Java Performance Optimization Tips
Java performance optimization is crucial for several reasons. First and foremost, it directly impacts the user experience. Faster and more responsive applications lead to a better user experience, reducing loading times and response delays.
Users expect applications to be snappy and efficient, and optimization helps achieve this, enhancing user satisfaction.
Furthermore, optimization improves resource efficiency, ensuring that the Java code consumes fewer system resources such as CPU, memory, and disk I/O. This efficiency allows the application to run more smoothly and leaves more resources available for other processes, contributing to overall system stability.
Scalability is another significant benefit of performance optimization. Well-optimized Java applications are more scalable, capable of handling increased loads and concurrent users without a significant drop in performance.
This scalability is crucial for web applications and services that need to accommodate growing demands over time.
Table of Content
- Why java optimization is crucial?
- Common Java Performance Pitfalls to avoid
- Tips to Enhance Java Performance
Why java optimization is crucial?
Java performance optimization is crucial for several reasons:
- Cost Savings: Optimized applications require fewer hardware resources to achieve the desired performance levels. This can result in cost savings, as less powerful hardware can be used to achieve the same performance or higher performance can be achieved with the same hardware.
- Energy Efficiency: Performance optimization often leads to reduced energy consumption. In energy-conscious environments, such as data centers or mobile devices, this can significantly impact operational costs and battery life.
- Competitiveness: In today's competitive software market, performance is a key differentiator. Faster and more efficient applications can attract and retain more users, giving a competitive advantage over slower alternatives.
- Reduced Latency: Optimized Java code can help reduce network and response latencies, improving the overall efficiency of networked applications, especially in distributed systems.
- Meeting SLAs (Service Level Agreements): For enterprise applications or services, meeting SLAs is critical. Performance optimization ensures that applications meet response time and throughput requirements.
- Better Resource Utilization: Proper optimization ensures that resources are used optimally, minimizing waste and improving system stability. This is particularly important in cloud computing environments, where resource usage can directly impact costs.
- Future-Proofing: As applications and datasets grow over time, unoptimized code may become a performance bottleneck. By optimizing early on, developers can ensure that the application can handle increasing workloads and maintain high performance over time.
- Compatibility with Target Devices: For Java applications running on mobile devices, embedded systems, or IoT devices, optimization is essential to ensure that the application runs smoothly within the constraints of the target hardware.
Java performance optimization is crucial to deliver efficient, fast, and responsive applications that can meet user expectations, scale with demand, and remain competitive in the ever-evolving software landscape. By focusing on optimization, developers can enhance resource efficiency, reduce costs, and improve overall system performance.
Common Java Performance Pitfalls to avoid
Java optimization, while important for improving performance, can also lead to common pitfalls if not done carefully. Here are some of the common downfalls to be aware of when optimizing Java:
- Premature Optimization
- Lack of Proper Benchmarking
- Overuse of Micro-Optimizations
- Sacrificing Readability and Maintainability
- Ignoring Algorithmic Complexity
- Not Considering Memory Usage
- Neglecting Garbage Collection
- Ignoring Platform-Specific Differences
- Not Utilizing JVM Optimizations
- Not Considering Parallelism
- Copy-Pasting Code for Optimization
- Unawareness of Library Optimizations
1. Premature Optimization
Premature optimization occurs when developers attempt to optimize code before identifying actual performance bottlenecks. In this example, the code performs some operation in a loop without any knowledge of whether this loop is a significant bottleneck in the application.
// Premature optimization without profiling
for (int i = 0; i < 1000000; i++) {
// Some code that might not be a significant bottleneck
}
2. Lack of Proper Benchmarking
Proper benchmarking is crucial to measure the impact of optimizations accurately. In this example, we measure the elapsed time for executing the optimized code using System.nanoTime()
. However, without proper comparisons to the unoptimized code or a larger sample size, the results may not be reliable.
long startTime = System.nanoTime();
// Optimized code here
long endTime = System.nanoTime();
long elapsedTime = endTime - startTime;
System.out.println("Elapsed Time: " + elapsedTime + " nanoseconds");
3. Overuse of Micro-Optimizations
Micro-optimizations focus on small, low-level code improvements. While they may provide minor performance gains, excessive use of micro-optimizations can hinder code readability and maintainability without delivering significant benefits.
// Overusing loop unrolling for micro-optimization
for (int i = 0; i < 1000000; i += 2) {
// Some code
}
4. Sacrificing Readability and Maintainability
Sacrificing code readability and maintainability for minor performance gains can lead to code that is difficult to understand and maintain. In this example, using a while loop instead of a for loop negatively impacts code readability.
// Sacrificing readability for optimization
int i = 0; while (i < 1000000) { /* Some code */ i++; }
5. Ignoring Algorithmic Complexity
Ignoring the algorithmic complexity of code can lead to suboptimal performance. In this example, the code uses an inefficient O(n^2) algorithm to find the maximum element in an array.
// O(n^2) algorithm for finding the maximum element in an array
int findMax(int[] arr) {
int max = arr[0];
for (int i = 0; i < arr.length; i++) {
for (int j = 0; j < arr.length; j++) {
if (arr[j] > max) {
max = arr[j];
}
}
}
return max;
}
6. Not Considering Memory Usage
Optimizing for speed alone without considering memory usage can lead to increased memory consumption. In this example, an ArrayList with a large initial capacity is used when a smaller data structure might suffice.
// Using a large array when a smaller data structure would suffice
List<Integer> list = new ArrayList<>(1000000);
7. Neglecting Garbage Collection
Failing to optimize object creation and garbage collection can lead to increased memory churn and performance degradation. In this example, string concatenation creates unnecessary intermediate String objects.
String concatenateStrings(String a, String b, String c) {
return a + b + c; // Creates intermediate String objects
}
8. Ignoring Platform-Specific Differences
Ignoring platform-specific differences can lead to suboptimal performance on specific JVM implementations or operating systems.
// Not considering differences in JVM implementations for optimization
String osName = System.getProperty("os.name");
if (osName.startsWith("Windows")) {
// Windows-specific code
} else {
// Non-Windows code
}
9. Not Utilizing JVM Optimizations
Explanation: Modern JVMs have sophisticated optimization techniques. In this example, not using the built-in array initializer may prevent certain JVM optimizations.
// Avoiding array initialization with 'new' (inefficient in older JVMs)
int[] arr = {1, 2, 3};
10. Not Considering Parallelism
Failing to leverage parallelism when applicable can limit the performance potential of multi-core processors.
// Sequential summing of an array (not leveraging parallelism)
int sum = 0;
for (int i = 0; i < arr.length; i++) {
sum += arr[i];
}
11. Copy-Pasting Code for Optimization
Copy-pasting similar code instead of using a method can lead to code duplication and maintenance issues.
// Copy-pasting similar code instead of using a method
int calculateArea(int length, int width) {
return length * width;
}
int calculateVolume(int length, int width, int height) {
return length * width * height;
}
12. Unawareness of Library Optimizations
Not utilizing built-in library optimizations can result in reinventing the wheel and missed opportunities for performance gains.
// Using a custom sorting algorithm instead of Collections.sort()
List<Integer> numbers = new ArrayList<>();
// ... add elements to the list ...
customSort(numbers); // Custom sorting algorithm
// Instead, use built-in library sorting
Collections.sort(numbers);
Tips to Enhance Java Performance
Below are some common pitfalls to avoid and tips to enhance the performance of your Java applications:
- Use Proper Data Structures
- Minimize Object Creation
- String Concatenation
- Use StringBuilder or StringBuffer
- Be Mindful of Autoboxing
- Optimize Loops
- Reduce Synchronization
- Use Primitives Instead of Wrapper Classes
- Properly Size Collections
- Optimize I/O Operations
1. Use Proper Data Structures
Choose the right data structures for your specific use case. ArrayLists are efficient for random access, LinkedLists for frequent insertions/deletions, HashMaps for quick key-value lookups, etc.
Understand the time complexity of operations on different data structures to make informed decisions.
List<Integer> arrayList = new ArrayList<>();
arrayList.add(10);
arrayList.add(20);
arrayList.add(30);
int element = arrayList.get(1); // Efficient random access
In this example, we have used an array list for random access.
2. Minimize Object Creation
Creating unnecessary objects can lead to increased garbage collection overhead. Reuse objects when possible, especially in loops, by employing object pooling or recycling mechanisms.
import java.util.LinkedList;
import java.util.Queue;
class ObjectPool {
private Queue<MyObject> pool = new LinkedList<>();
public MyObject getObject() {
if (pool.isEmpty()) {
return new MyObject(); // Create a new object if the pool is empty
} else {
return pool.poll(); // Reuse an existing object
}
}
public void releaseObject(MyObject object) {
// Reset the object state (if needed) before returning it to the pool
pool.add(object);
}
}
In this example, we have reused objects with object pool.
3. String Concatenation
Avoid using string concatenation (+) in loops as it creates a new String object each time. Instead, use StringBuilder
or StringBuffer
for efficient string manipulation.
StringBuilder sb = new StringBuilder();
for (int i = 0; i < 10000; i++) {
sb.append(i).append(", ");
}
String result = sb.toString();
This code creates a StringBuilder
and appends numbers from 0 to 9999, followed by a comma and a space, to it. The resulting string is stored in the result
variable.
4. Use StringBuilder or StringBuffer
When constructing strings dynamically, use StringBuilder
(non-thread-safe) or StringBuffer
(thread-safe) instead of repeated concatenation to avoid unnecessary object creation and copying.
StringBuilder sb = new StringBuilder();
for (int i = 0; i < 10000; i++) {
sb.append("Element ").append(i).append(", ");
}
String result = sb.toString();
In this example, we have used StringBuilder to concatenate strings efficiently.
5. Be Mindful of Autoboxing
Beware of autoboxing and unboxing when converting between primitive types and their wrapper classes. These operations can introduce unnecessary object creation and impact performance.
List<Integer> list = new ArrayList<>();
for (int i = 0; i < 10000; i++) {
list.add(i); // Prefer adding int directly, not Integer
}
In this code, an ArrayList
of Integer
objects is created, and a loop is used to add integers from 0 to 9999 to the list. The comment suggests that adding int
directly is preferable over using the Integer
wrapper type.
6. Optimize Loops
Minimize the work done inside loops, move invariant computations outside the loop, and use the most efficient loop constructs (e.g., for-each loop, while loop) depending on your requirements.
int length = someArray.length;
for (int i = 0; i < length; i++) {
// Some operation using 'i' and 'someArray[i]'
}
Here is an example of loop orientation where we move invariant computation outside the loop.
7. Reduce Synchronization
Synchronization can lead to contention and performance bottlenecks. Minimize synchronized blocks or methods when not necessary. Consider using concurrent data structures from the `java.util.concurrent` package for multi-threaded scenarios.
// Perform concurrent operations on the map without explicit synchronization
ConcurrentHashMap<String, Integer> map = new ConcurrentHashMap<>();
Here in this example, we have used a concurrent data structure instead of a synchronized block.
8. Use Primitives Instead of Wrapper Classes
Prefer using primitive data types (e.g., int, double) over their corresponding wrapper classes (e.g., Integer, Double) when dealing with large datasets or performance-critical sections.
int sum = 0;
for (int i = 0; i < 10000; i++) {
sum += i; // Prefer using int instead of Integer for better performance
}
In this example, we have used primitive data types instead of wrapper classes.
9. Properly Size Collections
When creating collections like ArrayLists or HashMaps, provide an initial capacity that is reasonably close to the expected size to reduce the frequency of resizing, which can be expensive.
int initialCapacity = 10000;
List<Integer> arrayList = new ArrayList<>(initialCapacity);
for (int i = 0; i < initialCapacity; i++) {
arrayList.add(i);
}
In this code, an ArrayList
is created with an initial capacity of 10000. Then, a loop is used to add integers from 0 to 9999 to the list. The specified initial capacity can help optimize memory usage and performance if you know the expected number of elements in advance.
10. Optimize I/O Operations
Use buffered I/O streams for reading and writing data to reduce disk access overhead. Also, avoid reading or writing data one byte at a time.
try (BufferedReader br = new BufferedReader(new FileReader("file.txt"))) {
String line;
while ((line = br.readLine()) != null) {
// Process each line
}
} catch (IOException e) {
e.printStackTrace();
}
In this example, we have used buffered I/O streams, for efficient file reading.
Other Java performance tips include:
- Use profiling tools like VisualVM or Java Mission Control to identify performance bottlenecks and hotspots in your application. This will help you target specific areas for optimization.
- The Just-In-Time (JIT) compiler optimizes your Java bytecode during runtime. Ensure you are running your application with an appropriate JVM version and optimize the JVM settings for your application's workload.
- Minimize the use of global variables, as they can make it harder for the JVM to optimize your code due to increased scope and potential aliasing issues. Declare variables as final wherever possible. Use immutable data structures to reduce the risk of unintended changes and enable certain optimizations.
- When working with resources like files or database connections, use try-with-resources to ensure proper resource handling and release. Consider offloading heavy computations or time-consuming tasks to background threads or separate worker services to keep the main application responsive.
- Choose the appropriate collection type based on your needs. For instance, use Set for unique elements, List for ordered elements with duplicates, and Map for key-value associations.
- Exceptions should be reserved for exceptional cases. Overusing exceptions for regular control flow can negatively impact performance. For performance-critical I/O operations, consider using external libraries like Apache Commons IO or Google Guava, which may offer better performance than standard Java I/O classes.
Conclusion
Java performance optimization is pivotal for delivering responsive and efficient applications. By optimizing code, developers can enhance user satisfaction, reduce resource consumption, and lower operational costs.
Additionally, well-optimized applications are better prepared to handle scalability demands and maintain high performance over time, ensuring a competitive edge in the software landscape.
Striking a balance between enhancements, maintainability, and readability yields exceptional software experiences in today's competitive landscape.
Monitor your Java application for errors & exceptions with Atatus
When it comes to ensuring the optimal performance and reliability of your Java applications, monitoring is key. The ability to detect bottlenecks, identify slowdowns, and troubleshoot issues in real-time can significantly enhance the user experience and streamline your development workflow. That's where Atatus comes in.
With its powerful features and intuitive interface, you can gain deep insights into your application's performance metrics, traces, and errors. By visualizing the entire request lifecycle, pinpointing problematic areas becomes a breeze.
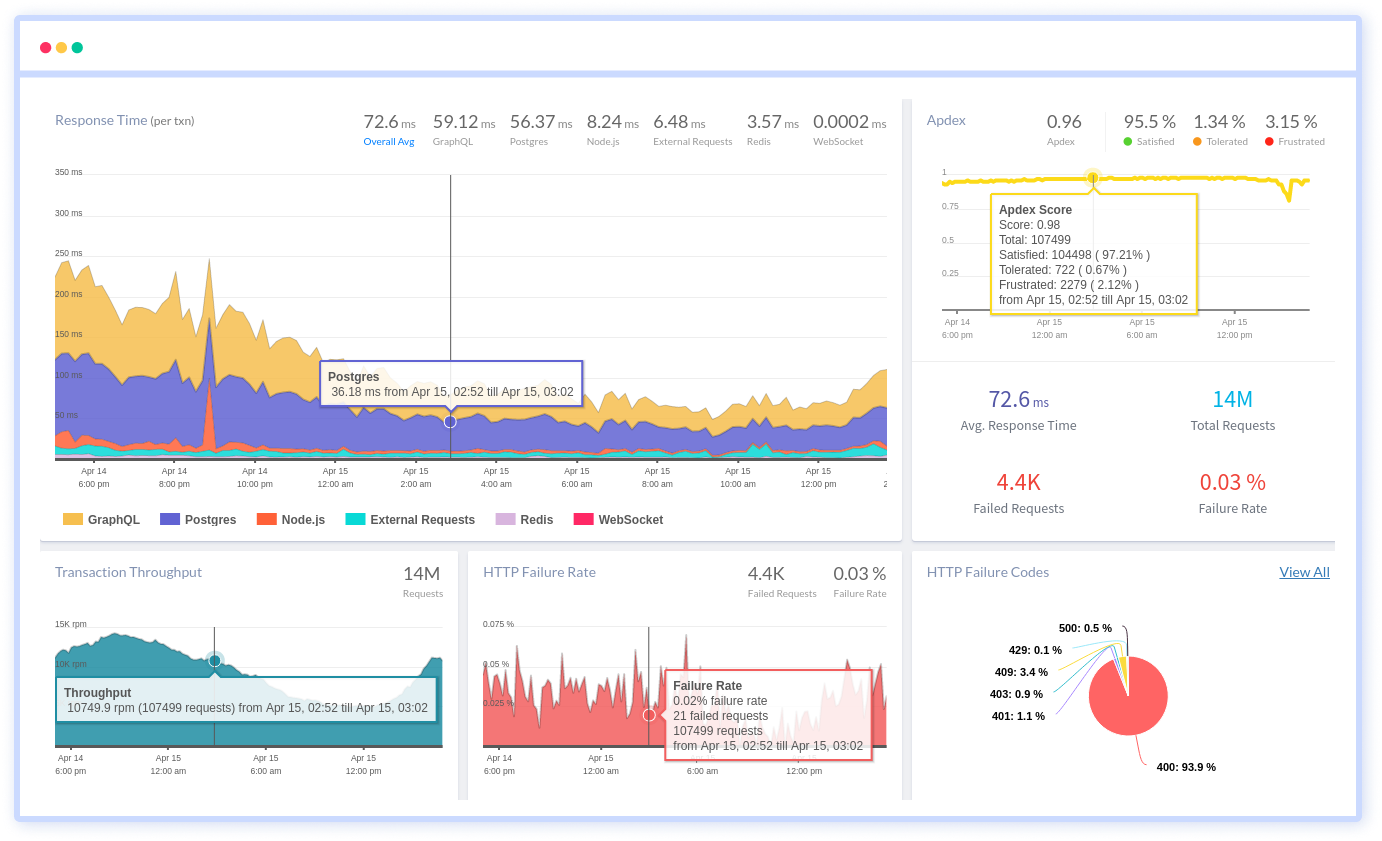
With Atatus Java performance monitoring you can:
- Root Cause Analysis: Quickly pinpoint the root causes of performance regressions.
- Improve User Experience: Deliver faster response times and smoother interactions for users.
- Code Profiling: Profile code execution to identify CPU-intensive or slow sections.
- Reduced Downtime: Minimize downtime through early identification and resolution of issues.
If you are not yet a Atatus customer, you can sign up for a 14-day free trial .
#1 Solution for Logs, Traces & Metrics
APM
Kubernetes
Logs
Synthetics
RUM
Serverless
Security
More