Read/Write a JSON file with Node.js
JSON (JavaScript Object Notation) is a lightweight data-interchange format. It is easy for humans to read and write. It is easy for machines to parse and generate.
JSON is a text format that is completely language independent but uses conventions that are familiar to programmers of the C-family of languages (C, C++, JavaScript, and so on). These properties make JSON an ideal data-interchange language.
If you're working with Node.js, chances are you'll need to read and write data to a JSON file at some point.
Imagine you have a purchase_history.json
file that contains a record of customer purchase order information.
{
"name": "John Smith",
"purchase_id": "20223",
"price": "$23.95",
"shipping_address": {
"name": "Jane Smith",
"address": "123 Maple Street",
"city": "Pretendville",
"state": "NY",
"zip": 10005
},
"billing_address": {
"name": "John Smith",
"address": "123 Maple Street",
"city": "Pretendville",
"state": "NY",
"zip": 10005
},
"is_reverse_charge_applied": false,
"warehouse": [
{
"warehouse_name": "ABC Warehouse",
"warehouse_id": 143
}
]
}
In this post, we'll show you how to read, write and update data in the JSON file with Node.js using the fs-module
.
Table of Contents
Reading a JSON file
There are two different methods to read the above purchase_history.json
JSON file in Node.js.
Method 1: Using require() or import() method
In Node.js, the require keyword is used to import external modules. Modules are JavaScript files that export functions and objects. The require()
is used to import these modules.
The require() function takes an argument, which is the name of the module. The module name should be a string. The require() function will return an object, which is the module.
This is one of the simplest way to read and parse a JSON object.
const data = require("path/to/file/filename");
A JSON file can be synchronously read and parsed into JavaScript objects by passing require() with its path.
const data = require("home/json/purchase_history.json");
console.log(data)
There is a disadvantage of using the require() function, besides its simplicity:
Since require()
caches the result, changes to the .json
file won't be visible when reading JSON more than once, unless the result is explicitly cleared from the cache.
In node.js, the process of importing a file using the import keyword is a relatively simple one. In order to do so, all you need to do is use the import keyword followed by the path to the file you wish to import.
For example, if you were to import a file named purchase_history.json
from the same directory as the file in which you were working, you would use the following code:
import { purchase_history } from "purchase_history.json";
Method 2: Using Node.js fs module
Node JS provides a built in module, fs
, that allows you to interact with the file system in your environment. There are two function available in fs
module:
The major differences between fileReadSync()
and fileRead()
:
fileReadSync() | fileRead() |
Blocking process | Non-Blocking process |
Uses variable to store content | Uses callback |
Synchronous nature | Asynchronous nature |
The fs
module can be imported using the following syntax:
var fs = require("fs")
i.) Using fs.readFileSync
This function allows you to read a file synchronously, which can be extremely helpful when you need to read a large file or do some processing on the data.
When the fs.readFileSync()
method is called the original node program stops executing, and node waits for the fs.readFileSync()
function to get executed, after getting the result of the method the remaining node program is executed.
Syntax:
fs.readFileSync( path, options )
Parameters:
path
- relative path of the file that you wish to read, it can be of URL type or file descriptor.options
- this is an optional parameter which contains the encoding format of the file content to read, by default it is set to null.
const { readFileSync } = require("fs");
const path = "home/json/purchase_history.json";
const jsonString = readFileSync(path);
console.log(JSON.parse(jsonString));
In the above code snippet, we have read the purchase_history.json
file using the readFileSync
function.
ii.) Using fs.readFile
The fs.readFile
function reads a file asynchronously, meaning that it will not block the execution of your program while it is reading the file. The data is read as a buffer, which is an array of bytes.
The fs.readFile
function takes three arguments: the path of the file to read, encoding-type, a callback function that will be called with the contents of the file.
Syntax:
fs.readFile( filename, encoding, callback_function )
The above function returns the data stored in the JSON file.
Example:
const fs = require("fs");
const path = "home/json/purchase_history.json";
fs.readFile(path, "utf8", (err, jsonString) => {
if (err) {
console.error(err);
return;
}
console.log(jsonString);
});
The encoding_type parameter is an optional parameter, if it is not specified the fs.readFile
function will return buffer instead of string.
The (err, jsonString) => {}
callback function is called after the file is read, and if the function is executed correctly, the JSON string is returned. If the function failed, an error will be returned.
To use the JSON string in the Node.js code you will have to parse it. You can parse it using the JSON.parse()
function.
const fs = require("fs");
const path = "home/json/purchase_history.json";
fs.readFile(path, "utf8", (err, jsonString) => {
if (err) {
console.log("Error reading the JSON file:", err);
return;
}
try {
const purchase_hist = JSON.parse(jsonString);
console.log(purchase_hist);
} catch (err) {
console.log("Error parsing JSON string:", err);
}
});
const path = "home/json/purchase_history.json";
Write to a JSON file
The fs
module does provide built-in methods to write JSON files. Similar to the read methods it also has two functions to write JSON file:
We should create a JSON string before writing it to a file with the JSON.stringify
function. The same way we parse data into an object when reading a file, we must turn our data into a string to write it.
JSON.stringify(value, replacer, space)
Parameters:
value
- It is the value to be converted to a JSON string.replacer
- It is an optional parameter. As a filter, this parameter value can be either a function or an array. If the value is empty or null then all properties of an object are included in a string.space
- It is also an optional parameter. The JSON.stringify() function uses this argument to control spacing in its final output. It can be a number or a string. If a number is specified, the specified number of spaces is indented, and if it is a string, the string is indented (up to 10 characters).
i.) Using fs.writeFileSync
fs.writeFileSync
is a function in Node.js that allows for writing data to a file in a synchronous manner. This can be useful when you need to ensure that data is written to a file before continuing on with other tasks.
Syntax:
fs.writeFileSync( path, data, options )
Parameters:
path
- The path is a string, buffer, URL, or file description integer that specifies where the file should be written. The method will behave similarly to fs.write() if a file descriptor is used.data
- The data to be written to the file can be a string, buffer, typed array, or dataview.options
- The options parameter is a string or object that specifies optional parameters that will affect the output. There are three optional parameters:
encoding : A file's encoding is specified by this string. It is set to 'utf8' by default.
mode : A file mode is specified by an integer. 0o666 is the default value.
flag: It is a string which specifies the flag used while writing to the file. The default value is ‘w’.
const { writeFileSync } = require("fs");
const path = "home/json/purchase_history.json";
const purchase_hist = {"name":"John Smith","purchase_id":"20223","price":"$23.95","shipping_address":{"name":"Jane Smith","address":"123 Maple Street","city":"Pretendville","state":"NY","zip":10005},"billing_address":{"name":"John Smith","address":"123 Maple Street","city":"Pretendville","state":"NY","zip":10005},"is_reverse_charge_applied":false,"warehouse":[{"warehouse_name":"ABC Warehouse","warehouse_id":143}]};
try {
writeFileSync(path, JSON.stringify(purchase_hist, null, 2), "utf8");
console.log("Data successfully saved");
} catch (error) {
console.log("An error has occurred ", error);
}
ii.) Using fs.writeFile
fs.writeFile is a function in Node.js that allows you to write data to a file. This function is asynchronous and takes two arguments:
path
- The path of the file to write to and the data to write.data
- It can be a string, a Buffer, or an integer. If you pass a string, it will be written to the file as-is. If you pass a Buffer, the contents of the buffer will be written to the file. If you pass an integer, it will be written to the file as a 32-bit integer.
const { writeFile } = require("fs");
const path = "home/json/purchase_history.json";
const purchase_hist = {"name":"John Smith","purchase_id":"20223","price":"$23.95","shipping_address":{"name":"Jane Smith","address":"123 Maple Street","city":"Pretendville","state":"NY","zip":10005},"billing_address":{"name":"John Smith","address":"123 Maple Street","city":"Pretendville","state":"NY","zip":10005},"is_reverse_charge_applied":false,"warehouse":[{"warehouse_name":"ABC Warehouse","warehouse_id":143}]};
writeFile(path, JSON.stringify(purchase_hist, null, 2), (error) => {
if (error) {
console.log("An error has occurred ", error);
return;
}
console.log("Data written successfully to the file");
});
How to update data in a JSON file?
When working with JSON files, it is often necessary to append new data to the file. This cannot be accomplished using the built-in JSON object in JavaScript.
Now we are going to update the name of the "shipping_address" address in the below JSON file from "Jane Smith" to "John Smith".
{
"name": "John Smith",
"purchase_id": "20223",
"price": "$23.95",
"shipping_address": {
"name": "Jane Smith",
"address": "123 Maple Street",
"city": "Pretendville",
"state": "NY",
"zip": 10005
},
"billing_address": {
"name": "John Smith",
"address": "123 Maple Street",
"city": "Pretendville",
"state": "NY",
"zip": 10005
},
"is_reverse_charge_applied": false,
"warehouse": [
{
"warehouse_name": "ABC Warehouse",
"warehouse_id": 143
}
]
}
The following steps will show you how to update data to a JSON file.
It is first necessary to read and parse the data, and then we can write it into the file. We can read and write using the fs
module.
const { writeFile, readFile } = require("fs");
Here we have used the readFile()
method from fs module to read the file to update the new JSON data.
readFile(path, (error, data) => {
if (error) {
console.log(error);
return;
}
console.log(data)
Before using the data directly from the callback function, you must turn it into a JavaScript object. You can parse the JSON data using the JSON.parse
method. Otherwise, we would just have a string of data with properties we can’t access.
const parsedData = JSON.parse(data);
Now we can update the data in the parsed JSON data. We have update the name from "Jane Smith" to "John Smith". You can access the key "name" inside the object as shown below:
parsedData.shipping_address.name = "John Smith";
To write it in the file, we will have to stringify the new JSON data using the JSON.stringify
method and write the appended data in the JSON file, here we have used the writeFile
method.
writeFile(path, JSON.stringify(parsedData, null, 2), (err) => {
if (err) {
console.log("Failed to write updated data to file");
return;
}
The complete snippet is as follows:
const { writeFile, readFile } = require("fs");
const path = "home/json/purchase_history.json";
readFile(path, (error, data) => {
if (error) {
console.log(error);
return;
}
console.log(data)
const parsedData = JSON.parse(data);
// updating name in shipping_address
parsedData.shipping_address.name = "John Smith";
writeFile(path, JSON.stringify(parsedData, null, 2), (err) => {
if (err) {
console.log("Failed to write updated data to file");
return;
}
console.log("Updated file successfully");
});
});
Conclusion
To conclude, JSON is a way to serialise data, which facilitates data exchange. It is a simple, text-based, self-describing data format that is generally used for storing data on the Web. JSON is supported by most modern web browsers and programming languages. t is an extensible format, including a number of optional features to allow further customization of behavior.
The above tutorial will help you read JSON data from a file and process those records with Node.js. You will learn how to read and write the JSON data with the fs
module.
The fs
module helps us accessing various file system-based operations. The JSON module reads JSON files and writes JSON files. It serializes objects to JSON, and unserializes JSON text from files.
We have discussed how to use fs.readFile
and fs.writeFile
to asynchronously and fs.readFileSync and fs.writeFileSync
to synchronously work with the filesystem, parsing data into and out of JSON format and updating the JSON content.
Monitor Your Node.js Applications with Atatus
Atatus keeps track of your Node.js application to give you a complete picture of your clients' end-user experience. You can determine the source of delayed response times, database queries, and other issues by identifying backend performance bottlenecks for each API request.
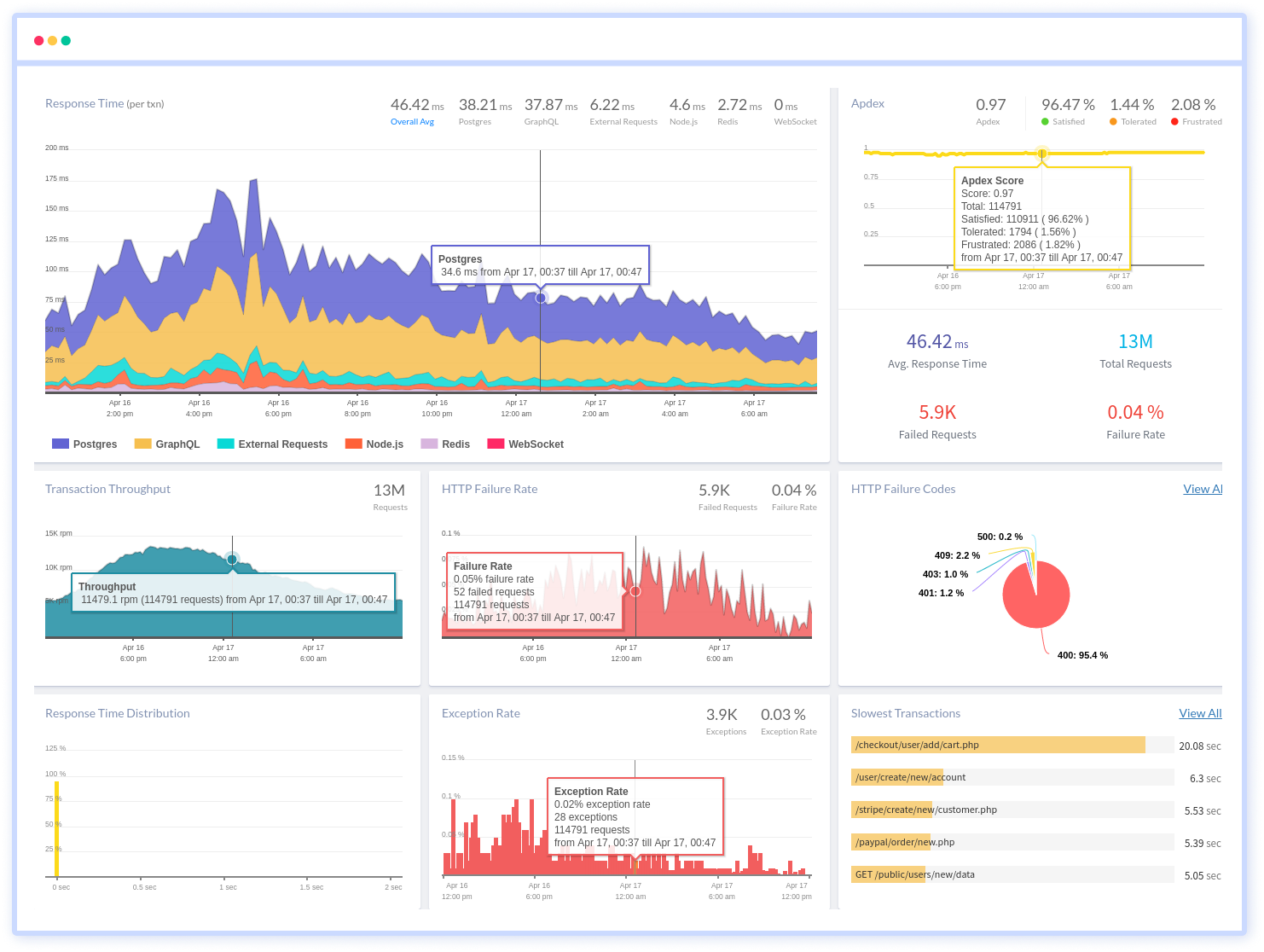
Node.js performance monitoring made bug fixing easier, every Node.js error is captured with a full stack trace and the specific line of source code marked. To assist you in resolving the Node.js error, look at the user activities, console logs, and all Node.js requests that occurred at the moment. Error and exception alerts can be sent by email, Slack, PagerDuty, or webhooks.
#1 Solution for Logs, Traces & Metrics
APM
Kubernetes
Logs
Synthetics
RUM
Serverless
Security
More