A Beginner's Guide to PHP cURL Functions
cURL is a PHP library and a command-line tool that enables us to receive and transmit data utilizing URL syntax. It can transmit data in a wide range of forms, including HTTP posts, HTTP gets, FTP uploads and downloads, cookies, SOAP requests and responses, and so forth.
Engaging a web server with this PHP extension may entail the most appropriate data which can be retrieved in the webpage database. An increasing proportion of the data included on webpages is acquired support from external sources, extensively across a Web application.
The data imparted by other internet services can be easily managed with cURL. Programmers can test their API requests and quickly receive performance results using cURL's versatile tool. Leveraging cURL's embedded libraries, you can also gather data from many other sites.
cURL has intuitive support for SSL, security management, certificate authentication, and HTTP cookie. It can be installed concurrently as a terminal framework or as a dynamic library (libcurl) which can be embedded across other applications.
Table Of Contents
- The PHP cURL
- Working of PHP cURL() extension
- Basic PHP cURL Functions
- Instances for PHP cURL
- Multiple cURL requests in parallel
- Additional Functions of PHP's cURL
- Uses of PHP cURL function
The PHP cURL
Let's first look at what is cURL in PHP? The cURL library allows us to engage with other servers using a wide range of protocols. It empowers the user to transmit and receive data using URL syntax. cURL may further improve security, send and receive cookies, import data to websites, and evaluate troubleshooting.
cURL strives as 'Client for URLs,' with "URL" transcribed in upper case letters to highlight how it interfaces with URLs. The PHP cURL model includes two options: libcurl and cURL. libcURL is a library that programmers can incorporate for a wide range of applications. cURL is a command-line data transmission tool.
cURL is a command-line feature for fetching and transmitting data through URL syntax. Since cURL is primarily focused on libcurl, it currently supports a wide range of frequently identified protocols, such as HTTP, HTTPS, FTP, FTPS, GOPHER, TELNET, DICT, and FILE.
The cURL library is employed to collaborate with most other servers using a range of protocols. And since cURL is intended to operate without user interaction, it can be employed in scripts or even cron jobs.
libcurl is a basic client-side URL transfer library that offers TTPS certificates, HTTP POST, HTTP PUT, FTP uploading, Kerberos, HTTP-based upload, proxies, cookies, user and password authentication, HTTP proxy tunneling, and numerous other functionalities.
Working of PHP cURL() extension
In order to use PHP's cURL functions you need to install the libcurl package. cURL is enabled by default in PHP, but if not, you can enable it in your environment by following these steps:
- Open the PHP configuration file
php.ini
. - Look for the line
extension=php_curl.dll
. - Remove the semicolon (;) from the beginning of the line.
- Restart the Apache server.
To execute a basic PHP cURL request-response cycle, follow these steps:
i) Set up the cURL session
$curl = curl_init();
ii) The session can be configured with varied options
curl_setopt($handle, CURLOPT_URL, $url);
iii) Execution fetches/sends data from/to the server.
$response = curl_exec($handle);
iv) Terminate the session
curl_close($handle);
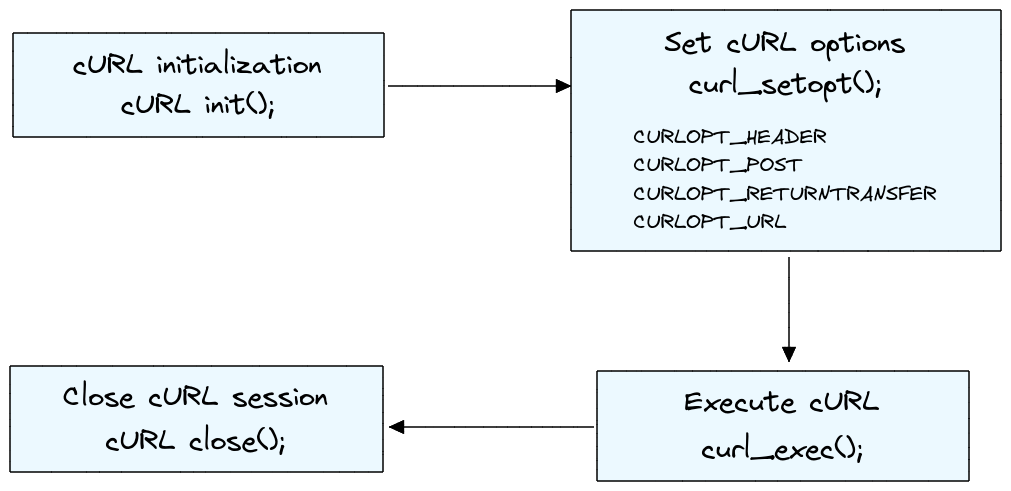
Here's a simple example of using cURL in PHP to make a GET request to a URL:
<?php
// Initialize cURL session
$curl = curl_init();
// Set the URL to be requested
curl_setopt($curl, CURLOPT_URL, "https://www.example.com");
// Return the response as a string instead of outputting it
curl_setopt($curl, CURLOPT_RETURNTRANSFER, true);
// Execute the cURL request and get the response
$response = curl_exec($curl);
// Check if the cURL request was successful
if ($response === false) {
echo "cURL Error: " . curl_error($curl);
} else {
// Print the response
echo $response;
}
// Close the cURL session
curl_close($curl);
?>
This example initializes a cURL session with curl_init()
, sets the URL to be requested with curl_setopt($curl, CURLOPT_URL, "https://www.example.com")
, tells cURL to return the response as a string with curl_setopt($curl, CURLOPT_RETURNTRANSFER, true)
, and executes the cURL request with curl_exec($curl)
.
The response is then checked for success, and if successful, printed. Finally, the cURL session is closed with curl_close($curl)
.
Basic PHP cURL Functions
Function | Description |
curl_init() | initiates a cURL session. |
curl_setopt() | specifies various options for cURL sessions. |
curl_setopt($ch, option, value) | Sets the value and option for a cURL session using the "ch" parameter. |
curl_exec() | It accomplishes the cURL session, the data transfer, and the http request. |
curl_close() | Using this function, cURL sessions can be closed. |
Instances for PHP cURL
Here are some examples of how to use cURL in PHP for various purposes:
1. Making a GET request in PHP cURL
To make a GET request using cURL in PHP, you can use the following code:
<?php
$url = "https://api.example.com/endpoint?key1=value1&key2=value2";
$ch = curl_init();
curl_setopt($ch, CURLOPT_URL, $url);
curl_setopt($ch, CURLOPT_RETURNTRANSFER, true);
$response = curl_exec($ch);
curl_close($ch);
echo $response;
?>
This code will make a GET request to the API endpoint located at $url
. The response from the API will be stored in the $response
variable and can be output using echo
.
2. Making a POST request using PHP cURL
Here's an example of using cURL in PHP to make a POST request with JSON data to an API endpoint:
<?php
$url = "https://api.example.com/endpoint";
$data = array("key1" => "value1", "key2" => "value2");
$ch = curl_init();
curl_setopt($ch, CURLOPT_URL, $url);
curl_setopt($ch, CURLOPT_POST, 1);
curl_setopt($ch, CURLOPT_POSTFIELDS, json_encode($data));
curl_setopt($ch, CURLOPT_HTTPHEADER, array('Content-Type: application/json'));
curl_setopt($ch, CURLOPT_RETURNTRANSFER, true);
$response = curl_exec($ch);
curl_close($ch);
echo $response;
?>
This code will make a POST request to the API endpoint located at $url
with the data $data
encoded as JSON. The CURLOPT_HTTPHEADER
option is set to specify the Content-Type
header as application/json
. The response from the API will be stored in the $response
variable.
3. Downloading a file from the internet using PHP cURL
To download a file from the internet using cURL in PHP, you can use the following code:
<?php
$url = "https://www.example.com/file.zip";
$file = "file.zip";
$ch = curl_init();
curl_setopt($ch, CURLOPT_URL, $url);
curl_setopt($ch, CURLOPT_RETURNTRANSFER, 1);
curl_setopt($ch, CURLOPT_SSL_VERIFYPEER, false);
$data = curl_exec($ch);
curl_close($ch);
$fp = fopen($file, "w");
fwrite($fp, $data);
fclose($fp);
?>
The above code will download the file located at $url
and save it to a file named $file
in the current directory. The CURLOPT_SSL_VERIFYPEER
option is set to false
to skip SSL verification, but in a production environment you may want to keep this enabled for security reasons.
4. Handling redirects (HTTP 301,302) using cURL in PHP
To handle redirects (HTTP 301 and 302 status codes) using cURL in PHP, you can set the CURLOPT_FOLLOWLOCATION
option to true
in your cURL request.
Here's an example:
<?php
$ch = curl_init();
curl_setopt($ch, CURLOPT_URL, "https://www.example.com");
curl_setopt($ch, CURLOPT_RETURNTRANSFER, 1);
curl_setopt($ch, CURLOPT_FOLLOWLOCATION, true);
$result = curl_exec($ch);
curl_close($ch);
echo $result;
?>
This will cause cURL to automatically follow any redirects and return the final content of the target URL.
5. Logging cURL Errors with PHP cURL to a File
To log cURL errors into a file using cURL in PHP, you can add the following code to your cURL request:
<?php
$url = "https://api.example.com/endpoint";
$ch = curl_init();
curl_setopt($ch, CURLOPT_URL, $url);
curl_setopt($ch, CURLOPT_RETURNTRANSFER, true);
$response = curl_exec($ch);
if (curl_errno($ch)) {
$error_message = curl_error($ch);
$log_file = "curl_error.log";
$log = fopen($log_file, "a");
fwrite($log, "[" . date("Y-m-d H:i:s") . "] " . $error_message . "\n");
fclose($log);
}
curl_close($ch);
echo $response;
?>
This code will write any cURL errors to a log file named curl_error.log
in the same directory as your script. The error log will include the error message and the date and time of the error.
These examples demonstrate how you can use cURL to make HTTP requests in PHP, but there are many other options and settings available.
Multiple cURL requests in parallel
In PHP, you can make multiple cURL requests at the same time using the curl_multi_exec
function. This allows you to increase the speed and efficiency of your scripts by reducing the wait time between requests.
Here is a simple example of how to use curl_multi_exec
:
<?php
// Initialize multiple cURL requests
$mh = curl_multi_init();
// Add multiple cURL handles
$curl1 = curl_init();
curl_setopt($curl1, CURLOPT_URL, "http://example.com/api1");
curl_multi_add_handle($mh, $curl1);
$curl2 = curl_init();
curl_setopt($curl2, CURLOPT_URL, "http://example.com/api2");
curl_multi_add_handle($mh, $curl2);
// Execute multiple cURL requests
$running = null;
do {
curl_multi_exec($mh, $running);
} while ($running);
// Get the results of each cURL request
$result1 = curl_multi_getcontent($curl1);
$result2 = curl_multi_getcontent($curl2);
// Clean up cURL resources
curl_multi_remove_handle($mh, $curl1);
curl_multi_remove_handle($mh, $curl2);
curl_multi_close($mh);
?>
In this Php curl example, we initialize multiple cURL requests using the curl_multi_init function
, and then add two cURL handles using curl_multi_add_handle
.
We execute the requests using curl_multi_exec
, and wait for all the requests to finish by checking the value of the $running
variable.
Once the requests are complete, we can retrieve the results of each request using curl_multi_getcontent
, and clean up the resources using curl_multi_remove_handle
and curl_multi_close
.
Additional Functions of PHP's cURL
curl_reset
- Reconfigures all parameters of a libcurl sessioncurl_copy_handle
- Duplicating a cURL Handle with its Preferences in PHPcurl_multi_init
— Returns a new cURL multi handlecurl_multi_add_handle
- Incorporating a Single cURL Handle into a cURL Multi Handlecurl_multi_select
- Wait for activity on any curl_multi connectioncurl_multi_setopt
- Set an option for the cURL multi handlecurl_multi_exec
- Execute the sub-components of the active cURL handlecurl_multi_getcontent
- IfCURLOPT_RETURNTRANSFER
is set, returns the content of a cURL handlecurl_multi_close
- Terminating Multiple cURL Handlescurl_multi_errno
- Obtaining the Error Code of the Last cURL Multi Requestcurl_multi_info_read
- Get information about the current transferscurl_multi_remove_handle
- Remove a multi handle from a set of cURL handlescurl_multi_strerror
- Return string describing error codecurl_version
- Provides information about the version of cURLcurl_pause
- The curl session can be paused and unpausedcurl_error
- Returns the string representing the error encountered during the current session.curl_file_create
- Creates a cURLFile objectcurl_getinfo
- Obtains information about a transfer
Uses of PHP cURL function
Here are some of the common uses of the PHP cURL library:
- Sending HTTP requests: cURL can send HTTP requests to a server, including GET, POST, PUT, and DELETE requests. This can be used for various purposes, such as fetching data from an API, submitting form data, or uploading files to a server.
- Scraping web pages: cURL can be used to scrape data from web pages, allowing you to extract information from websites and use it in your PHP code.
- Downloading files: cURL can be used to download files from a server, such as images or documents, and save them on your local system.
- Uploading files: cURL can be used to upload files to a server, such as images or documents, using methods like POST or PUT requests.
- Checking URLs: cURL can be used to check the status of a URL and determine if it is accessible. This can be used to check if a website is down or not, for example.
- Transferring data between servers: cURL can be used to transfer data between servers, such as sending data from one server to another for processing or storage.
- Sending requests with different protocols: cURL supports various protocols, including HTTP, HTTPS, FTP, FTPS, and more, so it can be used for many network-related tasks.
These are just a few of the many uses of the PHP cURL library. Its versatility makes it a powerful tool for working with data and interacting with servers.
Final thoughts
cURL is an effective tool for automating repetitive tasks and testing APIs. libcurl is a prevalently used, open-source, cross-platform library that is compatible with several well languages.
You can enhance almost each attribute of cURL employing one of it's more than 380 flags. With cURL, developers can rapidly & firmly fetch data from the website, User interfaces, datasets, and others.
Collaborating with other webpages, notably those that have access to third party data, rapidly and effortlessly by using cURL in PHP extension. The extension is especially helpful because it makes it easier to get information from other websites.
Without having to manually write complicated code, it enables us to quickly and securely retrieve information. For many web developers who want to quickly access data from websites without having to worry about writing the code to do it manually, cURL has become a renowned extension.
Atatus: PHP Performance Monitoring and Log Management
Atatus is an Application Performance Management (APM) solution that collects all requests to your PHP applications without requiring you to change your source code. However, the tool does more than just keep track of your application's performance.
Monitor logs from all of your PHP applications and systems into a centralized and easy-to-navigate user interface, allowing you to troubleshoot faster using PHP monitoring.
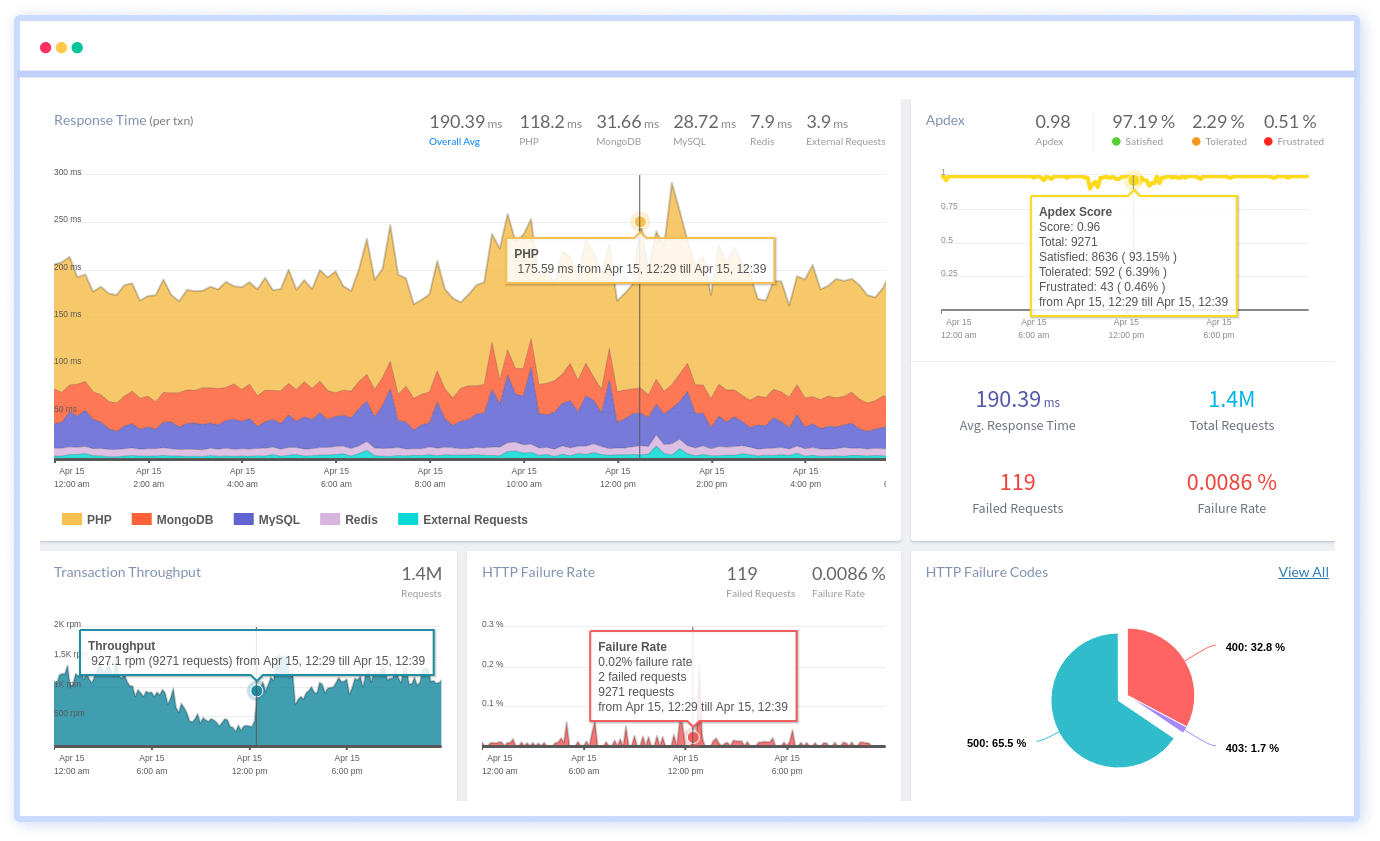
We offer Application Performance Monitoring, Real User Monitoring, Serverless Monitoring, Logs Monitoring, Synthetic Monitoring, Uptime Monitoring, and API Analytics. It works perfectly with any application, regardless of framework, and has plugins.
#1 Solution for Logs, Traces & Metrics
APM
Kubernetes
Logs
Synthetics
RUM
Serverless
Security
More