Node.js Timeout Tactics: A Complete Guide
Node.js is a powerful platform for building scalable and efficient server-side applications. Implementing effective timeout strategies in Node.js is crucial for preventing performance bottlenecks and ensuring application responsiveness. One essential feature of Node.js development is managing timeouts effectively. Timeouts help handle various scenarios, such as preventing requests from hanging indefinitely or executing tasks within a specific timeframe.
Let's get into the timeouts as time is out, what timeouts mean, and why they are necessary for Node.js applications.
- What are timeouts in Node.js
- Why are timeouts essential for Node.js applications?
- Types of timeouts
- Why are timeouts essential for Node.js applications?
- Best Practices for Timeout Management
What are timeouts in Node.js?
Implementing timeouts in Node.js allows developers to control the duration of various operations, enhancing application performance and preventing indefinite hanging of processes. If the task or operation exceeds this predefined time limit, Node.js interferes by terminating or interrupting the execution, thereby preventing potential bottlenecks or performance issues.
To prevent the indefinite hanging of certain operations, to improve application responsiveness, and to optimize resource allocation, timeouts are essential. They play an important role in maintaining the reliability, efficiency, and responsiveness of Node.js applications.
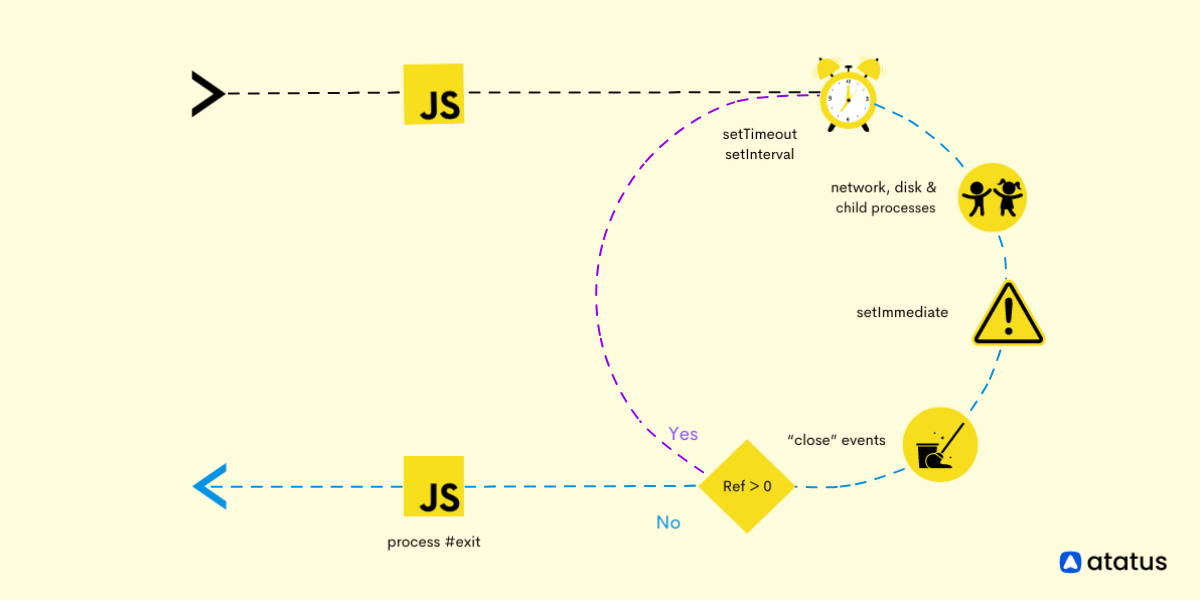
Why are timeouts essential for Node.js applications?
- Preventing Blocking Operations: If a particular operation takes too long to complete, it can block the event loop, causing other operations to be delayed or preventing new incoming requests from being processed. Timeouts help prevent such blocking operations by enforcing a maximum time limit for execution.
- Improving Application Responsiveness: By setting timeouts for various operations, Node.js applications can remain responsive to user requests and interactions. For example, suppose an HTTP request to an external API takes too long to respond. In that case, a timeout can prevent the application from waiting indefinitely and instead handle the timeout properly, returning an appropriate response to the user.
- Reducing Resource Exhaustion: Long-running operations can consume significant resources such as memory, CPU, and network bandwidth. By enforcing timeouts, Node.js applications can limit the resources allocated to a particular operation, preventing resource exhaustion and maintaining the overall stability and performance of the application.
- Handling Unpredictable Scenarios: Network delays, database timeouts, and other unexpected issues can occur in distributed systems. Timeouts provide a medium for handling such scenarios by specifying a maximum acceptable wait time. If the operation exceeds this time limit, the application can take suitable action, such as retrying the operation, returning an error message, or terminating the operation.
- Improving Failures: Timeouts contribute to the fault tolerance of Node.js applications by preventing them from becoming unresponsive or entering into an indefinite wait state when facing issues such as network failures or unresponsive external services. By setting suitable timeouts, applications can recover from transient failures more effectively and operate reliably under unfavorable conditions.
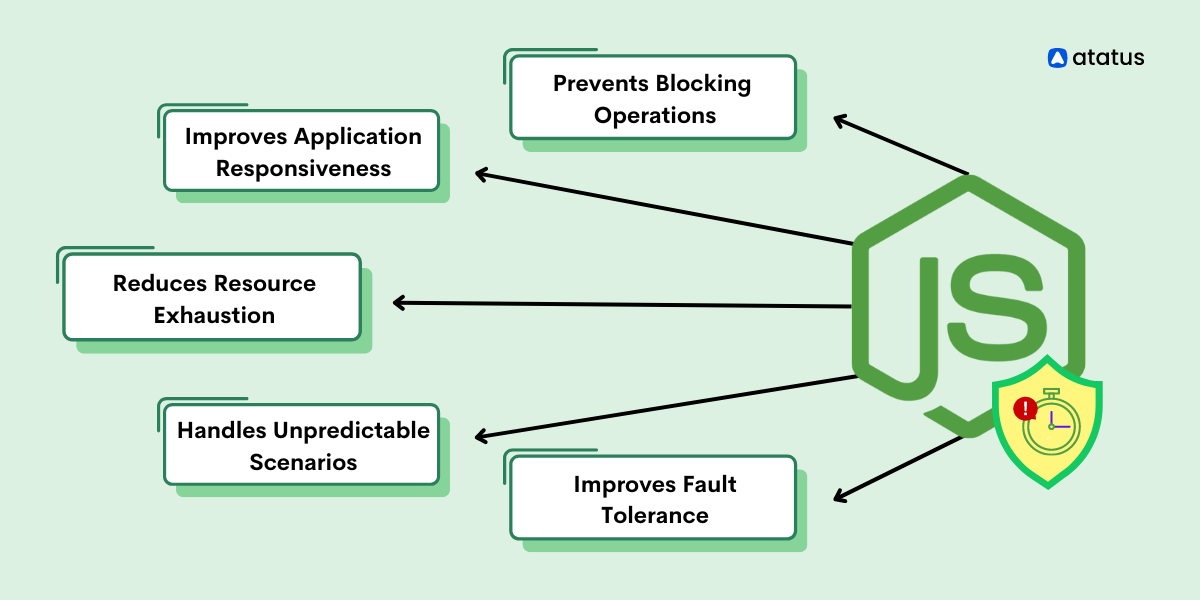
Types of timeouts
Three main types of timeouts are essential for different aspects of Node.js application development:
1. Request Timeouts
Request timeouts are used to handle situations where a server or client does not receive a response within a specified timeframe. These timeouts are necessary for securing that a system does not hang indefinitely while waiting for a response, which could lead to performance degradation or even denial of service in certain scenarios.
There are two main types of request timeouts in Node.js:
a. Incoming Request Timeouts
- Incoming request timeouts are applied to server-side operations, particularly when a Node.js server is waiting for a client to send a request.
- When a client establishes a connection to a Node.js server and sends a request, the server starts a timer. If the server does not receive the complete request within the specified timeout period, it will terminate the connection and possibly respond with an error or take other actions.
- Incoming request timeouts are handled at the HTTP server level using frameworks like Express.js or directly with the Node.js core HTTP/HTTPS modules.
b. Outgoing Request Timeouts
- Outgoing request timeouts are applied to client-side operations, particularly when a Node.js application requests an external server or service.
- When a Node.js application sends an HTTP request to an external server, it starts a timer to wait for the response. If the response does not arrive within the specified timeout period, the client-side operation is terminated, and the application can handle the timeout event accordingly (e.g., retrying the request, logging an error, etc.).
- Outgoing request timeouts can be managed using various mechanisms, such as setting timeout options in libraries like Axios or using the setTimeout function in combination with Promise-based HTTP request libraries like node-fetch.
Implementing Request Timeouts
Implementing request timeouts in Node.js, particularly in an Express.js application, is important for handling where the server takes too long to respond to incoming HTTP requests.
Several methods to implement request timeouts include using existing libraries like express-timeout-handler
, creating custom middleware, or directly using the built-in setTimeout
function in Node.js.
Using express-timeout-handler:
Install the library using npm or yarn
npm install express-timeout-handler
Combine it into your Express.js application
const express = require('express');
const timeout = require('express-timeout-handler');
const app = express();
// Set request timeout to 30 seconds
app.use(timeout.handler({ timeout: 30000 }));
// Error handler for timeouts
app.use((err, req, res, next) => {
if (err.timeout) {
res.status(503).send('Request timeout');
} else {
next(err);
}
});
// Start the server
const PORT = process.env.PORT || 3001;
app.listen(PORT, () => {
console.log(`Server running on port ${PORT}`);
});
Creating custom middleware:
Define a middleware function that sets a timeout for incoming requests
function requestTimeout(req, res, next) {
req.setTimeout(30000, () => {
res.status(503).send('Request timeout');
});
next();
}
Use this middleware in your Express.js application
const express = require('express');
const bodyParser = require('body-parser'); // Import body-parser
const app = express();
// Define your custom middleware function for request timeouts
function requestTimeout(req, res, next) {
req.setTimeout(30000, () => {
res.status(503).send('Request timeout');
});
next();
}
// Use the custom middleware for request timeouts
app.use(requestTimeout);
// Use body-parser to parse JSON request bodies
app.use(bodyParser.json());
// Define your routes...
// Start the server
const PORT = process.env.PORT || 3000;
app.listen(PORT, () => {
console.log(`Server running on port ${PORT}`);
});
Using setTimeout directly
Set a timeout for each incoming request using setTimeout
const express = require('express');
const bodyParser = require('body-parser');
const app = express();
// Middleware to set request timeout
app.use((req, res, next) => {
const timeout = setTimeout(() => {
res.status(503).send('Request timeout');
}, 30000); // Set timeout to 30 seconds
// Clear the timeout if the request is completed before the timeout
res.on('finish', () => {
clearTimeout(timeout);
});
next();
});
// Parse JSON bodies
app.use(bodyParser.json());
// Other middleware and routes
// Define your routes...
// Start the server
const PORT = process.env.PORT || 3000;
app.listen(PORT, () => {
console.log(`Server running on port ${PORT}`);
});
2. Task Timeouts
Task timeouts refer to operations or tasks within the application that have a predefined timeframe within which they should be completed. This could include asynchronous tasks such as database queries, file operations, or computational tasks.
By setting task timeouts, developers can understand that these operations do not exceed the allotted time limit, thereby preventing potential bottlenecks or resource contention within the application.
Implementing Task Timeouts
Implementing task timeouts for asynchronous operations involves setting a time limit for the completion of a task and handling scenarios where the task exceeds this time limit. This can be achieved using ‘setTimeout’ to schedule a function to run after a specified delay and ‘clearTimeout’ to cancel the scheduled function if the task completes within the allotted time.
Below are examples of implementing task timeouts for various asynchronous operations:
Database Query Timeout
const db = require('./db'); // Assuming you have a database module
function queryWithTimeout(query, timeout) {
return new Promise((resolve, reject) => {
const timer = setTimeout(() => {
reject(new Error('Query timeout'));
}, timeout);
db.query(query, (err, result) => {
clearTimeout(timer);
if (err) {
reject(err);
} else {
resolve(result);
}
});
});
}
// Example usage:
queryWithTimeout('SELECT * FROM users', 5000) // Timeout set to 5 seconds
.then(result => console.log(result))
.catch(error => console.error(error));
File Operation Timeout
const fs = require('fs');
function readFileWithTimeout(filePath, timeout) {
return new Promise((resolve, reject) => {
const timer = setTimeout(() => {
reject(new Error('File read timeout'));
}, timeout);
fs.readFile(filePath, (err, data) => {
clearTimeout(timer);
if (err) {
reject(err);
} else {
resolve(data);
}
});
});
}
// Example usage:
readFileWithTimeout('example.txt', 3000) // Timeout set to 3 seconds
.then(data => console.log(data.toString()))
.catch(error => console.error(error));
API Call Timeout
const axios = require('axios');
function fetchDataWithTimeout(url, timeout) {
return new Promise((resolve, reject) => {
const timer = setTimeout(() => {
reject(new Error('API request timeout'));
}, timeout);
axios.get(url)
.then(response => {
clearTimeout(timer);
resolve(response.data);
})
.catch(error => {
clearTimeout(timer);
reject(error);
});
});
}
// Example usage:
fetchDataWithTimeout('https://api.example.com/data', 8000) // Timeout set to 8 seconds
.then(data => console.log(data))
.catch(error => console.error(error));
3. Idle Timeouts
Idle timeouts manage inactive connections within the application, particularly in scenarios involving connections such as websockets or TCP servers. In Node.js, idle timeouts enable developers to detect and terminate idle connections that have remained inactive for a specified duration.
By applying idle timeouts, you can maximize resource utilization, prevent resource exhaustion, and improve the scalability and performance of your applications.
Implementing Idle Timeouts
The use of idle timeouts helps manage resources effectively, especially in long-lived connections such as websockets or TCP servers, where connections remain open even when not in use. Implementing idle timeouts allows servers to detect inactive connections and close them after a certain period of inactivity, thus freeing up resources and optimizing performance.
Below is an explanation of how to implement idle timeouts in various scenarios:
Websockets Idle Timeout
Websockets are consistent connections that can remain open for a long time, even when there is no activity between the client and the server. To manage idle timeouts in websockets, you can implement a method to track the last activity time and close the connection if there is no activity for a specified duration.
const WebSocket = require('ws');
const wss = new WebSocket.Server({ port: 8080 });
// Track last activity time for each websocket connection
const connections = new Map();
wss.on('connection', (ws) => {
ws.on('message', () => {
// Update last activity time when a message is received
connections.set(ws, Date.now());
});
});
// Check for idle connections and close them
setInterval(() => {
const now = Date.now();
for (const [ws, lastActivityTime] of connections.entries()) {
if (now - lastActivityTime > 60000) { // Close connection if idle for more than 1 minute
ws.terminate();
connections.delete(ws);
}
}
}, 30000); // Check every 30 seconds
In this example, the server tracks the last activity time for each websocket connection. A periodic check is performed to detect idle connections, and connections idle for more than 1 minute are terminated.
TCP Server Idle Timeout
Similarly to websockets, TCP servers can also have idle connections that need to be managed to optimize resource usage. You can implement idle timeouts by monitoring the activity on each TCP connection and closing inactive connections after a certain duration of inactivity.
const net = require('net');
const server = net.createServer((socket) => {
// Track last activity time for each TCP connection
let lastActivityTime = Date.now();
socket.on('data', () => {
// Update last activity time when data is received
lastActivityTime = Date.now();
});
// Handle socket close event
socket.on('close', () => {
console.log('Socket closed');
});
// Check for idle connections and close them
setInterval(() => {
const now = Date.now();
if (now - lastActivityTime > 60000) { // Close connection if idle for more than 1 minute
socket.end();
}
}, 30000); // Check every 30 seconds
});
server.listen(3000, () => {
console.log('TCP server listening on port 3000');
});
In this example, the TCP server tracks the last activity time for each connection. A periodic check is performed to detect idle connections, and connections idle for more than 1 minute are closed.
Implementing idle timeouts helps prevent resource wastage by closing inactive connections and freeing up resources for active connections, thereby optimizing the performance of servers handling long-lived connections. Adjust the timeout duration according to your specific use case and performance requirements.
Best Practices for Timeout Management
Here are some best practices for timeout management in Node.js:
#1 Set Appropriate Timeout Values:
- Determine timeout values based on your application's requirements and expected response times.
- Consider factors such as network latency, third-party service response times, and the complexity of operations.
- Set timeouts conservatively to avoid blocking resources for too long and make sure they are not too short to accommodate variations in workload and network conditions.
#2 Handle Timeout Errors Properly:
- Implement error handling mechanisms to handle timeout errors properly.
- Provide informative error messages to users or log timeout errors for troubleshooting purposes.
- Consider retrying the operation if appropriate or offering alternative actions to users in case of timeout errors.
#3 Monitor and Adjust Timeout Settings:
- Continuously monitor application performance and load to identify potential areas where timeouts may need adjustment.
- Use performance monitoring tools to track response times, error rates, and timeouts.
- Adjust timeout settings dynamically based on real-time data to optimize performance and deliver timely responses.
- Conduct load testing to simulate high-traffic conditions and validate timeout settings under different scenarios.
#4 Use Libraries and Frameworks with Built-in Timeout Support:
- Make use of libraries and frameworks that provide built-in support for timeout management.
- For example, Express.js middleware like
express-timeout-handler
can handle request timeouts, reducing the need for manual timeout management. - Choose third-party modules or libraries that are actively maintained and well-documented to increase reliability and compatibility with your application.
#5 Implement Circuit Breaker Patterns:
- Consider carefully implementing circuit breaker patterns to handle timeouts and failures, especially in distributed systems.
- Circuit breakers can detect and prevent repeated timeouts by temporarily stopping requests to a failing service and redirecting traffic to alternative services or cached data.
- Implement circuit breaker libraries like
hystrix
orbrakes
to manage timeouts and failures effectively in Node.js applications.
#6 Use Promises and Asynchronous APIs:
- Take advantage of Node.js's asynchronous nature and use Promises or asynchronous APIs to manage timeouts efficiently.
- Promises allow you to set timeouts for asynchronous operations using
Promise.race()
to race between the promise resolution and a timeout promise. - Use asynchronous APIs for I/O operations, network requests, and other potentially long-running tasks to avoid blocking the event loop and improve scalability.
Handling Timeout Errors
Here are some key points for identifying, debugging, and resolving timeout errors:
#1 Identifying Timeout Errors:
- Timeout errors typically occur when an operation takes longer than the specified timeout duration to complete.
- Common scenarios leading to timeout errors include slow network connections, unresponsive third-party services, or blocking I/O operations.
- Look for error messages indicating timeouts, such as
ETIMEDOUT
,ECONNRESET
, or custom error messages specific to your application or libraries.
#2 Logging Timeout Errors:
- Implement logging methods to capture timeout errors for debugging and monitoring purposes.
- Use logging libraries like
winston
or built-in logging features in Node.js to log timeout errors along with relevant contextual information such as timestamps, request parameters, and stack traces. - Log timeout errors at appropriate levels (e.g., error or warning) to confirm that they are easily identifiable and actionable.
#3 Strategies for Troubleshooting Timeout Issues:
- Review application logs to identify patterns or trends associated with timeout errors, such as specific endpoints or operations consistently experiencing timeouts.
- Monitor system resources (CPU, memory, disk I/O) to identify potential bottlenecks causing timeouts, such as high CPU usage or insufficient memory.
- Use profiling tools like Node.js's built-in
--inspect
flag or external tools likenode-clinic
to analyze application performance and identify areas of improvement. - Review network logs to identify network-related issues contributing to timeouts, such as network latency or packet loss.
- Evaluate third-party services or dependencies for reliability and performance issues that may cause timeouts in your application.
#4 Resolving Timeout Issues:
- Adjust timeout settings based on the analysis of application performance and workload characteristics.
- Maximize application code to improve performance and reduce the chance of timeouts, such as optimizing database queries, implementing caching methods, or using asynchronous programming patterns.
- Implement retry methods for important operations that may experience occasional timeouts, providing flexibility against transient failures.
- Consider scaling application resources horizontally or vertically to handle increased load and reduce the chance of timeouts during peak usage periods.
- Communicate with third-party service providers to address performance or reliability issues impacting your application's interactions with their services.
- Continuously monitor and iterate on timeout management strategies based on ongoing performance metrics and user feedback.
Closing Remarks
In conclusion, timeouts are necessary for Node.js applications to manage various aspects of asynchronous operations, network interactions, and resource utilization.
Recap of key concepts related to timeouts in Node.js:
Proper timeout management involves setting appropriate timeout values based on application requirements, handling timeout errors, monitoring and adjusting timeout settings based on performance metrics, and applying strategies for troubleshooting and resolving timeout issues. Timeouts are commonly used in scenarios such as handling HTTP requests, asynchronous operations, database queries, file operations, API calls, websockets, and TCP connections.
Incorporating timeouts effectively in Node.js development workflows is important for building robust and reliable applications. It helps prevent resource exhaustion, improves application responsiveness, and improves overall user experience. By carefully implementing and managing timeouts, you can make sure that their Node.js applications are flexible to various failure scenarios, provide timely responses to users, and maintain optimal performance under different workload conditions.
Finally, proper timeout management is required for building robust and reliable Node.js applications. By understanding the importance of timeouts, implementing best practices for timeout management, and continuously monitoring and adjusting timeout settings, you can be sure that your applications are resilient, responsive, and performant in various usage scenarios.
Monitor Your Node.js Applications with Atatus
Atatus keeps track of your Node.js application to give you a complete picture of your clients' end-user experience. You can determine the source of delayed response times, database queries, and other issues by identifying backend performance bottlenecks for each API request.
Node.js performance monitoring made bug fixing easier, every Node.js error is captured with a full stack trace and the specific line of source code marked. To assist you in resolving the Node.js error, look at the user activities, console logs, and all Node.js requests that occurred at the moment. Error and exception alerts can be sent by email, Slack, PagerDuty, or webhooks.
#1 Solution for Logs, Traces & Metrics
APM
Kubernetes
Logs
Synthetics
RUM
Serverless
Security
More