Containers are great for developers, but containers are also an excellent way to create reproducible, secure, and portable applications.
Applications can be deployed reliably and migrated to multiple computing environments quickly using containers.
It could be the developer's laptop to the testing environment or staging to the production environment.
To use Dockers, Kubernetes, etc., it has been necessary to build containers. A Docker is used as a configuration management tool and a container engine that helps run an application with a high level of abstraction and security.
It provides your application with key components that make it run efficiently in different environments.
It is possible to write code quickly, test it, and deploy it to production with quick shipping, testing, and deployment with docker methodologies.
In this section, we will cover the following topics;
Table of Contents
- What is a Dockerfile?
- SHELL and EXEC FORM
- Docker CMD
- Docker ENTRYPOINT
- When to use CMD and ENTRYPOINT
- Using ENTRYPOINT with CMD
#1 What is a Dockerfile?
Basically, Dockerfiles are simple text files with instructions on how to create Docker images.
The containers created by Dockerfiles can run on any Linux server. Business applications can be more flexible and portable using Dockerfiles. Applications and their dependencies are packaged using Dockerfiles and run in virtual containers, either on premises or in public clouds.
There are three specific types of commands that can be used to build and run Dockerfiles:
- RUN - To run this command you will need a separate new layer and this command is mainly used to build images, and install packages and applications.
- CMD - The CMD describes the default container parameters or commands. The user can easily override the default command when you use this.
- ENTRYPOINT - A container with an ENTRYPOINT is preferred when you want to define an executable. You can only override it if you use the --entrypoint flag.
#2 SHELL and EXEC FORM
To understand the docker daemon process, you will need to build a docker file. To build a sample dockerfile using the two commands:
SHELL FORM:
The Syntax for any command in SHELL form:
<instruction> <command>
EXEC FORM:
The syntax for any command in EXEC form:
<instruction> ["excecutable" "parameter"]
To write docker CMD/ENTRYPOINT in both forms:
- CMD SHELL form - CMD echo "Atatus - Full-stack monitoring tool"
- CMD EXEC form - CMD ["echo", "Atatus - APM monitoring solution"]
- ENTRYPOINT SHELL form - ENTRYPOINT echo "Atatus - Observability platform"
- ENTRYPOINT EXEC form - ENTRYPOINT ["echo", "Atatus - Observability Platform"]
#3 Docker CMD
Docker CMD produces a default executable for a Docker image. A container can be built on top of this image without command-line arguments. A docker image will run whatever command is specified by the CMD command. It is the default executable for a docker image.
Using the CMD command to create a Dockerfile and build an image
Step-1: Create a new folder named docker-test to store the new images.
mkdir docker-test
Step-2: Move to the new folder that you have created using the below command.
cd docker-test
touch Dockerfile
Step-3: Open the docker file with the text editor of your preference.
vi Dockerfile
Step-4: Add the following content in your docker file.
FROM ubuntu
RUN apt-get update
CMD ["echo" , "Try Best APM Monitoring Tool at atatus.com"]
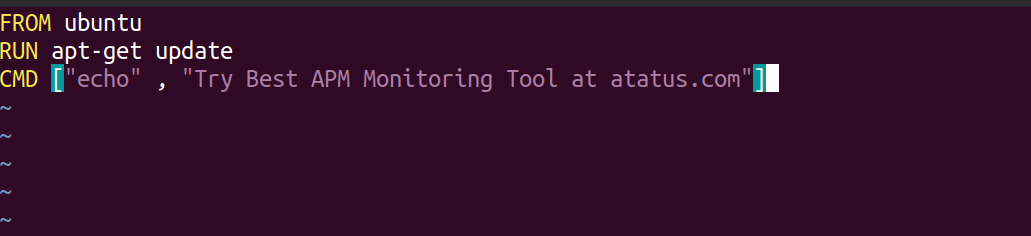
Step-5: Save and exit from the file.
Step: 6 You can now build the docker image from the newly created docker file. As long as you are in the same directory, you need not specify the location of the docker directory. Just build the image by running the following command:
sudo docker build .
To add the tag to the build use the below command:
sudo docker build -t [tag_name] .
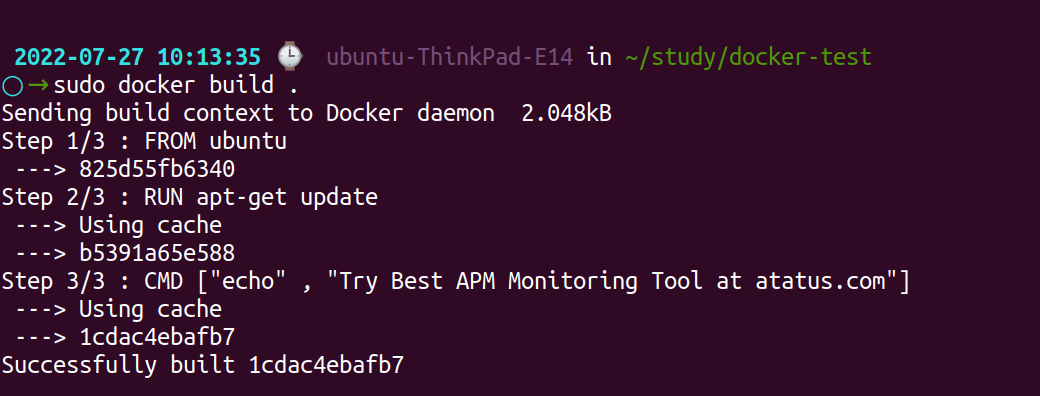
Step: 7 Use the following command to find out if the newly created image is stored locally.
sudo docker images
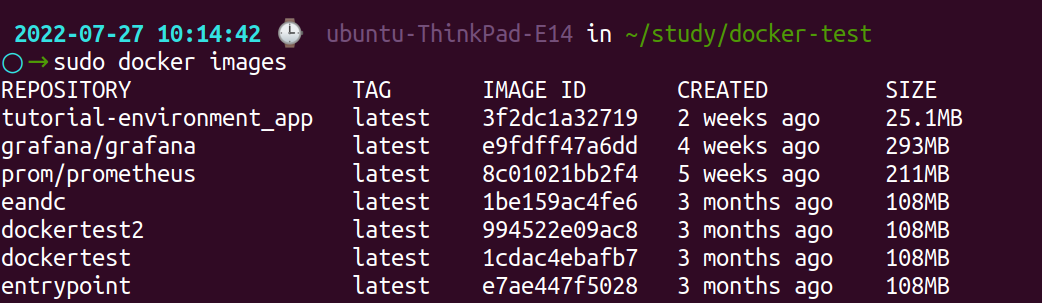
Step: 8 Run the container with the following command.
sudo docker run [image_name/image_id]
Upon running the command, the container will run the default CMD instructions, displaying the text entered.

If we add an argument to the run command it overrides the default instruction.
sudo docker run dockertest hostname

With the overridden CMD instructions the above command will run and display the hostname ignoring the echo instruction in the docker file.
#4 Docker ENTRYPOINT
It is used to set executables that will run only when the container is initiated. ENTRYPOINT commands cannot be overridden or ignored even if command-line arguments are stated.
This can be written in both Exec and SHELL form.
Using the ENTRYPOINT command to create a Dockerfile and build an image
Step: 1 Here I have used the same directory and docker file to use the ENTRYPOINT command. You can also create a new docker folder and a docker file. Open the existing file with the vi editor:
vi Dockerfile
Step: 2 Replace the content with the below one:
FROM ubuntu
RUN apt-get update
ENTRYPOINT ["echo" , "Try Best APM Monitoring Tool at atatus.com"]
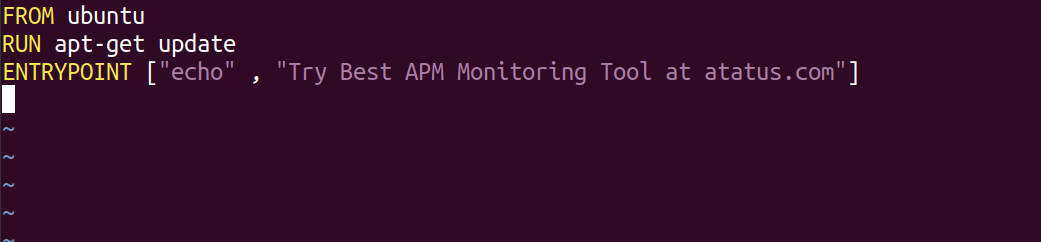
Step: 3 Save the file and exit.
Step: 4 You can now build the docker image from the newly created docker file. As long as you are in the same directory, you need not specify the location of the docker directory. Just build the image by running the following command:
sudo docker build .
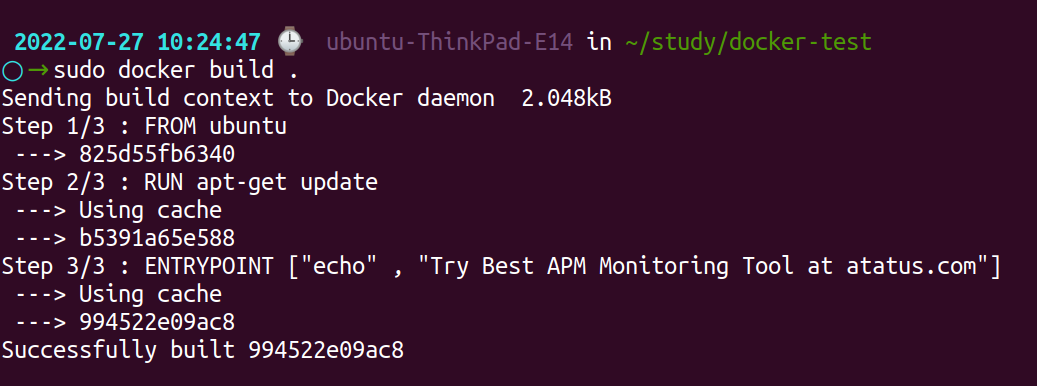
To add the tag to the build, use the below command:
sudo docker build -t tagname .
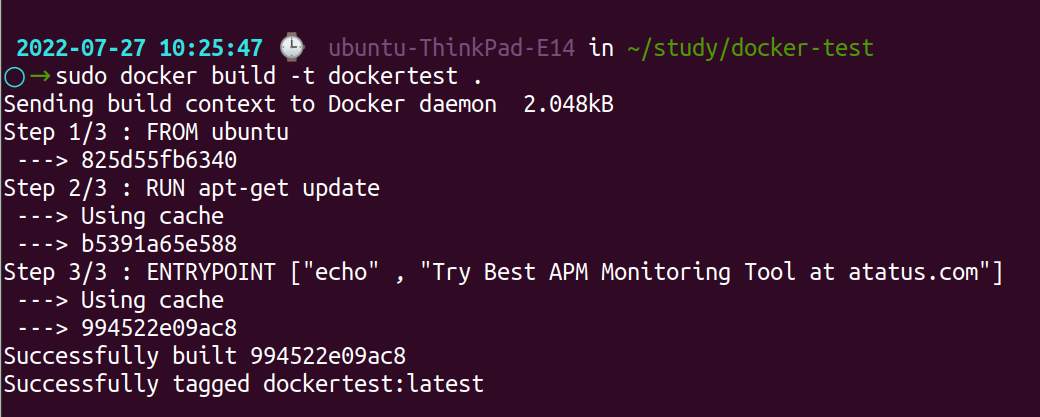
Step: 4 Use the following command to find out if the newly created image is stored locally.
sudo docker images
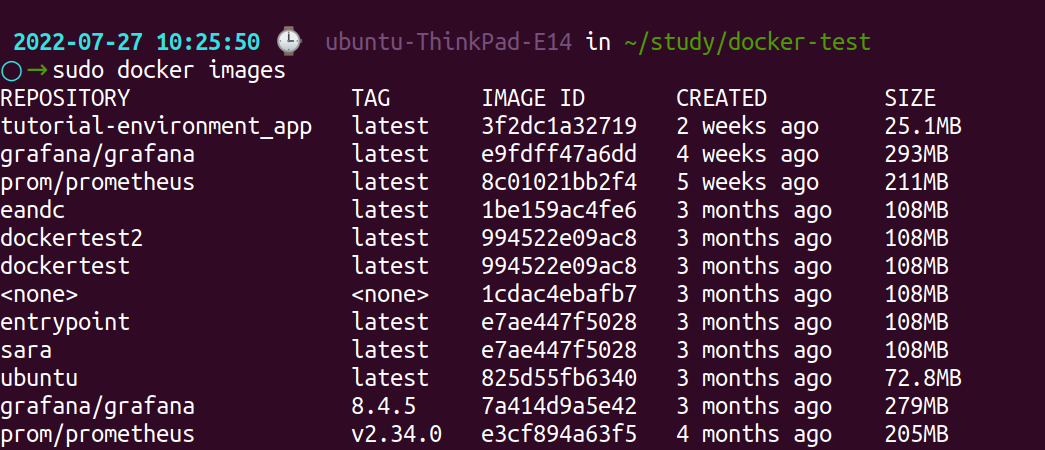
Step: 5 Run the container with the following command.
sudo docker run [image_name]

#5 When to use CMD and ENTRYPOINT
CMD can be used effectively if the default instructions are set in advance to run when no arguments are typed in the command line.
This will lead the CMD argument to store and load the container image as soon as possible. To override instructions, docker-run can be executed through a CLI.
ENTRYPOINT can be used in both single purpose and multi-mode images, which will contain a specific command to run the container.
Besides building wrapper container images for legacy programs, it is also used to ensure that programs will always run, which is achieved by using an ENTRYPOINT instruction.
#6 Using ENTRYPOINT with CMD
ENTRYPOINT and CMD both are not similar and the instructions are not manually exclusive. Still, they both can be used in your Docker file.
There are many such cases where we can use both ENTRYPOINT and CMD. The thing is that you will have to define the executable with the ENTRYPOINT and the default parameters using the CMD command.
Maintain them in exec form at all times.
To run a container with ENTRYPOINT and CMD
Step: 1 Open and modify the existing docker file using the below command or create a new file.
vi Dockerfile
Step: 2 Add the below content to the file. The docker file should contain the ENTRYPOINT instruction specifying the executable and CMD instruction specifying the default parameters.
FROM ubuntu
RUN apt-get update
ENTRYPOINT ["echo", "Affordable Observability Platform - atatus.com"]
CMD [/bin/bash]
Step: 3 Build a new image for the changed docker file.
sudo docker build .
Step: 4 Test the container with no parameters.
sudo docker run [container_name]
The above command will display the text entered in the docker file.
Step: 5 Try adding parameters to the docker run command and see what happens.
sudo docker run [container_name] [your_name/some_text]
The output would have been changed and this is because we cannot override the ENTRYPOINT instructions, whereas CMD instructions can be overridden.
There are use cases where both ENTRYPOINT and CMD can be used in the same dockerfile.
Conclusion
Both docker CMD and ENTRYPOINT instructions are necessary while building and executing a dockerfile.
Choosing the most suitable one completely depends on your use case.
- If you have an auxiliary set of arguments, use CMD instructions as a default method, unless there is an explicit command line reference when a docker container runs. It can be used if a user needs a default command that may be easily overridden.
- You can use ENTRYPOINT if you want to define a container with specific executables.
Experiment with both the instructions and find the best solution that works for you.
Monitor Your Entire Application with Atatus
Atatus is a Full Stack Observability Platform that lets you review problems as if they happened in your application. Instead of guessing why errors happen or asking users for screenshots and log dumps, Atatus lets you replay the session to quickly understand what went wrong.
We offer Application Performance Monitoring, Real User Monitoring, Server Monitoring, Logs Monitoring, Synthetic Monitoring, Uptime Monitoring and API Analytics. It works perfectly with any application, regardless of framework, and has plugins.
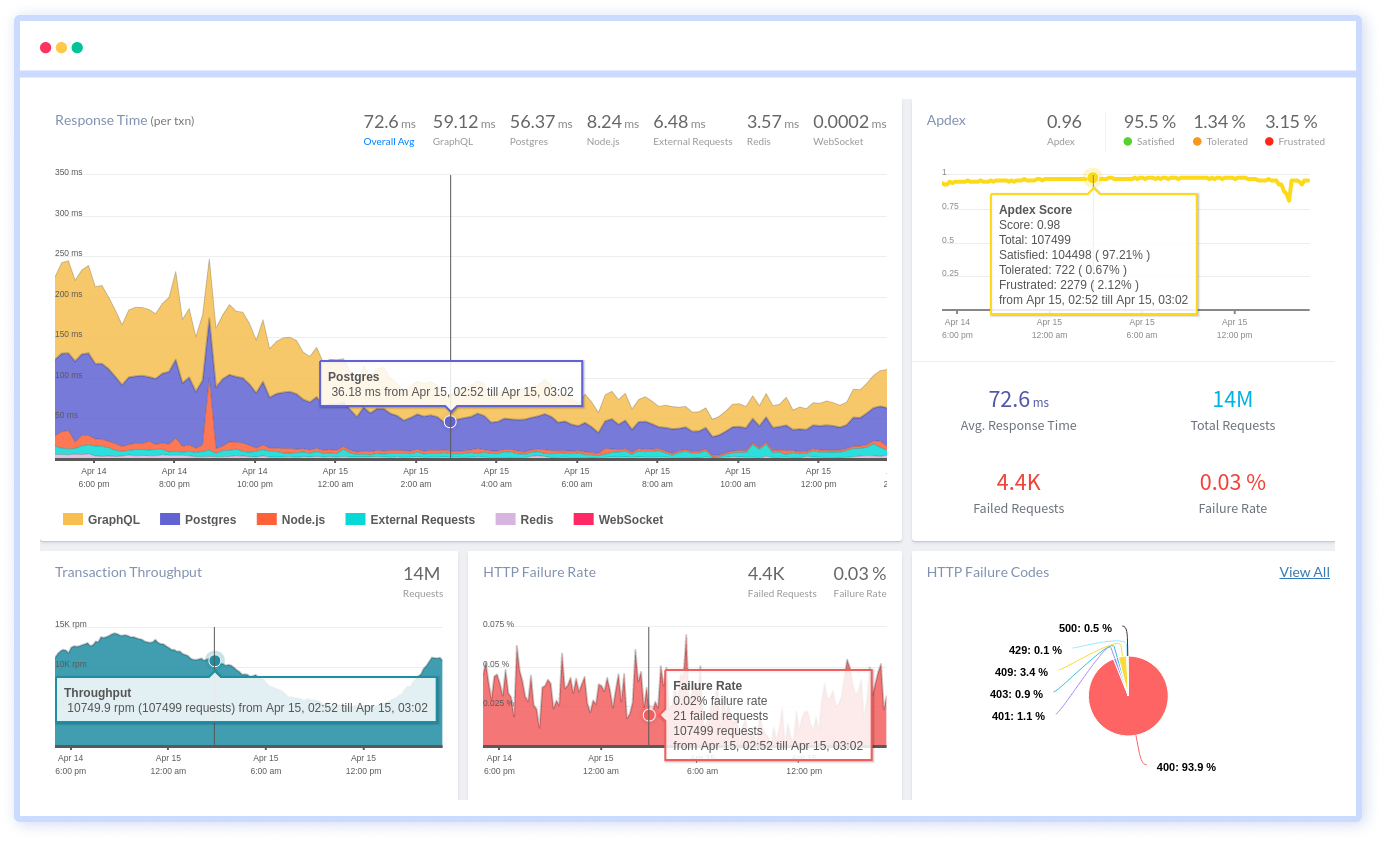
Atatus can be beneficial to your business, which provides a comprehensive view of your application, including how it works, where performance bottlenecks exist, which users are most impacted, and which errors break your code for your frontend, backend, and infrastructure.
If you are not yet a Atatus customer, you can sign up for a 14-day free trial .