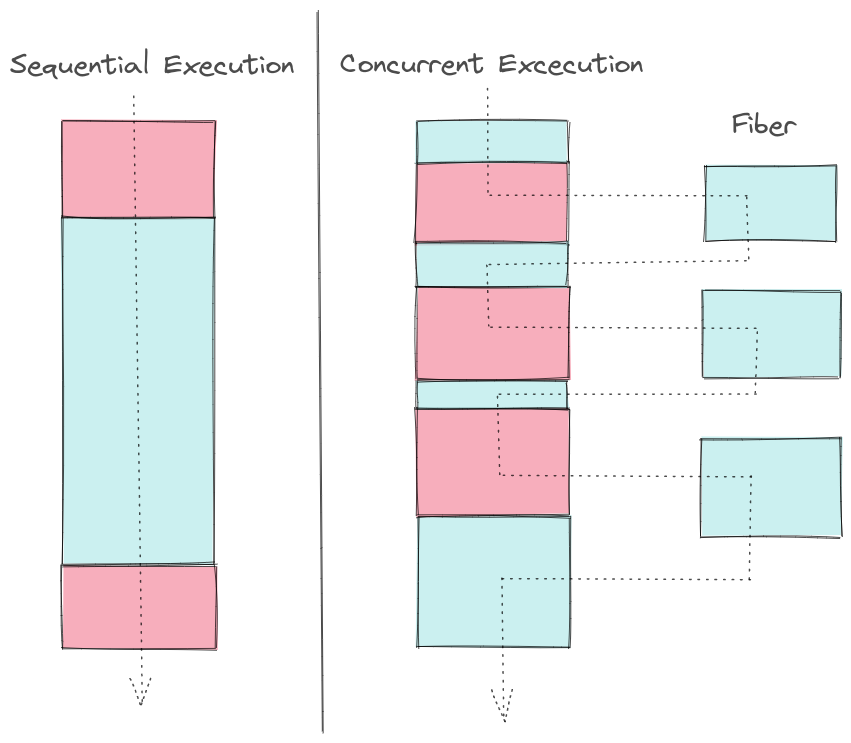
The main execution flow and the fiber execution does not happen at the same time. The main execution flow starts the fiber and the fiber does it exclusively once it is started. The main thread's task is to start the fiber, it cannot observe, terminate or suspend the fiber's flow while it is executed. The fiber can resume by itself but i cannot restart again, only the main thread can start the fiber once it is resumed. Simultaneous execution of multiple fibers is not allowed.
Example of using Fibres
$fiber = new Fiber(function(): void {
echo "Hello this is Fiber...\n";
Fiber::suspend();
echo "Fiber...\n";
});
echo "Starting Fiber program...\n";
$fiber->start();
echo "Taken back the control...\n";
echo "Resuming Fiber...\n";
$fiber->resume();
echo "Program exits...\n";
Fiber Class
<?php
final class Fiber
{
/**
* @param callable $callback Function to invoke
* when starting the fiber.
*/
public function __construct(callable $callback) {}
/**
* Starts execution of the fiber.
* Returns when the fiber suspends or terminates.
*
* @param mixed ...$args Arguments passed to
* fiber function.
*
* @return mixed Value from the first suspension
* point or NULL if the fiber returns.
*
* @throw FiberError If the fiber has already
* been started.
* @throw Throwable If the fiber callable throws
* an uncaught exception.
*/
public function start(mixed ...$args): mixed {}
/**
* Suspend execution of the fiber. The fiber may be
* resumed with {@see Fiber::resume()} or
* {@see Fiber::throw()}.
*
* Cannot be called from {main}.
*
* @param mixed $value Value to return from
* {@see Fiber::resume()} or {@see Fiber::throw()}.
*
* @return mixed Value provided to
* {@see Fiber::resume()}.
*
* @throws FiberError Thrown if not within a
* fiber (i.e., if called from {main}).
* @throws Throwable Exception provided to
* {@see Fiber::throw()}.
*/
public static function suspend(mixed $value = null): mixed {}
/**
* Resumes the fiber, returning the given value
* from {@see Fiber::suspend()}.
* Returns when the fiber suspends or terminates.
*
* @param mixed $value
*
* @return mixed Value from the next suspension
* point or NULL if the fiber returns.
*
* @throw FiberError If the fiber has not
* started, is running, or has terminated.
* @throw Throwable If the fiber callable throws
* an uncaught exception.
*/
public function resume(mixed $value = null): mixed {}
/**
* @return self|null Returns the currently executing
* fiber instance or NULL if in {main}.
*/
public static function getCurrent(): ?self {}
/**
* @return mixed Return value of the fiber
* callback. NULL is returned if the fiber does
* not have a return statement.
*
* @throws FiberError If the fiber has not terminated
* or the fiber threw an exception.
*/
public function getReturn(): mixed {}
/**
* Throws the given exception into the fiber
* from {@see Fiber::suspend()}.
* Returns when the fiber suspends or terminates.
*
* @param Throwable $exception
*
* @return mixed Value from the next suspension
* point or NULL if the fiber returns.
*
* @throw FiberError If the fiber has not started,
* is running, or has terminated.
* @throw Throwable If the fiber callable throws
* an uncaught exception.
*/
public function throw(Throwable $exception): mixed {}
/**
* @return bool True if the fiber has been started.
*/
public function isStarted(): bool {}
/**
* @return bool True if the fiber is suspended.
*/
public function isSuspended(): bool {}
/**
* @return bool True if the fiber is currently running.
*/
public function isRunning(): bool {}
/**
* @return bool True if the fiber has completed
* execution (returned or threw).
*/
public function isTerminated(): bool {}
}
Fiber Class Methods:
Here are the list of fiber class methods.
Fiber::__construct
Fiber::start
Fiber::suspend
Fiber::resume
Fiber::getCurrent
Fiber::getReturn
Fiber::throw
Fiber::isStarted
Fiber::isSuspended
Fiber::isRunning
Fiber::isTerminated
#3 String-keyed array unpack
PHP 7.4 has array unpacking using array spread operator(...), but this supported only numeric values. Since there is no consent on how to merge array duplicates in string they weren't supported in the older versions.
You can unpack the array using another array with the help of the spread operator.
Example:
$array_1 = ['mon', 'tue'];
$array_2 = ['wed', 'thurs'];
$unpacked_array = [...$array_1, ...$array_2, ...['fri']];
$merged_array = array_merge($array_1, $array_2, ['fri']);
var_dump($array_unpacked);
// ['mon', 'tue', 'wed', 'thurs', 'fri'];
var_dump($array_merged);
// ['mon', 'tue', 'wed', 'thurs', 'fri'];
array_merge() function combines all the passed values in the given order. The duplicate keys will get overwritten with the later values.
When u pass a string key to unpack it will result in error.
$array = [...['a' => 'mon'], ...['b' => 'tue']];
Fatal error: Cannot unpack array with string keys in ... on line ...
But now with PHP 8.1 it not only supports numericals but also supports string keys.
Example:
$array = [...['a' => 'mon'], ...['b' => 'tue']];
// ['a' => 'mon', 'b' => 'tue'];
Array unpacking and the array merge functions are similar to each other since they ignore duplicates and combines array in the given order.
#4 Readonly Properties
A read-only property will be supported in PHP 8.1 in which a class property can be initialized only once in which you cannot make any further modifications.
It can be declared only with the keyword readonly
in a typed property.
class Employee {
public readonly int $eid;
public function __construct(int $eid) {
$this->eid = $eid;
}
}
$employee = new Employee(2);
#5 final class constants
Final keyword helps you in preventing the child classes from overriding a method. Classes and methods can only be declared as final whereas properties and constants cannot be declared using the final keyword.
class vehicle
{
final public const X = "car";
}
class swift extends vehicle
{
public const X = "swift";
}
#6 Intersection Types
Intersection type is a bit similar to union types in PHP 8.0 but in union types the input should be one of the declared types, here in intersection types it can be any of the declared type.
function generateSlug(HasTitle&HasId $post) {
returnstrtolower($post->getTitle()) . $post->getId();
}
#7 Never Return Type
It is a new return type which is a function or method that can be declared using the keyword never. This will not return any value, either it will throw an exception or terminates with a die/exit call.
function abc(mixed $input): never
{
// dump
exit;
}
It is similar to the void return type but the small difference between these both are: the never return type terminates or throws an exception. This function helps you to stop the program flow which can be done using the exit() method.
#8 fsync and fdatasync Functions
These are similar to fflush function which helps in flushing the buffered data to the operating system. fsync and fdatasync function not only flushes data in the operating system but also requests the operating system to write buffers in the actual storage media.
- fsync: helps you to synchronize changes to the file along with the metadata. Metadata includes created and updated date, filename, author’s name and so on.
- fdatasync: helps you to synchronize data to the file without metadata.
Usage Example:
$file = fopen("abc.txt", "w");
fwrite($file, "Hello Sara!!!");
if (fsync($file)) {
echo "File has been successfully persisted to disk.";
}
fclose($file);
For better understanding let me explain with an example:
Think of you are copying bulk amount of data to an USB drive, here the OS will put your files in some internal buffer and then it physically copies your file to your USB drive.
The fsync and fdatasync functions helps you in copying the file quicker by processing the packets of data instead of bit by bit.
#9 array_is_list function Function
The array_is_list() function returns whether the given array is an array with all the successive integers starting from 0.
It also returns true for the following conditions if the given array is:
- semantic list of values
- all the keys are integers
- keys starting from 0
- with no gaps in between
- additionally on empty arrays
Usage:
array_is_list([]); // true
array_is_list([1, 2, 3]); // true
array_is_list(['sara', 2, 3]); // true
array_is_list(['sara', 'regi']); // true
array_is_list([0 => 'sara', 'regi']); // true
array_is_list([0 => 'sara', 1 => 'regi']); // true
The array_is_list() returns true for all of the above conditions.
// Key does not start with 0
array_is_list([1 => 'apple', 'orange']); // false
// Keys are not in order
array_is_list([1 => 'apple', 0 => 'orange']); // false
// Non-integer keys
array_is_list([0 => 'apple', 'fruit' => 'orange']); false
// Non-sequential keys
array_is_list([0 => 'apple', 2 => 'orange']); false
It returns false for the conditions where an array with keys not starting from 0 and any array in which the keys do not have integers in sequential order.
The array_is_list() only allows array as an parameter and it throws an TypeError exception if any other datatype is passed as an parameter. It is declared in the global namespace.
#10 Explicit octal numeral notation
In general, PHP supports various numeral systems such as decimal, integers and so on along with the default decimal (base-10), binary (base-2), octal (base-8), and hex (base-16).
In the new version PHP 8.1 it supports octal numeral with the 0o (zero, followed by o as in Oscar) prefix that is octal numeric literals are made readable. It also supports 0O (zero followed by uppercase O).
Usage:
echo 0xff; // 255
echo 0b111; // 7
echo 77; // 77
echo 077; // 63
echo 0o77; // 63, In PHP 8.1
echo 0O77; // 63, In PHP 8.1
Start noticing your PHP errors in your application with Atatus
Track and measure all the performance bottlenecks using PHP Monitoring and resolve the issues in your PHP application. You can find out the PHP errors all at one place with which you can easily solve the errors.
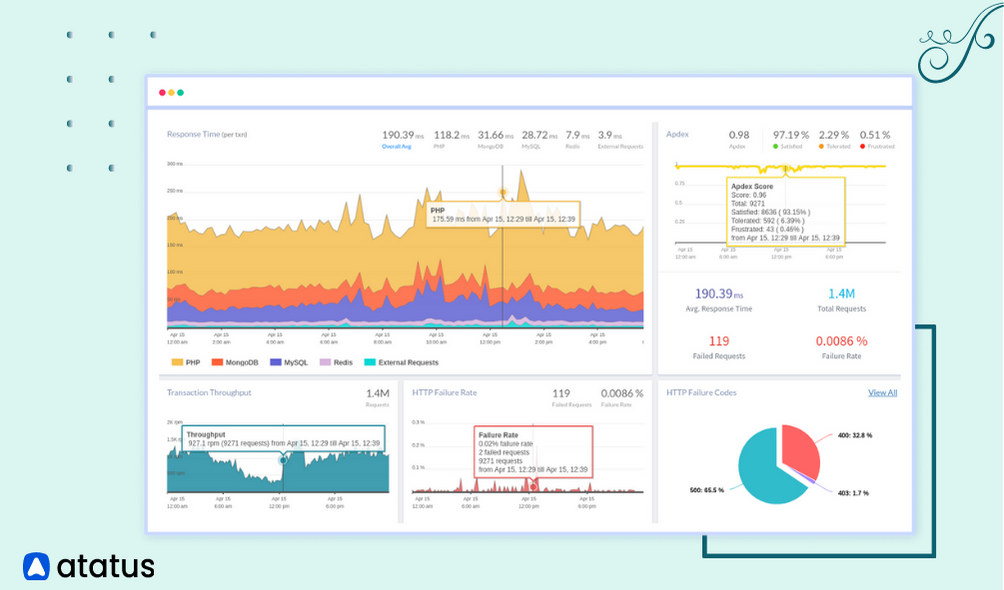
With the features listed below you can easily track all the PHP errors and fix the issues at the right time.
- Transaction Monitoring
- Database Monitoring
- External Requests
- Transaction Traces
- API Failures
- Error Tracking
Start your free trial with Atatus. No credit card required.
Share your thoughts about the new features that you might know in PHP 8.1 with us in the below comment section.
#1 Solution for Logs, Traces & Metrics
APM
Kubernetes
Logs
Synthetics
RUM
Serverless
Security
More