Pretty Print JSON in Ruby, Python, Java, Node.js, Golang, .NET, and PHP
JSON (JavaScript Object Notation) is a format for storing and exchanging data. It is a text-based, human-readable format for representing simple data structures and associative arrays (called objects).
JSON is a language-independent format. That means JSON code can be written in any language, including Ruby, Python, Java, .NET, PHP, Node.js, and Golang. JSON code is often more readable than code written in other languages.
If you work with JSON data often, you know how important it is to be able to read and write it in a way that is both easy for humans and machines to understand. Pretty printing JSON can make it much easier to read, and can also help with debugging.
In this blog post, we will show you how to pretty print JSON in Ruby, Python, Java, .NET, PHP, Node.js, and Golang.
Table of Contents
- Pretty Print JSON with Ruby
- Pretty Print JSON with Python
- Pretty Print JSON with Java
- Pretty Print JSON with Node.js
- Pretty Print JSON with Golang
- Pretty Print JSON with .NET
- Pretty Print JSON with PHP
1. Pretty Print JSON with Ruby
We have a JSON string and when we try to print it to the console, it prints a single line of the JSON data without any format or indentation.
json_example = '[{"Customer": 1, "name": "Alice", "country": ["Spain", "Madrid"]},{"Customer": 2, "name": "Jack", "country": ["UK", "London"]}]'
puts json_example
Output:
[{"Customer": 1, "name": "Alice", "country": ["Spain", "Madrid"]},{"Customer": 2, "name": "Jack", "country": ["UK", "London"]}]
You can pretty print JSON data with proper indentation and white spaces by the following methods:
i.) Using pretty_generate method
If you're working with JSON in Ruby, make it look nice when you print it out. Here's how you can pretty print JSON in Ruby.
The JSON library pretty_generate method
takes the minified, parsed JSON string and returns the prettified JSON format.
Example code
require "json"
json_example = '[{"Customer": 1, "name": "Alice", "country": ["Spain", "Madrid"]},{"Customer": 2, "name": "Jack", "country": ["UK", "London"]}]';
puts JSON.pretty_generate(JSON.parse(json_example))
Output:
[
{
"Customer": 1,
"name": "Alice",
"country": [
"Spain",
"Madrid"
]
},
{
"Customer": 2,
"name": "Jack",
"country": [
"UK",
"London"
]
}
]
ii.) Using awesome_print method
Indentation and colored styles are provided in awesome_print library for formatting ruby objects as JSONs.
In order to use awesome_print
, you must install awesome_print package. This method calls JSON.parse() and returns a pretty JSON object.
Install awesome_print
with the below command:
gem install awesome_print
Usage
The awesome_print
offers a method ap
with which you can pretty print the Ruby objects in the console. It contains two parameters: the JSON object & options.
require "awesome_print"
ap object, options = {}
You can explore the various options offered by the ap method here.
2. Pretty Print JSON with Python
To process the JSON data python has a built-in module called json
. We can use the module to pretty print the JSON data.
i.) Pretty print JSON string in Python
As the first step you have to convert the json string to a python object. And then with the help of the json.dumps()
you can convert the python object into JSON formatted string.
import json
cust_info = '{"destination_addresses":["Washington, DC, USA","Philadelphia, PA, USA","Santa Barbara, CA, USA","Miami, FL, USA","Austin, TX, USA","Napa County, CA, USA"],"origin_addresses":["New York, NY, USA"],"rows":[{"elements":[{"distance":{"text":"227 mi","value":365468},"duration":{"text":"3 hours 54 mins","value":14064},"status":"OK"},{"distance":{"text":"94.6 mi","value":152193},"duration":{"text":"1 hour 44 mins","value":6227},"status":"OK"},{"distance":{"text":"2,878 mi","value":4632197},"duration":{"text":"1 day 18 hours","value":151772},"status":"OK"},{"distance":{"text":"1,286 mi","value":2069031},"duration":{"text":"18 hours 43 mins","value":67405},"status":"OK"},{"distance":{"text":"1,742 mi","value":2802972},"duration":{"text":"1 day 2 hours","value":93070},"status":"OK"},{"distance":{"text":"2,871 mi","value":4620514},"duration":{"text":"1 day 18 hours","value":152913},"status":"OK"}]}],"status":"OK"}'
cust_obj = json.loads(cust_info)
cust_formatted_str = json.dumps(cust_obj, indent=2)
print(cust_formatted_str)
Output:
{
"destination_addresses": [
"Washington, DC, USA",
"Philadelphia, PA, USA",
"Santa Barbara, CA, USA",
"Miami, FL, USA",
"Austin, TX, USA",
"Napa County, CA, USA"
],
"origin_addresses": [
"New York, NY, USA"
],
"rows": [
{
"elements": [
{
"distance": {
"text": "227 mi",
"value": 365468
},
"duration": {
"text": "3 hours 54 mins",
"value": 14064
},
"status": "OK"
},
{
"distance": {
"text": "94.6 mi",
"value": 152193
},
"duration": {
"text": "1 hour 44 mins",
"value": 6227
},
"status": "OK"
},
{
"distance": {
"text": "2,878 mi",
"value": 4632197
},
"duration": {
"text": "1 day 18 hours",
"value": 151772
},
"status": "OK"
},
{
"distance": {
"text": "1,286 mi",
"value": 2069031
},
"duration": {
"text": "18 hours 43 mins",
"value": 67405
},
"status": "OK"
},
{
"distance": {
"text": "1,742 mi",
"value": 2802972
},
"duration": {
"text": "1 day 2 hours",
"value": 93070
},
"status": "OK"
},
{
"distance": {
"text": "2,871 mi",
"value": 4620514
},
"duration": {
"text": "1 day 18 hours",
"value": 152913
},
"status": "OK"
}
]
}
],
"status": "OK"
}
There are number of parameters in the json
module:
json_object
: defines the JSON object that you wish to prettify and print.skipkeys
: by default, it is set to false, when it is set to true it skip keys that are not a string, int, float, boolean or None.ensure_ascii
: it is set to true by default, it will return Non-ascii characters escaped when it is set to true.check_circular
: if set to true, skips circular references for container types. If not, returnOverflowError
.allow_nan
: NaN, Infinity, or -Infinity are substituted for out-of-range floats if set to true.indent
: you can specify the indent-level with theindent
function, the function will print only new lines if the indentation level is set to 0 or any negative integer.seperators
: specifies the item and the key seperate characterssort_keys
: sorts the output python dict by its keys if it is set to true.
json.dumps(
json_obj,
*,
skipkeys=False,
ensure_ascii=True,
check_circular=True,
allow_nan=True, cls=None,
indent=None,
separators=None,
default=None,
sort_keys=False,
**kw
)
ii.) Pretty print JSON file using pprint method in Python
Another popular and common way to display JSON data in Python code is by using pretty prints. You can import Python's pprint module for prettifying JSON data.
A formatted structure of your JSON data can be printed using its pprint() method. JSON data can be formatted with the PrettyPrinter class, which is part of the pprint module.
import json
import pprint
with open("cust_info.json", "r") as cust_data_file:
cust_obj = json.load(cust_data_file)
print(cust_obj)
cust_formatted_str = pprint.PrettyPrinter(indent = 2, width = 75, compact = False)
print("Pretty Printing JSON Data using pprint module")
cust_formatted_str.pprint(cust_obj)
We have imported two modules: json
and pprint
. We have opened the customer info json file in read mode and load the json using the json_load()
function.
Our next step is to set the indent, width, con=compact, and other attributes using the pprint.PrettyPrinter()
constructor. As a final step, we will pretty print our data using the pprint()
method.
3. Pretty Print JSON with Java
There are three different libraries with which you can easily pretty print the JSON data.
Java program to serialize the customer object.
public class Customer
{
private Integer id;
private String name;
private String[] country;
private String email;
//Constructors
//Getters and setters
}
i.) Pretty print JSON data using GSON library in Java
The JSON data is printed in a compact format by default with GSON. It is created by Google.
Using the GsonBuilder
, we can configure the Gson instance
to enable Pretty Print and then use the setPrettyPrinting
method to invoke the configuration.
In GSON, output JSON is formatted as 80 characters long, with 2 characters indentation, and 4 characters right margin.
// enabling gson pretty print
Gson gson = new GsonBuilder()
.setPrettyPrinting()
.create();
String jsonOutput = gson.toJson(someObject);
Java program to pretty print the customer JSON data.
Example:
import com.google.gson.Gson;
import com.google.gson.GsonBuilder;
public class Main
{
public static void main(String[] args)
{
Customer customerObj = new Customer(13, "Aldrin", {"Spain", "Madrid"}, "aldrin@gmail.com");
Gson gson = new GsonBuilder().setPrettyPrinting().create();
System.out.println(gson.toJson(customerObj));
}
}
Output:
{
"id": 13
"name": "Aldrin",
"country": [
"Spain",
"Madrid"
],
"email": "john.doe@example.com",
}
ii.) Pretty print JSON data using JSON-Java library in Java
JSON-Java Library users can convert the JSON object to pretty-printed JSON using JSONObject.toString(indentFactor)
. Here is an example of how this method might be invoked:
Example:
import org.json.JSONException;
import org.json.JSONObject;
public class Main {
public static void main(String[] args) {
Customer customerObj = new Customer(13, "Aldrin", {"Spain", "Madrid"}, "aldrin@gmail.com");
try {
JSONObject json = new JSONObject(customerObj);
String prettyJsonString = json.toString(4);
System.out.println(prettyJsonString);
} catch (JSONException e) {
e.printStackTrace();
}
}
}
Output:
{
"id": 13
"name": "Aldrin",
"country": [
"Spain",
"Madrid"
],
"email": "john.doe@example.com",
}
iii.) Pretty print JSON data using Jackson library in Java
To serialize objects with the pretty printer for indentation, the Jackson library (com.fasterxml.jackson.databind)
provides the factory method writerWithDefaultPrettyPrinter()
.
I have outlined the code below:
import com.fasterxml.jackson.databind.ObjectMapper;
public class Main {
public static void main(String[] args) {
Customer customerObj = new Customer(13, "Aldrin", {"Spain", "Madrid"}, "aldrin@gmail.com");
ObjectMapper mapper = new ObjectMapper();
String prettyJsonString = mapper.writerWithDefaultPrettyPrinter()
.writeValueAsString(customerObj);
System.out.println(prettyJsonString);
}
}
Output:
{
"id": 13
"name": "Aldrin",
"country": [
"Spain",
"Madrid"
],
"email": "john.doe@example.com",
}
4. Pretty Print JSON with Node.js
To pretty-print the JSON object in JavaScript you can use the JSON_stringify()
method. It accepts three parameters: JSON object, replacer and the space.
json_object
- The JSON data that you wish to pretty print.replacer
- an array or function can be used to manipulate the result.space
- string or a number to insert whitespace including indentation, line-breaks, etc.
custObj = { id:1, name: "Alice", country: ["Spain", "Madrid"] };
// convert JSON object to string
const custStr = JSON.stringify(custObj , null, "\t");
console.log(custStr);
Output:
{
"id": 1,
"name": "Alice",
"country": [
"Spain",
"Madrid"
]
}
5. Pretty-print JSON with Golang
To display the JSON data with appropriate indentation there are various ways using the encoding/json
package.
i.) Pretty print JSON string
The function json.Indent
is useful to prettify the JSON data with proper indentation. It includes parameters such as JSON, indent, output buffer and prefix.
package main
import (
"bytes"
"encoding/json"
"fmt"
"log"
)
func formatJSON(data []byte) ([]byte, error) {
var out bytes.Buffer
err := json.Indent(&out, data, "", " ")
if err == nil {
return out.Bytes(), err
}
return data, nil
}
func main() {
data := []byte(`{"destination_addresses":["Philadelphia, PA, USA"],"origin_addresses":["New York, NY, USA"],"rows":[{"elements":[{"distance":{"text":"94.6 mi","value":152193},"duration":{"text":"1 hour 44 mins","value":6227},"status":"OK"}]}],"status":"OK"}`)
prettyJSON, err := formatJSON(data)
if err != nil {
log.Fatal(err)
}
fmt.Println(string(prettyJSON))
}
Output:
{
"destination_addresses": [
"Philadelphia, PA, USA"
],
"origin_addresses": [
"New York, NY, USA"
],
"rows": [
{
"elements": [
{
"distance": {
"text": "94.6 mi",
"value": 152193
},
"duration": {
"text": "1 hour 44 mins",
"value": 6227
},
"status": "OK"
}
]
}
],
"status": "OK"
}
ii.) Pretty print JSON by marshalling value
The marshal
function generates a JSON data without any indentation or formatting. The json.MarshalIndent
is used to marshall an object, while indent
is for []byte
input.
package main
import (
"encoding/json"
"fmt"
"log"
)
func formatJson(custInfo interface {}) (string, error) {
val, err := json.MarshalIndent(custInfo, "", " ")
if err != nil {
return "", err
}
return string(val), nil
}
type CustomerInfo struct {
FirstName string `json:"firstname"`
MiddleName string `json:"middlename"`
LastName string `json:"lastname"`
Country []string `json:"country"`
}
func main() {
customer := CustomerInfo {
FirstName: "Mary",
MiddleName: "Elizabeth",
LastName: "Smith",
Country: []string{"Spain", "Madrid" },
}
res, err := formatJson(customer)
if err != nil {
log.Fatal(err)
}
fmt.Println(res)
}
When you run the above code you will get the formatted JSON data.
Output:
{
"firstname": "Mary",
"middlename": "Elizabeth",
"lastname": "Smith",
"country": [
"Spain",
"Madrid"
]
}
iii.) By encoding value
In json.Encode
, the Encoder.SetIndent
method can be used to set indentation similarly to marshaling by setting a prefix
and indent
.
package main
import (
"encoding/json"
"fmt"
"log"
"bytes"
"io"
)
func formatJson(custInfo interface{}, out io.Writer) error {
enc := json.NewEncoder(out)
enc.SetIndent("", " ")
if err := enc.Encode(custInfo); err != nil {
return err
}
return nil
}
type CustomerInfo struct {
FirstName string `json:"firstname"`
MiddleName string `json:"middlename"`
LastName string `json:"lastname"`
Country []string `json:"country"`
}
func main() {
customer := CustomerInfo {
FirstName: "Achmad",
MiddleName: "Rizky",
LastName: "Syahrani",
Country: []string { "Spain","Madrid" },
}
var buffer bytes.Buffer
err := formatJson(customer, &buffer)
if err != nil {
log.Fatal(err)
}
fmt.Println(buffer.String())
}
Output:
{
"firstname": "Achmad",
"middlename": "Rizky",
"lastname": "Syahrani",
"country": [
"Spain",
"Madrid"
]
}
6. Pretty Print JSON with .NET
The Newtonsoft.Json
namespace provides the class called JsonConvert
which helps in serializing and deserializing the JSON objects.
JsonConvert.SerializeObject(object, Formatting)
helps in serializing a particular object into .NET JSON string using Formatting.
using System;
using Newtonsoft.Json;
namespace FormatJson {
internal class Program {
private static void Main(string[] args) {
Customer customer = new Customer {
Id = 34,
Name = "Alice",
Country = new [] {
"Spain",
"Madrid"
}
};
string formatted_json = JsonConvert.SerializeObject(customer, Formatting.Indented);
Console.WriteLine(formatted_json);
Customer deserializedProduct = JsonConvert.DeserializeObject < Customer > (formatted_json);
}
}
internal class Customer {
public int Id {
get;
set;
}
public String Name {
get;
set;
}
public String[] Country {
get;
set;
}
}
}
Output:
{
"Id": 34,
"Name": "Alice",
"Country": [
"Spain",
"Madrid"
]
}
7. Pretty Print JSON with PHP
The json_encode()
is a built-in function in PHP which takes a value and returns the JSON representation of the particular value.
It accepts two arguments value and options
and return the JSON string.
- value - Value parameters of mixed type are required.
- options - The options include
JSON_HEX_QUOT
,JSON_HEX_TAG
,JSON_HEX_AMP
,JSON_HEX_APOS
,JSON_NUMERIC_CHECK
,JSON_PRETTY_PRINT
,JSON_UNESCAPED_SLASHES
,JSON_FORCE_OBJECT
.
As shown in this example, we have formatted our messy JSON data using the JSON_PRETTY_PRINT
option.
<?php
$cust_info = array( "Id"=> "13", "Name" => "Alice", "Country" => array( "Spain", "Madrid" ), "Mobile Number" => "897XXXXXXX");
$format_json = json_encode($cust_info, JSON_PRETTY_PRINT);
echo($format_json)
?>
Output:
{
"Id": "13",
"Name": "Alice",
"Country": [
"Spain",
"Madrid"
],
"Mobile Number": "897XXXXXXX"
}
Ensure your JavaScript program begins with header('Content-Type: application/json');
if you're passing JSON data.
<?php
header('Content-Type: application/json');
$cust_info = '{"Id": 1, "Name":"Alice", "Country": ["Spain", "Madrid"]}';
$format_json = json_encode(json_decode($cust_info), JSON_PRETTY_PRINT);
echo($format_json);
?>
Conclusion
In summary, JSON is a standard way to represent structured information. It is actually a data interchange format, meaning it is a language-neutral method of storing data so that one application can control how its data is transferred via HTTP to another application.
Pretty printing doesn't just make your JSON a little more human readable; it can drastically improve readability, especially if you're using JSON as a data structure.
Monitor Your Entire Application with Atatus
Atatus is a Full Stack Observability Platform that lets you review problems as if they happened in your application. Instead of guessing why errors happen or asking users for screenshots and log dumps, Atatus lets you replay the session to quickly understand what went wrong.
We offer Application Performance Monitoring, Real User Monitoring, Server Monitoring, Logs Monitoring, Synthetic Monitoring, Uptime Monitoring and API Analytics. It works perfectly with any application, regardless of framework, and has plugins.
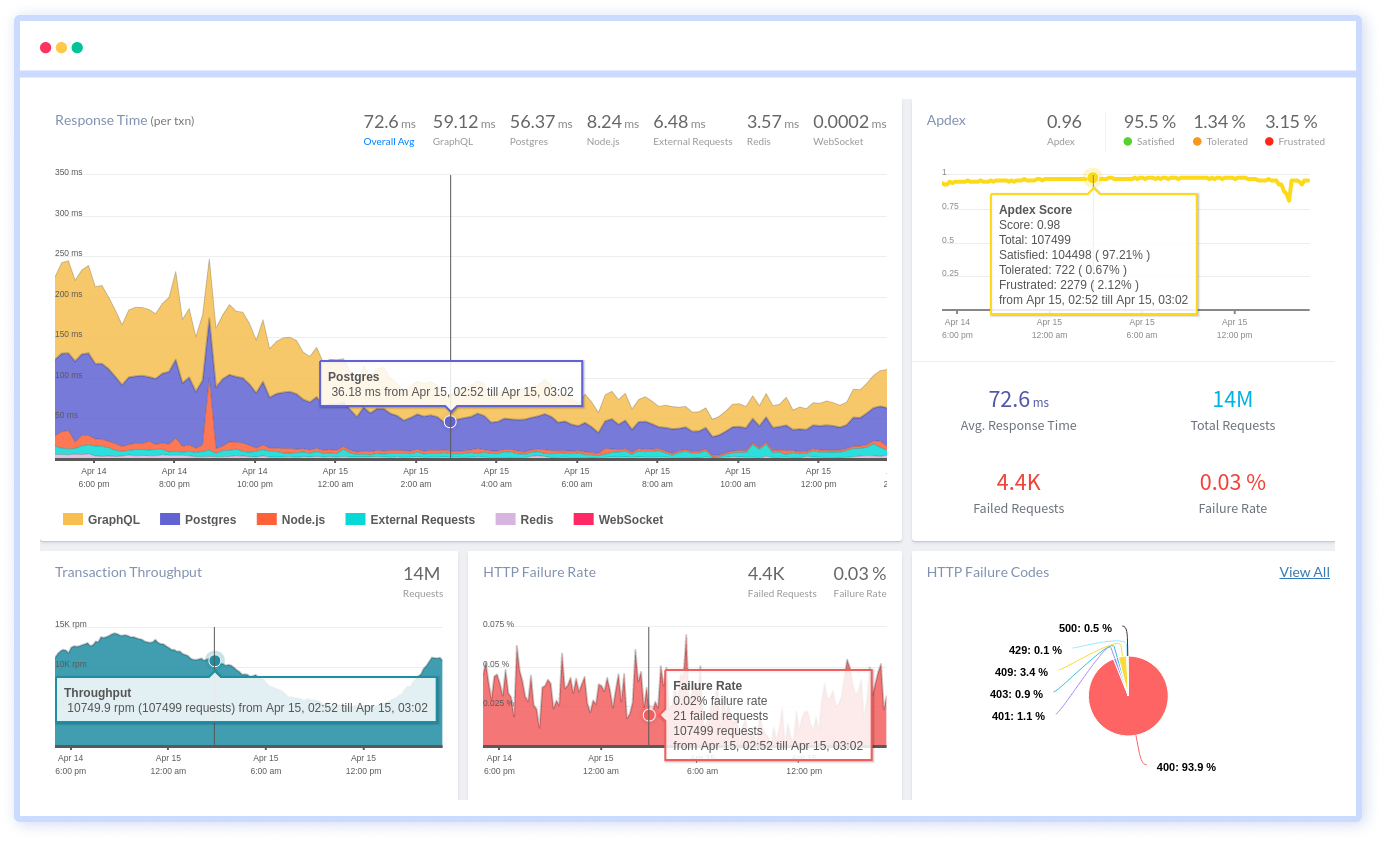
Atatus can be beneficial to your business, which provides a comprehensive view of your application, including how it works, where performance bottlenecks exist, which users are most impacted, and which errors break your code for your frontend, backend, and infrastructure.
If you are not yet a Atatus customer, you can sign up for a 14-day free trial .
#1 Solution for Logs, Traces & Metrics
APM
Kubernetes
Logs
Synthetics
RUM
Serverless
Security
More