"Many hands make light work" applies well to the concept of parallel processing. It means that a task becomes easier and quicker when many people work together towards a common goal.
In the context of computing, parallel processing refers to the ability to divide a task into smaller parts and distribute them across multiple processors or cores to complete the task more efficiently.
Parallel processing involves breaking down a complex task into simpler ones, which can be executed in parallel. This approach can lead to significant improvements in the speed and efficiency of a program, especially when dealing with large amounts of data or complex computations.
In this blog, we will dive deep into the concept of parallel processing, understand its benefits and limitations, and learn about the tools and techniques available for running PHP code in parallel.
Table of Content
- What is Parallel Processing in PHP?
- How Does it Work?
- Tools for Running PHP Code in Parallel
- PHP Parallel Processing Use Cases
- Common Pitfalls and How to Avoid Them
What is Parallel Processing in PHP?
Parallel processing in PHP refers to the ability of executing multiple tasks simultaneously, instead of sequentially, on a single processor or multiple processors. In simple terms, it means dividing a larger task into smaller, independent tasks that can be executed concurrently to increase the overall speed and efficiency of the program.
Let's take an example of a web application that needs to fetch data from multiple APIs before it can generate a response for the user. Without parallel processing, the application would have to wait for the response from one API before moving on to the next API, which could result in a significant delay in response time.
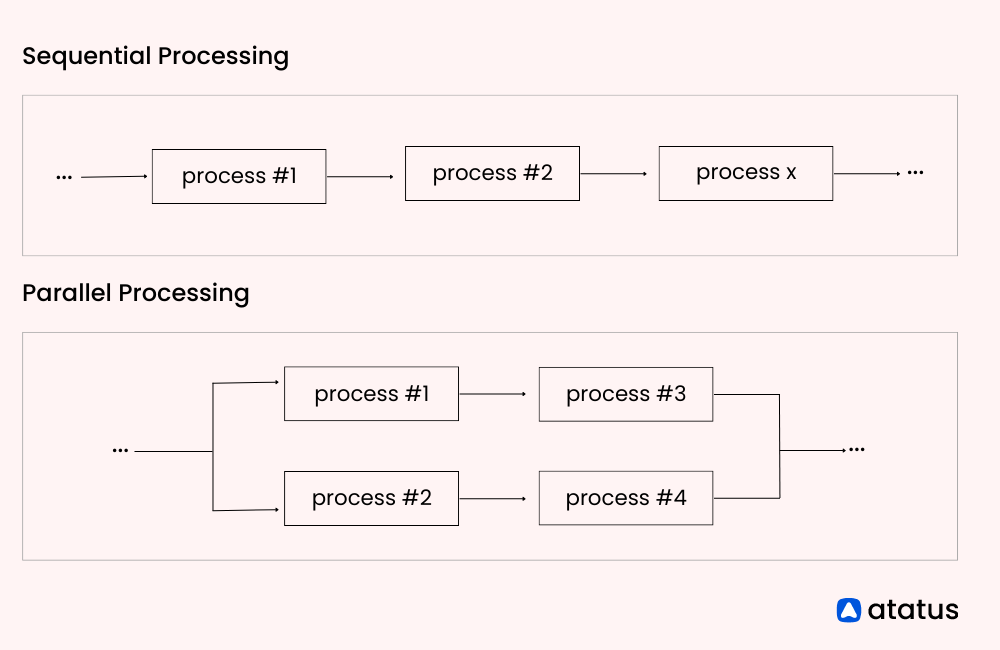
However, by using parallel processing, the application can make requests to all the APIs simultaneously, reducing the overall response time and improving the user experience.
How does it Work?
The process of parallel processing can be broken down into the following steps:
- Dividing the task: The first step is to identify the task that needs to be executed in parallel and then break it down into smaller, independent sub-tasks that can be executed concurrently.
- Assigning the sub-tasks: The next step is to assign the sub-tasks to individual processors or threads. This can be done using various techniques, such as round-robin, where each sub-task is assigned to a different processor or thread in a round-robin fashion.
- Executing the sub-tasks: Once the sub-tasks have been assigned, they can be executed concurrently on individual processors or threads. Each processor or thread will execute its assigned sub-task independently of the others.
- Synchronizing the results: Finally, once all the sub-tasks have been executed, their results need to be combined to produce the final output. This can be done using various synchronization techniques, such as merging the results, or using a reduction operation to combine the results.
Parallel processing is a technique that allows for the simultaneous execution of multiple sub-tasks, resulting in faster and more efficient processing of large tasks. By breaking down a large task into smaller, independent sub-tasks and executing them concurrently, parallel processing can significantly improve the performance of PHP applications.
Tools for Running PHP Code in Parallel
Running PHP code in parallel refers to the ability to execute multiple PHP processes simultaneously, which can significantly improve the performance and efficiency of your applications. Parallel processing is especially beneficial for tasks that involve heavy computation, I/O operations, or handling multiple concurrent requests.
There are several tools and techniques available for running PHP code in parallel. These tools generally aim to leverage multi-threading, multi-processing, or asynchronous programming to achieve parallel execution.
Here are some interesting facts about parallel processing in PHP:
- Multi-threading: Traditional PHP implementations like PHP-FPM are not inherently designed for multi-threading due to the way the language is structured. However, you can still achieve parallelism by using extensions like pthreads or parallel, which provide thread management functionality in PHP.
- Multi-processing: PHP has built-in support for multi-processing through extensions such as pcntl and POSIX, which allow you to fork multiple child processes from a parent process. Each child process can then execute PHP code independently, providing parallelism.
- Asynchronous programming: Asynchronous programming enables parallel execution by allowing tasks to run concurrently without blocking the main thread. Libraries like ReactPHP, Swoole, and Amp provide asynchronous event-driven architectures and non-blocking I/O operations, making it easier to write PHP code that runs in parallel.
Now let us discuss the top five tools commonly used for running PHP code in parallel:
1. Swoole
Swoole is a high-performance coroutine-based networking framework for PHP. It offers event-driven architecture, asynchronous I/O, timers and coroutines to achieve parallel execution. It supports both multi-threading and multi-processing, making it suitable for various use cases.
Swoole is commonly used for developing web servers, APIs, WebSocket servers, and other networked applications.
Here's a basic example of using Swoole in PHP to create a simple HTTP server:
<?php
// Create an HTTP server using Swoole
$server = new Swoole\Http\Server("127.0.0.1", 8000);
// Handle incoming requests
$server->on('request', function ($request, $response) {
// Set the response header
$response->header('Content-Type', 'text/plain');
// Send the response body
$response->end('Hello, Swoole!');
});
// Start the server
$server->start();
2. ReactPHP
ReactPHP is an event-driven, non-blocking I/O framework for PHP. It enables writing asynchronous PHP code using event loops and promises. ReactPHP is particularly useful for building scalable network servers or handling concurrent HTTP requests.
It is widely used for building real-time applications, microservices, and other network-based solutions.
Here's a basic example of using ReactPHP in PHP to create a simple HTTP server:
<?php
require 'vendor/autoload.php';
use React\Http\Server;
use React\Http\Response;
use Psr\Http\Message\ServerRequestInterface;
$loop = React\EventLoop\Factory::create();
$server = new Server(function (ServerRequestInterface $request) {
return new Response(
200,
array('Content-Type' => 'text/plain'),
'Hello, ReactPHP!'
);
});
$socket = new React\Socket\Server('127.0.0.1:8000', $loop);
$server->listen($socket);
$loop->run();
3. Amp
Amp is another asynchronous concurrency library for PHP. It provides components like event loops, promises, and concurrency primitives to write non-blocking, parallel PHP code. Amp is known for its simplicity and ease of use.
Here's a basic example of using Amp in PHP to perform asynchronous operations:
<?php
require 'vendor/autoload.php';
use Amp\Loop;
use Amp\Promise;
use Amp\Success;
use Amp\Delayed;
function asyncOperation(): Promise
{
return new Success('Async operation completed');
}
Loop::run(function () {
$promise = asyncOperation();
$promise->onResolve(function ($exception, $result) {
if ($exception) {
echo 'Async operation failed: ' . $exception->getMessage() . PHP_EOL;
} else {
echo 'Async operation result: ' . $result . PHP_EOL;
}
});
yield new Delayed(1000);
});
4. Pthreads
Pthreads is a PHP extension that allows multi-threading in PHP. It provides an object-oriented API for creating and managing threads, sharing data between threads, and synchronizing their execution.
Pthreads enables parallel processing within a single PHP process. With Pthreads, developers can execute code concurrently, leveraging the power of multiple CPU cores.
Here's a basic example of using Pthreads in PHP to create and run a simple multithreaded application:
<?php
class MyThread extends Thread
{
public function run()
{
echo "Hello from thread " . $this->getThreadId() . "\n";
}
}
$thread1 = new MyThread();
$thread2 = new MyThread();
$thread1->start();
$thread2->start();
$thread1->join();
$thread2->join();
These extensions provide different approaches to parallel processing in PHP, allowing you to choose the one that best suits your requirements and programming style. It's important to carefully consider the specific needs of your application and evaluate the performance, scalability, and complexity trade-offs associated with each tool before making a decision.
PHP Parallel Processing Use Cases
Parallel processing in PHP can be beneficial in various scenarios where concurrent execution of tasks can significantly improve performance and efficiency. Here are some example use cases where parallel processing can be applied in PHP:
1. Image Processing
When dealing with image manipulation or processing a large number of images, parallel processing can be utilized to distribute the workload across multiple threads or processes. For example, resizing or applying filters to multiple images simultaneously can be done in parallel, reducing the overall processing time.
For example, imagine you have a PHP wizard that can resize, apply filters, and add special effects to images. However, the wizard can only process one image at a time, leading to a long waiting time for your users. How can you make the wizard work faster and handle multiple images simultaneously? Parallel processing to the rescue! By employing parallel techniques, you can run multiple copies of the wizard, each working on a different image, making the whole process enchantingly quick.
2. Data Processing and Analysis
PHP is commonly used for data processing tasks, such as parsing and analysing large data sets or logs. Parallel processing can accelerate these operations by dividing the data into smaller chunks and processing them concurrently. This approach can be particularly useful for tasks like data aggregation, statistical analysis, or generating reports.
For example, imagine you have a detective PHP script that analyzes a massive pile of data, searching for clues and patterns. However, the detective can only process one chunk of data at a time, leaving you waiting for hours or even days to crack the case. How can you speed up the investigation and make the detective work on multiple data chunks concurrently? Parallel processing to the rescue! By dividing the data into smaller pieces and assigning them to different detectives working in parallel, the investigation becomes a thrilling race against time.
3. Web Scraping and API Calls
When scraping websites or making multiple API requests, parallel processing can greatly enhance efficiency. By distributing the requests across multiple threads or processes, you can fetch data from multiple sources simultaneously, reducing the overall scraping or API response time.
Common Pitfalls and How to Avoid Them
When working with parallel processing in PHP, there are common pitfalls that developers may encounter. Being aware of these pitfalls and knowing how to avoid them can help ensure smooth and efficient implementation. Here are some common pitfalls and ways to avoid them:
1. Data Synchronization Issues
Parallel processing involves handling multiple tasks concurrently, which can lead to data synchronization issues. When multiple processes access and modify shared data simultaneously, conflicts and inconsistencies may arise.
How to Avoid: Implement proper synchronization techniques such as locks, semaphores, or mutexes to control access to shared data. Utilize synchronization mechanisms provided by PHP, such as the synchronized
keyword or use thread-safe data structures. Carefully design your code to minimize dependencies on shared data whenever possible.
2. Load Balancing Challenges
Dividing work among multiple processing units requires effective load balancing. Uneven distribution of tasks can result in some units being overwhelmed with work, while others remain idle.
How to Avoid: Implement dynamic load balancing algorithms that distribute tasks evenly across processing units. Monitor the workload and adjust task allocation dynamically based on processing power and availability. Utilize load balancing techniques provided by PHP frameworks or libraries, or implement custom load balancing logic tailored to your specific requirements.
3. Overhead and Resource Management
Parallel processing comes with additional overhead, such as thread or process creation, inter-process communication, and memory consumption. Inefficient resource management can lead to excessive resource usage and performance degradation.
How to Avoid: Optimize resource usage by minimizing unnecessary overhead. Use lightweight thread or process creation techniques, reuse resources where possible, and avoid excessive context switching. Monitor resource utilization and optimize memory management to avoid memory leaks or excessive memory consumption.
4. Limited Scalability
Parallel processing can hit scalability limitations, especially when working with a large number of concurrent tasks or when scaling across multiple machines.
How to Avoid: Employ distributed computing techniques such as task partitioning and workload distribution across multiple machines. Utilize distributed processing frameworks or technologies that support parallel execution across multiple nodes. Design your system with scalability in mind from the beginning, considering horizontal scaling options and efficient communication protocols.
5. Debugging and Testing Challenges
Debugging parallel code can be complex due to non-deterministic behaviour and interleaved execution. It can be challenging to reproduce and track down bugs in parallelized code. Additionally, testing parallel code for different scenarios can be demanding.
How to Avoid: Implement proper logging and debugging mechanisms to track the execution of parallel tasks. Use debugging tools and profilers specifically designed for parallel code. Write comprehensive unit tests that cover different parallel scenarios and ensure proper synchronization and behaviour of shared data.
Conclusion
Parallel processing in PHP is a transformative force that empowers developers to tackle complex tasks with speed, efficiency, and scalability. From image processing to data mining, web scraping to real-time analytics, parallel processing unlocks new possibilities and enhances the performance of PHP applications.
By harnessing the potential of parallel processing, developers can unleash a symphony of code, where multiple tasks harmoniously work together, accelerating the execution time and delivering impressive results. The power of parallel processing turns ordinary operations into extraordinary feats, enabling faster image editing, efficient data analysis, seamless web scraping, and real-time insights.
As the digital landscape evolves, parallel processing becomes increasingly crucial in meeting the demands of today's data-intensive and time-sensitive applications. It unleashes the true potential of PHP, enabling developers to push boundaries, unravel insights, and create robust, scalable solutions. So, unlock the potential of parallel processing and embark on a journey of limitless possibilities in the PHP realm.
Atatus: PHP Performance Monitoring and Error Tracking
Atatus is an Application Performance Management (APM) solution that collects all requests to your PHP applications without requiring you to change your source code. However, the tool does more than just keep track of your application's performance.
Atatus PHP monitoring, tracks and provides real-time notifications for every error, exception, and performance problem that occurs within the application. .
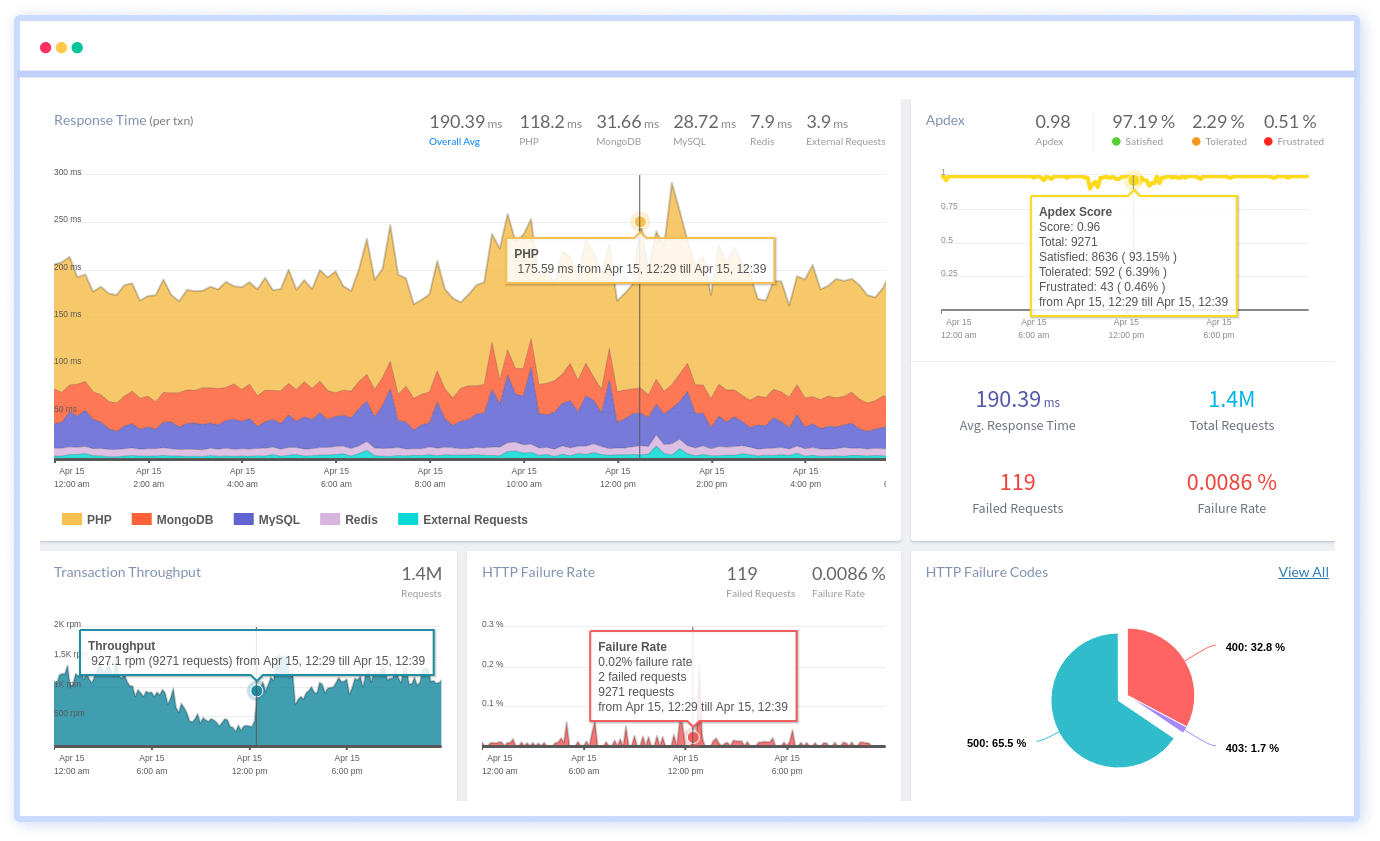
By tracking KPIs such as response times, throughput, HTTP failure rates, and error rates, it can help users identify and address any performance issues before they impact the application's users.
Furthermore, Atatus supports multiple PHP framework such as WordPress, Magento, Laravel, CodeIgniter, CakePHP, Symfony, Yii and Slim making it an adaptable tool that can be used to monitor applications across different frameworks and platforms.