Welcome to our exploration of Java threads—a fascinating feature that let your computer multitask like a pro!
Threads are like mini-workers inside your computer, each handling a different job at the same time. Normally, your computer works on one task at a time. But with threads, it can tackle multiple tasks concurrently, making things faster and more efficient. Threads can speed up your programs and make them work better.
But wait, there's a twist! With great power comes great complexity. Threads can be unpredictable sometimes. This unpredictability arises due to the concurrent nature of threads.
So in this blog, we will understand these intricacies and learn how to guide threads seamlessly, creating efficient and reliable software.
Let's get started!
Table of Contents
- Multitasking in Java
- Threads in Java
- Thread Priority
- Thread Lifecycle
- How to Generate a Thread in Java?
- Methods of Thread Class
- Challenges and Solutions
Multitasking in Java
Multitasking in Java refers to the ability to execute multiple task simultaneously. Multitasking is achieved through the use of threads. As we already know, threads are separate paths of execution within a single program, allowing multiple tasks to run concurrently.
There are two distinct methods for achieving concurrent execution: process-based multitasking and thread-based multitasking. Let's examine each approach individually,
1. Process-Based Multitasking
In process-based multitasking, every active application or program is considered a distinct process. The process is an independent entity with its own space for memory, system resources and execution context. Each of these process has its own protected memory space, which prevents one process from directly interfering with another.
2. Thread-Based Multitasking
In thread-based multitasking, a single program can have multiple threads of execution. These threads use the same memory and resources within the program, making communication between them quicker and smoother than communicating between separate programs. Threads in a program can work together or handle different tasks simultaneously, like a team working together.
Threads in Java
In Java, a thread is a fundamental unit of execution that operates independently within a process. Threads enable simultaneous execution of tasks, allowing multiple sequences of instructions to run concurrently and potentially in parallel.
Threads are created using the java.lang.Thread
class, which handles the process of making and controlling threads, as well as ensuring they work together smoothly.
Different Types of Java Thread
In Java, there are mainly two types of threads,
- User threads
- Daemon threads
User Threads: User threads are regular threads that are created by the application to perform specific tasks. They continue running even if the main program has finished, and the program won't exit until all user threads have completed their execution.
Thread userThread = new Thread(() -> {
// Task to be performed by the user thread
});
userThread.start();
Daemon Threads: Daemon threads are background threads that provide support to user threads. They are terminated automatically when all user threads have finished executing. They are used for non-essential tasks that can be abandoned if the main program ends.
Thread daemonThread = new Thread(() -> {
// Task to be performed by the daemon thread
});
daemonThread.setDaemon(true);
daemonThread.start();
In both the examples, the tasks defined within the thread run concurrently with the main program. With user threads, the main program waits until those threads finish their work before it ends. Daemon threads, on the other hand, might stop working if the main program finishes its tasks.
Thread Priority
In Java, thread priority means giving a number to a task to show how important it is for the computer to handle. Threads with higher priority values are generally given preferential treatment in terms of CPU time compared to threads with lower priority values.
Java provides thread priority values as integers ranging from 1 to 10, where 1 is the lowest priority and 10 is the highest. Threads are assigned a default priority value of 5.
You can set a thread's priority using the setPriority(int priority)
method provided by the Thread class.
Thread thread = new Thread(new MyRunnable());
thread.setPriority(Thread.MAX_PRIORITY);
thread.start();
However, it is important to understand that thread priority doesn't guarantee precise execution order and can be influenced by various factors, including the operating system's scheduling algorithm and the overall system load.
Thread Lifecycle
The thread lifecycle in Java outlines the various stages a thread undergoes while being active within a program. These stages delineate the trajectory of a thread from its instantiation to its termination. Java, as a programming language, establishes a set of clearly defined thread states that encompass this progression.
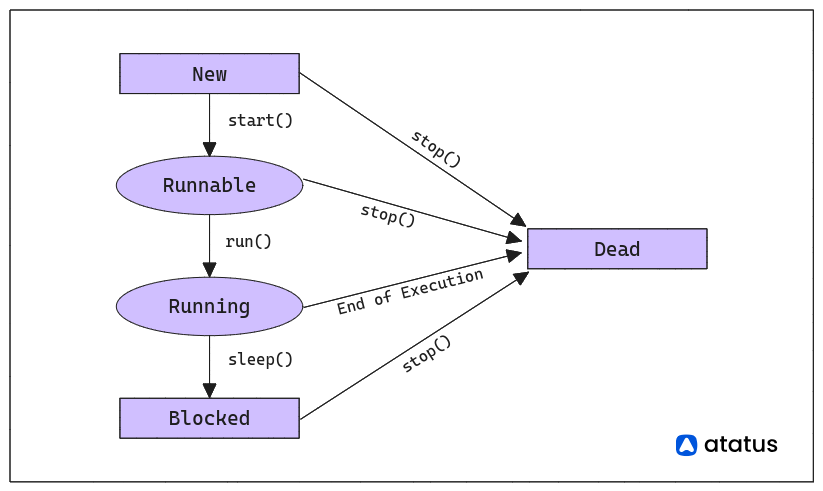
i.) New State: When you make a new thread with the "new"
command, the thread is in the new state, also called the Born state. This happens right after the thread is created but before it starts working. In this state, the thread's setup is done, but it hasn't actually begun doing its job yet.
ii.) Active State: Initiating a thread using the "start()" method
transitions it from the "New"
state to the widely recognized "Runnable"
state. Within this state, the thread may await its turn for execution or actively engage in instruction execution within the "Running" state.
- Runnable State: Threads within the
"runnable"
state are either actively processing or prepared for execution upon assessment. Threads within this state await their turn in a queue, prepared for task execution. - Running State: In the
"running"
state, a thread seizes CPU control for its task execution. In Java, threads are allocated a defined time duration for execution. The thread scheduler transition a thread from the"runnable"
state to the"running"
state, facilitating its execution.
iii.) Blocked/Waiting: In Java, when a thread becomes temporarily inactive for a period (not permanently), it enters what's known as the waiting or blocked state. This happens when a thread needs something specific to continue but has to pause until that condition is met or the necessary resource becomes accessible.
iv.) Timed waiting state: The timed waiting state occurs when a thread temporarily halts its execution for a predefined time interval. During this state, the thread remains inactive and "waits" for the specified time duration. Once the time elapses, the thread becomes runnable again and re-enters the execution cycle. This state is often used when a thread needs to wait for a certain event or resource, but with a time limit to avoid indefinite waiting.
v.) Terminated State: The terminated state indicates that a thread has finished its execution, which happens when it completes its assigned task. Additionally, a thread can enter the terminated state due to errors or exceptional circumstances. Threads in the terminated state no longer actively participate in the program's operation. When a thread is mishandled or triggers other unexpected executions, it might undergo abnormal termination, leading to its termination in an irregular manner.
How to Generate a Thread in Java?
As we already know, threads enable us to perform multiple operations concurrently, such as handling user input, performing background tasks, and more.
So, by mastering how to create threads in Java, you are essentially equipping yourself with the tools to build applications that are not only more dynamic and scalable but also well-equipped to deal with intricate tasks in parallel.
In Java, you can create a thread by either extending the Thread
class or implementing the Runnable
interface. let's delve deeper into both the approaches in detail for better understanding.
Approach 1: Extending Thread Class
When you extend the Thread
class, you are essentially creating a new type of thread with customized behaviour. This involves defining a new class that inherits from the Thread
class. Within this new class, you'll override the run()
method. This method holds the code you want the new thread to execute.
public class MyThread extends Thread
{
public void run()
{
System.out.println("Thread is running.");
}
public static void main(String[] args)
{
MyThread myThread = new MyThread();
myThread.start();
}
}
This Java code defines a class named MyThread
that extends the built-in Thread
class. It overrides the run()
method to contain the code executed when the thread starts, printing "Thread is running." The main
method creates an instance of MyThread
and invokes start()
to begin the thread's execution.
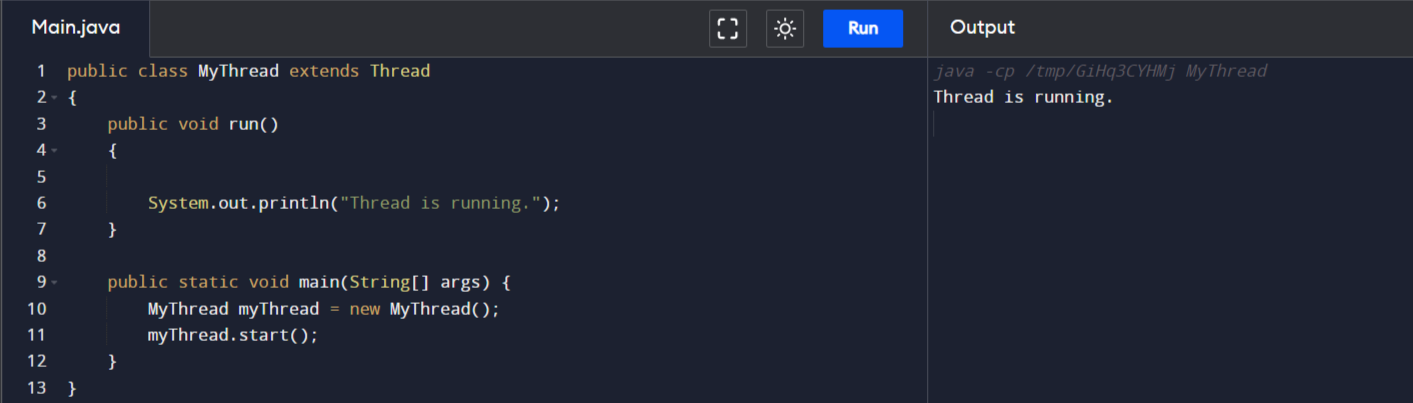
When the start()
method is called on the myThread
instance, it initiates the execution of the run()
method you have defined in the MyThread
class. This causes the "Thread is running." message to be printed to the console.
Approach 2: Implementing Runnable Interface
In this approach, you create a class that implements the Runnable
interface. The Runnable
interface defines a single method called run()
, where you put the code you want to execute in the thread. You create a thread object and provide an instance of your Runnable
class to its constructor. By calling the start()
method on the thread object, it starts running the code from the run()
method concurrently.
public class MyRunnable implements Runnable
{
public void run()
{
System.out.println("Thread is running.");
}
public static void main(String[] args)
{
MyRunnable myRunnable = new MyRunnable();
Thread thread = new Thread(myRunnable);
thread.start();
}
}
The code defines a class named MyRunnable
that implements the Runnable
interface. It contains a run()
method with the code to be executed when the thread starts. In this case, it prints "Thread is running."
In the main
method, an instance of MyRunnable
is created, and a new thread is constructed using it. By calling start()
on this thread, it commences the execution of the run()
method. This leads to the message being printed to the console, indicating the thread is running.
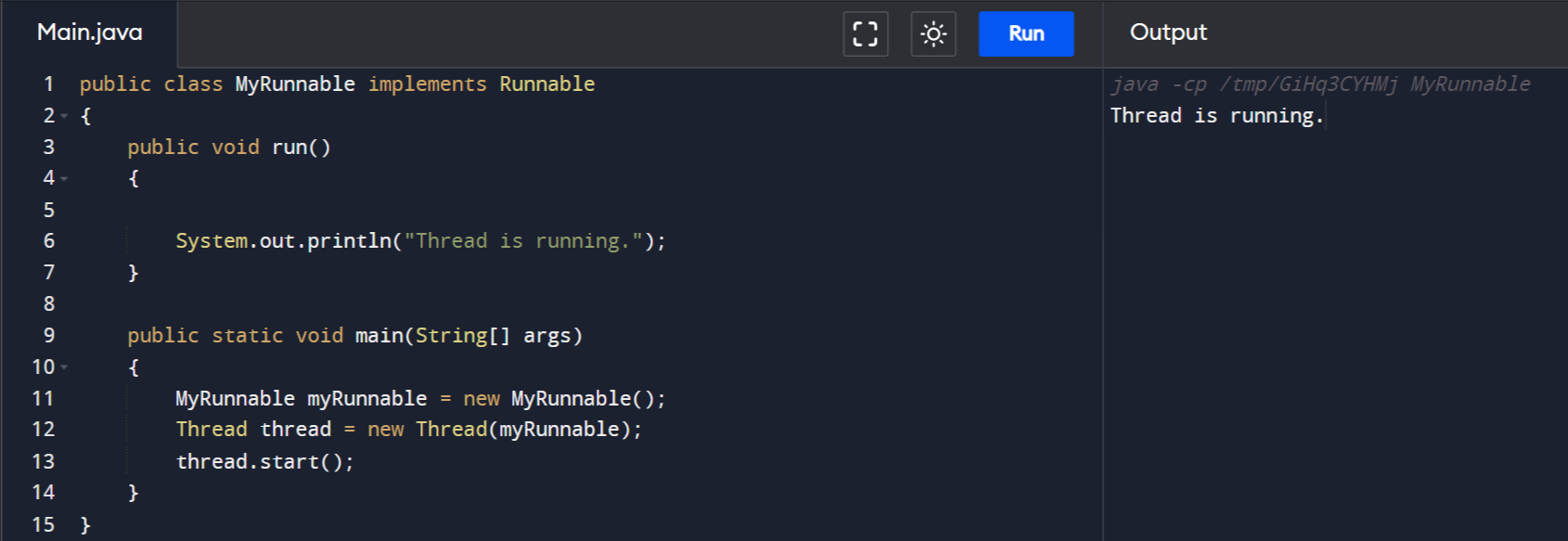
Both methods facilitate concurrent programming, yet the second approach, which involves utilizing the Runnable interface, is generally preferred. This is because it separates the thread's behaviour from the management of the thread itself. This makes your code more modular and flexible when working with concurrent tasks.
Methods of Thread Class
Method Name | Function |
---|---|
start() | Initiates thread execution by calling its run() method. |
run() | Contains the code executed by the thread. |
sleep(long millis) | Pauses the thread's execution for a specified time. |
yield() | Suggests yielding the CPU to other threads. |
join() | Waits for a thread to complete its execution. |
isAlive() | Checks if a thread is still executing. |
interrupt() | Requests a thread to interrupt its execution. |
isInterrupted() | Checks if a thread's interrupt status is set. |
currentThread() | Returns the currently executing thread. |
setName(String name) | Sets the thread's name for identification. |
setPriority(int priority) | Sets the thread's priority for scheduling. |
getState() | Retrieves the current state of the thread. |
Challenges and Solutions
Here are some common complexities that arises while accessing threads, let see one by one.
i.) Synchronization Issues: When multiple threads access shared resources (like variables, data structures, or files) simultaneously, synchronization issues can occur. This can lead to inconsistent data or unexpected behaviour.
class Synchronization
{
private int sharedCounter = 0;
public void incrementCounter()
{
sharedCounter++;
}
}
Use synchronization mechanisms to ensure only one thread can access the shared resource at a time, preventing simultaneous conflicting modifications.
ii.) Race Conditions: A race condition occurs when the final outcome of a program depends on the order of thread execution.
class RaceCondition
{
private int sharedValue = 0;
public void incrementValue()
{
sharedValue++;
}
}
iii.) Deadlocks: Deadlocks occur when two or more threads are stuck waiting for each other to release resources, resulting in a standstill. Deadlocks can halt program execution and require careful handling to prevent.
class DeadlockExample
{
private Object lock1 = new Object();
private Object lock2 = new Object();
public void method1()
{
synchronized (lock1)
{
synchronized (lock2)
{
// ...
}
}
}
public void method2()
{
synchronized (lock2)
{
synchronized (lock1)
{
// ...
}
}
}
}
Thus, arrange the acquisition of locks in a uniform sequence across all threads, preventing circular waits and thereby reducing the likelihood of encountering deadlocks.
iv.) Performance Overhead: While threads can enhance performance, they also introduce overhead due to thread creation, context switching, and synchronization.
When threads are created and destroyed frequently, it can introduce performance overhead due to the associated setup and cleanup operations. This overhead can impact the overall efficiency of your application.
In order to avoid overhead issues, you can set up a pool of reusable threads using the ExecutorService
interface in Java, rather than creating new threads every time a task needs to be executed.
Conclusion
Multi-threading in Java is a powerful mechanism that enables concurrent execution of multiple threads within a single application. It allows developers to harness the full potential of modern CPUs and create more responsive, efficient, and versatile software.
Understanding the complexities that come with multi-threading is essential. However, by carefully planning how threads work together, using tools to manage their interactions, and learning how Java's thread tools function, developers can make sure their programs work smoothly and quickly, handling many tasks at once without causing problems.
Thus, threading in Java presents both opportunities and challenges. A balanced approach, combining the benefits of multi-threading with an attentive approach to potential issues, ensures the creation of robust, efficient, and dependable software solutions.
Monitor Java Application for errors & exceptions with Atatus
When it comes to ensuring the optimal performance and reliability of your Java applications, monitoring is key. The ability to detect bottlenecks, identify slowdowns, and troubleshoot issues in real-time can significantly enhance the user experience and streamline your development workflow. That's where Atatus comes in.
With its powerful features and intuitive interface, you can gain deep insights into your application's performance metrics, traces, and errors. By visualizing the entire request lifecycle, pinpointing problematic areas becomes a breeze.
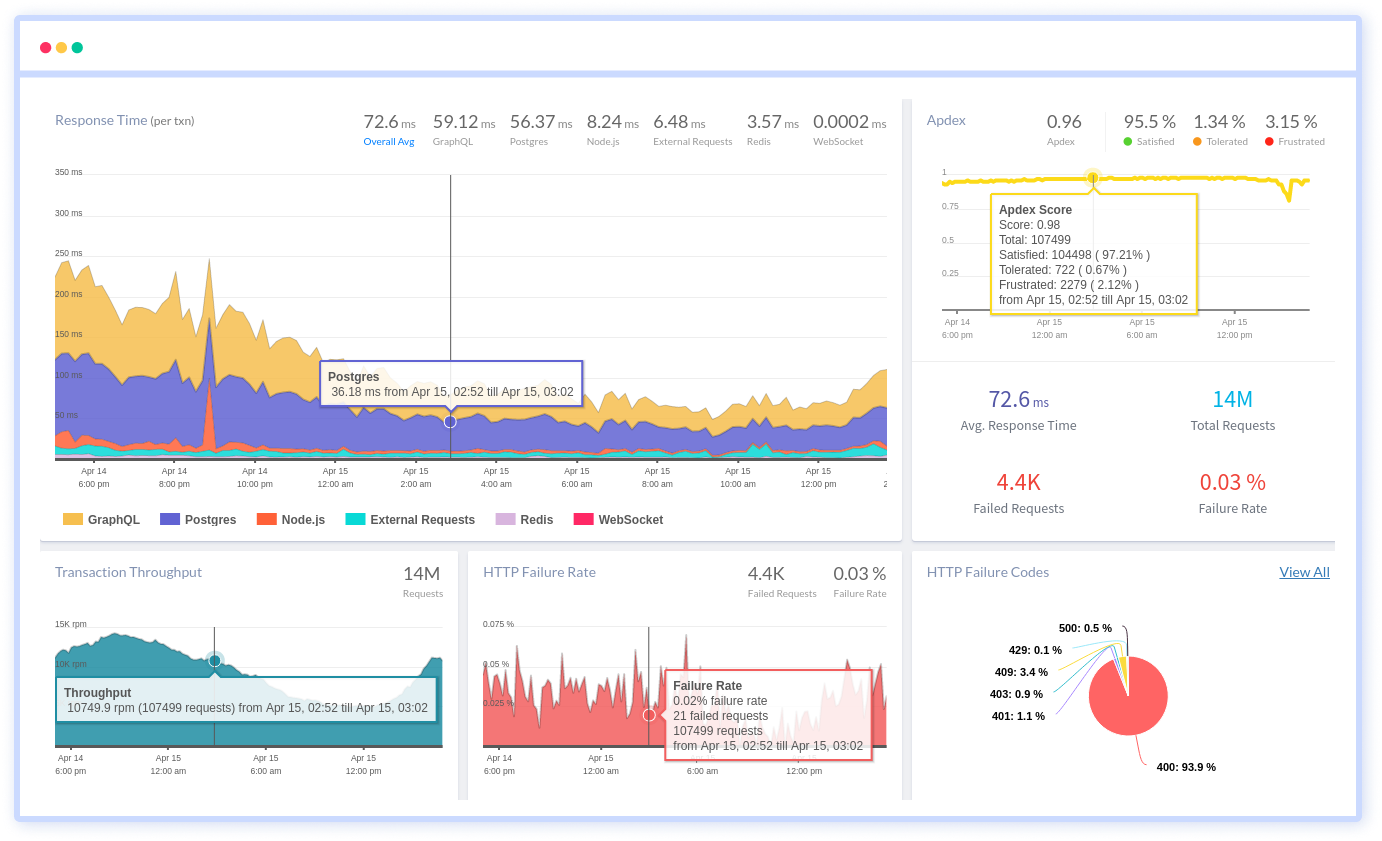
With Atatus Java Performance Monitoring:
- Root Cause Analysis: Quickly pinpoint the root causes of performance regressions.
- Improve User Experience: Deliver faster response times and smoother interactions for users.
- Code Profiling: Profile code execution to identify CPU-intensive or slow sections.
- Reduced Downtime: Minimize downtime through early identification and resolution of issues.
If you are not yet a Atatus customer, you can sign up for a 14-day free trial .