How to Display All PHP Errors: For Basic and Advanced Use
During the execution of a PHP application, it can generate a wide range of warnings and errors. For developers seeking to troubleshoot a misbehaving application, being able to check these errors is critical. Developers, on the other hand, frequently encounter difficulties when attempting to display errors from their PHP applications. Instead, their applications simply stop working.
First and foremost, you should be aware that with PHP, some errors prevent the script from being executed. Some errors, on the other hand, just display error messages with a warning. Then you'll learn how to use PHP to show or hide all error reporting.
In PHP, there are four types of errors:
- Notice Error – When attempting to access an undefined index in a PHP code, an error occurs. The execution of the PHP code is not halted by the notice error. It just alerts you to the fact that there is an issue.
- Warning Error – The most common causes of warning errors are omitting a file or invoking a function with improper parameters. It's comparable to seeing a mistake, but it doesn't stop the PHP code from running. It just serves as a reminder that the script has a flaw.
- Fatal Error – When undefined functions and classes are called in a PHP code, a fatal error occurs. The PHP code is stopped and an error message is displayed when a fatal error occurs.
- Parse Error – When a syntax issue occurs in the script, it is referred to as a parse error. The execution of the PHP code is also stopped when a parse error occurs, and an error message is displayed.
You've come to the right place if you're having troubles with your PHP web application and need to see all of the errors and warnings. We'll go through all of the different ways to activate PHP errors and warnings in this tutorial.
- To Show All PHP Errors
- .htaccess Configuration to Show PHP Errors
- Enable Detailed Warnings and Notices
- In-depth with the error_reporting() function
- Use error_log() Function to Log PHP Error
- Use Web Server Configuration to Log PHP Errors
- Collect PHP Errors using Atatus
1. To Show All PHP Errors
Adding these lines to your PHP code file is the quickest way to output all PHP errors and warnings:
ini_set ('display_errors', 1);
ini_set ('display_startup_errors', 1);
error_reporting (E_ALL);
The ini_set() function will attempt to override the PHP ini file's configuration.
The display_errors and display_startup_errors directives are just two of the options.
- The display_errors directive determines whether or not the errors are visible to the user. After development, the dispay_errors directive should usually be disabled.
- The display_startup_errors directive, on the other hand, is separate since display errors do not handle errors that occur during PHP's startup sequence.
The official documentation contains a list of directives that can be overridden by the ini_set() function. Unfortunately, parsing problems such as missing semicolons or curly brackets will not be displayed by these two directives. The PHP ini configuration must be changed in this scenario.
To display all errors, including parsing errors, in xampp, make the following changes to the php.ini example file and restart the apache server.
display_errors = on
In the PHP.ini file, set the display errors directive to turn on. It will show all errors that can't be seen by just calling the ini_set() function, such as syntax and parsing errors.
2. .htaccess Configuration to Show PHP Errors
The directory files are normally accessible to developers. The .htaccess file in the project's root or public directory can also be used to enable or disable the directive for displaying PHP errors.
php_flag display_startup_errors on
php_flag display_errors on
.htaccess has directives for display_startup_errors and display_errors, similar to what will be added to the PHP code to show PHP errors. The benefit of displaying or silencing error messages in this way is that development and production can have separate .htaccess files, with production suppressing error displays.
You may want to adjust display_errors in .htaccess or your PHP.ini file depending on which files you have access to and how you handle deployments and server configurations. Many hosting companies won't let you change the PHP.ini file to enable display errors.
3. Enable Detailed Warnings and Notices
Warnings that appear to not affect the application at first might sometimes result in fatal errors under certain circumstances. These warnings must be fixed because they indicate that the application will not function normally in certain circumstances. If these warnings result in a large number of errors, it would be more practical to hide the errors and only display the warning messages.
error_reporting(E_WARNING);
Showing warnings and hiding errors are as simple as adding a single line of code for a developer. The parameter for the error reporting function will be “E_WARNING | E_NOTICE” to display warnings and notifications. As bitwise operators, the error reporting function can accept E_ERROR, E_WARNING, E_PARSE, and E_NOTICE parameters.
For ease of reference, these constants are also detailed in the online PHP Manual.
To report all errors except notices, use the option "E_ALL & E_NOTICE," where E_ALL refers to all of the error reporting function's potential parameters.
4. In-depth with the error_reporting() Function
One of the first results when you browser "PHP errors" is a link to the error_reporting() function documentation.
The PHP error_reporting() function allows developers to choose which and how many errors should be displayed in their applications. Remember that this function will set the error reporting directive in the PHP ini configuration during runtime.
error_reporting(0);
The value zero should be supplied to the error_reporting() function to delete all errors, warnings, parse messages, and notices. This line of code would be impractical to include in each of the PHP files. It is preferable to disable report messages in the PHP ini file or the .htaccess file.
error_reporting(-1);
An integer value is also passed as an input to the error_reporting() function. In PHP, we may use this method to display errors. In PHP, there are many different types of errors. All PHP errors are represented by the -1 level. Even with new levels and constants, passing the value -1 will work in future PHP versions.
error_reporting(E_NOTICE);
Variables can be used even if they aren't declared in PHP. This isn't recommended because undeclared variables can create issues in the application when they're utilized in loops and conditions.
This can also happen when the stated variable is spelled differently than the variable used in conditions or loops. These undeclared variables will be displayed in the web application if E_NOTICE is supplied in the error_reporting() function.
error_reporting(E_ALL & ~E_NOTICE);
You can filter which errors are displayed using the error_reporting() function. The “~” character stands for “not” or “no,” hence the parameter ~E_NOTICE indicates that notices should not be displayed.
In the code, keep an eye out for the letters "&" and "|." The “&” symbol stands for “true for all,” whereas the “|” character stands for “true for either.” In PHP, these two characters have the same meaning: OR and AND.
5. Use error_log() Function to Log PHP Error
Error messages must not be displayed to end-users during production, but they must be logged for tracing purposes. The best approach to keep track of these error messages in a live web application is to keep track of them in log files.
The error_log() function, which accepts four parameters, provides a simple way to use log files. The first parameter, which contains information about the log errors or what should be logged, is the only one that must be provided. This function's type, destination, and header parameters are all optional.
error_log("Whoosh!!! Something is wrong!", 0);
If the type option is not specified, it defaults to 0, which means that this log information will be appended to the web server's log file.
error_log("Email this error to Justin!", 1, "Justin@mydomain.com");
The error logs supplied in the third parameter will be emailed using the type 1 parameter. To use this feature, the PHP ini must have a valid SMTP configuration to send emails.
Host, encryption type, username, password, and port are among the SMTP ini directives. This kind of error reporting is recommended for logging or notifying errors that must be corrected as soon as possible.
error_log("Write this error down to a file!", 3, "logs/php-errors.log");
Type 3 must be used to log messages in a different file established by the web server's configuration. The third parameter specifies the log file's location, which must be writable by the webserver. The log file's location might be either a relative or absolute library to where this code is invoked.
You can also refer to the error_log() function documentation to know more.
6. Use Web Server Configuration to Log PHP Errors
The ideal technique to record errors is to define them in the web server configuration file, rather than modifying parameters in the .htaccess or adding lines in the PHP code to show errors.
ErrorLog "/var/log/apache2/my-website-error.log"
These files must be added to the virtual host of a specific HTML page or application in Apache, which is commonly found in the sites-available folder in Ubuntu or the httpd-vhosts file in Windows.
error_log /var/log/nginx/my-website-error.log;
The directive is just called error_log() in Nginx, as it is in Apache. The log files for both Apache and Nginx web servers must be writable by the webserver. Fortunately, the folders for the log files of these two web servers are already writable after installation.
7. Collect PHP Errors using Atatus
Atatus is an Application Performance Management (APM) solution that collects all requests to your PHP applications without requiring you to change your source code. However, the tool does more than just keep track of your application's performance. Atatus' ability to automatically gather all unhandled errors in your PHP application is one of its best features.
To fix PHP exceptions, gather all necessary information such as class, message, URL, request agent, version, and so on. Every PHP error is logged and captured with a full stack trace, with the precise line of source code marked, to make bug fixes easy.
Read the guide to know more about PHP Performance Monitoring.
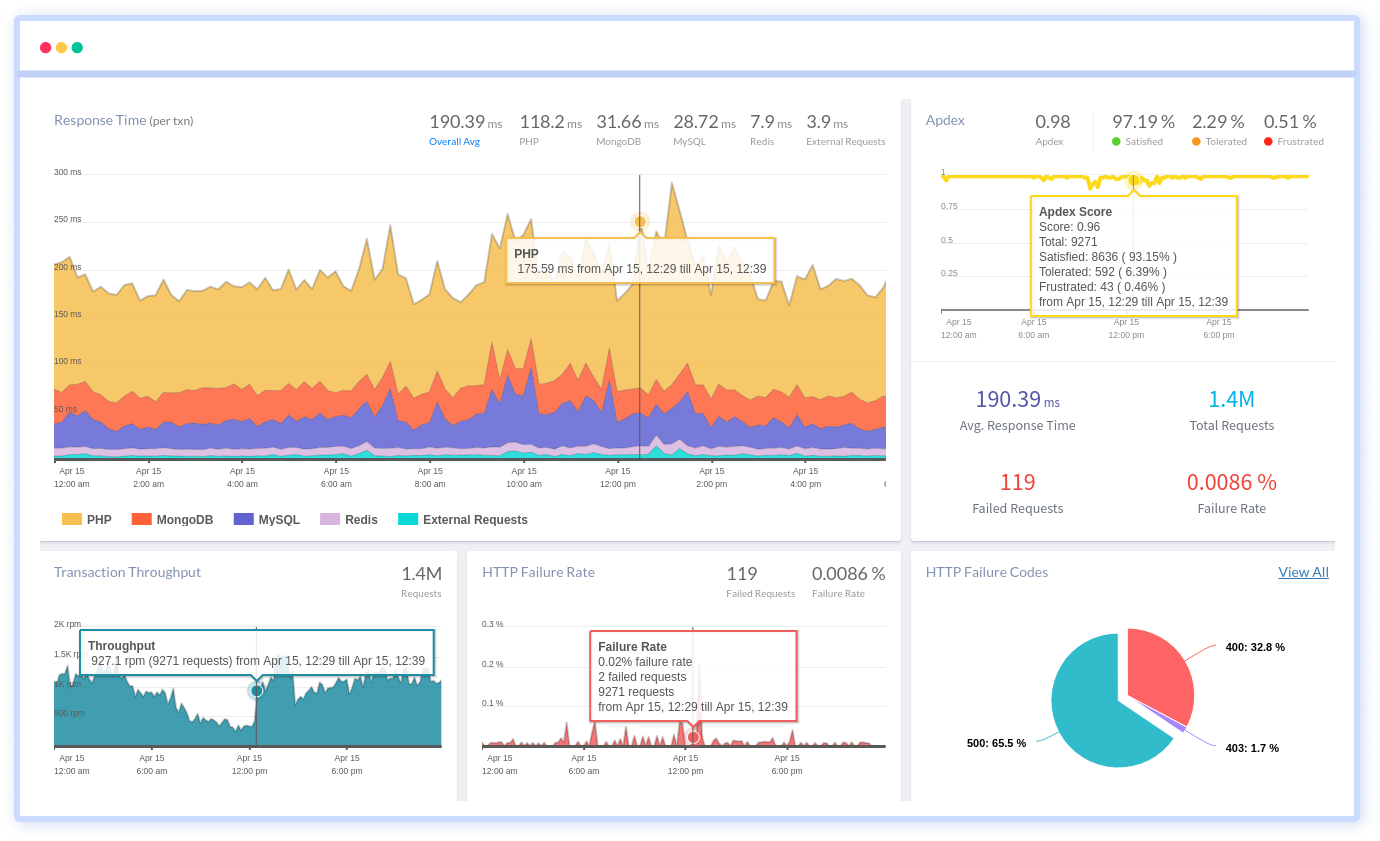
All errors are automatically logged and structured in Atatus so that they can be easily viewed. Not only will Atatus show you what errors have happened, but it will also examine where and why they occurred. The logs also display the time and number of occurrences, making it much easier to focus on which issue to address.
Start your 14-day free trial of Atatus, right now!!!
Summary
When there is an issue with the PHP code, a PHP error occurs. Even something as simple as incorrect syntax or forgetting a semicolon can result in an error, prompting a notification. Alternatively, the cause could be more complicated, such as invoking an incorrect variable, which can result in a fatal error and cause your system to crash.
This tutorial has shown you several ways to enable and display all PHP errors and warnings. You can boost your ability to debug PHP issues by receiving error notifications fast and precisely.
Let us know what you think about displaying PHP errors in the below comment section.
#1 Solution for Logs, Traces & Metrics
APM
Kubernetes
Logs
Synthetics
RUM
Serverless
Security
More