Switch or Match in PHP
In the world of PHP programming, match and switch statements are both powerful tools for performing value comparisons. While they share some similarities, such as comparing a value against multiple conditions, they also have important differences that set them apart.
In this discussion, we'll explore the relationship between match and switch statements, as well as their differences. Specifically, we'll see how the match statement has certain advantages over the switch statement, such as its more expressive syntax and advanced type checking capabilities.
However, we'll also consider situations where the switch statement may still be the best choice, especially if backwards compatibility with older versions of PHP is a concern. Ultimately, both match and switch statements have their strengths and weaknesses, and knowing when to use each one can help you write cleaner, more efficient code.
"Match expression" was the original name for the match statement in PHP 8, but it was changed to simply "match" due to concerns about confusion and for conciseness.
Switch or Match in PHP? Confused which one to use? Let's Dive in and Find Out!
Table of contents
- Introduction to Switch and Match
- Syntax for Switch and Match Statement in PHP
- Advanced Type Checking Capabilities
- Control Flow Differences Between Switch and Match
- Using Switch Over Match in PHP
- Which Control Structure is the Best Choice?
Introduction to Switch and Match
Switch Statement
A switch statement in PHP is a programming construct that allows you to test a variable or expression against multiple cases and execute different blocks of code based on the value of the variable.
It's a convenient and readable way to write code that needs to perform different actions based on the value of a variable.
The syntax of a switch statement includes the expression to evaluate, followed by multiple case statements that test for specific values of the expression.
The code block for the first matching case is executed, and the break statement is used to exit the switch statement. A default case can also be included as a fallback option.
Match Statement
The match statement is a new feature introduced in PHP 8 that provides a new way to perform value comparisons. It is similar to the switch statement, but with a few key differences.
The match statement introduces the concept of pattern matching to PHP, which allows you to write more expressive and concise code when you want to perform complex value comparisons.
With the match statement, you can compare a value against a set of patterns and execute code based on which pattern matches the value. Each pattern can be either a value or an expression that evaluates to a value. You can also use the default
keyword to specify what code to execute if none of the patterns match the value.
Syntax for Switch and Match Statement in PHP
Both match
and switch
are conditional statements in PHP that allow you to execute different code blocks based on different conditions. switch
is a traditional control structure that has been around in PHP for a long time.
It works by evaluating an expression and then executing the code block associated with the first matching case
label. The syntax looks like this,
switch ($value) {
case 1:
// code to execute if $value is 1
break;
case 2:
// code to execute if $value is 2
break;
default:
// code to execute if $value doesn't match any of the above cases
}
In contrast, match
is a new feature introduced in PHP 8.0. It provides a more concise syntax for performing pattern matching on a value. The syntax looks like this,
match ($value)
{
pattern1 =>
{
// code to execute if $value matches pattern1
},
pattern2 =>
{
// code to execute if $value matches pattern2
},
// more patterns...
default =>
{
// code to execute if none of the patterns match
}
}
match
is the keyword that starts the match statement. It is followed by the value you want to compare.pattern1
,pattern2
, etc. are the patterns you want to match against the value. Each pattern can be a value, a variable, or an expression that evaluates to a value. You can specify multiple patterns separated by commas.=>
is the comparison operator that maps a pattern to a block of code. If the value matches the pattern, the block of code associated with that pattern is executed.- The code to execute is enclosed in curly braces
{ }
and can contain any valid PHP code, including function calls, conditional statements, loops, etc. default
is an optional keyword that specifies what code to execute if none of the patterns match the value. It is followed by the block of code to execute, which is enclosed in curly braces{ }
.
Now that we came to know the syntax of both switch and match, let us discuss about their features and differences to know which is more effective ?
The match
statement provides a more concise and expressive syntax for performing pattern matching on a value. While the traditional switch
statement has been around in PHP for a long time, match
offers several advantages over switch
that make it a better choice in most cases.
One key advantage of match
is its type safety. match
checks the type of the value being matched against each possible pattern, and throws a TypeError if there is a type mismatch. This helps catch errors earlier in the development process and makes your code more reliable.
Additionally, match
provides a more expressive syntax for matching patterns, which includes matching against arrays, using logical operators to combine patterns, and matching against object properties or method return values.
Another advantage of match
is its simplicity. match
provides a simpler and more concise syntax than switch
, making it easier to read and write. match
also supports early return using the return
keyword, which can make your code more efficient and easier to follow.
Overall, while switch
may still be useful in some situations, match
is generally considered to be a better choice in PHP 8.0 or later. Its type safety, expressiveness, simplicity, and early return support make it a powerful and versatile tool for matching patterns and executing code blocks based on different conditions.
Advanced Type Checking Capabilities
Both switch
and match
provide advanced type checking capabilities, which allow you to match against different types of values in a flexible and powerful way. However, there are some differences between the two that are worth discussing.
With switch
, you can use type checking to match against different types of values, including strings, integers, floats, and booleans. For example,
switch($value) {
case 'string':
// Do something for string values
break;
case 1:
// Do something for integer values
break;
case 1.5:
// Do something for float values
break;
case true:
// Do something for boolean values
break;
default:
// Do something for all other values
}
In contrast, match
provides more advanced type checking capabilities that are not available in switch
. For example, you can match against specific types, including scalar types, arrays, and objects. You can also use match
to match against class names, interface names, and trait names. Additionally, you can use match
to match against values that implement a specific interface or extend a specific class.
match($value) {
'string' => // Do something for string values,
1 => // Do something for integer values,
1.5 => // Do something for float values,
true => // Do something for boolean values,
array => // Do something for arrays,
object => // Do something for objects,
MyClass::class => // Do something for instances of MyClass,
MyInterface::class => // Do something for instances of classes that implement MyInterface,
MyTrait::class => // Do something for instances of classes that use MyTrait,
default => // Do something for all other values
}
While both switch
and match
offer advanced type checking capabilities, match
provides a more flexible and expressive syntax for matching against different types of values, including scalar types, arrays, objects, class names, interface names, and trait names. match
also allows you to match against values that implement a specific interface or extend a specific class.
Therefore, for advanced type checking in PHP, match
is the recommended option over switch
. It provides a more powerful and versatile tool for matching patterns and executing code blocks based on different conditions.
Control Flow Differences Between Switch and Match
In PHP, switch
and match
are two control structures that allow you to match a value against multiple conditions and execute different code blocks based on the matching condition. One key difference between the two is their control flow.
With switch
, the control flow is based on a "fallthrough" mechanism. This means that when a condition is matched, the code block for that condition is executed, and then execution continues with the next code block, regardless of whether or not it matches.
This continues until a break
statement is encountered, which ends the switch
block and skips to the end of the block.
$value = 2;
switch ($value) {
case 1:
echo "Value is 1";
break;
case 2:
echo "Value is 2";
case 3:
echo "Value is 3";
break;
}
In this example, since $value
is 2, the second condition is matched and the code block for that condition (echo "Value is 2";
) is executed. Then, since there is no break
statement, execution continues to the next code block, and the third message (echo "Value is 3";
) is also printed.
With match
, the control flow is based on a "return on match" mechanism. This means that when a condition is matched, the code block for that condition is executed, and then the match
statement immediately ends and returns the value of the code block. This means that only one code block is executed, even if multiple conditions match.
$value = 2;
$result = match ($value) {
1 => "Value is 1",
2 => "Value is 2",
3 => "Value is 3",
};
echo $result;
In this example, since $value
is 2, the second condition is matched and the code block for that condition ("Value is 2"
) is executed. Then, the match
statement immediately ends and returns the value of the code block ("Value is 2"
), which is stored in the $result
variable and printed with the echo
statement.
The control flow for switch
is based on a "fallthrough" mechanism, while the control flow for match
is based on a "return on match" mechanism. This can impact the behaviour of your code and should be considered when deciding which control structure to use in your PHP code.
Using Switch Over Match in PHP
While match
provides a more powerful and flexible syntax for pattern matching and type checking, there may still be situations where the traditional switch
statement is the best choice.
One such situation is when backwards compatibility with older versions of PHP is a concern. Since match
was introduced in PHP 8.0, code that uses match
will not be compatible with older versions of PHP.
Another situation where switch
may be a better choice is when you need to match against more complex patterns that are not easily expressed with match
. While match
allows you to match against arrays, logical operators, object properties, and method return values, there may be cases where you need to match against more complex patterns, such as regular expressions or nested structures. In these cases, switch
may be a more suitable choice.
It is worth noting that switch
may still be a good option if you're already familiar with its syntax and find it easier to read and understand than match
. While match
provides a more concise and expressive syntax, some developers may prefer the more explicit syntax of switch
.
Consider factors such as backwards compatibility, complex pattern matching, and personal preference when deciding which statement to use in your PHP code.
Which Control Structure is the Best Choice?
Both switch
and match
are powerful control structures that offer different features and syntax options in PHP. match
offers a more concise syntax, advanced type checking capabilities, and the ability to return values, making it a great choice for many situations.
However, switch
still has its place, particularly if backwards compatibility with older versions of PHP is a concern, or if you need to handle more complex control flow logic.
Ultimately, the best choice between switch
and match
will depend on the specific requirements of your code and the features that you need. Consider the type of data you're working with, the complexity of the control flow logic, and any performance considerations.
By understanding the differences and capabilities of both structures, you can make an informed decision and choose the best one for your use case.
Atatus: PHP Performance Monitoring and Log Management
Atatus is an Application Performance Management (APM) solution that collects all requests to your PHP applications without requiring you to change your source code. However, the tool does more than just keep track of your application's performance.
Monitor logs from all of your PHP applications and systems into a centralized and easy-to-navigate user interface, allowing you to troubleshoot faster using PHP monitoring.
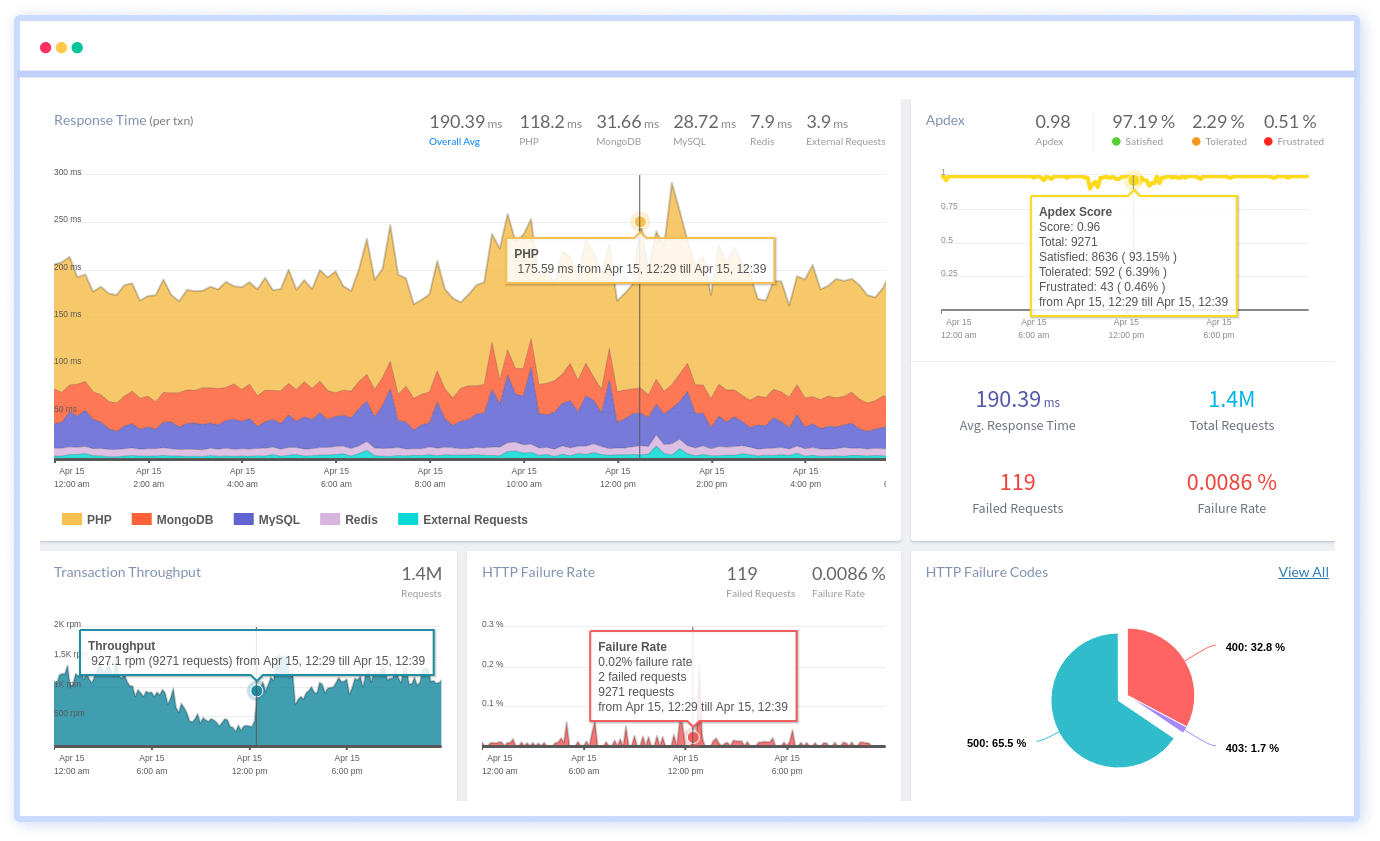
We give a cost-effective, scalable method to centralized PHP logging, so you can obtain total insight across your complex architecture. To cut through the noise and focus on the key events that matter, you can search the logs by hostname, service, source, messages, and more. When you can correlate log events with APM slow traces and errors, troubleshooting becomes easy.
#1 Solution for Logs, Traces & Metrics
APM
Kubernetes
Logs
Synthetics
RUM
Serverless
Security
More