What is gRPC framework? How can we use it more effectively?
It was in 2015 that Google first developed gRPC as an extension of RPC(Remote Procedure Call) to link all its microservices. It was a closed tool operated only in their infrastructure then, but later, they opened it to the public, and gRPC has grown since then with community support. Now, it’s part of the CNFC project.
gRPC is designed to enable efficient and high-performance communication between distributed systems, making it easier for applications to call methods or functions on remote servers as if they were local.
It offers simple service definition, automatically generates idiomatic stubs in a variety of programming languages, has the ability to scale to millions of RPCs within a second along with bi-directional streaming and integrated pluggable authentication with HTTP/2-based transport.
Table Of Contents:-
gRPC Basic Concepts
At the core of gRPC is the service definition, which is defined using Protocol Buffers (Protobuf). A service is defined by specifying the methods it exposes, along with the input and output message types for each method. Protobuf
is a language-agnostic, efficient serialization format that allows developers to define the service interface in a clear and concise manner.
Once you define your gRPC service using Protobuf
, you can use the gRPC tools to generate client and server code in various programming languages. This code generation process creates language-specific classes and stubs that help developers interact with the gRPC service without worrying about the low-level details of communication.
In a gRPC architecture, there are typically two main components:
- gRPC client
- gRPC server.
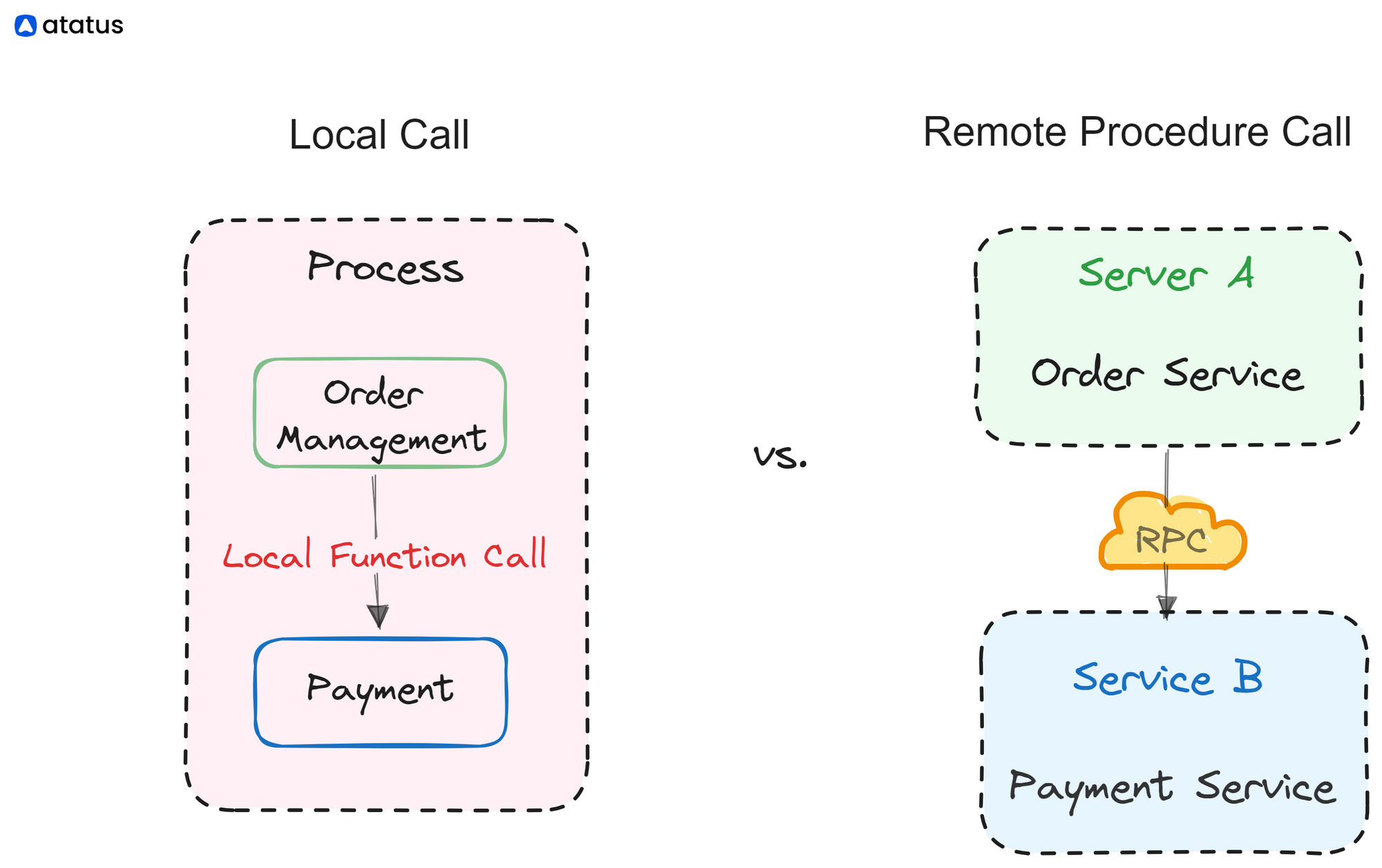
The client sends RPC requests to the server, and the server processes these requests and sends back responses. Both the client and server can be written in different programming languages as long as they support gRPC.
Advantages of using gRPC:
1. Serialization and Deserialization
gRPC uses Protobuf
for serialization and deserialization of messages. This binary serialization format is highly efficient and performs better than text-based formats like JSON. It reduces the size of data sent over the network and is also language-agnostic, allowing communication between services written in different programming languages.
2. HTTP/2
gRPC relies on the HTTP/2 protocol as its transport layer. HTTP/2 offers several advantages over its predecessor, including multiplexing multiple RPCs over a single connection, header compression, and support for bidirectional streaming. These features make gRPC suitable for high-performance and low-latency communication.
3. RPC Methods
gRPC services define a set of RPC methods, each of which corresponds to a specific operation that the client can request from the server. These methods are defined in the Protobuf
service definition and include input and output message types. gRPC supports four types of RPC methods:
- Unary: A simple request-response RPC.
- Server Streaming: The client sends a request, and the server streams a sequence of responses.
- Client Streaming: The client streams a sequence of requests, and the server sends a single response.
- Bidirectional Streaming: Both client and server can stream a sequence of messages independently.
4. Interceptors
gRPC allows you to define interceptors on both the client and server side. Interceptors are functions that can be used to perform common tasks such as authentication, logging, and monitoring for all RPC calls.
5. Error Handling
gRPC provides a well-defined error model that includes status codes and details to help clients and servers communicate errors effectively. This enables robust error handling in distributed systems.
gRPC Architecture Model
gRPC's architecture model is centered around defining services and their methods using Protocol Buffers, generating client and server code, and enabling efficient communication between services using HTTP/2 and binary serialization. It is designed to be highly performant, language-agnostic, and suitable for modern distributed systems and microservices architectures.
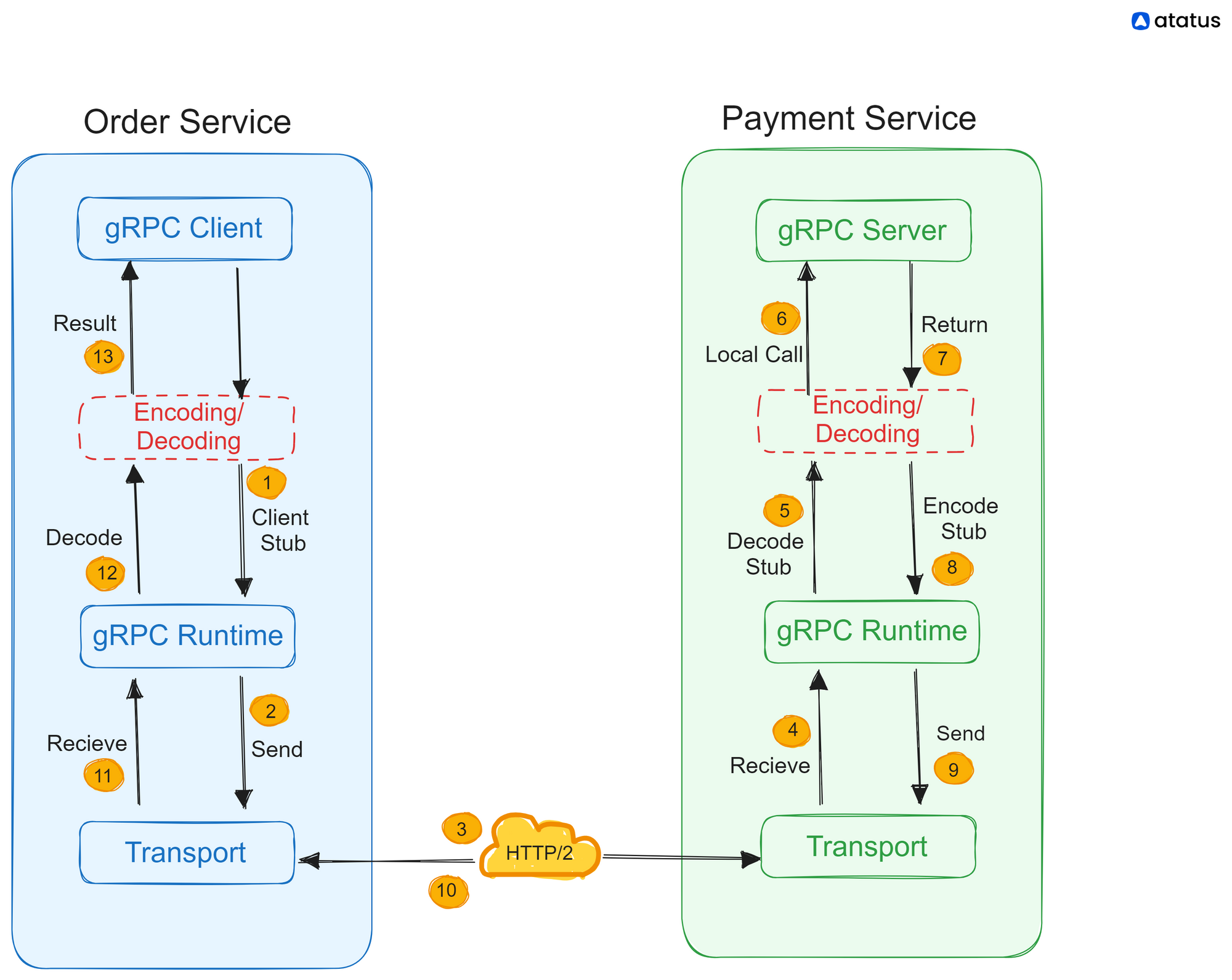
Middleware: You can add middleware to gRPC services to implement cross-cutting concerns like authentication, authorization, and logging in a reusable way. Middleware can be applied to all RPC methods or specific methods as needed.
How to use gRPC?
Using gRPC involves several steps, from defining your service and message types to implementing the server and client code in your chosen programming language. Here's a step-by-step guide on how to use gRPC:
#1 Start by defining your service and its methods using Protocol Buffers (Protobuf). You'll need to create a .proto file that defines your service interface and message types. Here's a simple example:
syntax = "proto3";
package myapp;
service MyService {
rpc SayHello(HelloRequest) returns(HelloResponse);
}
message HelloRequest {
string name = 1;
}
message HelloResponse {
string message = 1;
}
This example defines a service named MyService
with one RPC method, SayHello
, which takes a HelloRequest
message as input and returns a HelloResponse
message.
#2 Next, use the gRPC tools to generate client and server code based on your .proto file. The exact commands and options may vary depending on your programming language. For example, to generate Python code, you can use the grpc_tools package
:
python -m grpc_tools.protoc -I. --python_out=. --grpc_python_out=. myapp.proto
This command generates Python code for the client and server in the current directory.
#3 Now, write the server-side code that implements your gRPC service. You'll need to create a class that inherits from the auto-generated base class and provide implementations for your service methods. Here's a simplified Python example:
import grpc
import myapp_pb2
import myapp_pb2_grpc
class MyService(myapp_pb2_grpc.MyServiceServicer):
def SayHello(self, request, context):
return myapp_pb2.HelloResponse(message=f"Hello, {request.name}!")
def serve():
server = grpc.server(grpc.insecure_channel())
myapp_pb2_grpc.add_MyServiceServicer_to_server(MyService(), server)
server.add_insecure_port('[::]:50051')
server.start()
server.wait_for_termination()
if __name__ == '__main__':
serve()
#4 Write the client-side code to make gRPC calls to your service. You'll use the auto-generated client code to create a client stub and make RPC calls. Here's how you do it:
import grpc
import myapp_pb2
import myapp_pb2_grpc
def run():
channel = grpc.insecure_channel('localhost:50051')
stub = myapp_pb2_grpc.MyServiceStub(channel)
response = stub.SayHello(myapp_pb2.HelloRequest(name='Alice'))
print("Server Response:", response.message)
if __name__ == '__main__':
run()
#5 Before running your client, make sure the gRPC server is running. In the server implementation, we specified that it listens on port 50051. Start the server first and then run the client code.
In production, you should use secure communication by enabling Transport Layer Security (TLS) for your gRPC connections. This involves obtaining and configuring TLS certificates for both the server and client.
Remember that gRPC supports various programming languages, so the specific code and tools you use may vary depending on your chosen language. However, the core concepts and steps for using gRPC remain consistent across languages.
gRPC vs. REST
gRPC and REST are two different architectural styles for designing and implementing APIs. Here's a comparison of gRPC and REST in various aspects:
gRPC | REST | |
---|---|---|
Communication Protocol | It uses HTTP/2 as the underlying communication protocol, which offers features like bidirectional streaming, multiplexing, and header compression. This makes gRPC more efficient for high-throughput and low-latency scenarios. | REST typically uses HTTP/1.1 or HTTP/2 for communication. While HTTP/2 provides some improvements over HTTP/1.1, it lacks some of the advanced features of gRPC, such as bidirectional streaming. |
Serialization | Uses Protocol Buffers (Protobuf) by default for message serialization. Protobuf is binary and more compact than text-based formats like JSON, leading to reduced data size and faster serialization/deserialization. | Uses text-based formats like JSON or XML for data serialization, which are human-readable but less efficient in terms of size and parsing speed. |
Language Support | Provides support for multiple programming languages, including Java, Python, Go, C++, and more. Code generation tools generate client and server code in these languages based on the service definition. | Is language-agnostic and can be used with any programming language that supports HTTP. |
API Contract | Requires the definition of a service contract using Protocol Buffers, making it strongly typed and self-descriptive. This enforces a clear API contract and enables tooling for code generation. | Uses HTTP methods (GET, POST, PUT, DELETE, etc.) and relies on URI endpoints to define resources and actions. While it can use OpenAPI or Swagger for documentation, the API contract is not as strongly enforced as with gRPC. |
Error Handling | Provides a well-defined status code system that makes error handling more structured and clear. It includes rich details for error reporting. | Typically relies on HTTP status codes and custom error payloads in response bodies. While it can convey errors effectively, it may not be as standardized as gRPC. |
Performance | Generally offers better performance due to binary serialization, HTTP/2 efficiency features, and support for multiplexing and streaming. It's well-suited for scenarios where high throughput and low latency are essential. | While it can be performant, REST APIs may require additional optimization efforts to achieve the same level of performance as gRPC. |
Use Cases | Well-suited for microservices architectures, real-time applications, and scenarios requiring efficient and high-throughput communication. It's commonly used in internal microservices communication within a data center. | Remains popular for public-facing APIs and web services, where compatibility with a wide range of clients is important, and human readability of data is valued. |
Ecosystem and Tooling | Has a growing ecosystem of tools and libraries for various languages. It's widely adopted in cloud-native and containerized environments. | Has a mature ecosystem with numerous libraries and frameworks available for building and consuming RESTful APIs. |
Complexity | May have a steeper learning curve due to Protocol Buffers and code generation, but it can result in cleaner and more structured code. | Often considered simpler to understand and implement, especially for small to medium-sized projects. |
Disadvantages of gRPC
- gRPC may have a steeper learning curve, especially if you are new to Protocol Buffers and code generation tools. Understanding and configuring aspects like streaming and middleware can be challenging for beginners.
- The additional structure imposed by Protobuf and strongly typed contracts can lead to more complex code and development processes, especially for small or simple projects.
- While gRPC clients and servers can be implemented in various languages, there may be challenges in ensuring compatibility between different language versions of gRPC libraries.
- Debugging gRPC-based systems can be more challenging due to the binary nature of Protobuf messages. Tools for visualizing and debugging may not be as mature as those for text-based formats.
- For public-facing APIs where human readability and ease of use are paramount, gRPC may not be the best choice. RESTful APIs with JSON payloads are often preferred in such cases.
- Implementing secure communication in gRPC with Transport Layer Security (TLS) can be more involved and may require additional configuration compared to basic HTTP/HTTPS.
Conclusion
Both gRPC and REST have their own strengths and weaknesses, and the choice between them depends on your project requirements and constraints. Many organizations use a combination of both technologies, leveraging the strengths of each where they are most appropriate and the context in which each API will be used.
Due to its fast and efficient nature, gRPC has gained a lot of traction over the years, especially in microservices architectures. However, it also presents a new set of security challenges such as content validation, authentication, and authorization.
Monitor Your Entire Application with Atatus
Atatus is a Full Stack Observability Platform that lets you review problems as if they happened in your application. Instead of guessing why errors happen or asking users for screenshots and log dumps, Atatus lets you replay the session to quickly understand what went wrong.
We offer Application Performance Monitoring, Real User Monitoring, Server Monitoring, Logs Monitoring, Synthetic Monitoring, Uptime Monitoring, and API Analytics. It works perfectly with any application, regardless of framework, and has plugins.
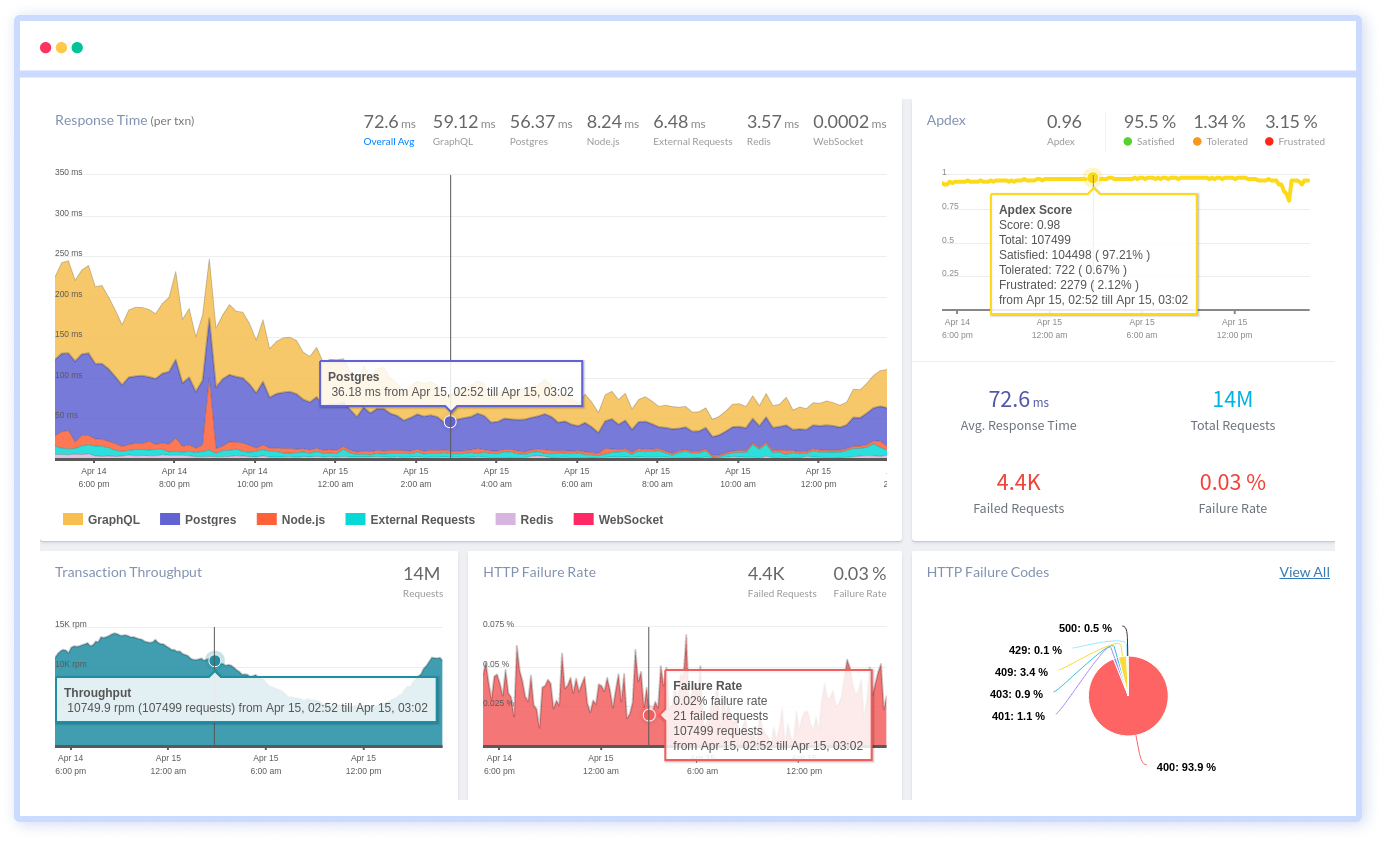
Atatus can be beneficial to your business, which provides a comprehensive view of your application, including how it works, where performance bottlenecks exist, which users are most impacted, and which errors break your code for your frontend, backend, and infrastructure.
If you are not yet an Atatus customer, you can sign up for a 14-day free trial.
#1 Solution for Logs, Traces & Metrics
APM
Kubernetes
Logs
Synthetics
RUM
Serverless
Security
More