Guide to Using Break and Continue Statements in Python
Do you find yourself frustrated with loops that never seem to end? Do you find yourself wishing you could skip certain iterations or exit a loop prematurely?
Python's break and continue statements are here to save you!
One of the key features of Python is its ability to control the flow of code using control flow statements. Two of the most commonly used control flow statements in Python are break and continue.
With these statements, you can take control of your loops and make your code more efficient than ever before. These statements are used to alter the behaviour of loops, allowing developers to break out of a loop prematurely or skip certain iterations of a loop based on the specific conditions.
In this blog, we'll explore the functionality of break and continue, and how they can be used to make your Python code more effective.
Table of contents
- What is a Loop in Programming?
- Python Break Statement
- Python Continue Statement
- Use Cases of Break and Continue Statements
What is a Loop in Programming?
In programming, loops are commonly used to repeatedly execute a set of instructions or iterate through a collection of data. There are two main types of loops in python.
- for loops
- while loops
Here is a simple example to understand the loop concept:
Consider a scenario in which you are a teacher and you need to determine the overall performance of students. You have a list of each student's scores, and you want to calculate the average score by adding up all the scores and dividing it by the total number of students. You could use a loop to iterate over the list of scores and add up all the scores.
Python Break Statement
In Python, the break statement is used to immediately terminate a loop, regardless of whether the loop has finished iterating over all its items.
When a break statement is encountered within a loop, the program execution immediately exits the loop and continues with the statement immediately following the loop. The conditional statement, such as an if statement is used in combination with break statement to exit the loop when a certain condition is met.
The break statement is typically used with one of the following three types of loops in python : for loops, while loops, and do-while loops.
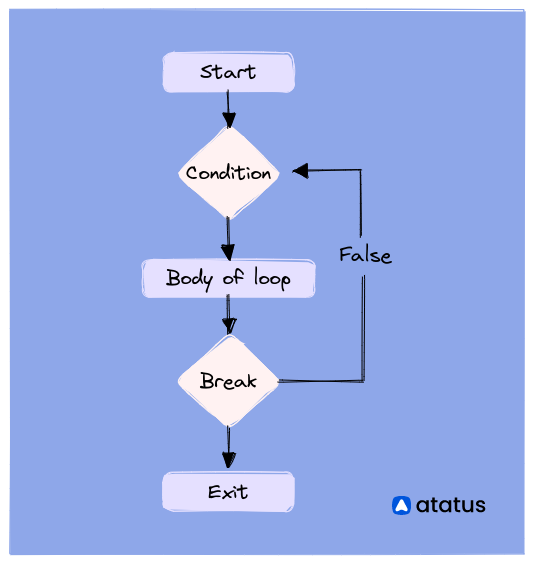
1. Execution Flow
At the beginning of each iteration of the loop, the program evaluates the condition of the decision box.
If the condition is true, the program executes the statement within the loop and then jumps back to the decision box to evaluate the condition again.
If the condition is false, the program skips over the statements within the loop and jumps to the statement immediately following the loop.
If the break statement is encountered within the loop, regardless of whether the condition of the decision box is true or false, the program immediately exits the loop and continues with the statement following the loop.
2. Break Statement inside for Loop
Here's an example of using the break statement with a for loop in Python:
Example:
for i in range(1, 11):
if i == 5:
break
print(i)
Output:

In this example, there is a condition given inside the loop. The for loop iterates through the number and checks if the current number i is equal to 5.
If i equals 5, the break statement is triggered, which immediately stops the loop from continuing to the next iteration.
As a result of the break statement being executed, the loop ends prematurely when the value of i becomes 5. Only the numbers from 1 to 4 are printed, since the loop has been terminated before it could continue to print the remaining numbers.
A for loop is a suitable option when you have a sequence of elements that you want to iterate over individually, one after the other.
3. Break Statement inside while Loop
Here's an example of using the break statement with a while loop in Python:
Example:
password = ""
while True:
password = input("Enter your password: ")
if password == "d8H^ku?@4!":
print("Password accepted.")
break
else:
print("Incorrect password. Try again.")
In this example, a while loop is used with an infinite condition(True) to keep asking the user for a password until the user enter the correct password. Inside the loop, an if statement is used to check if the entered password matches the correct password .
If the password entered by the user matches with the correct password, then the program print a message indicating that the password has been accepted and the loop is exited using the break statement.
If the user enters any other password, the loop will continue to ask for a password until the user enter the correct password.
A while loop is a good choice when you want to repeat a set of instructions until a certain condition is met, and you don't know how many times the loop will need to run before that happens.
4. Benefits of Break Statement
- Improves efficiency: When processing large amounts of data or performing a time-consuming operation, using the
break
statement can help you optimize your code by exiting the loop as soon as you have found what you are looking for. This saves you time and computing resources that would otherwise be wasted on unnecessary iterations. - Simplifies code: Using the
break
statement can help you simplify your code by allowing you to eliminate unnecessary code blocks that would be needed to achieve the same result without thebreak
statement. - Increases readability: Code that uses the
break
statement is often more readable and easier to understand than code that uses complex logic to achieve the same result. This is because thebreak
statement provides a clear and concise way to communicate the intent of the code. - Reduces the risk of errors: By allowing you to exit a loop immediately when a certain condition is met, the
break
statement reduces the risk of logic errors that can occur when a loop continues iterating unnecessarily.
5. Limitations of Break Statement
- Using break can make your code harder to read and debug, especially if it is used in complex ways.
- In some cases, using break can lead to unexpected errors, such as infinite loops or off-by-one errors.
Python Continue Statement
In Python, the continue statement is used inside loops such as for or while loops to skip over the current iteration of the loop and move on to the next iteration. It can only be used inside a loop (for loop or while loop) and is usually accompanied by a conditional statement.
When the continue statement is executed within a loop, the code inside the loop will immediately stop executing for the current iteration, and the loop will jump to the next iteration.
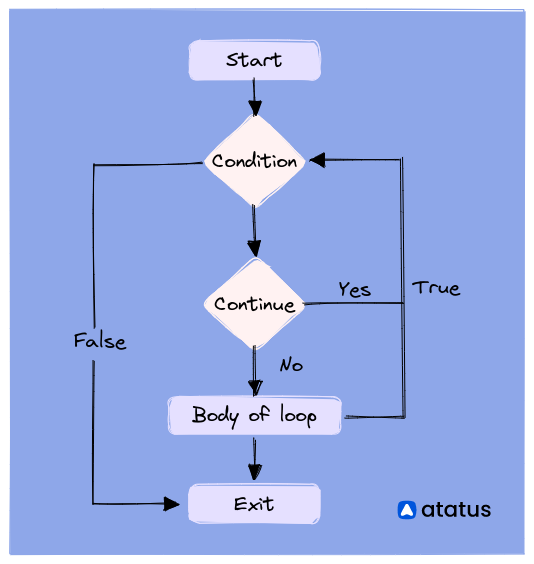
1. Execution Flow
The loop begins to iterate over each item in case of for loop or executes the loop body repeatedly in case of while loop. When a continue statement is encountered within the loop body, the execution of the loop body for the current iteration stops immediately.
The loop immediately moves on to the next iteration, skipping any remaining code for the current iteration. If the loop condition is no longer true, the loop terminates. If there are more items or the loop condition is still true, the loop body is executed again for the next iteration.
2. Continue Statement inside for Loop
Here's an example of using the continue statement with a for loop in Python:
Example:
for i in range(1, 11):
if i == 5:
continue
print(i)
Output:
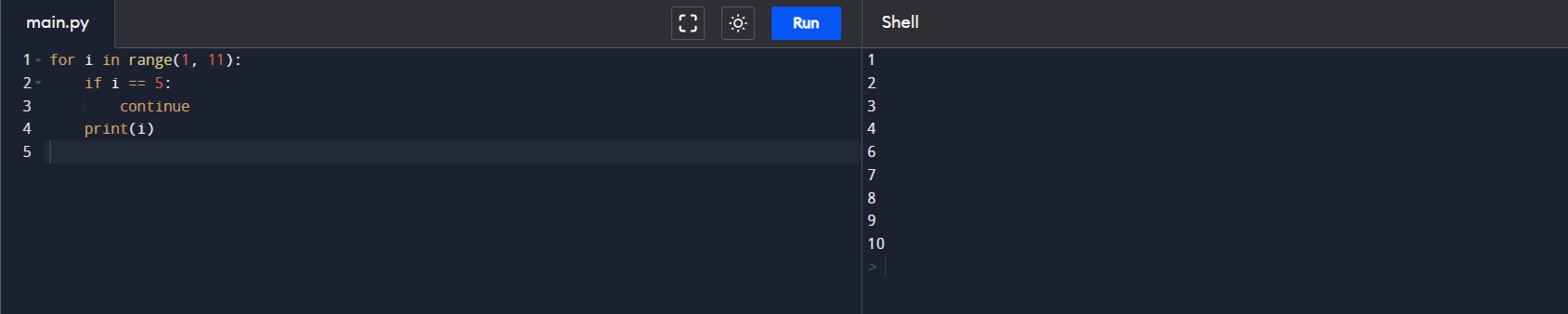
In the given example, the for loop iterates from 1 to 10 as per the range given in the code.
When the loop variable i equals 5, the continue statement is triggered, causing the program to skip the remaining statements inside the loop for that specific iteration. The loop then moves on to the next iteration and continues until it has iterated through all the values in the range.
3. Continue Statement inside while Loop
Here's an example of using the continue statement with a while loop in Python:
Example:
i = 1
while i <= 10:
if i % 2 == 0:
i += 1
continue
print(i)
i += 1
Output:

The above code example shows the use of the continue statement in a while loop. Initially, i is set to 1 and the while loop continues until i is less than or equal to 10.
Whenever the value of i is even, the continue statement is executed and the statements that come after it (in this case, the print(i) statement) are skipped. The loop then moves on to the next iteration and continues until it has iterated through all the values until 10.
4. Benefits of Continue Statement
- Increases maintainability: By allowing you to skip over specific iterations of a loop, the continue statement can make it easier to add or modify code inside the loop without affecting the overall logic of the loop.
- Reduces nesting: By allowing you to skip over specific iterations of a loop, the continue statement can help you reduce the number of nested if statements that you need to use in your code. This can make your code more readable and easier to maintain.
- Improves performance: When processing large amounts of data or performing a time-consuming operation, using the continue statement can help you optimize your code by skipping over iterations that are not necessary. This saves you time and computing resources that would otherwise be wasted on unnecessary iterations.
- Enhances code clarity: The continue statement can help make your code more evident by explicitly indicating that specific iterations of the loop should be skipped. This can make it easier for other developers to understand and modify your code in the future.
5. Limitations of Continue Statement
- Limited to loops: The
continue
statement can only be used within loops such as for loops, while loops, and do-while loops. It cannot be used outside of a loop or in a function. - Can lead to infinite loops: Using the
continue
statement incorrectly can cause an infinite loop, where the loop never terminates. For example, if you accidentally place thecontinue
statement outside of the conditional statement in a loop, it will skip every iteration of the loop and continue indefinitely. - Can make code harder to read: While the
continue
statement can improve the readability of some code, it can also make code more difficult to read if used excessively or inappropriately. It can also make it harder to understand the overall flow of the loop. - Can be overused: The
continue
statement should be used judiciously, as overuse can lead to overly complex code that is difficult to maintain. In some cases, it may be better to use a different control flow statement, such asif-else
statements or nested loops.
Use Cases of Break and Continue Statements
Here are some examples of how to use the break
and continue
statements in Python:
1. Break
- Terminating a loop when a certain condition is met:
Example 1:
for i in range(1, 11):
if i == 5:
break
print(i)
# Output: 1 2 3 4
Example 2:
fruits = ['apple', 'banana', 'cherry', 'orange', 'kiwi']
for fruit in fruits:
if fruit == 'orange':
break
print(fruit)
# Output: apple banana cherry
In both examples, the loop is terminated when a certain condition is met. In Example 1, the loop stops when i
is equal to 5, while in Example 2, the loop stops when the fruit
variable is equal to 'orange'.
- Ending a program when an error occurs or a certain condition is met:
Example 1:
for i in range(1, 11):
if i == 5:
print('An error has occurred.')
break
print(i)
# Output: 1 2 3 4 An error has occurred.
Example 2:
while True:
response = input('Enter your name (or "quit" to exit): ')
if response == 'quit':
print('Exiting program.')
break
print(f'Hello, {response}!')
In Example 1, the loop is terminated and an error message is printed when i
is equal to 5. In Example 2, the program is ended when the user enters the word 'quit' at the prompt.
2. Continue
- Skipping over certain items in a loop:
Example 1:
for i in range(1, 11):
if i % 2 == 0:
continue
print(i)
# Output: 1 3 5 7 9
Example 2:
fruits = ['apple', 'banana', 'cherry', 'orange', 'kiwi']
for fruit in fruits:
if len(fruit) > 6:
continue
print(fruit)
# Output: apple kiwi
In both examples, the continue
statement is used to skip over certain items in the loop. In Example 1, the loop skips over even numbers, while in Example 2, the loop skips over fruits that have more than 6 characters in their name.
- Handling errors or special cases in a loop:
numbers = [1, 2, 3, 4, 5, 6, 7, 8, 9, 10]
for number in numbers:
if number == 7:
print('This number is not allowed.')
continue
print(number)
# Output: 1 2 3 4 5 6 This number is not allowed. 8 9 10
In the above example, the loop skips over the number 7, which is not allowed in the sequence.
Conclusion
In summary, the break and continue statements are valuable tools that can simplify your Python loops and make your code more efficient. The break statement lets you exit a loop early if a specific condition is met, saving time and resources by avoiding unnecessary iterations. The continue statement skips over certain iterations, allowing you to execute only specific parts of the loop.
By using these statements carefully, you can make your code more readable and effective. However, it's important to use them appropriately to avoid confusing code. With a good understanding of these statements, you can create more effective loops and write better Python code.
Atatus: Python Performance Monitoring
Atatus is an Application Performance Management (APM) solution that collects all requests to your Python applications without requiring you to change your source code. However, the tool does more than just keep track of your application's performance.
Monitor logs from all of your Python applications and systems into a centralized and easy-to-navigate user interface, allowing you to troubleshoot faster using Python monitoring.
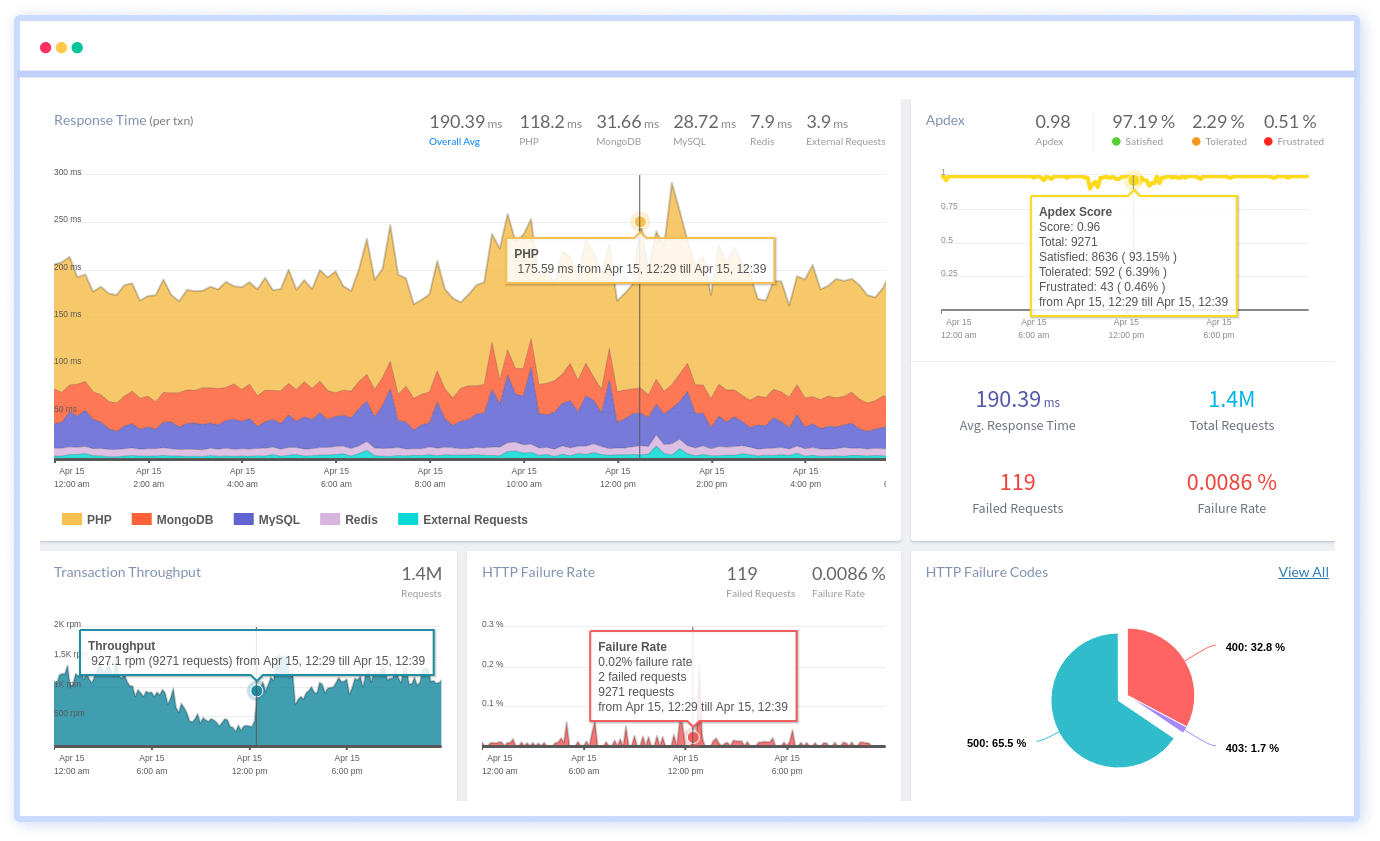
We give a cost-effective, scalable method to centralized Python logging, so you can obtain total insight across your complex architecture. To cut through the noise and focus on the key events that matter, you can search the logs by hostname, service, source, messages, and more. When you can correlate log events with APM slow traces and errors, troubleshooting becomes easy.
#1 Solution for Logs, Traces & Metrics
APM
Kubernetes
Logs
Synthetics
RUM
Serverless
Security
More