What is MEVN Stack? Building a CRUD Application Using MEVN Stack
Nowadays, developers prefer a one-stop solution for web development where developers can build their entire web application on the go.
Full-stack development platforms such as MEVN, MEAN, and MERN are the contemporary solutions for building an end-to-end application.
Developers can create every part of the applications in the same language for every aspect - including a front-end, backend, database, API, security, web server, and more.
It reduces cost, time consumption, and code usage, enhances flexibility, and manages changes regarding scalability.
In this blog, we will explore one of the trending stacks: the MEVN stack. It is an open-source JavaScript software stack that has emerged as a new and evolving way to build powerful and dynamic web applications.
Table Of Contents:
- MEVN Stack Overview
- MEVN Stack Architecture
- Real-time Use Cases of MEVN Stack
- Building a CRUD Application using MEVN Stack
- Advantages and Disadvantages of MEVN Stack
MEVN Stack Overview
The MEVN stack, which stands for MongoDB, Express.js, Vue.js, and Node.js, is a full-stack software development solution built on JavaScript and Vue. It is the most preferable full-stack development platform for software community for websites and web app development.
It's a collection of four JavaScript-related tools with excellent functionality for fast software development. These tools help to create both the front and back ends of your website or app.
Let's explore the four significant technologies that comprise the stack:
- MongoDB is a popular NoSQL database that enables you to store data in a customizable, JSON-like format. It offers a flexible and scalable solution for managing and querying large amounts of data.
- Express.js This Node.js web application framework makes building scalable and modular online applications easier. The functionalities that Express.js offers make building online and mobile applications fast and with little effort.
- Vue.js is a progressive JavaScript framework for developing user interfaces. It is designed to be gradually adaptable, which means that it can be seamlessly integrated into existing projects. Vue.js is well-known for its simplicity and adaptability, making it a popular front-end development framework.
- Node.js is a server-side JavaScript runtime that lets developers create scalable, high-performance online apps. It is event-driven and non-blocking, making it ideal for managing several concurrent connections.
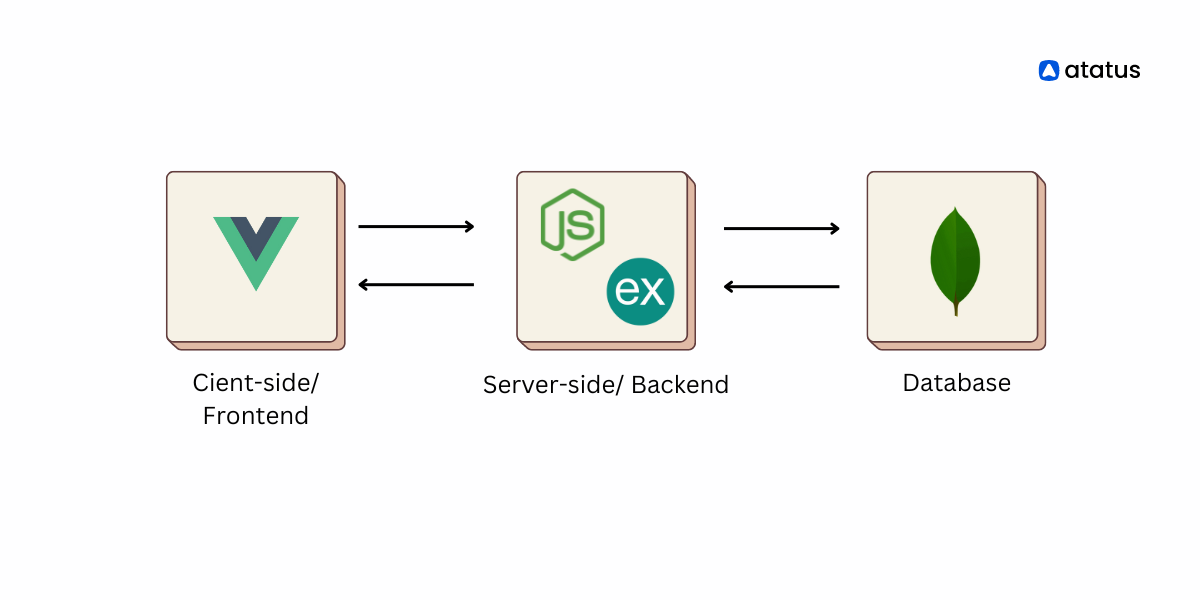
MEVN Stack Architecture
i.) Front-end (Vue.js) -Vue.js creates the user interface and manages client-side interactions. Because of its reactive and component-based architecture, managing and updating the user interface dynamically is simple.
ii.) Backend (Node.js and Express.js) -Node.js and Express.js collaborate to handle server-side logic and maintain the app's backend. They improve communication between the front end and the database by effectively handling requests and responses.
iii.) Database (MongoDB) - A versatile, JSON-like format is used by MongoDB, a NoSQL database, to store and retrieve data. It offers scalability and permits smooth integration with Express.js and Node.js.
iv.) Communication -HTTP/HTTPS is a language that Vue.js (the user interface) and Node.js (the server) use to communicate with one another.
v.) API (Application Programming Interface) - Serves as an intermediary between Vue.js and Express.js. It handles all HTTP requests from the frontend, processes them, interacts with the database, and returns back responses to the frontend framework.
vi.) RESTful API - This is an interface that Vue.js uses to communicate with Node.js and request information from it. It is comparable to having a waiter take orders and deliver food.
vii.) Middleware -Think of this as an Express.js assistant. Its additional responsibilities include managing problems and determining whether the user is authorized to access particular areas.
viii.) Build Tools and Package Managers - For example, Webpack and Babel: These tools help organize and prepare the code to ensure that the website or app functions properly.
ix.) npm or Yarn - These are similar to magic bullets in that they assist with organizing and monitoring all the equipment and supplies required for the task.
x.) Deployment Hosting Services - Once the website or app is complete, it requires a home on the Internet. Hosting providers, such as AWS or Heroku, function like landlords, providing a space to dwell.
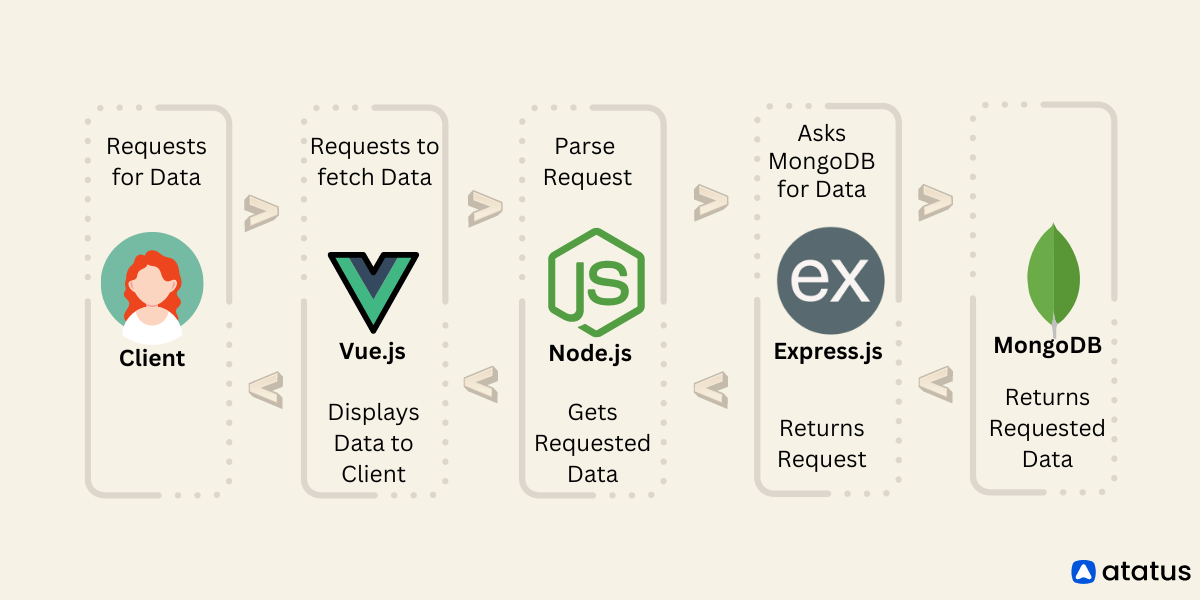
Real-time Use Cases of the MEVN Stack
The MEVN stack supports a variety of use cases, including:
1. Real-time Applications
MEVN is a good choice for developing real-time applications, such as live-streaming platforms, chat apps, and collaboration tools. Vue.js's reactivity and Node.js's event-driven design enable smooth processing of real-time updates.
2. Single Page Applications (SPAs)
MEVN is the best tool for creating SPAs where a fluid and dynamic user interface is essential. With its component-based architecture, Vue.js makes it possible to create dynamic and adaptable user interfaces, while Node.js effectively manages server-side logic.
3. Content Management Systems (CMS)
MEVN is useful for developing powerful content management systems that require dynamic content creation, editing, and publishing. Vue.js provides a user-friendly admin interface, while MongoDB's versatility enables simple content storage and retrieval.
4. E-commerce Platforms
MEVN-based e-commerce systems are a potential solution. Vue.js can handle dynamic and interactive user interface elements, while Express.js and Node.js handle server-side functionality. MongoDB's scalability makes it ideal for managing big product databases.
5. Startups and Prototyping
Startups frequently pick MEVN because of its simplicity and ability to prototype and produce minimum viable products (MVPs) quickly. The full-stack approach lowers the need to transition between multiple technologies, resulting in speedier development cycles.
6. Mobile Applications (Hybrid)
MEVN can be used to create hybrid mobile apps with frameworks such as Cordova or Capacitor. Vue.js can be used to design the mobile app's user experience, while Node.js is responsible for business logic and data storage.
7. Data Dashboards and Analytical Tools
MEVN is highly suited to creating data dashboards and analytics solutions. Vue.js allows you to construct visually beautiful and interactive charts, whereas Node.js handles server-side data processing. MongoDB's versatility makes it ideal for storing and retrieving analytical data.
Building a CRUD Application Using MEVN Stack
The MEVN stack consists of the front-end framework Vue.js, the backend framework Express.js, the database MongoDB, and the runtime environment Node.js.
Building a CRUD (Create, Read, Update, Delete) application involves creating the components required for each operation. Below is a condensed illustration of an MEVN stack CRUD application.
Verify that MongoDB, npm, and Node.js are installed on your computer.
Step 1: Set up the backend (Express.js and Node.js)
Make a new project folder and go to it as follows:
mkdir mevn-crud-app
cd mevn-crud-app
Launch a Node.js project and install the required libraries:
npm init -y
npm install express mongoose cors body-parser
Create an index.js
file in your express server to handle CRUD Operations:
// index.js
const express = require('express');
const mongoose = require('mongoose');
const bodyParser = require('body-parser');
const cors = require('cors');
const app = express();
const PORT = process.env.PORT || 3000;
// Middleware
app.use(bodyParser.json());
app.use(cors());
// MongoDB connection
mongoose.connect('mongodb://localhost:27017/my_database', {
useNewUrlParser: true,
useUnifiedTopology: true
});
// Define Schema
const TodoSchema = new mongoose.Schema({
title: String,
description: String,
});
const Todo = mongoose.model('Todo', TodoSchema);
// Routes
app.get('/api/todos', async (req, res) => {
try {
const todos = await Todo.find();
res.json(todos);
} catch (err) {
res.status(500).json({ message: err.message });
}
});
app.post('/api/todos', async (req, res) => {
const todo = new Todo({
title: req.body.title,
description: req.body.description
});
try {
const newTodo = await todo.save();
res.status(201).json(newTodo);
} catch (err) {
res.status(400).json({ message: err.message });
}
});
app.put('/api/todos/:id', async (req, res) => {
try {
await Todo.findByIdAndUpdate(req.params.id, req.body);
res.json({ message: 'Todo updated successfully' });
} catch (err) {
res.status(500).json({ message: err.message });
}
});
app.delete('/api/todos/:id', async (req, res) => {
try {
await Todo.findByIdAndDelete(req.params.id);
res.json({ message: 'Todo deleted successfully' });
} catch (err) {
res.status(500).json({ message: err.message });
}
});
// Start server
app.listen(PORT, () => {
console.log(`Server is running on port ${PORT}`);
});
Here's a rundown of what each part of the code does:
Middleware Setup:
body-parser
: Parses incoming request bodies.cors
: Enables Cross-Origin Resource Sharing, allowing requests from different origins.
MongoDB Connection:
mongoose.connect()
: Connects to the MongoDB database named 'my_database' running on localhost.
Define Schema:
TodoSchema
: Defines the schema for the Todo model withtitle
anddescription
fields.
Routes:
GET /api/todos
: Fetches all todos from the database.POST /api/todos
: Creates a new todo.PUT /api/todos/:id
: Updates a todo with the specified id.DELETE /api/todos/:id
: Deletes a todo with the specified id.
Server Setup:
app.listen()
: Starts the server on the specified port.
This code provides a solid foundation for a CRUD API with basic error handling. You can extend it further by adding validation, authentication, pagination, and more depending on your project requirements.
Make sure you have MongoDB running on your local machine and have installed the necessary Node.js packages (express
, mongoose
, body-parser
, cors
) before running this code. You can start the server by running node index.js
assuming you saved the file as index.js
.
Step 2: Set up the frontend (Vue.js)
Globally install the Vue CLI:
npm install -g @vue/cli
Make a project in Vue:
vue create client
cd client
Set up Axios to send HTTP requests:
npm install axios
Change src/main.js
and src/App.vue
to build a basic frontend:
<template>
<div id="app">
<h1>MEVN CRUD App</h1>
<AddTodo @add="addTodo" />
<TodoList :todos="todos" @edit="editTodo" @delete="confirmDelete" />
<EditTodo v-if="editMode" :todo="selectedTodo" @update="updateTodo" />
<DeleteTodo v-if="deleteMode" @delete="deleteTodo" @cancel="cancelDelete" />
</div>
</template>
<script>
import axios from 'axios';
import TodoList from './components/TodoList.vue';
import AddTodo from './components/AddTodo.vue';
import EditTodo from './components/EditTodo.vue';
import DeleteTodo from './components/DeleteTodo.vue';
export default {
name: 'App',
components: {
TodoList,
AddTodo,
EditTodo,
DeleteTodo
},
data() {
return {
todos: [],
editMode: false,
deleteMode: false,
selectedTodo: null
};
},
methods: {
async fetchTodos() {
try {
const response = await axios.get('http://localhost:3000/api/todos');
this.todos = response.data;
} catch (error) {
console.error(error);
}
},
async addTodo(todo) {
try {
await axios.post('http://localhost:3000/api/todos', todo);
await this.fetchTodos();
} catch (error) {
console.error(error);
}
},
editTodo(todo) {
this.selectedTodo = todo;
this.editMode = true;
},
async updateTodo(updatedTodo) {
try {
await axios.put(`http://localhost:3000/api/todos/${updatedTodo._id}`, updatedTodo);
this.editMode = false;
this.selectedTodo = null;
await this.fetchTodos();
} catch (error) {
console.error(error);
}
},
confirmDelete(todo) {
this.selectedTodo = todo;
this.deleteMode = true;
},
async deleteTodo() {
try {
await axios.delete(`http://localhost:3000/api/todos/${this.selectedTodo._id}`);
this.deleteMode = false;
this.selectedTodo = null;
await this.fetchTodos();
} catch (error) {
console.error(error);
}
},
cancelDelete() {
this.deleteMode = false;
this.selectedTodo = null;
}
},
mounted() {
this.fetchTodos();
}
};
</script>
This file will initialize our Vue.js application and mount it to an HTML element with the id app
.
import Vue from 'vue';
import App from './App.vue';
Vue.config.productionTip = false;
new Vue({
render: h => h(App),
}).$mount('#app');
Step 3: Run the application
In one terminal, launch your Express.js server:
node server.js
In another terminal, navigate to the client folder and launch the Vue.js application:
cd client
npm run serve
Visit http://localhost:8080
in your browser to see the MEVN CRUD application in action.
Keep in mind that this is only a basic example; in a real-world setting, you would also need to take security, validation, and other factors into account. Furthermore, you may wish to utilise a more complex state management system, like Vuex, for the front end.
Advantages and Disadvantages of the MEVN Stack
Advantages
- Full Stack in JavaScript - With the MEVN stack, developers can use JavaScript for both front-end and back-end development, giving them a uniform language to work with from start to finish.
- Flexibility and Scalability - The stack can be used to construct applications of various sizes and complexity because each component is made to be flexible and scalable.
- Modularity - Because Vue.js, Express.js, and Node.js are modular, developers can construct and manage various application components independently, which facilitates a more structured and effective development process.
- Community Support - Due to the MEVN stack's increased popularity, there is a sizable and vibrant community. Thanks to this community support, developers can access many tools, tutorials, and third-party libraries.
Disadvantages
- Learning Curve - Despite its reputation for simplicity, Vue.js may present a learning curve for new developers. If a team is already familiar with other front-end frameworks, such as React or Angular, migrating to Vue.js may take some time to adjust.
- Maturity - Compared to Angular and React, Vue.js is comparatively more recent. Despite its quick rise to fame, it may not have the same level of maturity and sustained community support as its contemporaries. This may affect the framework's stability and resource availability.
- Corporate Backing - Corporate support for Vue.js is not as strong as it is for React (Facebook) or Angular (Google). Some businesses may find this concerning when it comes to ongoing maintenance, updates, and support.
- Integration Issues - Due to the stack's recent age, Integrating third-party tools and services may be more difficult. Compatibility concerns may develop when attempting to add specific libraries or tools to the MEVN stack.
- Complexity for Simple Applications - The MEVN stack may be seen as overkill for simple or small-scale applications. The full-stack approach and use of MongoDB, a NoSQL database, may add extra complexity to simpler projects.
Conclusion
The MEVN technology stack offers a comprehensive, JavaScript-focused method for creating scalable and reliable online applications.
With MEVN, developers can create engaging and dynamic user experiences by leveraging a single language, robust components, and a vibrant ecosystem. Despite some difficulties, the advantages and useful uses of the MEVN stack demonstrate its promise in the rapidly changing field of web development today.
MEVN Stack Development creates responsive website applications for desktop computers, laptop computers, mobile devices, and gaming systems using Java stack components.
A use cases' requirements, the experience of the development team, the need for scalability, and long-term maintainability should all be considered while selecting the MEVN stack.
Monitor Your Entire Application with Atatus
Atatus is a Full Stack Observability Platform that lets you review problems as if they happened in your application. Instead of guessing why errors happen or asking users for screenshots and log dumps, Atatus lets you replay the session to quickly understand what went wrong.
We offer Application Performance Monitoring, Real User Monitoring, Server Monitoring, Logs Monitoring, Synthetic Monitoring, Uptime Monitoring and API Analytics. It works perfectly with any application, regardless of framework, and has plugins.
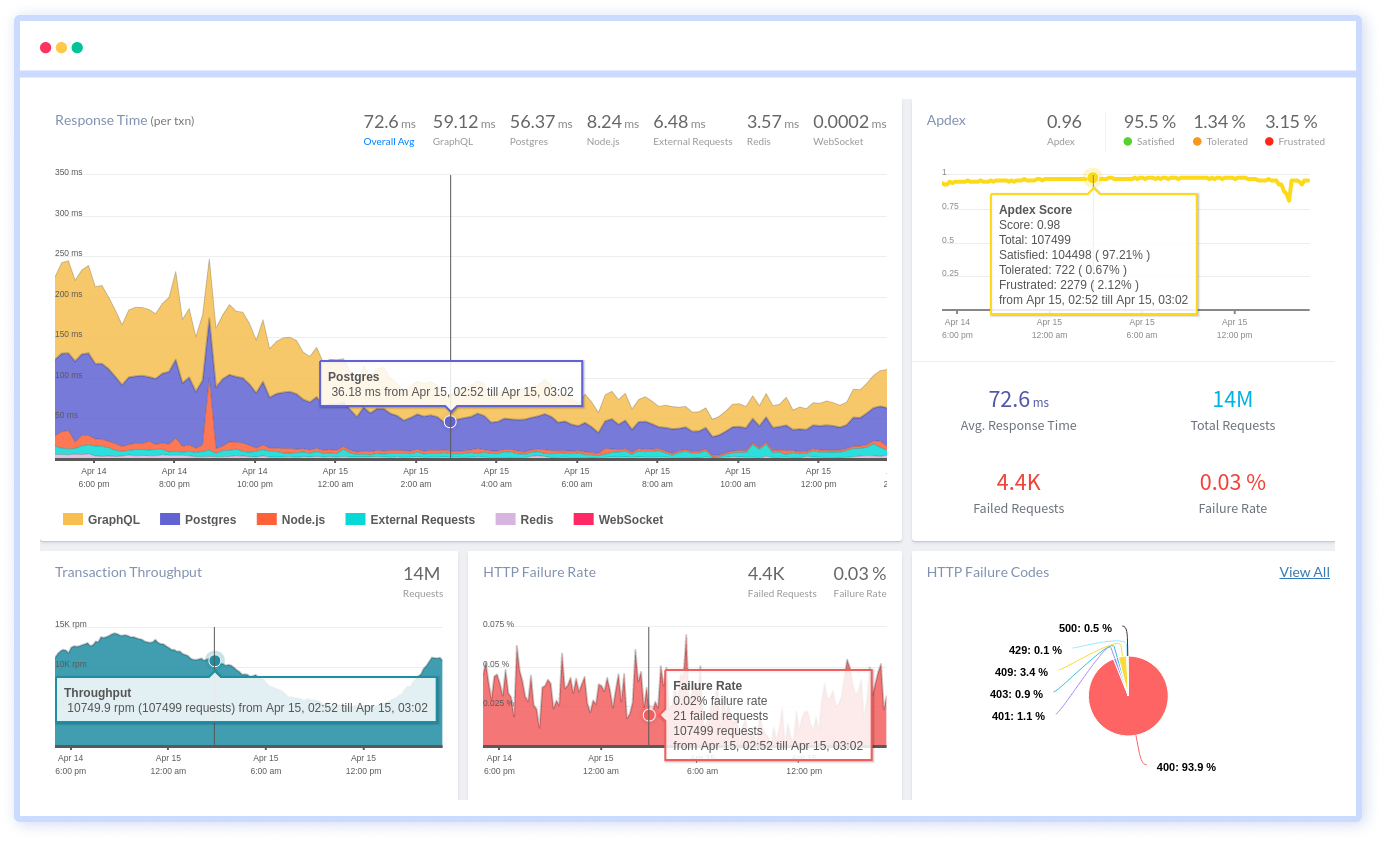
Atatus can be beneficial to your business, which provides a comprehensive view of your application, including how it works, where performance bottlenecks exist, which users are most impacted, and which errors break your code for your frontend, backend, and infrastructure.
If you are not yet a Atatus customer, you can sign up for a 14-day free trial .
#1 Solution for Logs, Traces & Metrics
APM
Kubernetes
Logs
Synthetics
RUM
Serverless
Security
More