Introduction to Python arrays
An Python array is a set of items kept close to one another in memory. With just an offset added to a base value, it is possible to determine the position of each element when storing multiple items of the same type together.
In Python, an "array" module is used to manage Python arrays. Although lists can be used like Python arrays, users cannot specify the kind of elements that can be stored there. Therefore, when using the array module to create Python arrays, all array components ought to be of the same sort.
Arrays in Python, are a widely employed module. Both the NymPy package and the Python Standard Library can be used to create them. However, the NumPy array is the standard since it is more versatile and reliable.
Using NumPy arrays allows you to perform arithmetic operations on the entire array, which is a unique variable that can hold more than one value at once.
Receding code size and eliminating complicated syntax, arrays in Python might save time and effort. Additionally, it reduces the amount of code that must be written because only one variable must be created, and multiple values must be stored.
Table Of Contents
- what are Arrays in Python?
- Difference between Python Arrays and Lists
- Aspects of Implying Arrays in Python
- Basic Array Operations
- Python Array Methods
what are Arrays in Python?
Several programming languages adopt Python arrays as an intrinsic data structure since they are containers that can enable multiple items concurrently. Furthermore, they are an ordered set of items, with each value of the same data type.
Arrays in Python are data structures that may hold several objects of the same kind, making it simple to retain, manage, retrieve, and modify the values.
Python Arrays are highly efficient because they perform a wide range of operations, including filtering the data, applying mathematical functions to chunks of data, and even formulating visual user interfaces.
Arrays in Python are a profound data model across most coding languages, and they function as Python warehouses that can retain over one at a time. Arrays in Python are the organized set of elements that share the same dataset with each value, aiming to make each other a valuable data framework while applied to data that might be noted as a single unit.
Python arrays enable it to retrieve and manage data efficiently, and they're notably reliable when functioning with extensive databases. Data might be appropriately searched for and analyzed employing Python arrays.
By importing an array module, you can be creating an array in Python. A data type and value list are specified in an array using the array( data_type, value_list )
.
from array import *
arrayName = array(typecode, [Initializers])
In an Python array's typecode determines what values it can contain. The following are some commonly used type codes in Python arrays:
Typecode | Value |
b | Integer with size 1 byte/td |
B | Unsigned integer of size 1 byte |
c | A character with a size of 1 byte |
i | A signed integer with a size of 2 bytes |
I | The size of the unsigned integer is 2 bytes |
f | This is a floating point representation of size 4 bytes |
d | A floating point value of 8 bytes in size |
Difference between Python Arrays and Lists
Python lists and arrays are both used to store collections of data, but there are some key differences between them:
- Data types: In Python, a list can store values of any data type, including other lists. On the other hand, an Python array can only store values of the same data type. This means that if you want to store a collection of integers, you can use an Python array for better performance, but if you need to store a collection of mixed data types, you'll need to use a list.
- Memory allocation: When you create a Python list, the memory is allocated dynamically, and the list can grow or shrink as needed. This means that the size of a list can be easily changed. In contrast, when you create a Python array, the memory is pre-allocated and the size of the Python array cannot be changed dynamically. This means that if you need to add or remove elements from an Python array, you'll need to create a new array in Python and copy the elements over.
- Performance: Since arrays in Python are pre-allocated with a fixed size, they are generally faster and more efficient than lists when it comes to accessing and manipulating elements. This is because Python arrays use contiguous blocks of memory, which makes them easier to access and process.
Aspects of Implying Arrays in Python
- Python arrays will be used to process data dynamically and store a lot of the same type of variables.
- Memory-efficient to compute.
- Python arrays are faster than lists and can save time or effort by modifying the code and removing unusual syntax.
- Arrays in Python easily manage massive amounts of data.
- In addition to being more storage than lists, NumPy arrays are also much more functional & annex minimal memory.
- Quicker operations and analysis than lists.
Basic Array Operations
In an Python array can be used to perform the following operations:
- Traverse - The elements are printed one by one.
- Insertion - Adds elements at the specified index.
- Deletion - Deletes the specified index of an element.
- Search - Using an index or value; it searches for an element.
- Update - This method updates the index of an element.
1. Adding Elements
The built-in insert()
function can be used to add elements to the Python array. The insert function allows several data elements to be inserted into the Python array. Depending upon the requisition, adding an element to an array can occur at any index, whether at the beginning, end, or middle. An array's value is also appended at the end using append()
.
# Python program to demonstrate
# Adding Elements to a Array
# importing "array" for array creations
import array as arr
# array with int type
a = arr.array('i', [1, 2, 3])
print ("Array before insertion : ", end =" ")
for i in range (0, 3):
print (a[i], end =" ")
print()
# inserting array using
# insert() function
a.insert(1, 4)
print ("Array after insertion : ", end =" ")
for i in (a):
print (i, end =" ")
print()
# array with float type
b = arr.array('d', [2.5, 3.2, 3.3])
print ("Array before insertion : ", end =" ")
for i in range (0, 3):
print (b[i], end =" ")
print()
# adding an element using append()
b.append(4.4)
print ("Array after insertion : ", end =" ")
for i in (b):
print (i, end =" ")
print()
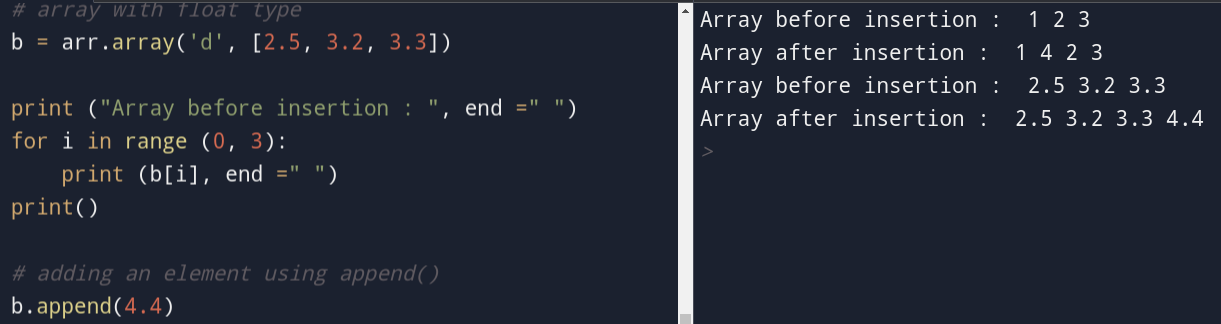
2. Accessing Elements
Python uses indices to access array elements. An array item can be accessed using the index operator [ ]
. Similarly to lists, indices begin at zero. Indexes must be integers.
# Python program to demonstrate
# accessing of element from list
# importing array module
import array as arr
# array with int type
a = arr.array('i', [1, 2, 3, 4, 5, 6])
# accessing element of array
print("Access element is: ", a[0])
# accessing element of array
print("Access element is: ", a[3])
# array with float type
b = arr.array('d', [2.5, 3.2, 3.3])
# accessing element of array
print("Access element is: ", b[1])
# accessing element of array
print("Access element is: ", b[2])
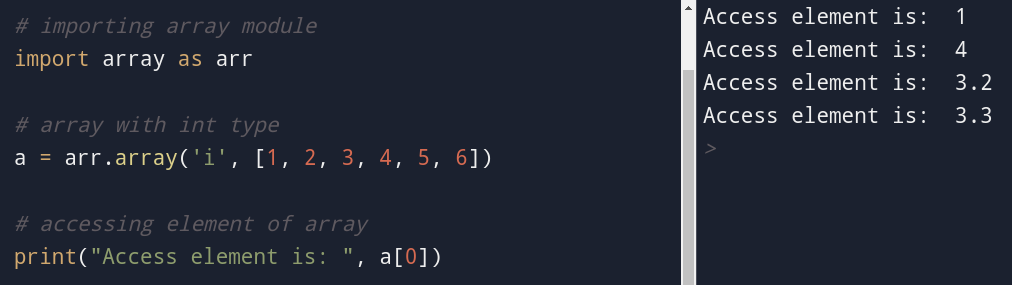
3. Removing Elements
A built-in function, remove()
, can be used to remove elements from the Python array, but an error is thrown if the element doesn't exist in the set. When using the remove ()
method, an iterator is used to remove a range of elements rather than one at a time.
Pop()
has the ability to return elements in addition to removing them from an array in Python, but it typically only returns the last element in the array. When using Pop()
to remove an element from a specific location in the Python array, an element's index is passed as an argument.
# Python program to demonstrate
# Removal of elements in a Array
# importing "array" for array operations
import array
# initializing array with array values
# initializes array with signed integers
arr = array.array('i', [1, 2, 3, 1, 5])
# printing original array
print("The new created array is : ", end ="")
for i in range (0, 5):
print (arr[i], end =" ")
print ("\r")
# using pop() to remove element at 2nd position
print("The popped element is : ", end ="")
print(arr.pop(2))
# printing array after popping
print ("The array after popping is : ", end ="")
for i in range (0, 4):
print (arr[i], end =" ")
print("\r")
# using remove() to remove 1st occurrence of 1
arr.remove(1)
# printing array after removing
print ("The array after removing is : ", end ="")
for i in range (0, 3):
print (arr[i], end =" ")
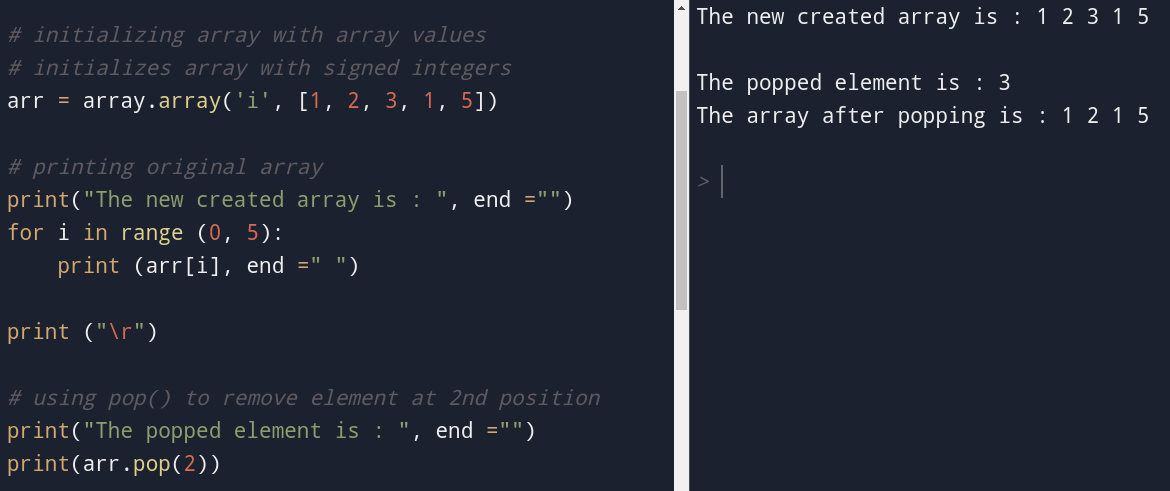
4. Slicing
The slicing operator (:)
in Python allows access to a range of elements in an array. A range can be printed by using [:Index]
, an end range can be printed by [:-Index]
.
A specific index can be printed up until the end using [Index:]
, elements can be printed within a range with [Start Index:End Index]
, and a list can be printed by using [:]
. Then, to reverse the array, use [::-1]
.
# Python program to demonstrate
# slicing of elements in a Array
# importing array module
import array as arr
# creating a list
l = [1, 2, 3, 4, 5, 6, 7, 8, 9, 10]
a = arr.array('i', l)
print("Initial Array: ")
for i in (a):
print(i, end =" ")
# Print elements of a range
# using Slice operation
sliced_array = a[3:8]
print("\nSlicing elements in a range 3-8: ")
print(sliced_array)
# Print elements from a
# pre-defined point to end
sliced_array = a[5:]
print("\nElements sliced from 5th "
"element till the end: ")
print(sliced_array)
# Printing elements from
# beginning till end
sliced_array = a[:]
print("\nPrinting all elements using slice operation: ")
print(sliced_array)
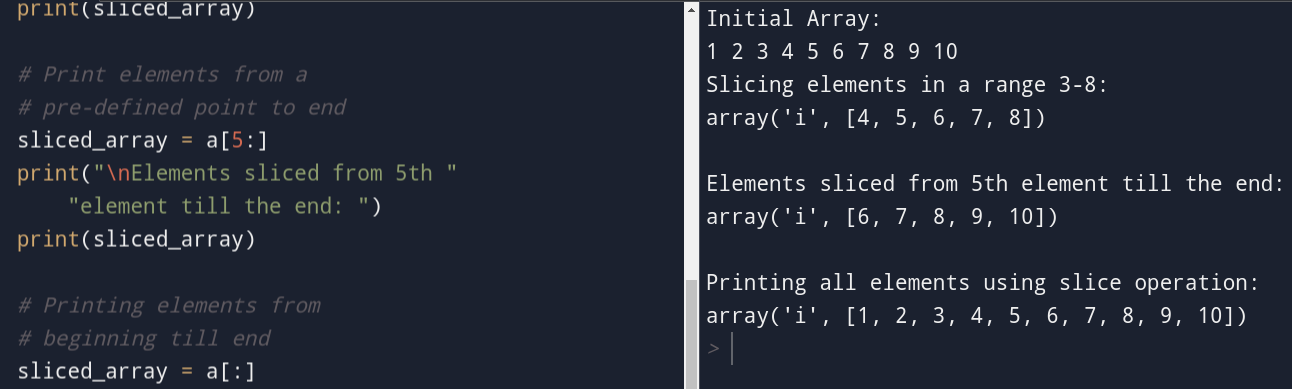
5. Searching element
An Python array can be searched for an element based on its index or value. Python array elements can be found using the index()
method based on their values. An element's index is returned by this method.
# Python code to demonstrate
# searching an element in array
# importing array module
import array
# initializing array with array values
# initializes array with signed integers
arr = array.array('i', [1, 2, 3, 1, 2, 5])
# printing original array
print ("The new created array is : ", end ="")
for i in range (0, 6):
print (arr[i], end =" ")
print ("\r")
# using index() to print index of 1st occurrence of 2
print ("The index of 1st occurrence of 2 is : ", end ="")
print (arr.index(2))
# using index() to print index of 1st occurrence of 1
print ("The index of 1st occurrence of 1 is : ", end ="")
print (arr.index(1))
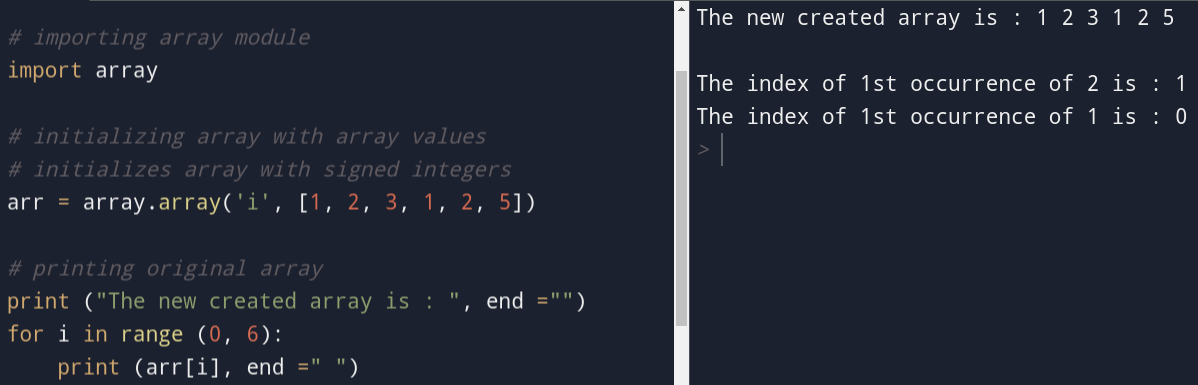
6. Updating Elements
At a given index, an update operation updates an existing element. An Python array element is updated when a new value is assigned to an array index.
# Python code to demonstrate
# how to update an element in array
# importing array module
import array
# initializing array with array values
# initializes array with signed integers
arr = array.array('i', [1, 2, 3, 1, 2, 5])
# printing original array
print ("Array before updation : ", end ="")
for i in range (0, 6):
print (arr[i], end =" ")
print ("\r")
# updating a element in a array
arr[2] = 6
print("Array after updation : ", end ="")
for i in range (0, 6):
print (arr[i], end =" ")
print()
# updating a element in a array
arr[4] = 8
print("Array after updation : ", end ="")
for i in range (0, 6):
print (arr[i], end =" ")
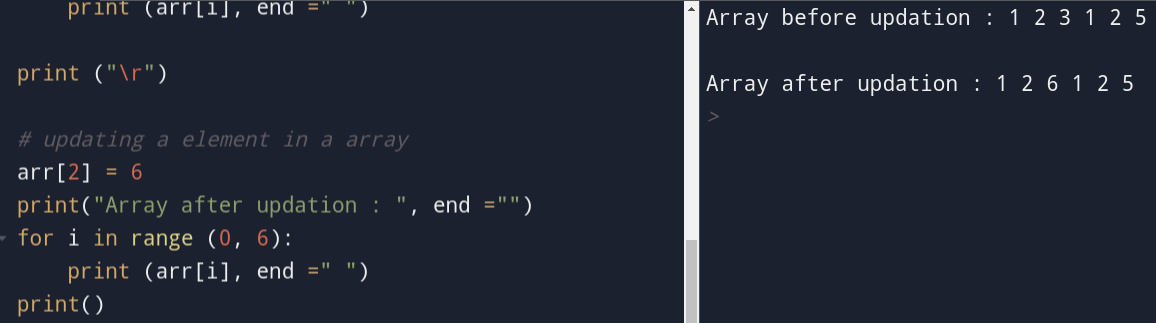
Python Array Methods
There are a number of built-in array methods in Python, including:
1. append()
This method appends an element to the end of the array.
import array
my_array = array.array('i', [1, 2, 3])
my_array.append(4)
print(my_array) # Output: array('i', [1, 2, 3, 4])
2. clear()
This method removes all elements from the array.
import array
my_array = array.array('i', [1, 2, 3])
my_array.clear()
print(my_array) # Output: array('i')
3. copy()
This method returns a shallow copy of the array.
import array
my_array = array.array('i', [1, 2, 3])
new_array = my_array.copy()
print(new_array) # Output: array('i', [1, 2, 3]
4. count()
This method returns the number of occurrences of a given element in the array.
import array
my_array = array.array('i', [1, 2, 2, 3])
print(my_array.count(2)) # Output: 2
5. len()
The len()
function in Python returns the number of elements in an array. Here is an example:
import array
my_array = array.array('i', [1, 2, 3, 4, 5])
array_length = len(my_array)
print("The length of my_array is:", array_length)
6. extend()
This method extends the array by appending all the elements from the iterable object.
import array
my_array = array.array('i', [1, 2, 3])
new_elements = [4, 5, 6]
my_array.extend(new_elements)
print(my_array) # Output: array('i', [1, 2, 3, 4, 5, 6])
7. index()
This method returns the index of the first occurrence of a given element in the array.
import array
my_array = array.array('i', [1, 2, 3])
print(my_array.index(2)) # Output:
8. insert()
This method inserts an element at the specified position in the array.
import array
my_array = array.array('i', [1, 2, 3])
my_array.insert(1, 4)
print(my_array) # Output: array('i', [1, 4, 2, 3])
9. pop()
This method removes and returns the element at the specified position, or the last element if no index is specified.
import array
my_array = array.array('i', [1, 2, 3])
print(my_array.pop(1)) # Output: 2
print(my_array) # Output: array('i', [1, 3])
10. remove()
This method removes the first occurrence of the specified element from the Python array.
import array
my_array = array.array('i', [1, 2, 3])
my_array.remove(2)
print(my_array) # Output: array('i', [1, 3])
11. reverse()
This method reverses the order of the elements in the array.
import array
my_array = array.array('i', [1, 2, 3])
my_array.reverse()
print(my_array) # Output: array('i', [3, 2, 1])
12. sort()
The sort()
method is used to sort the elements of an Python array in ascending order. Here is an example:
import array
my_array = array.array('i', [3, 2, 1, 5, 4])
my_array.sort()
print(my_array)
In this example, we created an array my_array
of integers, and used the sort()
method to sort the elements in ascending order. The resulting array is printed to the console.
Note that the sort()
method modifies the original array in place and does not return a new array. If you want to sort a copy of the array, you can use the sorted()
function instead.
Conclusion
The array in Python is one of the key data structures in nearly every programming language. A lot of time and space is saved by encrypting data sets using arrays in Python.
As Python arrays are dynamically sized, users can manage distinct sizes of data without worrying about storage space. Aside from this, using arrays in Python can hold all kinds of data, including complex objects, strings, and integers.
Data structures such as Python arrays require that all elements have the same type. The "array" module operates arrays in Python programs. Python Arrays can be created, manipulated, and accessed with the array module.
Python arrays are added with the insert (i, x) syntax and accessed via indices. They are mutable and can be converted to Unicode. They differ from lists because they can use an index to access any array item. Python's array module has distinct functions for running array operations.
Atatus: Python Performance Monitoring
Atatus is an Application Performance Management (APM) solution that collects all requests to your Python applications without requiring you to change your source code. However, the tool does more than just keep track of your application's performance.
Monitor logs from all of your Python applications and systems into a centralized and easy-to-navigate user interface, allowing you to troubleshoot faster using Python monitoring.
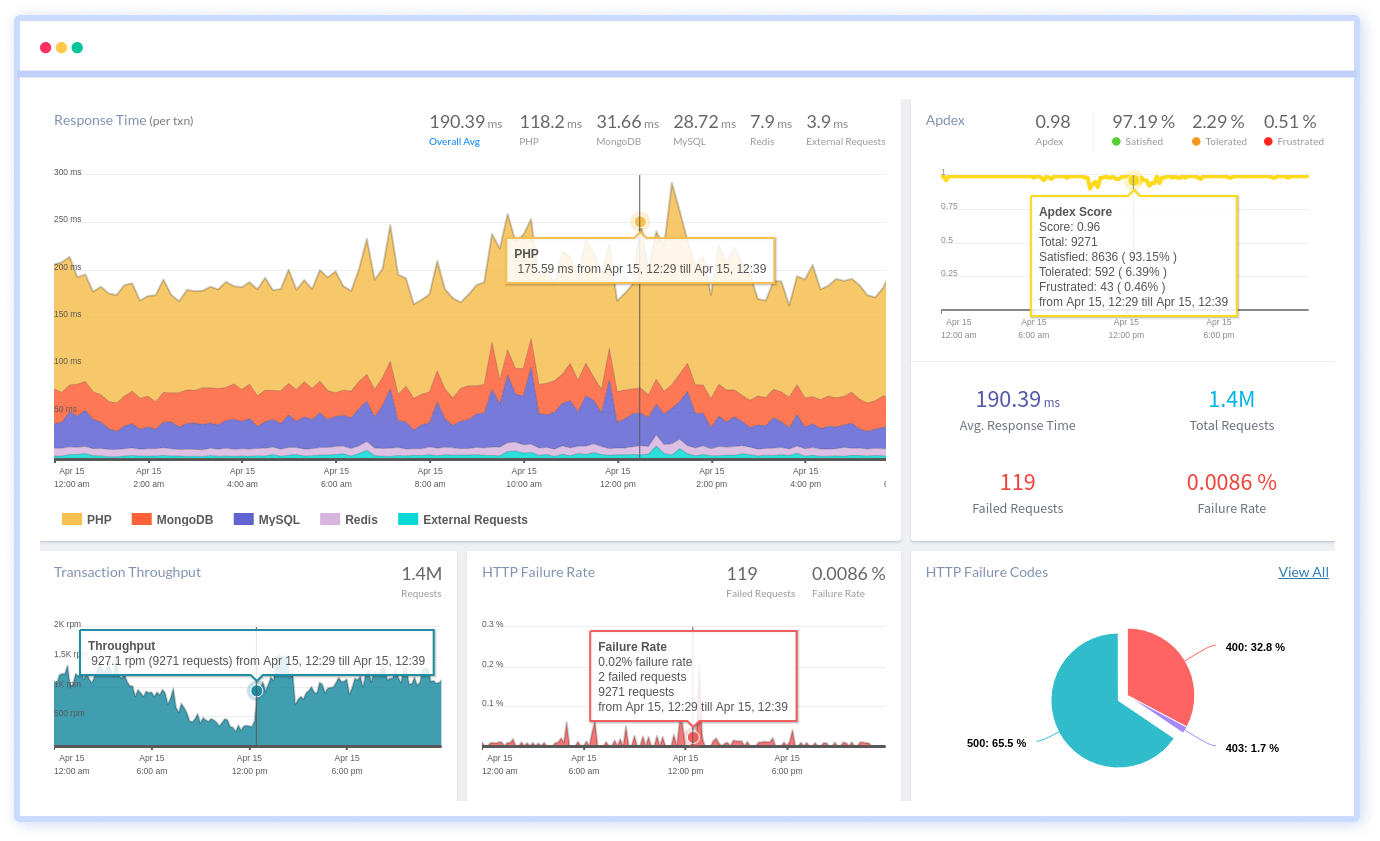
We give a cost-effective, scalable method to centralized Python logging, so you can obtain total insight across your complex architecture. To cut through the noise and focus on the key events that matter, you can search the logs by hostname, service, source, messages, and more. When you can correlate log events with APM slow traces and errors, troubleshooting becomes easy.
#1 Solution for Logs, Traces & Metrics
APM
Kubernetes
Logs
Synthetics
RUM
Serverless
Security
More