REST Client Made Easy: Exploring Top Libraries Across Languages
REST Client is like a messenger that helps applications talk to a server that provides a RESTful API. It handles tasks such as sending requests for data, updating resources, creating new records, or deleting existing ones.
Imagine you need to retrieve information from a remote server or update data in a database. REST clients act as intermediaries, helping your application send requests to the server and receive responses back. They handle the complexities of communication, such as making HTTP requests, managing headers, and handling authentication, so you can focus on utilizing the data in your application.
In this blog, we'll dive into the concept of REST clients and explore their role in modern application development. Let's begin!
Table of Contents
- What is a REST Client?
- What does the REST Client Do?
- Characteristics of REST
- Structure of REST Client Request
- REST Client Tools
- Popular REST Client Libraries
- Benefits of REST Client
What is a REST Client?
REST(REpresentational State Transfer) client is a software application or a tool that interacts with a RESTful web service. It initiates HTTP requests to the server, specifying the desired action and resource, and receives HTTP responses containing the requested data or status information.
REST is an architectural style that establishes a standardized approach for communication between clients and servers using stateless HTTP. It is not a programming language like HTML or XML, but rather a set of principles and guidelines that API developers adhere to when interacting with other RESTful APIs.
Imagine you have a weather app on your phone. When you open the app and ask for the weather of your location, the app acts as a REST client. It sends a request to a weather service's server, asking for the current weather information.
The server processes the request, gathers the weather data, and sends it back as a response. The REST client app receives the response and displays the weather details on your phone. The app and the weather service communicate using HTTP requests and responses, following the REST principles.
What does the REST Client Do?
The primary function is to send HTTP requests to the server, specifying the desired action (such as retrieving data or performing an operation) and the resource involved. The REST client then receives the HTTP responses from the server, which contain the requested data or status information.
These requests define the desired action to be performed on a resource in the server's database. There are four main types of requests that a client can issue:
- GET Request: This request is used to retrieve information from the server. It reads the data of a specific resource or a collection of resources. For example, a GET request can be used to fetch details about a book from an online library.
- PUT Request: This request is used to update or modify the information of a specific resource in the server's database. It changes the existing data according to the provided request payload. For example, a PUT request can be used to update the title or author of a book in a library's catalog.
- POST Request: This request is used to create a new resource in the server's database. It submits data to the server to be stored as a new record. For example, a POST request can be used to add a new customer to an online store's customer database.
- DELETE Request: This request is used to remove a specific resource from the server's database. It deletes the corresponding record, making it no longer accessible. For example, a DELETE request can be used to remove a user account from a social media platform.
Learn how to optimize your API by incorporating efficient filtering, sorting, and pagination strategies.
Characteristics of REST
REST defines a set of characteristics that contribute to its unique nature. The key characteristics of REST are:
- It is stateless, meaning that each request from a client to a server contains all the necessary information for the server to understand and process the request. The server does not store any session state between requests, which improves scalability and simplifies communication.
- It consists of a set of well-defined HTTP methods (GET, POST, PUT, DELETE) for performing different actions on resources.
- It separates the client and server components, allowing them to evolve independently. The client is responsible for the user interface and user interactions, while the server handles data storage, processing, and management. This improves scalability by simplifying server components.
- It is a layered system, ensuring that clients are unaware of any intermediaries between them and the server. This means that the presence of load balancers or proxies does not affect the communication between the client and server. No modifications to the client or server code are required to accommodate these intermediaries.
- HATEOAS(Hypermedia as the Engine of Application State) is a key principle in REST where responses contain links or hypermedia controls that guide clients on the available actions and transitions. By following these links, clients can navigate and interact with the API without prior knowledge of its structure.
Structure of REST Client Request
RESTful APIs have become the backbone of modern web applications, allowing different software systems to interact and exchange data. To effectively utilize RESTful APIs, it is essential to understand the role of a REST client and how it enables communication with these services. It follows a specific pattern to communicate the desired action and associated data. Here's an explanation of the key elements:
- HTTP Method: The request starts with specifying the HTTP method, which indicates the type of action to be performed on the server. The commonly used methods are GET, POST, PUT, and DELETE. Each method has a different purpose: GET for retrieving data, POST for creating new data, PUT for updating data, and DELETE for removing data.
- Request URL: The URL (Uniform Resource Locator) identifies the specific resource or endpoint on the server that the request is targeting. It helps the server understand which data or functionality the client wants to interact with.
- Headers: Headers provide additional information about the request, such as the content type, authentication credentials, or caching directives. They convey important instructions or metadata related to the request.
- Request Body (Optional): For some requests, such as POST or PUT, a request body can be included. It contains the data that the client wants to send to the server, typically in formats like JSON or XML. The body carries information needed for creating or updating resources.
- Query Parameters (Optional): Query parameters are appended to the URL and provide additional instructions or filtering criteria for the request. They help narrow down the desired data or control the behaviour of the server.
Here's an example of the structure of a REST client request:
POST /api/users
Content-Type: application/json
Authorization: Bearer YOUR_TOKEN
{
"name": "John Doe",
"email": "john@example.com"
}
- The method is POST, indicating that a new resource will be created.
- The URL is
/api/users
, representing the endpoint for creating user resources. - The request includes headers like Content-Type (set to
application/json
) and Authorization (using a bearer token for authentication). - The request body contains JSON data representing the user's name and email.
REST Client Tools
REST client libraries and tools play a crucial role in simplifying the development and interaction with RESTful APIs. They provide utilities, and pre-built functionality to handle HTTP communication and facilitate the consumption of RESTful services. Here are some popular REST client libraries and tools:
1. cURL
cURL is a command-line tool for making HTTP requests. It is widely used and supports various protocols, including HTTP, HTTPS, and more. It provides a straightforward way to send requests and receive responses from RESTful APIs.
Here are a few examples of APIs you can interact with using cURL:
GitHub API:
curl -X GET -H "Authorization: Bearer YOUR_TOKEN" "https://api.github.com/user/repos"
This example retrieves the list of repositories for the authenticated user on GitHub.
Twitter API:
curl -X GET -H "Authorization: Bearer YOUR_TOKEN" "https://api.twitter.com/1.1/statuses/user_timeline.json?screen_name=USERNAME&count=10"
This example uses the Google Maps Geocoding API to retrieve the geographical coordinates (latitude and longitude) for a given address.
Weather API (OpenWeatherMap):
curl -X GET "https://api.openweathermap.org/data/2.5/weather?q=London&appid=YOUR_API_KEY"
This example fetches the current weather information for a specific location (in this case, London) using the OpenWeatherMap API.
Ensure to replace the placeholders "YOUR_TOKEN" or "YOUR_API_KEY" with the actual authentication or API key required for the specific API you are working with. It is recommended to refer to the API documentation to gain insights into the available endpoints and parameters for making accurate requests.
2. Postman
Postman is a powerful API development and testing tool that offers a user-friendly interface for making REST API requests. It allows you to construct and send requests, view responses, set headers, and manage collections of API endpoints.
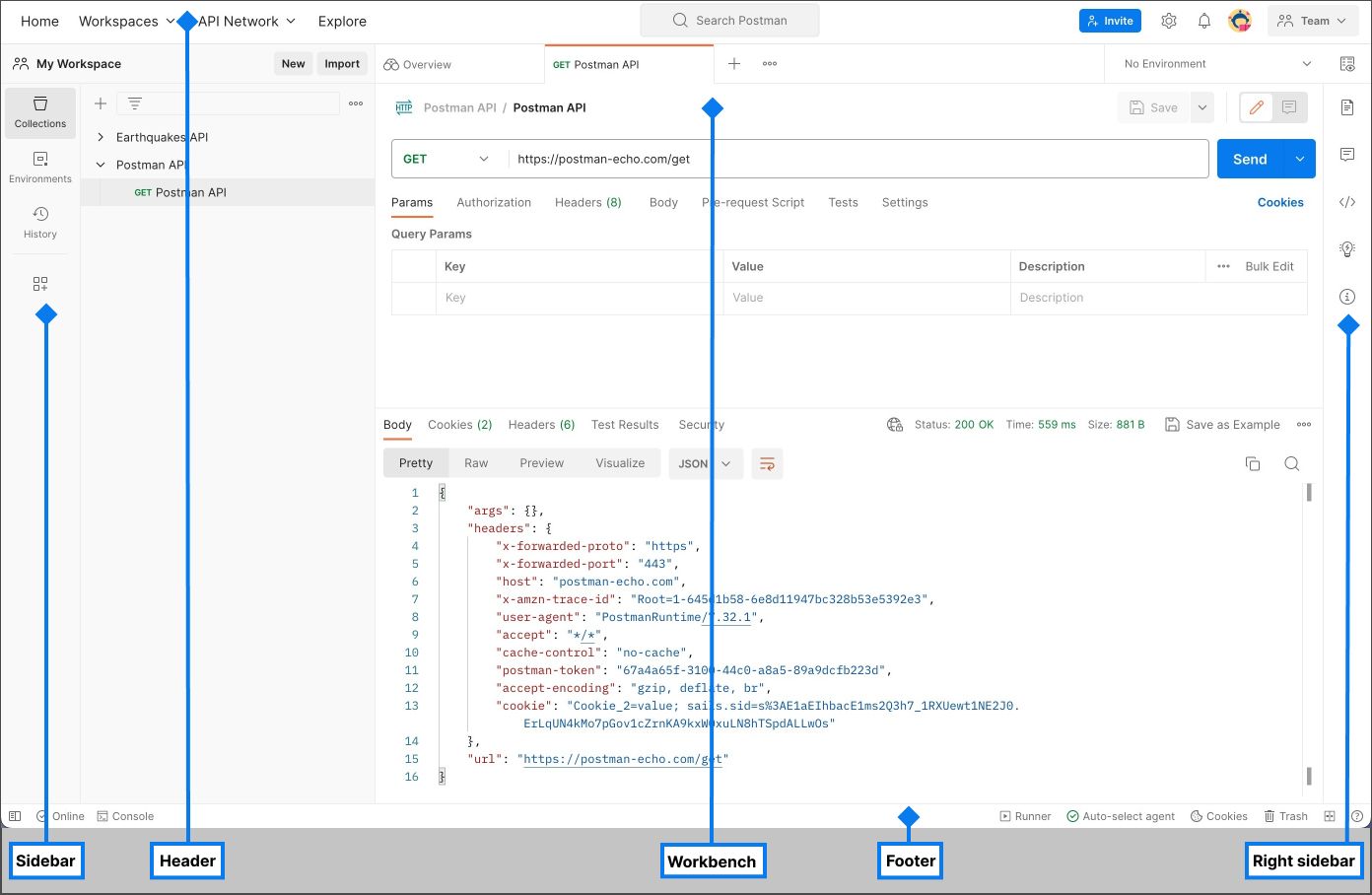
To send an API request in Postman through the Postman UI:
Step-1: Create a New Collection by clicking on the "+" icon in the left sidebar.
Step-2: Add a New Request by clicking on the "Add a request" link.
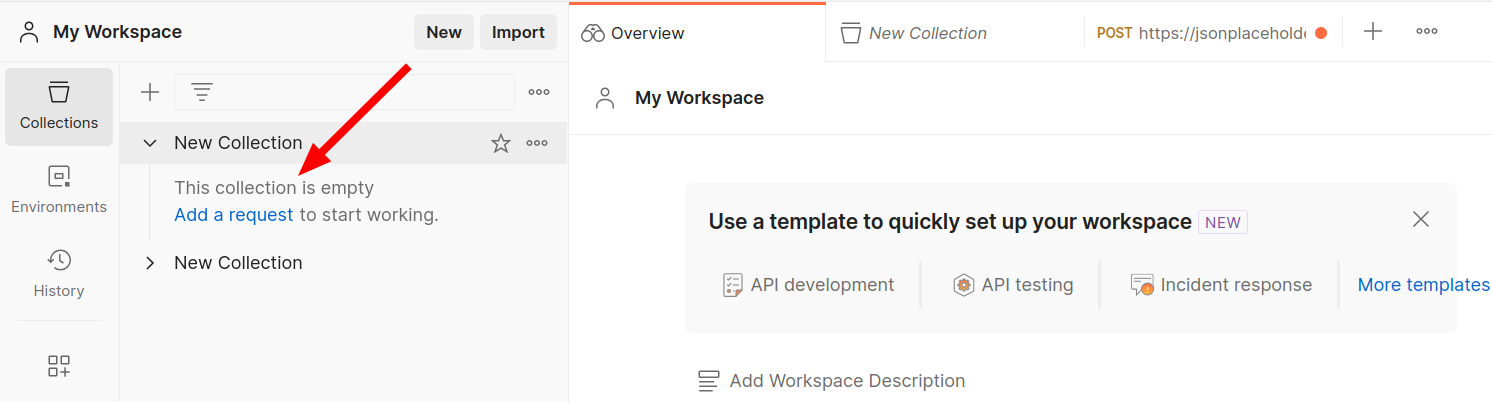
Step-3: Choose Request Type: Select the HTTP method for your API request (e.g., GET, POST).
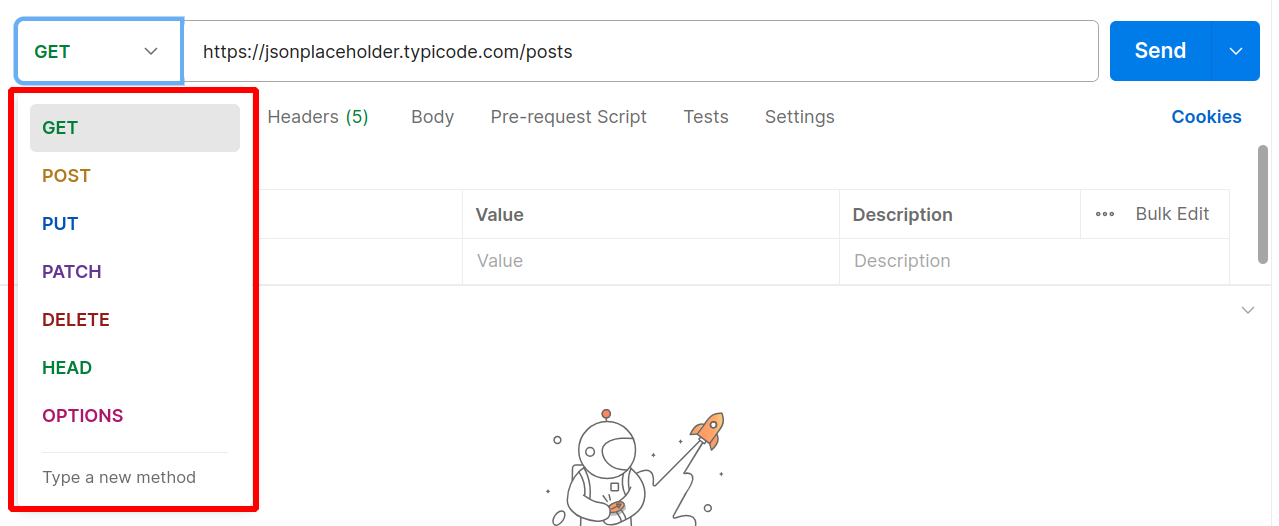
Step-4: Enter API URL: Type the URL of the API endpoint in the URL field.
Step-5: Add Parameters: Include query parameters, headers, or request body data if needed. Here I have added the body as raw text, you can choose the format as per your requirement.
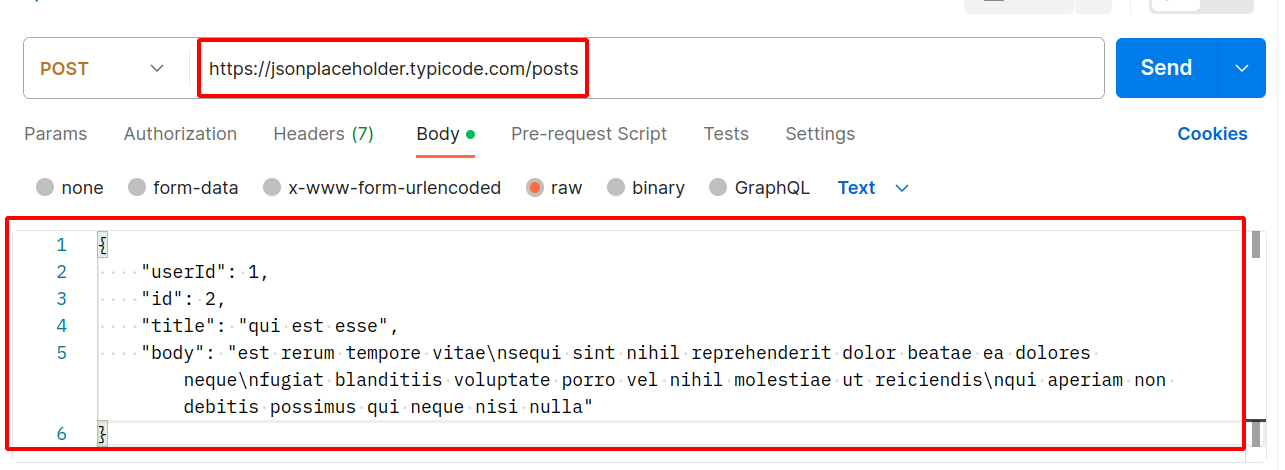
Step-6: Send the Request: Click "Send" to send the API request to the specified URL.
Step-7: View Response: Observe the response headers, status code, and response body.
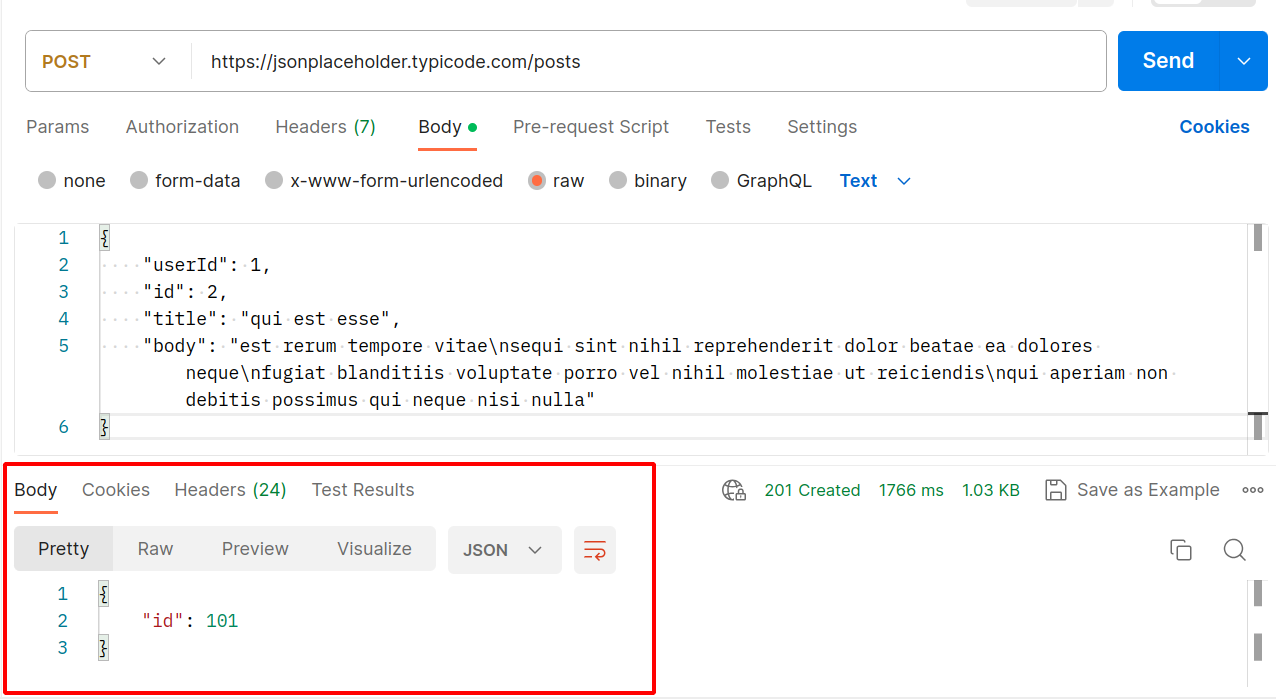
3. Insomnia
Insomnia is another popular API testing tool that provides a clean and intuitive interface for creating and managing REST API requests. It supports various authentication methods, environment variables, and code snippets generation.
Below is an example of how you can utilize Insomnia, a widely-used REST client tool, to execute a REST API request:
a.) Launch Insomnia and create a new request by clicking on the "Create" button in right corner of the Insomnia website. In the request editor, specify the HTTP method, URL, and other relevant details for the request.
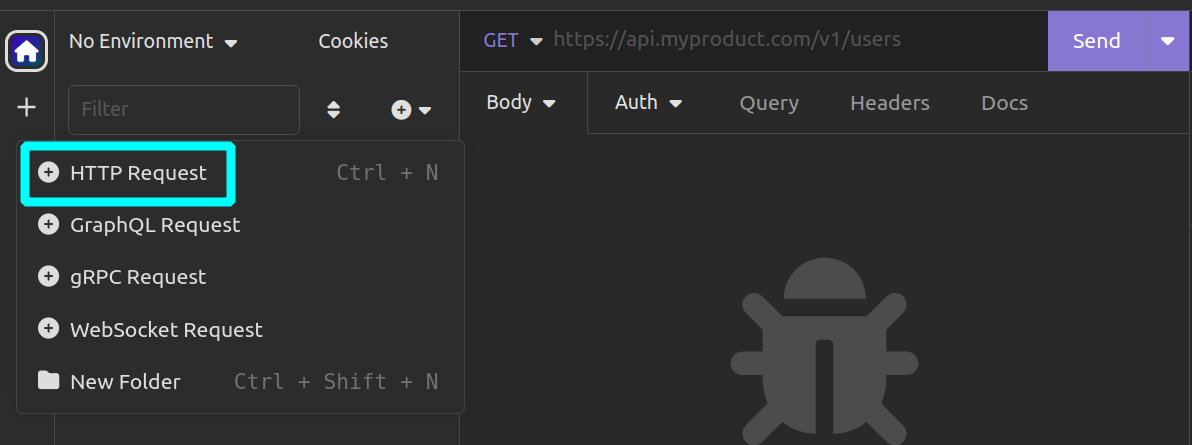
For instance:
- Method:
GET
- URL:
https://www.giveleads.net/leads/list
b.) If necessary, add request headers, query parameters, or a request body using the dedicated sections within the request editor.
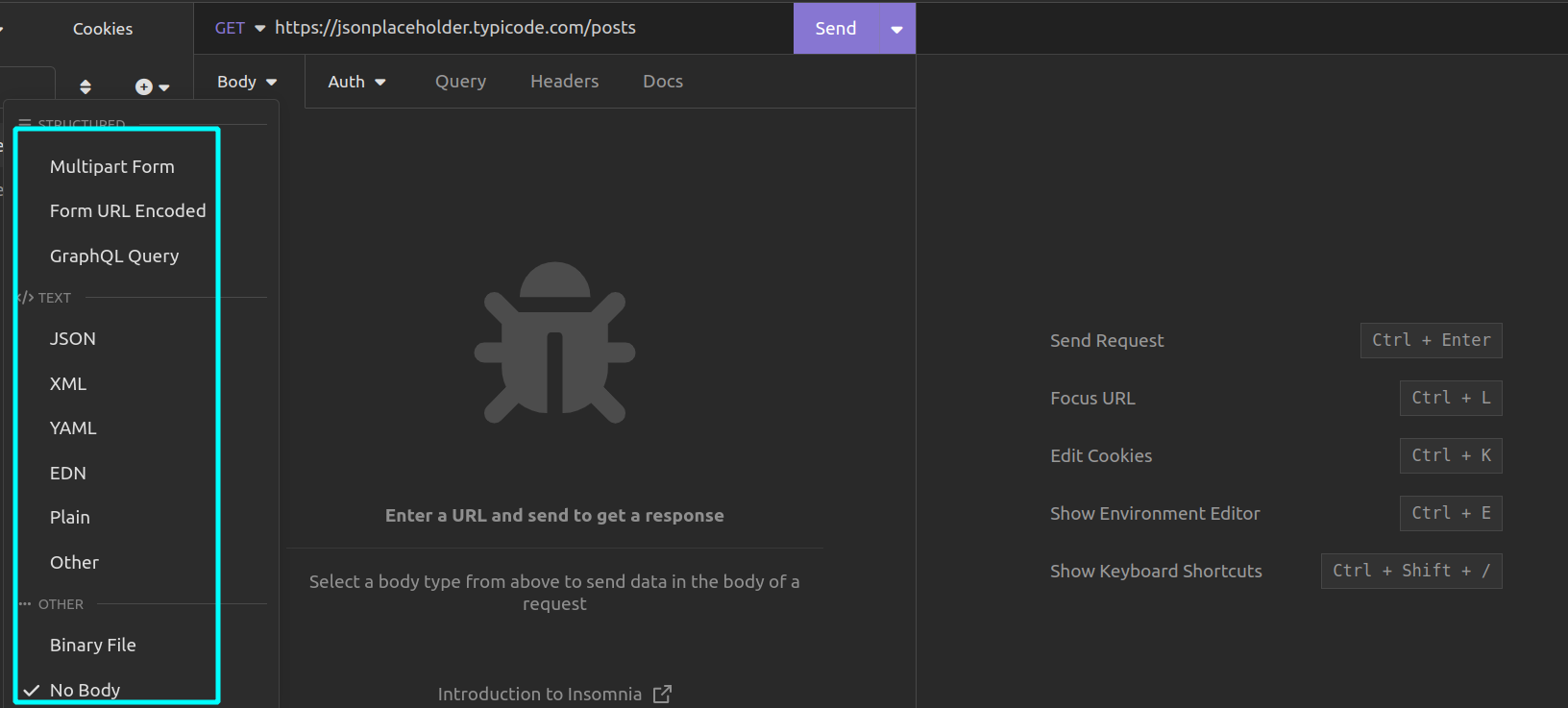
c.) Initiate the request by clicking on the "Send" button or by using the keyboard shortcut Ctrl + Enter
(Windows) or Cmd + Enter
(Mac).

d.) Insomnia will display the response obtained from the server, providing you with insights into the response body, headers, and other pertinent information within the response pane.
e.) Furthermore, you can include assertions or tests to verify the response's validity. Insomnia facilitates this through an "Assertions" section where you can define specific expectations regarding the response status code, body content, headers, and more.
Popular REST Client Libraries
1. Node.js
a.) axios
axios is a JavaScript library commonly used in web development for making HTTP requests, including REST API calls. It can be used both in the browser and Node.js environments, providing a simple and elegant API for handling requests and responses.
Usage:
const axios = require('axios');
// Set the base URL for the API
axios.defaults.baseURL = 'https://api.example.com';
// Make a GET request to retrieve user data
axios.get('/users/123')
.then(function (response) {
// Handle the response data
console.log('Response:', response.data);
})
.catch(function (error) {
// Handle any errors
console.error('Error:', error.message);
});
b.) node-fetch
node-fetch
is a lightweight and straightforward HTTP client for Node.js. It is similar to the Fetch API available in web browsers, making it familiar for developers who have experience with front-end development. Its simplicity and ease of use have contributed to its popularity.
Usage:
const fetch = require('node-fetch');
const url = 'https://api.example.com/data';
fetch(url)
.then(response => response.json())
.then(data => console.log(data))
.catch(error => console.error('Request failed:', error.message));
2. Python
a.) requests
The requests
library is a popular and easy-to-use HTTP library in Python. It provides a simple API to make HTTP requests and handle responses effectively. Due to its user-friendly design and widespread adoption, it has become the go-to choice for many developers when working with REST APIs.
Usage:
import requests
url = 'https://api.example.com/data'
response = requests.get(url)
if response.status_code == 200:
data = response.json()
print(data)
else:
print('Request failed with status code:', response.status_code)
b.) httpx
httpx
is a modern, asynchronous HTTP client library for Python. It builds upon the foundation of requests
but adds support for asynchronous requests using Python's asyncio
. This makes it an attractive option for projects that require high concurrency and performance.
Usage:
import httpx
url = 'https://api.example.com/data'
response = httpx.get(url)
if response.status_code == 200:
data = response.json()
print(data)
else:
print('Request failed with status code:', response.status_code)
3. Golang
a.) net/http
The net/http
package is part of Go's standard library, making it readily available and widely used for making HTTP requests. While it is relatively low-level, its simplicity and ease of integration with other Go code make it a popular choice for many developers.
Usage:
package main
import (
"fmt"
"io/ioutil"
"net/http"
)
func main() {
url := "https://api.example.com/data"
response, err := http.Get(url)
if err != nil {
fmt.Println("Request failed:", err)
return
}
defer response.Body.Close()
if response.StatusCode == 200 {
body, _ := ioutil.ReadAll(response.Body)
fmt.Println(string(body))
} else {
fmt.Println("Request failed with status code:", response.StatusCode)
}
}
b.) http.Client
The http.Client
is another major library for making HTTP requests in Go. It offers more control and customization options, such as setting custom timeouts and managing cookies, making it suitable for projects with specific requirements.
Usage:
package main
import (
"fmt"
"io/ioutil"
"net/http"
"time"
)
func main() {
url := "https://api.example.com/data"
client := &http.Client{Timeout: 10 * time.Second}
response, err := client.Get(url)
if err != nil {
fmt.Println("Request failed:", err)
return
}
defer response.Body.Close()
if response.StatusCode == 200 {
body, _ := ioutil.ReadAll(response.Body)
fmt.Println(string(body))
} else {
fmt.Println("Request failed with status code:", response.StatusCode)
}
}
4. Java
a.) java.net.HttpURLConnection
java.net.HttpURLConnection
class is part of Java's standard library and provides basic functionality for making HTTP requests.
Although it is relatively low-level, its simplicity and the fact that it comes with Java make it widely used, especially in smaller projects or when other libraries are not available.
Usage:
import java.io.BufferedReader;
import java.io.IOException;
import java.io.InputStreamReader;
import java.net.HttpURLConnection;
import java.net.URL;
public class Main {
public static void main(String[] args) {
try {
URL url = new URL("https://api.example.com/data");
HttpURLConnection conn = (HttpURLConnection) url.openConnection();
conn.setRequestMethod("GET");
if (conn.getResponseCode() == 200) {
BufferedReader br = new BufferedReader(new InputStreamReader(conn.getInputStream()));
String line;
StringBuilder response = new StringBuilder();
while ((line = br.readLine()) != null) {
response.append(line);
}
br.close();
System.out.println(response.toString());
} else {
System.out.println("Request failed with status code: " + conn.getResponseCode());
}
conn.disconnect();
} catch (IOException e) {
System.out.println("Request failed: " + e.getMessage());
}
}
}
b.) Apache HttpClient
The Apache HttpClient
library is a powerful and feature-rich library for making HTTP requests in Java. Its extensive feature set, ease of use, and the ability to handle complex HTTP workflows make it a popular choice for developers working on Java-based projects.
Usage:
import org.apache.http.client.methods.CloseableHttpResponse;
import org.apache.http.client.methods.HttpGet;
import org.apache.http.impl.client.CloseableHttpClient;
import org.apache.http.impl.client.HttpClients;
import org.apache.http.util.EntityUtils;
import java.io.IOException;
public class Main {
public static void main(String[] args) {
CloseableHttpClient httpClient = HttpClients.createDefault();
HttpGet httpGet = new HttpGet("https://api.example.com/data");
try (CloseableHttpResponse response = httpClient.execute(httpGet)) {
if (response.getStatusLine().getStatusCode() == 200) {
String responseBody = EntityUtils.toString(response.getEntity());
System.out.println(responseBody);
} else {
System.out.println("Request failed with status code: " + response.getStatusLine().getStatusCode());
}
} catch (IOException e) {
System.out.println("Request failed: " + e.getMessage());
}
}
}
5. PHP
a.) Guzzle
Guzzle is a comprehensive HTTP client library for PHP that provides a wide range of features, including handling HTTP methods, cookies, authentication, and more.
Its feature-rich nature, ease of use, and solid documentation make it one of the most popular choices for making HTTP requests in PHP.
Usage:
<?php
require 'vendor/autoload.php'; // Assuming you've installed Guzzle via Composer
use GuzzleHttp\Client;
$url = 'https://api.example.com/data';
$client = new Client();
$response = $client->get($url);
if ($response->getStatusCode() == 200) {
$data = $response->getBody()->getContents();
echo $data;
} else {
echo 'Request failed with status code: ' . $response->getStatusCode();
}
Benefits of REST Client
- REST can be implemented in various programming languages and used across different platforms.
- REST are compatible with a wide range of devices, including desktop computers, mobile devices, and Internet of Things (IoT) devices.
- REST promotes interoperability by adhering to standard HTTP protocols and formats like JSON or XML. This adherence to standards enables seamless communication and data exchange.
- REST boasts faster response times and optimized bandwidth utilization, resulting in efficient communication between clients and servers.
- REST is flexible and works well with various technology platforms, including cloud computing environments.
Conclusion
REST clients make it easier for applications to talk to and work with APIs. They act as messengers between the application and the API server, handling all the technical stuff like sending requests and getting responses.
With REST clients, developers can effortlessly retrieve data, update information, create new records, and delete existing ones from the server.
They simplify the process, so developers can focus on using the data in their applications without getting caught up in the technical details. Thus REST clients have become indispensable tools in the developer's toolkit.
Atatus API Monitoring and Observability
Atatus provides Powerful API Observability to help you debug and prevent API issues. It monitors the consumer experience and is notified when abnormalities or issues arise. You can deeply understand who is using your APIs, how they are used, and the payloads they are sending.
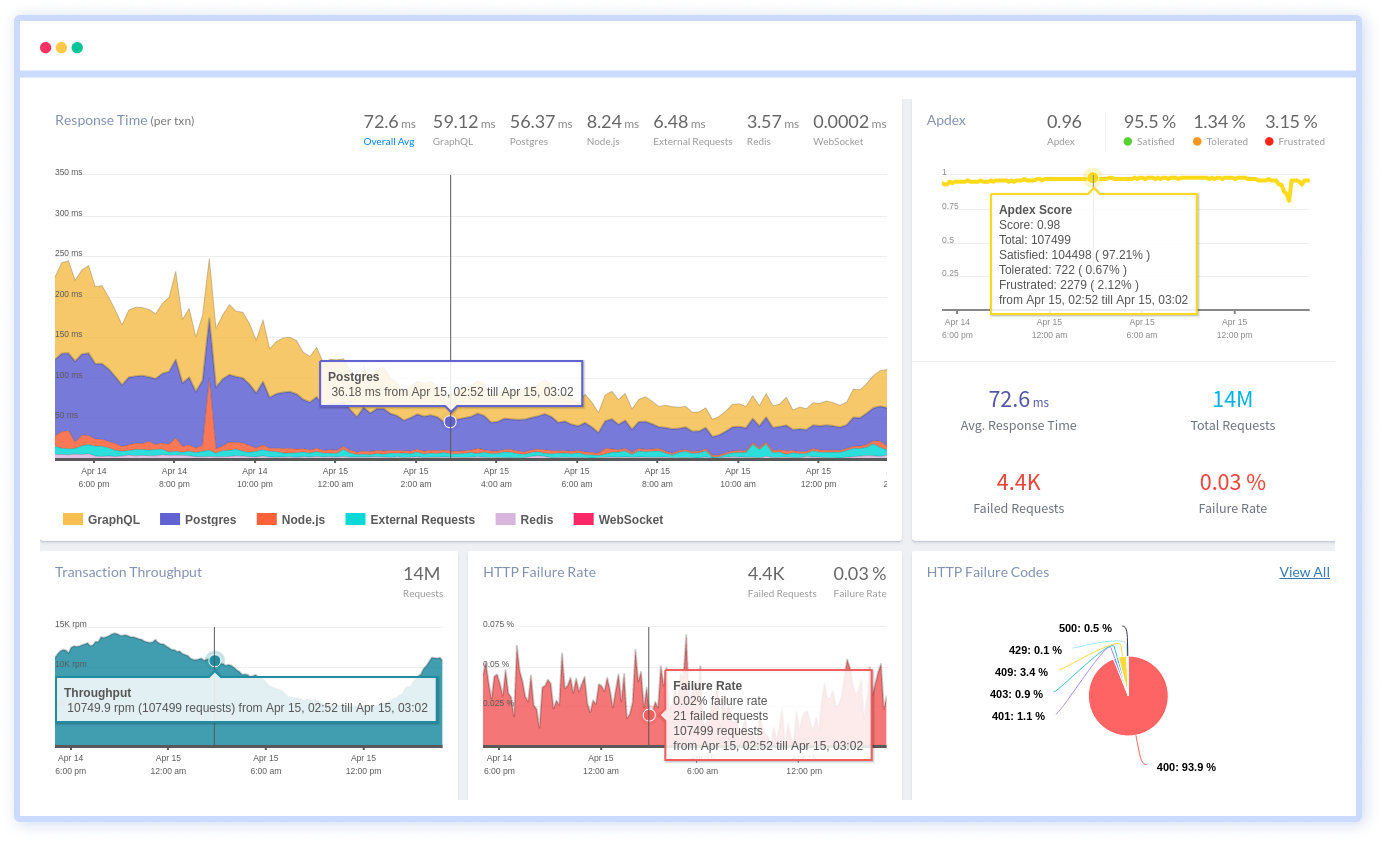
Atatus's user-centric API observability tracks how your actual customers experience your APIs and applications. Customers may easily get metrics on their quota usage, SLAs, and more.
It monitors the functionality, availability, and performance data of your internal, external, and third-party APIs to see how your actual users interact with the API in your application. It also validates rest APIs and keeps track of metrics like latency, response time, and other performance indicators to ensure your application runs smoothly.
#1 Solution for Logs, Traces & Metrics
APM
Kubernetes
Logs
Synthetics
RUM
Serverless
Security
More