A Complete Guide To React Fragments
React is one of the development languages that’s rising in popularity exponentially amongst front-end developers. However, the language comes with its own set of challenges and can make common tasks such as returning multiple HTML components a space-consuming task when handled using generic methods.
This makes it essential for developers to learn about react functions such as React Fragments in order to create an effectively readable and easy-to-execute codebase.
With this blog, we will take an in-depth look into how React fragments can help you execute multiple HTML components without affecting the DOM nodes in your codebase for a faster and improved development experience.
- What are React Fragments and When to use them?
- Difference between React Fragment and React Component
- Step-by-Step Guide to using React Fragments
- Real-Time Uses Of React Fragments
- Reasons React Fragments Is A Must For Developers
- Avoiding Common Pitfalls when working with React Fragments
What are React Fragments and When to use them?
React Fragments were first introduced with version 16.2.0 in 2017 and since then it has become mainstream for react developers. React fragments can help you wrap multiple components within an HTML tag without disrupting the DOM nodes in your codebase.
Issue: In React, when you create a component that returns multiple HTML tags, you'll often need to wrap them in a single parent element like a <div>. This is because React requires that each component have a single root element.
However, sometimes using an <div> as the parent element can be inconvenient or semantically incorrect. For example, you should return a list of items that should be wrapped in <li>
tags, where you can't use a div
or span
as the parent element.
To solve this problem, React provides a feature called fragments, with which you can use it by wrapping your elements in <React.Fragment>
tags, or by using the shorthand syntax <> </>
.
Earlier to achieve this developers would usually use div tags however they expand your codebase since these tags usually add up in the DOM nodes making your codebase expand exponentially. However, in the case of React Fragments, its components can easily render without needing a DOM node wrapping it.
A common approach in component-based development is for a component to render a list of child components or elements as part of its output.
For example:
<Columns />
must return multiple elements to render valid HTML. If a parent div
was used inside the render()
, then the resulting HTML will be invalid.
class Columns extends React.Component {
render() {
return (
<div>
<td>Hello</td>
<td>World</td>
</div>
);
}
}
class Table extends React.Component {
render() {
return (
<table>
<tr>
<Columns />
</tr>
</table>
);
}
}
In the above example, we have wrapped the <td>
elements inside the <div>
which will be considered as invalid in HTML.
In HTML this will translate into:
<table>
<tr>
<div>
<td>Hello</td>
<td>World</td>
</div>
</tr>
</table>
We can resolve the above issue using React fragments.
class Columns extends React.Component {
render() {
return (
<React.Fragment>
<td>Hello</td>
<td>World</td>
</React.Fragment>
);
}
}
The above code results in a correct <Table />
output.
<table>
<tr>
<td>Hello</td>
<td>World</td>
</tr>
</table>
Difference between React Fragment and React Component
React Fragment and React Component are two important concepts in React. Both are used to create reusable and modular pieces of UI. However, there are some key differences between the two.
A React Component is a JavaScript class or function that returns a React element. A React element is a description of what you want to see on the screen, typically in the form of a virtual DOM node.
Here's an example of a simple React Component that renders a greeting message:
import React from 'react';
class Greeting extends React.Component {
render() {
return <h1>Hello, {this.props.name}!</h1>;
}
}
export default Greeting;
In this example, we define a class called Greeting
that extends the React.Component
class. The render()
method of this class returns a React element that renders a greeting message with the name of the person passed in as a prop.
A React Fragment is a way to group a list of children without adding extra nodes to the DOM. When you render a list of components, you usually wrap them in a container element.
However, this can create unnecessary DOM elements that can slow down your app. With React Fragment, you can group a list of children without adding a container element to the DOM. Here's an example:
import React from 'react';
function GreetingList(props) {
return (
<>
<Greeting name="Alice" />
<Greeting name="Bob" />
<Greeting name="Charlie" />
</>
);
}
export default GreetingList;
In this example, we define a function called GreetingList
that returns a React Fragment containing three instances of the Greeting
component. The <>
and </>
syntax is a shorthand for React.Fragment
. This will render three greeting messages without creating any extra DOM elements.
React Component is a reusable piece of UI that returns a React element, while a React Fragment is a way to group a list of children without adding extra nodes to the DOM.
Step-by-Step Guide to using React Fragments
React fragments can be particularly useful when you have a component that returns multiple elements, but you don't want to add an unnecessary container element to the DOM. In this step-by-step guide, we'll walk through how to use React Fragments in your project.
Step 1: Import React
The first step is to import React into your project. This is typically done at the top of your file.
import React from 'react';
Step 2: Create Your Component
Next, create a component that returns multiple elements. For this example, let's create a component that returns a list of items.
Step 3: Add React Fragments
To use React Fragments, you need to wrap your list items in a set of empty angle brackets (<>
) and closing angle brackets (</>
). This tells React to group the list items together without creating a container element.
function MyListComponent() {
return (
<React.Fragment>
<li>Application performance monitoring</li>
<li>Real user monitoring</li>
<li>Synthetic monitoring</li>
</React.Fragment>
);
}
Step 4: Render Your Component
Finally, render your component in your application.
function MyApp() {
return (
<div>
<h1>My List Component</h1>
<ul>
<MyListComponent />
</ul>
</div>
);
}
ReactDOM.render(<MyApp />, document.getElementById('root'));
And that's it! You've successfully used React Fragments in your project. Remember that React Fragments can be used anywhere that you need to group a list of elements together without adding an unnecessary container element to the DOM.
Real-Time Uses Of React Fragments
Now that you've gained in-depth insights into what react fragments are moving further let’s take a look into some of the real-life instances where using react fragments can be of great aid in your development journey.
1. To wrap multiple HTML elements
One of the core functionalities of React Fragments is that it can help you wrap multiple HTML elements in one fragment tag. To achieve this whenever you want to return multiple HTML elements wrap them with React. Fragment tag or empty tag.
Here’s a working example of returning multiple HTML elements with React Fragments:
function FragmentExample () {
// returning 4 <div>s without
// adding an extra wrapper <div>
return (
<React.Fragment>
<div> Application monitoring </div>
<div> Synthetic Monitoring </div>
<div> Logs Monitoring </div>
<div> API monitoring </div>
</React.Fragment>
);
}
ReactDOM.render(
<FragmentExample/>,
document.getElementById('container')
);
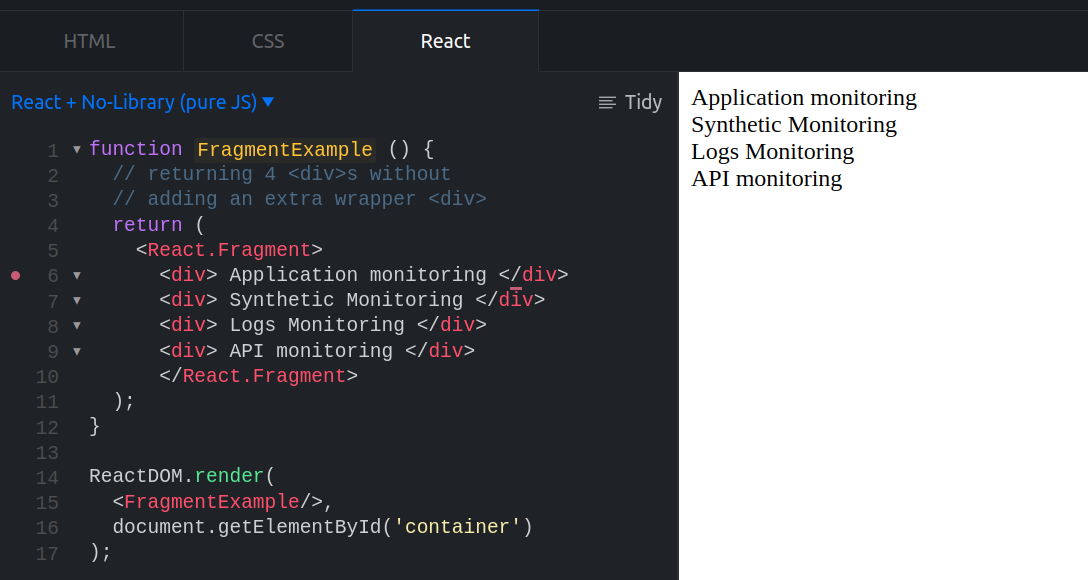
2. Using keyed React Fragments
The React.Fragment comes with keys that can make dealing with data collections such as arrays in Javascript much easier. Although if you’re using empty tags for react fragments this might not be a viable option for you.
You can easily use key props to map elements in React to that of HTML elements. In the example below we are taking an array and we will use react fragment keys to map them with their corresponding HTML elements effectively.
function FragmentExample(props) {
writers = [
{
id: 1,
fullName: "Jhonny",
birthDate: "09/08/1990",
},
{
id: 2,
fullName: "Mary",
birthDate: "12/20/2000",
},
{
id: 3,
fullName: "Olive",
birthDate: "10/08/1997",
},
];
return (
<div>
<h3>Writers</h3>
{writers.map((writers) => {
// the short syntax cannot be used here!
return (
<React.Fragment key={writers.id}>
<p>{`${writers.fullName} (${writers.birthDate})`}</p>
</React.Fragment>
);
})}
</div>
);
}
ReactDOM.render(<FragmentExample />, document.getElementById("container"));
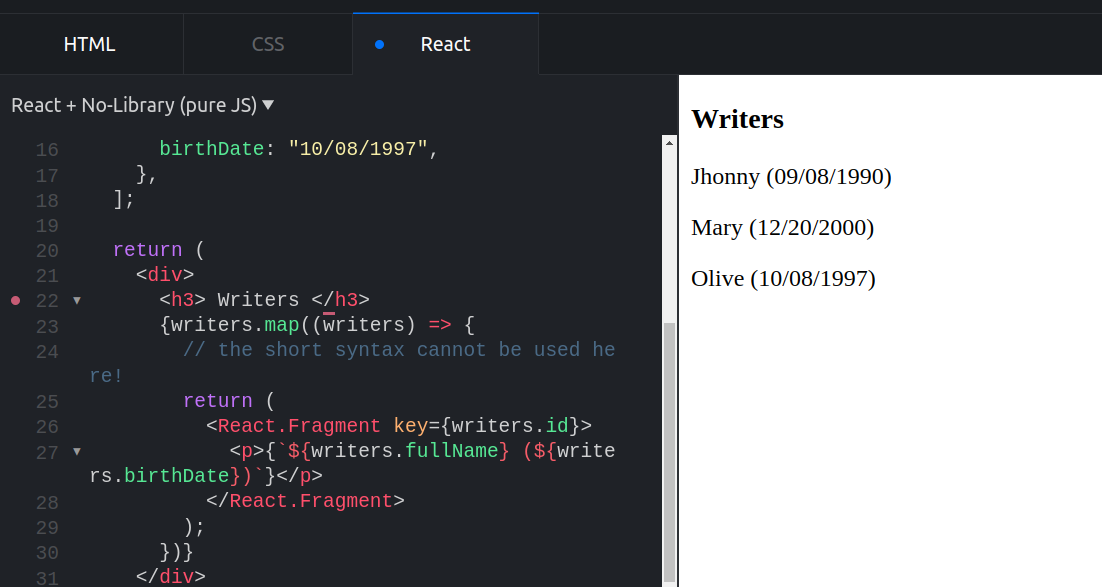
3. Implementing Fragments in CSS flexbox layout
You can further effectively place your CSS elements in the browser by using react.fragments. With this, you can easily add more certainty to your UI design and make it consistent throughout your web development projects.
In the example below we will place three colored boxes adjacent to each other with the help of React fragment in this example to ease the code you can even replace react fragment with its shorthand as we discussed in the earlier chapters.
import "./styles.css";
import React from "react";
const Row = ({ children }) => <div className="row">{children}</div>;
const Column = ({ children }) => <div className="col">{children}</div>;
const Box = ({ color }) => (
<div className="box" style={{ backgroundColor: color }}></div>
);
export default function App() {
return (
<Row>
<ChildComponent />
<Column>
<Box color="green" />
</Column>
</Row>
);
}
const ChildComponent = () => (
<React.Fragment>
<Column>
<Box color="pink" />
</Column>
<Column>
<Box color="black" />
</Column>
</React.Fragment>
);
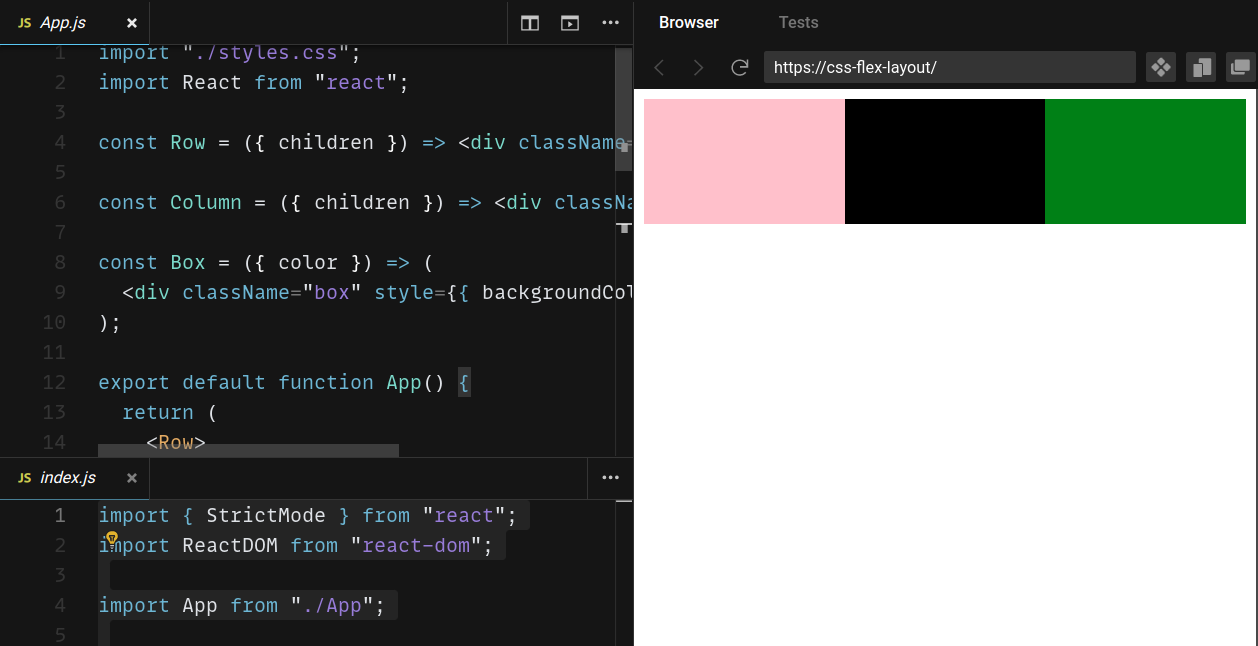
4. Rendering HTML lists with React Fragments
Lists are essential UI components and it always comes in handy if you’re trying to showcase data effectively to your users. However, if you’re working with a long list of objects, using div to render it in the UI can be exhausting and it can even make your codebase unnecessarily long and hard to manage.
In such cases using the react fragment feature can easily help you render the list without stretching the codebase.
In the example below we will use react fragment to render a basic ordered list in the browser.
import React from 'react'
const Countries = [{
id : "1",
Name : "United Kingdom",
Currency : "Euro"
},{
id : "2",
Name : "America",
Currency : "Dollar"
}, {
id : "3",
Name : "Tokyo",
Currency : "Japanese-Yen"
}]
function App() {
return (
<>
{
Countries.map(item => (
<React.Fragment key = {item.id}>
<h3> Name of the Country is : {item.Name} </h3>
<h3> Currency is : {item.Currency} </h3>
</React.Fragment>
))
}
</>
);
}
export default App;
5. Using React Fragment for Conditional Renders
Conditional renders are essential elements in web development as it helps users to reach the right information by meeting certain conditions. However, in traditional methods, this would mean adding unnecessary HTML tags and expanding your codebase. With React fragments you can make this process faster and more reliable.
In the example below, we will set two messages one for admin and the other for non-admins and we will set a boolean field isadmin
you can manually set it to true and false in order to see the designated messages for each group of users. You can use this concept for dynamic cases as well.
function FragmentExample(props) {
const { isAdmin } = props;
return (
<div className="card">
{isAdmin ? (
<>
<h3> message</h3>
<p>We are using React Fragment!</p>
</>
) : (
<>
<h3>Sorry you can't see this text </h3>
<p>change isadmin to true for seeing the text</p>
</>
)}
</div>
);
}
ReactDOM.render(
<FragmentExample
// set isAdmin to false to see the other message
isAdmin={false}
/>,
document.getElementById("container")
);
Reasons React Fragments is must developers
Although every functionality you achieve with React Fragments can also be achieved via the generally used div element, in the long run integrating react fragments can be of great importance for developers. It provides you with amazing perks such as:
- React Fragment is a one-time use tag, unlike div which you need to use multiple times for rendering UI components. In a small-sized application, the difference it makes might not be much visible however for large-scale projects using react fragments would make your code much more manageable.
- Since react fragment helps you reduce the size of DOM markup making it easier for the browser to read your application, it also significantly improves the overall performance of your web applications.
- Also, React fragments reduce the lines of code you need to write for executing functionalities which eventually improves code readability.
- For specific parent-child structures divs can be a disruptive element to work with however react fragment disrupts the initial UI hierarchy and helps in rendering accurate UI for users.
Avoiding Common Pitfalls when working with React Fragments
React Fragments are a useful tool for grouping multiple elements together without adding an extra node to the DOM. However, working with fragments can lead to some common pitfalls if you're not careful. Here are some tips for avoiding those pitfalls:
- Fragments should have a unique key: When rendering a list of fragments, each fragment should have a unique key. This is important for React's reconciliation process, which uses the key to determine if a component should be updated, added, or removed.
- Avoid using too many nested fragments: While nesting fragments is possible, it can make your code more difficult to read and maintain. Try to avoid using too many nested fragments, and consider other approaches such as using arrays or higher-order components.
- Remember to add a key prop to mapped fragments: When using fragments with arrays, make sure to add a key prop to each mapped fragment. This is important for performance reasons, as it allows React to identify which elements have changed and need to be updated.
- Fragments can't have props or state: Fragments are not actual components, so they cannot have their own props or state. If you need to pass props to a fragment, you'll need to wrap it in a higher-order component or use a different approach.
- Fragments can be used with React Router: If you're using React Router, you can use fragments as children of route components. This is useful for grouping multiple components together without adding an extra node to the DOM.
- Use
<React.Fragment>
instead of<>
and</>
: While using the shorthand syntax for fragments can make your code more concise, it can also make it more difficult to read and maintain. Consider using the<React.Fragment>
component instead, which provides a clear indication of where your fragments begin and end.
Final Words on React Fragments
React Fragment is one of those features which you can implement in day-to-day coding tasks to improve the development experience. The feature not only helps you improve the overall speed of your project but also makes your codebase more manageable.
So try out the above-mentioned examples on react fragment today so you can develop a strong grasp of its concepts. Also to further advance your expertise you can even try fragments with dynamic UI components.
React Performance Monitoring with Atatus RUM
Visualize React errors and performance issues influencing your end-user experience. With React performance monitoring of Atatus you can identify and resolve problems faster with in-depth data points that assist you in analyzing and resolving them.
Using Atatus, you can get an overview of how your customers experience your React app. Learn how to identify the root cause of a slow load time, route change, and more on the front end by identifying performance bottlenecks in the front end.
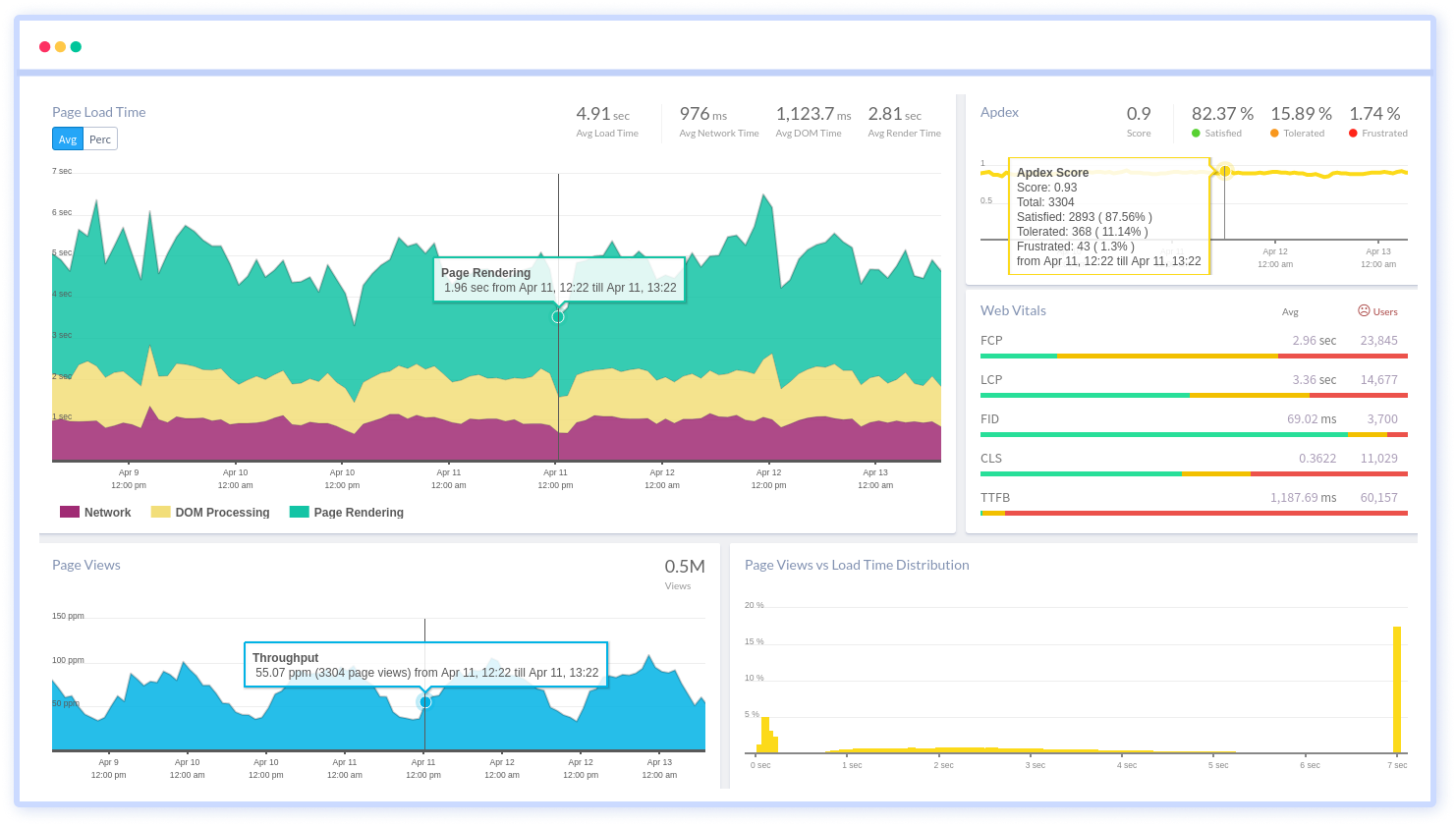
Ensure that your React app sends all XHR requests. Monitoring and measuring the response times and failure rates of XHR calls. Optimize AJAX request performance by identifying slow and failed calls. Analyze AJAX calls in real time based on the browser, the user, the version, the tag, and other attributes.
Identify the reasons behind bad front-end performance and slow page loading that are impacting your customers. Inspect individual users who are experiencing poor performance because of slow pages, React exceptions or a failing AJAX method.
Identify how your end users' experience is impacted by the network or geography. In addition to identifying slow React assets, long load times, and errors in sessions, session traces provide a waterfall view of the session.
Take a closer look at your React app's performance with Atatus. To get started activate Atatus free-trial for 14 days!
#1 Solution for Logs, Traces & Metrics
APM
Kubernetes
Logs
Synthetics
RUM
Serverless
Security
More