An essential data structure in programming is a queue. A queue is open at both ends and operates according to the FIFO (First In First Out) concept. Data insertion occurs at one end of the queue, known as the back end or tail, while data deletion occurs at the other end, known as the front end or head of the queue.
We will cover the following:
What is Queue?
A queue is an organized group of objects where new items are added at one end, known as the "rear," and old items are taken out at the other end, sometimes referred to as the "front." When an element joins the queue, it moves from the back to the front, waiting until it is the next element to be taken out of the line.
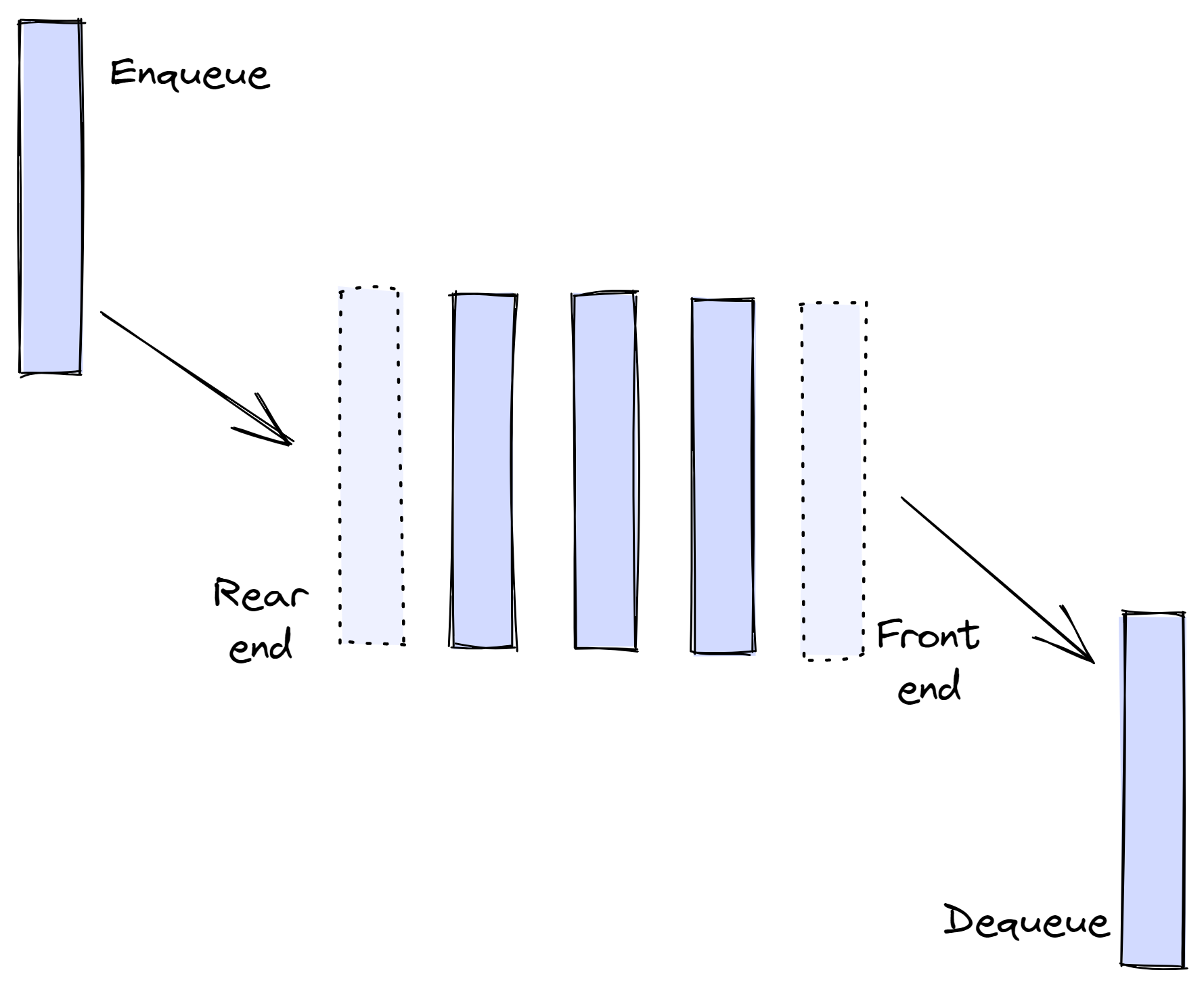
The last thing in the queue to be processed must wait until the end of the collection. At the front is the piece that has been around the longest in the collection. First-in first-out, or FIFO, is another name for this ordering tenet. It is also known as "first-come first-served."
The typical line that we all occasionally wait in is the simplest example of a queue. We wait in the restaurant line, the grocery store checkout line, and the line to see a movie (so that we can pop the tray stack).
The fact that there is only one entrance and exit makes orderly queuing extremely constrictive. There is no skipping the front and no leaving before you have waited the required length of time.
In real life, queues are a highly common model for data flow. Think about a workplace with 30 PCs connected through a network to a single printer.
Printing requests "get in line" with all the other printing requests that are waiting when someone wants to print anything. The following task is to finish the first one. The jobs in front of you must print before you if you are last in line.
Additionally, a variety of queues are used by operating systems to manage computer processes. A queuing algorithm is often used to schedule what gets done next and aims to serve as many people as possible while executing programs as quickly as possible.
Furthermore, occasionally as we type, keystrokes occur before the characters that are displayed on the screen. This is because the computer was simultaneously working on other tasks.
To ensure that the keystrokes are eventually presented on the screen in the correct order, they are stored in a buffer that resembles a queue.
Types of Queue
A queue is a helpful data structure in programming. It is comparable to the queue for tickets outside a movie theater, where the person who joins the queue first receives the first ticket.
There are four different categories of queues:
- Simple Queue
- Circular Queue
- Priority Queue
- Double Ended Queue
1) Simple Queue
In a simple queue, insertion happens at the back and removal happens at the front. It firmly adheres to the First in First out (FIFO) concept.

2) Circular Queue
In a circular queue, the last element makes a circular relationship with the initial element.
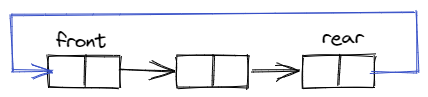
Better memory utilization is the key benefit of a circular queue over a simple queue. We can insert an element in the first position if the last position is full and the first position is vacant. In a basic queue, this action cannot be accomplished.
3) Priority Queue
A special kind of queue called a priority queue is one in which each element has a priority assigned to it and is handled by that priority. If two items with the same priority appear, they are handled in the order they were placed in the queue.
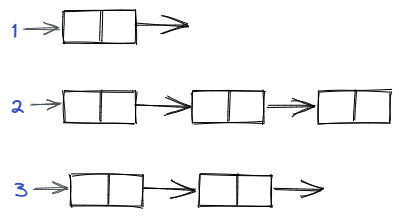
According to the arrival of the data, insertion takes place, and removal takes place according to priority.
4) Deque (Double-Ended Queue)
A double-ended queue allows for the insertion and removal of elements from either the front or the rear. As a result, it deviates from the FIFO (First In First Out) concept.

Queue Implementation
The fact that a queue theoretically lacks a fixed capacity is one of its characteristics. No matter how many elements are already there, more can always be added. It can also be empty, in which case it will not be allowed to remove an element until another element has been added.
Although fixed-length arrays have a finite number of elements, it is untrue that things must be copied to the front of the queue. It is unnecessary to ever move objects contained in the array because of the straightforward approach of converting the array into a closed circle and allowing the head and tail to endlessly float around in that circle.
Computing indices modulo n will transform the array into a circle if n is the array's size. This is still the conceptually simplest way to create a queue in a high-level language, but it does slow things down a little because the array indices must be compared to zero and the array size, which is comparable to the time it takes to check whether an array index is out of bounds, which some languages do.
Nevertheless, this will undoubtedly be the preferred method for a quick and dirty implementation or for any high-level language that does not have pointer syntax.
Although some implementations simply double the given array size when overflow occurs, the array size must be declared in advance. Most contemporary languages that support objects or pointers can implement dynamic lists or include libraries for doing so.
In addition to memory restrictions, such data structures might not have defined a set capacity limit. Attempting to add an element to a full queue will cause a queue overflow while attempting to remove an element from an empty queue will cause a queue underflow.
A queue that can hold just a certain number of items is said to be bounded.
FIFO queues can be implemented in several effective ways. En-queuing and de-queuing activities must be completed in O(1) time for an implementation to be considered effective.
1) Linked List
- A doubly linked list makes sense for queues since it has O(1) insertion and deletion at both ends.
- Regular singly-linked lists can insert and delete efficiently only at one end. An effective queue can be implemented with just a small adjustment of a pointer to the last node in addition to the first one.
2) Deque
- A deque implemented with a modified dynamic array
Applications of Queue
When we need a process to run in the First in First Out sequence, we use the queue. It is advantageous to use queue data structures in circumstances when synchronous data transfer is not required.
Breadth-First Search
This is one of the methods for moving through a tree's nodes. In this case, a queue of the nearby nodes of the current node that must be traversed before moving to the following level of depth is kept.
Single Shared Resources
The queue keeps track of the order in which different requesting processes should be given access to the same resource. Operating systems also use a queue to keep track of the processes that must be retrieved for execution from their waiting states, like priority queues.
MM1 Queue
This queuing model is being used to simulate a variety of real-world scenarios. The following categories for its use are:
- There is just a single server available.
- The service time for a non-empty queue has an exponential distribution with the rate mu/min.
- A queue has an exponential distribution of lambda per minute and an inter-arrival time.
What are Queues Used For?
Queues, which are common in computer applications, can be utilized in any circumstance when you want things to happen in the order they were called but the machine cannot keep up.
A queue data structure requires that after a new element is added, all previous elements that were added must be removed to make room for the new element. In practically every form of software development, such circumstances occur.
Take as an example a website that serves files to thousands of users or a multitasking operating system.
Queues are used in operating systems to regulate access to shared resources like printers, files, and communication lines. For these situations, queues are the best data structure to utilize because:
- A website cannot fulfill every request, so it treats them in the order of arrival according to the principle of "first-come first-served".
- An operating system schedules tasks by some policy since it is impossible to do all tasks at once.
Queues operate behind the scenes like a temporary database in computing systems that require high throughput and significant scale-out. A durable queue can recreate where it was and ensure that things are delivered if the service fails by maintaining elements in the same way a database would.
Conclusion
The components are stored in the queue data structure, a linear type of data structure. The FIFO method is used to store elements in this data structure. When creating a queue data structure, an array or linked list was employed. At the REAR end of the queue, items are added, and at the FRONT end, items are deleted. A single shared resource uses this kind of data structure to handle a variety of queries.
Also Read:
Monitor Your Entire Application with Atatus
Atatus is a Full Stack Observability Platform that lets you review problems as if they happened in your application. Instead of guessing why errors happen or asking users for screenshots and log dumps, Atatus lets you replay the session to quickly understand what went wrong.
We offer Application Performance Monitoring, Real User Monitoring, Server Monitoring, Logs Monitoring, Synthetic Monitoring, Uptime Monitoring, and API Analytics. It works perfectly with any application, regardless of framework, and has plugins.
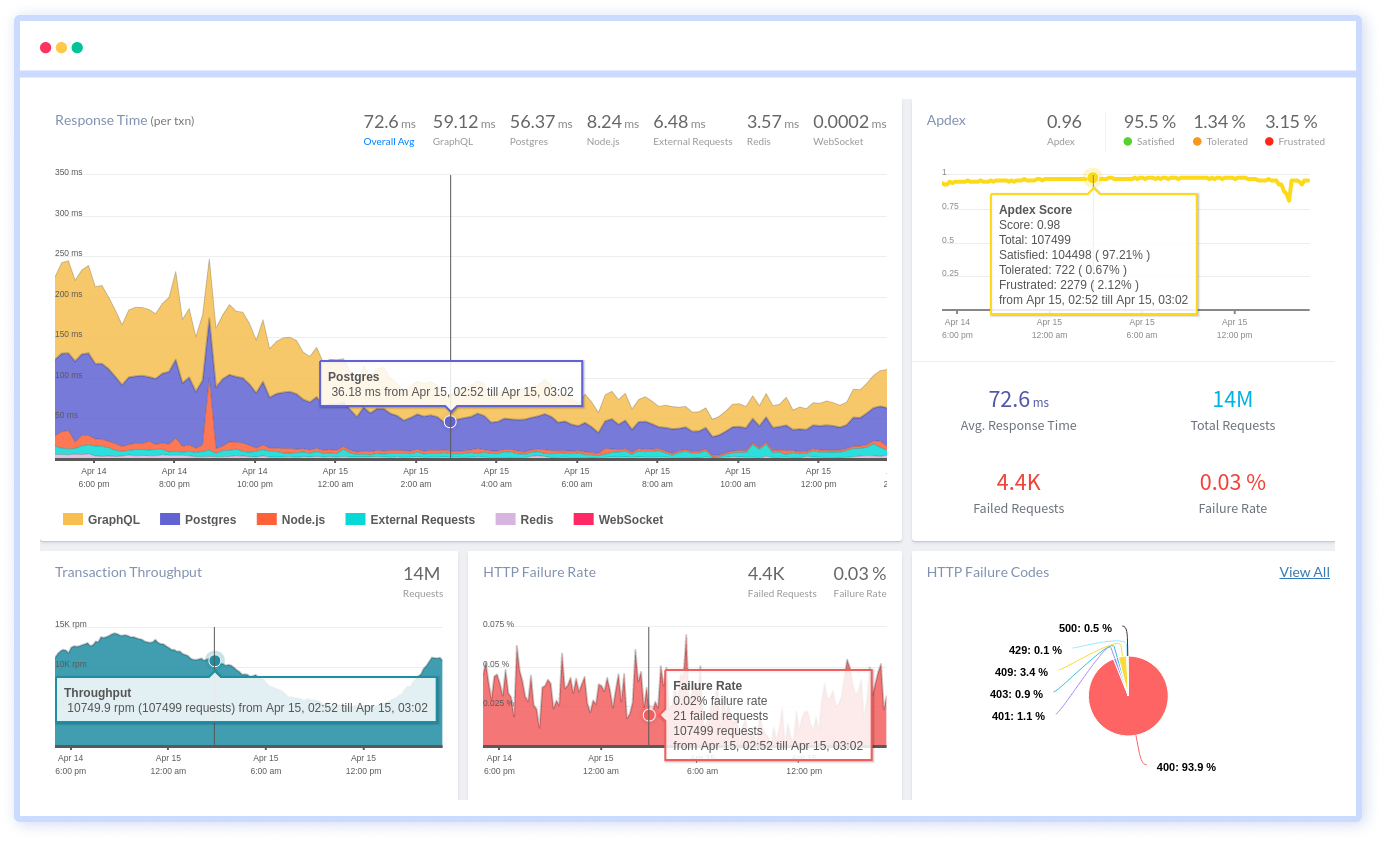
Atatus can be beneficial to your business, which provides a comprehensive view of your application, including how it works, where performance bottlenecks exist, which users are most impacted, and which errors break your code for your frontend, backend, and infrastructure.
If you are not yet an Atatus customer, you can sign up for a 14-day free trial.