What is Git Checkout Remote Branch? Benefits, Best Practices & More
Git is a terrific tool that many developers use to keep track of their projects’ versions. Despite the fact that there are many different version control systems, git is by far the most used. The focus on distributed development and the ease with which branches can be used for good reasons.
We will cover the following:
- What is Branch?
- What is a Remote Branch?
- What is Git Checkout Remote Branch?
- How to Git Checkout Remote Branch?
- Different Options In Git Checkout
- Benefits of Git Checkout Remote Branch
- Best Practices for Git Checkout Remote Branch
What is Branch?
A branch is a simple approach of departing from the main development flow. It's typically used in a branch to add a new feature or correct an issue. You'll be able to encapsulate the modifications and maintain your master or main branch tidy this way.
Branches in git are extremely valuable because they are simple and inexpensive to establish. Unlike other version control systems, git's branch is simply a pointer to the original node where the branch began, rather than a copy of your code.
When using git, you'll be working in a master environment. This branch contains the source code that will be deployed when your application is ready for use.
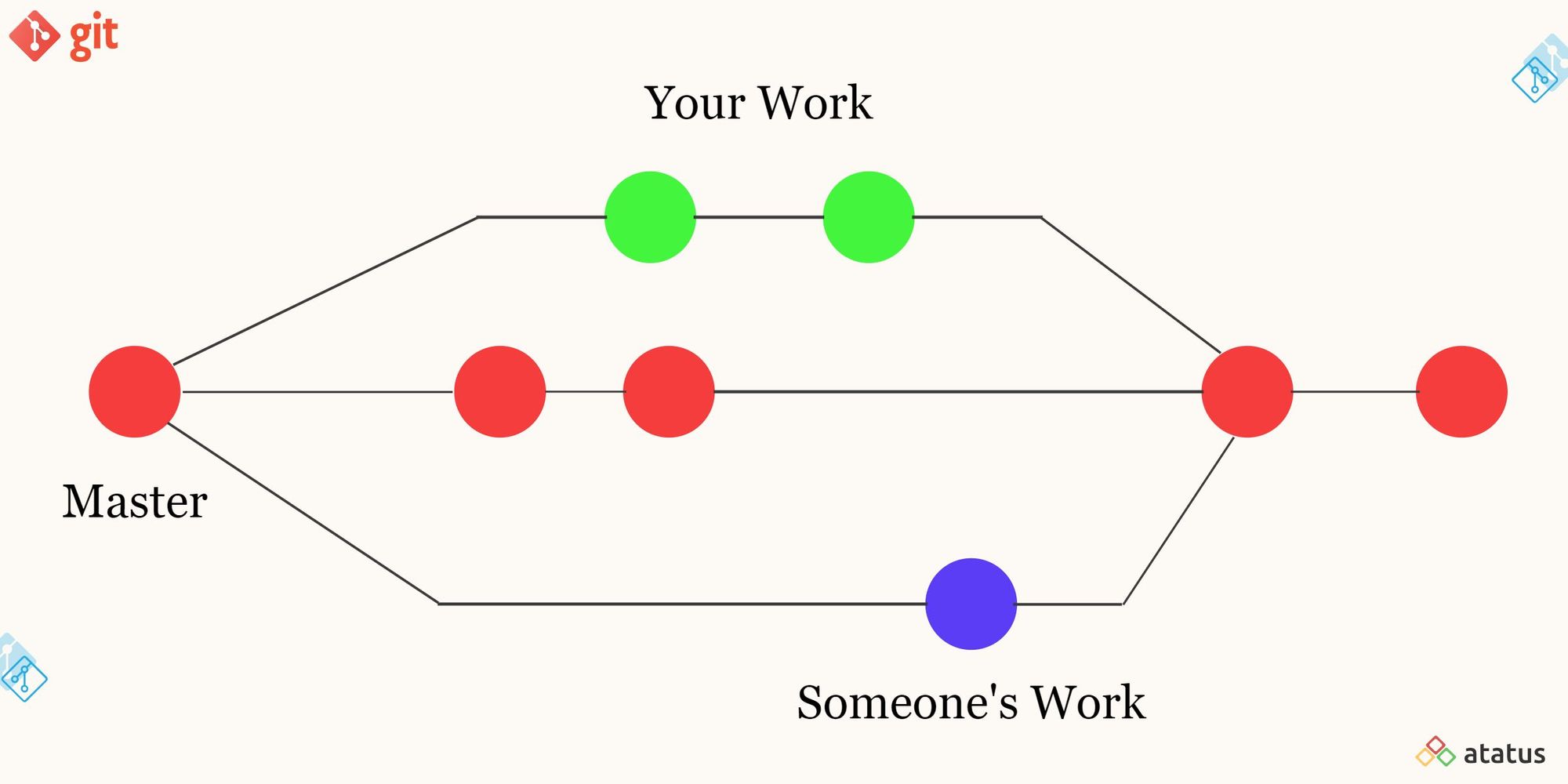
You can also add other changes to this branch when you wish to update your application. This may not be a big concern for minor adjustments, but it is not ideal for major changes. That is why there are additional branches.
What is a Remote Branch?
A remote branch is a branch that exists in a remote git repository. Once configured, your local Git repository will seek for external commit changes in these remote repositories, which are referred to as "remotes".
The Git branch command can be used to see which branches are available. The branch with a star (*) is the one that is currently active.
How to Checkout a Remote Branch?
The ideal option to share your development work with other members of your team is to use a remote branch. You must first use git fetch to check out a remote branch that someone has published. This command downloads the references from your remote repository, including the reference to the remote branch, to your local system.
All you have to do now is execute the command
git checkout <remote_branch_name>
What is Git Checkout Remote Branch?
A programmer can utilize the Git checkout remote branch to examine and collaborate on the work of a colleague or collaborator. The command “git checkout remote branch” does not exist. It's merely a slang term for checking out a remote branch.
Git is a software development tool that allows programmers to track changes to their code. It keeps track of all the different versions in a single database. Git enables numerous developers to collaborate on the same code at the same time. A programmer may need to access a colleague's independent work, or "branch," on occasion. This is made feasible via the git checkout remote branch command.
Here some of the git commands that developers use often:
Git Branch Command
The git branch command is used to create, list, and delete branches in Git. It does not allow you to swap between branches and reconstruct a branched history. Branching is supported by the majority of version control systems. Its purpose is to provide a snapshot of your changes. A new branch is formed for summarising the modifications if you intend to fix bugs or add new properties.
Git Fetch Command
The git fetch command is used to fetch commits, references, and files from a remote repository and save them to a local repository. You can use it to check what other members of the group have been up to. The git checkout command should be used to precisely check out the content that has been fetched.
Git Checkout Command
The git checkout command is used to switch branches and restore working tree files. It may be used on commits, branches, and files. It comes in handy when moving between multiple features in a single repository.
Git Reset Command
A git reset command is a handy tool for reverting Git changes. It has three invocation forms that correspond to Git's internal state management mechanisms. Three Git trees are the names given to these systems. The HEAD, your staging index, and, lastly, the working directory are all included.
How to Git Checkout Remote Branch?
Let's imagine you wish to pull a remote branch that has been produced by another developer. The following is how you go do it:
#1 Fetch All Remote Branch
git fetch origin
This retrieves all of the repository's remote branches. The distant name you're aiming for is called the origin. You can use git fetch upstream if you have an upstream remote name.
#2 List the Branches Available for Checkout
git branch -a
This command returns a list of branches that can be available for checkout. You'll see that the remote branches are prefixed with remotes/origin.
#3 Pull Changes from a Remote Branch
It's worth noting that you can't make modifications to a remote branch directly. As a result, you'll require a duplicate of that branch. Let's say you wanted to replicate the <branch-new> remote branch. Here how it’s done:
git checkout -b origin/feature_branch_name
It creates and checkouts a new branch called <branch-new>. It also pushes modifications to that branch from origin/<branch-new>.
With newer versions, you can simply use:
git fetch
git checkout
Different Options In Git Checkout
Using the above mentioned commands you can fetch, list and switch to branches. Git Checkout command is also used to undo changes in your working directory.
#1 To Checkout an Existing Branch
git checkout <branch_name>
You can simply checkout the existing branch using the above command.
#2 To Create and Switch Branch Simultaneously
git checkout -b <new-branch>
Here the git command first runs the git branch command and creates a new branch before running git checkout operation. With this you can automatically switch to the new branch.
#3 To Checkout a New Branch or Reset a Branch to a Start Point
You can use the same checkout command but with the -B flag and an optional START_POINT parameter.
git checkout -B <branch-name> START-POINT
Here if the branch name doesn't exist git will automatically create a new branch and starts it at the START-POINT. If the branch already exists it resets it to the START_POINT.
#4 To Force Checkout a Branch
To force git to switch branches you can pass the -f or --force option in the command as given below.
git checkout -f <branch-name>
# Alternative
git checkout --force <branch-name>
#5 To Undo Changes in Your Working Directory
To undo the changes that you have made in your file you can use the below command. Revert back the changes in the HEAD. This will delete all your modification. Be careful when running this command
git checkout -- FILE-NAME
eg:
git checkout -- src/server.js
#6 How To Checkout a Specific Commit
Get the specific commit id by running the following command.
git log
To checkout a particular commit you can us the below command.
git checkout specific-commit-id
Benefits of Git Checkout Remote Branch
Git is a fantastic tool for programmers who want to collaborate on coding projects. Consider ten programmers working on the same piece of code, each attempting to make their own changes and then attempting to integrate those changes without the use of a version control system.
- Multiple developers can work on a single piece of software using the git checkout remote branch, each making their own changes in a secure manner, without adding unstable code to functional software.
- Git checkout remote branch allows for failsafe review and collaboration with others.
- It helps bug management by making it more difficult for unstable code to be merged into the master repository.
- It's also useful for checking out remote branches.
- It helps in the uniform management of the branching mechanism during feature development and release management.
- It allows you to switch branches with a single command.
- Maintains a clean and readable repository and procedure.
Best Practices for Git Checkout Remote Branch
Since the git checkout, remote branch methods stated above are a subset of Git as a whole, working with git checkout remote branch follows the same recommended practices as dealing with Git as a whole, including:
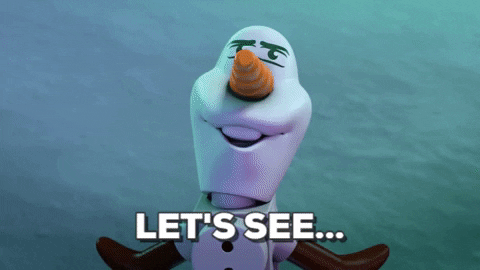
- Make Frequent Commitments
Keep commitments short and share work more regularly when committing regularly. It will be easier to prevent significant merge disputes as a result of this. - Don't Make a Commitment to Work that You haven't Finished
Break down the code for your feature into manageable bits. After you've finished a chunk, test it before committing it. This technique of working avoids the potential for conflicts that can arise when integrating huge amounts of code at once. At the same time, it ensures that you don't commit non-working code snippets. - Test Before You Commit
Don't commit anything until you've thoroughly tested it. Untested shared code can cause a lot of issues and wasted time for an entire team. - Commit Modifications That are Related
Make your commits small and limited to changes that are directly connected. When you repair two independent bugs, you should do it with two separate commits. - Create Short and Clear Commit Messages
Include a one-sentence summary of the changes you've made. After that, describe why the modification was made and how it differs from the prior version. - Make Use of Branches
Branches are a great way to minimize misunderstanding and keep development lines separate. - Make a Plan for Your Workflow
Before the project begins, your team should agree on a workflow. The project will determine whether this is done using topic-branches, git-flow, long-running branches, or another workflow.
Conclusion
During application development, Git branching makes collaboration simple like a snap. Different developers can easily work on different portions of the application at the same time via branches. Developers can copy remote branches locally on their systems, make changes, then push to the remote branches with git checkout remote branch, making collaboration even more smooth.
Do share your thoughts about Git Checkout Remote Branch with us at Atatus.
#1 Solution for Logs, Traces & Metrics
APM
Kubernetes
Logs
Synthetics
RUM
Serverless
Security
More