Class Variables vs Instance Variables in Python
Variables are a fundamental aspect of any programming language, and Python is no exception. As we already know, python variables are used to store values, which can then be utilised in your program. The values can be of different data types, such as integers, strings, lists, dictionaries, and more.
Are you familiar with the different types of variables in Python and when to use them? Well, when you are working with objects in python, you may come across two different types, which you can use within class - Class variables and instance variables.
Understanding the difference between class variables and instance variables is important in object-oriented programming, as it allows for more efficient and organized code.
Class variables are useful for storing values that are constant across all instances of a class, while instance variables are useful for storing unique values for each object created from the class.
The main aim of this blog is to dive deep into the concept of class variables and instance variables in object-oriented programming within Python.
Let's get started!
Table of contents
- What are Python Class Variables?
- Accessing a Class Variable in python
- Modify value of a Class Variable in python
- What are Python Instance Variables?
- Accessing an Instance Variable in python
- Modify value of an Instance Variable in python
- Delete an Instance Variable in python
- Class Variables vs Instance Variables
- Illustration of Python Class and Instance Variable
What are Python Class Variables?
In Python, a class variable is a variable that is defined within a class and outside of any class method. It is a variable that is shared by all instances of the class, meaning that if the variable's value is changed, the change will be reflected in all instances of the class. Class variables help store data common to all instances of a class.
Creating a Class Variable
Here's an example of how to create a class variable in Python:
class Employee:
office_name = "XYZ Private Limited" # This is a class variable
In this example, the office_name
variable is a class variable, and it is shared among all instances of the Employee
class.
Accessing a Class Variable in Python
In Python you can access class variables in the constructor of a class. The constructor is defined using the special method __init__()
and is called automatically when an instance of a class is created.
Example 1:
To access the value of the class variable within the constructor, you can use the name of the class followed by the class variable as shown in the following example.
class Employee:
office_name = "XYZ Private Limited"
def __init__(self):
print(Employee.office_name)
my_instance = Employee()
Output:
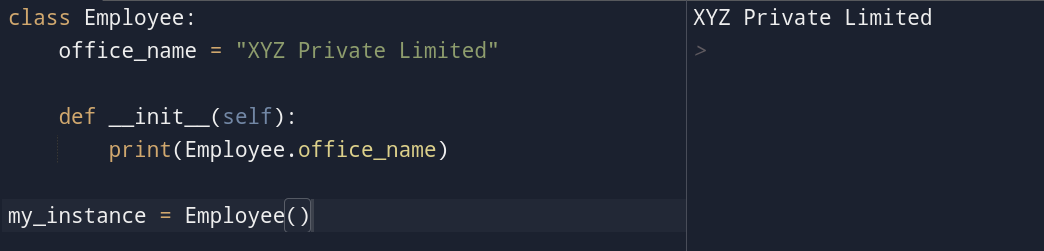
Example 2:
To access the value of the class variable within the constructor, you can use self to access the class variable instead of using the class name as shown in the following example.
class Employee:
office_name = "XYZ Private Limited"
def __init__(self, name):
self.name = name
print(self.office_name)
my_instance = Employee("Ram")
Output:
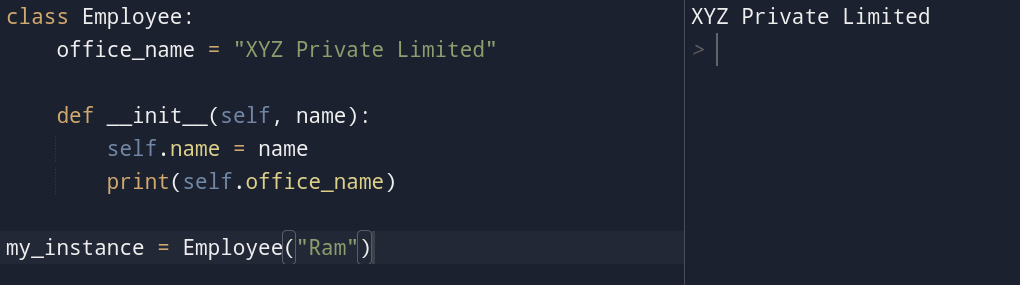
Example 3:
To access the value of the class variable, you can use the dot notation. Since the class variable is associated with a class, you don’t even need to declare an instance of the class to see its value.
class Employee:
office_name = "XYZ Private Limited"
print(Employee.office_name)
Output:

Modify value of Class Variable in Python
Like any other variables, class variables can also be changed. In Python, you can modify a class variable using the class name or an instance of the class.
Though you can modify a class variable using the class name or an instance of the class, it is important to note that modifying a class variable using an instance of the class only modifies the variable for that instance, not for all instances of the class or the class itself.
Modifying a class variable helps you to change the behaviour or attributes of all instances of the class, without having to modify each instance individually.
Example:
class Employee:
# Class variable
office_name = 'XYZ Private Limited'
# Constructor
def __init__(self, employee_name, employee_ID):
self.employee_name = employee_name
self.employee_ID = employee_ID
def show(self):
print("Name:", self.employee_name)
print("ID:", self.employee_ID)
print("Office name:", Employee.office_name)
# create Object
e1= Employee('Ram', 'T0166')
print('Before')
e1.show()
# Modify class variable
Employee.office_name = 'PQR Private Limited'
print('After')
e1.show()
Output:
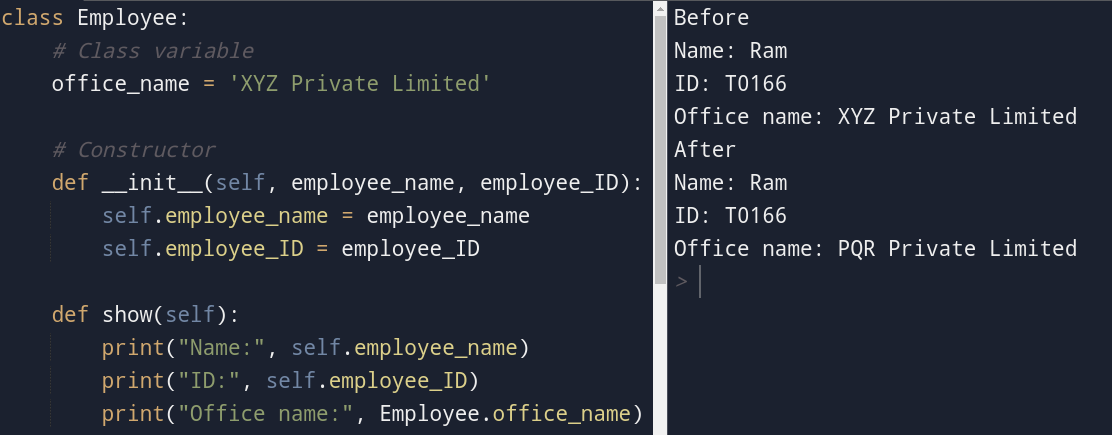
What are Python Instance Variables?
A class in which the value of the variable vary from object to object is known as instance variables. An instance variable, in object-oriented programming, is a variable that is associated with an instance or object of a class.
It holds data or state that is specific to that instance and can be accessed or modified through the instance's methods. Instance variables are typically declared within the class definition and can have different values for different instances of the same class.
Creating an Instance Variable
Here is an example of how to create an instance variable:
class Employee:
def __init__(self, name, id):
self.name = name
self.id = id
self.salary = 0
In this example, we define an Employee
class with three instance variables, name
, id
, and salary
. We set the first two instance variables, name
and id
, in the __init__
method. We also set the salary
instance variable to a default value of 0.
Accessing an Instance Variable in Python
In Python, you can access an instance variable by using the dot notation on an instance of a class. Here's an example:
class Employee:
def __init__(self, name, id):
self.name = name
self.id = id
employee1 = Employee("John Doe", 123)
employee2 = Employee("Jane Smith", 456)
print(employee1.name)
print(employee1.id)
print(employee2.name)
print(employee2.id)
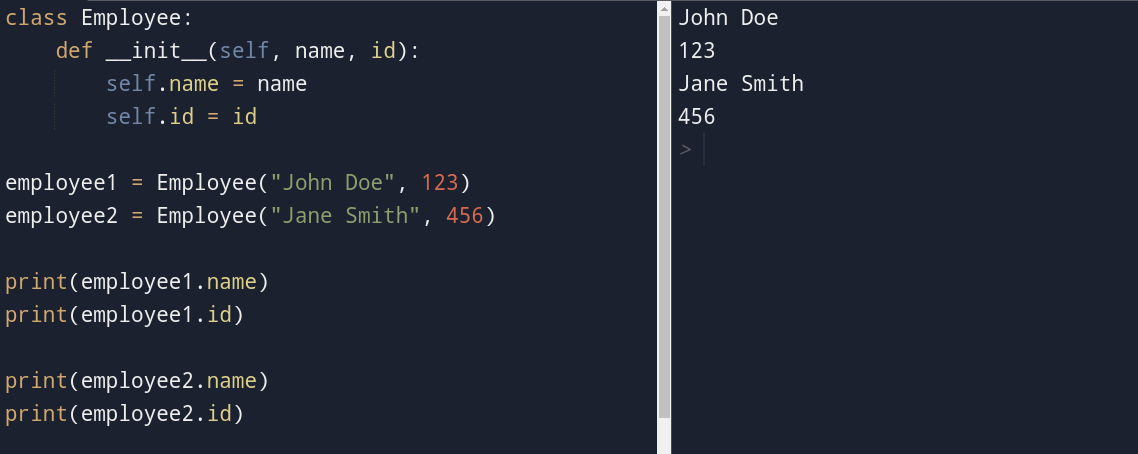
In this example, we define an Employee
class with two instance variables, name
, and id
. We then create two class instances, employee1
, and employee2
, and set their name
and id
instance variables using the init
method.
We can then access the instance variables of each employee instance using the dot notation, as demonstrated in the print statements. For example, employee1.name
returns the first employee's name, "John Doe".
Instance variables are unique to each instance of a class. This means that each instance of a class has its copy of the instance variables, and changes to one instance variable do not affect the values of the same variable in other instances of the class.
Modify value of an Instance Variable
The value of the instance variables can be changed by adding a new value to it using the object reference. It is important to note that the changes made in one in object does not reflect in any of the other objects because the instance variable is maintained separately by each object.
Example:
class Employee:
# constructor
def __init__(self, employee_name, employee_ID):
# Instance variable
self.employee_name = employee_name
self.employee_ID = employee_ID
# create object
e1 = Employee("Ram", "T0166")
print("Before")
print("Employee name:", e1.employee_name, "Employee_ID:", e1.employee_ID)
# modify instance variable
e1.employee_name = "John"
e1.employee_ID = "T0167"
Output:
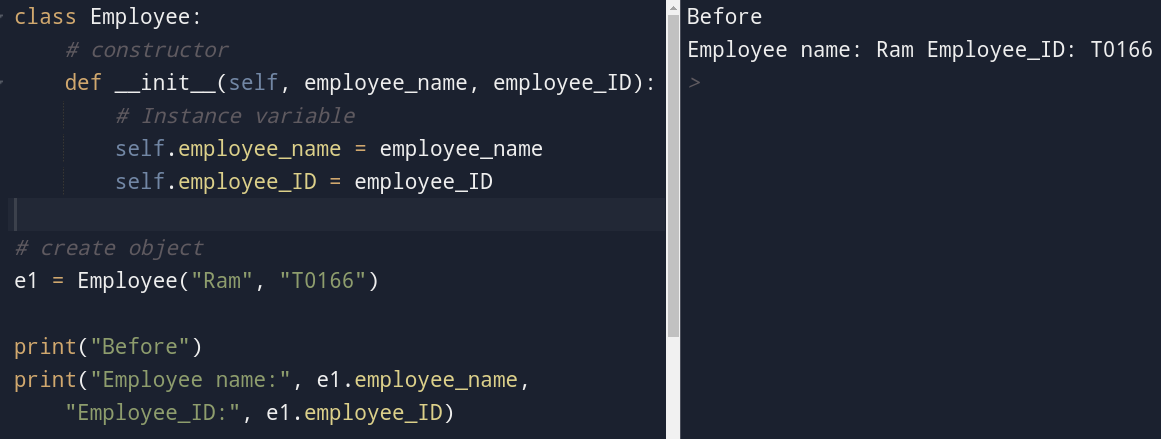
Delete an Instance Variable in Python
In Python, you can delete an instance variable by using the del statement and specifying the variable you want to delete using the dot notation.
Example 1:
class Employee:
def __init__(self, employee_name, employee_ID):
# Instance variable
self.employee_name = employee_name
self.employee_ID = employee_ID
# create object
e1 = Employee('Ram', 'T0166')
print(e1.employee_name, e1.employee_ID)
# del name
del e1.employee_name
print(e1.employee_name)
Output:
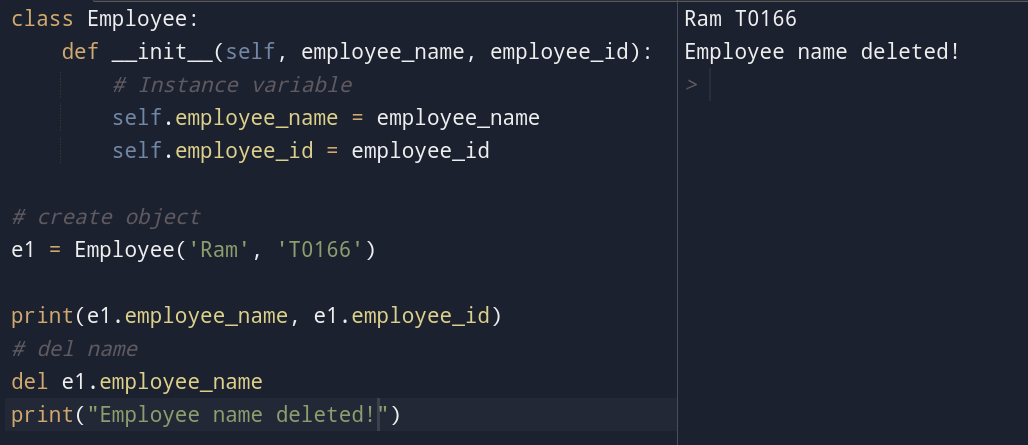
Class Variables vs Instance Variables
Parameter | Class Variables | Instance Variables |
---|---|---|
Definition | Class variables are defined within the class but outside of any class methods. | Instance variables are defined within class methods, typically the constructor. |
Scope | Changes made to the class variable affect all instances. | Changes made to the instance variable does not affect all instances. |
Initialization | Class variables can be initialized either inside the class definition or outside the class definition. | Instance variables are typically initialized in the constructor of the class. |
Access | Class variables are accessed using the class name, followed by the variable name. | Instance variables are accessed using the instance name, followed by the variable name. |
Usage | Class variables are useful for storing data that is shared among all instances of a class, such as constants or default values. | Instance variables are used to store data that is unique to each instance of a class, such as object properties. |
Illustration of Python Class and Instance variable
The class Product
has three instance variables name
, price
, and quantity
. These instance variables are initialized using the constructor __init__()
method, which takes three arguments name
, price
, and quantity
.
The class also has several methods:
getName()
,setName()
: getter and setter methods for thename
instance variable.getPrice()
,setPrice()
: getter and setter methods for theprice
instance variable.getQuantity()
,setQuantity()
: getter and setter methods for thequantity
instance variable.increaseQuantity(int amount)
: increases the quantity of the product in stock by the specified amount.decreaseQuantity(int amount)
: decreases the quantity of the product in stock by the specified amount, as long as there is enough of the product in stock.toString()
: returns aString
representation of the product, showing its name, price, and quantity in parentheses.
class Product:
def __init__(self, name, price, quantity):
# instance variable
self.name = name
self.price = price
self.quantity = quantity
def getName(self):
return self.name
def setName(self, name):
self.name = name
def getPrice(self):
return self.price
def setPrice(self, price):
self.price = price
def getQuantity(self):
return self.quantity
def setQuantity(self, quantity):
self.quantity = quantity
def increaseQuantity(self, amount):
self.quantity += amount
def decreaseQuantity(self, amount):
if (self.quantity < amount):
print("Not enough " + self.name + " in stock")
else:
self.quantity -= amount
def toString(self):
return self.name + ": $" + str(self.price) + " (" + str(self.quantity) + ")"
bread = Product("Bread", 2.99, 10)
milk = Product("Milk", 3.99, 5)
# display initial state of the products
print("Initial state of the products:")
print(bread.toString())
print(milk.toString())
# sell some bread and milk
bread.decreaseQuantity(3)
milk.decreaseQuantity(2)
# display updated state of the products
print("State of the products after selling some:")
print(bread.toString())
print(milk.toString())
# add more bread and milk to the inventory
bread.increaseQuantity(5)
milk.increaseQuantity(3)
# display final state of the products
print("Final state of the products:")
print(bread.toString())
print(milk.toString())
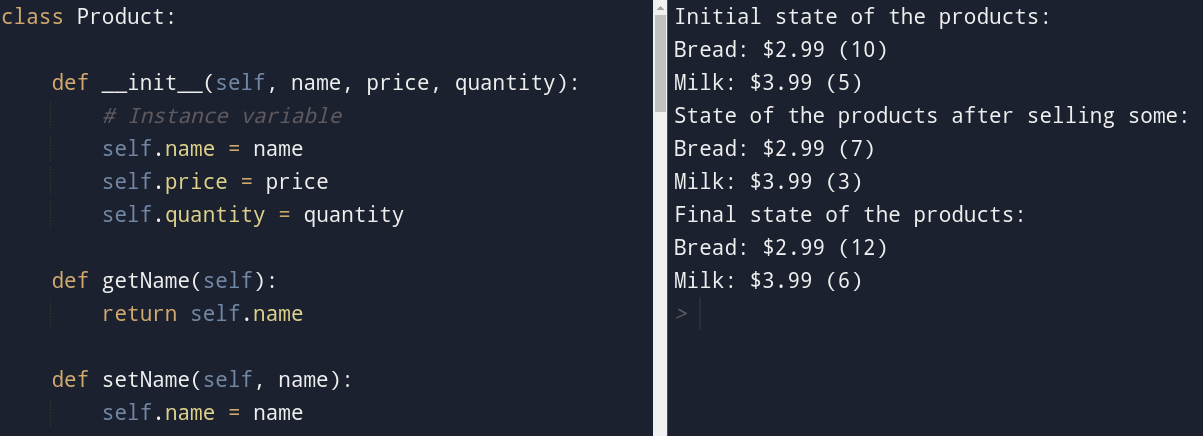
We also create two instances of the Product
class: bread
and milk
. It then displays the initial state of these products using the toString()
method.
Next, we sell some bread and milk by calling the decreaseQuantity()
method, and displays the updated state of the products.
Finally, we add more bread and milk to the inventory using the increaseQuantity()
method, and displays the final state of the products.
Conclusion
Class variables and instance variables are both important concepts in object-oriented programming. Class variables are shared across all instances of a class, while instance variables are unique to each instance.
The choice between using class variables or instance variables depends on the specific needs of the program. If a variable needs to be shared across all instances of a class, then a class variable is appropriate. On the other hand, if a variable needs to be unique to each instance, then an instance variable is the way to go.
To create robust and maintainable object-oriented programs, it is important to have a clear understanding of the distinctions between class variables and instance variables.
Selecting the appropriate variable type for each use case is critical to achieve efficiency, flexibility, and effectiveness in the code. Hence, developers must be meticulous in their choice of variable types to build successful object-oriented programs.
Atatus: Python Performance Monitoring
Atatus is an Application Performance Management (APM) solution that collects all requests to your Python applications without requiring you to change your source code. However, the tool does more than just keep track of your application's performance.
Monitor logs from all of your Python applications and systems into a centralized and easy-to-navigate user interface, allowing you to troubleshoot faster using Python monitoring.
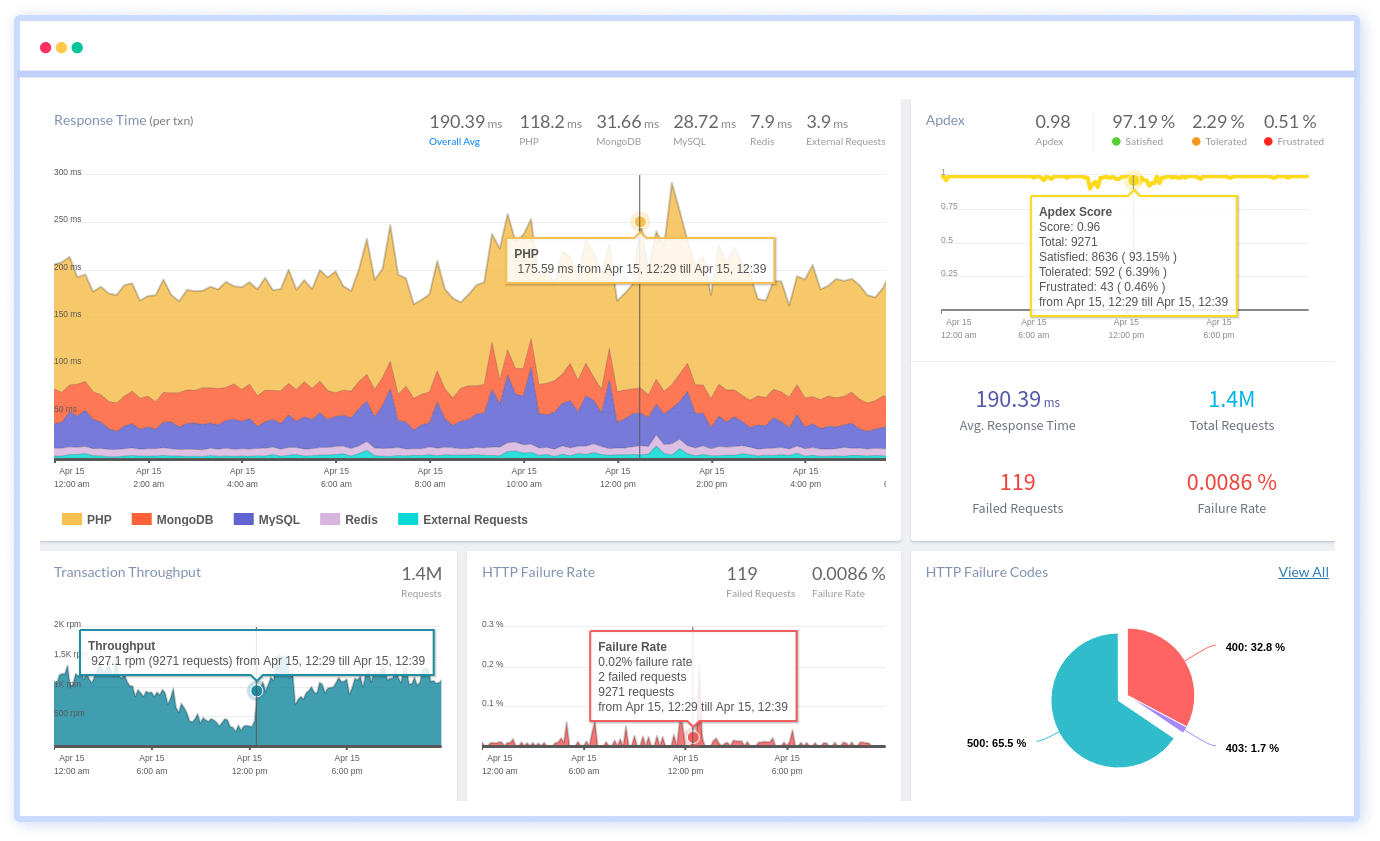
We give a cost-effective, scalable method to centralized Python logging, so you can obtain total insight across your complex architecture. To cut through the noise and focus on the key events that matter, you can search the logs by hostname, service, source, messages, and more. When you can correlate log events with APM slow traces and errors, troubleshooting becomes easy.
#1 Solution for Logs, Traces & Metrics
APM
Kubernetes
Logs
Synthetics
RUM
Serverless
Security
More