HTTP Status Code to Use for CRUD Operation
When responding to our clients, we can utilize various status codes defined by the HTTP protocol. Others wish to fully utilize HTTP's library of codes to inform their clients of any issues; some APIs simply use the most fundamental codes and develop their own error-signaling systems on top of them.
This tutorial explains the CRUD actions and the status codes you should employ for a clean API design.
We will cover the following:
What is CRUD?
At most, models should be able to perform the four fundamental operations of Create, Read, Update, and Delete (CRUD).
We want our models to offer four fundamental forms of functionality when we are creating APIs. The model has to provide Create, Read, Update, and Delete operations on resources. These operations are frequently referred to by the abbreviation CRUD by computer scientists.
For a model to be complete, it must be able to accomplish no more than these four tasks. An action should potentially have its own model if it cannot be explained by one of these four operations.
The CRUD concept is frequently used when building web applications because it offers a simple foundation that developers can refer to when they forget how to build complete, useable models.
Imagine a system that records information about employees, for instance. We can envision that there would be an employee details database in which employee objects would be stored.
Say the employee object has the following appearance:
"employee": {
"id": "701",
"position": "Lead",
"dept": "Marketing",
"mob_no": "9876543210"
}
We would want to make sure that there were explicit procedures for carrying out the CRUD operations to make this employee detail system usable:
- Create
A function would be called in this case whenever a new employee detail is added to the database. The values for "position," "dept," and "mob_no" would be supplied by the application that called the function. When this function is used, a new entry for this new employee should appear in the employee information. Additionally, a unique id is given to the new entry, which can be used to access this resource in the future. - Read
This would entail calling a method to view every employee currently stored in the database. This function call would only obtain the resource and display the results, not change the employee information stored in the database. The ability to access a single employee's details would also be available, and we could enter the employee's position, department, or mobile number. Once more, only retrieval would be done with this employee detail. - Update
When information about an employee needs to be modified, a function should be available to call. The function would get the updated values for "position," "dept," and "mob_no" from the calling program. The employee resource entry corresponding to the function call would include the updated fields afterward. - Delete
An employee detail should be able to be deleted from the database by using a certain function. The employee would be identified by the program invoking the function using one or more values (such as "position," "dept," and/or "mob_no"), and would then be deleted from the employee resource. The employee resource should have all the employee details it had before, except the one that was just deleted, after calling this function.
HTTP Status Codes
An HTTP status code is the outcome of a server's response to a browser's request. When you visit a website, your browser sends a request to the server, which response with a three-digit code known as the HTTP status code.
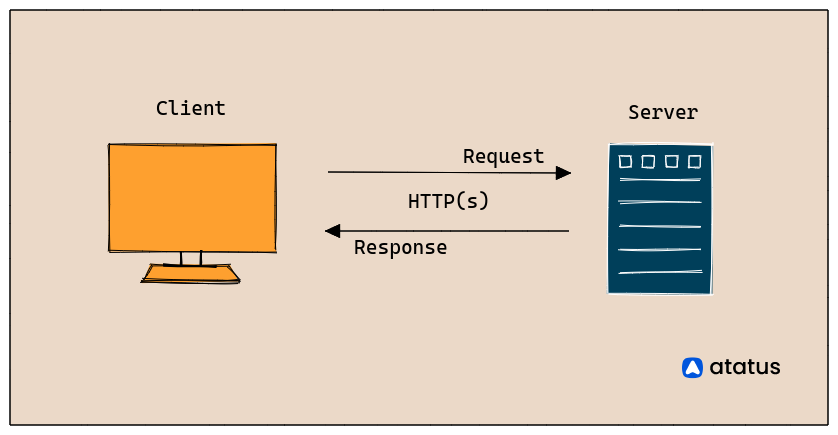
These status codes represent a conversation between your browser and the server on the internet. They communicate when anything is amiss, when things are touched and go, or when everything is fine between the two.
You can diagnose site issues quickly to reduce downtime by understanding status codes and knowing how to use them. Some of these status codes can even be used to facilitate website access for users and search engines.
A 301 redirect, for instance, notifies users and search engines that a page has relocated permanently. Each three-digit status code's first digit starts with one of the five integers from 1 to 5, and you might see this stated as 1xx or 5xx to denote status codes in that range.
Each of the bands includes a certain type of server response:
- 1xxs (Informational responses)
The request is being considered by the server. - 2xxs (Success!)
The request was successfully processed by the server, and the browser received the required result. - 3xxs (Redirection)
You were taken to another location. Even if the request was received, there was some form of rerouting. - 4xxs (Client errors)
Page not found. The website or page was inaccessible. (Although the page is invalid, the request was made. This is an error on the website's end of the discussion and frequently occurs when a page doesn't exist on the site.) - 5xxs (Server errors)
Failure. Although the client's request was valid, the server was unable to accommodate it.
HTTP Status for CRUD Operation
The simplest API actions for persistent storage are defined by the CRUD concept. Create, read, update, and delete. They account for the majority of API endpoints. Let's see which status codes satisfy their demands.
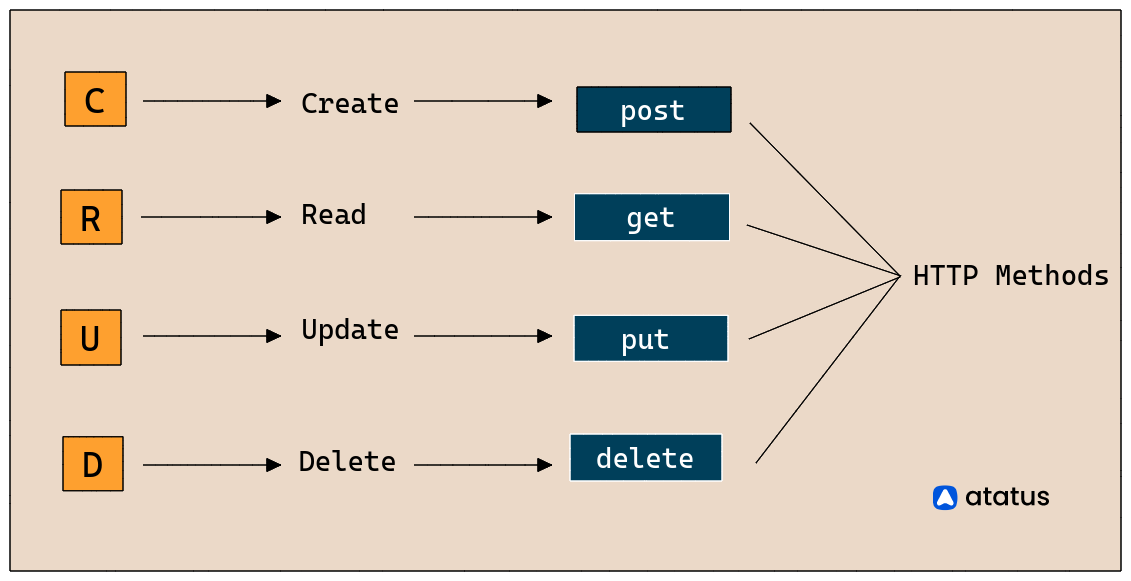
CREATE
The HTTPs POST method is typically used to implement the create action. These endpoints are used to create new resources or access tokens in RESTful APIs.
How does it function?
The CREATE operation in CRUD accomplishes what its name suggests. It entails entering data. A task, a post, a user profile, or an account could all be included in this entry.
As we mentioned previously, the POST method of the HTTP protocol supports a CREATE action.
To construct in a SQL database is to INSERT. With the insert() method, you can construct a NoSQL database like MongoDB.
Status Code for CREATE
- 200 (OK)
It’s the basic status code to tell the client everything went well. We may use the status code 200 to create an access token because we don't generate any endpoint-accessible resources. - 201 (Created)
The one that works best for CREATE operations. This code should indicate the construction of a backend-side resource and be accompanied by a Location header specifying the most precise URL for the newly created resource. Additionally, it's a good idea to provide in the response body an acceptable representation of the resource or at least one or more URLs to that resource. - 202 (Accepted)
It is commonly utilized for asynchronous processing. The client is informed by this code that although the request was valid, processing will take some time to complete. An URL to the final resource with some information about when it will be accessible should be included in the response body, as should a URL to a monitoring endpoint that notifies the client when the resource is ready. - 303 (See Other)
Similar to the 202 code, but with the addition of a Location header field to inform the client of the resource's location or an endpoint that enables the client to verify the status of the creation process. Some clients automatically follow the status codes of the Redirect class. Only POST requests typically make use of this code.
READ
The read action uses the HTTPs GET method to implement it and is used to retrieve resource representations. The reason for asynchronous processing is frequently that the resource doesn't yet exist and must be produced, thus it's a CREATE action anyhow, therefore asynchronous responses aren't much of an issue here.
How does it function?
Getting access to inputs or entries in the UI is what the READ operation entails. I.e., noticing it. Once more, the entry might consist of anything, such as user information or posts from social media.
This access could refer to the user having immediate access to newly created entries or looking for them. The usage of searching enables users to eliminate entries they don't require.
The GET method of the HTTP protocol provides a READ action. To read an entry in a SQL database is to do a SELECT. The find() or findById() method is used to read data from a NoSQL database like MongoDB.
Status Code for READ Action
- 200 (OK)
The majority of read activities will return a 200 OK status. - 206 (Partial Content)
This code is helpful for lists of content that are too lengthy to be given in a single response. The request must include a Range header field to use it. Although the unit can be freely assigned as long as both parties understand it, this header field typically specifies the byte range the backend should deliver to the client. - 300 (Multiple Choices)
If a resource has many representations and the client (or its user) must select one of them, this redirect is utilized. - 308 (Permanent Redirect)
This instructs the client to stop using the current URL and instead use a different one to access the resource. It is useful when we have several endpoints for a single resource but do not want to implement reading from every one of them. - 304 (Not Modified)
The client will be directed to its locally cached representation from a previous read because it is utilized similarly to 200 but without a body. - 307 (Temporary Redirect)
If a resource's URL is subject to change in the future, clients should always request the most recent URL before accessing the actual one.
UPDATE
Using the HTTP PUT or PATCH methods, an update can be implemented. The quantity of data the client must send to the backend differs.
How does it function?
The operation that enables you to change already-existing data is UPDATE. In other words, data editing.
Contrary to READ, the UPDATE operation modifies already-existing data. Depending on your needs, you can implement an UPDATE operation using either the PUT or PATCH HTTP protocols.
When updating a complete record, PUT should be used, and PATCH should be used if only part of the entry has to be changed.
- To update a resource using PUT, the client must submit the full representation of the resource. (Instead of the current one, use the new one.)
- PATCH simply requires the client to submit a portion of the resource's representation to update the resource. (Add, update, or delete these parts in the earlier version.)
You use UPDATE to update an entry in a SQL database. The findByIdAndUpdate() method in a NoSQL database like MongoDB can be used to build an updating feature.
Status code for Update
- 200 (OK)
For the majority of use cases, this is the most suitable code. - 204 (No Content)
It is a suitable code for updates that don't provide data to the client, such as when just saving a document that is being modified. - 202 (Accepted)
This code may be applied if the update is carried out asynchronously. An URL to the updated resource or a URL to check whether the update was successful should be included. It may also contain an estimation of the update's duration.
DELETE
The HTTP DELETE method can be used to implement the delete action.
How does it function?
A record is deleted when it is taken out of the database and user interface. The HTTP protocol for carrying out a DELETE action is called DELETE.
DELETE is the command used to remove an entry from a SQL database. The findByIdAndDelete() method can be used to implement delete in a NoSQL database like MongoDB.
Status code for Delete
- 200 (OK)
Some people believe that any delete function should return the deleted element so that the response body can contain a representation of the deleted element. - 204 (No Content)
The status code that best describes this situation. It's preferable to minimize bandwidth, inform the client that the deletion has been completed, and not return any response body (as the resource has been deleted). - 202 (Accepted)
It could be appropriate to return this code along with some information or a URL to alert the client when it will be erased if the deletion is asynchronous and takes some time, as is the case with distributed systems.
API Changes
If our API is around for a long enough time, it will eventually change how it is organized. Avoiding breaking changes is great practice, and the redirection class of status codes can assist with this since certain clients automatically follow their Location header.
Status Codes for API Changes
- 307 (Temporary Redirect)
If the resource might become available at a different URL in the future, but we want the current endpoint to decide where the client is redirected to, then this is the correct code to use. Every time a request is made using this status code, the client can return to the current URL. - 308 (Permanent Redirect)
If the resource will now be accessible at a new URL and the client should directly access it via the new URL in the future, this is the correct code. If the resource URL changes again, a subsequent redirect will need to be sent from the new URL as the current endpoint cannot influence the behavior of the clients following the request.
Conclusion
This article showed you what CRUD means and the status codes for CRUD operation. When developing HTTP, its developers considered a variety of status codes and even included a ton of brand-new ones over time.
They can dramatically enhance the developer experience if used properly by utilizing automatic client redirect follows and providing clearer explanations of what happened.
Although there may be numerous codes that we could employ in a given situation, we must maintain consistency in our usage across the entire API surface.
Atatus API Monitoring and Observability
Atatus provides Powerful API Observability to help you debug and prevent API issues. It monitors the consumer experience and is notified when abnormalities or issues arise. You can deeply understand who is using your APIs, how they are used, and the payloads they are sending.
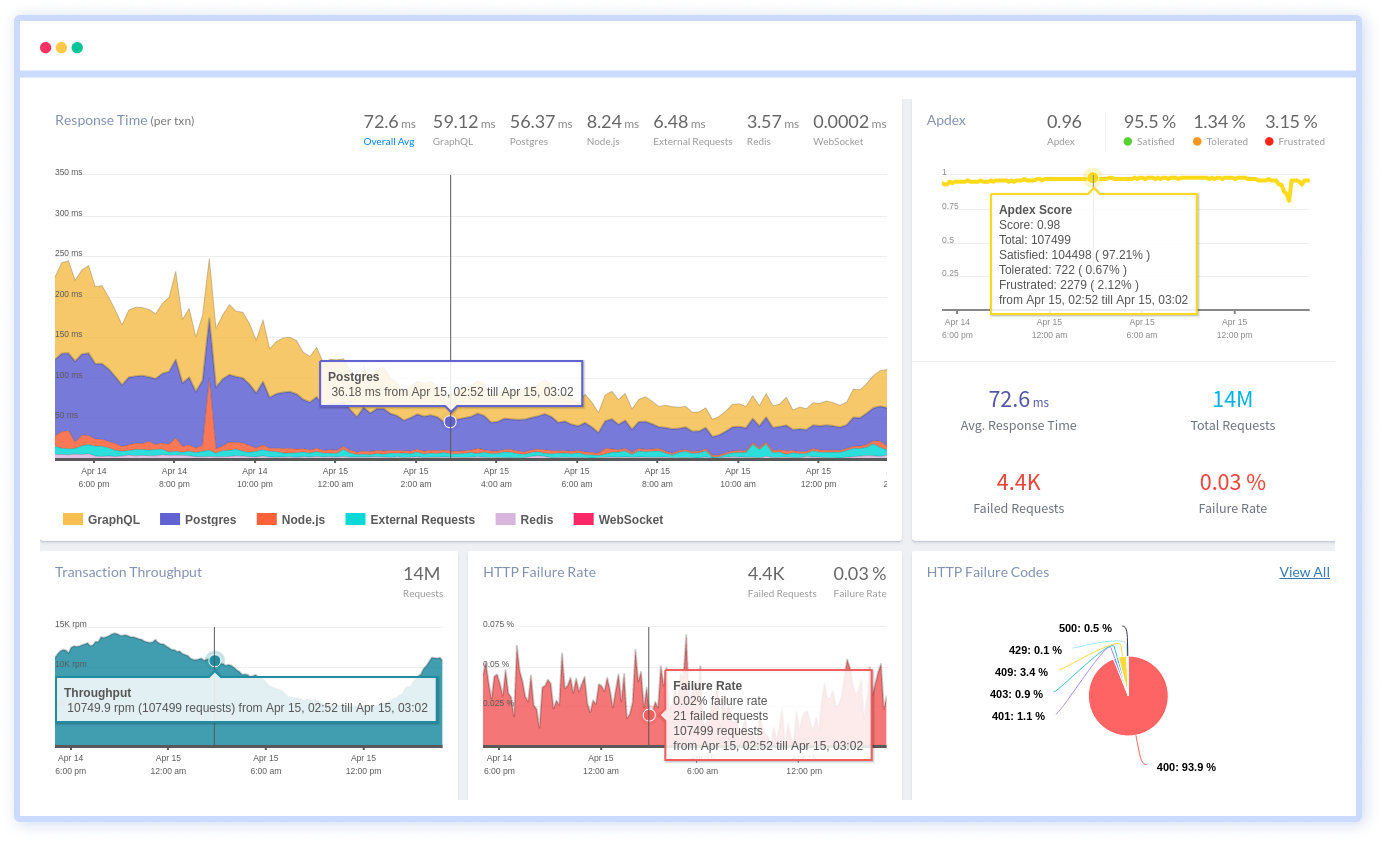
Atatus's user-centric API observability tracks how your actual customers experience your APIs and applications. Customers may easily get metrics on their quota usage, SLAs, and more.
It monitors the functionality, availability, and performance data of your internal, external, and third-party APIs to see how your actual users interact with the API in your application. It also validates rest APIs and keeps track of metrics like latency, response time, and other performance indicators to ensure your application runs smoothly.
#1 Solution for Logs, Traces & Metrics
APM
Kubernetes
Logs
Synthetics
RUM
Serverless
Security
More