An in-depth overview of Java Ternary Operators
Java is a widely used programming language that has several unique features that make coding efficient and organized.
One such feature is the Java ternary operator. This operator is a shorthand way of writing an if-else statement in Java, making code more concise and readable. With the increasing demand for speedy coding and reduced lines of code, the Java ternary operator is gradually becoming a popular choice among developers.
In this blog post, we'll explore the basics of Java ternary operators, including their syntax, how they work, and when to use them.
We'll also cover some best practices for using ternary operators in your code and discuss common mistakes to avoid. Whether you're new to programming or an experienced developer, this guide will help you become a master of Java ternary operators.
Table Of Contents
- Java Ternary Operator
- Aspects of the Java ternary operator
- Examples of the Java ternary operator
- Usage of the Java ternary operator
- Advantages of Ternary Operator
Java Ternary Operator
The Java ternary operator is a shorthand way of writing an if-else statement that evaluates to a single value. It is also called the conditional operator because it uses a condition to determine which value to return.
The syntax of the ternary operator is:
condition ? value_if_true : value_if_false
Here, the condition is an expression that evaluates to a boolean value; value_if_true
is the value to retrieve if the condition is true, as well as value_if_false
, is the value to retrieve if the condition is false.
For example, the following code uses the ternary operator to verify if a number is positive or negative:
int number = -5;
String result = (number >= 0) ? "positive" : "negative";
System.out.println(result);
In this example, the condition (number >= 0)
is evaluated. If it is true, the value "positive" is returned; otherwise, the value "negative" is returned. The result is then assigned to the variable result and printed to the console.
Aspects of the Java ternary operator
In general, the Java ternary operator is the only one that takes three operands. Java programmers often use it as a one-line replacement for if-then-else statements.
Some of the aspects of the Java ternary operators are listed below:
- Java ternary operator can be used to assign a value to a variable based on a condition.
- The ternary operators in Java can be nested to evaluate multiple conditions.
- Java ternary operator can be used instead of an
if-else
statement when a simple decision needs to be made. However, it can make code harder to read and understand if it is used excessively or in complex situations. - The expressions
value_if_true
andvalue_if_false
can have different data types as long as they are compatible. The operands' data type determines the entire expression's data type. - The ternary operator in Java has got more elevated precedence than the = operator, so it is essential to use parentheses when assigning the result to a variable.
- The ternary operator in Java is right-associative, which evaluates from right to left.
- The ternary operator in Java can be nested to handle more complex conditional statements, but this can make code harder to read and maintain.
Java ternary operator Examples
Here are some examples of the ternary operator in Java:
1. Assigning a value based on a condition:
int age = 18;
String message = (age >= 18) ? "You are an adult" : "You are a minor";
System.out.println(message); // "You are an adult"
2. Returning a value based on a condition:
int x = 5;
int y = 10;
int max = (x > y) ? x : y;
System.out.println(max); // 10
3. Nesting ternary operators:
int a = 5;
int b = 10;
int c = (a > b) ? 1 : ((a < b) ? -1 : 0);
System.out.println(c); // -1
4. Assigning a value to a boolean:
int score = 80;
boolean isPassing = (score >= 60) ? true : false;
System.out.println(isPassing); // true
5. Check if a number is even or odd:
int num = 7;
String parity = (num % 2 == 0) ? "even" : "odd";
System.out.println(parity); // Output: odd
Usage of Java ternary operator
The Java ternary operator is often used to impute a value to a variable depending on a condition like this:
int x = 5;
int y = (x > 3) ? 10 : 20; // if x is greater than 3, y will be assigned 10; otherwise, y will be assigned 20
In this example, condition x > 3
is evaluated first. If it is true, then the expression 10 is returned and assigned to y. Otherwise, the expression 20 is returned and assigned to y.
The Java ternary operator can also be implied in more complex expressions, such as:
int a = 5;
int b = 10;
int c = (a > 3 && b < 5) ? 15 : 20; // if both conditions are true, c will be assigned 15; otherwise, c will be assigned 20
Here, the condition (a > 3 && b < 5)
evaluates to false since b is not less than 5. Therefore, the expression 20 is returned and assigned to c.
It is generally known that the Java ternary operator is useful for creating more concise code when a simple if-else
statement is required. In spite of this, ternary expressions should be used sparingly since overly complex ternary expressions can be challenging to understand.
The ternary operator in Java is a shorthand way to write an if-else
statement. The syntax of the Java ternary operator is as follows:
variable = (condition) ? value1 : value2;
Here, variable is the variable you want to assign a value to, condition is the condition that you want to evaluate, value1
is the value to be assigned to the variable if condition is true, and value2
is the value to be assigned to the variable if condition is false.
For example, suppose you have two variables, x and y, and you want to assign the larger value to a variable called max. You can use the ternary operator to do this as follows:
int x = 5;
int y = 10;
int max = (x > y) ? x : y;
In this example, x > y
is the condition that is evaluated. If this condition is true, the value of x is assigned to the max. Otherwise, the value of y is assigned to the max. Since y is more significant than x, the value of the max will be 10.
Advantages of Ternary Operator
Here are some advantages of using the ternary operator in your code:
- Concise Code: The ternary operator allows you to write conditional statements concisely, making your code easier to read and understand. It can be beneficial when working with complex conditional statements.
- Faster Execution: The ternary operator can be faster than if-else statements because it is a single line of code. This can result in better performance if the code is executed many times.
- Avoid Repetition: The ternary operator can help you avoid repeating code in if-else statements. This can make your code more maintainable and reduce the chances of introducing bugs.
- More Readable Code: The ternary operator can make your code more readable by reducing the nesting in if-else statements. This can make it easier to understand the logic of your code.
Here are some scenarios where you might consider using the ternary operator:
- Simple Conditionals: The ternary operator is great for simple conditional statements. For example, you can use it to assign a value to a variable based on a simple condition.
- Inline Conditionals: The ternary operator is great for inline conditionals. For example, you can use it to determine the value of a variable used in a more prominent expression.
- Non-Complex Conditionals: The ternary operator is best used for non-complex conditionals. It may be better to use if-else statements for complex conditionals to make the code easier to understand.
Conclusion
A number of operators on the compiler are used to instruct it to perform a specific operation. The Ternary Operator is also an operator available in Java, just like Arithmetic, Bitwise, Unary, and others.
Using the ternary operator in Java improves the readability and quality of code by shorthand conditional statements. Its name is derived from three expressions that make up the ternary operator in Java.
As a simplified Java if statement, the Java ternary operator works similarly. If the condition is true, a value is returned; if it is false, the value is returned. Java Ternary operators involve a condition that can be either true or false and a value that returns if it is true or false.
Java Ternary operators permit you to control the flow of your code more efficiently with if statements in Java. These operators have three operands, which is why they are referred to as ternary operators.
Monitor your Java application for errors & exceptions with Atatus
With Atatus Java performance monitoring, you can monitor the performance and availability of your Java application in real-time and receive alerts when issues arise. This allows you to quickly identify and troubleshoot problems, ensuring that your application is always running smoothly.
One of the key features of Atatus is its ability to monitor the performance of your Java application down to the individual request level. This allows you to see exactly how long each request is taking to process, as well as any errors or exceptions that may have occurred. You can also see a breakdown of the different components of your application, such as the web server, database, and external services, to see how they are affecting performance.
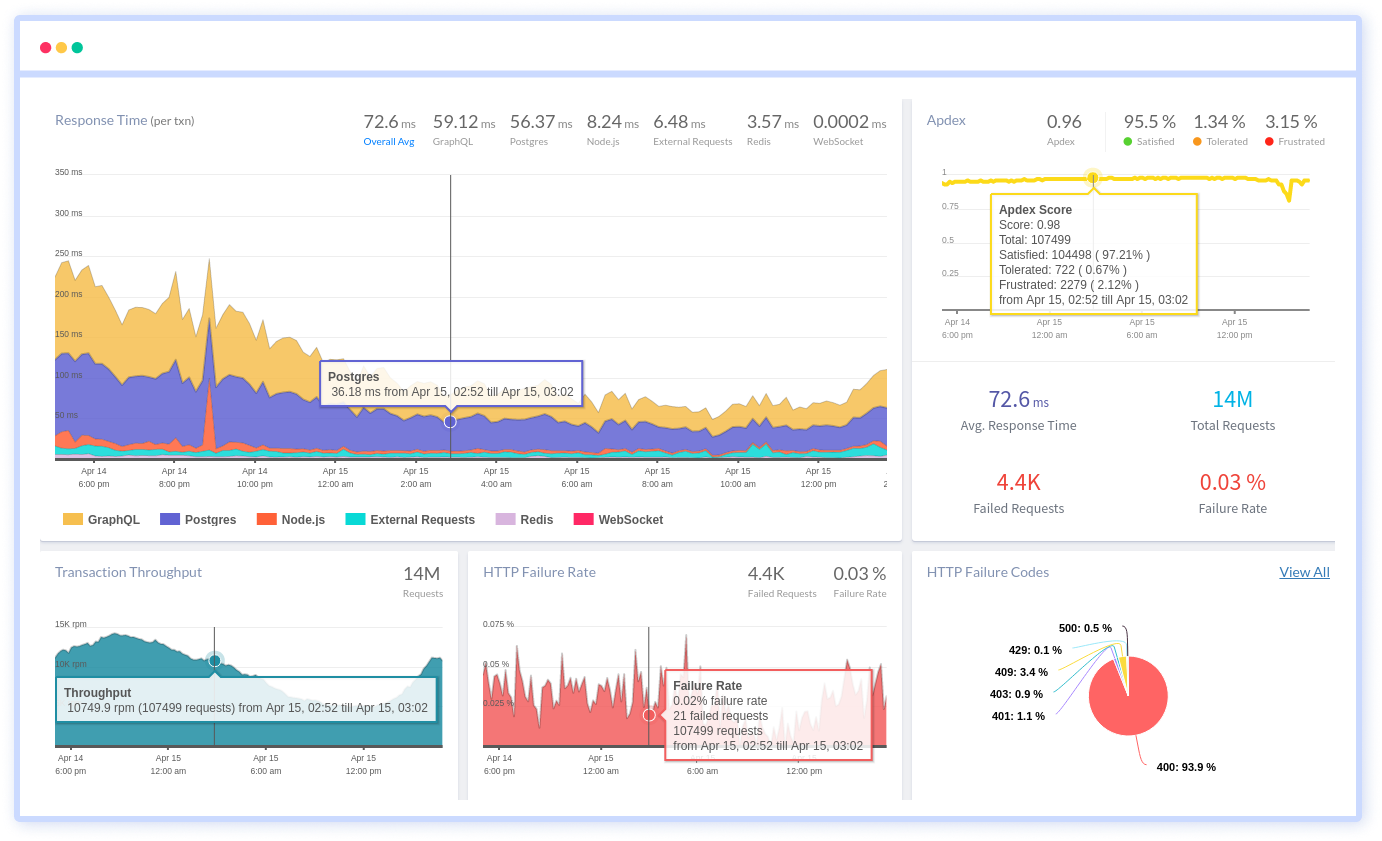
In addition to monitoring performance, Atatus also allows you to track the availability of your Java application. This means that you can see when your application is down or experiencing errors, and receive alerts when this occurs. You can also see how long your application has been running, as well as any uptime or downtime trends over time.
Atatus also offers a range of tools and integrations that can help you to get the most out of your monitoring. For example, you can integrate Atatus with popular tools like Slack, PagerDuty, and Datadog to receive alerts and notifications in your preferred channels. You can also use Atatus's APIs and SDKs to customize your monitoring and build custom integrations with your own tools and systems.
Overall, Atatus is a powerful solution for monitoring and managing the performance and availability of your Java application. By using Atatus, you can ensure that your application is always running smoothly and that any issues are quickly identified and resolved.
If you are not yet a Atatus customer, you can sign up for a 14-day free trial .
#1 Solution for Logs, Traces & Metrics
APM
Kubernetes
Logs
Synthetics
RUM
Serverless
Security
More