Understanding API Calls: A Comprehensive Overview
Application Programming Interface (API), is like a hidden gateway that holds the key to unlocking a world of interconnected possibilities. An API acts as the intermediary software that sends a request to a server and subsequently transmits the server's response back to the client.
It acts as a secret passage connecting various software systems, enabling them to communicate, collaborate, and exchange information seamlessly.
Now that we have a gist of what an API is and what it does, let's now shift our focus towards the concept of an API call. API calls offer a world of opportunities for developers, allowing them to tap into the functionalities of external services, leverage powerful algorithms, or integrate data from multiple sources. Each call is an invitation to explore, discover, and create.
Now that you have been introduced to the topic of API calls, let us dive deep into the details of this topic in our blog.
We will explore the ins and outs of API calls, understanding their importance and discovering the endless opportunities they present.
Table of Contents
- Introduction to API Calls
- Methods of API Calls
- Different Modes of Executing an API Call
- Error Handling in API Calls
- Securing API Calls with HTTPS and SSL/TLS
- Common Mistakes to Avoid When Making API Calls
Introduction to API Calls
An API call refers to making a request to an API to retrieve or manipulate data or perform a specific action. An API call is typically made by a client application or system to communicate with a server-side API.
API calls facilitate seamless communication and integration between applications, enabling developers to leverage the capabilities of external services, retrieve data, perform transactions, and automate processes.
Still, trying to get the concept? Okay, let us look into an example to understand the concept better. Imagine you are using a travel planning application that provides information about flights. You enter your search criteria, such as the departure and arrival cities, the date of travel, and any other preferences.
When you hit the "Search" button, the application behind the scenes makes an API call to a flight booking API. The API call includes the necessary information, such as the origin and destination airports, the date of travel, and any additional parameters.
The API processes the request, queries its database or interacts with external flight booking systems, and retrieves a list of available flights that match your criteria. The API then sends back a response to the travel planning application, which includes the flight details, such as the airline, departure, and arrival times, ticket prices, and other relevant information.
In this scenario, searching for available flights by hitting the "Search" button triggered an API call to the flight booking API. The API call facilitated the communication between the travel planning application and the flight booking service, allowing you to retrieve and view the relevant flight information conveniently within the application.
Here's an example of how to make an API call using Node.js:
const axios = require('axios');
async function fetchData() {
try {
const response = await axios.get('https://api.example.com/data');
console.log(response.data);
// Process the data
} catch (error) {
console.error(error);
}
}
fetchData();
If the API call is successful, the response data is logged to the console.
If an error occurs during the API call, it will be caught in the catch
block. In this example, we simply log the error to the console using console.error(error)
. You can customize the error handling logic based on your application requirements.
Methods of API Calls
API calls can be classified into different types based on the actions and operations they perform. Here are several common categories of API calls:
1. GET Method
The GET
method is useful when you want to fetch information or resources from the server. This is one of the most frequently utilized methods in APIs and websites.
Retrieving a user's profile information from a social media API:
GET /api/users/{user_id}
Fetching a list of products from an e-commerce API:
GET /api/products
2. POST Method
The POST method is utilized for sending data to a server in order to create or update a resource. It is commonly employed when submitting forms or transmitting data to be processed on the server.
Creating a new user account using a user registration API:
POST /api/users
Request Body: {
"name": "John Doe",
"email": "john@example.com",
"password": "secretpassword"
}
Adding a new product to an e-commerce store using a product creation API:
POST /api/products
Request Body: {
"name": "New Product",
"price": 19.99,
"description": "A description of the new product"
}
3. PUT Method
The PUT method is utilized to modify an already existing resource on the server. It resembles the POST method but is specifically designed for updating pre-existing data. For instance:
Updating a user's profile information using a user update API:
PUT /api/users/{user_id}
Request Body: {
"name": "Updated Name",
"email": "updated@example.com"
}
Modifying product details using a product update API:
PUT /api/products/{product_id}
Request Body: {
"name": "Updated Product Name",
"price": 29.99,
"description": "An updated description"
}
4. DELETE Method
The DELETE method is used for the purpose of removing a resource from the server. It is utilized to delete or eliminate a specific resource identified by a URL or URI. For example:
Deleting a user account using a user deletion API:
DELETE /api/users/{user_id}
Removing a product from an e-commerce store using a product deletion API:
DELETE /api/products/{product_id}
5. PATCH Method
The PATCH method is utilized to make partial updates to a resource on the server. It differs from the PUT method, as it allows for sending only the specific modifications or updates that are required for the resource, rather than sending the entire updated version.
Updating specific fields of a user's profile using a user profile update API:
PATCH /api/users/{user_id}
Request Body: {
"name": "Updated Name"
}
Modifying certain attributes of a product using a product partial update API:
PATCH /api/products/{product_id}
Request Body: {
"price": 39.99
}
Different Modes of Executing an API Call
When it comes to interacting with APIs, developers have different options for making requests and receiving responses. Two common approaches are synchronous and asynchronous API calls. These approaches determine how the client application handles the process of sending a request to an API and waiting for the corresponding response.
1. Synchronous API Calls
In synchronous API calls, the client application sends a request to the API and waits for a response before proceeding with further actions. The client blocks and remains idle during this waiting period.
Once the response is received, the client processes it and continues executing the subsequent code. Synchronous API calls are straightforward to implement and follow a sequential execution pattern.
Example for Synchronous API call:
const axios = require('axios');
function fetchData() {
try {
const response = axios.get('https://api.example.com/data');
console.log(response.data);
// Process the data
} catch (error) {
console.error(error);
}
}
fetchData();
In the synchronous API call example, we're making use of the synchronous behavior of Axios. The fetchData
function does not have the async
keyword, and we don't use await
when making the API call. Instead, the response is returned directly by axios.get()
.
We can access the response data using response.data
. However, in this approach, if an error occurs during the API call, it will throw an exception, and we need to handle it using a try-catch block.
2. Asynchronous API Calls
Asynchronous API calls, on the other hand, allow the client application to send a request and continue its execution without waiting for a response. The client doesn't block or remains idle while waiting for the API response.
Instead, it can perform other tasks or make additional API calls concurrently. When the response is eventually received, the client processes it through a callback function or by using asynchronous programming techniques.
Example for Asynchronous API call:
const axios = require('axios');
async function fetchData() {
try {
const response = await axios.get('https://api.example.com/data');
console.log(response.data);
// Process the data
} catch (error) {
console.error(error);
}
}
fetchData();
In the asynchronous API call example, we're using the async
keyword in the fetchData
function declaration to indicate that it is an asynchronous function. Inside the function, we use await
before axios.get()
to await the response from the API call.
This allows the function to pause and wait for the response before continuing with further processing. Using await
ensures that the API call is non-blocking and does not block the execution of other code.
Note that to use await
, the enclosing function must be marked as async
.
In both examples, error handling is done within the catch
block. If an error occurs during the API call, it is caught and logged to the console.
Understanding the differences between synchronous and asynchronous API calls can help developers choose the most suitable method for their specific use cases.
Error Handling in API Calls
When it comes to error handling in API calls, following best practices is crucial for maintaining a robust and user-friendly system. Start by using a consistent error response format with relevant information. Utilize appropriate HTTP status codes to indicate the outcome of the API call.
Provide descriptive and user-friendly error messages that offer guidance on resolving the issue. Implement exception handling mechanisms to gracefully handle errors and prevent application crashes. Validate and sanitize user input to ensure data integrity and protect against security vulnerabilities.
Implement logging and error tracking to aid in debugging and troubleshooting. Document the possible errors and provide guidance on how to handle them. Consider security implications and avoid exposing sensitive information in error responses. Implement throttling mechanisms to prevent abusive requests.
Finally, thoroughly test error scenarios to ensure the effectiveness of your error handling mechanisms.
Here's an example of how you can handle errors in API calls using Node.js:
const axios = require('axios');
async function fetchData() {
try {
const response = await axios.get('https://api.example.com/data');
console.log(response.data);
// Process the data
} catch (error) {
if (error.response) {
// Handle error response from the server
console.error(`Status code: ${error.response.status}`);
console.error(`Response data: ${error.response.data}`);
} else if (error.request) {
// Handle request error (e.g., no response received)
console.error(`No response received: ${error.request}`);
} else {
// Handle other request setup errors
console.error(`Error: ${error.message}`);
}
}
}
fetchData();
In this example, we use the Axios library to make the API call. The fetchData
function is declared as async
to allow the use of await
for making the HTTP GET request to https://api.example.com/data
. If the request is successful, the response data is logged and can be further processed.
If an error occurs, it will be caught in the catch
block. Inside the error handling block, we examine different properties of the error object to determine the type of error encountered.
- If
error.response
is defined, it means the server responded with a non-2xx status code, so we can access the response status code and data usingerror.response.status
anderror.response.data
, respectively. - If
error.request
is defined, it means the request was made but no response was received. We can handle this scenario by logging an appropriate message, such asNo response received: ${error.request}
. - For any other errors that occur during the request setup, we can access the error message using
error.message
. This can be used to handle errors related to setting up the request.
Securing API Calls with HTTPS and SSL/TLS
Securing API calls with HTTPS and SSL/TLS is like creating a protective shield for the data that travels between applications over the internet. It ensures that the information remains confidential, and reaches its intended destination without falling into the wrong hands.
Imagine you are sending a secret message to someone. You put it in a locked box and send it through a secure courier service. The recipient has the key to unlock the box and read the message. In this analogy, HTTPS is like the locked box, SSL/TLS is the courier service, and the client and server are the sender and recipient, respectively.
HTTPS, which stands for Hypertext Transfer Protocol Secure, adds an extra layer of security to API calls. It uses encryption to scramble the data, making it unreadable to anyone trying to intercept it. This encryption process takes place using SSL/TLS protocols, which create a secure connection between the client and the server.
Common Mistakes to Avoid When Making API Calls
Integrating APIs is a common practice in modern software development, enabling applications to leverage external services, retrieve data, and perform various operations. It is crucial for developers to be aware of these common pitfalls to ensure smooth communication with APIs and optimize the overall performance and reliability of their applications.
- Not Caching Responses: Neglecting response caching, leading to redundant API calls and decreased application performance.
- Lack of Proper Documentation and Versioning: Insufficient documentation of API endpoints and lack of versioning guidelines, causing confusion and compatibility issues.
- Poor Testing and Monitoring: Inadequate testing and monitoring of API calls, leading to undetected issues and unreliable application behaviour.
- Inadequate Error Messaging: Providing vague or uninformative error messages, making it challenging to identify and resolve issues effectively.
- Ignoring API Lifecycle Management: Neglecting to keep track of API changes, updates, and deprecations, resulting in compatibility issues and potential disruptions in functionality.
- Ignoring API Versioning: Neglecting to utilize proper versioning techniques can result in compatibility issues and break existing integrations when API changes are introduced. Adhere to versioning guidelines to ensure seamless transitions and maintain backward compatibility.
- Insufficient Error Handling: Neglecting proper handling of errors and failure scenarios, resulting in unpredictable behaviour and compromised application stability.
- Lack of Authentication and Authorization: Failing to implement secure authentication and authorization measures, exposing sensitive data and compromising the security of the API integration.
- Overfetching or Underfetching Data: Retrieving excessive or insufficient data from the API, leading to performance issues, increased network overhead, or incomplete information.
- Ignoring Rate Limits and Throttling: Disregarding rate limits imposed by the API provider, potentially causing service disruptions, temporary blocks, or API restrictions.
- Inefficient Use of Batch or Bulk Operations: Failing to utilize batch or bulk operations where applicable, resulting in slower performance and increased network overhead.
Conclusion
API calls empower developers to unlock a treasure of possibilities. They serve as the bridge that connects systems, allowing developers to leverage the functionalities and resources of external services to enhance their own applications.
The significance of API calls goes beyond mere technical integration. They have become the backbone of modern digital experiences, powering the interconnected web of services and applications that we rely on daily.
From mobile applications to e-commerce platforms, social media networks to Internet of Things (IoT) devices, API calls are at the core of seamless user experiences and efficient data flow.
As technology continues to evolve, API calls will remain a fundamental building block for seamless integration, data sharing, and collaboration between applications. They will continue to empower developers to innovate, create, and build transformative solutions that shape the digital landscape.
Atatus API Monitoring and Observability
Atatus provides Powerful API Observability to help you debug and prevent API issues. It monitors the consumer experience and is notified when abnormalities or issues arise. You can deeply understand who is using your APIs, how they are used, and the payloads they are sending.
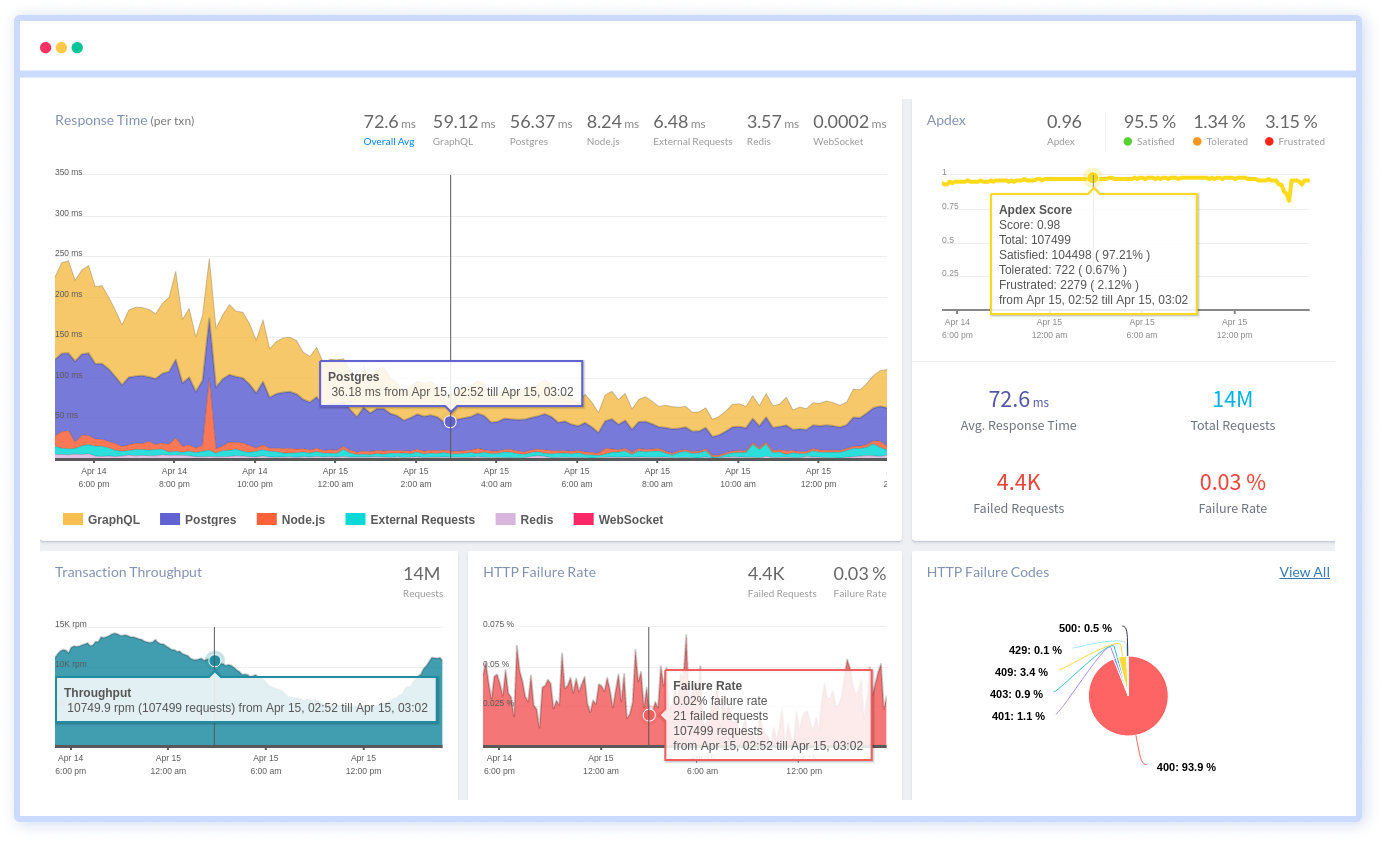
Atatus's user-centric API observability tracks how your actual customers experience your APIs and applications. Customers may easily get metrics on their quota usage, SLAs, and more.
It monitors the functionality, availability, and performance data of your internal, external, and third-party APIs to see how your actual users interact with the API in your application. It also validates rest APIs and keeps track of metrics like latency, response time, and other performance indicators to ensure your application runs smoothly.
#1 Solution for Logs, Traces & Metrics
APM
Kubernetes
Logs
Synthetics
RUM
Serverless
Security
More