Parsing PHP data with JSON encode and decode functions
PHP is a powerful backend developer language. In fact, it is the most used server-side scripting language today.
There is a lot of coding that goes behind building a successful website. And all these work on data. The I/O commands written on a disk forms the data, and the resource material you’ve already embedded becomes data. Data can be written in any format - as a string, object, or array.
It may not be much to see on your final application page, but the code that goes behind making your website pretty is humongous. And all this adds up to the data you feed onto your servers. Their format becomes important here. My conviction is based on its serialization procedures.
In server-side programming, data can be exchanged and stored in several formats, including:
- JSON (JavaScript Object Notation) - A lightweight, text-based data format that is easy to read and write for humans and machines.
- XML (eXtensible Markup Language) - A markup language that is used to store and transport data, often over the internet.
- CSV (Comma Separated Values) - A simple text format used to store tabular data, where each line represents a row and each field is separated by a comma.
- YAML (Yet Another Markup Language) - A human-readable data serialization format that is often used for configuration files and data storage.
- Protocol Buffers - A high-performance, compact binary format used for serializing structured data.
- Binary formats - Used to store data in a compact and efficient format, often used in high-performance applications and to store images, audio, and video files.
The choice of data format depends on the use case, performance requirements, and the tools and libraries available in the programming language being used.
Let's look at the following:
What is JSON?
JSON (JavaScript Object Notation) is a lightweight data-interchange format that uses human-readable text to transmit data objects consisting of attribute–value pairs. It primarily transmits data between a server and a web application.
Mostly, it works as an alternative to XML.
PHP 5.2.0 supports JSON by default. In other words, when you install PHP, JSON support is automatically installed, and no additional installation or configuration is necessary.
JSON structure consists of key-value pairs and has the following syntax:
{
"key1": "value1",
"key2": "value2",
...
}
Where the keys are strings, and the values can be strings, numbers, objects (another nested JSON), arrays, boolean values (true/false), or null.
Note:
Strings - A String is a sequence of characters. Generally, a string is an immutable object, meaning the string's value can not be changed.
Array - The Array is a collection of similar data type elements. It uses a contiguous memory location to store the elements.
Object - Objects represent a special data type that is mutable and can be used to store a collection of data (rather than just a single value).
JSON encode and decode Functions
This entire article is devoted to helping you understand the manner in which you can convert data sets into JSON format for ease of readability and working. And the two functions that will help us do so are discussed below.
JSON encode() Function
The json_encode() function is a built-in function in PHP that converts a PHP value to a JSON-formatted string. The function takes a single argument, which can be any type of PHP value (e.g., array, object, string, number, boolean), and returns a JSON-encoded string representation of that value. The returned string can be used to transmit the data over a network or store the data in a file for later use.
Example:
$array = array("name" => "John Doe", "age" => 35, "city" => "New York");
$json = json_encode($array);
In this example, we are defining a name, age, and city for the value set of an array key. Followed by including this key as a value in the json_encode function. And running this gives us the below output:
// Output: {"name":"John Doe","age":35,"city":"New York"}
The json_encode() function is commonly used in web development to:
- Transfer data between a client-side JavaScript application and a server-side PHP application using AJAX (Asynchronous JavaScript and XML).
- Store data on a client-side as a JSON encoded string in a cookie or local storage.
- Share data between different applications in a standardized format.
- Serialize data for storage in a NoSQL database like MongoDB.
- Provide an API (Application Programming Interface) for third-party developers to access data from your application.
- Serialize complex data structures, such as arrays and objects, into a compact string representation that can be easily transported over a network.
JSON decode Function
The next function of importance to us is json_decode. The json_decode() function is a built-in function in PHP that converts a JSON-formatted string into a PHP value (e.g. array, object, string, number, boolean). The function takes a single argument, which is the JSON-encoded string, and returns a PHP value that can be used in PHP scripts.
By default, json_decode() converts JSON objects into associative arrays. However, you can also ask json_decode() to return the JSON data as an object by setting the second argument to true.
Example:
$json = '{"name":"John Doe","age":35,"city":"New York"}';
$array = json_decode($json);
Output:
Array ( [name] => John Doe [age] => 35 [city] => New York )
Here are some common uses of the json_decode() function:
- Processing JSON data received from a REST API or an AJAX request.
- Converting JSON data stored in a cookie or local storage into a PHP value.
- Decoding JSON data received as part of a message queue or in an event-driven architecture.
- Reading JSON configuration files and converting the data into usable PHP values.
- Deserializing complex data structures, such as arrays and objects, that were previously serialized into JSON format.
- Converting JSON data received from a third-party service into usable PHP values.
Encoding JSON Data in PHP
We are going to take a PHP value( string. Object, and array) and convert them to easily readable and executable JSON formats.
1. PHP String to JSON
A string is the easiest value that you can process in PHP. You use the same string as the value key while performing encode() function here. This would return the exact string as output.
$phpString = '{"name": "John", "age": 30, "city": "New York"}';
$json = json_encode($phpString);
Output:
{ "name":John, "age":30, "city":NewYork }
2. PHP Object to JSON
An object contains a number of data fields, like a record, and also offers a number of subroutines for accessing or modifying them, called methods. Most of your work would have to do with PHP objects rather than Strings or arrays.
$phpObject = new stdClass;
$phpObject->name = "John";
$phpObject->age = 30;
$phpObject->city = "New York";
$json = json_encode($phpObject);
Output:
3. PHP Array to JSON
$phpArray = array("name" => "John", "age" => 30, "city" => "New York");
$json = json_encode($phpArray);
The output of the above code would be a JSON-encoded string representation of the PHP array:
{"name":"John","age":30,"city":"New York"}
Decoding JSON Data in PHP
The json_decode() function is a built-in PHP function that is used to convert a JSON-encoded string into a PHP value (either an object, an associative array, or a scalar value).
$json = '{"name":"John","age":30,"city":"New York"}';
$phpArray = json_decode($json, true);
The second argument of json_decode() is a Boolean value that determines the type of output. If it is true, the function will return an associative array. If it is false, it will return an object.
If NULL is passed as the second argument, the function will return an object if the JSON string represents an object and an array if it represents an array.
Conclusion
If you look at the statistics, JSON is one of the most used data formats for exchanging information on the internet. And this necessitates a premium language like PHP to be able to parse data in JSON.
The problem with XML data type is that it is verbose, large, and expressing node relationship is difficult; this creates redundancy and will slow down your applications’ processing powers. This problem is sorted with the lightweight text-based data transfer format - JSON.
The above tutorial will help you understand how to parse any data in PHP to JSON and vice-versa using its encode and decode functions. For those of you who are new to PHP and JSON, these articles can help you get started -
Check your PHP compatibility and Performance
Atatus: PHP Performance Monitoring and Log Management
Atatus is an Application Performance Management (APM) solution that collects all requests to your PHP applications without requiring you to change your source code. However, the tool does more than just keep track of your application's performance.
Monitor logs from all of your PHP applications and systems into a centralized and easy-to-navigate user interface, allowing you to troubleshoot faster using PHP monitoring.
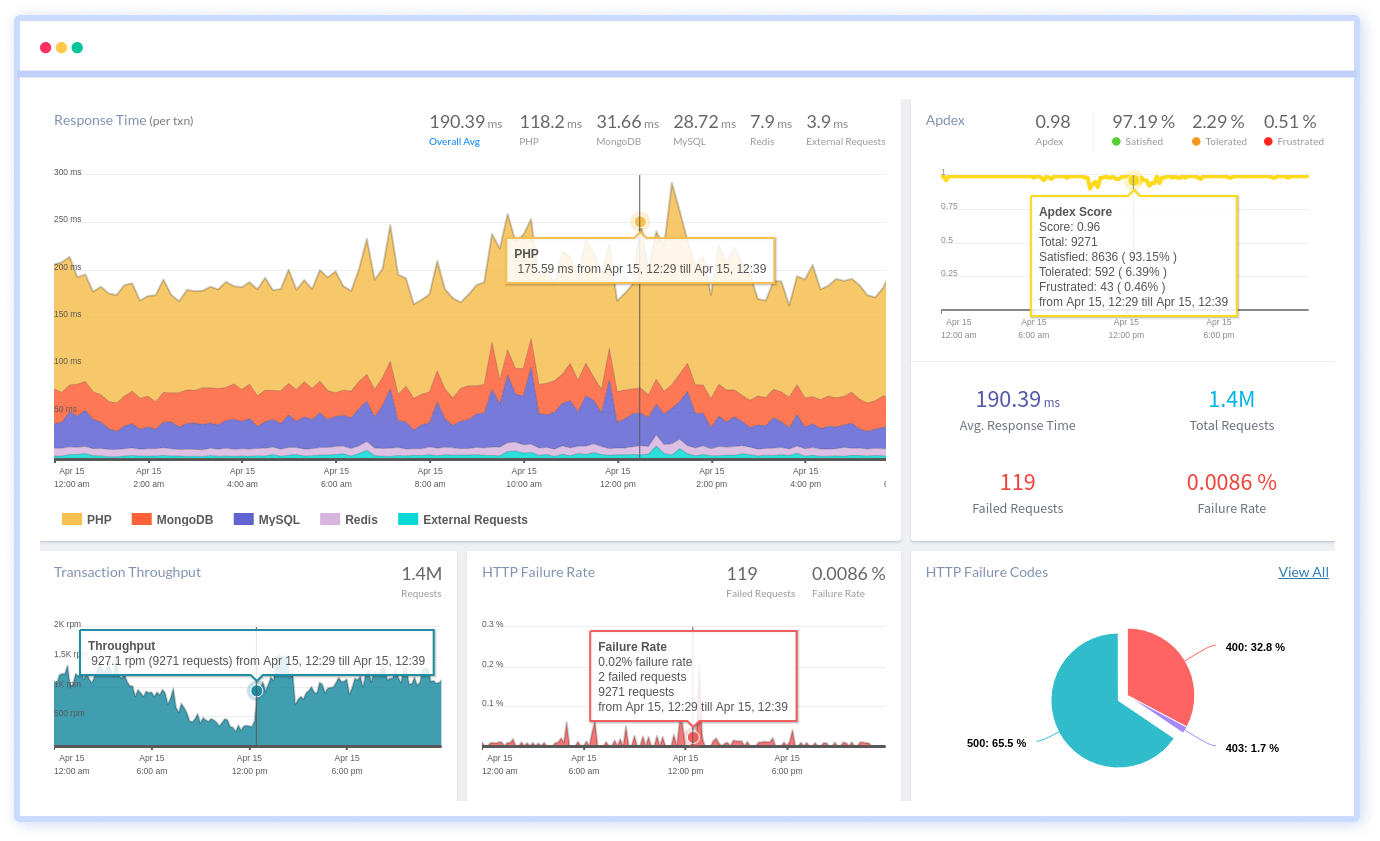
We give a cost-effective, scalable method to centralized PHP logging, so you can obtain total insight across your complex architecture. To cut through the noise and focus on the key events that matter, you can search the logs by hostname, service, source, messages, and more. When you can correlate log events with APM slow traces and errors, troubleshooting becomes easy.
#1 Solution for Logs, Traces & Metrics
APM
Kubernetes
Logs
Synthetics
RUM
Serverless
Security
More