8 Best Node.js Schedulers to Know
Job scheduling is the process of starting a job, task, or function at a specific time or when specific events take place. Cron, the time-based job scheduler used in Unix-like systems, is the basis for the majority of job schedulers.
Numerous job schedulers are available for the Node.js runtime environment. While some of these schedulers operate in both the browser and Node, others exclusively in Node. Choosing the best scheduler for your use case can be challenging and time-consuming because each of these schedulers has its own characteristics.
We will walk you through the most common Node.js job schedulers in this article, highlighting their important characteristics, differences, and similarities. We will highlight helpful metrics and information, such as bundle size, GitHub stars, release count, npm downloads, package licenses, dependencies, and maintenance, among others, in addition to the major features and functions provided by each package.
Here’s how it’s done:
#1 Agenda
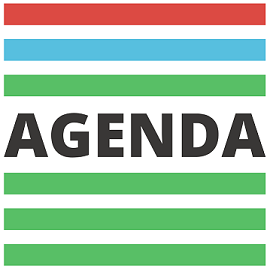
Agenda provides the ability to flexible schedule jobs using cron and a more approachable syntax. To utilize Agenda as a job scheduler, your MongoDB database must be operational.
Create a Docker container or use MongoDB's cloud database Atlas to try out this scheduler if you don't want to download and install MongoDB on your system. If you don't want to use MongoDB, this package is probably not a viable choice for you.
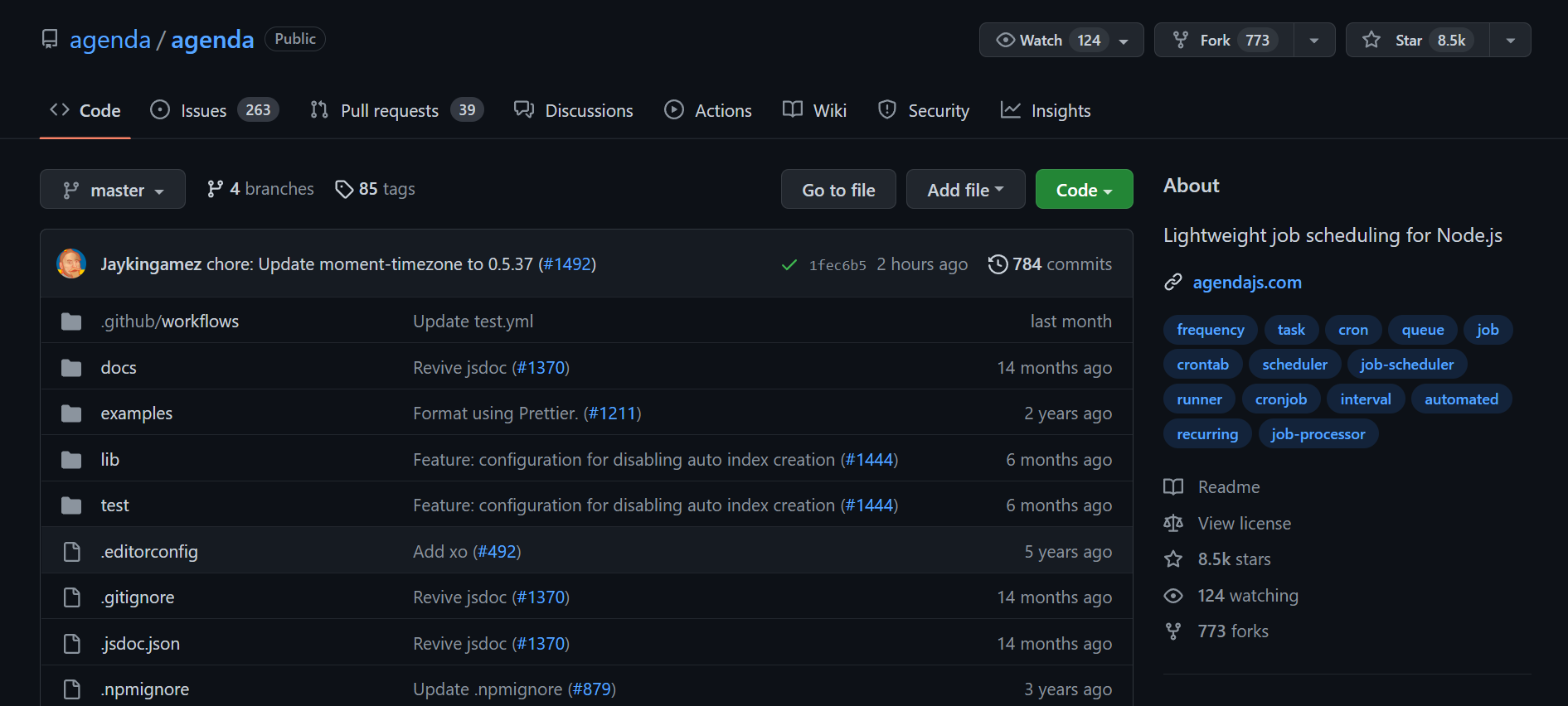
I have defined a simple job that logs to the console once every minute in the code snippet below:
const mongoConnectionString = "mongodb://127.0.0.1/agenda";
const agenda = new Agenda({
db: {
address: mongoConnectionString
}
});
agenda.define("Delete old users", async (job) => {
await User.remove({
lastLogIn: {
$lt: twoDaysAgo
}
});
});
(async function() {
// IIFE to give access to async/await
await agenda.start();
await agenda.every("3 minutes", "Delete old users");
// Alternatively, you could also do:
await agenda.every("*/3 * * * *", "Delete old users");
})();
Agenda is a Node scheduler that is MIT-licensed. It was initially released eight years ago; the most recent version is 4.3.0. It has 8.5k GitHub stars and 90,562 npm weekly downloads.
Agenda is a pretty sophisticated package, especially given the number of releases. It has a lot of features, but it also has a lot of dependencies. Agenda has an install size of 18.8MB and a publish bundle size of 339.4 KB.
#2 Node Schedule
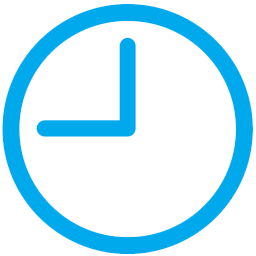
Node Schedule is a versatile job scheduler for Node.js that works both like and unlike cron. With configurable recurrence criteria, it enables you to schedule jobs (arbitrary functions) for execution at particular dates. It doesn't evaluate upcoming jobs every second or minute, just using one timer at a time. Instead of using interval-based scheduling, Node Schedule uses time-based scheduling.
This is another MIT-licensed open-source tool for Node.js job scheduling. According to the number of downloads on npm, it is the second most used Node scheduler. Node-schedule has had plenty of opportunities to adapt and advance over the years, with 44 versions produced before the current version 2.1.0.
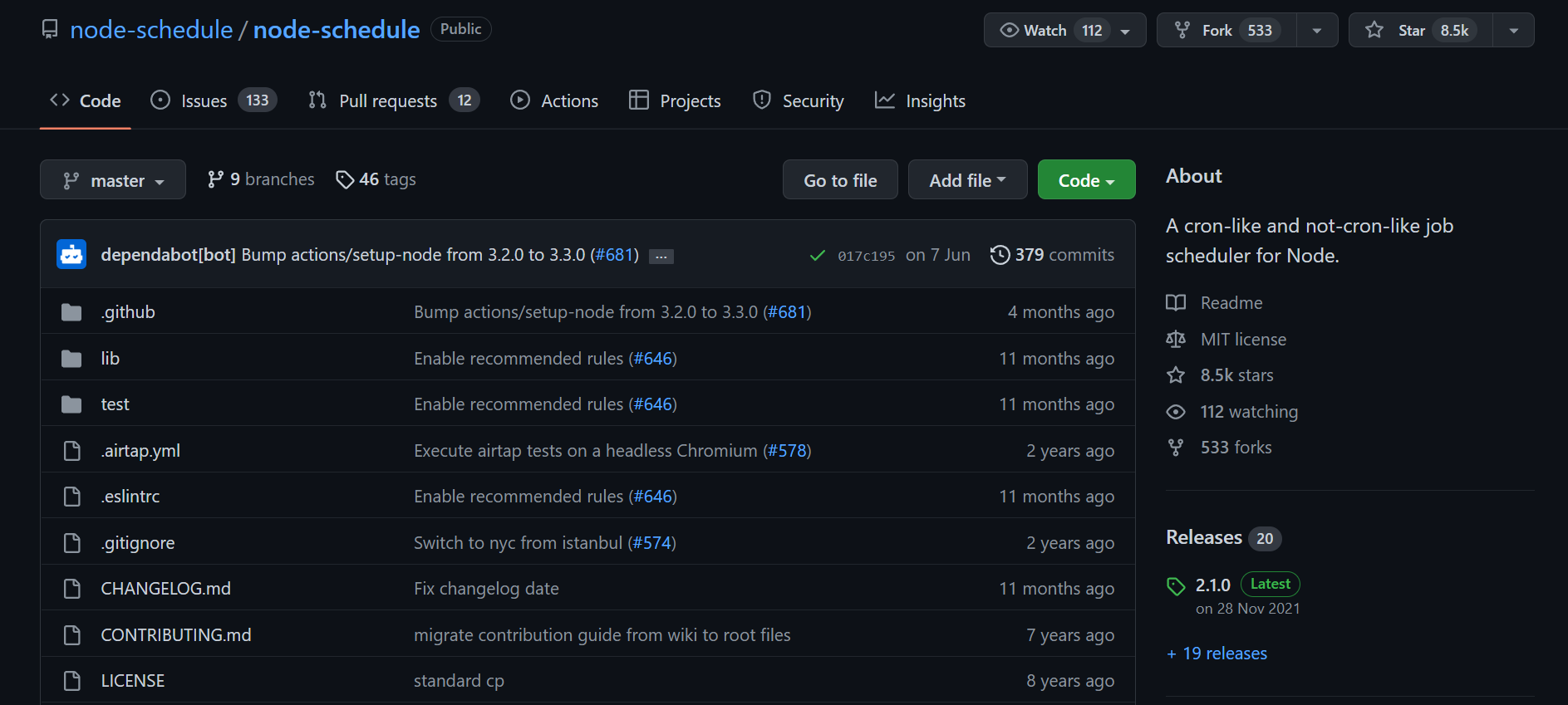
Although it can also be used flexibly, Node-schedule is mostly for time-based scheduling as opposed to interval-based scheduling. You can quickly schedule a job to run on particular dates with the help of Node-schedule, which also offers an optional recurring configuration.
You have the choice of using date-based scheduling and cron-style scheduling with Node-schedule. When utilizing cron-style scheduling, you can pass a cron expression and have cron-parser parse it to indicate when the job is triggered:
const nodeSchedule = require('node-schedule');
const job = nodeSchedule.scheduleJob('* * * * *', function() {
console.log('Job has been triggered at: ', new Date.toLocaleTimeString());
})
Contrarily, date-based scheduling allows you to pass a real JavaScript date object to determine a job's specific execution date. Additionally, it offers the choice to bind current data for usage in the future.
Like most Node schedulers, Node-schedule has the restriction that the job will only be triggered when the script is active. Consider utilizing Cron if you want to schedule a job that will continue to execute even if the script is not. Similarly, if you wish to keep the job between restarts, it is not a good alternative.
Node-schedule has an install size of 3.15MB and a publish size of 33.2KB after running through Packagephobia.
#3 Node Cron
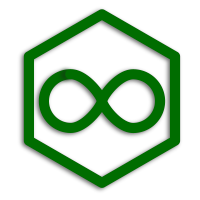
An open-source, ISC-licensed package is node cron. Another established, reliable, and tried-and-true Node scheduler is Node-cron, which was first released six years ago. On npm, the most recent version, 3.0.2, receives roughly 355,569 downloads per week.
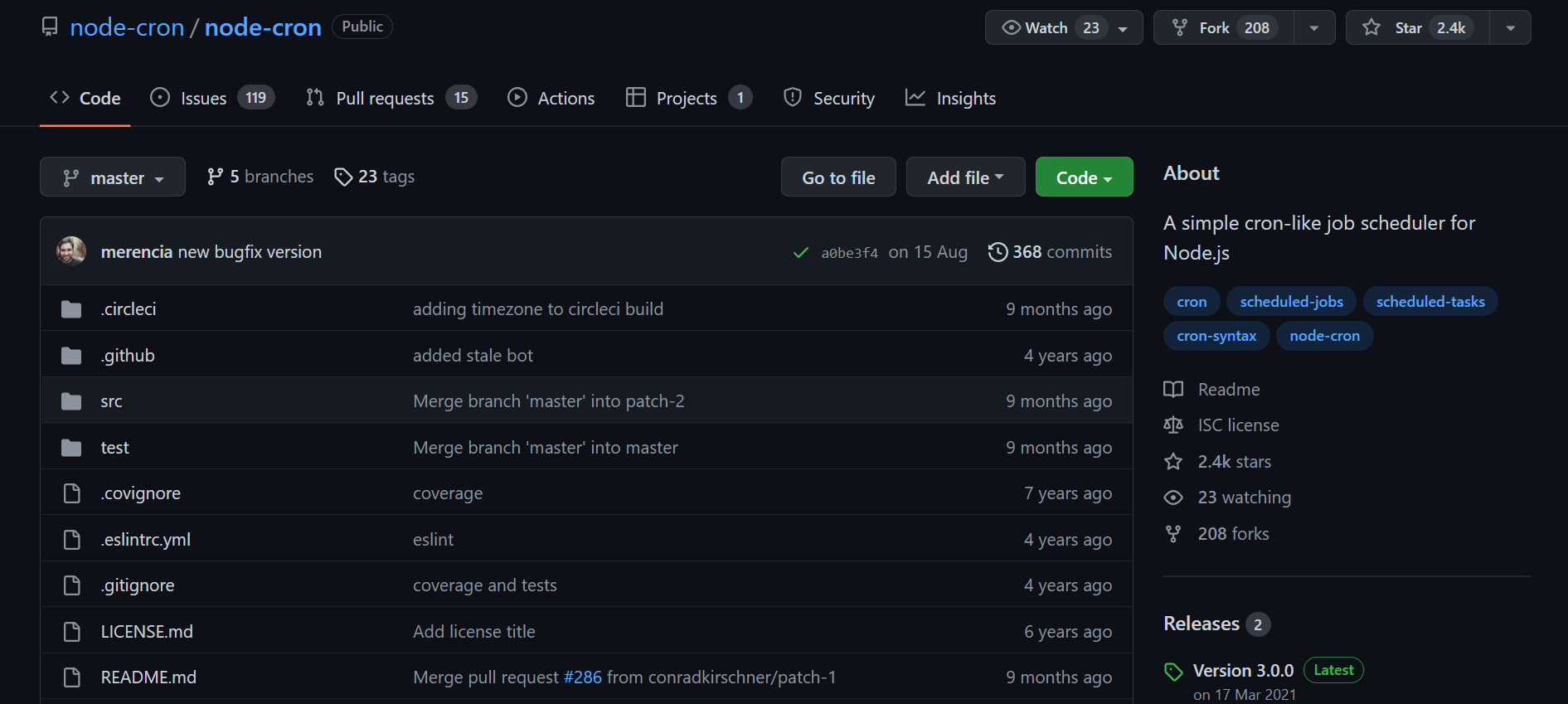
The node-cron module is a tiny GNU crontab-based job scheduler for node.js written entirely in JavaScript. This module enables complete crontab syntax job scheduling in node.js. Node-cron can be programmed to start the job at predetermined times or periodically:
const nodeCron = require('node-cron');
nodeCron.schedule('* * * * * *', () => {
// This job will run every second
console.log(new Date().toLocaleTimeString());
})
Node-cron includes methods for validating cron expressions, and beginning, halting, and terminating scheduled jobs in addition to the schedule method for scheduling a job.
Node-cron will be helpful if you need to schedule a simple job in Node or on the server side, but it won't be much use if you also need a scheduler that supports worker threads or runs in the browser.
There is only one first-level dependency for Node-cron. According to Packagephobia, the publish and install sizes for Node-cron are 65.6KB and 5.26MB, respectively.
#4 Bree
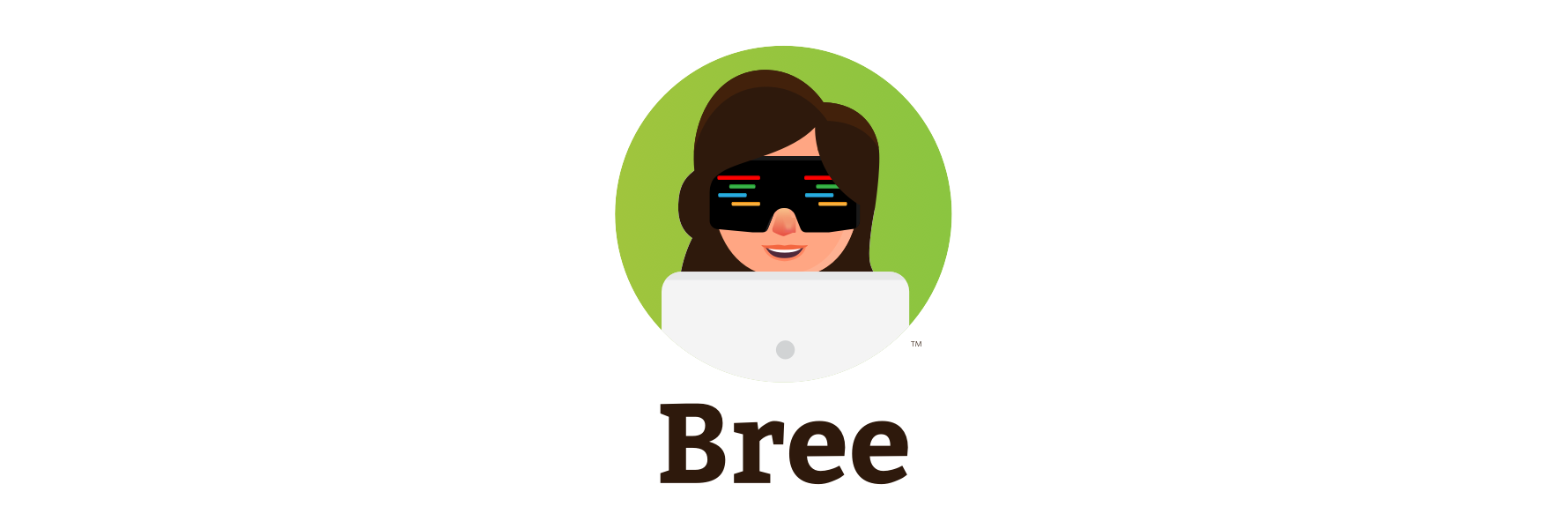
Bree is another JavaScript job scheduler that is MIT-licensed. Both Node and the browser are used to run it.
In comparison to the other schedulers mentioned above, this package is quite new. Since it was first released about nine months ago, there have been about 72 publications of it. On npm, the most recent version, 9.1.2, receives roughly 21,417 downloads per week.
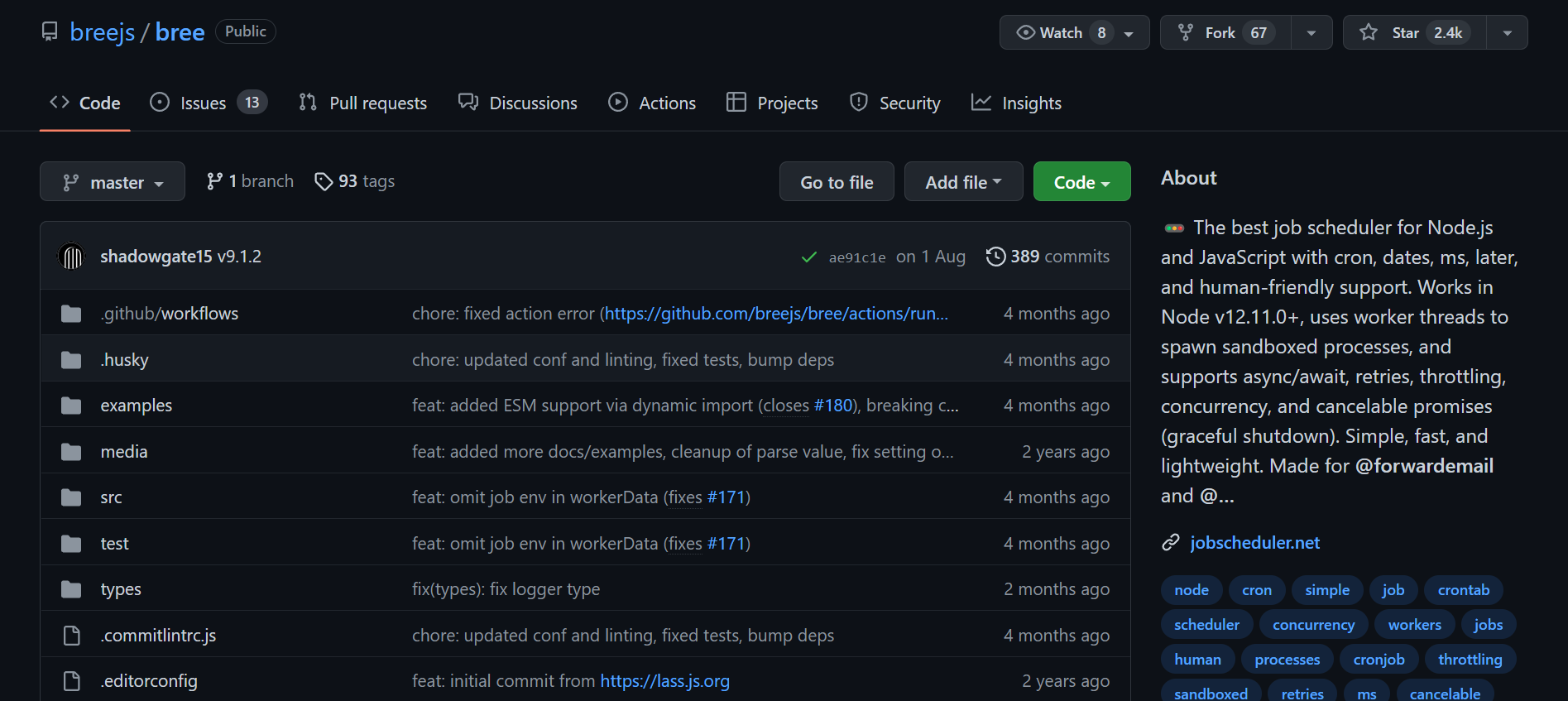
Bree, the newest scheduler, offers significantly more features than most of the competition and is the only one that works with Node and the browser. According to the documentation, Bree spawns sandboxed processes using worker threads in Node and web workers in the browser.
It supports throttling, long-running operations, concurrency, and a lot more. To view a complete list of features, read the documentation.
You can use Bree to plan a simple job by looking at the code excerpt below. The functions scrape-data.js, backup-database.js, and send-email.js are listed in the jobs folder at the project directory's root:
const Bree = require('bree');
const jobs = [{
name: 'scrape-data',
interval: 'every 1 minute'
},
{
name: 'backup-database',
timeout: 'at 12:00 am'
},
{
name: 'send-email',
timeout: '1m',
interval: '5m'
}
];
const bree = new Bree({
jobs
});
bree.start();
There are 18 first-level dependencies on this package. After running it through Packagephobia, the publish size is 1.38MB and the install size is 5.64MB.
Similarly, after running the package through Bundlephobia, Bree's minified and gzipped bundle size is 64.3KB if you're considering executing it on the client side.
#5 Cron
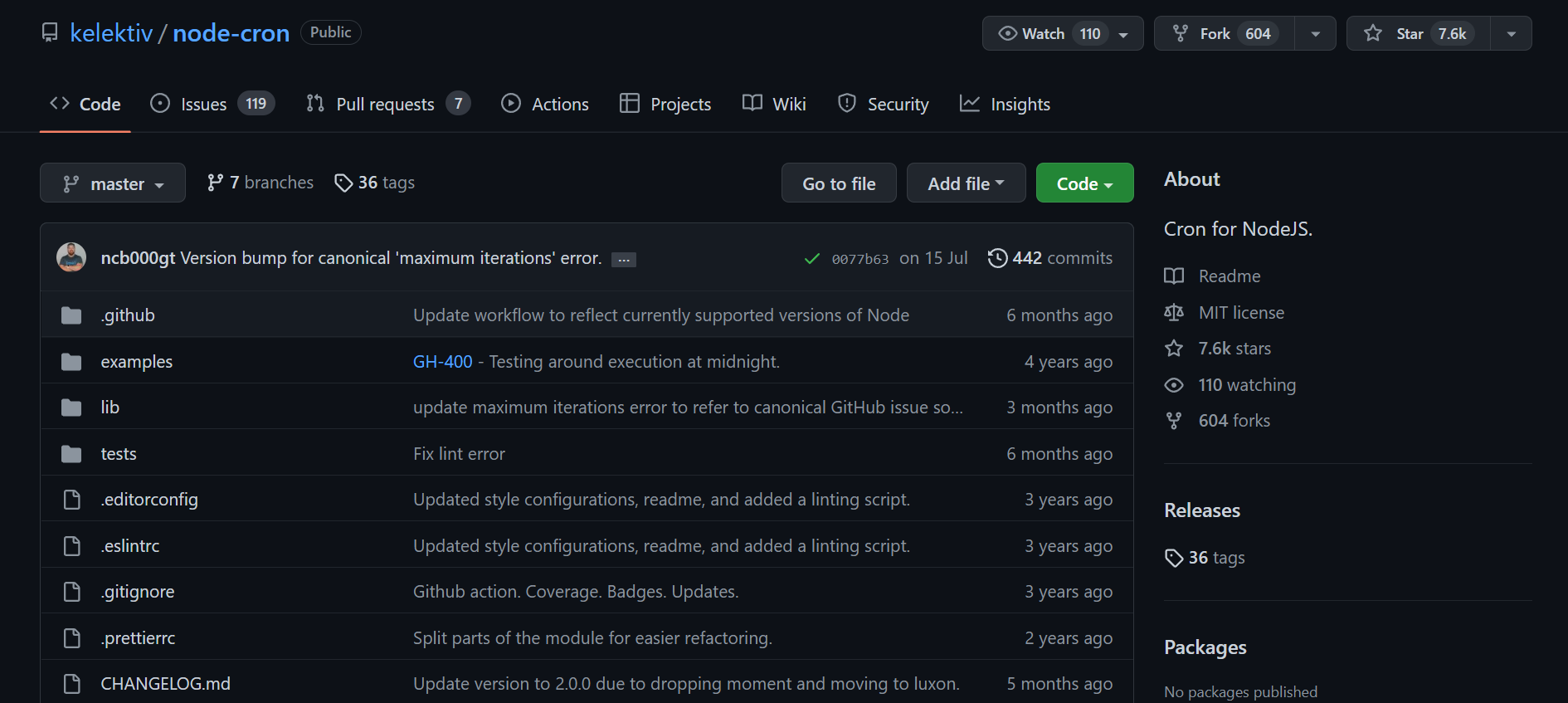
Be careful not to mix this MIT-licensed Node scheduler with Node-cron. The GitHub repository name for both Cron and Node-cron is node-cron. You could assume that one is a fork of the other if you search GitHub.
Since its initial release ten years ago, 40 versions of this package have been released. The Node scheduler has the most downloads of those mentioned here, with 1,243,355 weekly downloads as of version 2.1.0 and the highest download count.
The crontab syntax, "* * * * *," can be used to plan when a job will be executed. The expression adds a seconds option, extending the Unix pattern. The seconds option defaults to zero if it is not given.
Additionally, it gives the option of giving a date object rather than the cron expression. Cron's functionality is pretty similar to Node- cron's.
const {
CronJob
} = require('cron');
const job = new CronJob('* * * * * *', function() {
console.log('This job is triggered each second!');
});
job.start();
In addition to 1,701 other dependencies, Cron has one first-level reliance on npm. The dependencies for Cron and Node-cron are the same.
Its install size is 5.25MB, and its publish size is 69.3KB. As you can see, the publish size is only 3.7KB larger than Node- cron's.
#6 Bull
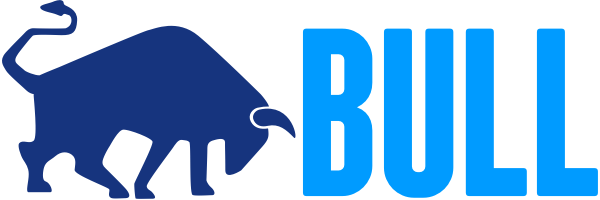
Bull is a queuing system for Node that uses a Redis server that must be running. You will have to do with the other schedulers if you don't want to use Redis. If installing Redis on your system is not something you want to do, there are Redis hosting services that integrate well with Bull.
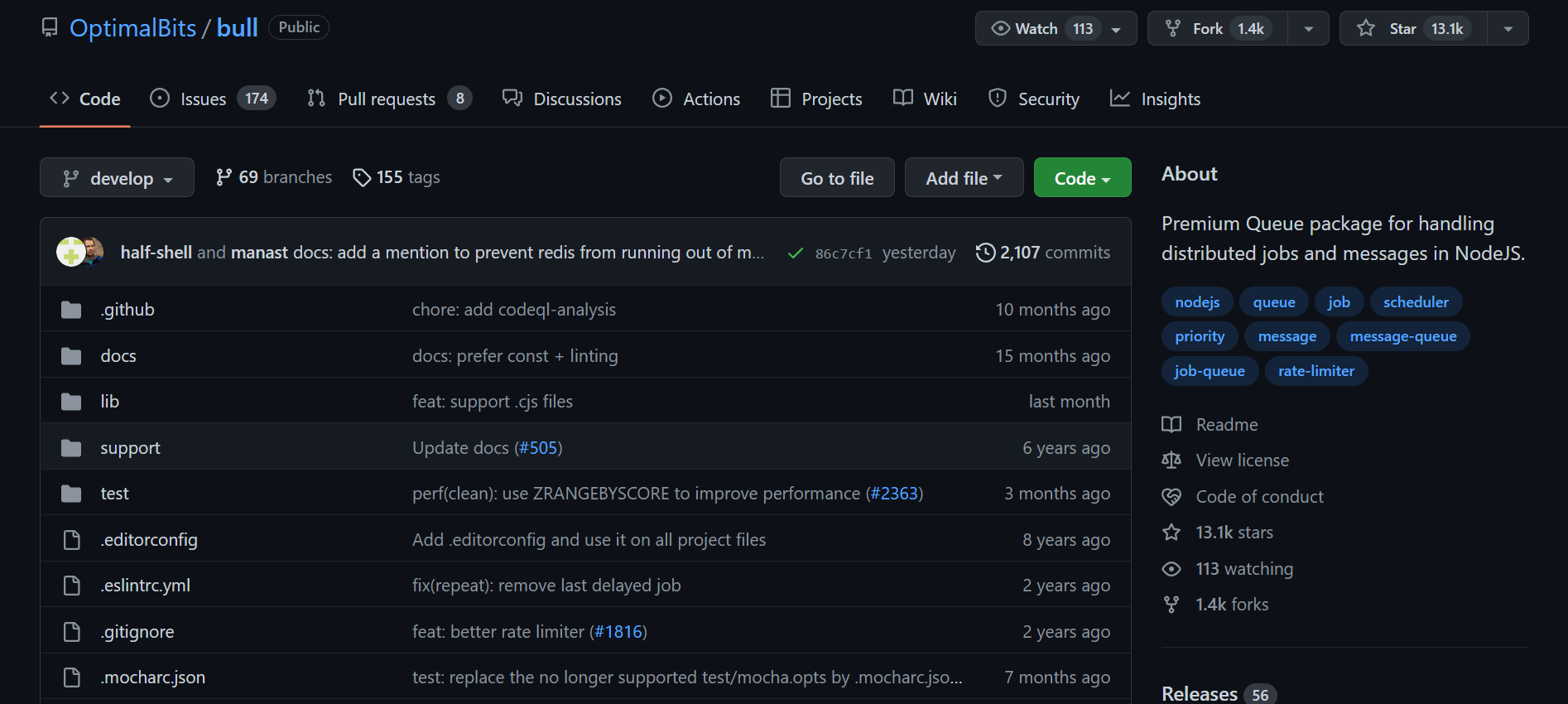
Bull boasts the quickest and most dependable Redis-based queue system for Node runtime environments. This MIT-licensed package has 421,580 weekly downloads and was published nine years ago. The fact that it is currently at version 4.10.0 shows how mature and reliable it is.
Among other things, Bull provides cron syntax-based job scheduling, rate-limiting of jobs, concurrency, running multiple jobs per queue, retries, and job priority. You can see the documentation for a complete list of features.
With the default Redis configuration, the simple example below will write "Hello World!" to the terminal after five seconds:
const Queue = require('bull');
const helloWorldQueue = new Queue('hello-world-queue');
const data = {
someData: 'Hello World!'
};
const options = {
delay: 5000,
attempts: 3
};
helloWorldQueue.add(data, options);
helloWorldQueue.process((job) => {
console.log(job.data.someData);
});
There are 10 first-level dependencies on this package. The package has an install size of 9.14MB and a publish size of 170KB.
#7 Bottleneck
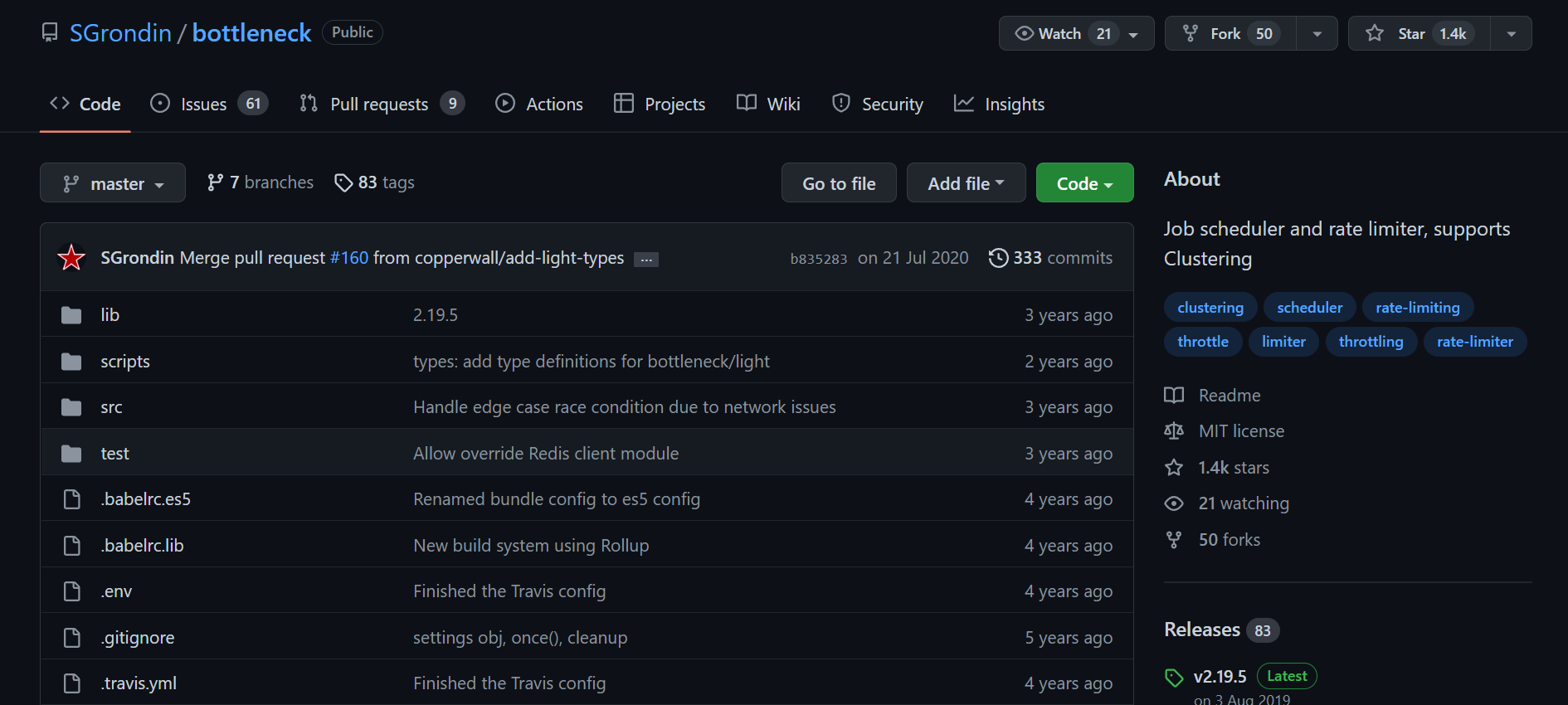
The bottleneck is a rate limiter and job scheduler for Node.js and a browser that is small and independent. On npm, the most recent version, 2.19.5, receives roughly 1,500,861 downloads per week.
Since the bottleneck adds very little complexity to your code, it is a simple solution. It is widely utilized by private businesses and open-source software and is battle-tested, dependable, and production-ready.
It is capable of rate-limiting jobs over several Node.js instances and enables clustering. To maintain dependability in the presence of unstable clients and networks, it uses Redis and strictly atomic operations. Redis Cluster and Redis Sentinel are also supported.
The majority of APIs have a rate limit. For instance, to process three requests every second:
const limiter = new Bottleneck({
minTime: 333
});
If you wish to stop more than one request from running at once and there's a potential that certain requests might take longer than 333ms, add maxConcurrent: 1:
const limiter = new Bottleneck({
maxConcurrent: 1,
minTime: 333
});
Bottleneck creates a queue of tasks and completes them quickly. The jobs will typically be carried out in the order that they were received by default.
#8 Neoplan
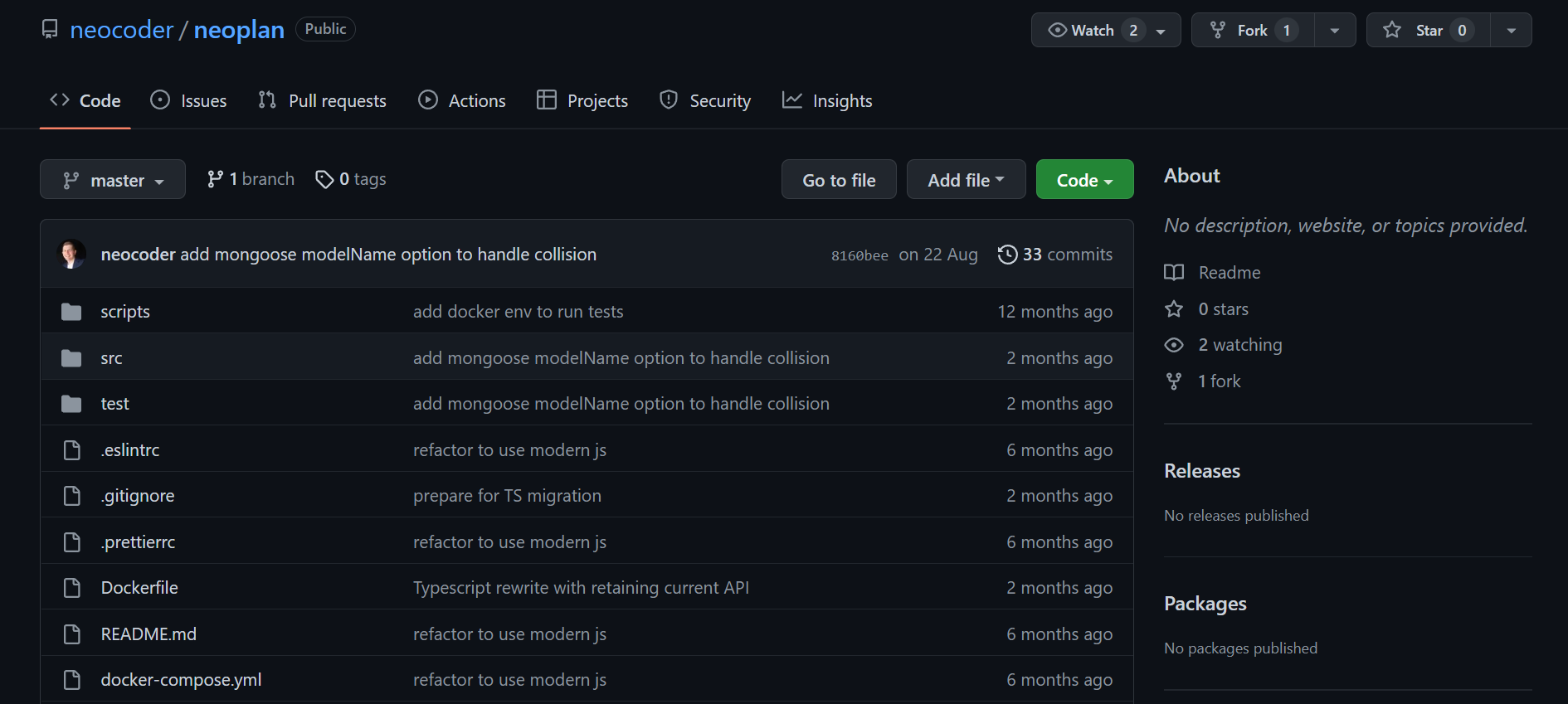
Neoplan is a simple, Agenda-inspired Node.js job scheduler built on MongoDB. On npm, the most recent version, 1.2.1, receives roughly 7 downloads per week.
Neoplan differs from Agenda in several ways, including the fact that it doesn't have as many features and lets you schedule tasks with the same name but different input values.
A neoplan is an example of a simple control structure. The jobs are loaded into Neoplans by mapping them to a database collection.
var neoplan = new Neoplan({
db: {
address: 'localhost:27017/neoplan-example'
}
});
neoplan.connect();
neoplan.define('delete old users', function(data, done) {
User.remove({
lastLogIn: {
$lt: twoDaysAgo
}
}, done);
});
neoplan.every('3 minutes', 'delete old users');
// Alternatively, you could also do:
neoplan.every('*/3 * * * *', 'delete old users');
Neoplan must be started for it to begin processing jobs from the database. This will set up a check for new jobs and a run interval (depending on processEvery). The line can also be stopped.
Multiple node instances or machines processing from the same queue may be desired occasionally. Neoplan has a locking feature to prevent the same job from being processed by different queues.
There is no built-in web interface for Neoplan. Nevertheless, neoplan-UI is a standalone web interface that is available.
Summary
The Node package ecosystem offers a variety of job schedulers for users to choose from. Your choice of a scheduler will mostly depend on your requirement because the majority of the packages contain features that will satisfy your basic job scheduling needs.
If you want to execute straightforward and easy work scheduling on the server side, Cron, Node-cron, and Node-schedule could be worth looking into because they are simple, well-established, popular, dependable, and have accommodating licenses. For easy tasks, we advise using one of the three.
Both Agenda and Bull provide simple job scheduling, but with extra capabilities and assistance for database persistence. If you aren't planning to use the extra functionality those bundles and dependencies offer, you wouldn't want them in your application.
On the other hand, Bree might be your best option if you're looking for a scheduler with additional features like support for worker threads or the ability to run in both the browser and Node (in addition to basic job scheduling).
Monitor Your Node.js Applications with Atatus
Atatus keeps track of your Node.js application to give you a complete picture of your clients' end-user experience. You can determine the source of delayed response times, database queries, and other issues by identifying backend performance bottlenecks for each API request.
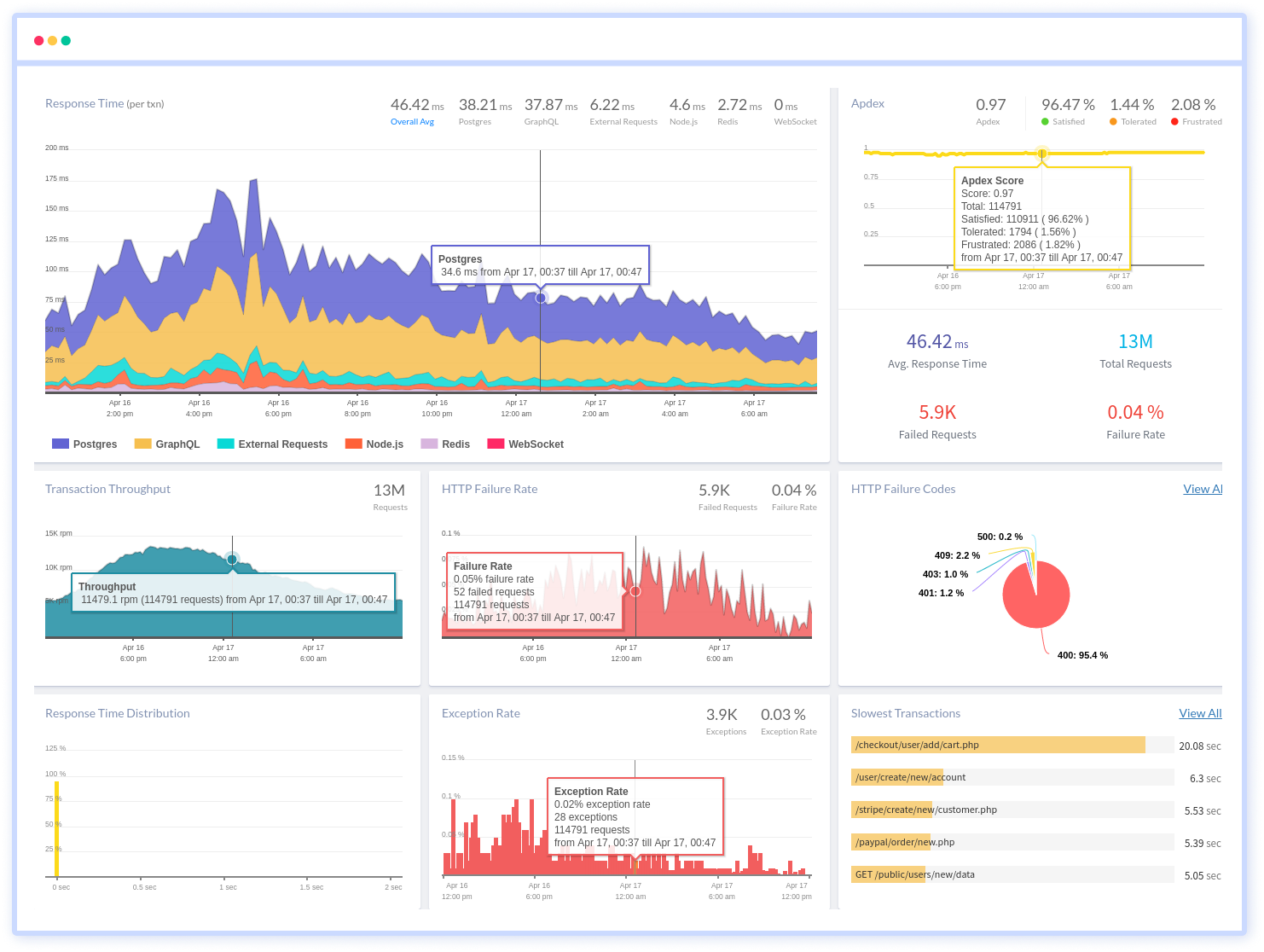
To make bug fixing easier, every Node.js error is captured with a full stack trace and the specific line of source code marked. To assist you in resolving the Node.js error, look at the user activities, console logs, and all Node.js requests that occurred at the moment. Error and exception alerts can be sent by email, Slack, PagerDuty, or webhooks.
#1 Solution for Logs, Traces & Metrics
APM
Kubernetes
Logs
Synthetics
RUM
Serverless
Security
More