Python: Converting Lists to Dictionaries
The list and dictionary are the data structures that captivates data. In Dictionary, data is stored as key-value pairs, while in List, heterogeneous data types are stored. Python lists are converted into dictionaries here.
As lists are ordered, and dictionaries are unordered, results can differ based on the order of inputs. Python collections encompass objects like lists and dictionaries. They enable you to hold numerous values equivalent to a specific data structure.
Lists enable the storage of values in an ordered manner, whereas dictionaries offer a means of storing data with particular keys that match the values they comprise.
Python is a powerful programming language with several built-in data structures, including lists and dictionaries. In this article, let's dive deep into Python and see how to convert a list to a dictionary python.
Table Of Contents
Python Lists and Dictionaries
Python List is simply an ordered collection of elements, which can be of any data type, such as integers, strings, or even other lists. Lists are defined using square brackets []. Here's an example of a Python List that contains three integers:
my_list = [1, 2, 3]
You can also create an empty list using the following syntax:
my_list = []
In Python, a Dictionary is a built-in data structure that stores key-value pairs. Dictionaries are sometimes called associative python arrays or hash tables in other programming languages.
Python dictionary is created using curly braces {} or the built-in dict()
function. Here is an example of creating a dictionary:
my_dict = {'apple': 1, 'banana': 2, 'orange': 3}
In this example, my_dict is a dictionary with three key-value pairs. The keys are 'apple', 'banana', and 'orange', and the corresponding values are 1, 2, and 3, respectively.
my_dict = {}
Python conversion from List to Dictionary
Converting a list to a dictionary python is a common task in Python programming. In simple words, the dictionary is just a data structure that upholds a collection of key-value pairs, while a list is a collection of elements.
1. Using a for loop method
One way to convert a list to a dictionary python is to use a for loop to iterate through the list and create dictionary from list python and from its elements.
my_list = ['a', 'b', 'c']
my_dict = {}
for index, element in enumerate(my_list):
my_dict[index] = element
print(my_dict)
In the above code, we create an empty dictionary my_dict
, then loop through the list my_list
Applying the enumerate()
function. The enumerate()
function returns a tuple incorporating the value and index of every individual element in the list.
We use the index as the key and the value as the value in the dictionary. The output of the above code will be:
{0: 'a', 1: 'b', 2: 'c'}
2. Using the map and dict method
Convert list into dictionary python, by implying array slicing, the first step is to create an python array with keys and values. By using the map method, key-value pairs are created as tuples. To make it suitable for dictation, it should be typecast.
The output of the above code will be:
# Python3 program to Convert a
# list to dictionary
def Convert(lst):
res_dct = map(lambda i: (lst[i], lst[i+1]), range(len(lst)-1)[::2])
return dict(res_dct)
# Driver code
lst = ['a', 1, 'b', 2, 'c', 3]
print(Convert(lst))
3. Using the zip() method
The alternative method to convert a list into a dictionary is to use the zip() function, which takes two or more iterables and returns a tuple of their corresponding elements.
We can use the zip()
function to create a list of tuples, where each tuple comprises an index and a value from the original list, and then convert this list of tuples to a dictionary.
my_list = ['a', 'b', 'c']
my_dict = dict(zip(range(len(my_list)), my_list))
print(my_dict)
In the above code, we use the range()
function to generate a sequence of integers from 0 to the length of the list my_list
and then use the zip()
function to create a list of tuples that incorporates both value and index of every individual element in the list.
Finally, we convert this list of tuples to a dictionary using the dict()
function, make dict from list python. The output of the above code will be:
{0: 'a', 1: 'b', 2: 'c'}
4. Using dictionary comprehension method
We can also use a dictionary comprehension to convert a list to a dictionary in one line of code.
my_list = ['a', 'b', 'c']
my_dict = {index: element for index, element in enumerate(my_list)}
print(my_dict)
In the above code, we use a dictionary cognition to generate a dictionary from the list my_list
. The output of the above code will be:
{0: 'a', 1: 'b', 2: 'c'}
5. Using a list of keys method
Converting a list to a dictionary can be done by using the list of key method. Create two lists, one for keys and one for values. Zip the two lists together using the zip()
function to create a list of key-value pairs.
Convert the list of key-value pairs to a dictionary using the dict()
function.
Here's an instance code snippet that exhibits how to convert a list to a dictionary, implying the list of key method:
keys = ['apple', 'banana', 'orange']
values = [3, 6, 4]
# zip the two lists together to create a list of key-value pairs
key_value_pairs = zip(keys, values)
# convert the list of key-value pairs to a dictionary
my_dict = dict(key_value_pairs)
print(my_dict)
# Output: {'apple': 3, 'banana': 6, 'orange': 4}
In the instance above, the zip()
function is used to combine the keys and values lists into a list of key-value pairs. The output list of key-value pairs is then passed to the dict()
function to create a dictionary. Finally, the resulting dictionary is printed to the console.
6. Using dict.fromkeys() method
Convert list into dictionary python by dictionary keys that are converted to dictionary values, which are assigned in the process of dict.fromkeys()
. All keys will be assigned the same value.
l1=['red','blue','orange']
d1=dict.fromkeys(l1,"colors")
print (d1)
#Output:{'red': 'colors', 'blue': 'colors', 'orange': 'colors'}
7. Using Counter() method
A counter subclass of dict is designed to count hashable objects. A dictionary collection contains elements linked by dictionary keys, and dictionary values link their counts.
collections.Counter(iterable-or-mapping)
The Python documentation states that “counts can be any integer value, including zeros and negatives.” List items will be converted to keys, and their frequencies will be converted to values using counter()
.
from collections import Counter
c1=Counter(['c','b','a','b','c','a','b'])
#key are elements and corresponding values are their frequencies
print (c1)#Output:Counter({'b': 3, 'c': 2, 'a': 2})
print (dict(c1))#Output:{'c': 2, 'b': 3, 'a': 2}
The Final Shot
Develop a Python program to create dictionary from list python, with all the odd elements having keys and all the even elements having values. It is possible to iterate through the list using the zip function and to have the output in any order.
Among the many data structures used to store data, lists, and dictionaries are the most commonly used. Key-value pairs are stored in the dictionary, whereas various data types are stored in the list.
Dictionaries and Lists differ in how they store data. If you retrieve data from a dictionary, the order in which it was stored may differ from the order in which you stored it.
Convert list into dictionary python, implying the dict.fromkeys()
method, hence creating a dictionary from a list. A Python zip()
function can be used to create a dictionary from two lists. Based on a list of values, you can create a new dictionary or convert list to dictionary python by applying the dictionary comprehension.
Atatus: Python Performance Monitoring
Atatus is an Application Performance Management (APM) solution that collects all requests to your Python applications without requiring you to change your source code. However, the tool does more than just keep track of your application's performance.
Monitor logs from all of your Python applications and systems into a centralized and easy-to-navigate user interface, allowing you to troubleshoot faster using Python monitoring.
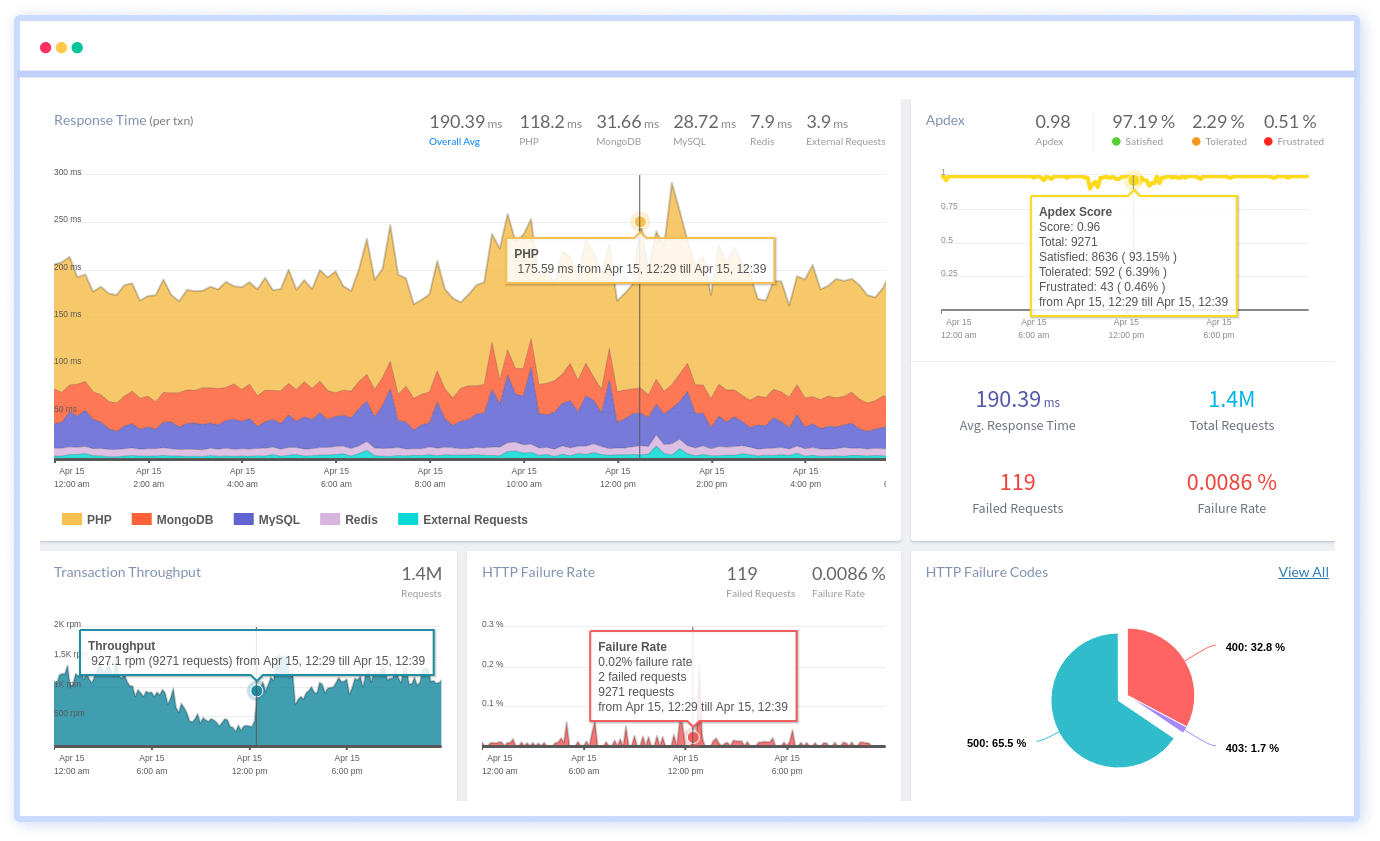
We give a cost-effective, scalable method to centralized Python logging, so you can obtain total insight across your complex architecture. To cut through the noise and focus on the key events that matter, you can search the logs by hostname, service, source, messages, and more. When you can correlate log events with APM slow traces and errors, troubleshooting becomes easy.
#1 Solution for Logs, Traces & Metrics
APM
Kubernetes
Logs
Synthetics
RUM
Serverless
Security
More