PHP Exception Handling Using Try Catch: For Basic and Advanced Use
An error is unexpected data generated by a computer program that the computer program cannot handle. PHP Error Handling is a single-step technique for catching all errors generated by your primary computer program and taking suitable action.
Error handling in PHP with try-catch blocks is remarkably similar to error handling in other programming languages. The PHP runtime looks for a catch statement that can handle a PHP exception when it is thrown. It will keep inspecting the calling methods all the way up the stack trace until it finds a catch statement.
As you read, you will learn more about PHP Exception Handling:
- What is Exception Handling?
- PHP Exception Handling
- PHP Exception Handling Keywords
- How to Implement Exception Handling?
- When Should You Use try-catch-finally?
- Creating Custom PHP Exception Types
- Global Exception Handler
- Handling Multiple Exception
- Re-throwing Exceptions
- How to Log Exceptions in Your PHP try-catch Blocks?
- How to Use try-catch with MySQL?
- View All PHP Exceptions in One Place
#1 What is Exception Handling?
Exception handling is the process of making accommodations for errors and responding to them programmatically, which results in the execution of an alternate, but previously planned, sequence of code. It can be found in a variety of places, including software, hardware, and electrical systems, such as the motherboard of your computer.
Invalid form inputs, erroneous programming logic, network errors, software compatibility issues, unauthorized access, and a lack of memory, among other things, can cause problems on the software side.
Exception handling helps developers debug and users better grasp an application's needs by resolving conflicts via error messages. Good Exception practices protect users from a bad user experience while also assisting developers in determining what went wrong with the application.
PHP, like many other programming languages, contains some built-in exceptions such as ArgumentCountError, ArithmeticError, DivisionByZeroError, CompileError, ParseError, and TypeError. Exception handling systems, on the other hand, can be utilized to generate problem-specific constraints. If the requirements aren't followed, they will result in exceptions that can be handled by programming.
#2 PHP Exception Handling
When an error occurs, PHP displays an error message in the web browser with information about the error that happened, depending on your configuration settings.
Errors can be handled in a variety of ways in PHP. We'll look at three ways that are often used:
- Die Statements
The echo and exit functions are combined in the die function. When we want to produce a notice and stop the script execution when an error occurs, it's quite handy. - Custom Error Handlers
When an error occurs, these are user-defined functions that are invoked. - PHP Error Reporting
Depending on your PHP error reporting settings, the error message will be different. When you don't know what caused the error, this solution comes in handy in the development environment. The data given can assist you in debugging your application.
#3 PHP Exception Handling Keywords
For PHP exception handling, the following keywords are utilized.
Try – The code that could potentially throw an exception is contained in the try block. Until an exception is thrown, the code in the try block is performed in its entirety.
Throw – The throw keyword is used to indicate that a PHP exception has occurred. After that, the PHP runtime will look for a catch statement to handle the exception.
Catch – Only if an exception occurs within the try code block will this block of code be called. The exception thrown must be handled by the code in your catch statement.
Finally – The "finally" statement was added in PHP 5.5. Finally, instead of or in addition to "catch" blocks, the "finally" block can be specified. Regardless of whether an exception has been thrown, code in the "finally" block will always be run after the try and catch blocks and before normal execution resumes. This is handy in situations where you want to close a database connection regardless of whether or not an exception occurred.
#4 How to Implement Exception Handling?
Exception handling mechanisms such as 'try,' 'throw,' and 'catch' is widely used.
Try
The try block is used to include code that is likely to result in an error. Any erroneous condition that happens in the code within a try block can be addressed programmatically.
<?php
try {
// code likely to give error comes in this block
}
?>
Throw
Exceptions can be raised either automatically by the programming language as a result of syntactical errors and warnings, or manually by code when a condition is or isn't met. Throwing is the term used in programming to describe the act of raising errors. Only from within the try block can errors be thrown manually, as shown below:
<?php
try {
throw new Exception("Something breaks. Please fix it!");
}
?>
The throw keyword is used to create an instance of the exception class with a string that describes the exception that was thrown. Any code in the try block that comes after the throw call is not performed because program control is transferred to the catch block, which is where the exception is handled.
Catch
Let's look at how exceptions are dealt with or managed now that we know where and how they can be thrown. To catch an exception, use the proper terminology. After the try scope, catch procedures are declared that take an instance of the exception class as a parameter.
<?php
try {
// if some condition is met or not met
throw new Exception("Error Message!!!");
// below lines are not executed
echo "I am not executed!";
} catch(Exception $e) {
echo "\n Exception caught - ", $e->getMessage();
}
?>
#5 When Should You Use try-catch-finally?
You may wish to utilize a "finally" section in your PHP error handling code. Finally, it's used for more than simply managing exceptions; it's also used to run cleaning code like closing a file, closing a database connection, and so on.
When the try-catch block finishes, the "finally" block is always executed.
Example:
try {
print "this is our try block n";
throw new Exception();
} catch (Exception $e) {
print "something went wrong, caught yah! n";
} finally {
print "this part is always executed n";
}
The program's operation is illustrated in the diagram below.
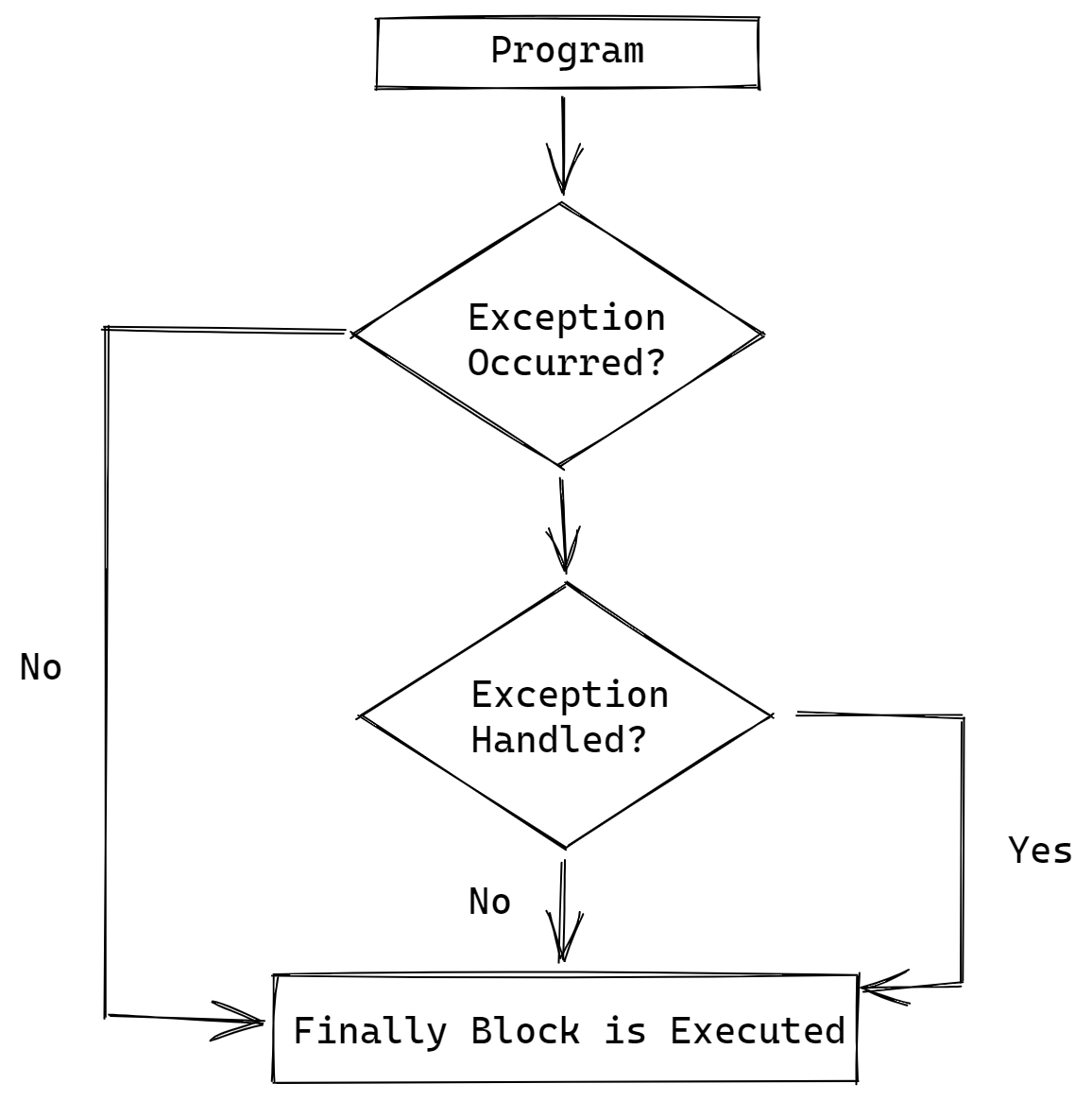
#6 Creating Custom PHP Exception Types
Custom exception types are also possible in PHP. This can be useful for defining custom exceptions in your application, which you can then handle differently.
We must construct a new class with functions that can be called when an exception occurs in order to develop a custom exception handler. The class must be an exception class extension.
You can add custom functions to the custom exception class, which inherits properties from PHP's Exception class. You may not want to show the user all of the details of an exception; instead, show a user-friendly message and log the error message internally for monitoring.
A custom PHP exception is used in the following example:
<?php
// custom exception class
class DivideByZeroException extends Exception {
// define constructor
function __construct() {
// we can even take arguments if we want
}
// we can define class methods if required
}
?>
The DivideByZeroException() and DivideByNegativeException() classes are extensions of the current Exception class, inheriting all of the Exception class's methods and properties.
#7 Global Exception Handler
There's a good chance you didn't account for the potential of an exception. To be on the safe side, it's a good idea to create a global, top-level function that handles any unexpected exceptions. This method will help you in dealing with exceptions not covered by try blocks.
The set_exception_handler function in PHP allows us to define such an exception handler, which takes as an argument the name of the function that is responsible for catching an unexpected exception:
function our_global_exception_handler($exception) {
// This code should log the exception to console and an error tracking system
echo "Exception:" . $exception->getMessage();
}
set_exception_handler(‘our_global_exception_handler’);
#8 Handling Multiple Exception
To handle thrown exceptions, multiple exceptions utilize numerous try-catch blocks. Multiple exceptions is a good idea when
- You'd like to show a customized message based on the exception that was thrown
- You wish to run a unique operation based on the exception thrown
In simple terms, if a piece of code can throw several types of exceptions and we need to do different actions depending on the type of exception, we can have multiple catch blocks.
<?php
try {
// calling the function
triggerException();
} catch (StudytonightException $e) {
// do something here...
} catch (Exception $e) {
echo "Oops! Some unexpected error occured...";
}
?>
When handling multiple exceptions with several catch blocks, there are a few things to keep in mind:
- The catch block for the Exception class's child class must be positioned above the catch block for the Exception class. Exception class handling catch block, in other words, should be placed last.
- Since all exception classes are child classes of the Exception class, the catch block handling the Exception class can also handle other exceptions.
The flowchart below shows how multiple exceptions are handled.
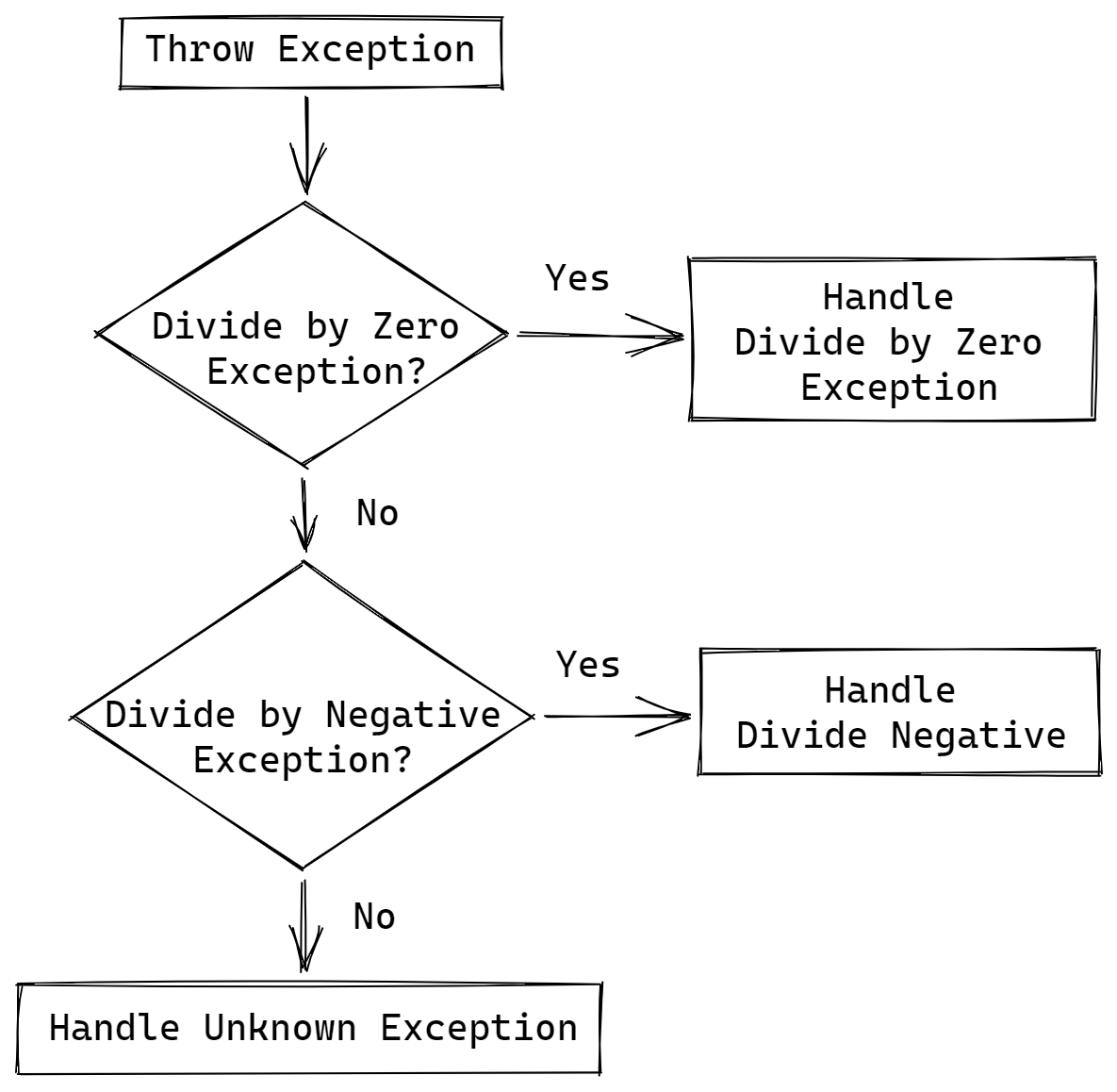
#9 Re-throwing Exceptions
When an exception is thrown, you might want to handle it in a different way than usual. Within a "catch" block, it is possible to throw an exception a second time.
Users should not be aware of system errors if a script is used. System errors may be significant to programmers, but they are uninteresting to users. You can re-throw the exception with a user-friendly message to make things easy for the user.
<?php
try {
try {
// throw exception
throw new Exception($e);
} catch(Exception $e) {
// re-throw exception
throw new customException($e);
}
} catch (customException $e) {
// display custom message
echo $e->errorMessage();
}
?>
#10 How to Log Exceptions in Your PHP try-catch Blocks?
During development, error logs are essential because they allow developers to observe warnings, errors, alerts, and other events that occurred while the application was operating. That you can use the PHP exception handling try-catch method to appropriately handle them.
Depending on the PHP framework you're using, such as Laravel, Codeigniter, Symfony, or others, built-in logging frameworks may be available. Monolog, a standard PHP logging package, is another option. You should always log essential exceptions thrown in your code, regardless of the logging system you're using.
Here's a sample of a Monolog try/catch that reports errors:
require_once(DIR.'/vendor/autoload.php');
use MonologLogger;
use MonologHandlerStreamHandler;
$logger = new Logger('channel-name');
$logger->pushHandler(new StreamHandler(DIR.'/app.log', Logger::DEBUG));
try {
// Code does some stuff
// debug logging statement
$logger->info('This is a log!');
} catch (Exception $e) {
$logger->error('Oh no an exception happened! ');
}
#11 How to Use try-catch with MySQL?
Error handling is handled differently in the PHP libraries for MySQL, PDO, and mysqli. You can't use try-catch blocks if you don't have exceptions enabled for those libraries. This makes error handling unique and possibly more difficult.
PDO
When making a connection in PDO, you must activate ERRMODE_EXCEPTION.
ERRMODE_EXCEPTION is the default mode as of PHP 8.0.0. PDO will throw a PDOException and adjust its attributes to reflect the error code and details about the error in order to update the error code. This feature is especially handy while debugging because it literally blows the script at the point of the error, pointing a finger at potential trouble spots in your code very fast. If the script crashes due to an exception, the transactions are automatically rolled back.
// connect to MySQL
$conn = new PDO('mysql:host=localhost;dbname=atatusdb;charset=utf8mb4', 'username', 'password');
//PDO error mode to exception
$conn->setAttribute(PDO::ATTR_ERRMODE, PDO::ERRMODE_EXCEPTION);
MySQL
You must do something similar for mysqli too:
mysqli_report(MYSQLI_REPORT_ERROR | MYSQLI_REPORT_STRICT);
#12 View All PHP Exceptions in One Place
In PHP, proper exception handling is critical. You don't want to just log your exceptions to a log file and never know they happened as part of that.
Use an error tracking tool like Atatus as a solution. All errors are logged and captured together with key information about them, such as class, message, URL, request agent, version, and so on.
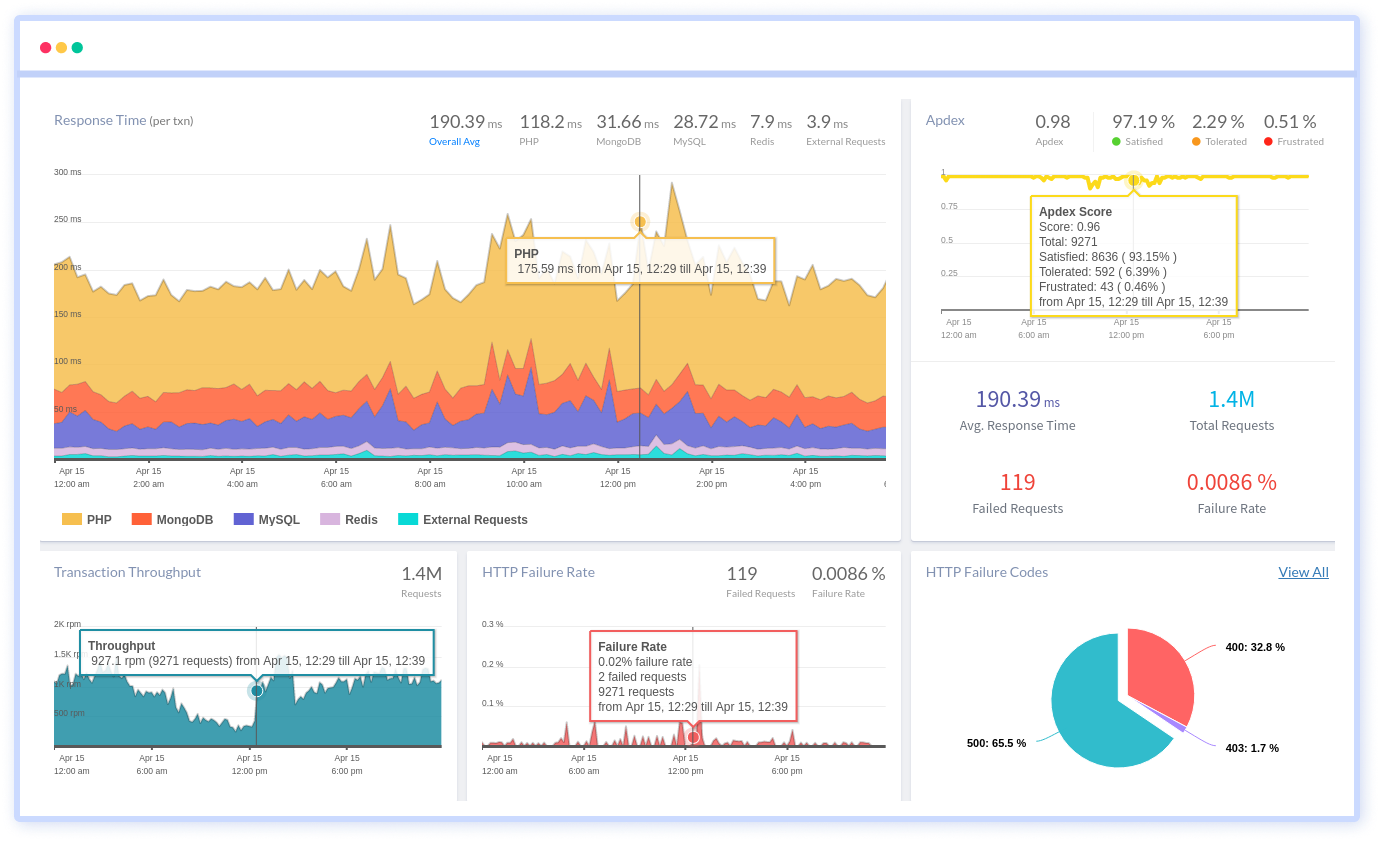
In Atatus, all errors are automatically logged and organized so they may be easily accessed. Atatus will not only show you what errors have occurred, but it will also investigate where and why they happened. The timing and number of incidents are also displayed in the logs, making it much easier to pinpoint which issue needs to be addressed first.
Error tracking and monitoring tools are included in Atatus. Among the features is the ability to see all errors in one location, the ability to identify unique errors, the ability to locate new errors faster after a deployment, email notifications about errors, and more.
The error and log management solution from Atatus can help you in easily monitoring and troubleshooting your application.
Wrapping It
We realized that it's necessary to be prepared for unexpected scenarios that require exceptions at all times. To do this, we can wrap our code in try blocks, have proper catch methods to deal with thrown errors, and a global exception handler to deal with anything else. Now that you've gathered all of the necessary information, go ahead and make your own exceptions!
Also, do share what you think of this article with us in the below comment section.
#1 Solution for Logs, Traces & Metrics
APM
Kubernetes
Logs
Synthetics
RUM
Serverless
Security
More