PHP Code Simplification: How Array Destructuring Can Help?
Have you ever struggled with writing lengthy code just to extract values from an array in PHP? If so, there is no need to worry anymore. Array destructuring in PHP offers a quick and efficient solution to this problem, allowing you to extract array values in just a few lines of code. By utilizing array destructuring, you can effortlessly extract values from arrays and make your code more readable and structured.
But what is array destructuring, exactly? Well, it's a feature in PHP that allows you to extract values from an array and assign them to variables in a single line of code. This can be incredibly useful for a variety of tasks, from parsing API responses to working with complex data structures.
In this post, we'll dive into the world of array destructuring in PHP, exploring its many use cases and advanced techniques. We will cover everything from basic syntax to more complex applications, and we will look into plenty of code examples to help you understand how it all works.
So, whether you're a seasoned PHP developer or just starting out, get ready to learn all about the power of array destructuring. How can you use it to simplify your code? What are some common mistakes to avoid? And most importantly, how can it help you write better, more efficient PHP code? Let's find out!
"Array destructuring is like a Swiss Army knife for PHP developers - you never know when you'll need it, but when you do, it's a lifesaver."
Table of Contents
- Introduction to Array Destructuring in PHP
- Basic syntax of Array Destructuring
- Array Destructuring with list() Function
- Swapping variable values using array destructuring
- Advanced use cases of array destructuring
- Potential Pitfalls to Avoid when Using Array Destructuring
Introduction to Array Destructuring in PHP
In PHP, an array is a data structure that can hold a collection of values, including integers, floats, strings, or other arrays. An array can be indexed by numbers, starting at zero, or by strings. You can access individual elements of an array using their index.
Array destructuring is a feature in PHP that allows you to extract values from an array and assign them to individual variables in a single statement. This can be useful when you want to quickly extract multiple values from an array and avoid writing multiple lines of code.
To use array destructuring in PHP, you specify a list of variables, enclosed in square brackets, and assign them the array you want to extract the values from. PHP will automatically assign each variable a value from the corresponding position in the array.
Say goodbye to the time-consuming process of extracting values from arrays and hello to the simplicity of array destructuring.
Basic syntax of array destructuring
In PHP, array destructuring is a way to extract values from an array and assign them to individual variables. Here is the basic syntax of array destructuring in PHP.
[$var1, $var2, ...] = $array;
Here, $array
is the array from which you want to extract values, and $var1
, $var2
, etc. are the variables in which you want to store those values.
For example, let's say you have an array with three values, say 10, 20 and 30,
$array = [10, 20, 30];
Now, you can extract these values into individual variables like this as shown,
[$a, $b, $c] = $array;
Now, $a
will be 10, $b
will be 20, and $c
will be 30.
Also, when using array destructuring in PHP, you may encounter situations where the number of variables specified is not the same as the number of values in the array you are destructuring. In such cases, PHP has some behavior that you should be aware of.
If the array has more values than the number of variables specified, the additional values will be ignored. For example, consider the following code,
$array = [10, 20, 30, 40];
[$a, $b] = $array;
Here, $a
will be assigned the value 10 and $b
will be assigned the value 20. The remaining values in the array (30 and 40) will be ignored.
On the other hand, if there are fewer values than variables specified, the remaining variables will be assigned null
as shown below.
$array = [10];
[$a, $b, $c] = $array;
Here, $a
will be assigned the value 10, but $b
and $c
will be assigned null, since there are no corresponding values in the array to assign to them.
To prevent your code from producing unexpected results or errors, it is essential to be mindful of this behaviour when using array destructuring in PHP.
Array Destructuring with list() Function
While square brackets are commonly used for array destructuring, the list()
function provides an alternative syntax for this process.
$array = [10, 20, 30];
list($a, $b, $c) = $array;
The list()
function assigns values from an array to a list of variables in one statement. In this example, the list()
function is used to assign the values 10, 20, and 30 from the $array variable to the variables $a
, $b
, and $c
, respectively. This code is functionally equivalent to the square bracket syntax.
However, the list()
function can be useful in certain situations where you prefer the syntax or need to skip certain values in the array. For example, you can use an empty space in the list of variables to skip certain values in the array as shown below.
$array = [10, 20, 30];
list($a, , $c) = $array;
In this case, the second value in the $array
variable is skipped by using an empty space in the list of variables, and $a
is assigned the value 10, while $c
is assigned the value 30 respectively.
You can also use the list()
function to extract values from associative arrays using the keys as variable names.
In PHP, the list()
function can be used to extract values from associative arrays using the keys as variable names. This can be useful when you have an array with named keys and you want to assign the values to individual variables using the same key names. Here is an example of using the list()
function to extract values from an associative array.
$assoc_array = ['name' => 'John', 'age' => 30, 'gender' => 'male'];
list('name' => $name, 'age' => $age, 'gender' => $gender) = $assoc_array;
In this example, the $assoc_array
variable is an associative array with three key-value pairs. The list()
function is used to assign the values corresponding to the keys 'name', 'age', and 'gender' to the variables $name
, $age
, and $gender
, respectively.
After the list()
function is executed, the variable $name will contain the value 'John', the variable $age
will contain the value 30, and the variable $gender
will contain the value 'male'.
Using the list()
function with associative arrays can be a convenient way to extract values from an array with named keys and assign them to variables with the same names. However, it is important to note that the keys in the list()
function must match the keys in the associative array, otherwise the variables will not be assigned the correct values.
Swapping variable values using array destructuring
In PHP, array destructuring allows you to assign values from an array to individual variables in a single statement. This feature can be used to swap the values of two variables without needing a temporary variable.
Suppose we have an array of customer data that includes their name, email address, and phone number. We might want to swap the email address and phone number values for some reason. We can do this easily using array destructuring.
$customer = [
'name' => 'John Doe',
'email' => 'johndoe@example.com',
'phone' => '555-1234',
];
[$customer['email'], $customer['phone']] = [$customer['phone'], $customer['email']];
This code creates a new array containing the values of $customer['phone']
and $customer['email']
, in that order, and then uses array destructuring to assign those values to $customer['email']
and $customer['phone']
, respectively.
<?php
$customer =
[
'name' => 'John Doe',
'email' => 'johndoe@example.com',
'phone' => '555-1234',
];
echo "Customer data before swap: \n";
print_r($customer);
[$customer['email'], $customer['phone']] = [$customer['phone'], $customer['email']];
echo "Customer data after swap: \n";
print_r($customer);
?>
After this code runs, the email address and phone number values in the $customer
array will be swapped as shown below:
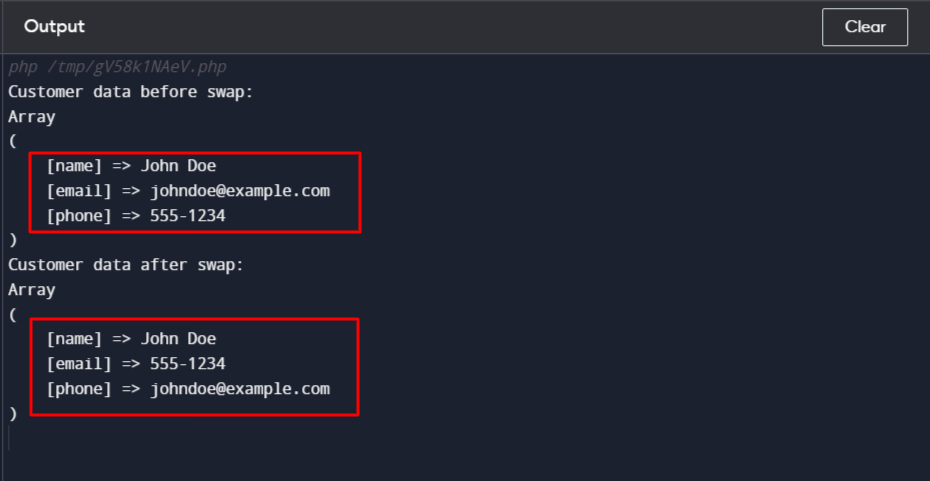
Advanced use cases of array destructuring
In addition to the basic use cases, array destructuring can be used in more advanced scenarios, such as extracting values from nested arrays and objects, setting default values for missing array keys, and extracting values from function returns. Here are some examples of how array destructuring can be used in more advanced ways.
1. Extracting values from nested arrays
Extracting values from nested arrays means that you have an array that contains other arrays nested within it, and you want to extract specific values from those nested arrays.
The given following code creates an array called $people
which contains two sub-arrays, each representing a person's information, including their name and address. Each address is also an array, containing the street, city, state, and zip.
<?php
$people = [
[
'name' => 'John Doe',
'address' => [
'street' => '123 Main St',
'city' => 'Anytown',
'state' => 'CA',
'zip' => '12345',
],
],
[
'name' => 'Jane Smith',
'address' => [
'street' => '456 Oak St',
'city' => 'Othertown',
'state' => 'NY',
'zip' => '67890',
],
],
];
foreach ($people as ['address' => ['zip' => $zip]]) {
echo "Zip code: $zip\n";
}
?>
The foreach loop iterates over the $people
array and uses array destructuring to extract the zip value from the address sub-array for each person.
The syntax ['address' => ['zip' => $zip]] is used to extract the zip value from the nested address array. This means that for each person in the $people
array, the zip value will be assigned to the $zip
variable.
Finally, the code uses the $zip
variable to output the zip code for each person, along with the string "Zip code: " using the echo statement.
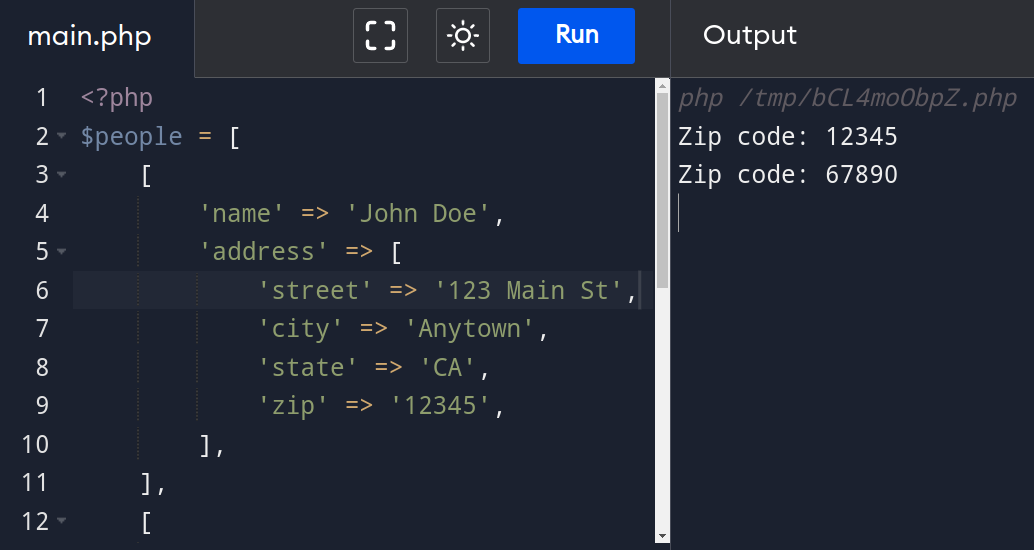
2. Extracting values from function returns
Using array destructuring to extract values from function returns can be a convenient way to handle complex data structures and simplify your code.
In this example, we define a function called getPerson
which returns an array containing information about a person, including their name, age, and email.
<?php
function getPerson() {
return [
'name' => 'John Doe',
'age' => 30,
'email' => 'johndoe@example.com',
];
}
['name' => $name, 'age' => $age] = getPerson();
echo "Name: $name\n";
echo "Age: $age\n";
?>
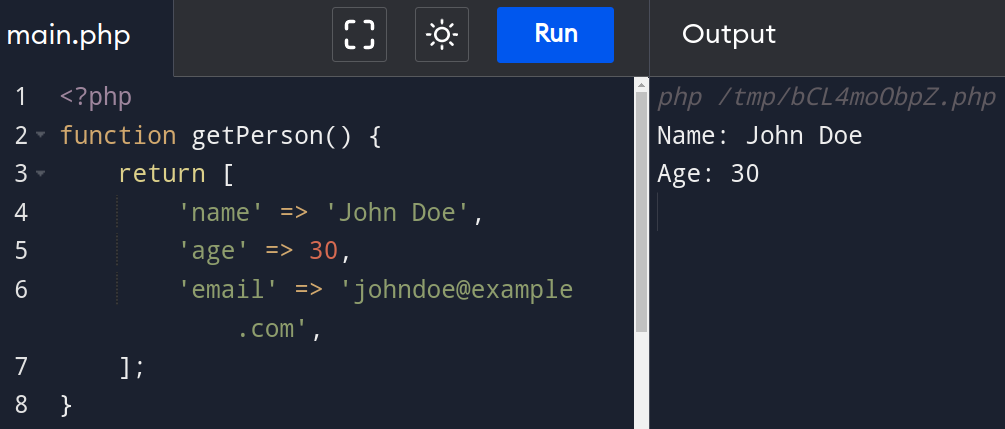
We then use array destructuring to extract the name and age values from the array returned by the getPerson
function. Finally, we output the values of $name
and $age
using the echo
statement.
3. Using default values for missing array keys
Using default values for missing array keys can be important in situations where you want to avoid errors or handle missing data gracefully. When working with arrays, it's common to encounter situations where a key is missing, especially when working with data that comes from external sources.
For example, consider the following code that retrieves a value from an array using the ['key'] syntax.
$data = ['foo' => 'bar'];
$value = $data['key'];
echo $value;
This code will result in a Notice: Undefined index error
, because the key
index does not exist in the $data
array.
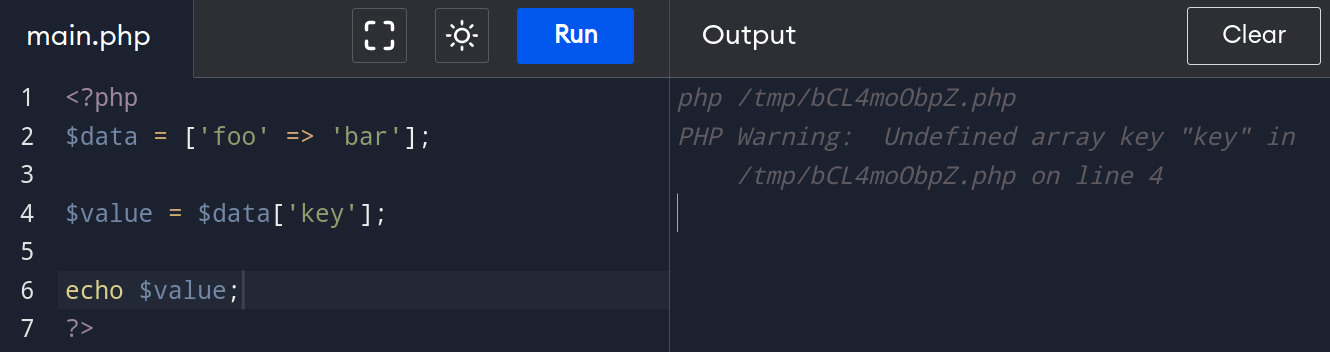
To avoid this error, you can use the null coalescing operator (??)
to specify a default value that will be used if the key is missing.
<?php
$data = ['foo' => 'bar'];
$value = $data['key'] ?? 'default';
echo $value;
?>
In this example, the $value
variable will be assigned the value 'default' because the 'key' index does not exist in the $data
array.
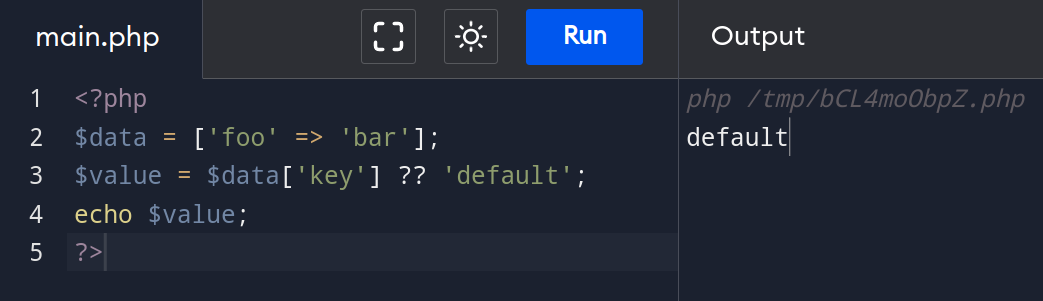
4. Extracting values from objects
In PHP, you can use object destructuring to extract values from objects. Object destructuring works in a similar way to array destructuring, but instead of using square brackets to specify keys, you use curly braces and property names.
<?php
class Person {
public string $name;
public int $age;
public function __construct(string $name, int $age) {
$this->name = $name;
$this->age = $age;
}
}
$person = new Person('John Doe', 30);
['name' => $name, 'age' => $age] = (array) $person;
echo "Name: $name\n";
echo "Age: $age\n";
?>
In this example, we define a Person
class with two public properties: $name and $age. We then create a new Person object with the name "John Doe" and age 30.
We use object destructuring to extract the name and age properties from the $person
object, and assign them to variables $name
and $age
. Finally, we output the values of $name
and $age
using the echo
statement.
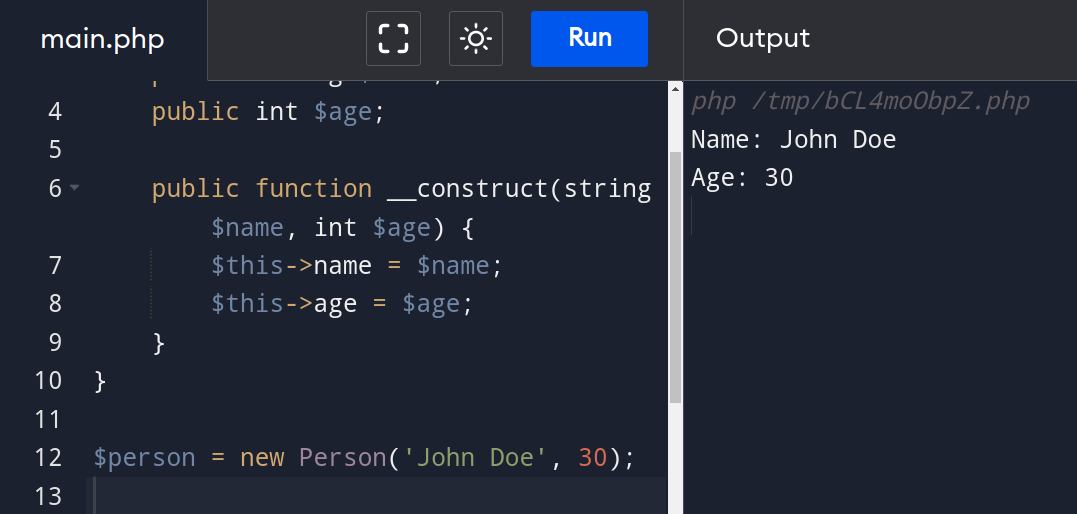
Using these advanced features of array destructuring in PHP can help you write code that is easier to read, understand, and maintain. This can result in faster development times and fewer errors, leading to a more enjoyable development experience overall.
Potential Pitfalls to Avoid when Using Array Destructuring
Here are some potential pitfalls to avoid when using array destructuring in PHP with examples:
1. Undefined or missing array keys:
$data = ['name' => 'John Doe'];
[$name, $email] = $data; // PHP Notice: Undefined offset: 1
In the example above, we are trying to extract the $name
and $email
variables from the $data
array using array destructuring. However, the $email
key does not exist in the array, causing PHP to throw a notice. To avoid this issue, we can check if the $email
key exists in the array before extracting it:
$data = ['name' => 'John Doe'];
[$name, $email] = $data + [null, null];
Now, the array will be augmented with null
values for the $email
key, preventing any undefined offset errors.
2. Incorrect array structures:
$data = ['John Doe', 'john@example.com'];
[$name, $email, $phone] = $data; // PHP Fatal error: Uncaught Error: [] operator not supported for strings
In the example above, we are trying to extract the $name
, $email
, and $phone
variables from the $data
array using array destructuring. However, the $data
array has only two elements, causing PHP to throw a fatal error. To avoid this issue, we can ensure that the array structure matches the variable list:
$data = ['John Doe', 'john@example.com'];
[$name, $email] = $data;
Now, the array will be destructured into only $name
and $email
variables, preventing any fatal errors.
Conclusion
As we come to the end of this blog on array destructuring in PHP, it's clear that this feature is a game-changer for developers. Array destructuring simplifies the process of extracting values from arrays, making code more concise and readable. But beyond its practical applications, array destructuring represents a shift in the way we think about code.
By embracing array destructuring, developers can unlock new levels of creativity and innovation. It allows for a more streamlined approach to coding, freeing up mental space for bigger and better ideas. In many ways, array destructuring is a symbol of the ongoing evolution of PHP and the broader tech landscape.
So, Let's explore its full potential and see where it takes us. The future of PHP development is bright, and array destructuring is just the beginning.
Atatus: PHP Performance Monitoring and Log Management
Atatus is an Application Performance Management (APM) solution that collects all requests to your PHP applications without requiring you to change your source code. However, the tool does more than just keep track of your application's performance.
Monitor logs from all of your PHP applications and systems into a centralized and easy-to-navigate user interface, allowing you to troubleshoot faster using PHP monitoring.
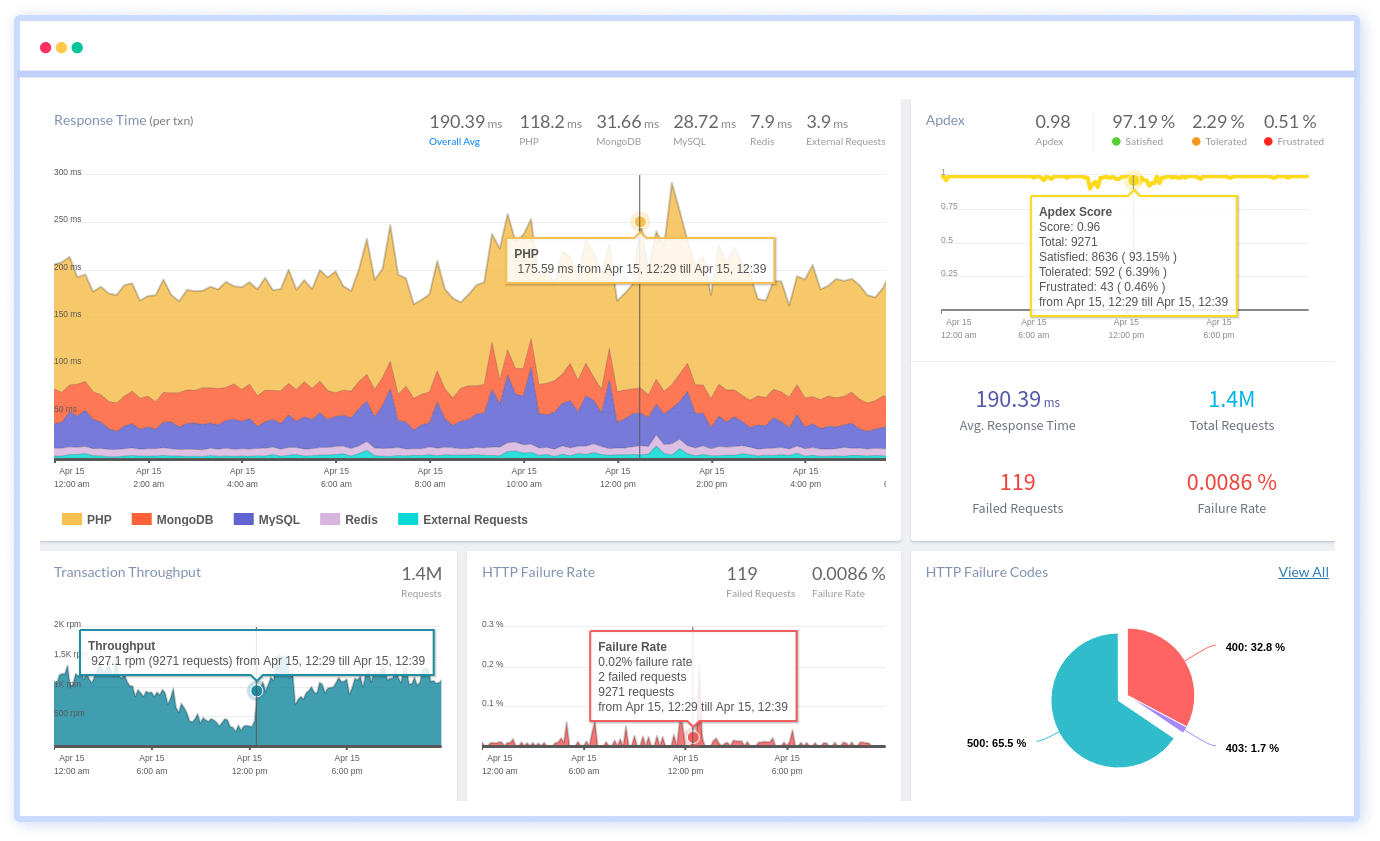
We give a cost-effective, scalable method to centralized PHP logging, so you can obtain total insight across your complex architecture. To cut through the noise and focus on the key events that matter, you can search the logs by hostname, service, source, messages, and more. When you can correlate log events with APM slow traces and errors, troubleshooting becomes easy.
#1 Solution for Logs, Traces & Metrics
APM
Kubernetes
Logs
Synthetics
RUM
Serverless
Security
More