typeof in JavaScript: An introduction
Checking data types for variables is vital in any programming language to ensure a smooth and error-free development process. However, this becomes even more essential for accuracy when it comes to dynamically typed languages such as Javascript.
In Javascript, one variable can hold multiple value types within the same file as shown below:
let x = 1;
x = 'blue';
x = true;
x = Boolean(true);
x = String('Be positive');
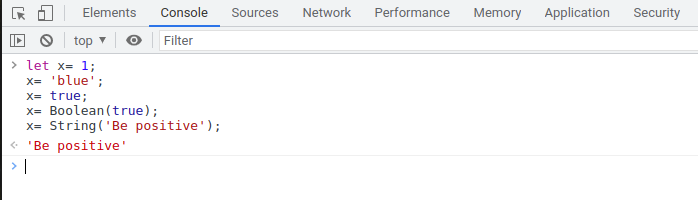
To achieve this, one of the most commonly used operators is typeof
. With this article, we will take an in-depth look into the functionalities of typeof
operators and the ways they can help craft a reliable and error-free codebase.
Table of contents
- JavaScript Data Types
- Introduction to typeof and How to use them
- Function based typeof Syntax Vs. Basic Syntax
JavaScript Data Types
Before we jump into implementing typeof operators, let us first understand the data types we can use in JavaScript for enhancing their overall functionality. In JS you can use the following seven primitive types of data:
- Number
- BigInt
- String
- Boolean
- Symbol
- Null
- Undefined
The rest of the data types are known as reference types. Apart from these the arrays, regular expressions and functions fall under special kinds of objects. Further, you can also use special object class constructs for crafting other necessary objects for further functionality such as:
- Date- For date objects
- RegExp- For crafting Regular Expressions Error- For crafting JS Errors
Introduction to typeof and How to use them
The typeof operators use unary operators only to get their original data type and return the result in the form of strings. Using them can help enhance the accuracy of your functions. Here’s the basic syntax for using typeof operators:
typeof 7; // returns 'number'
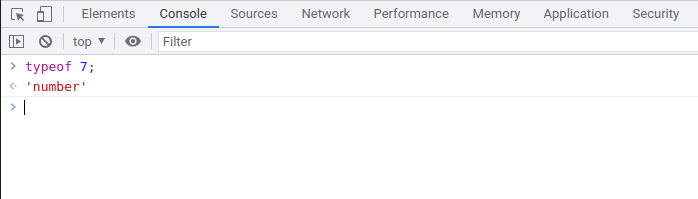
For those with a Java background using typeof in the function syntax as below can add up a hint of familiarity. You can further use the syntax to evaluate expressions as well.
typeof(operand) // Syntax
typeof(typeof 7); // returns 'string'
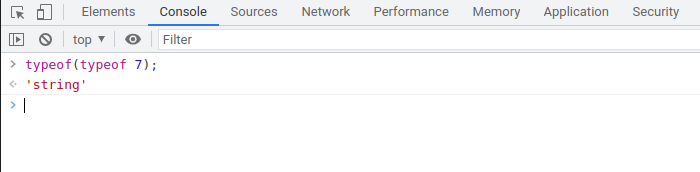
The use of the above syntax instead of the first one also ensures that the results are more accurate and reliable. This increases the overall accuracy of the codebase and helps developers avoid run-time bugs.
Function based typeof Syntax Vs. Basic typeof Syntax
To understand how the typeof operator syntax results effects the overall accuracy, let’s try to get the datatype of expression 88-44
using the typeof operator in both methods.
Method 1:
typeof 88-44; // returns, NaN
Method 2:
typeof(88-44); // returns, "number"
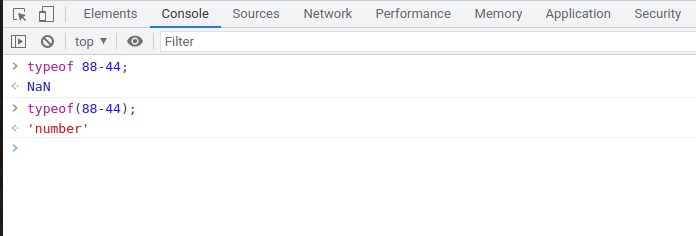
As we can see, without the parenthesis, the expression of -44
becomes a string for the operator interacting with a number. Hence it results in the final output of NaN
(Not a Number). However, the parenthesis used allows the operator to view and understand expressions.
Here is a list of type checks on different data types and their return result for a better idea.
typeof 1; // 'number'
typeof +1; // 'number'
typeof -1; // 'number'
typeof Math.sqrt(4); // 'number'
typeof Infinity; // 'number'
typeof NaN; // 'number', even if it is Not a Number
typeof Number('13'); // 'number', After successfully coerced to number
typeof Number('Happy'); // 'number', despite it can not be coerced to a number
typeof true; // 'boolean'
typeof false; // 'boolean'
typeof Boolean(0); // 'boolean'
typeof 2n; // 'bigint'
typeof ''; // 'string'
typeof 'Joy'; //'string'
typeof `Joy is Bliss`; // 'string'
typeof '80'; // 'string'
typeof String(80); // 'string'
typeof Symbol(); // 'symbol'
typeof Symbol('joy'); // 'symbol'
typeof {blog: 'typeof', author: 'A'}; // 'object';
typeof ['It’s', 'a', 10]; // 'object'
typeof new Date(); // 'object'
typeof Array(5); // 'object'
typeof new Boolean(true); // 'object';
typeof new Number(10); // 'object';
typeof new String('Happy’); // 'object';
typeof new Object; // 'object'
typeof alert; // 'function'
typeof function () {}; // 'function'
typeof (() => {}); // 'function' - an arrow function so, parenthesis is required
typeof Math.sqrt; // 'function'
let c;
typeof c; // 'undefined'
typeof d; // 'undefined'
typeof undefined; // 'undefined'
typeof null; // 'object'
To track different data types and their type check returns, refer to the table below.
Error safety for typeof operators
Before the launch of ES6, the typeof operator always returned a string regardless of the operand. Hence, even type checks on undeclared variables would result in undefined, which can cause application crashes and errors.
To solve this in ES6, we can declare block scope variables with let and constant keywords. Further, if developers type checks before the declaration of a variable, it throws a reference error to ensure accuracy in the codebase.
typeof x; // ReferenceError
let x = 'Apple';
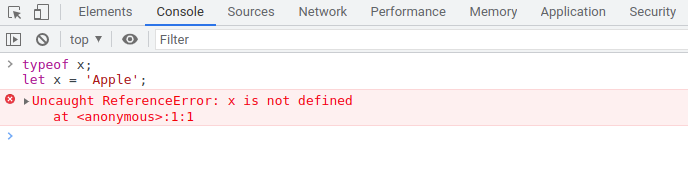
Limitations in typeof
From the above discussion, you might have observed that implementing typeof is quite simplistic. However, when it comes to its efficiency, it lacks in multiple aspects. Here is a list of common instances where you can not use the typeof operator for accurate results:
#1 Unable to Detect NaN
When we use the typeof operator on NaN it returns a number even though NaN isn’t. Hence, failing to recognize the true data type. In such scenarios instead of using the typeof operator for checking "NaN" a better alternative is to use the "isNaN()" operator, which returns the value in the boolean form as shown below:
isNaN(0/0); // returns, true
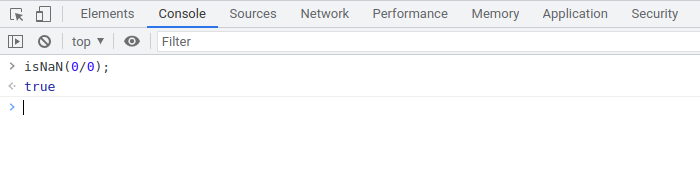
Note: However, when we check NaN for undefined, it returns the value as True.
isNaN(undefined); // returns true for 'undefined'
Yet, in ES6, "isNaN" is added to the global number object, so NaN is preferred.
Number.isNaN(0/0); // returns, true
Number.isNaN(undefined); // returns, false
#2 Fails To Detect Null Value
Using the typeof operator to detect Null values can lead to errors in real-time scenarios. A suitable alternative for detecting Null values whenever needed is by adapting to a strict quality operator(===) as shown below:
function isNull(input)
{
return input === null;
}
Note: Strict operators have the syntax of “===” replacing it with “==” can lead to misleading type checking.
#3 Lack Of Array Detection Capabilities
Since the typeof only detects primitive data types, you can not use it to identify an array. Yet, array detection in JS is essential to ensure you are working with the appropriate datasets. For Array detection, you can use “Array.isArray” available in ES6.
Array.isArray([]); // returns true
Array.isArray({}); // returns false
If your system does not support ES6, another alternative is to use the “instanceof” operator for detecting arrays as shown in the example:
function isArray(input)
{
return input instanceof Array;
}
#4 Object Type Checking in JS
As discussed earlier, the typeof operator can not check object types. However, type checking is essential to ensure developers are working with the right data sets as the web application expands. For this, you can use the Object.prototype.toString
method and check the object types.
If you invoke it using either call() or apply() method, you will get the object type in the format of [object type], where type denotes the type of object. Below is an example of how you can easily use the Object.prototype.toString method for type-checking the object in the JS file effectively.
// returns '[object Array]'
Object.prototype.toString.call([]);
// returns '[object Date]'
Object.prototype.toString.call(new Date());
// returns '[object String]'
Object.prototype.toString.call(new String('atatus.com'));
// returns '[object Boolean]'
Object.prototype.toString.call(new Boolean(true));
// returns '[object Null]'
Object.prototype.toString.call(null);
To further enhance the accuracy and get the exact object type, we can easily extract the "Type" with the following program:
function check (value)
{
const val = Object.prototype.toString.call(value);
const Objtype = value.substring(
val.indexOf(" ") + 1,
val.indexOf("]"));
return type.toLowerCase();
}
Now the check
function we crafted above can easily further detect the object type.
check([]); // 'array'
check(new Date()); // 'date'
check(new String('Hello')); // 'string'
check(new Boolean(true)); // 'boolean'
check(null); // 'null' of any object you’re using
To Wrap Up
From the discussion above, we can easily conclude that the typeof
operator is essential to maintain accuracy in your JS codebase and avoid unwanted real-time errors and application crashes.
Yet, typeof
operator comes with multiple drawbacks such as lack of array detection, Null value recognition, and faulty results when applied on NaN
, which can negatively affect the overall accuracy and efficiency.
Hence, type checking via other alternatives becomes essential for developers. Try the above examples for type-checking the data types and object types today to sharpen your JS skill and craft unique JS solutions.
Monitor Your Node.js Applications with Atatus
Atatus keeps track of your Node.js application to give you a complete picture of your clients' end-user experience. You can determine the source of delayed response times, database queries, and other issues by identifying backend performance bottlenecks for each API request.
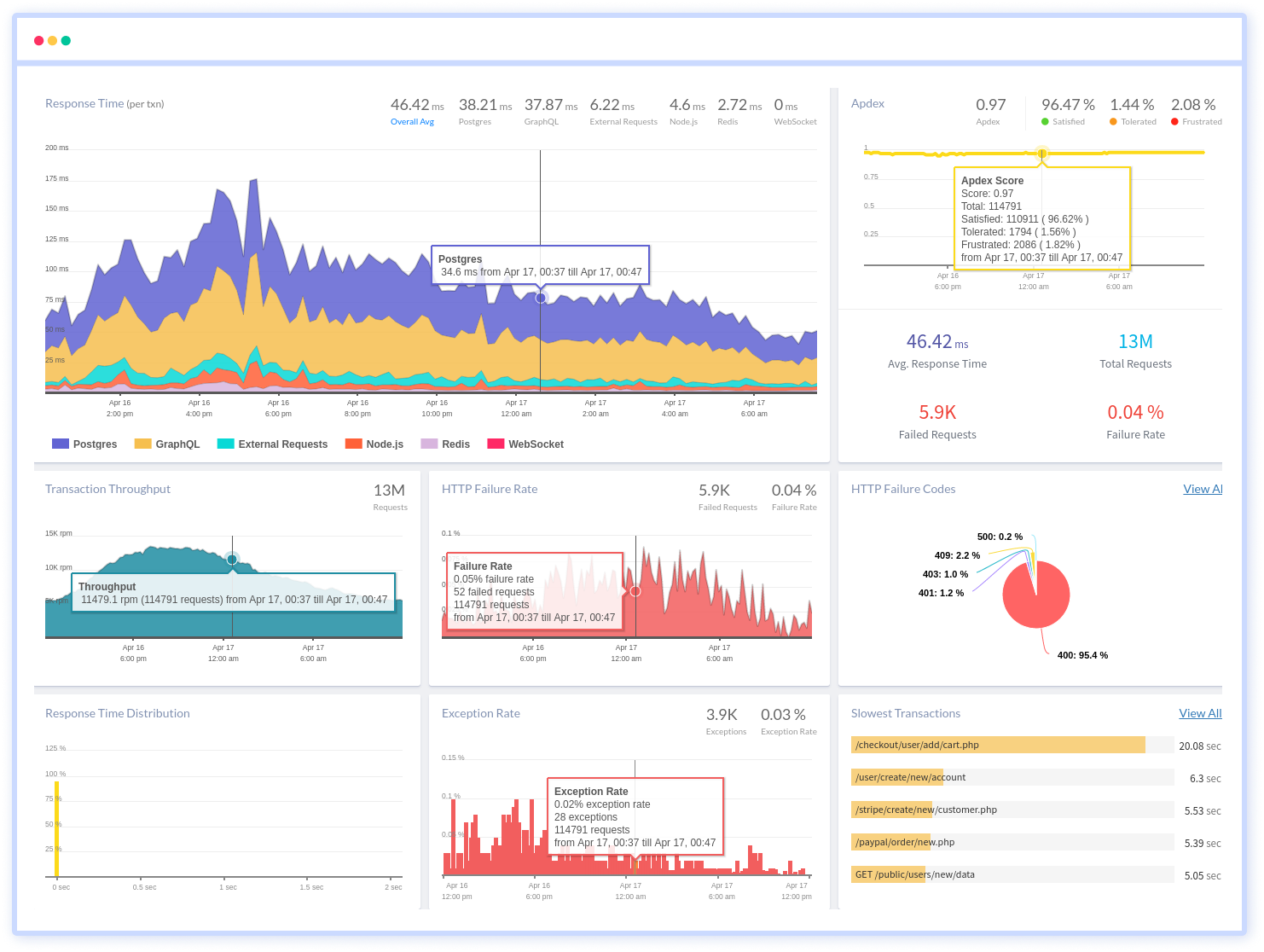
To make bug fixing easier, every Node.js error is captured with a full stack trace and the specific line of source code marked. To assist you in resolving the Node.js error, look at the user activities, console logs, and all Node.js requests that occurred at the moment. Error and exception alerts can be sent by email, Slack, PagerDuty, or webhooks.
#1 Solution for Logs, Traces & Metrics
APM
Kubernetes
Logs
Synthetics
RUM
Serverless
Security
More