Code Like a Pro: 10 tips for aspiring coders
The definition of good code varies for each person, but most define it as code that is fully tested. There are those who define that the performance and speed of this product are incredible, moreover, it runs perfectly on old hardware.
Though precisely, a clean good code focuses on adaptability and extensibility for an organization and lasts beyond its original timeframe. Coding is the language in which all computers intercommunicate and comprehend.
In computer programming, programmers give instructions to a computer and tell it how to execute certain actions. In software development, coding is a foundational element and therefore it must be simple to understand and modify.
Communication with specific systems mandates the use of diverse programming languages. It is very typical to use the terms code writing, coding, and programming interchangeably.
The function of writing good code is generally to provide instructions to a computer on its actions and how to carry them out. The codes are comprised of several languages, such as JavaScript, C#, Python, and various others.
Table Of Contents
Code and Coding
Rather than just knowing one programming language, it is important to know several, since they are used for different purposes. The relationship and connections between some languages are stronger than those between others.
Code is a written language with spelling conventions and syntax made up of letters, numbers, and different symbols. Programmers and code writers have a wide range of career options because they can use their skills to build anything from websites to aircraft.
Code is responsible for making all computer-related activities possible. There are countless other products that use code, notably web pages, vehicle controls, electronic games, smartphones, aircraft, and dozens more.
There is an ever-increasing demand for programming professionals thanks to the digitization of products. Businesses expect innovative and intense coders to deliver exceptional good code for new products in order to comply with buyer demand for modern and advanced products.
Significance of writing code
There are many instances where poorly written internal codes lead to the failure of big businesses and great products that could have been even better. The creation and deployment of any code base are made efficient by clean code.
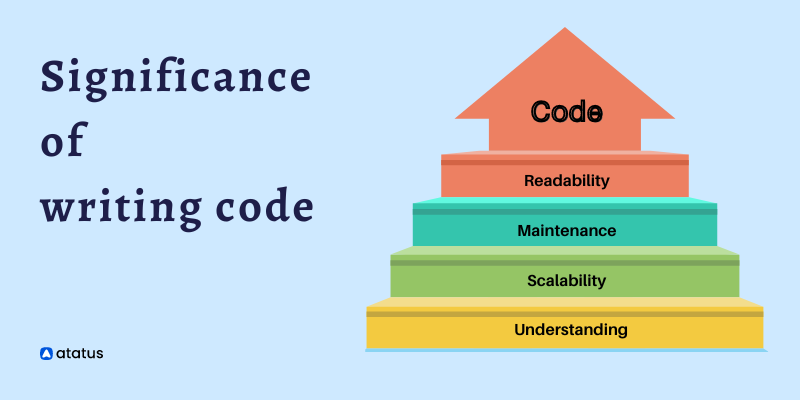
High-quality clean code has the following characteristics:
- Readability is a measure of how well a programmer can understand the code, whatever their level of programming experience may be. In terms of understanding and using the code, it does not require a lot of time.
- The time spent on maintenance is reduced. When you have clean code, you have less time to figure out what certain segments actually do, and more time to make it better, revise, and extend it.
- As with any successful product, the scalability is an essential part of success. With a stable, clean, and well-written code base, scaling and extending the code is easier.
- A better understanding of ideas is achieved. The more clean code you write, the less likely you are to have misunderstandings with your fellow programmers. That also results in fewer bugs in the long run.
10 must-know tips to write a clean code
In this article 10 effective methods are listed, with which you can imply instantly to begin writing more reliable good code. The subsequent are some beginner coding tips to help you improve your coding skills and to write better code right now!
1. Determine your goals
Make sure you consider your goals before you begin writing code. Programming generally involves the following techniques. Identifying the problem and designing a solution are the first steps in writing good code.
Prior to initiating to write a code, think about the outcome you want for the code to achieve. Start by writing pseudocode. Until real code is written, pseudocode exists. A code sketch is beneficial for scribbling out the overall structure without getting lost in details.
Drawing out a diagram of the issue will help you understand the steps that must be taken and how they relate to one another. You should also proofread your work. After drafting your plan, verify that it is justifiable by comparing it to any unreasonable assumptions.
Include inline comments while writing the code, describing your reasoning. Comments like these can be extremely useful when you or someone else revisits the code in the future. Developing good code requires testing and debugging to ensure it works as planned.
2. Use descriptive names for variables
Several names will be needed for variables, functions, classes, arguments, modules, packages, and directories. Keep your code named meaningfully. Regardless of the names you use, your code should serve three functions;
- Define its purpose,
- Justify its existence,
- Explain its function.
Ensure that the names are pronounceable. Be careful not to use strange or confusing names. Nouns should be used when naming variables, constants, and classes, and verbs should be used when naming functions and variables.
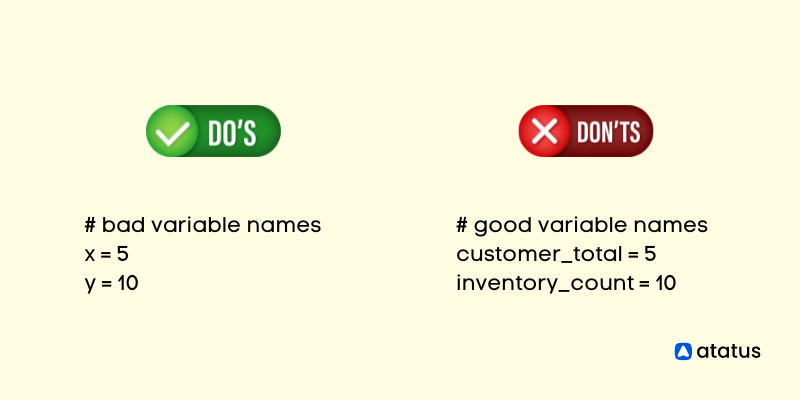
You may need to spend some time choosing meaningful names, but you will find that it will make your code look very clean and easy to read for other developers and yourself. In addition, try to make the names as concise as possible.
Writing good code requires proper naming. Using self-descriptive names for your methods, variables, and other custom definitions and simple to understand is extremely advantageous, not just for those who will interact with your code, but even for you as the code developer. Editing and extending it later will be easier.
3. Repetition is not allowed (code)
Automating is one of the fundamental functions of nearly all applications. All code should adhere to this principle, including web applications. It is not a good idea to keep repeating the same code.
The same function is sometimes implemented in multiple places, which can be harmful to the program. Code repetition will cause a document to grow very long and disrupt the reader's flow.
Creating a separate file and adding the path as necessary may be preferable if you frequently use the same code. For say, a standard web application is composed of many pages. A few elements from the previous page are probably present on this one as well.
This is usually best done in headers and footers. These headers and footers shouldn't be copied and pasted every time. Rather than putting them in separate source files, put them in separate source files that you can retain for later use.
Do's
const express = require('express');
const app = express();
// Avoid repeating code by extracting it into a separate function.
const calculateTotal = (price, tax) => {
return price + price * tax;
};
app.get('/orders', (req, res) => {
const orders = getOrders();
const processedOrders = orders.map((order) => {
const total = calculateTotal(order.price, order.tax);
return { ...order, total };
});
res.send(processedOrders);
});
app.get('/invoices', (req, res) => {
const invoices = getInvoices();
const processedInvoices = invoices.map((invoice) => {
const total = calculateTotal(invoice.price, invoice.tax);
return { ...invoice, total };
});
res.send(processedInvoices);
});
app.listen(3000, () => {
console.log('Server listening on port 3000');
});
Don'ts
const express = require('express');
const app = express();
// Don't include unnecessary code that serves no purpose.
const unnecessaryVariable = 'This variable is not used';
app.get('/orders', (req, res) => {
const orders = getOrders();
res.send(orders);
});
...
app.listen(3000, () => {
console.log('Server listening on port 3000');
});
4. Get rid of unnecessary code
Comment lines in code are normally used by developers to clarify a line's intention. The importance of comments is that they provide a lot of information about how your code works, but they entail more frequent maintenance.
It's common for code to be moved around a lot while it's being generated, but when a comment remains in one spot, problems can emerge. In addition to creating chaos among programmers, unworkable comments can also divert them.
When used appropriately, comments have no negative connotations; they occasionally serve a particular objective. For instance, only include comments if you are using third-party APIs and you need to explain a particular behavior. Otherwise, avoid doing so.
The old code should be commented out because keeping it is pointless. It will simply appear disorganized and excessively long. A line of code in modern programming languages is explained simply through English-like syntax. Make sure the variables, functions, and files you create have meaningful names so you do not have to add any comments.
Do's
const express = require('express');
const app = express();
// Only include the code that is necessary for the program to function.
app.get('/orders', (req, res) => {
const orders = getOrders();
res.send(orders);
});
app.listen(3000, () => {
console.log('Server listening on port 3000');
});
Don'ts
const express = require('express');
const app = express();
// Don't include unnecessary code that serves no purpose.
const unnecessaryVariable = 'This variable is not used';
app.get('/orders', (req, res) => {
const orders = getOrders();
res.send(orders);
});
app.listen(3000, () => {
console.log('Server listening on port 3000');
});
5. Single out a single problem
In addition to defining a clear objective, each class, function, or method should be good at doing one thing. By doing so, functions will remain compact and be easier to test, and other developers will find it simple to add additional functions as needed.
Coding quality control is an important aspect of your software development process. There can never be more than one task being worked on at once. Consider one issue and try to resolve it before moving on to another. For instance,
function subtract(x, y) {
return x - y;
}
The most common error made by new programmers is to create functions that are almost universally useful. Developers have a difficult time finding code or fixing bugs when attempting to locate a specific piece.
In an ideal world, every function and class would serve only one purpose, and every concept would be represented by a single class. Despite its simplicity, ensuring the work is clean, is the best approach.
6. Simple is best
When you're trying to fix something, you can't find it if you have a bunch of code. Instead, you should break it up into smaller projects based on features and functions.
Retaining the whole code in files and folders is very good for maintainability, especially if different teams and people are working on it. Coders should keep things simple when they code. In addition to being understandable and manageable, simple code is also easier to maintain. The problem with good coding is that bugs are also created.
Be careful not to overdo it. The quality of your code will determine whether you are a faster coder from the start. A good rule of thumb is to write less code. Code that is simpler to read and understand can be reused and altered more easily.
It will also reduce iterations and avoid any confrontations between the manager and developer later on if you align your code at the start of the project. Keeping the project objectives in mind while coding prevents future unnecessary changes by keeping them in mind during the coding process.
7. Ensure quality
Maintain a high standard of good code quality and consistency, because your code is your accomplishment. Maintainability, reusability, and readability are all important in writing a good code.
An example of writing good, efficient, and well-documented code:
// Use a linter to catch syntax errors
/* eslint-disable no-console */
const express = require('express');
const app = express();
// Write tests for your code
describe('My API', () => {
it('should return a 200 status code', () => {
// Test code goes here
});
});
// Use version control to track changes
const getUsers = () => {
// Function code goes here
};
// Document your code with clear and concise comments
/**
* Calculates the total price of an order.
* @param {number} price - The base price of the order.
* @param {number} tax - The tax rate for the order.
* @returns {number} The total price of the order.
*/
const calculateTotal = (price, tax) => {
return price + price * tax;
};
app.listen(3000, () => {
console.log('Server listening on port 3000');
});
A perfect programmer does not exist in the world. It is inevitable that a programmer will make mistakes while working on a project hence coding consistently with high-quality is significant. But In order to rectify a bug, you need to search further for the cause and the solution.
8. Optimize your code with arrays and loops
In order to write more suitable good code, you can be productive by employing the fundamental but effectual tools of arrays and loops. The topics are probably already acquainted to you if you have started to learn to code. An array allows you to organize data in a systematic manner.
Using this method can improve the efficiency and readability of your code. Automating repetitive tasks can be accomplished with loops, in contrast. If you know how to utilize them correctly, you can save a great deal of time and energy.
By using them, one frequently does not have to create nested conditional blocks that are complex and intricate. Since there are so numerous forks in the logic flow with nested-if statements, they are complicated to read.
Avoid using unnecessary nested for
loops:
const numbers = [1, 2, 3, 4, 5];
// DO: Use a loop to iterate over the elements in the array
for (let i = 0; i < numbers.length; i++) {
console.log(numbers[i]);
}
// DON'T: Nest loops unnecessarily
const numbers = [1, 2, 3, 4, 5];
for (let i = 0; i < numbers.length; i++) {
for (let j = 0; j < numbers.length; j++) {
console.log(numbers[i] * numbers[j]);
}
}
// This is unnecessarily verbose and inefficient. The inner loop is unnecessary, as we only need to multiply each number by itself.
Try using the appropriate loop types such as while
, for
, do-while
:
const numbers = [1, 2, 3, 4, 5];
// Use a for loop if you need to iterate over a fixed number of elements
for (let i = 0; i < numbers.length; i++) {
console.log(numbers[i] * numbers[i]);
}
let i = 0;
// Use a while loop if you need to keep looping until a certain condition is met
while (i < numbers.length) {
console.log(numbers[i] * numbers[i]);
i++;
}
Use the break and continue statements to exit a loop or skip an iteration:
const numbers = [1, 2, 3, 4, 5];
for (let i = 0; i < numbers.length; i++) {
if (numbers[i] % 2 === 0) {
// Skip the current iteration if the number is even
continue;
}
console.log(numbers[i] * numbers[i]);
}
for (let i = 0; i < numbers.length; i++) {
if (numbers[i] > 3) {
// Exit the loop when the number is greater than 3
break;
}
console.log(numbers[i] * numbers[i]);
}
Use loop variables to track the current iteration and make use of their values:
const numbers = [1, 2, 3, 4, 5];
for (let i = 0; i < numbers.length; i++) {
console.log(`The square of ${numbers[i]} is ${numbers[i] * numbers[i]}`);
}
9. Compose good comments
Commenting on your code regularly is a good habit. A great way to improve the clarity of your code is to use this approach. Adding comments to your writing is useful if you keep them to a minimum and limit them to key passages.
No matter how neat the style is and how consistent the indentation is, there will be too many comments on every single line, making the code unreadable and chaotic.
They are not here to elucidate your code in depth; rather, they are there to furnish documentation. Your remarks ought to be succinct, concise, and direct. Use comments to emphasize the following points to help:
- Apparently declare the intent of a function or a block of code.
- Alert users to any potential exceptions or programming errors.
- Code sections and functions should be highlighted based on their primary function.
Do's
const express = require('express');
const app = express();
// Good comments explain the purpose of the code and how it works.
/**
* Calculates the total price of an order.
* @param {number} price - The base price of the order.
* @param {number} tax - The tax rate for the order.
* @returns {number} The total price of the order.
*/
const calculateTotal = (price, tax) => {
return price + price * tax;
};
// Good comments use proper grammar and spelling.
app.get('/users', (req, res) => {
// Get a list of users from the database
const users = getUsers();
// Send the list of users back to the client
res.send(users);
});
app.listen(3000, () => {
console.log('Server listening on port 3000');
});
Don'ts
const express = require('express');
const app = express();
// Don't write comments that are redundant or unnecessary.
const calculateTotal = (price, tax) => {
// Calculate the total price of an order
return price + price * tax;
};
// Don't use poor grammar or spelling in your comments.
app.get('/users', (req, res) => {
// get list of users from db
const users = getUsers();
// send list of users back to client
res.send(users);
});
app.listen(3000, () => {
console.log('Server listening on port 3000');
});
10. Refactoring code
Refactoring is the process of making changes to code without altering its functionality. For the intention of enhancing clarity and efficiency, you can think of it as decluttering.
This excludes both bug fixes and the inclusion of any unique attributes. The term "refactoring" is simply a fancy way of saying "cleaning up the code" without changing how it functions.
During your freshest thought, it may be a good idea to refactor the code that you wrote the day before so that it is more accessible and portable beyond your immediate view. Refactoring is an opportunity to take advantage of any best practices that improve the readability of the good code.
Besides, editing is a noteworthy part of writing, and refactoring is a noteworthy part of good coding. This tip is truly the most influential one to assume, as the disinclination to refactor is the briefest way to initiate inaccessible code.
const express = require('express');
const app = express();
// Extract complex logic into separate functions to make the code easier to read and understand.
const calculateTotal = (price, tax) => {
return price + price * tax;
};
app.get('/orders', (req, res) => {
const orders = getOrders();
const processedOrders = orders.map((order) => {
const total = calculateTotal(order.price, order.tax);
return { ...order, total };
});
res.send(processedOrders);
});
app.listen(3000, () => {
console.log('Server listening on port 3000');
});
Recapitulations
There isn't a right way to write good code, but there are plenty of wrong ways, and it will take years to master. A developer needs to be able to write good code but comprehends to write good code endure time and preparation.
Programmers who are learning how to write good code are like novelists learning how to write clean prose. If you wish to become a competent programmer, you must follow these tips to enhance the code and your coding skills.
By writing code regularly, you will be able to improve its quality. Stay on top of your development skills by learning from experienced developers and practicing it frequently.
A developer's ability to read and write good code is fundamental to his or her ability to maintain the code and work with his or her team. Hence a developer must design or make a code which enriches the readability of the good code.
Monitor Your Entire Application with Atatus
Atatus is a Full Stack Observability Platform that lets you review problems as if they happened in your application. Instead of guessing why errors happen or asking users for screenshots and log dumps, Atatus lets you replay the session to quickly understand what went wrong.
We offer Application Performance Monitoring, Real User Monitoring, Server Monitoring, Logs Monitoring, Synthetic Monitoring, Uptime Monitoring and API Analytics. It works perfectly with any application, regardless of framework, and has plugins.
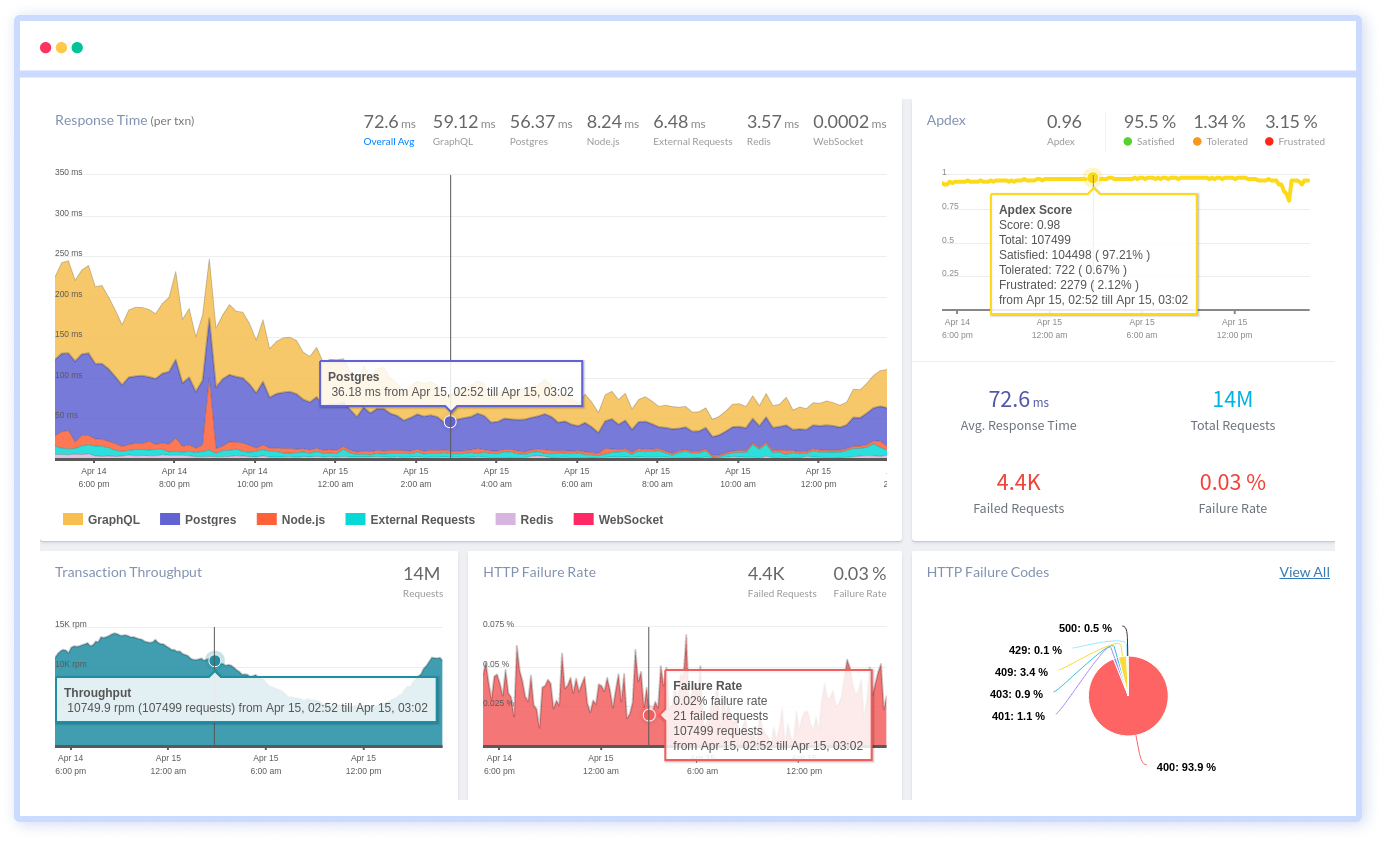
Atatus can be beneficial to your business, which provides a comprehensive view of your application, including how it works, where performance bottlenecks exist, which users are most impacted, and which errors break your code for your frontend, backend, and infrastructure.
If you are not yet a Atatus customer, you can sign up for a 14-day free trial .
#1 Solution for Logs, Traces & Metrics
APM
Kubernetes
Logs
Synthetics
RUM
Serverless
Security
More