Typescript vs Javascript: Should You Make the Switch?
Today, 98% of the websites are dependent on JavaScript for their client-side programming.
JavaScript is an exceptionally curated scripting language capable of creating dynamically updating content, developing games and making workable web applications.
These are some of the basic features that make JavaScript an ever-popular option for developers.
- Open source
- Enhances user interaction
- Wide range of frameworks and libraries
- Cross-browser compatibility
- High speed
- Simple semantics
JavaScript was at its helm when the same developers who created JavaScript, designed another scripting language called TypeScript.
Programmatically it is a superset of JavaScript with an additional static typing feature. Typescript is considered as a good programming language for implementing large-scale or enterprise-level applications.
Typescript and JavaScript have been two trending topics of all time. Let's see which one fares better.
- What is TypeScript?
- Features of TypeScript
- Overview of TypeScript
- TypeScript vs JavaScript - Key Differences
- Advantages of TypeScript over JavaScript
- Will TypeScript replace JavaScript?
- Should you migrate your program to TypeScript?
- How to migrate from JavaScript to TypeScript?
- When to choose JavaScript and When to choose TypeScript?
What is TypeScript?
Typescript is a free and open-source programming language developed and maintained by Microsoft. It is designed for developing large applications and transpiles into JavaScript. Typescript provides several additional features like interfaces, type aliases, abstract classes, function overloading, tuple, generics etc.
TypeScript is better than JavaScript in terms of language features, reference validation, project scalability, collaboration within and between teams, developer experience, and code maintainability.
Typescript is essentially JavaScript that improves developer experience.
It can be run on Node.js or any browser which supports ECMAScript 3 or newer versions. Recently typescript jumped 4.64% points from its 2021 standing to overtake Java as one of the most popular programming languages in the world.
JavaScript was a phenomenal language but it had just one shortcoming, it could not rise up to the standard of an object-oriented programming language when developers started using Js for developing server-side technologies. This led to the rise of a new scripting language - TypeScript.
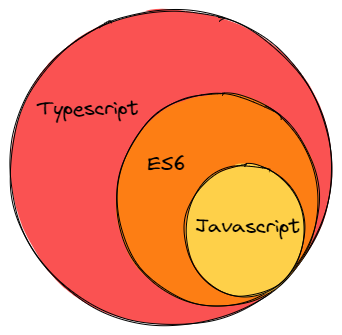
TypeScript - Frontend or Backend Programming?
Having been compiled into JavaScript, TypeScript has the advantage of being able to be used on both the front end as well as the back end of an application.
It is also worth noting that JavaScript is one of the most popular programming languages for developing the front end of web pages and applications. Therefore, TypeScript may be used for the same reason, but it may also be used for complex and large-scale enterprise projects on the server.
However, you can also build next-generation apps with other top front-end frameworks such as React, Angular, and Vue which are all compatible with typescript.
Features of TypeScript
Typescript has these additional qualities over JavaScript:-
i.) Compatible with all the versions of JavaScript including ES7 and ES12.
ii.) Supports namespaces, null checking, interfaces, generics and access modifiers.
iii.) Any code written in JavaScript can be converted to typescript by changing the extension from .js
to .ts
.
iv.) Typescript is syntactically the same as JavaScript, although all codes compiled in JavaScript may not be processed by the typescript compiler. For example,
let a = 'a';
a = 1; // throws: error TS2322: Type '1' is not assignable to type 'string'.
v.) Transpiling codes - Browsers are incapable of interpreting typescript codes, thus any program written in .ts will be converted to JavaScript for execution.
vi.) Static typing is a reliable option:
- Allows avoiding hidden ninja errors like "undefined" which is not a function.
- Refactoring code without breaking it fully
vii.) Supports JavaScript libraries - TypeScript can use already existing JS libraries while coding. Also, IntelliSense helps you navigate and discover new interfaces.
viii.) Full-featured IDE support and scalable HTML5 client-side development.
Overview of TypeScript
By understanding its benefits, you'll be able to determine if TypeScript is the right choice for your next project. Here we'll discuss some of the key benefits of using TypeScript and how you can use it to create a better development experience.
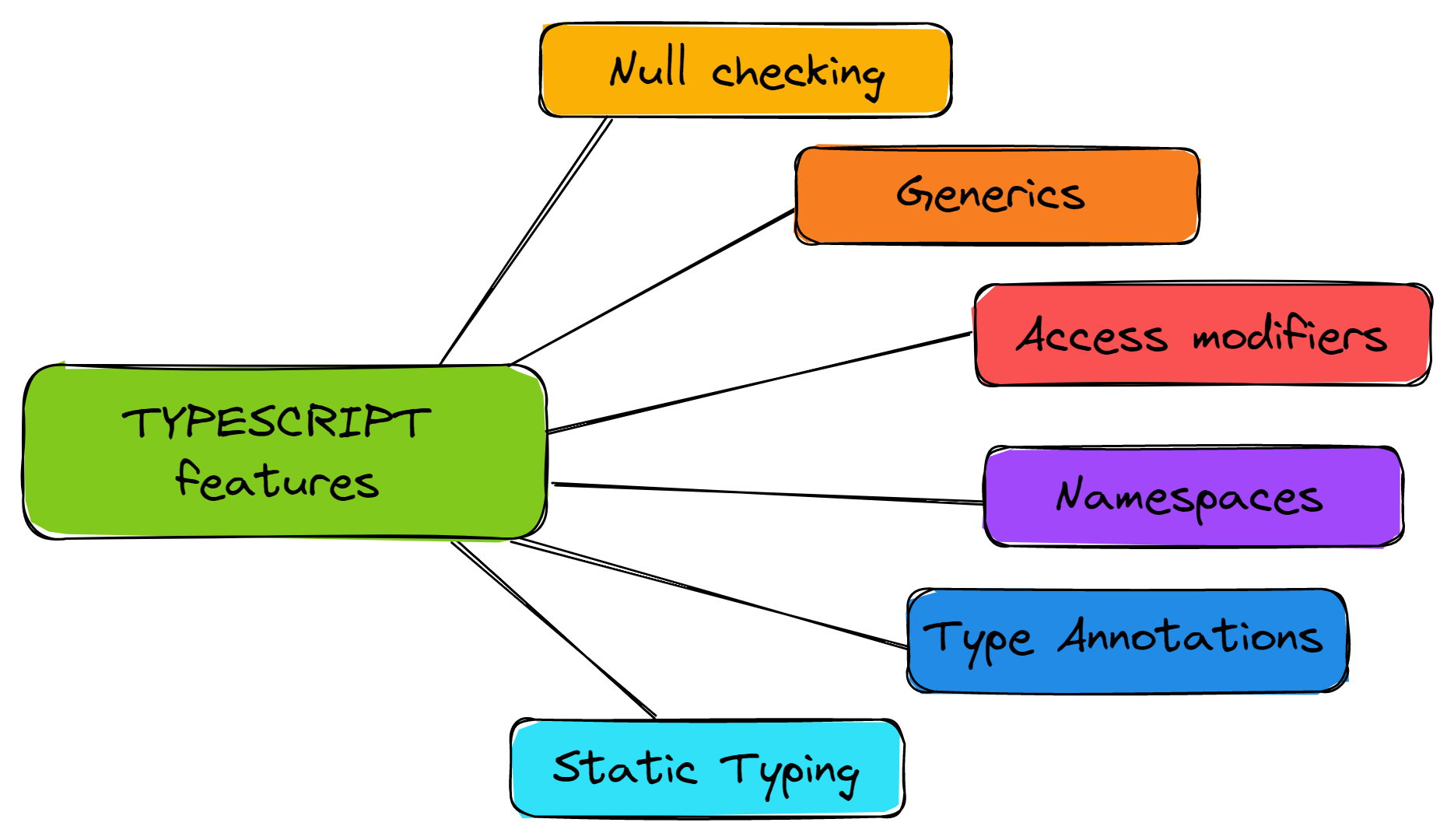
1. Null checking
Null refers to a value that is either empty or doesn't exist. In typescript, unassigned values are termed undefined. Thus we have to assign a null value to a variable if we want its output to be null. For that purpose, we can use, typeof
or comparison operators to check whether a variable is null or undefined in typescript.
type Name = null | undefined | string;
let name: Name;
// check if undefined or null
if (typeof name === null) {
console.log("Variable is null");
}
// check if NOT undefined or null
if (name != null) {
console.log("variable is not undefined or null");
}
2. Generics
Generics in Typescript are one of the most powerful and versatile features in the language. It provide a way to write code that can work with multiple types of data without having to specify it in the code.
With generics, developers can create code with fewer restrictions and more flexibility, allowing for better use of resources, improved code readability, and improved code reuse. With generics, developers can create code that is scalable, secure, and maintainable.
function getRandomNumber(items: number[]): number {
let randomIndex = Math.floor(Math.random() * items.length);
return items[randomIndex];
}
let numbers = [1, 5, 7, 4, 2, 9];
console.log(getRandomNumberElement(numbers));
Furthermore, they enable developers to create components that are both type-safe and efficient.
3. Access modifiers
Typescript supports three access modifiers - public, private, protected and read-only modifiers. Public members are accessible anywhere within the class. The access modifier increases the security of the class members and prevents them from invalid use.
class employeeDetails {
public name: string;
protected id: number;
private empcode: number;
constructor(name: string, id: number, empcode: number) {
this.name = name;
this.id = id;
this.empcode = empcode;
}
}
We can also use it to control the visibility of data members of a class. If the class does not have to be set any access modifier, TypeScript automatically sets the public access modifier to all class members.
4. Namespaces
When writing typescript applications, using namespaces is an effective way to define, organize, and maintain code in a large project. Namespaces allow developers to organize their code into smaller, manageable parts that are easier to interpret and use.
namespace <name> {
// ...
}
By using namespaces, developers are also able to avoid naming collisions and organize their code into logical components.
// string validator
namespace stringValidation {
export interface StringValidator {
isValid (s: string): boolean;
}
}
5. Type annotations
Type annotations provide a way to ensure that the correct data types are being passed as arguments to functions and that correct methods are being called on objects. By using type annotations, developers can also gain a better understanding of the structure of their applications and help prevent runtime errors.
This example shows how to annotate parameters with types.
// Basic JavaScript
function display(id:number, name:string)
{
console.log("Id = " + id + ", Name = " + name);
}
The TypeScript compiler can still infer the data type of variables based on the JavaScript way of declaring variables.
The properties of an object can also be declared with inline annotations.
let employee : {
id: number;
name: string;
};
employee = {
id: "20",
name : "Adam"
}
The TypeScript compiler will produce the following error if you try to assign a string to id.
error TS2322: Type '{ id: string; name: string; }' is not assignable to type
'{ id:number; name: string; }'.Types of property 'id' are incompatible.
Type 'string' is not assignable to type 'number'.
6. Static Type Analysis
Static type analysis is an important feature of TypeScript that allows developers to detect errors and enforce type safety in their applications. This feature can help improve the overall codebase quality and maintainability as well as reduce the number of runtime errors.
TypeScript vs JavaScript - Key Differences
JavaScript is a lightweight, client-side programming language while typescript is a more powerful object-oriented programming system including generics and other prominent features.
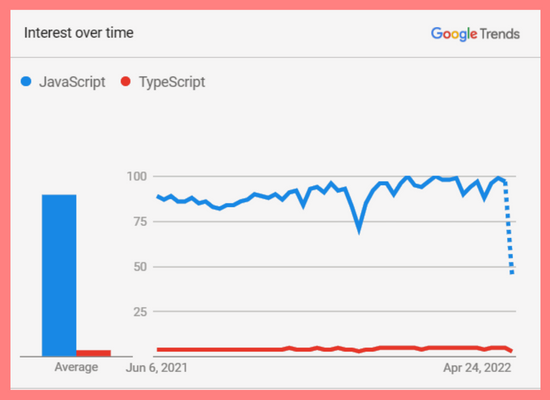
Learning curve - JavaScript is flexible and easy to learn, meanwhile typescript demands prior scripting knowledge and has a higher learning curve.
Performance - TypeScript is definitely more powerful than JavaScript. Typescript was created in the first place to overcome the limitations of JavaScript for running large-scale codes. As their tagline says
“TypeScript is JavaScript that scales.”
Compilation - JavaScript can be run as such, there is no need for compilation. Typescript on the other hand cannot be interpreted by browsers directly, so it is first converted into JavaScript and then run to compile programs.
Ecosystem - Static Typing feature in typescript allows the usage of several union types, intersections and discriminated unions. all these make typescript more powerful programmatically with respect to JavaScript. JavaScript offers the option to explore and create code without a build step
Static typing - TypeScript supports static typing and errors are highlighted during the development phase itself. While JavaScript doesn't support static typing and displays errors only during runtime.
Prototyping and annotation - While prototyping and annotation are favorable choices for developers using typescript to enhance their program's capability, these options are not available for JavaScript developers.
Popularity - TypeScript is a relatively new entrant amongst all the heavier scripting languages so far, but it also enjoys a large fanbase due to its enhanced performance. Microsoft, Accenture, and Alibaba use typescript while other bigshots like Instagram, Codecademy, Amazon, Twitter, LinkedIn and Airbnb still use JavaScript for their web servers.
JavaScript Sample Code
var Person = (function() {
function Person(personName) {
this.name = personName;
}
Person.prototype.name = function() {
return "My name is " + this.name;
}
return Person;
})();
TypeScript Sample Code
class Person {
private name: string;
constructor(private name: string) {
this.name = name;
}
name() {
return "name is " + this.name;
}
}
Advantages of TypeScript over JavaScript
- TypeScript is a preferred choice for the development of large or complex applications, whereas JavaScript is preferred for smaller projects.
- Typescript supports optional static typing to the language which makes checking type correctness easy during compilation. But this feature is not available in JavaScript.
- TypeScript always points out the compilation errors at the time of development (pre-compilation). Because of this getting runtime errors is less likely, whereas JavaScript is an interpreted language.
- Typescript allows for easier code restructuring when compared to JavaScript. This also makes identifying and fixing code errors in Typescript faster.
- At the time of development, TypeScript identifies the compilation bugs. As a result, the scope of evaluating errors at runtime is very less. JavaScript, on the other hand, is an interpreted language.
Will TypeScript replace JavaScript?
JavaScript is an in-demand scripting language used by multitudes of corporations and even individual developers. It is an advantageous tool when making simple interface programs without much complication.
Even if typescript is more powerful in terms of performance capabilities, typescript cannot run on its own, any program in typescript needs to be transpiled into JavaScript before a web browser can read its contents and execute the program.
Both languages have pros and cons when compared, but solitarily, they both need to be used in different contexts - JavaScript while designing a simple interface small application and typescript when building a scalable larger project.
Thus, for an answer to “Will typescript replace JavaScript?”, it is always going to be a NO!
Typescript and JavaScript will coexist and help developers make beautiful web applications.
Should you migrate your program to TypeScript?
JavaScript is a leading web-development language used by some of the world’s mightiest corporations. It is easy to read, code and execute. It is a very fitting tool for building small-scale applications.
The problem comes in when we are trying to scale these existing applications or want to build a program that caters to a wide clientele database. Sadly, JavaScript is not equipped with handling such huge tasks. Thus, typescript was created. Typescript is ideal for developers building such scalable interfaces as used by Instagram or Twitter.
Keeping in mind all the pros and cons of both these languages, choose one that is appropriate for you and your program.
Read when to choose what? Section to get further insights on this topic.
How to migrate from JavaScript to TypeScript?
If at all you want to migrate, you can follow the steps below -
In order to migrate from JS to TS,
- Add tsconfig.json file to project
- Integrate with a build tool
- Change all .js files to .ts files
- Check for any errors
Add tsconfig.json file to project
By using the include option here, we are assigning it to include all the files placed under src. folder. The compilerOptions
, outDir
specifies that the output files should be redirected to a folder called "dist".The target option specifies that all JavaScript constructs should be translated to ECMAScript 5.
{
"compilerOptions": {
"outDir": "./dist",
"allowJs": true,
"target": "es5"
},
"include": [
"./src/**/*"
]
}
Integrating with a build tool
i.) Run the following command on your terminal:
npm install awesome-typescript-loader source-map-loader
awesome-typescript-loader
is a TypeScript loader, whereas source-map-loader
is used for debugging your source code.
ii.) Add/Edit the module config property in your webpack.config.js
file to include these two loaders:
module.exports = {
entry: "./src/index.ts",
output: {
filename: "./dist/bundle.js",
},
// Enable sourcemaps for debugging webpack's output.
devtool: "source-map",
resolve: {
// Add '.ts' and '.tsx' as resolvable extensions.
extensions: ["", ".webpack.js", ".web.js", ".ts", ".tsx", ".js"],
},
module: {
rules: [
// All files with a '.ts' or '.tsx' extension will be handled by 'ts-loader'.
{ test: /\.tsx?$/, loader: "ts-loader" },
// All output '.js' files will have any sourcemaps re-processed by 'source-map-loader'.
{ test: /\.js$/, loader: "source-map-loader" },
],
},
// Other options...
};
Converting .js files to .ts files
Rename your first .js
file to .ts
. Similarly, rename the .jsx
file to .tsx
. As soon as you do that, some of your code might start giving compilation errors.
Check for Errors
Since TypeScript has strict type checking, you may notice errors in your JavaScript code. For example, consider a function with too many or two few arguments
function displayPerson(name, age, height) {
let str1 = "Person named " + name + ", " + age + " years old";
let str2 = (height !== undefined) ? (" and " + height + " feet tall") : '';
console.log(str1 + str2);
}
displayPerson( "John", 32);
In the above code, we have a function named displayPerson()
that takes three arguments: name, age, and height. We call the above function with two values: "John", and 23. The above function is perfectly valid with JavaScript. This is because if an expected argument to a function is missing in JavaScript, it assigns the value undefined to the argument.
However, the same code in TypeScript will give the compilation error: error TS2554: Expected 3 arguments but got 2.
To rectify this, you can add an optional parameter sign to the argument height. Also, while you are removing errors, you can also annotate your code like below:
function displayPerson( name: string, age: number, height?: number) {
let str1: string = "Person named " + name + ", " + age + " years old";
let str2: string = (height !== undefined) ? (" and " + height + " feet tall") : '';
console.log(str1 + str2);
}
When to choose JavaScript and When to choose TypeScript?
Depending on the requirement, we can decide which of these scripting languages would be appropriate for our project.
When to choose JavaScript?
- You plan to build a small-scale application. Do not complicate it by coding it in typescript.
- When typescript doesn't support your choice of framework and thus leveraging it is not possible.
- TypeScript necessitates a build step to produce the final JavaScript to be executed. However, it is becoming increasingly rare to develop JavaScript applications without build tools of any kind.
- When we need to use libraries in typescript, we need to have knowledge of their type definitions. Every type definition in turn requires an npm package. The credibility and maintenance of these packages can prove risky.
- We are using a test-driven development workflow, and migrating to typescript would just be an additional cost burden!
When to choose TypeScript?
- You are working on large-scale projects or working with a team of developers.
- You need to use a new library or framework, like React, whose APIs you may be unfamiliar with. IntelliSense helps you to identify and navigate through such APIs. it is possible because typescript has declaration files for all the standardized built-in APIs available in JavaScript runtimes.
- Static type checking detects bugs even before running the programming by compiling errors at runtime.
Conclusion
The use of JavaScript is so widespread that most experienced developers are well-trained in it. JavaScript works with HTML to improve the quality of web pages, making it a comprehensive and reliable programming language.
Meanwhile, TypeScript is an ideal choice for developers seeking to create readable and clean code. TypeScript also provides live bug detection, static typing, and other features.
Both these languages have their own attributes and limitations. Development teams must take into account the needs of the project before choosing which scripting language to use. For more information on typeof
function in typescript, follow this link.
Atatus Real User Monitoring
Atatus is a scalable end-user experience monitoring system that allows you to see which areas of your website are underperforming and affecting your users. Understand the causes of your front-end performance issues and how to improve the user experience.
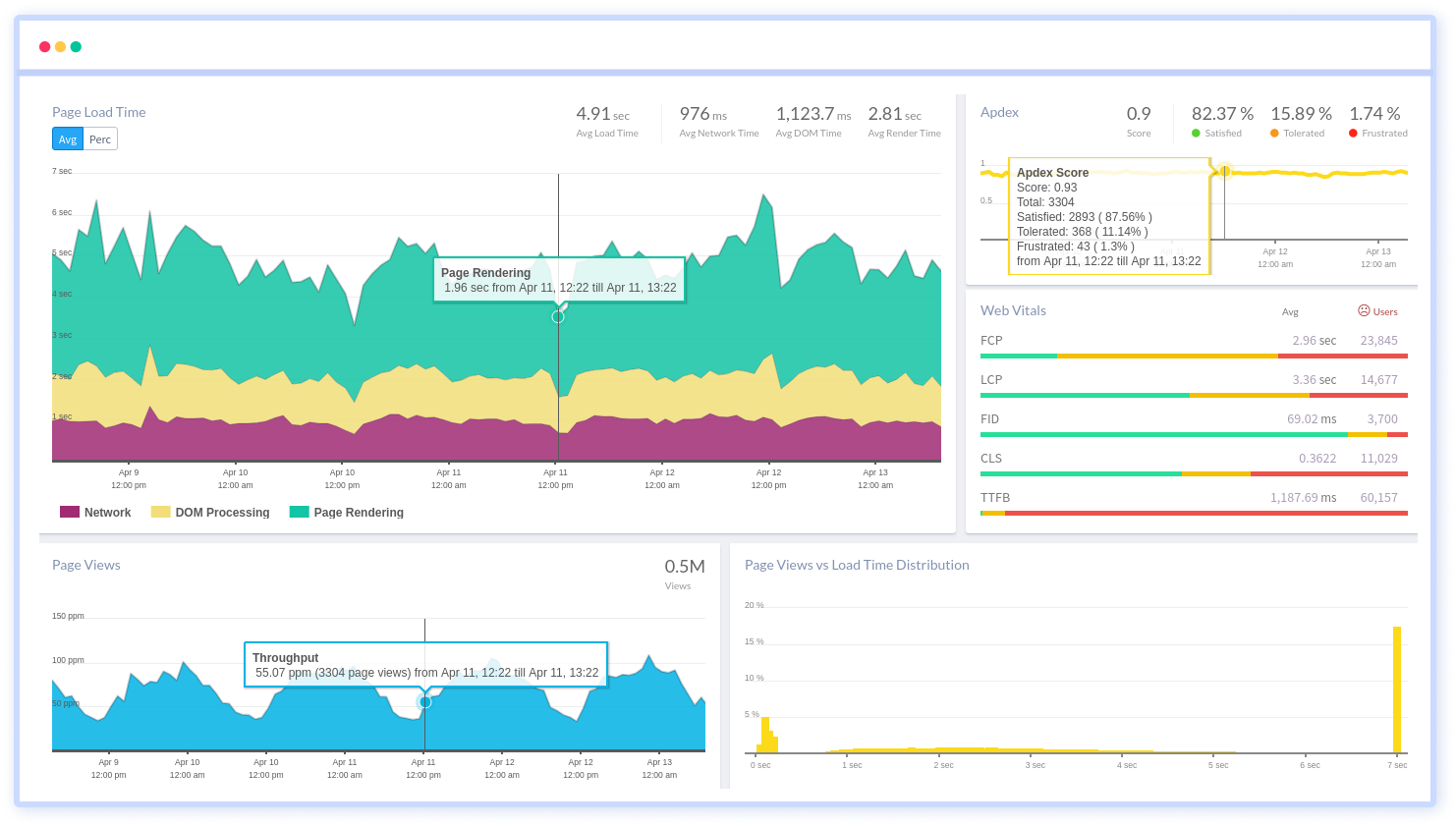
By understanding the complicated frontend performance issues that develop due to slow page loads, route modifications, delayed static assets, poor XMLHttpRequest, JS errors, core web vitals and more, you can discover and fix poor end-user performance with Real User Monitoring (RUM).
You can get a detailed view of each page-load event to quickly detect and fix frontend performance issues affecting actual users. With filterable data by URL, connection type, device, country, and more, you examine a detailed complete resource waterfall view to see which assets are slowing down your pages.
#1 Solution for Logs, Traces & Metrics
APM
Kubernetes
Logs
Synthetics
RUM
Serverless
Security
More