Achieve Optimal Performance with Nginx and Micro-Caching
The word "cache" is used in many different contexts. Typically, a cache is a small chunk of data stored locally for quick access. In this case, it’s about computer caches, i.e., the data stored in memory.
Micro-caching is a technique where a programmer uses this cached data to do some quick computations, which can lead to some amazing solutions.
In micro caching, dynamically generated content is cached for only a brief period rather than full-page caching.
Implementing micro-caching strategies on sites with high traffic and rapidly changing public content is only appropriate. This includes sites that offer real-time stock quotes, breaking news, or sports scores.
Table of Contents
- Introduction to Nginx and Micro-Caching
- Instance for Micro-Caching
- Micro-Caching with Nginx
- Features of Nginx with Micro-Caching
- Working of Micro-Caching with Nginx in Node.js
- Why Nginx is the ideal choice for Micro-Caching?
Introduction to Nginx and Micro-Caching
Nginx is a high-performance open-source web server and reverse proxy server commonly used in the industry. It is known for handling large amounts of traffic efficiently and effectively. Nginx is often used to serve static content, reverse proxying, load balancing, and caching.
Micro-caching is a technique used to cache small portions of data or responses for a short period of time, usually a few seconds. This technique is used to improve the performance of web applications by reducing the load on the backend servers and reducing the response time for the end-users.
Here are some examples of Nginx and micro-caching in action:
Nginx example: Suppose you have a website that receives traffic. Instead of handling all the requests directly on the backend server, you can use Nginx as a reverse proxy server to distribute the load and improve performance. Nginx can also serve static content, reducing the load on the backend server and improving the website's overall performance.
Micro-caching example: Suppose you have an e-commerce website that displays product information. The product information is likely to stay the same, and therefore it can be micro-cached for a short period of time.
Doing so can reduce the backend server load and improve the end-user's response time. For example, if a user visits the product page, the response can be cached for a few seconds so that subsequent requests for the same page can be served from the cache rather than being processed by the backend server.
How Micro-caching works?
Here is an example of how micro-caching can be used in a web application:
- A user requests a page from the server, which generates the page HTML and sends it back to the user's browser.
- The server also stores a copy of the generated HTML in a cache with a time-to-live (TTL) of a few seconds.
- If another user requests the same page within the TTL, the server can serve the cached HTML rather than generating it again, which reduces the load on the server and improves the response time for the user.
- Once the TTL expires, the cached HTML is removed from the cache and the process repeats for future requests.
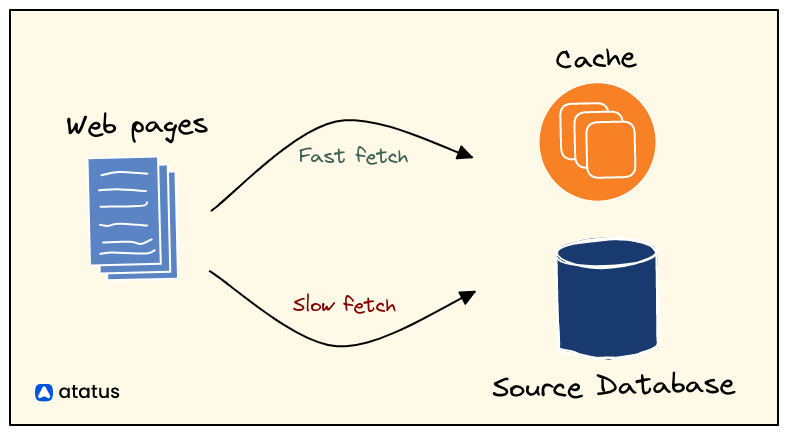
Micro-caching can improve web applications' performance by reducing the load on servers and databases, especially for frequently requested data or resources.
However, it is essential to carefully balance the benefits of micro-caching with the need to ensure that users always see the most up-to-date version of data or resources.
Instance for Micro-Caching
Imagine that you run an online store that sells a variety of products. Your store has a database that stores all of the products, along with their descriptions, prices, and other information. When a user visits your store and browses the product catalog, the store retrieves the list of products from the database and displays them to the user.
To improve the performance of the store, you decide to implement micro-caching. Here is how it might work:
- A user visits your store and requests a list of products.
- The store retrieves the list of products from the database and sends it back to the user's browser.
- The store also stores a copy of the list of products in a cache with a time-to-live (TTL) of a few minutes.
- If another user requests the same list of products within the TTL, the store can serve the cached list rather than querying the database again, which reduces the load on the database and improves the response time for the user.
- Once the TTL expires, the cached list is removed from the cache and the process repeats for future requests.
In this example, micro-caching is used to reduce the load on the database and improve the response time for users who request the list of products.
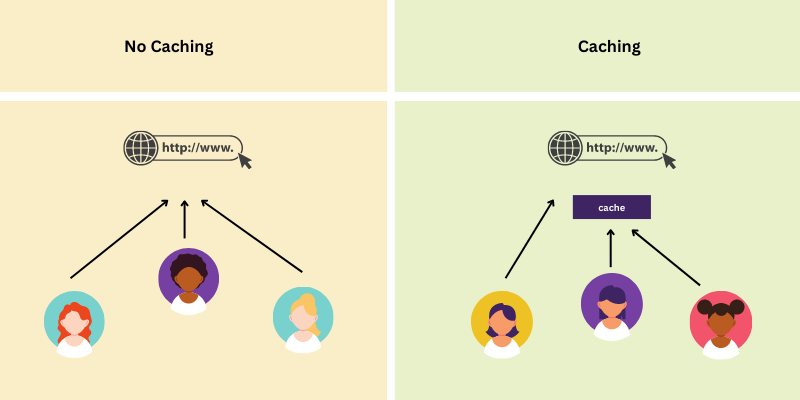
By storing a copy of the list in a cache with a short TTL, the store can serve the list from the cache rather than querying the database for each request, which can improve the overall performance of the online store.
Micro-caching with Nginx
Nginx is a high-performance web server that can be used with micro-caching to improve the performance and reliability of a web application.
Microcaching involves storing frequently accessed data or resources in a temporary storage location, called a cache, to reduce the server load and improve the response time for users or clients.
One of the key advantages of using Nginx with micro-caching is that Nginx handles a large volume of requests efficiently. In addition, by using Nginx as the front-end web server, you can offload the request handling from your application server, which can help improve your system's overall performance.
Nginx also has built-in support for microcaching, which means that you can easily configure it to cache small chunks of data or resources for a short period of time. This can help to reduce the load on your application server and improve the response time for users or clients.
With Nginx, you can cache static and dynamic content, which means you can cache a wide variety of data or resources. This can be particularly useful if you have a high volume of traffic or if you need to serve data or resources to users in different geographic locations.
Features of Nginx with Micro-Caching
Nginx is a high-performance web server that has a number of features that make it well-suited for microcaching, including:
- Configurable cache expiration times: Nginx allows you to specify the length of time that data or resources should be cached. This can be useful if you have data or resources that are only valid for a certain period of time.
- Cache keys based on request parameters: Nginx allows you to specify which request parameters should be used to generate the cache key. This can be useful if you have data or resources that vary based on the parameters of the request (e.g., language, user agent).
- Support for cache invalidation: Allows you to specify when the cache should be invalidated and the data or resources should be re-fetched from the backend server. This can be useful if you have data or resources that change frequently or that need to be updated in real-time.
- Load balancing and failover: Nginx can be configured to distribute requests across a group of servers to improve the system's performance and reliability. This can be useful if you have a high volume of traffic or if you want to ensure that your application is always available.
- Security features: Includes a number of security features that can help to protect your application from various types of attacks, including denial of service attacks, cross-site scripting attacks, and SQL injection attacks.
Working of Micro-Caching with Nginx in Node.js
Here is an example of how micro-caching might be implemented with Nginx in a Node.js web application:
First, you will need to install and configure Nginx as a web server for your Node.js application. You will also need to enable the Nginx cache module and set up a cache location in your Nginx configuration file:
http {
...
proxy_cache_path /path/to/cache levels=1:2 keys_zone=my_cache:10m max_size=10g inactive=60m use_temp_path=off;
...
}
The proxy_cache_path
directive sets up a cache in Nginx with the specified path, levels, keys zone, maximum size, and inactive time. The cache is named my_cache
and has a maximum size of 10 gigabyte.
Next, you will need to set up a route in your Node.js application that will be served by Nginx. In this example, we will set up a route that displays a list of products from a database:
app.get('/products', (req, res) => {
// Query the database for the list of products
const products = database.query('SELECT * FROM products');
// Send the list of products back to the client
res.send(products);
});
Finally, you will need to configure Nginx to cache the results of the /products
route for a specified period of time. To do this, you will need to add a location block to your Nginx configuration file that specifies the /products
route and the cache settings:
http {
...
server {
...
location /products {
proxy_cache my_cache;
proxy_cache_valid 200 10m;
proxy_pass http://localhost:3000;
}
...
}
...
}
This configuration tells Nginx to cache the results of the /products
route for 10 minutes (600 seconds) in the my_cache
cache zone.
When a user or client requests the /products
route, Nginx will check the cache to see if a copy of the response is stored there.
If a copy is found and it is still within the TTL period, Nginx will serve the cached response to the user or client.
If a copy is not found in the cache or the TTL has expired, Nginx will pass the request to the Node.js application, which will generate the response and send it back to Nginx.
Nginx will then store a copy of the response in the cache and serve it to the user or client.
By using micro-caching with Nginx, you can improve the performance of your Node.js web application's performance by reducing the backend server load and improving the response time for users or clients.
However, it is essential to carefully balance the benefits of micro-caching with the need to ensure that users or clients always see the most up-to-date version of the data or resources.
Why Nginx is the ideal choice for Micro-Caching?
There are several reasons why it might be beneficial to use Nginx with microcaching:
1. Improved performance
By serving frequently requested data or resources from a cache rather than generating them on demand, micro-caching can improve a web application's performance by reducing the backend server load and improving the response time for users.
Nginx is a high-performance web server that can quickly and efficiently handle many requests, making it well-suited for implementing micro-caching.
2. Reduced load on the backend
By serving cached data or resources rather than generating them on demand, Nginx can reduce the load on the backend server or database, which can help improve the application's scalability and reliability.
3. Reduced resource usage
By serving data or resources from a cache, micro-caching can reduce the amount of CPU, memory, and other resources used by the backend server. This can help to reduce the overall cost of running the application. Nginx is known for its low resource usage, which can further improve the efficiency of the application.
4. Improved response time
It is possible to improve the user experience of an application by serving data or resources that have been cached by Nginx in order to improve the response time for users.
5. Supports multiple protocols
The portability of Nginx, which supports HTTP, HTTPS, and HTTP/2, makes it a valuable choice for web applications that must support multiple protocols.
6. Simplified configuration
Nginx offers an easy-to-use configuration system for setting up micro-caching for web applications. The configuration can be tailored to the application's specific needs and modified as needed over time.
7. Compatibility with other caching mechanisms
Nginx can work with other caching mechanisms, such as browser caching or CDN caching, to provide an even more efficient and scalable solution for the application.
8. Simplified maintenance
By using micro-caching, developers can reduce the number of changes that need to be made to the backend server, making it easier to maintain the application over time.
Conclusion
Implementing micro-caching requires little time and effort. Successful micro-caching techniques appear to use no additional time or effort and are successful.
A micro-cache is pertinent only for data that does not impact processes, operations, or integrity. To increase the performance of dynamic content without sacrificing user experience, micro-caching is an intense method.
As a result, most visitors to the website get a copy of the website from the static content cache, rather than the origin server, when the web pages or websites are heavily loaded.By implementing this process, there will be less load on the server, as well as improved overall performance.
Enhance Your Server's Performance with Atatus Nginx Monitoring
Nginx performance monitoring offers essential insights into your web server's efficiency and reliability by providing real-time tracking and in-depth analysis of critical Nginx metrics. With continuous monitoring, you gain the power to proactively address issues, optimize resource allocation, and ultimately enhance the overall performance and user experience of your web applications.
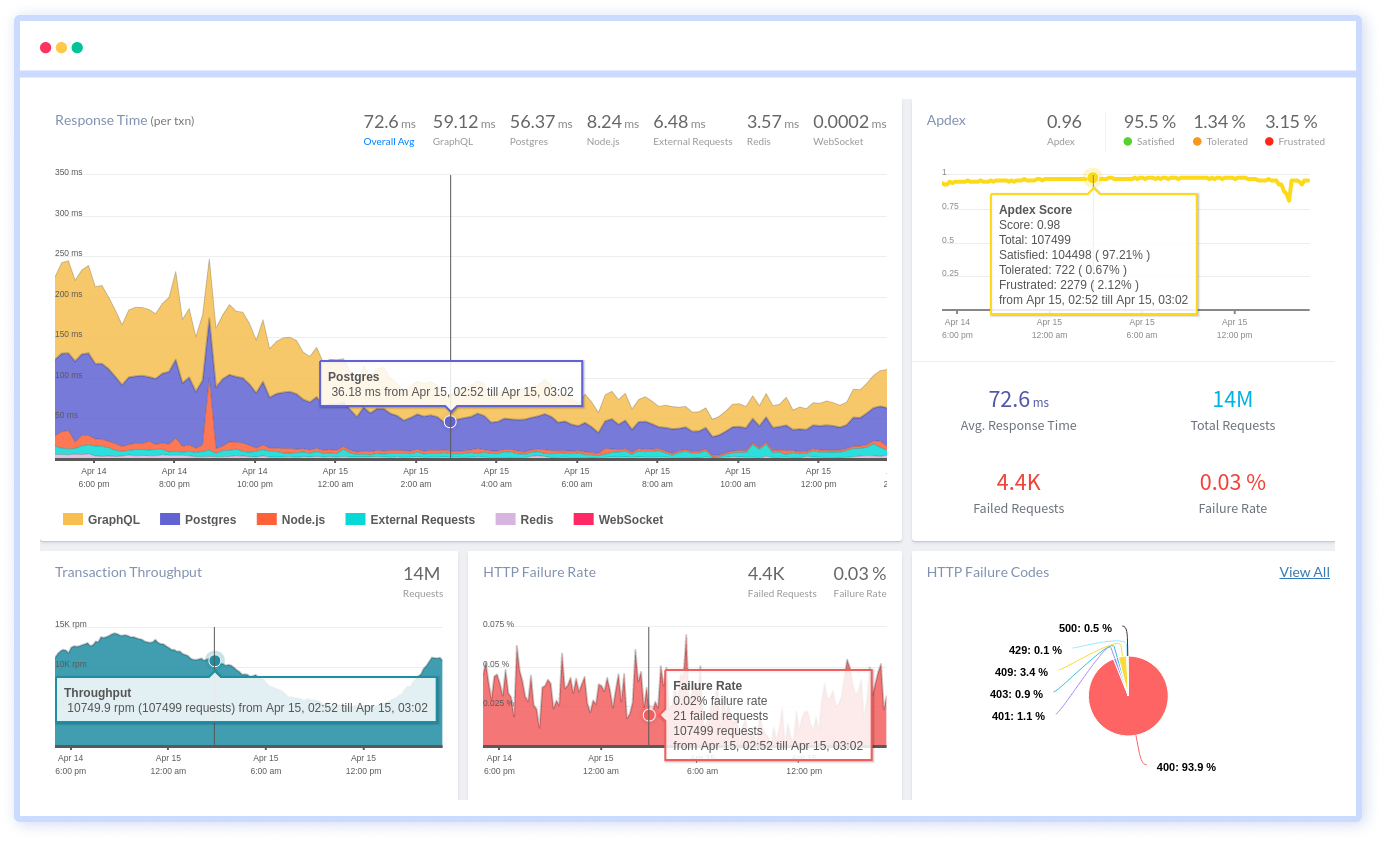
By closely monitoring request rates, error codes, and server load, you can pinpoint areas for improvement and optimization. Identifying bottlenecks and addressing them promptly ensures that your web server operates at its best, delivering a seamless user experience. It also streamlines resource management, reducing costs and improving scalability for efficient and budget-friendly server operations.
If you are not yet a Atatus customer, you can sign up for a 14-day free trial.
#1 Solution for Logs, Traces & Metrics
APM
Kubernetes
Logs
Synthetics
RUM
Serverless
Security
More