Understanding the Difference - Web APIs vs. Web Services
In today's digital world, where systems need to work together smoothly and communicate effectively, two important terms come into play, APIs and web services. These are the tools that help different applications and systems connect with each other seamlessly.
APIs are like secret keys that unlock specific functions and data, saving time for developers and allowing them to reuse code. On the other hand, web services act as bridges between different systems, breaking down language and platform barriers so they can collaborate effortlessly. APIs are the concept, and web services are a specific implementation of APIs using web technologies for communication.
Web APIs and Web services are similar concepts, but they are not identical in meaning. But what lies behind these intriguing terms? How do they impact the way applications communicate, and what sets them apart? Let's uncover the secrets.
In the context of Kubernetes, servers and other backend systems, the term "services" might be used differently. However, in this blog, our focus will be on web services, a way of enabling communication between different applications over a network.
So, let's avoid any confusion between the two and delve into the topic of Web API's vs Web Services.
Table of Contents
- What is an API?
- What are Web Services?
- Types of Web APIs and Web Services
- Features of an API
- Features of Web Services
- Request-Response Model
- Advantages and Disadvantages of Web APIs
- Advantages and Disadvantages of Web Services
What is a Web API?
An API, which stands for Application Programming Interface, is a collection of specific instructions that establish how various applications can interact and exchange information with each other.
An API is a broader term that encompasses a range of methods, protocols, and tools that allow software applications to communicate with each other. It enables two applications to communicate and work together seamlessly without requiring any manual intervention from the user.
What are Web Services?
A web service is a software system that facilitates the exchange of information between machines over a network in a standardized manner.
A web service, on the other hand, is a specific type of API that uses web technologies and protocols (such as HTTP) to enable machine-to-machine communication over a network, typically the internet.
It provides a defined interface, typically described in a machine-readable format like Web Service Definition Language (WSDL), allowing different systems to interact and communicate.
Types of Web APIs and Web Services
APIs and web services are essential components of modern application development and integration. They provide a standardized approach for different applications, systems, or services to communicate, exchange data, and collaborate seamlessly.
Types of APIs
1. RESTful APIs
Representational State Transfer (REST) APIs use HTTP methods (GET, POST, PUT, DELETE) to perform operations on resources. They are widely used for web development and provide a lightweight and scalable approach to building APIs. They emphasize simplicity, scalability, and the decoupling of client and server.
Real-world Applications: RESTful APIs are commonly used in web and mobile applications, social media platforms, e-commerce websites, and any system requiring resource-based interactions and stateless communication.
Example: Using Python's requests
library to interact with a RESTful API that manages a list of books.
import requests
# Fetch all books
response = requests.get("https://example.com/api/books")
if response.status_code == 200:
books = response.json()
for book in books:
print(book['title'])
# Add a new book
new_book = {"title": "Sample Book", "author": "John Doe"}
response = requests.post("https://example.com/api/books", json=new_book)
if response.status_code == 201:
print("Book added successfully.")
2. SOAP APIs
Simple Object Access Protocol (SOAP) APIs use the XML messaging protocol for communication. They support complex operations, data typing, and provide features like security and reliability. They tend to be more heavyweight and suitable for enterprise-level integrations.
Real-world Applications: SOAP APIs are commonly used in enterprise systems, financial institutions, healthcare applications, where security, reliability, and transactional capabilities are critical.
Example: Using Python's suds
library to interact with a SOAP-based weather service.
from suds.client import Client
# Create a SOAP client
url = "https://example.com/WeatherService?wsdl"
client = Client(url)
# Call a SOAP method to get weather information
response = client.service.GetWeather(city="New York")
print(response)
3. GraphQL APIs
GraphQL APIs enable clients to specify the exact data they need, reducing over-fetching or under-fetching of data. They provide a flexible and efficient way to request and manipulate data, allowing clients to define the structure of the response.
Real-world Applications: GraphQL APIs are popular in applications with complex data requirements, such as social networks, data-intensive applications, and where optimizing data transfer and minimizing network requests are essential.
Example: Using Python's requests
library to interact with a GraphQL API that retrieves user information.
import requests
# GraphQL query
query = """
query {
user(id: 123) {
name
email
age
}
}
"""
# Send GraphQL query to the API endpoint
response = requests.post("https://example.com/graphql", json={'query': query})
if response.status_code == 200:
data = response.json()
user = data['data']['user']
print(user['name'], user['email'], user['age'])
4. WebSocket APIs
WebSocket enable real-time bidirectional communication between clients and servers. They are commonly used for applications that require continuous data streaming, such as chat applications or live dashboards.
Real-world Applications: WebSocket are commonly used in real-time collaboration tools, chat applications, stock market tickers, multiplayer games, and any application that demands instant data transmission and real-time interactions.
Example: Using Python's websockets
library to connect to a WebSocket server.
import asyncio
import websockets
async def receive_messages():
async with websockets.connect('wss://example.com/socket') as websocket:
while True:
message = await websocket.recv()
print("Received:", message)
# Start receiving messages in the background
asyncio.get_event_loop().run_until_complete(receive_messages())
Types of Web Services
1. SOAP Web Service (Simple Object Access Protocol)
SOAP web services use the SOAP protocol for communication and XML for data exchange. They typically rely on the Web Services Description Language (WSDL) for defining the service interface and offer features like security, reliability, and transaction support.
Real-world Applications: Used for enterprise systems integration, financial transactions, and healthcare systems.
Example: Implementing a simple SOAP web service that calculates the sum of two numbers.
from spyne import Application, rpc, ServiceBase, Integer, Decimal
from spyne.protocol.soap import Soap11
from spyne.server.wsgi import WsgiApplication
class CalculatorService(ServiceBase):
@rpc(Integer, Integer, _returns=Decimal)
def add_numbers(ctx, num1, num2):
return num1 + num2
app = Application([CalculatorService], 'calculator',
in_protocol=Soap11(validator='lxml'),
out_protocol=Soap11())
if __name__ == '__main__':
from wsgiref.simple_server import make_server
wsgi_app = WsgiApplication(app)
server = make_server('localhost', 8000, wsgi_app)
print("SOAP server started on http://localhost:8000")
server.serve_forever()
2. RESTful Web Services
RESTful web services adhere to REST principles and use standard HTTP methods for communication. They focus on resource-based interactions, use lightweight data formats like JSON or XML, and provide scalability and simplicity in building web services.
Real-world Applications: Employed in web and mobile applications, social media platforms, and IoT applications.
We can create a simple RESTful web service in Python using the Flask framework. Flask is a lightweight and easy-to-use web framework that allows you to build RESTful APIs quickly.
Example: Implementing a simple RESTful web service that manages a list of books.
from flask import Flask, request, jsonify
app = Flask(__name__)
books = []
@app.route('/api/books', methods=['GET'])
def get_books():
return jsonify(books)
@app.route('/api/books', methods=['POST'])
def add_book():
data = request.json
books.append(data)
return jsonify({"message": "Book added successfully."}), 201
if __name__ == '__main__':
app.run(host='0.0.0.0', port=8000)
3. XML-RPC
XML-RPC is a remote procedure call protocol that allows for remote execution of procedures using XML as the data format. It enables communication between different systems and programming languages.
Real-world Applications: Utilized in content management systems for remote administration.
Here's a simple example of an XML-RPC web service using Python:
Server Code:
from xmlrpc.server import SimpleXMLRPCServer
# Function to add two numbers
def add_numbers(a, b):
return a + b
# Create the server
server = SimpleXMLRPCServer(("localhost", 8000))
print("Listening on port 8000...")
# Register the add_numbers function with the server
server.register_function(add_numbers, "add")
# Run the server's main loop
server.serve_forever()
Client Code:
import xmlrpc.client
# Connect to the server
server = xmlrpc.client.ServerProxy("http://localhost:8000/")
# Call the add function with two numbers
result = server.add(5, 3)
print("Result:", result)
When you execute the code, the client will send a request to the server, and the server will respond by adding the numbers and sending back the result.
4. JSON-RPC
JSON-RPC is a lightweight remote procedure call protocol that uses JSON for data exchange. It provides a simpler and more compact alternative to XML-RPC.
Real-world Applications: Cryptocurrency exchanges use JSON-RPC APIs to enable developers to programmatically interact with the exchange, perform operations like fetching account balances, placing trades, and managing orders.
Example: Implementing a simple JSON-RPC web service to add two numbers.
from jsonrpc import JSONRPCResponseManager, dispatcher
from flask import Flask, request, jsonify
app = Flask(__name__)
@dispatcher.add_method
def add_numbers(x, y):
return x + y
@app.route('/api', methods=['POST'])
def jsonrpc_api():
data = request.get_json()
response = JSONRPCResponseManager.handle(data, dispatcher)
return jsonify(response.data)
if __name__ == '__main__':
app.run(host='0.0.0.0', port=8000)
Wondering which web API style is better suited for your projects? Delve into the insightful blog - SOAP vs REST: What's the difference?, tackling the distinctions between SOAP and REST, and gain valuable insights for your development journey.
Features of an API
- Granularity: APIs provide a fine-grained level of control over the functionalities exposed by an application or service. They allow developers to access and manipulate specific data or services without exposing the underlying implementation details.
- Platform Independence: APIs can be implemented on various platforms and can be accessed by clients developed in different programming languages. They provide a standardized way for different systems to communicate and interact with each other.
- Time Effective: API allows developers to quickly integrate pre-built functionalities, services, or data, reducing development time and effort by leveraging standardized protocols and reusable components.
- Lightweight Communication: APIs typically use lightweight protocols such as HTTP or HTTPS for communication. This enables faster and efficient data transfer, making APIs suitable for web and mobile applications.
- Language Independent: API enables developers to build applications in different programming languages while still being able to interact with the API, promoting interoperability and flexibility in software development.
- Customizable: This feature of an API allows to tailor the API's functionalities, configurations, or data to meet specific application requirements, providing flexibility and adaptability in integration and usage.
Features of Web Services
- Interoperability: Web services enable communication and data exchange between different systems, platforms, and programming languages, promoting interoperability and integration across diverse environments.
- Standardized Protocols: Web services use standardized protocols like SOAP and REST, ensuring consistent and reliable interactions between clients and services, facilitating seamless integration and interoperability.
- XML-based Data Exchange: Web services use XML for data exchange, providing a flexible and structured format that enables seamless integration and sharing of information between systems.
- Security: Web services offer built-in security mechanisms, such as WS-Security, to ensure data integrity, confidentiality, and authentication, enabling secure communication between service providers and consumers.
- Loosely Coupled: Web services promote loose coupling between systems, allowing them to evolve independently, as they communicate through standardized protocols and interfaces, reducing dependencies and enabling flexibility.
Request-Response Model in Web API vs. Web Services
Aspects & Steps Involved | Web API | Web Services |
---|---|---|
Protocol | Primarily uses HTTP as the communication protocol. | Can use various communication protocols such as HTTP, SOAP, REST, XML-RPC, etc. |
Data Exchange Format | Supports various data formats like JSON, XML, and others. | Often relies on XML as the data exchange format, but can also use JSON, SOAP, etc. |
Request | The client sends a request to the API specifying the desired action and data. | The client application sends a request to the web service using a specific protocol. |
Processing | The API server receives and processes the request, executes business logic and access databases if necessary. | The web service receives and processes the request, performing the required actions or operations. |
Response | The API server generates a response based on the request processing and sends it back to the client. | The web service generates a response containing the requested data or the result of the operation and sends it back to the client application. |
Delivery | The API server delivers the response to the client for handling and data extraction. | The web service delivers the response to the client application for handling and processing. |
Client Handling | The client receives the API response and extracts the necessary data or performs the required actions based on the response. | The client application receives the web service response and processes it accordingly.It extracts the requested data from the response and performs any necessary actions based on the received data. |
Transport Security | Can use HTTPS for secure communication. | Can use different security mechanisms depending on the chosen protocol. |
Advantages and Disadvantages of Web APIs
Advantages of APIs
- Flexibility: APIs provide access to specific functions or data, enabling developers to customize and use them as needed.
- Reusability: APIs allow the reuse of code components, saving development time and effort.
- Integration: APIs facilitate seamless communication and data exchange between different systems or applications.
- Platform Independence: APIs can be implemented on various platforms and used with different programming languages.
- Ecosystem Expansion: APIs encourage third-party developers to build applications that extend the functionality of a platform.
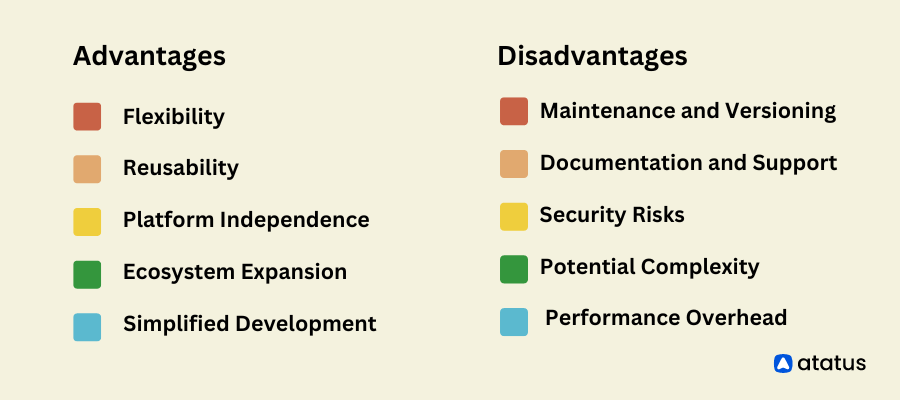
Disadvantages of APIs
- Maintenance and Versioning: APIs require ongoing maintenance and careful versioning to avoid breaking changes.
- Documentation and Support: Providing comprehensive and up-to-date documentation and support for APIs can be challenging.
- Security Risks: APIs need proper security measures to prevent unauthorized access or data breaches.
- Potential Complexity: APIs can have a learning curve and may require understanding specific technologies or protocols.
- Performance Overhead: Depending on implementation, APIs can introduce additional layers and protocols, potentially impacting performance.
Advantages and Disadvantages of Web Services
Advantages of Web Services
- Interoperability: Web services enable seamless communication and data exchange between different systems or platforms.
- Standardization: Web services adhere to standardized protocols and data formats, ensuring consistent interactions.
- Legacy System Integration: Web services facilitate the integration of older systems with newer applications.
- Security: Web services include built-in security mechanisms, ensuring secure communication and data protection.
- Compatibility: Web services can work across different platforms and programming languages, promoting interoperability.
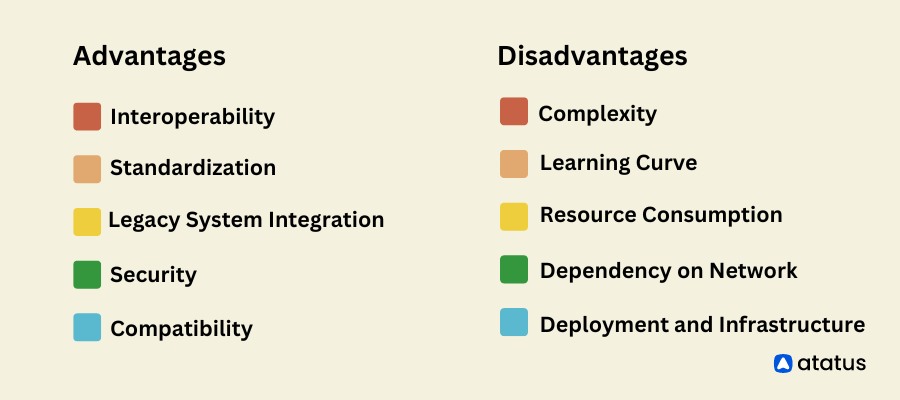
Disadvantages of Web Services
- Complexity: Implementing and working with web services can be more complex compared to simpler API approaches.
- Learning Curve: Utilizing web services may require understanding specific technologies and standards.
- Resource Consumption: Web services can consume more resources compared to lightweight API implementations.
- Dependency on Network: Web services rely on network connectivity, making them susceptible to network-related issues.
- Deployment and Infrastructure: Setting up and maintaining the necessary infrastructure for web services can be challenging.
Conclusion
In conclusion, while APIs and web services share similarities, they are not the same thing. APIs represent a broader concept of enabling communication between software applications, while web services specifically utilize web technologies for communication over the internet.
Understanding this distinction is essential for beginners to navigate the world of software development and integration effectively.
Atatus API Monitoring and Observability
Atatus provides Powerful API Observability to help you debug and prevent API issues. It monitors the consumer experience and is notified when abnormalities or issues arise. You can deeply understand who is using your APIs, how they are used, and the payloads they are sending.
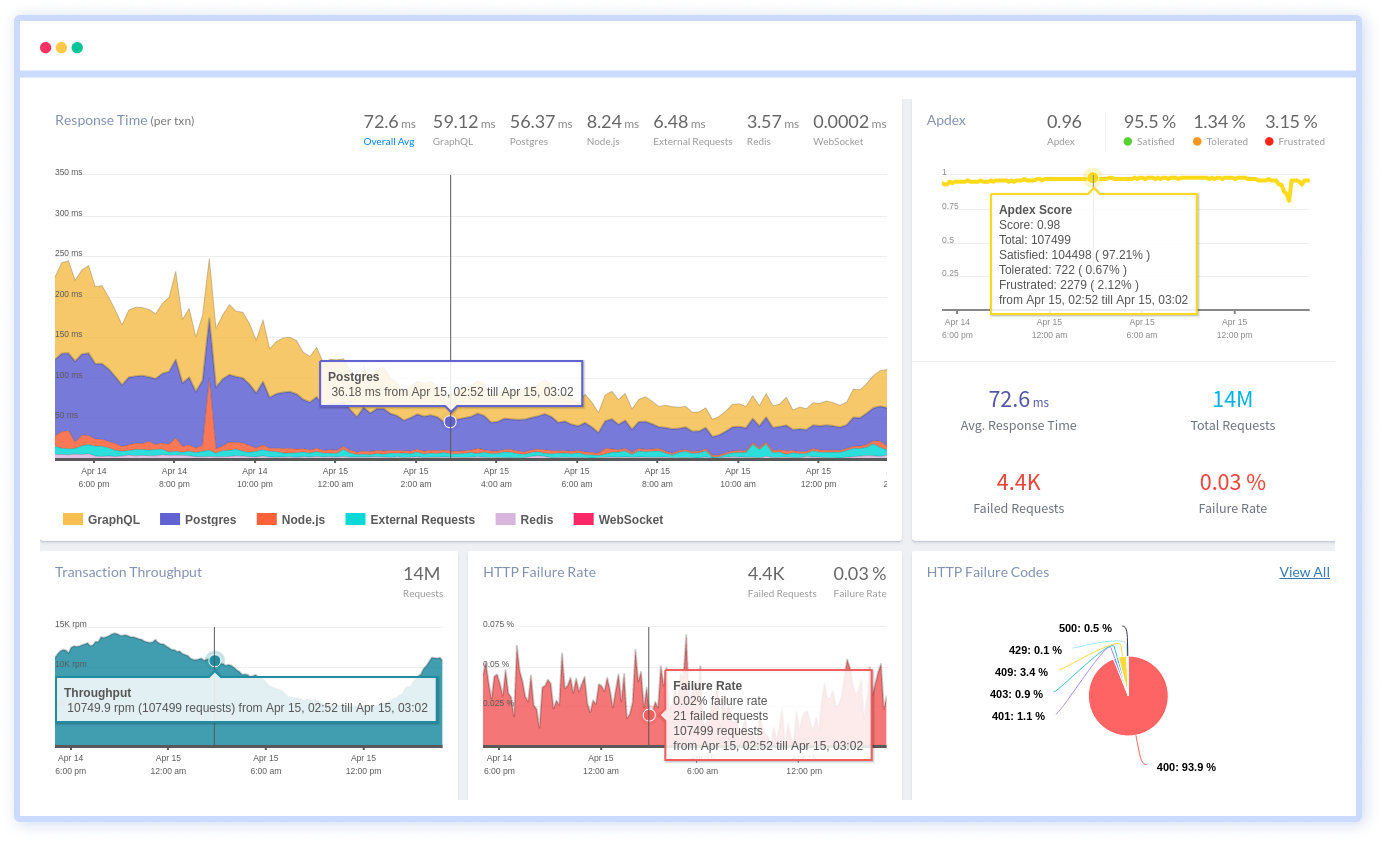
Atatus's user-centric API observability tracks how your actual customers experience your APIs and applications. Customers may easily get metrics on their quota usage, SLAs, and more.
It monitors the functionality, availability, and performance data of your internal, external, and third-party APIs to see how your actual users interact with the API in your application. It also validates rest APIs and keeps track of metrics like latency, response time, and other performance indicators to ensure your application runs smoothly.
#1 Solution for Logs, Traces & Metrics
APM
Kubernetes
Logs
Synthetics
RUM
Serverless
Security
More